Week 09 Input devices
Testing sensors
For this week, I'll be using the board I previously manufactured in Week 8. I added two analog sensors that will work together: on one side (left), there's an infrared distance sensor, and on the other (right), a simple joystick. My idea is that if the sensor detects an object within a minimum distance of 20 cm, it will turn on the two LEDs on my board. Meanwhile, the joystick—only when the LEDs are on—will control their brightness, with each axis of the joystick assigned to a different LED.
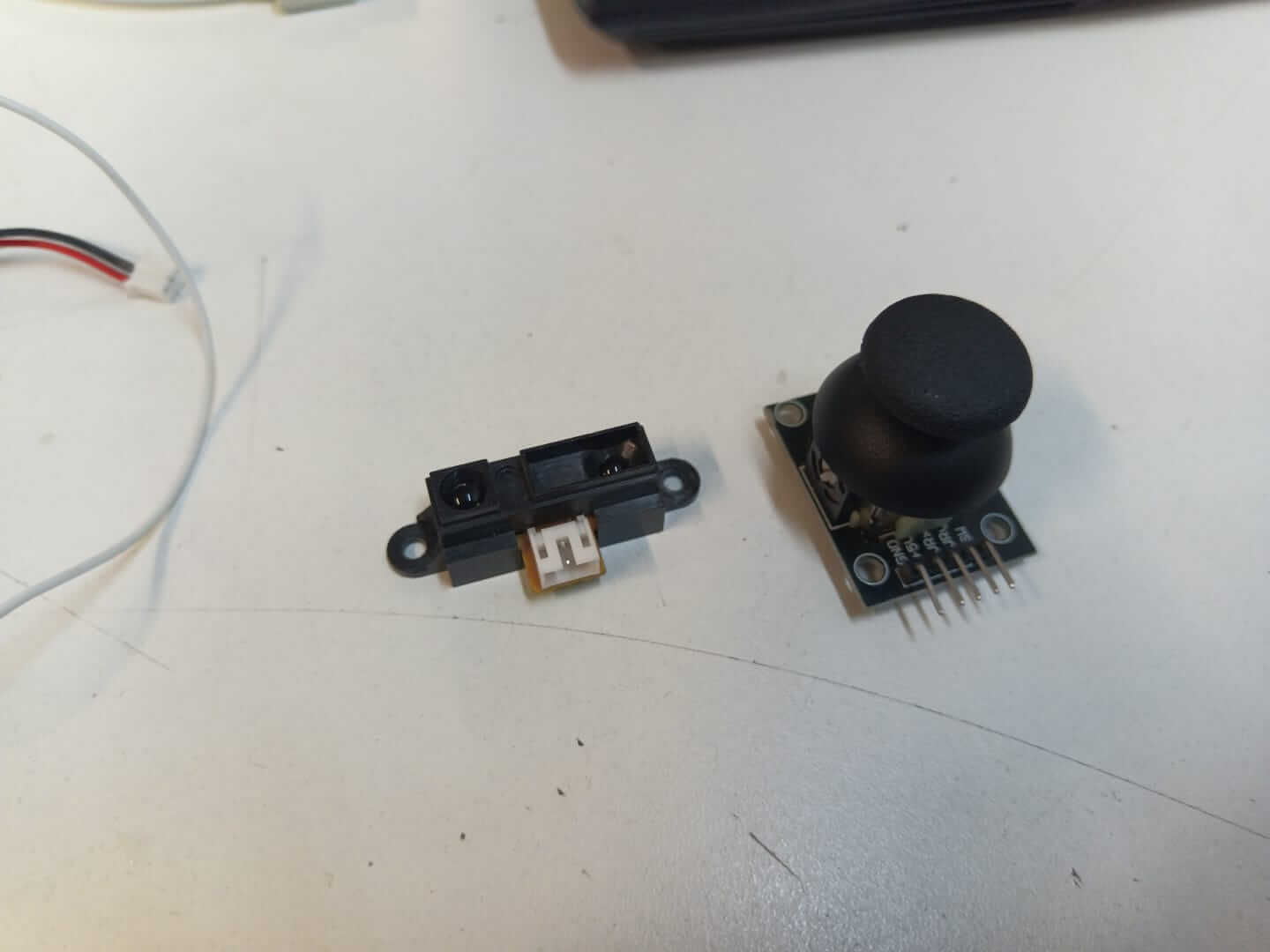
Analog signals are continuous and can take any value within a given range. This means that an analog signal varies smoothly over time, like the voltage output from a potentiometer or the sound waves detected by a microphone. Microcontrollers read these signals using an ADC (Analog-to-Digital Converter), as seen in the analogRead() function used for the potentiometers and the distance sensor. On the other hand, digital signals are discrete, meaning they can only have two possible states: HIGH (1) or LOW (0). This makes them ideal for binary operations, such