Interface & Application Programming
Individual assignment
To start with this week, I made some research to get general understanding of how interface and applications looks like, and as a result, the interface programming it is a process of designing user interfaces for software applications. A user interface is the point of interaction between a user and a computer program or device, and interface programming is concerned with making this interaction as intuitive and user-friendly as possible. In the other hand the application programming refers to the development of software applications themselves, including the underlying code, algorithms, and logic that enable the application to perform its intended functions.
Interface and application programming are closely related, as the design of an application's user interface is an important aspect of application programming. By creating an effective and intuitive user interface, application programmers can ensure that users are able to interact with their software in a way that is easy to understand and navigate.
I am planning to make an interface that allows me to control a LED connected on the board I have already made it on communication week.
The starting point was a bit hard sense it is the first time I want to make something like this, thanks to our instructor, Emma, she advised to start with software called processing, which is a free, open-source programming language and development environment used for creating interactive art, animation, and visualizations. Processing is based on the Java programming language and provides a simplified syntax and set of libraries for creating graphical and interactive applications. It includes built-in functions for drawing shapes, creating animations, and handling user inputs.
I downloaded the processing and run it directly, even the interface was looks like the arduino IDE and familiar, but still it is the first time I use it, so a lot of youtube tutorials explains how to use it and offers a lot explanation, this tutorial list is one of them.
Knowing the command or the function and how to use them properly is the half of the way, so I started trying some basic codes following the processing tutorial page.
I have to upload 2 codes, one for the ESP32-C3 and the other is for processing, Processing will communicate with the ESP32-C3 through the serial port, it is either allowing it to receive input from sensors or other hardware components connected to the board. It can then use this input to create dynamic visual displays or to control other software components. or sending data from the processing to ESP32-C3 through the serial port to make the board execute some commands.
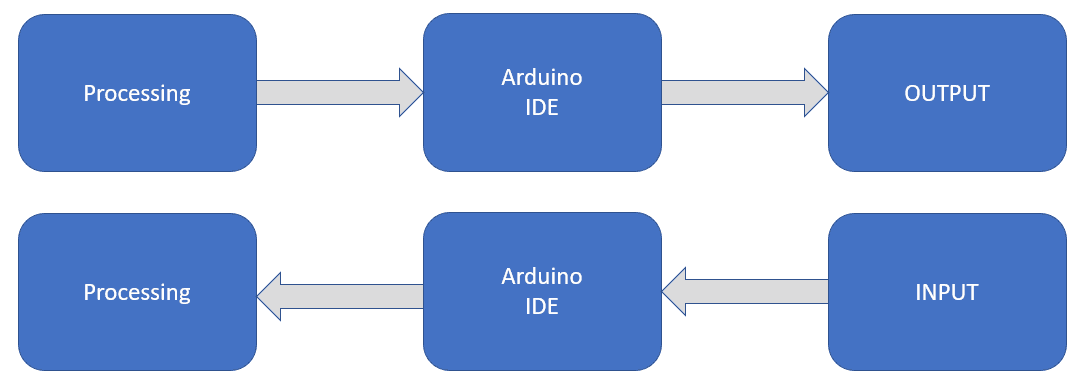
Processing:
I have found this link which helped me to start coding for both IDE and the Processing, For the processing the code is:
import processing.serial.*;
Serial sheqoo;
void setup() {
size(800, 500);
String[] portList = Serial.list();
if (portList.length > 0) { // check if the portList array is not empty
sheqoo = new Serial(this, portList[0], 9600);
}
}
void draw() {
background(255);
fill(0, 0, 255);
ellipse(width/4, height/2, width/3, height/3);
fill(0, 255, 0);
ellipse(3*width/4, height/2, width/3, height/3);
fill(0, 0, 0);
ellipse(width/2, height/2, width/3, height/3);
textAlign(CENTER, CENTER);
textSize(16);
fill(255);
text("Turn on blue color", width/4, height/2);
text("Turn on green color", 3*width/4, height/2);
text("Turn off all colors", width/2, height/2);
}
void mousePressed() {
float d1 = dist(mouseX, mouseY, width/4, height/2); // distance between mouse and center of 1st ellipse
float d2 = dist(mouseX, mouseY, 3*width/4, height/2); // distance between mouse and center of 2nd ellipse
float d3 = dist(mouseX, mouseY, width/2, height/2); // distance between mouse and center of 3rd ellipse
if (d1 < width/6 && sheqoo != null) {
sheqoo.write("b");
}
else if (d2 < width/6 && sheqoo != null) {
sheqoo.write("g");
}
else if (d3 < width/6 && sheqoo != null) {
sheqoo.write("OFF");
}
}
I used some new commands and a little bit different from the IDE:
1- Import processing.serial.*: In this command line, I am importing the Processing serial library, which allows communication with the ESP32-C3 through the serial communication.
2-Serial sheqoo : here I declare a serial object "sheqoo" that will be used to communicate with the ESP32-C3.
3- Size(800, 500): sets the size of the canvas to 500 x 500 pixels.
4- String[] portList = Serial.list(): here this command creates an array called "portList" containing the available serial ports on the computer.
5- If statements checks if there is at least one serial port available. If there is, it creates a new Serial object named "sheqoo" with the first port in the list, and a baud rate of 9600.
6- background(255): sets the background color of the canvas to white.
7- fill(0, 0, 255): sets the fill color(it depends on the combination number of the colors).
7- ellipse(A,B,C,D): to make circles, A,B values in pixel to set the location of the circle in x,y direction respectively. C,D to set width and height of the circle.
8- textAlign(CENTER, CENTER): to make the text in the center of the circles.
9- textSize(16) : sets the size of the text.
10- void mousePressed(): it is a built-in function that is called whenever the mouse button is pressed.checks the distance between the mouse click and the centers of the three circles that are drawn on the screen. If the mouse click is inside the blue circle, the Processing code sends the character 'b' to the Sheqoo board using the serial connection to turn on the blue LED connected to pin 8. If the mouse click is inside the green circle, the Processing code sends the character 'g' to the Sheqoo board to turn on the green LED connected to pin 9. Finally, if the mouse click is inside the black circle, the Processing code sends the character 'OFF' to the Sheqoo board to turn off both LEDs.
11- d1,d2,d3: functions to calculate the distance between the mouse and the center of each circle.
12- last three if statements: This line of code checks if the distance between the mouse and the center of the first ellipse is less than one-sixth of the width of the canvas, and if the sheqoo object is not null (meaning that the serial port has been successfully opened). If both of these conditions are true, then it sends the character "b or g of OFF" through the sheqoo serial connection.
The result by running the code on processing:
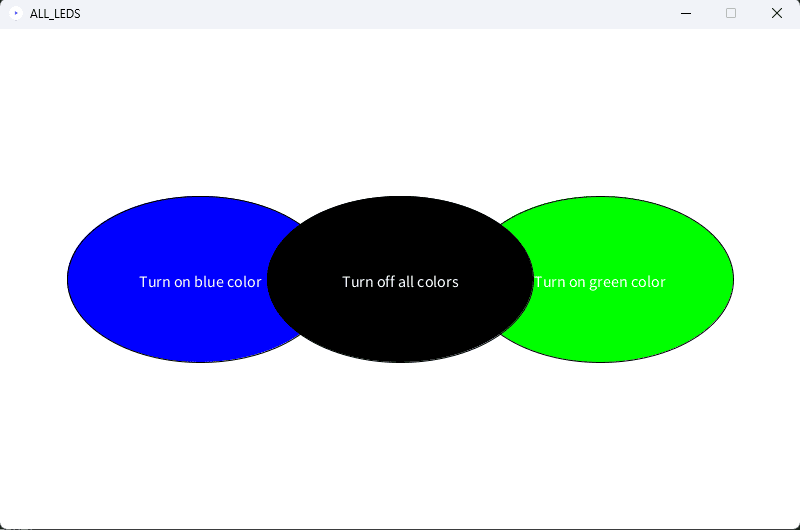
Arduino IDE:
The arduino IDE code, first I initialize the two led pins then in void setup I set them as an outputs pins, then in void loop I used Serial.available() which is a function in Arduino and Processing that checks if there is any incoming data available on the serial port. The function returns an integer value that represents the number of bytes (characters) available to be read. If there is no data available, the function will return 0. If there is data available, it is read as a string using the Serial.readString() function. Depending on the string that is received, the code turns on or off the green LED, blue LED, or both LEDs.
int greenPin = 9;
int bluePin = 8;
void setup() {
pinMode(greenPin, OUTPUT);
pinMode(bluePin, OUTPUT);
Serial.begin(9600);
}
void loop() {
if (Serial.available() > 0) {
String command = Serial.readString();
if (command == "g") {
digitalWrite(greenPin, LOW);
digitalWrite(bluePin, HIGH);
}
else if (command == "b") {
digitalWrite(greenPin, HIGH);
digitalWrite(bluePin, LOW);
}
else if (command == "OFF") {
digitalWrite(greenPin, HIGH);
digitalWrite(bluePin, HIGH);
}
}
}
I uploaded the code on the ESP32-C3, and I ran the processing code, and yeah it worked!
It is worth mentioning that I did not utilize the ControlP5 library. The ControlP5 is a popular library in Processing that provides a set of graphical user interface (GUI) components and controls, such as buttons, sliders, text fields, checkboxes, and more. It allows developers to easily create interactive and customizable interfaces for their Processing applications.
Instead of using the ControlP5, I made the code creates and draws circles on the canvas and handles mouse input to control the LED that connected to my MCU (the ESP32-C3 board). This approach demonstrates the basic principles of creating a custom interface without relying on external libraries.
Group assignment
In our group assignment, we explored different tools and platforms used for creating interactive applications. Here are the tools we discussed:
1- Processing: Processing is a powerful software sketchbook and programming language that simplifies the creation of interactive media, such as visual arts, animations, and multimedia applications. It provides a user-friendly interface, an integrated development environment (IDE), and a set of libraries that aid in coding and creative expression. With Processing, artists, designers, and programmers can bring their ideas to life through visual and interactive projects.
Example: Creating a simple animation of a bouncing ball on a canvas using Processing or making a game.
2- MIT app inventor:MIT App Inventor is a cloud-based tool that allows users to create mobile applications for Android devices without prior programming experience. It offers a drag-and-drop interface for assembling app components and a block-based programming language for defining app behavior. This platform enables individuals to transform their app ideas into reality quickly.
Example: Developing a mobile app that provides access to Fab Academy course materials, including lecture videos, assignments, and community forums.
3- Jupyter: Jupyter: Jupyter is an open-source web application widely used in scientific computing, data science, and machine learning. It enables users to create and share documents (notebooks) containing live code, equations, visualizations, and narrative text. Jupyter notebooks facilitate interactive data analysis, exploration, and collaboration.
Example: Using a Jupyter notebook with Python to analyze and visualize a dataset, such as plotting a line chart of some data.(similar to excel sheet) but jupyter notebooks provide a more flexible and customizable environment compared to Excel.
4- Bubble: Bubble: Bubble is a visual programming platform that empowers users to build web and mobile applications without coding. Its drag-and-drop interface simplifies the creation of user interfaces and allows for customization. Bubble offers hosting and scalable infrastructure for launching and running applications in the cloud.
Example: Building a web-based platform with Bubble for Fab Academy students and instructors to collaborate, share project documentation, and provide feedback on each other's work (That would add some fun).
In conclusion, we explored various tools and platforms for creating interactive applications. We discussed Processing, which is a versatile software sketchbook for visual arts and multimedia projects. MIT App Inventor was another tool we examined, enabling the creation of mobile apps without coding(just adding blocks). Jupyter, on the other hand, offered a flexible environment for data analysis and visualization. Lastly, Bubble stood out as a visual programming platform for building web and mobile applications. Overall, this assignment provided us with insights into diverse tools that facilitate interactive application development, catering to different skill sets and project requirements.