Week 11 Embedded Networking and Communications
Testing sensors
aaa
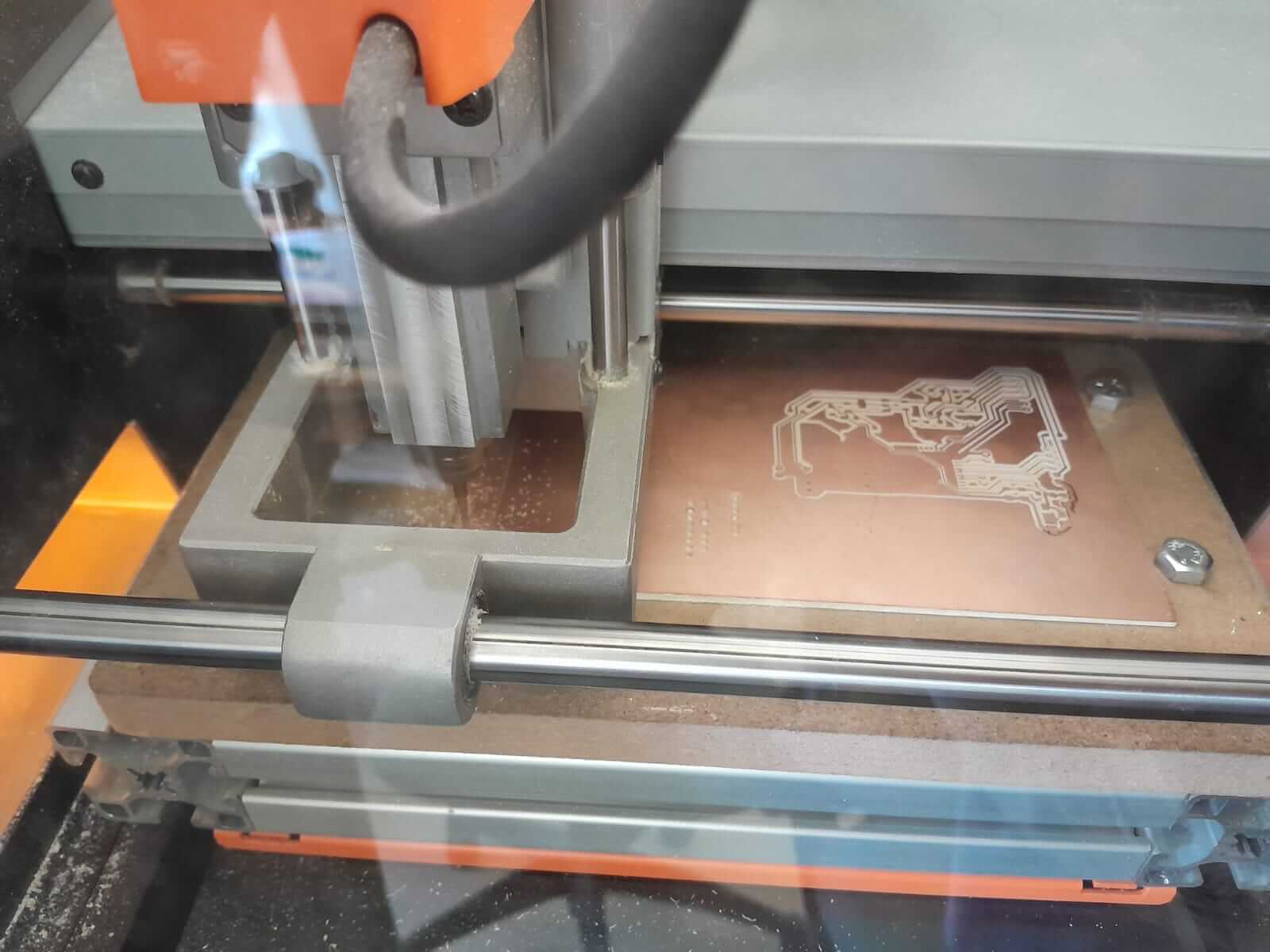
Here's the code I used:
//joystick y sensor de distancia
reflexion
Here you have the link to our group page
Here you can find the files of each process:
aaa
Here's the code I used:
//joystick y sensor de distancia
reflexion
Here you have the link to our group page
Here you can find the files of each process: