p5.js and Arduino¶
Ref. Lab: Serial Input to P5.js
p5.js and Arduino¶
When you use the p5.serialport library for P5.js, it communicates with a webSocket server in the P5.js IDE to give you access to serial devices attached to your computer. This lab shows you how to use P5 to control a microcontroller using asynchronous serial communication.
Install the P5.serialcontrol App¶
Download P5.serialcontrol
Arduino sketch¶
void setup() {
Serial.begin(9600); // initialize serial communications
}
void loop() {
if (Serial.available() > 0) { // if there's serial data available
int inByte = Serial.read(); // read it
Serial.write(inByte); // send it back out as raw binary data
analogWrite(13, inByte); // use it to set the LED brightness
}
}
Note
Check the serial port in ArduinoIDE.
Open p5.js Web Editor in browser¶
Find this line
<script src="https://cdnjs.cloudflare.com/ajax/libs/p5.js/1.4.0/p5.js"></script>
Then Add this in the next line
<script language="javascript" type="text/javascript" src="https://cdn.jsdelivr.net/npm/p5.serialserver@0.0.28/lib/p5.serialport.js"></script>
Open Sketch.js
and replace into this.
let serial; // variable to hold an instance of the serialport library
function setup() {
serial = new p5.SerialPort(); // make a new instance of the serialport library
serial.on('list', printList); // set a callback function for the serialport list event
serial.list(); // list the serial ports
}
// get the list of ports:
function printList(portList) {
// portList is an array of serial port names
for (var i = 0; i < portList.length; i++) {
// Display the list the console:
console.log(i + portList[i]);
}
}
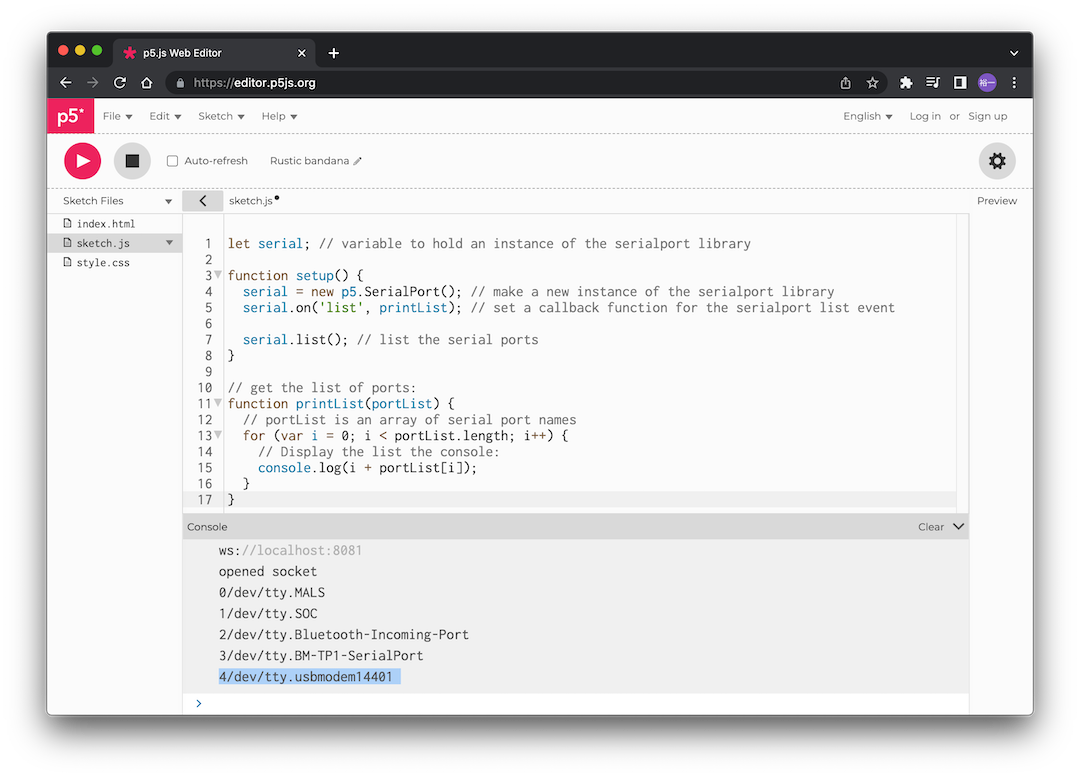
Attention
this page is not finished
Last update:
May 13, 2022