Week 11 - Machine Design¶
For more complete notes, please refer to the group page for this assignment.
Notes from Wednesday:¶
- T-slotted framing rails are good to make machine frame.
- Carriers can be printed or made from tape measure tape.
- Series elastics example are springs that make actuators more smooth.
- Roller wheels/pulley wheels.
First brainstorm¶
Benjamin will work remote so we will have to use ‘spiral’ design. Bente wants to make something with wooden gears, for instance a drawbridge. If possible a wave simulator because she needs it for her final project. Joany’s idea is a moving surface pattern wall-mounted thingy. There are videos showing the inner workings. Daniel Rozin like thing with mirrors. Reacts to movement. - https://www.youtube.com/watch?v=IvUU8joBb1Q&ab_channel=Wintergatan Jonathan’s idea was a general idea to build something useful for the lab, like the wax-cutting machine. Ben’s idea instrument guitar/flute mechatronic, xylophone on which marbles are dropped. - https://en.wikipedia.org/wiki/Computer_Controlled_Acoustic_Instruments_pt2 - http://www.markbischof.com/nl/ - https://www.youtube.com/watch?app=desktop&v=4f1J5LzRdIo
The shield should be 5V 2-5A.
Day 0: Printed out brackets, wrote non-blocking servo movement code. Created Mattermost channel. Day 1: Printed out brackets, tested sensors, milled pcb. Day 2: Wrote code that does a wave. Day 3: Debugging servo’s, found some broken ones, labelled the other ones, put together a prototype, tested it. Day 4: Lasercut a larger backboard, looked at different code that works better, printing bracket. Day 5: Putting everything together, fine-tuning dimensions.
#include <VarSpeedServo.h>
// configuration
const int arraySize = 3; // how many servos are connected
const int turnSpeed = 50; // how fast should they move
const int offset = 300; // how far apart should they start moving
const int pinArray[] = {2, 3, 4}; // what pins are the servos on
const int limitArray[] = {45, 135}; // sets the min and max degrees
VarSpeedServo servoArray[arraySize];
unsigned long currentTimeInCycle = 0;
bool stepArray[arraySize] = {true, true, true};
bool resetArray[arraySize] = {true, true, true};
void setup() {
Serial.begin(9600);
Serial.println("Serial connection setup..");
for(int i=0; i < arraySize; i++){
VarSpeedServo iServo;
iServo.attach(pinArray[i]);
servoArray[i] = iServo;
}
set45Degrees(); // put all pixels at same orientation
delay(2000); // wait two seconds before starting
}
void loop() {
unsigned long startTime = millis(); // tick
for(int i=0; i < arraySize; i++){
// check whether or not we are starting a new cycle, if so reset the cycle flags
if(currentTimeInCycle >= (arraySize * offset)) {
resetCycleFlags(resetArray, arraySize);
currentTimeInCycle = currentTimeInCycle % (arraySize * offset);
}
// check if the timer is above the limit for this servo and if it hasn't already been actuated
if(currentTimeInCycle >= i * offset && resetArray[i] == true) {
servoArray[i].write(limitArray[stepArray[i]], turnSpeed);
stepArray[i] = !stepArray[i];
resetArray[i] = false;
}
}
unsigned long endTime = millis(); // tock
currentTimeInCycle += endTime - startTime;
}
void resetCycleFlags(bool resetArray[], int arraySize) {
for(int i=0; i < arraySize; i++){
resetArray[i] = true;
}
}
void set45Degrees() {
for(int i=0; i < arraySize; i++){
servoArray[i].write(45);
}
}
I worked on a piece of code which worked on a small amount of servos to create a wave. At first I used the VarSpeedServo library for this, but this library was limited to 8 servos only. So I switched back to the more basic “Servo.h” library which supports up to 48 servos on the Arduino Mega. The issue was that some servos would move and others would not, from logging output I could see that the parameters being sent to the library method were correct, however, some servos would consistently not move. For smaller amounts of servos it seemed to work without problem.
A different example that we found that did work for large amounts of servos used a slightly different method from the same library.
for(int nServo = 0;nServo < CONNECTED_SERVOS;nServo++)
{
myServos[nServo].writeMicroseconds(nPulseWidth);
}
Also, in stead of giving the servos a destination orientation immediately the program moves the servos by a small amount each iteration. I adapted my above code a little to work with the writeMicroseconds method, however, I still wasn’t able to get the code running as expected. It looks like arrays work slightly differently from what I’m used to in Java/Python.
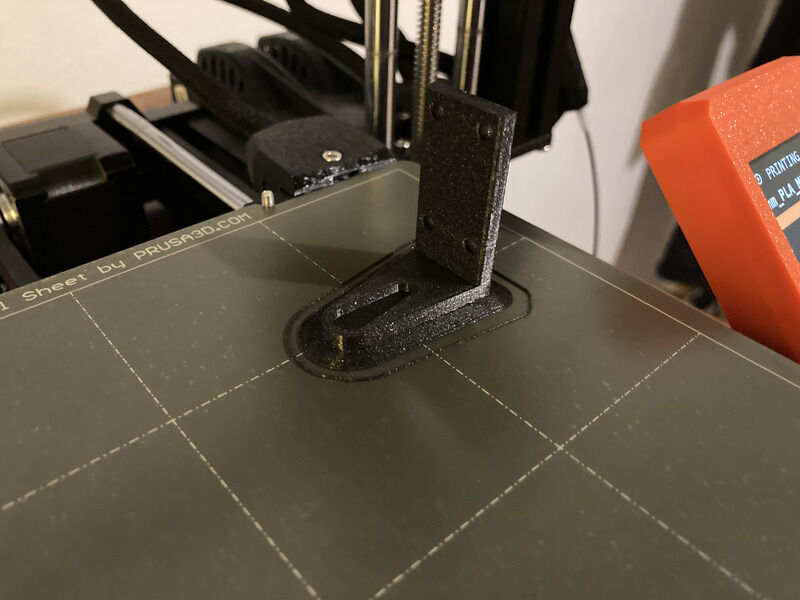
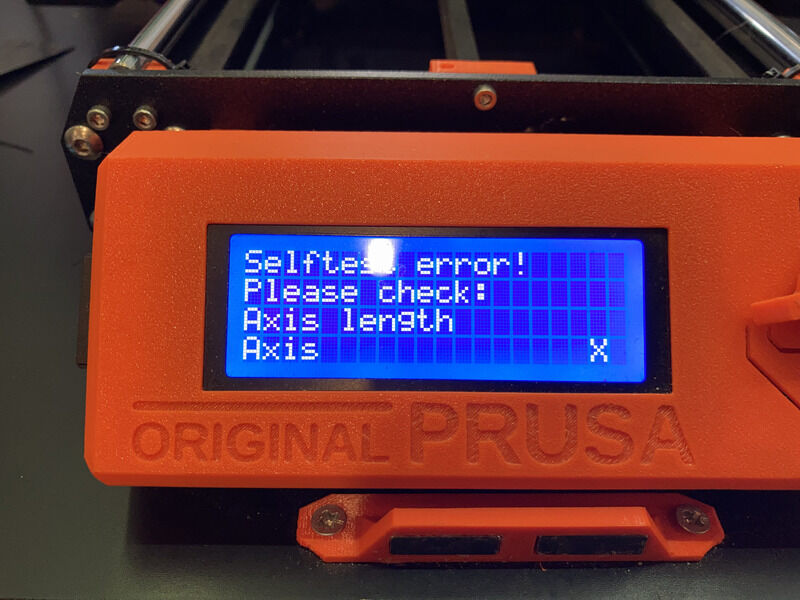
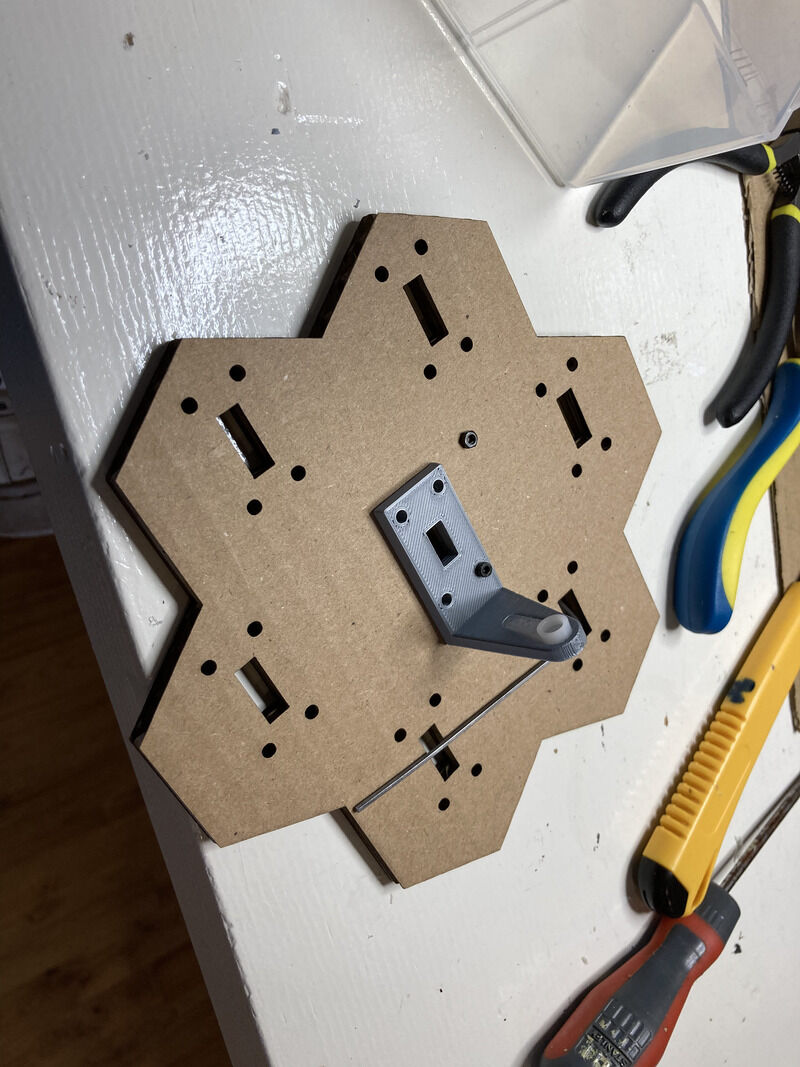
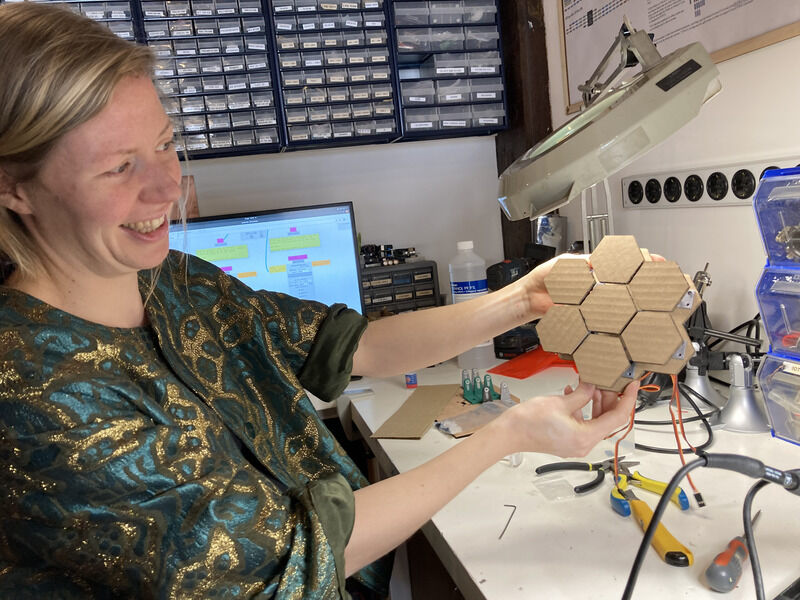
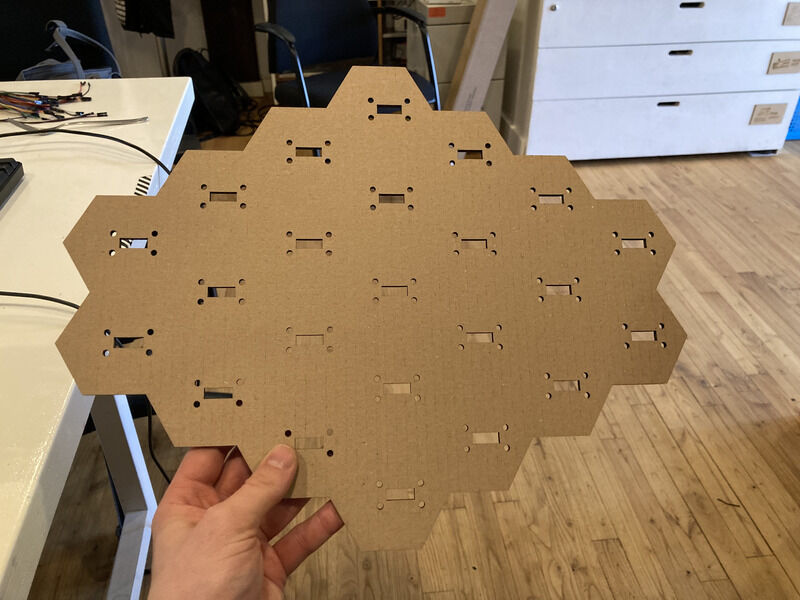
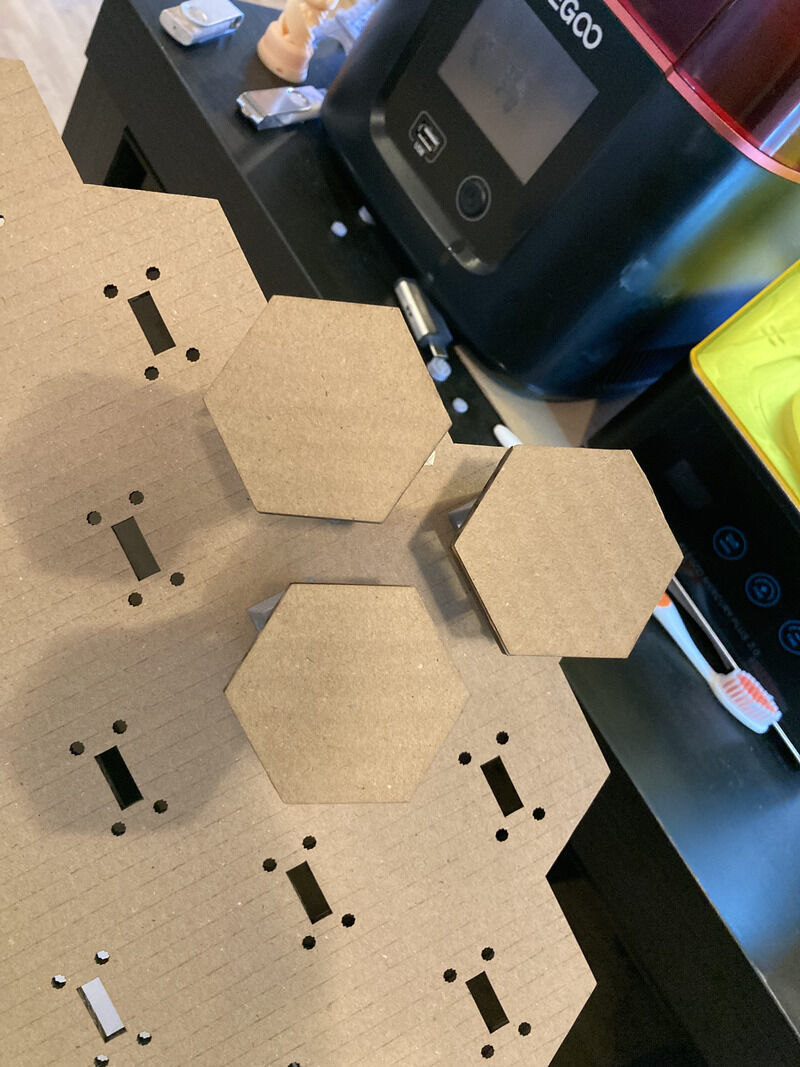
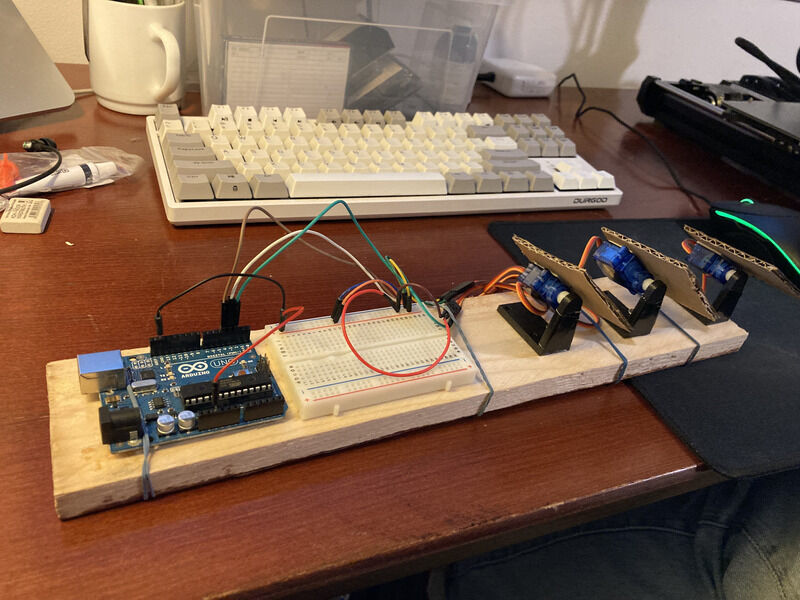
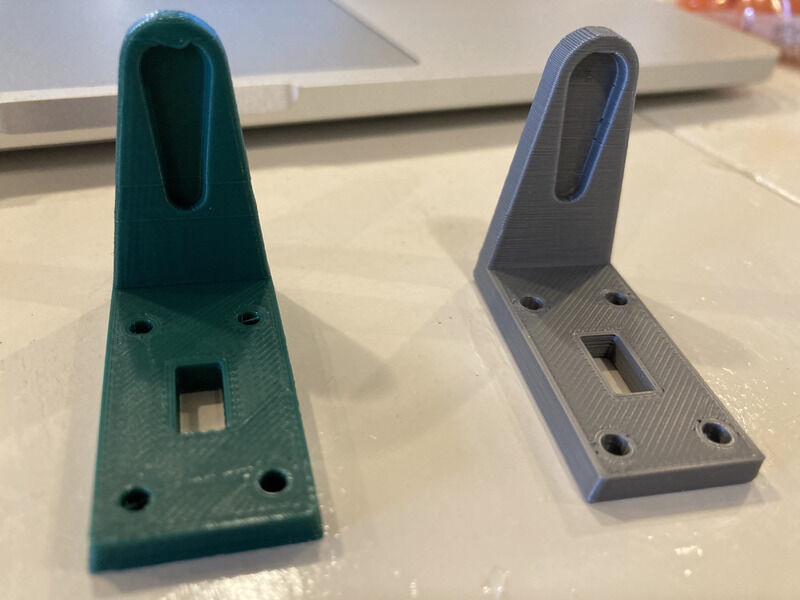
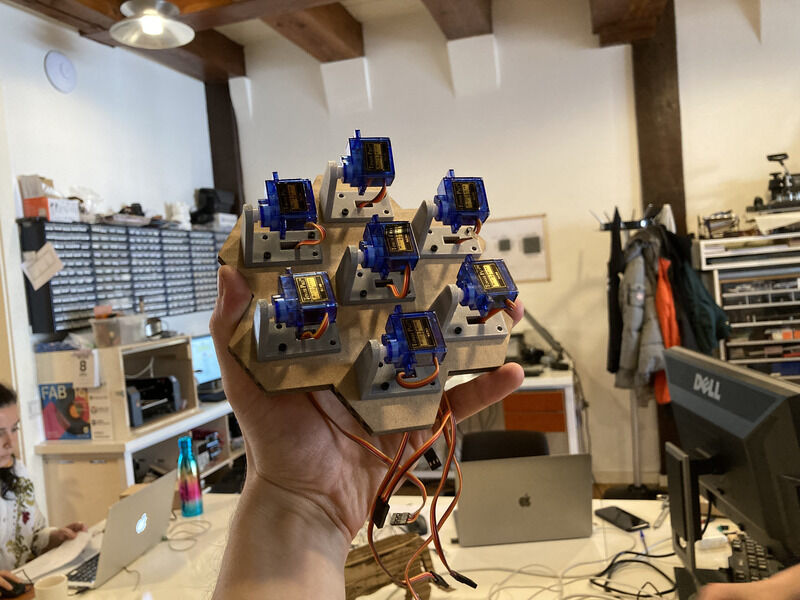
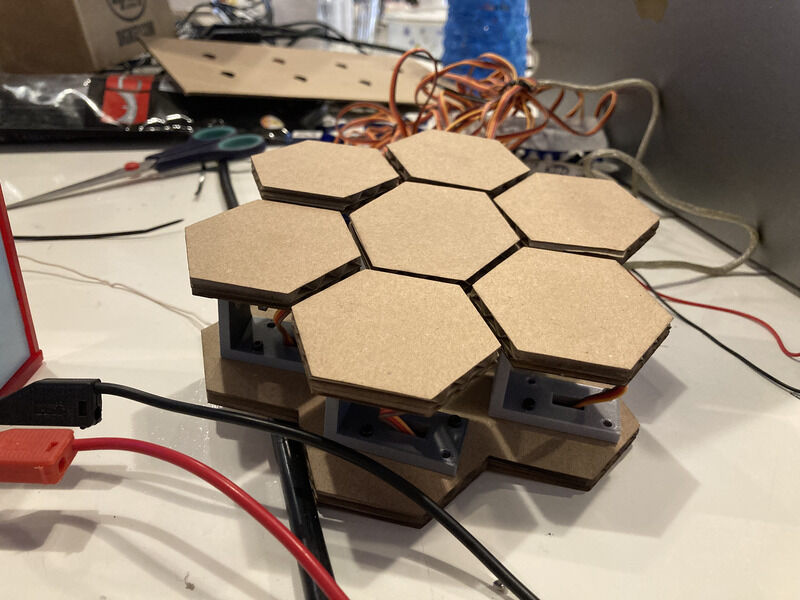
In this code written for the Adafruit board, the maker attaches the on-board oscillator to modulate the pulsewidth that drives the servos. I think this is also what we should have done rather than create timers in software. The maker also added code which accounts for unexpected states, such as an angle being beyond a limit. I think this is something we completely ignored because we expected the devices to work perfectly, which they don’t.
void loop() {
void loop() {
setC1((1+(1+sin(millis() - PI))/2) * 60);
setC2((1+(1+sin(millis() - PI * 0.66))/2) * 60);
setC3((1+(1+sin(millis() - PI * 0.33))/2) * 60);
setC4((1+(1+sin(millis()))/2) * 60);
setC5((1+(1+sin(millis() + PI * 0.33))/2) * 60);
setC6((1+(1+sin(millis() + PI * 0.66))/2) * 60);
setC7((1+(1+sin(millis() + PI))/2) * 60);
delay(delayMs);
}
}
Conclusions¶
- The devices we use aren’t perfect and it needs to be accounted for in the code.
- It is more stable to use timers and functions from the board directly than to create them in software.