week 10. Output devices
This Week i used the read data from the DHT22 Temperature and Humidity Sensor of last week Assignment and displayed them on a I2C display on the Board i designed in week 6 and produced in Week 8. I programmed the board with the Arduino IDE shown
In our group assignment, We showed, how we measured the powerdraw inside an project. I was responsible for the Pictures and the Website.
Prepwork
board setup
First i have to setted up the Pi Pico 2 W as Borad. For that i used the RP2040 BoardLibrary from "Earle F. Philhower, III". Step 1 was to open the preferences and add "https://github.com/earlephilhower/arduino-pico/releases/download/global/package_rp2040_index.json" to "Additional boards manager URLs"
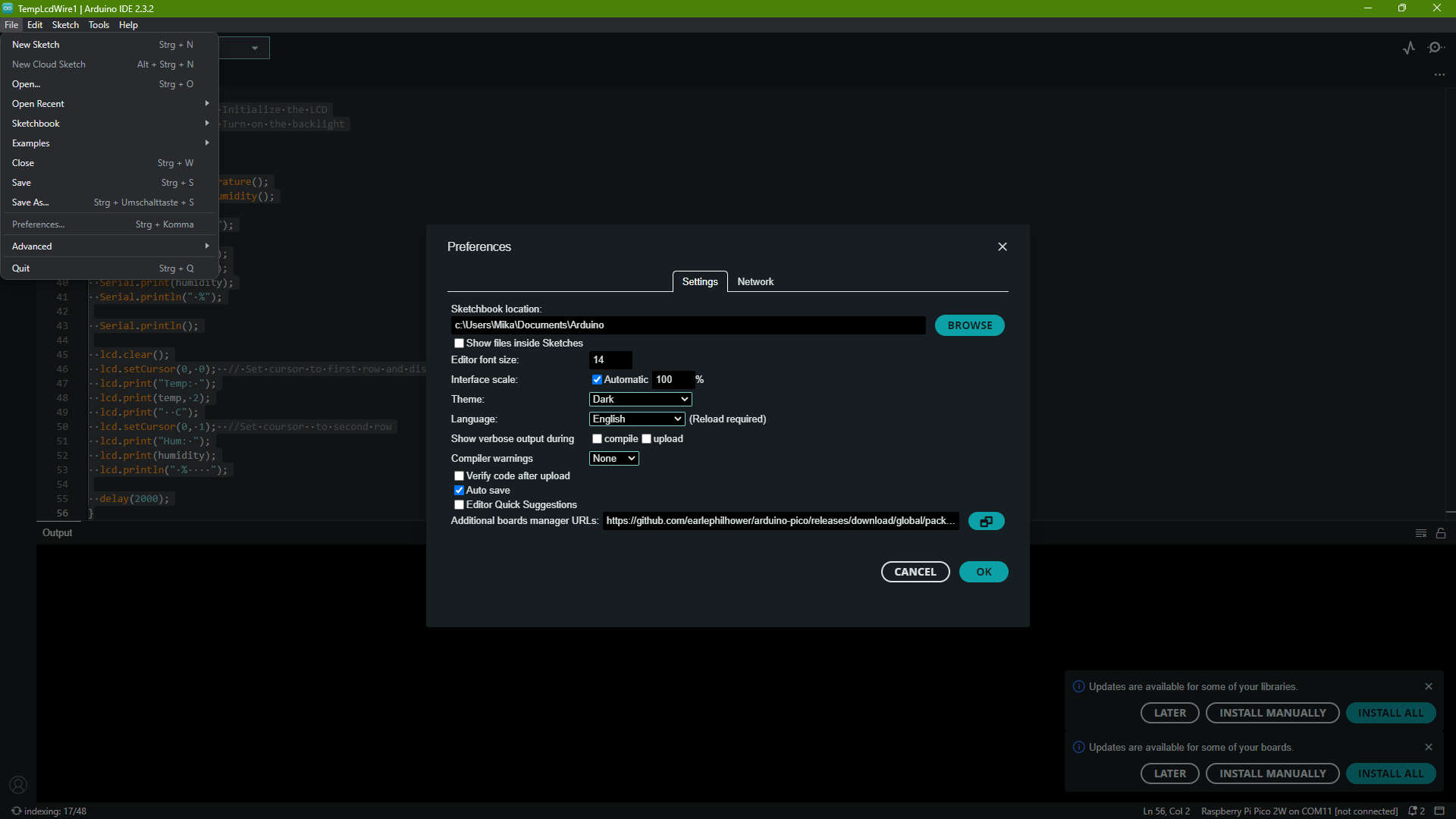
Second steo was to install the Boards in the Boards Manager.
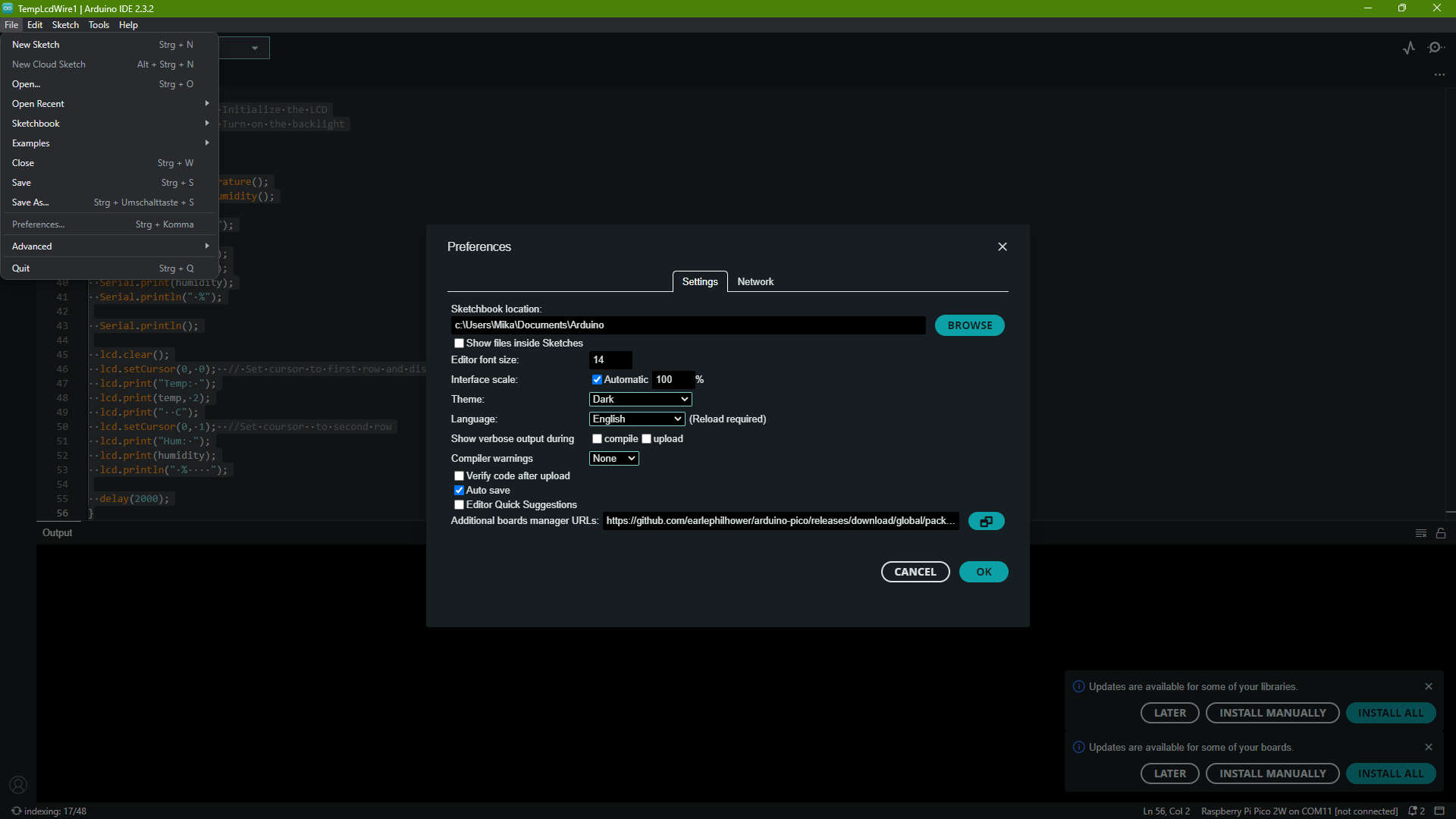
libraries
To make the code work with I2C1(second I2C-channel of the Pi Pico 2 W), I needed to change a library. As in Week 4 i used the LiquidCrystal I2C libray by Frank de Brabander. I changed all occurrences of "Wire" with "Wire1" in the LiquidCrystal_I2C.h and LiquidCrystal_I2C.cpp in the libraries folder. That means that i now uses the second i2c channel instead of the first, that also means that for futur projects i need to change it back in .cpp and .h file
connnecting the display and sensor
To Connect our Sensor, use the second GP-Pin, which is "DHT-DATA" and the power pins on our board (marked with "DHT-VCC" and DHT-GND). Every pin used for the DHT is colored Green. The Display is connected onto the Display I2C Connector colored yellow.
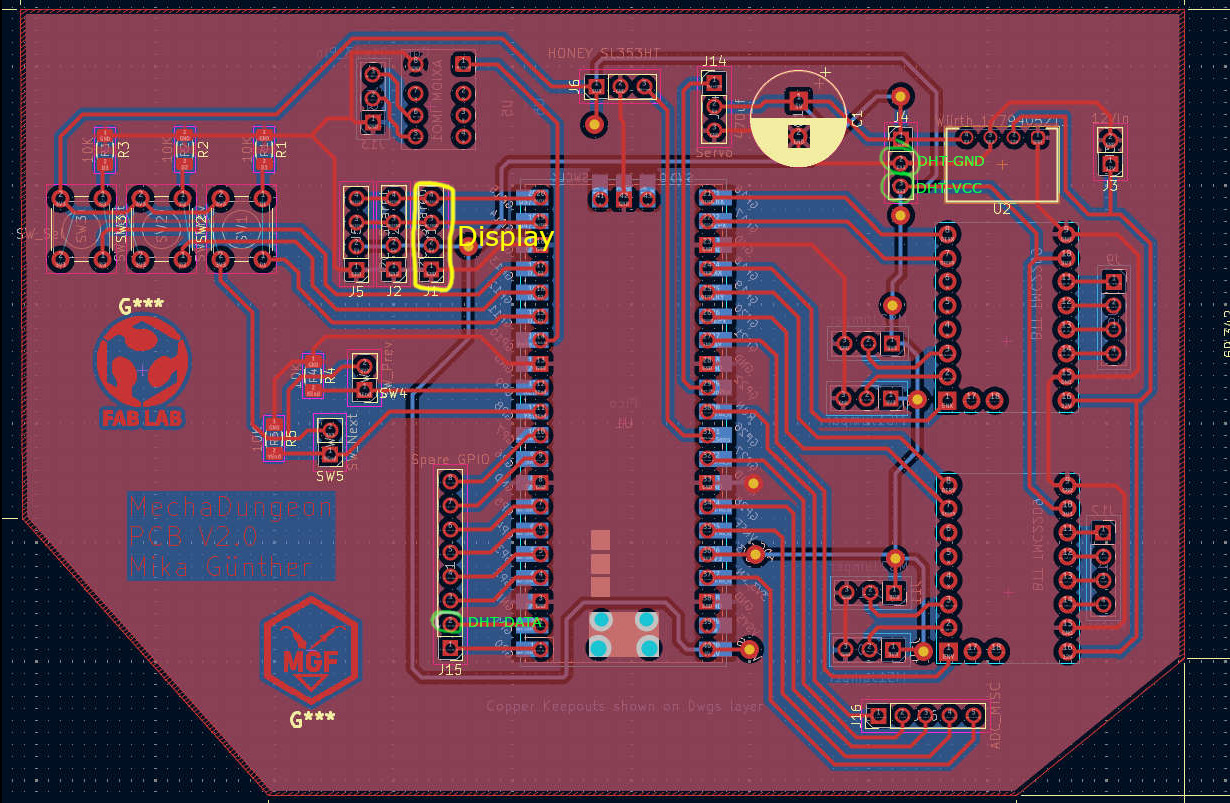
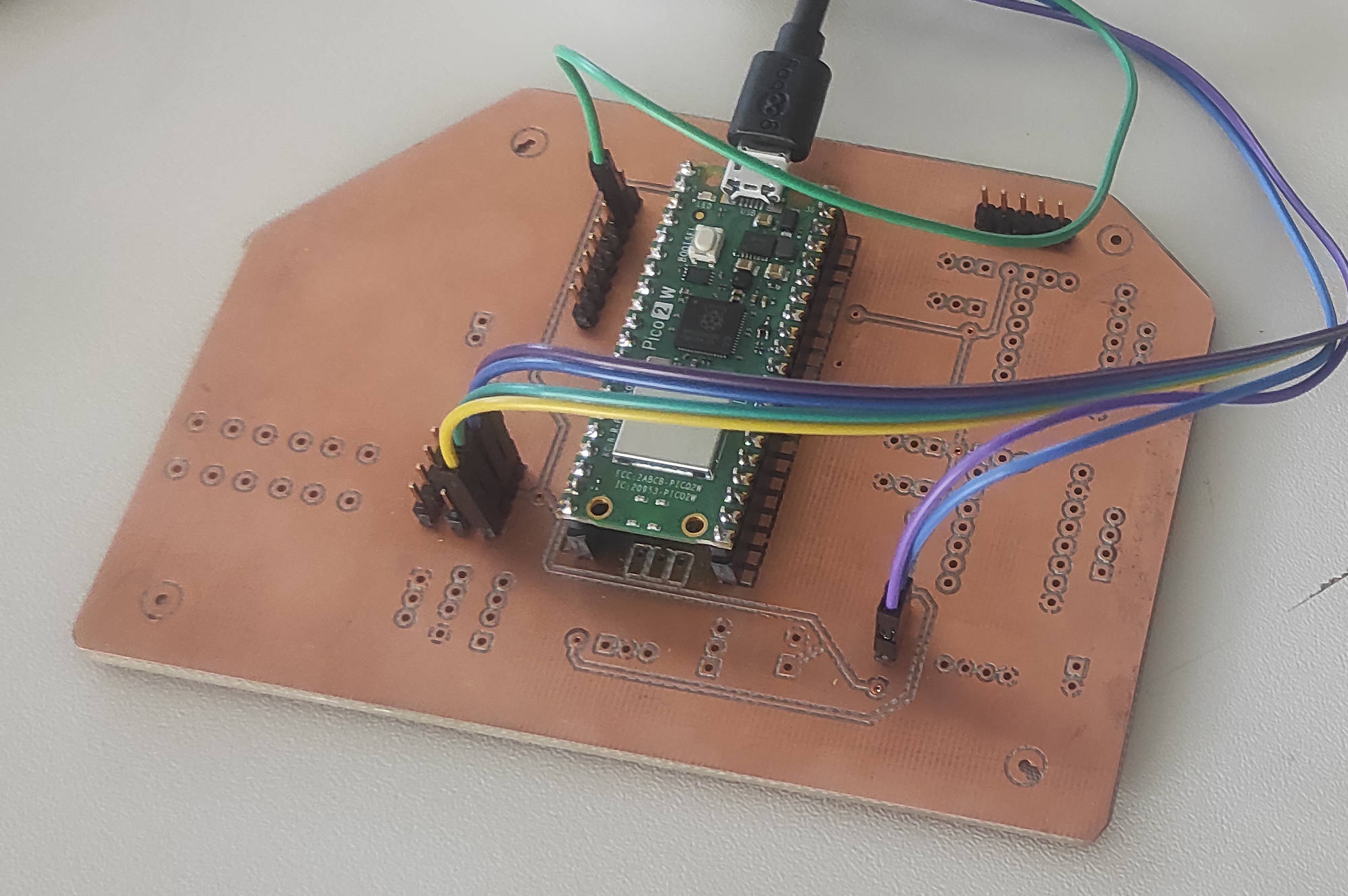
The Code
#include#include #include //DHT Setup #define DHTPIN 1 // GP1 Pin of the Pi Pico 2 W #define DHTTYPE DHT22 float temp; float humidity; DHT dht(DHTPIN, DHTTYPE); //LCD Setup LiquidCrystal_I2C lcd(0x27, 16, 2); void setup() { Serial.begin(9600); Wire1.setSDA(14); // set the SDA pin to GP14 of the Pi Pico 2W Wire1.setSCL(15); // set the SCL pin to GP15 of the Pi Pico 2W dht.begin(); lcd.init(); // Initialize the LCD lcd.backlight(); // Turn on the backlight } void loop() { // Read Sensor Data temp = dht.readTemperature(); humidity = dht.readHumidity(); // Debug Output of the SensorData to the serial Monitor Serial.print("Temp: "); Serial.print(temp); Serial.println(" *C"); Serial.print("Hum : "); Serial.print(humidity); Serial.println(" %"); Serial.println(); //Sensor Data Output on Display lcd.clear(); lcd.setCursor(0, 0); // Set cursor to first row and display lcd.print("Temp: "); lcd.print(temp, 2); lcd.print(" C"); lcd.setCursor(0, 1); //Set coursor to second row lcd.print("Hum : "); lcd.print(humidity); lcd.println(" % "); delay(2000); }