Interface and application programming
Introduction (sourced from chat gpt )
Interface programming involves designing and implementing the user interface (UI) of
software applications. This includes everything that users interact with, such as
buttons, menus, forms, and other graphical elements.
Application programming, on the other hand, refers to the development of software
applications themselves. This involves writing code to perform specific tasks or
functions, often utilizing various programming languages and frameworks..
Group Assignment
The Kannai team as usual was divided into two and i worked with Ahmad Tijjani Ishaq The task is to compare as many tool options as possible Our group assignment page
Individual Assignment
All source files can be found Here at the side bar.
Assignment for the week is to write an application that interfaces a user with an input &/or output device that you made
To complete my task i used the following:
- Arduino IDE
- Project board 1
- Processing Software
- Thumbprint sensor
what i did
I wrote a code that interfaces my thumbprint sensor using and arduino IDE and processing software. When a finger print is matched by the sensor, a signal "1" is printed and sent serially to the processing software. Then the processing software displays a canvass with my final project Logo TouchGuard .
More on thumbprint sensor can be found in my input device documentation
I connected the thumbprint sensor to my project board 1 and then wrote
the code on both arduino IDE and Processing software.
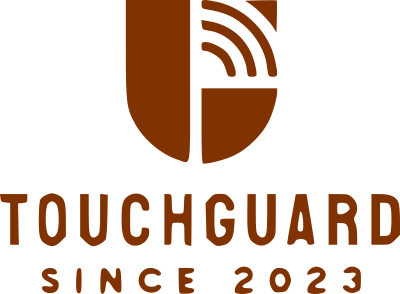
Code
I started by first loading the adafruit fingerprint sensor example for detecting a matched finger data.
#include
#if (defined(__AVR__) || defined(ESP8266)) && !defined(__AVR_ATmega2560__)
// For UNO and others without hardware serial, we must use software serial...
// pin #2 is IN from sensor (GREEN wire)
// pin #3 is OUT from arduino (WHITE wire)
// Set up the serial port to use softwareserial..
SoftwareSerial mySerial(2, 3);
#else
// On Leonardo/M0/etc, others with hardware serial, use hardware serial!
// #0 is green wire, #1 is white
#define mySerial Serial1
#endif
Adafruit_Fingerprint finger = Adafruit_Fingerprint(&mySerial);
void setup()
{
Serial.begin(9600);
while (!Serial); // For Yun/Leo/Micro/Zero/...
delay(100);
Serial.println("\n\nAdafruit finger detect test");
// set the data rate for the sensor serial port
finger.begin(57600);
delay(5);
if (finger.verifyPassword()) {
Serial.println("Found fingerprint sensor!");
} else {
Serial.println("Did not find fingerprint sensor :(");
while (1) { delay(1); }
}
Serial.println(F("Reading sensor parameters"));
finger.getParameters();
Serial.print(F("Status: 0x")); Serial.println(finger.status_reg, HEX);
Serial.print(F("Sys ID: 0x")); Serial.println(finger.system_id, HEX);
Serial.print(F("Capacity: ")); Serial.println(finger.capacity);
Serial.print(F("Security level: ")); Serial.println(finger.security_level);
Serial.print(F("Device address: ")); Serial.println(finger.device_addr, HEX);
Serial.print(F("Packet len: ")); Serial.println(finger.packet_len);
Serial.print(F("Baud rate: ")); Serial.println(finger.baud_rate);
finger.getTemplateCount();
if (finger.templateCount == 0) {
Serial.print("Sensor doesn't contain any fingerprint data. Please run the 'enroll' example.");
}
else {
Serial.println("Waiting for valid finger...");
Serial.print("Sensor contains "); Serial.print(finger.templateCount); Serial.println(" templates");
}
}
void loop() // run over and over again
{
getFingerprintID();
delay(50); //don't ned to run this at full speed.
}
uint8_t getFingerprintID() {
uint8_t p = finger.getImage();
switch (p) {
case FINGERPRINT_OK:
Serial.println("Image taken");
break;
case FINGERPRINT_NOFINGER:
Serial.println("No finger detected");
return p;
case FINGERPRINT_PACKETRECIEVEERR:
Serial.println("Communication error");
return p;
case FINGERPRINT_IMAGEFAIL:
Serial.println("Imaging error");
return p;
default:
Serial.println("Unknown error");
return p;
}
// OK success!
p = finger.image2Tz();
switch (p) {
case FINGERPRINT_OK:
Serial.println("Image converted");
break;
case FINGERPRINT_IMAGEMESS:
Serial.println("Image too messy");
return p;
case FINGERPRINT_PACKETRECIEVEERR:
Serial.println("Communication error");
return p;
case FINGERPRINT_FEATUREFAIL:
Serial.println("Could not find fingerprint features");
return p;
case FINGERPRINT_INVALIDIMAGE:
Serial.println("Could not find fingerprint features");
return p;
default:
Serial.println("Unknown error");
return p;
}
// OK converted!
p = finger.fingerSearch();
if (p == FINGERPRINT_OK) {
Serial.println("Found a print match!");
} else if (p == FINGERPRINT_PACKETRECIEVEERR) {
Serial.println("Communication error");
return p;
} else if (p == FINGERPRINT_NOTFOUND) {
Serial.println("Did not find a match");
return p;
} else {
Serial.println("Unknown error");
return p;
}
// found a match!
Serial.print("Found ID #"); Serial.print(finger.fingerID);
Serial.print(" with confidence of "); Serial.println(finger.confidence);
return finger.fingerID;
}
// returns -1 if failed, otherwise returns ID #
int getFingerprintIDez() {
uint8_t p = finger.getImage();
if (p != FINGERPRINT_OK) return -1;
p = finger.image2Tz();
if (p != FINGERPRINT_OK) return -1;
p = finger.fingerFastSearch();
if (p != FINGERPRINT_OK) return -1;
// found a match!
Serial.print("Found ID #"); Serial.print(finger.fingerID);
Serial.print(" with confidence of "); Serial.println(finger.confidence);
return finger.fingerID;
}
The code is complex but the explanation can be found Here block by block
Then i modified the function uint8_t getFingerprintID() and replaced "Serial.println("Found a print match!");" with "Serial.println(1);" .
uint8_t getFingerprintID() {
uint8_t p = finger.getImage();
switch (p) {
case FINGERPRINT_OK:
break;
case FINGERPRINT_NOFINGER:
delay(500);
return p;
case FINGERPRINT_PACKETRECIEVEERR:
return p;
case FINGERPRINT_IMAGEFAIL:
default:
return p;
}
p = finger.image2Tz();
switch (p) {
case FINGERPRINT_OK:
break;
case FINGERPRINT_IMAGEMESS:
case FINGERPRINT_PACKETRECIEVEERR:
case FINGERPRINT_FEATUREFAIL:
case FINGERPRINT_INVALIDIMAGE:
default:
return p;
}
p = finger.fingerSearch();
if (p == FINGERPRINT_OK) {
// Found a print match!
Serial.println(1);
} else if (p == FINGERPRINT_PACKETRECIEVEERR || p == FINGERPRINT_NOTFOUND) {
// Communication error or no match found
return p;
} else {
return p;
}
return finger.fingerID;
}
I did this because it sends the value "1" serially and it is detected by my running processing software. once obtained the processing software displays a canvass with Touchguard Logo, my processing code is shown below.
import processing.serial.*;
Serial myPort; // Create object from Serial class
PImage displayImage; // Image to display when "1" is received
int displayDuration = 5000; // Display duration in milliseconds
int lastDisplayTime = 0; // Variable to store the last time the image was displayed
void setup() {
size(400, 300);
// Change the portName to match your Arduino's port
String portName = "COM6"; // Change this to your Arduino's port
myPort = new Serial(this, portName, 9600);
// Load the image to be displayed when "1" is received
displayImage = loadImage("path1.png"); // Replace "image_to_display.png" with your image file
}
void draw() {
// Check if there is data available from Arduino
while (myPort.available() > 0) {
String data = myPort.readStringUntil('\n'); // Read until a newline character
if (data != null) {
// Convert the received string to an integer
int receivedNumber = int(data.trim());
// Check if the received number is 1
if (receivedNumber == 1) {
// Display the image on the window
background(255); // Clear the background
if (displayImage != null) {
image(displayImage, 0, 0, width, height);
lastDisplayTime = millis(); // Record the time when the image is displayed
} else {
// Display an error message if the image couldn't be loaded
textSize(16);
fill(255, 0, 0); // Set text color to red
text("Error loading image", 20, height / 2);
}
}
}
}
// Check if it's time to reset the display
if (millis() - lastDisplayTime > displayDuration) {
background(255); // Clear the background to make it blank
}
}
I was able to write the code with the help of my local instructor Yuichi Tamiya and chat gpt. The video is shown below of how the code works
With this i have come to the end of my weekly activity
All source files can be found Here at the side bar.