Week 14 - Interface and Application Design¶
Group assignment¶
For the group assignment we compared two development environments to create interfaces. Bas introduced Processing which is based on Java, and Bente told us how to use Node.js to communicate using the serial bus.
In the first example Bas procedurally explained how to write code that animates balls using Processing. Later he also showed how to add input using input from the serial port. Link to processing example code.
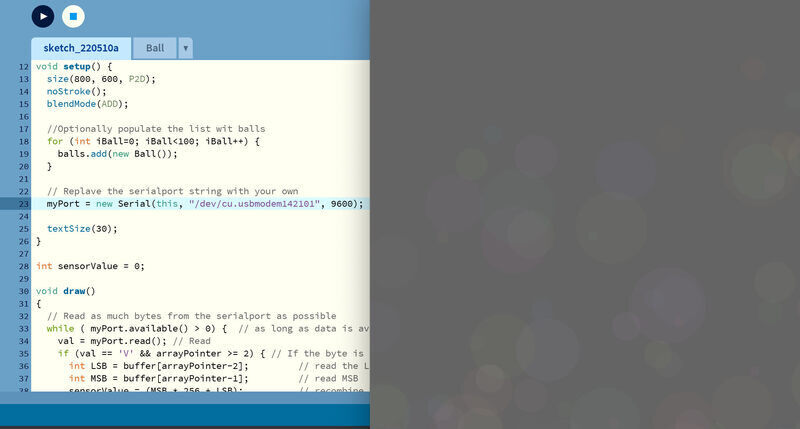
Afterwards Bente showed us how to use Node.js to turn our helloworld boards into a cat-generating device. She took us through the steps of setting up a server and a client.
Link to Node.js example code.
The code below is a part of server.js
, it uses a lambda function to define what happens when the server receives data.
// create a parser to parse the raw data
const parser = new ReadlineParser();
serialPort.pipe( parser );
// whenever we get data from the serial port....
parser.on("data", data => {
// send it to the clients
if (websocket) {
websocket.send(data);
}
else {
// or pass it to the console if there is no client connected
console.log(data);
}
});
Individual assignment¶
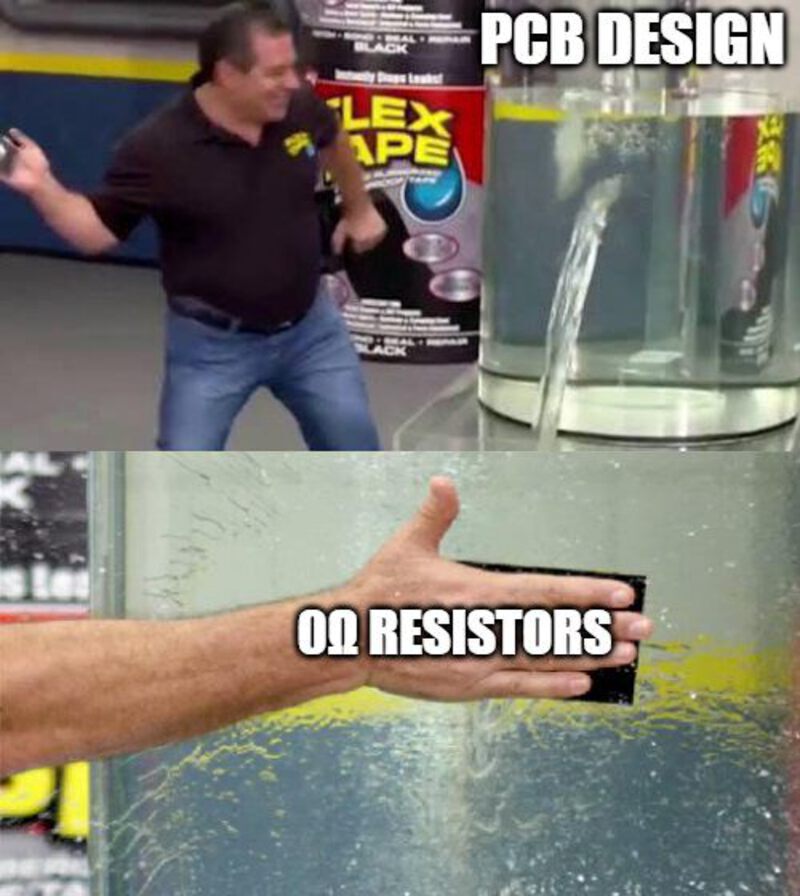
Because I’ve worked on enough web application for one lifetime, I decided to make a simple command-line interface using the examples given by Bente in Node.js. My first program uses Inquirer which is a package to create command-line dialogs. The full Node.js code can be found here.
setTimeout(() => {
choices = ["off", "on", "blink"];
inquirer.prompt([
{
type: "list",
name: "function",
message: "Select a LED function",
choices: choices
}
])
.then((answers) => {
response = answers["function"];
serialPort.write(String(choices.indexOf(response)));
console.log("Thank you..");
process.exit();
}
);
}, 1000);
The loop part of my Arduino code that listens for bytes being sent over the serial bus.
void loop() {
serialRead();
if(incomingByte == 48) { // turn LED off
digitalWrite(PIN_A1, LOW);
} else if (incomingByte == 49) { // turn LED on
digitalWrite(PIN_A1, HIGH);
} else while(incomingByte == 50) { // blink LED
digitalWrite(PIN_A1, HIGH);
delay(500);
digitalWrite(PIN_A1, LOW);
delay(500);
serialRead();
}
delay(500);
}


After this, I decided to make another CLI program which was more like a GUI. It should show the measured brightness on my hellworld board over time. For this, I used a really nice library called CLUI. Using the provided examples and combining them with the serial reading code that Bente provided for the group assignment I was able to get the UI to display the light measurements over time. The full Node.js code can be found here.
I uploaded the following simple sketch to my helloworld board to write the measurements over the serial bus.
void setup() {
Serial.begin(9600);
pinMode(PIN_PA3, INPUT);
}
void loop() {
Serial.println(analogRead(PIN_PA3));
delay(200);
}

Conclusions¶
- Node.js is very good for all kinds of stuff, web and cli, but I don’t like programming in it.
- Processing is a nice wrapper for Java so that you don’t need frames.
- It is probably better to communicate over a network using a protocol rather than raw serial data.