14. Interface and application programming¶
Group assignment:¶
- Compare as many tool options as possible
To see our group assignment click here
Individual assignment:¶
- Write an application that interfaces a user with input and/or output device(s) on a board that you made.
For this week’s assignment, we chose to use node red which is a nodejs based solution to develop an interface to communicate with two MCUs namely the ESP01 and the ESP32 presented in Week 13 (Networking and communications).
Indeed, the assignment that will be done is related to our final project. Regarding the operation we will have a RFID reader and an LCD screen connected to the ESP32 and a relay with a led connected to the esp 01. Our interface will allow to turn on or off the relay and to display the code recovered by the RFID reader.
Our work this assignment will be divided into 4 parts: Installation of node-red, wiring and programming the MCU, programming the interface and testing.
Installation of node-red¶
Node-RED is a programming tool for wiring together hardware devices, APIs and online services in new and interesting ways. It provides a browser-based editor that makes it easy to wire together flows using the wide range of nodes in the palette that can be deployed to its runtime in a single-click. for more details click here
For the installation of node red we will use the raspberry pi used in week 13.
To connect to the raspberry pi with ssh we used windows powershell.
- connection to the Raspberry pi
- now that we are connected to the raspberry pi we will update the pi before any other command.
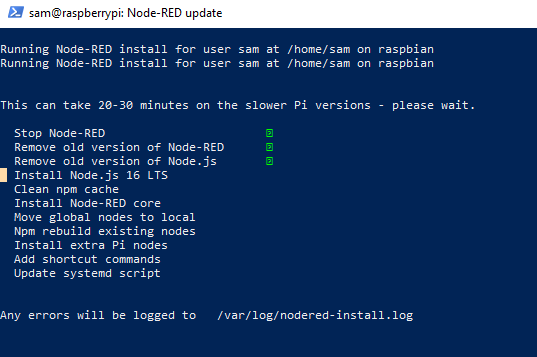
once the installation is finished, we entered the address of our Raspberry pi followed by “:1880” to access the node-red home page.
In order to program our interface with node-red we need to install an extension as shown below.
Once the installation is complete you can enter the IP address of the Raspberry followed by “:1880/ui” to access the dashboard.
Wiring and programming the MCU¶
ESP32¶
Here is our wiring diagram
For programming we used the Arduino IDE for more details on programming refer to week 9. and to week 13.
ESP32_program.ino | |
---|---|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 |
|
ESP01¶
For programming we used the Arduino IDE for more details on programming refer to week 13.
ESP01_program.ino | |
---|---|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 |
|
Programming the interface of Node-Red¶
For the programming of the node red interface we will use two switches, one to control the relay and the other to control the led on the ESP01 card. For the visualization of the RFID code retrieved by the ESP32 we will use an input field to display it.
- We chose the modules we were interested in from the palette on the left of the screen and made a click and drag as shown below.
- In order to set the different nodes, double click on them and the settings window will appear as shown below.
- once the nodes are set up we click on deploy and we should have the following result
- Now we can visualize our interface by entering the IP address of the raspberry pi followed by “:1880/ui”. Here is our interface.
I know more than one person is wondering but how to reproduce the interface don’t worry you just need to download my source file and go to the node red menu and click on import as shown below.
Testing¶
Below you will find our hero video. I hope our work will inspire you to do extraordinary things.