04 Assignment (Embedded Programming)
This week's assignment is all about embedded programming. What is embedded programming?
Embedded programming is code written and stored on a microcontroller. You probably have
one near you right now, like in your Bluetooth mouse! The microcontroller inside has a set
of instructions that tell it what to do when you move it or click a button.
Microcontrollers are perfect for small tasks, like turning on your Bluetooth
headset or controlling appliances. In contrast, your computer uses a different
system because it needs to run multiple programs at once.
There are many different types of microcontrollers, and we compared them in our
group assignment to see what the main differences are.
This weeks to-do list
Intro to the Arduino R3
The Arduino Uno R3 is a very popular microcontroller board, based on the ATmega328P chip.
It features 14 digital input and output pins, six of which can be used as PWM outputs,
six analog inputs, a USB connection that can be used for programming the board, and a power
jack—not to mention a reset button. The Arduino Uno R3 can be powered by either USB or an
external power supply. The ATmega328P is an 8-bit microcontroller and is part of the AVR family,
making it optimized for low power consumption and high performance.
features
For more in formation here is a link to the Data sheet.
How the R3 is setup
The boards itself is labeled giving you a hint on which pin you are using. Including the Grounds, 5 Volt and 3.3 Volt pins.
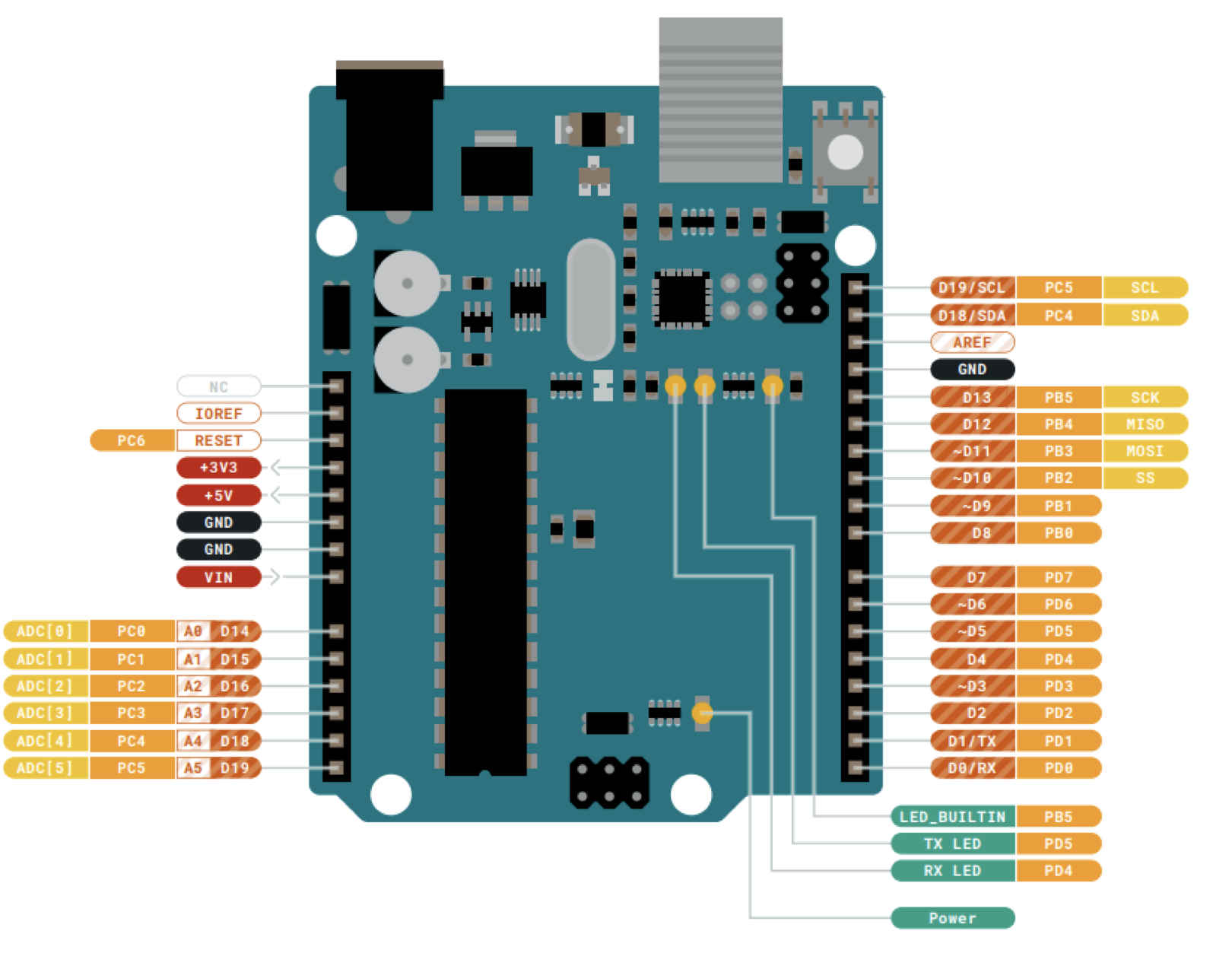
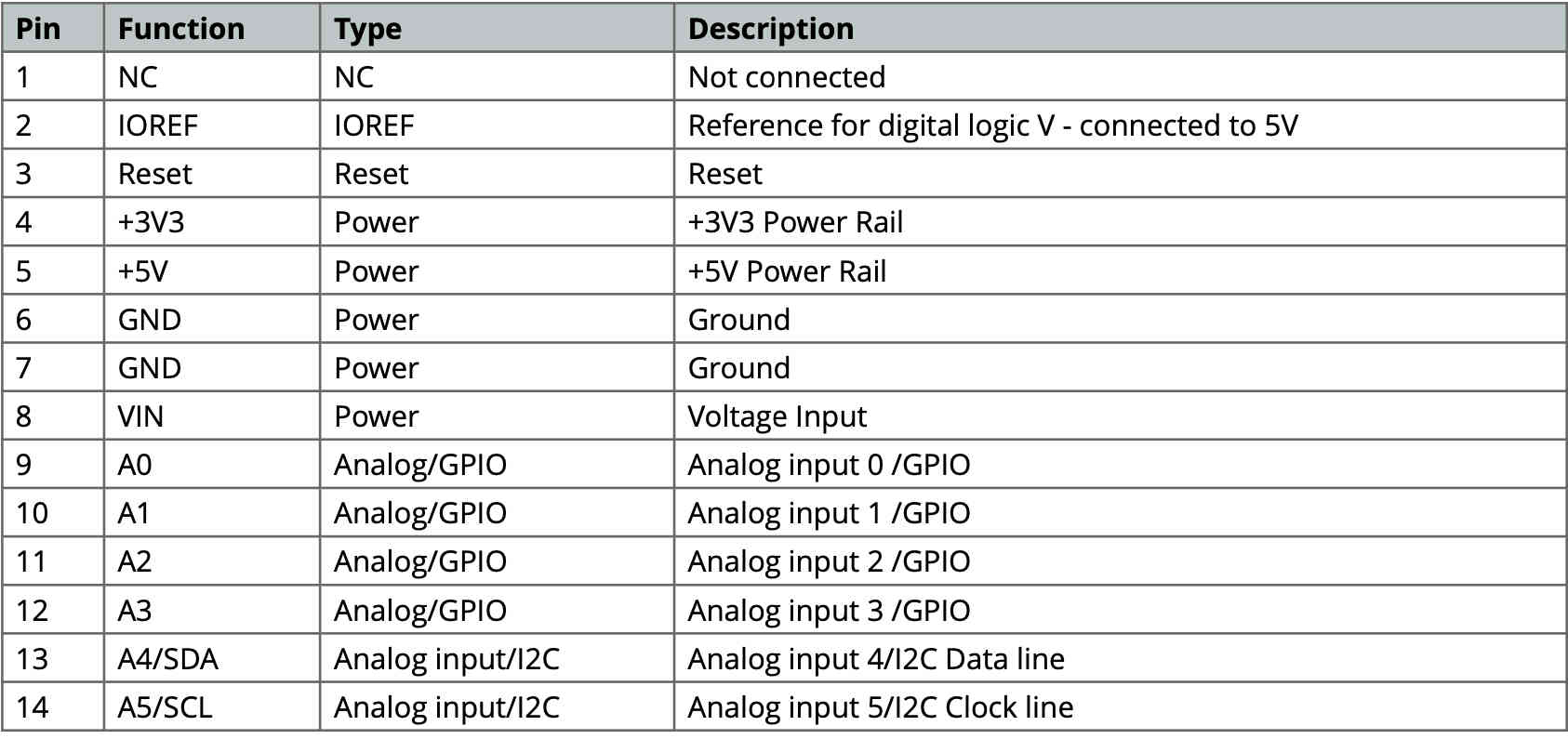
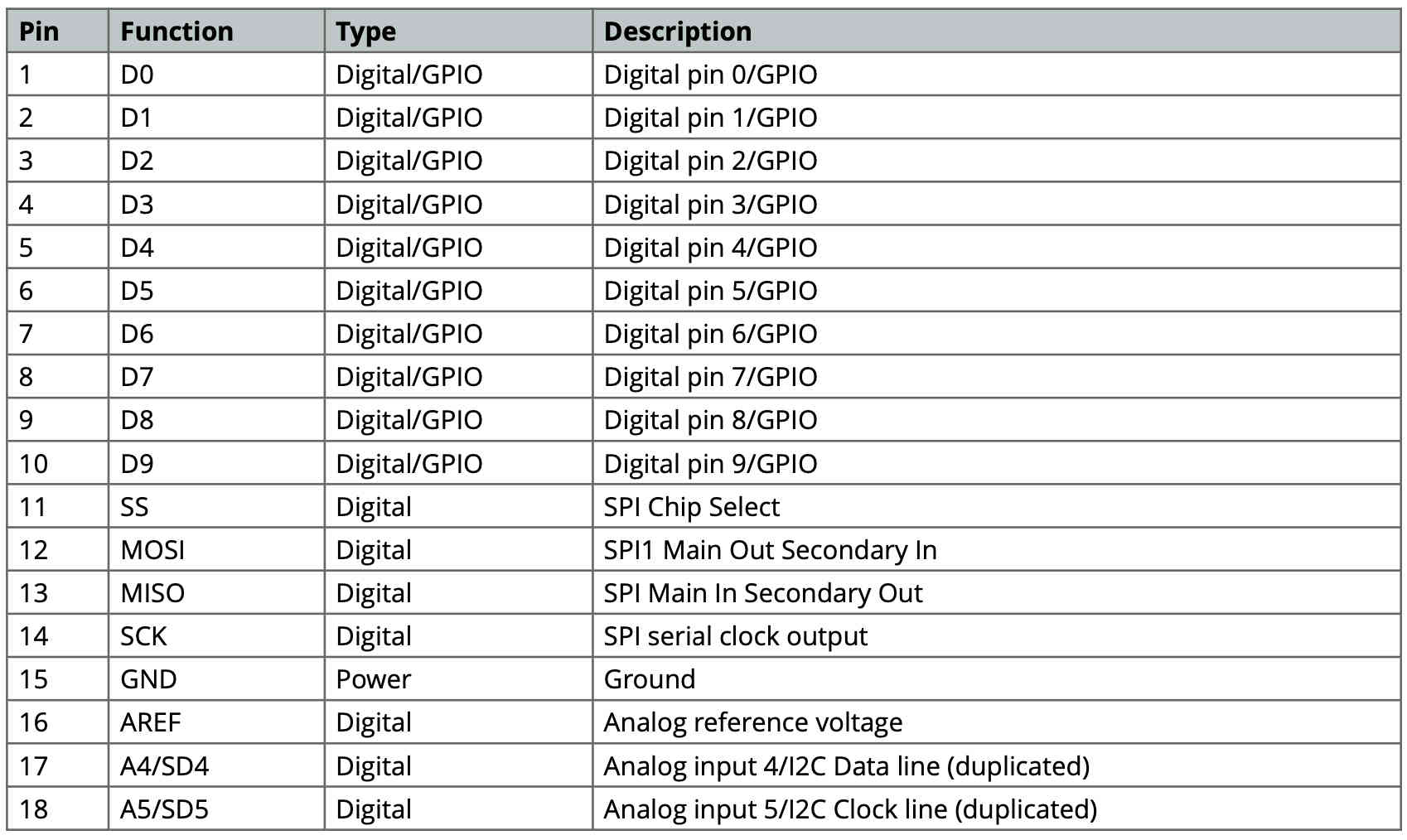