as turning an LED on or off, reading a button press, or communicating through digital protocols like I2C and SPI. In this program, digital signals are used when controlling the LEDs with digitalWrite(), where the LEDs are either fully on or completely off. The key difference between these types of signals lies in precision and processing. Analog signals provide more detailed and continuous information but require additional conversion to be processed digitally. Digital signals, in contrast, are simpler to handle and more resistant to noise, making them ideal for applications where only on/off states are needed.
This is how the connection of both sensors looks in relation to my microcontroller (I should clarify that I'm aware the sensor in the image, while it is a distance sensor, is ultrasonic, whereas the one I use is infrared—it's just there to give an idea of the connection, since the website doesn't have that specific sensor).
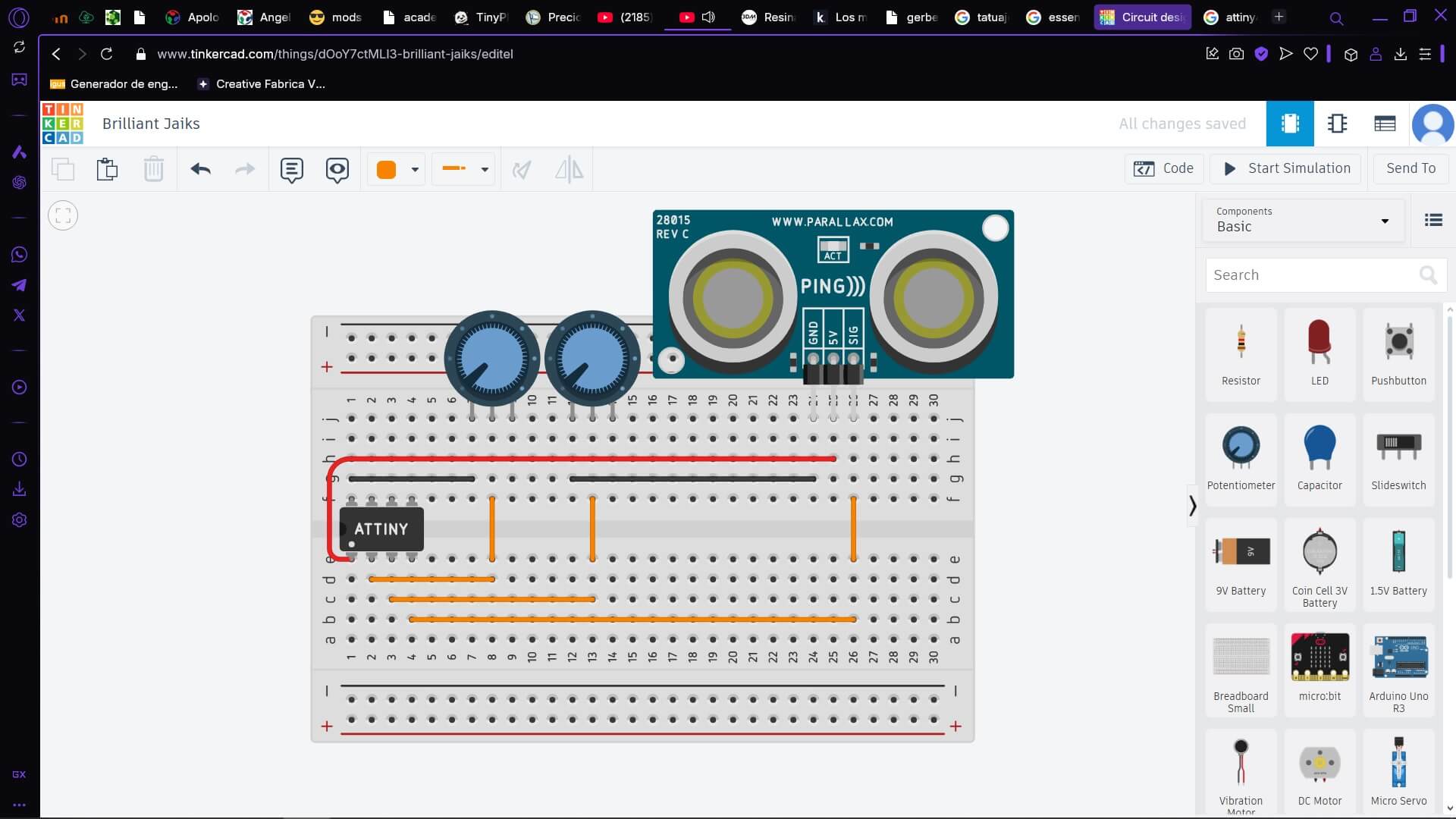
This is how my physical connections look like
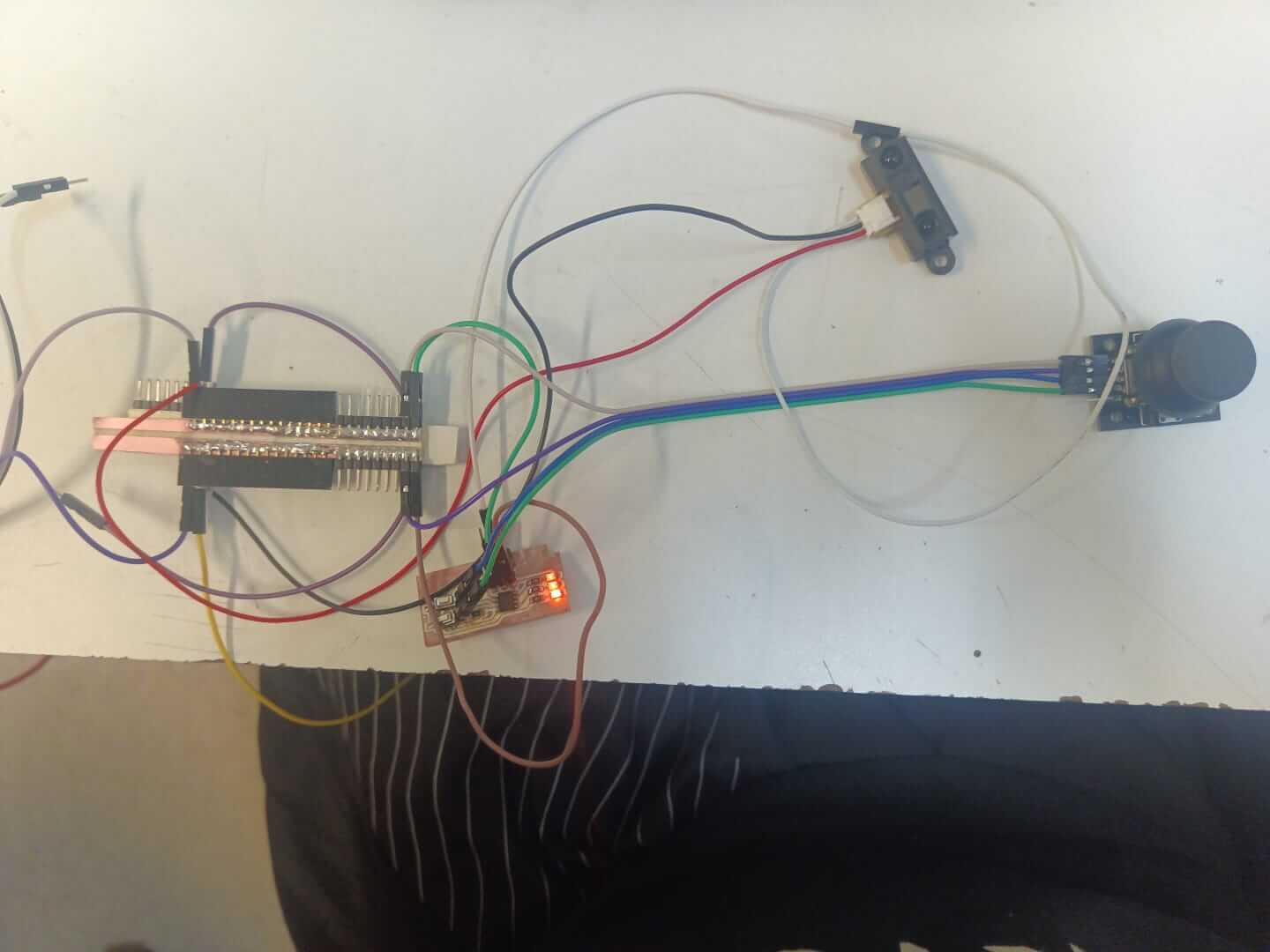
Here's the code I used:
// Joystick and distance sensor #define POT_PIN_1 0 // Analog pin where the first potentiometer is connected #define POT_PIN_2 1 // Analog pin where the second potentiometer is connected #define LED_PIN_1 4 // PWM pin where the first LED is connected #define LED_PIN_2 3 // PWM pin where the second LED is connected #define SENSOR_PIN 2 // Analog pin where the distance sensor is connected #define THRESHOLD_CM 20 // Distance in cm to activate the LEDs void setup() { pinMode(LED_PIN_1, OUTPUT); pinMode(LED_PIN_2, OUTPUT); pinMode(POT_PIN_1, INPUT); pinMode(POT_PIN_2, INPUT); pinMode(SENSOR_PIN, INPUT); } void loop() { // Read the values from the potentiometers int potValue1 = analogRead(POT_PIN_1); // Read the first potentiometer (0-1023) int potValue2 = analogRead(POT_PIN_2); // Read the second potentiometer (0-1023) // Map the potentiometer values to the PWM range (0-255) int brightness1 = map(potValue1, 0, 1023, 0, 255); int brightness2 = map(potValue2, 0, 1023, 0, 255); // Read the distance sensor value and convert it to distance in cm int sensorValue = analogRead(SENSOR_PIN); float distance = convertToDistance(sensorValue); // If the distance is less than or equal to the threshold, turn on the LEDs if (distance <= THRESHOLD_CM) { analogWrite(LED_PIN_1, brightness1); // Adjust the brightness of the first LED analogWrite(LED_PIN_2, brightness2); // Adjust the brightness of the second LED } else { digitalWrite(LED_PIN_1, LOW); // Turn off the first LED digitalWrite(LED_PIN_2, LOW); // Turn off the second LED } delay(100); // Small delay for stability } // Function to convert the analog reading to distance in cm float convertToDistance(int value) { // Approximate formula for the sensor return 2076.0 / (value - 11.0); }
Pin definitions
The first section of the code defines the pin assignments for the potentiometers, LEDs, and distance sensor. The potentiometers are connected to analog pins 0 and 1, allowing them to provide variable voltage readings between 0 and 1023. The LEDs are connected to PWM-enabled pins, meaning their brightness can be adjusted through pulse-width modulation. The distance sensor is connected to an analog pin and will be used to determine when the LEDs should turn on. The constant THRESHOLD_CM represents the maximum distance at which the LEDs should be activated.
