Week 10 Output devices
Testing motors
For this week, I'll be using the board I previously manufactured in Week 8. Likewise, since I didn't want to spend more than the 5 calories I burn thinking about what to do, I reused the code from my Week 4. However, I adjusted it for the ATtiny414, completely removing the use of an H-bridge and a button, simply because I don't have enough pins for so many functions.
This is how the physical connection of my circuit looks. I must say that I only used the breadboard to connect components and the power supply for the servomotors.
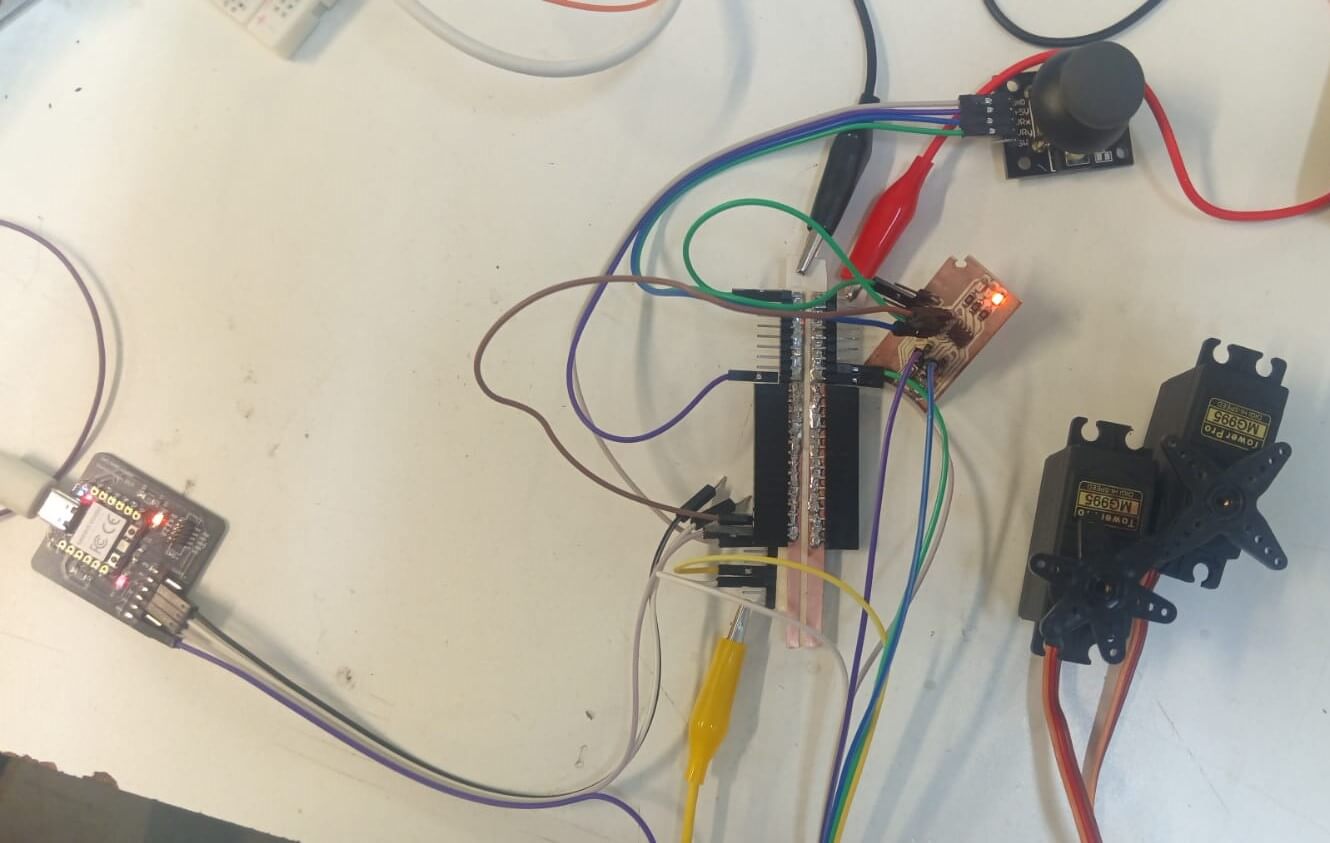
Here is a better wiring diagram made in Tinkercad, providing a clearer understanding of the connections.
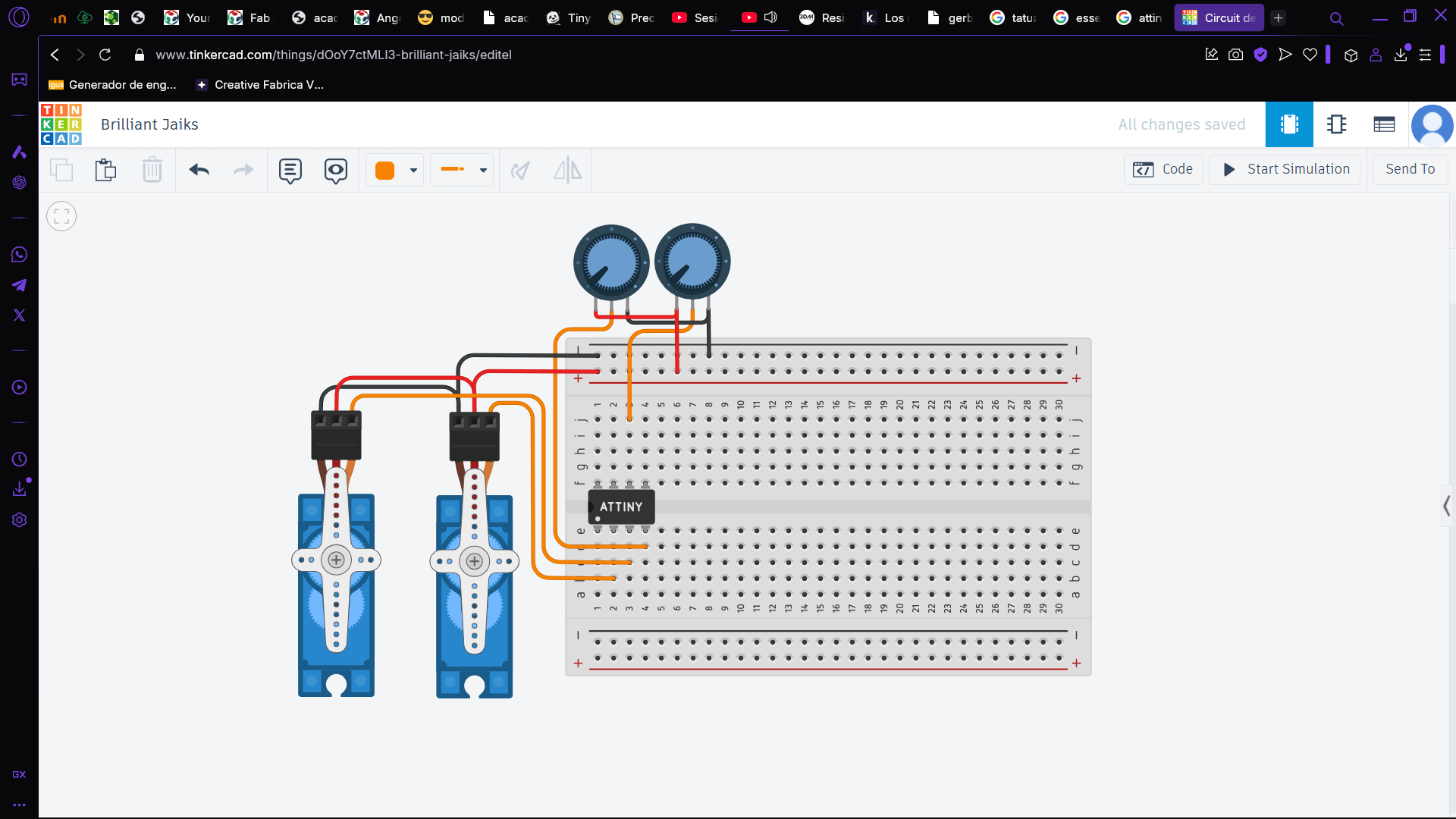
We consider that each servo typically operates at 5V and draws around 1.2A at no load, but can reach up to 1.5A under stress. Assuming an average current draw of 1.35A per servo, the power consumed by each servo is given by the formula P = V * I, where P is power in watts, V is voltage, and I is current. For one servo, P = 5V * 1.35A = 6.75W. For two servos, the total power consumption is 6.75W * 2 = 13.5W.
For the power supply, I set 7V for the servos and noticed a peak current consumption of 0.94A, as can be barely seen in the video.
Below are a video and images showing the PWM signal at its minimum and maximum levels.
Oh, you don't know what a PWM signal is?
PWM, or pulse-width modulation, is a technique used to control analog devices using digital signals. Instead of varying voltage levels continuously, PWM rapidly switches between HIGH and LOW states at a fixed frequency. The duty cycle, which represents the percentage of time the signal is HIGH within a given period, determines the effective power delivered to the device. In servo motors, PWM controls the position by sending pulses of different widths, where a pulse width of around 500µs positions the servo at 0°, 1500µs at 90°, and 2400µs at 180°. This method allows for precise control of motor angles while maintaining efficiency. PWM is widely used in motor control, LED dimming, and power regulation in embedded systems.
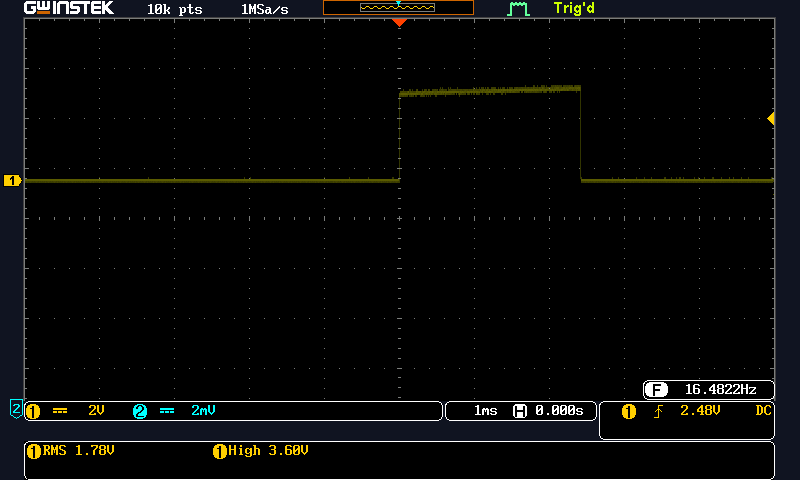
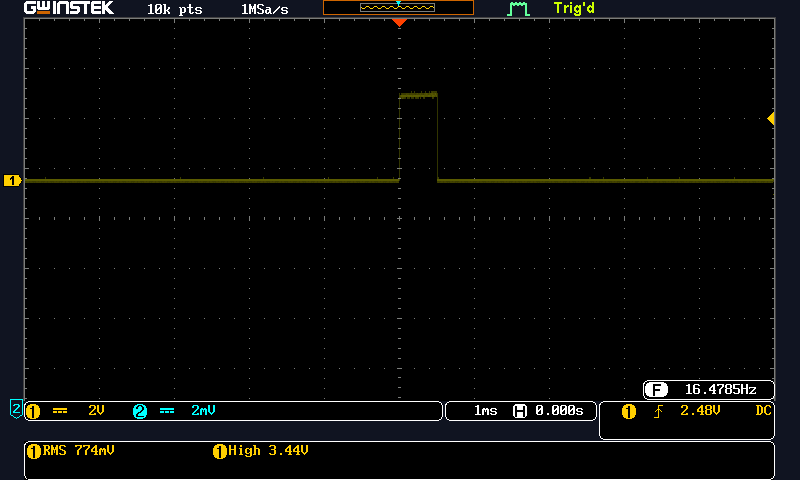
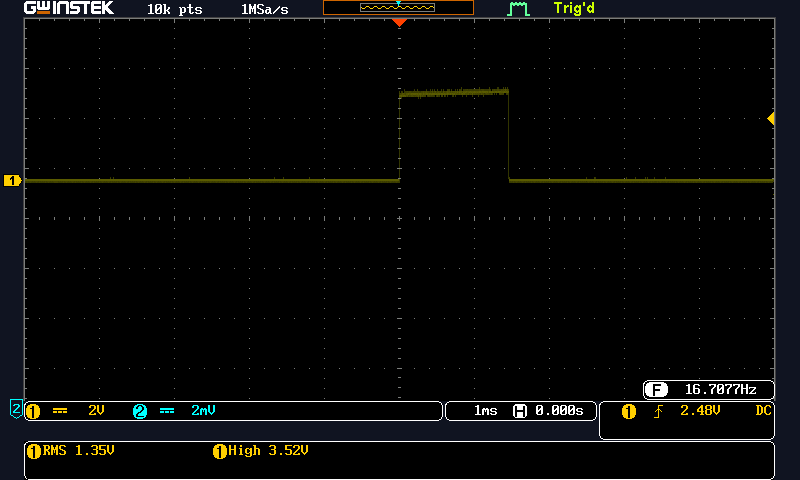
Here's the code I used:
// Joystick and Servo Control #include "Arduino.h" // Define pins const int servoPin1 = 0; // Servo 1 const int servoPin2 = 1; // Servo 2 const int potPin1 = 2; // Potentiometer 1 const int potPin2 = 5; // Potentiometer 2 void setup() { // Set servo pins as outputs pinMode(servoPin1, OUTPUT); pinMode(servoPin2, OUTPUT); } void loop() { // Read potentiometer values int potValue1 = analogRead(potPin1); int potValue2 = analogRead(potPin2); // Convert potentiometer values to angles (0° to 180°) int angle1 = map(potValue1, 0, 1023, 0, 180); int angle2 = map(potValue2, 0, 1023, 0, 180); // Convert angles to PWM pulse width int pulseWidth1 = map(angle1, 0, 180, 500, 2400); int pulseWidth2 = map(angle2, 0, 180, 500, 2400); // Send PWM signals to servos moveServo(servoPin1, pulseWidth1); moveServo(servoPin2, pulseWidth2); delay(20); // Small delay for stability } // Generate PWM signal manually for servos void moveServo(int pin, int pulseWidth) { digitalWrite(pin, HIGH); delayMicroseconds(pulseWidth); digitalWrite(pin, LOW); delayMicroseconds(20000 - pulseWidth); // 20ms period }
Joystick and servo pin definition
This section defines the pin assignments for both the servo motors and the potentiometers. The servos are connected to digital pins 0 and 1, while the potentiometers are connected to analog pins 2 and 5. These pin definitions allow the Arduino to interact with the hardware components by reading the potentiometer values and sending signals to the servos.
#include "Arduino.h" // Define pins const int servoPin1 = 0; // Servo 1 const int servoPin2 = 1; // Servo 2 const int potPin1 = 2; // Potentiometer 1 const int potPin2 = 5; // Potentiometer 2
setup() function
The setup() function initializes the pin modes for the servos, setting them as outputs. This means the Arduino will generate signals to control the servo motors. No input configuration is needed for the potentiometers because they are read using the analogRead() function, which does not require explicit pinMode configuration.
void setup() { pinMode(servoPin1, OUTPUT); pinMode(servoPin2, OUTPUT); }
loop() function
The loop() function continuously runs and first reads the analog values from both potentiometers. The values range from 0 to 1023, as the Arduino's ADC (analog-to-digital converter) converts voltage readings from 0V to 5V into a 10-bit resolution.
void loop() { int potValue1 = analogRead(potPin1); int potValue2 = analogRead(potPin2);
Mapping potentiometer values to angles
Since the potentiometer readings are in the range of 0 to 1023 but the servo motors require an angle input from 0° to 180°, the map() function is used to scale the values accordingly. This ensures that the potentiometer position directly corresponds to the servo rotation, allowing smooth control.
int angle1 = map(potValue1, 0, 1023, 0, 180); int angle2 = map(potValue2, 0, 1023, 0, 180);
Converting angles to PWM pulse width
Servo motors operate using PWM signals with pulse widths that typically range from 500µs (0°) to 2400µs (180°). The map() function scales the angle values to match the required pulse width. This conversion ensures that the servo receives the correct timing signal to position itself at the desired angle.
int pulseWidth1 = map(angle1, 0, 180, 500, 2400); int pulseWidth2 = map(angle2, 0, 180, 500, 2400);
sending PWM signals to servos
The moveServo() function is called to generate the PWM signals for each servo, using the calculated pulse width values. A short delay of 20 milliseconds is added to stabilize the motion and prevent rapid fluctuations in servo positioning.
moveServo(servoPin1, pulseWidth1); moveServo(servoPin2, pulseWidth2); delay(20); }
Manual PWM signal generation
Unlike using a dedicated servo library, this function manually generates a PWM signal by setting the pin HIGH for the desired pulse width duration and then setting it LOW for the remainder of the 20ms period. This timing mimics the standard PWM control used by servo motors, where pulses are sent approximately every 20 milliseconds.
void moveServo(int pin, int pulseWidth) { digitalWrite(pin, HIGH); delayMicroseconds(pulseWidth); digitalWrite(pin, LOW); delayMicroseconds(20000 - pulseWidth); // 20ms period }
Beyond that, I reinforced my understanding of interacting with output devices using microcontrollers. I learned how to control servo motors efficiently by fine-tuning PWM signals and ensuring proper power supply management. Additionally, I improved my ability to adapt and optimize code for different microcontrollers, considering their pin limitations and resource constraints.
Here you have the link to our group page
Here you can find the files of each process: