OUTPUT DEVICES
LCD I2C DISPLAY
For this week's assignment i decided to use an lcd display and plug it to the board I have already design. The main idea was for it to display the readings of the soil moisture sensor that was used in Week 9
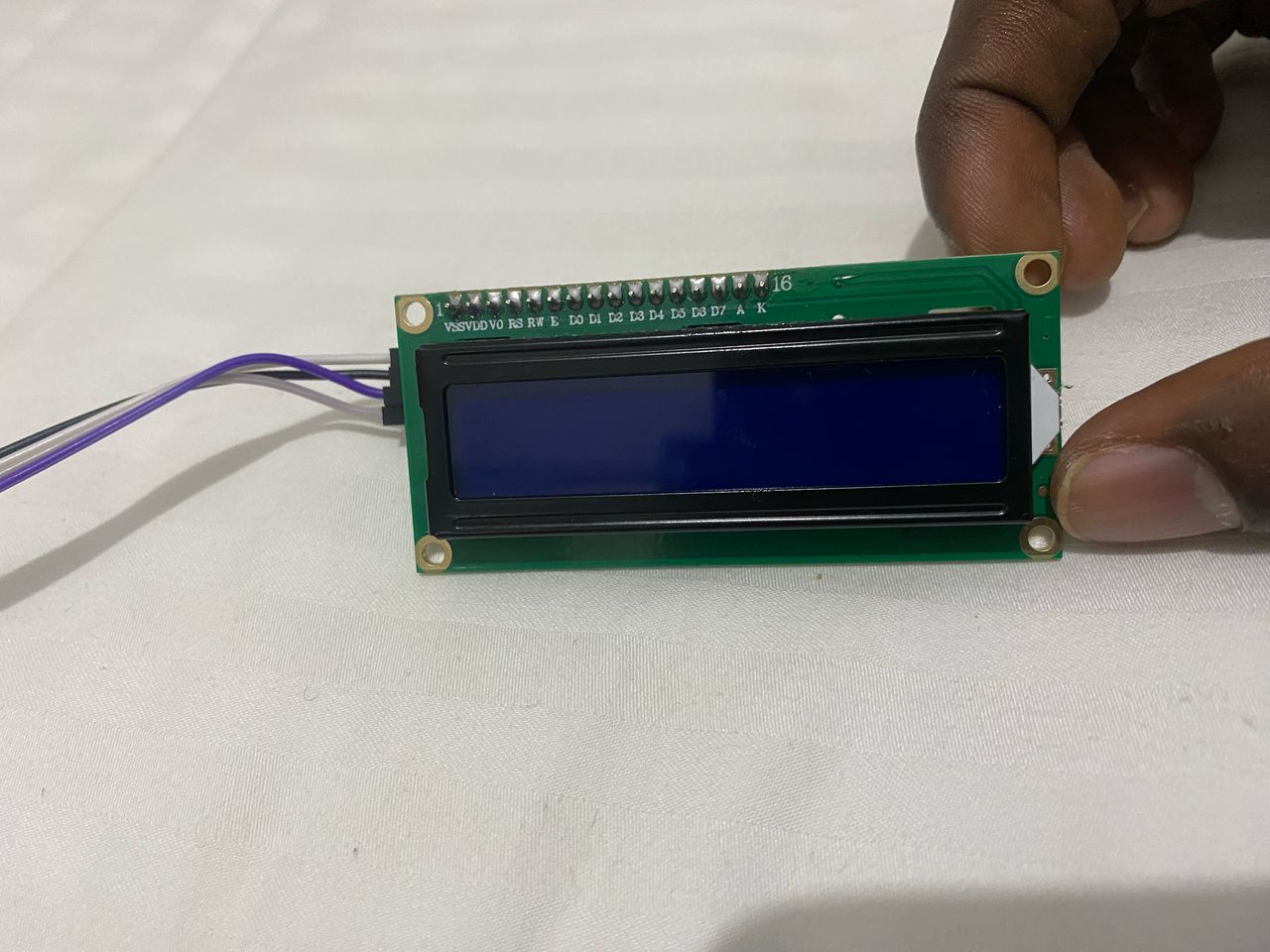
COMPONENTS USED
- PCB (DESIGNED ON WEEK 6)
- JUMPER WIRES
- SOIL MOISTURE SENSOR
- LCD I2C DISPLAY
I started off by connecting the lcd dispayed into the board that was already connected with the moisture sensor from the previous assignment. The LCD was connected to the board's I2C pins which are SDA and SCL.
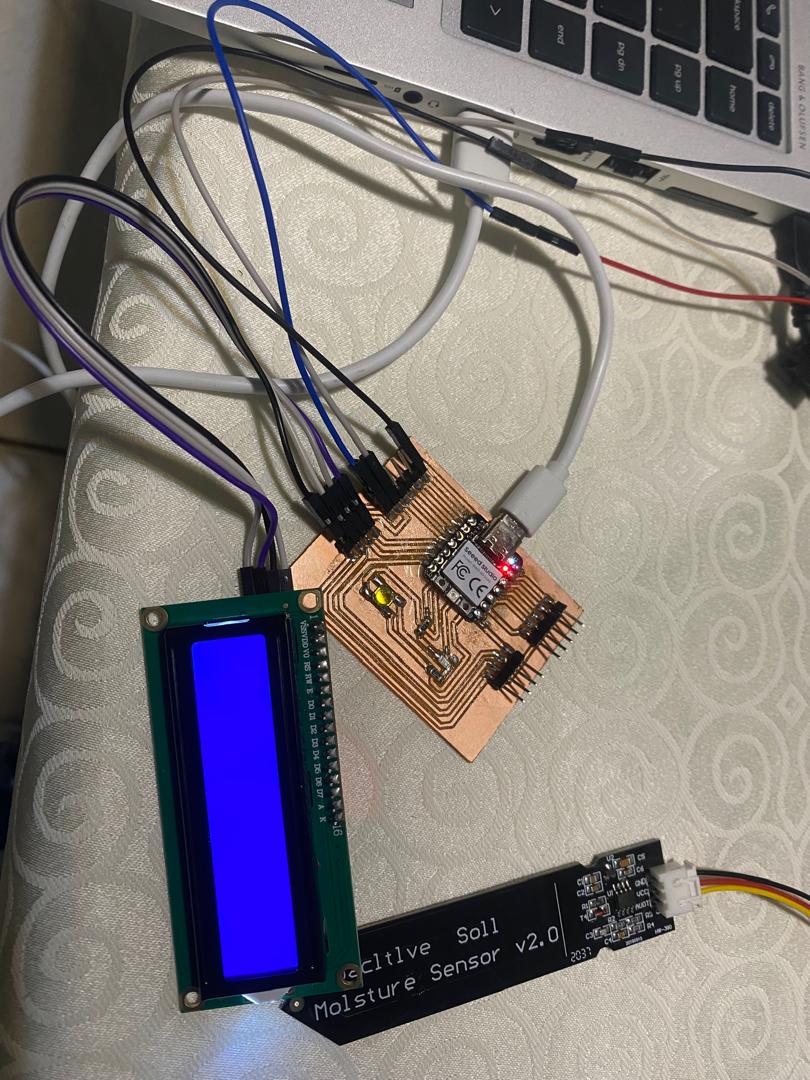
INSTALLING LC LIBRARY
After the connection of the hardware, I then launched Arduino IDE and then installed the LiquidCrystal_I2C library which is used to control the LCD I2C Display.
I then updated the moisture sensor code by adding the LCD code to it, so that the moisture sensor reading can be displayed in the LCD. The codes is as follows:
#include
// Initialize the LCD: address 0x27, 16 columns, 2 rows
LiquidCrystal_I2C lcd(0x27, 16, 2);
// Define the analog pin connected to the soil moisture sensor
const int sensorPin = A0;
int sensorValue;
void setup() {
Serial.begin(9600);
lcd.init(); // Initialize the LCD
lcd.backlight(); // Turn on the backlight
lcd.print("sensorValue"); // This will literally print the text "sensorValue"
delay(2000);
lcd.clear(); // Clear the LCD
pinMode(sensorPin, INPUT);
}
void loop() {
sensorValue = analogRead(sensorPin); // Read the analog value from the sensor
// Print the reading to the Serial Monitor
Serial.print("Soil Moisture Reading: ");
Serial.println(sensorValue);
// Display the reading on the LCD
lcd.print(sensorValue);
delay(1000); // Wait 1 second
lcd.clear(); // Clear the LCD for the next update
}
FILES