#define POT_PIN_1 0 // Analog pin where the first potentiometer is connected #define POT_PIN_2 1 // Analog pin where the second potentiometer is connected #define LED_PIN_1 4 // PWM pin where the first LED is connected #define LED_PIN_2 3 // PWM pin where the second LED is connected #define SENSOR_PIN 2 // Analog pin where the distance sensor is connected #define THRESHOLD_CM 20 // Distance in cm to activate the LEDs
Setup() function
In the setup() function, the microcontroller configures the LEDs as outputs, meaning they will receive signals from the board. The potentiometers and the distance sensor are set as inputs, allowing the microcontroller to read their values. Since setup() runs only once when the board starts, this initialization ensures that all components are correctly set up before the main loop begins execution
void setup() { pinMode(LED_PIN_1, OUTPUT); pinMode(LED_PIN_2, OUTPUT); pinMode(POT_PIN_1, INPUT); pinMode(POT_PIN_2, INPUT); pinMode(SENSOR_PIN, INPUT); }
loop() function
The loop() function continuously executes while the microcontroller is running. It reads sensor values, processes them, and adjusts the LEDs accordingly. It first reads the potentiometer values, maps them to a usable range for LED brightness, then reads the distance sensor and checks if the object is within the threshold distance. If the object is close enough, it adjusts LED brightness; otherwise, it turns the LEDs off.
void loop() { int potValue1 = analogRead(POT_PIN_1); // Read the first potentiometer (0-1023) int potValue2 = analogRead(POT_PIN_2); // Read the second potentiometer (0-1023) int brightness1 = map(potValue1, 0, 1023, 0, 255); int brightness2 = map(potValue2, 0, 1023, 0, 255); int sensorValue = analogRead(SENSOR_PIN); float distance = convertToDistance(sensorValue); if (distance <= THRESHOLD_CM) { analogWrite(LED_PIN_1, brightness1); analogWrite(LED_PIN_2, brightness2); } else { digitalWrite(LED_PIN_1, LOW); digitalWrite(LED_PIN_2, LOW); } delay(100); }
Mapping potentiometer values
The map() function scales the potentiometer readings from their original range (0-1023) to a range that corresponds to PWM signals (0-255). This allows the potentiometers to control LED brightness smoothly. The higher the potentiometer value, the brighter the LED will be.
int brightness1 = map(potValue1, 0, 1023, 0, 255); int brightness2 = map(potValue2, 0, 1023, 0, 255);
Reading and converting distance
The analog value from the distance sensor is read and stored in sensorValue. Since this raw reading does not represent a direct distance in centimeters, it is passed to the convertToDistance() function. This function applies a mathematical formula to estimate the actual distance based on the sensor's characteristics.
int sensorValue = analogRead(SENSOR_PIN); float distance = convertToDistance(sensorValue);
LED control logic
This section determines whether the LEDs should be turned on or off based on the measured distance. If the detected object is within THRESHOLD_CM, the LEDs are turned on with brightness levels determined by the potentiometers. Otherwise, they are turned off. This logic ensures that the LEDs react dynamically to both distance and user input from the potentiometers.
if (distance <= THRESHOLD_CM) { analogWrite(LED_PIN_1, brightness1); analogWrite(LED_PIN_2, brightness2); } else { digitalWrite(LED_PIN_1, LOW); digitalWrite(LED_PIN_2, LOW); }
Delay for stability
The delay(100); function pauses the execution of the loop for 100 milliseconds. This prevents excessive fluctuations in sensor readings and ensures smoother behavior. Without a delay, the LEDs might flicker due to rapid changes in sensor readings.
delay(100);
Convert sensor value to distance
This function converts the raw sensor reading into a meaningful distance value in centimeters. The formula 2076.0 / (value - 11.0) is derived from the sensor's specifications and provides an approximation of the actual distance. It allows the program to work with real-world measurements instead of arbitrary sensor values.
float convertToDistance(int value) { return 2076.0 / (value - 11.0); }
This is what the distance sensor signal looks like.
And this is what the PWM signal from the potentiometer looks like.
This is how it works.
This week I didn't have any issues beyond finding sensors that suited me better. This is because I've previously worked with both analog and digital sensors, although in this case I didn't show any digital ones.
Here you have the link to our group page
Here you can find the files of each process: