14. Networking and communications¶
Individual assignment:¶
Design, build, and connect wired or wireless node(s) with network or bus addresses¶
All Network¶
MQTTLens application web with ESP8266 NodeMcu Publisher and ESP8266 NodeMcu Subscriber.
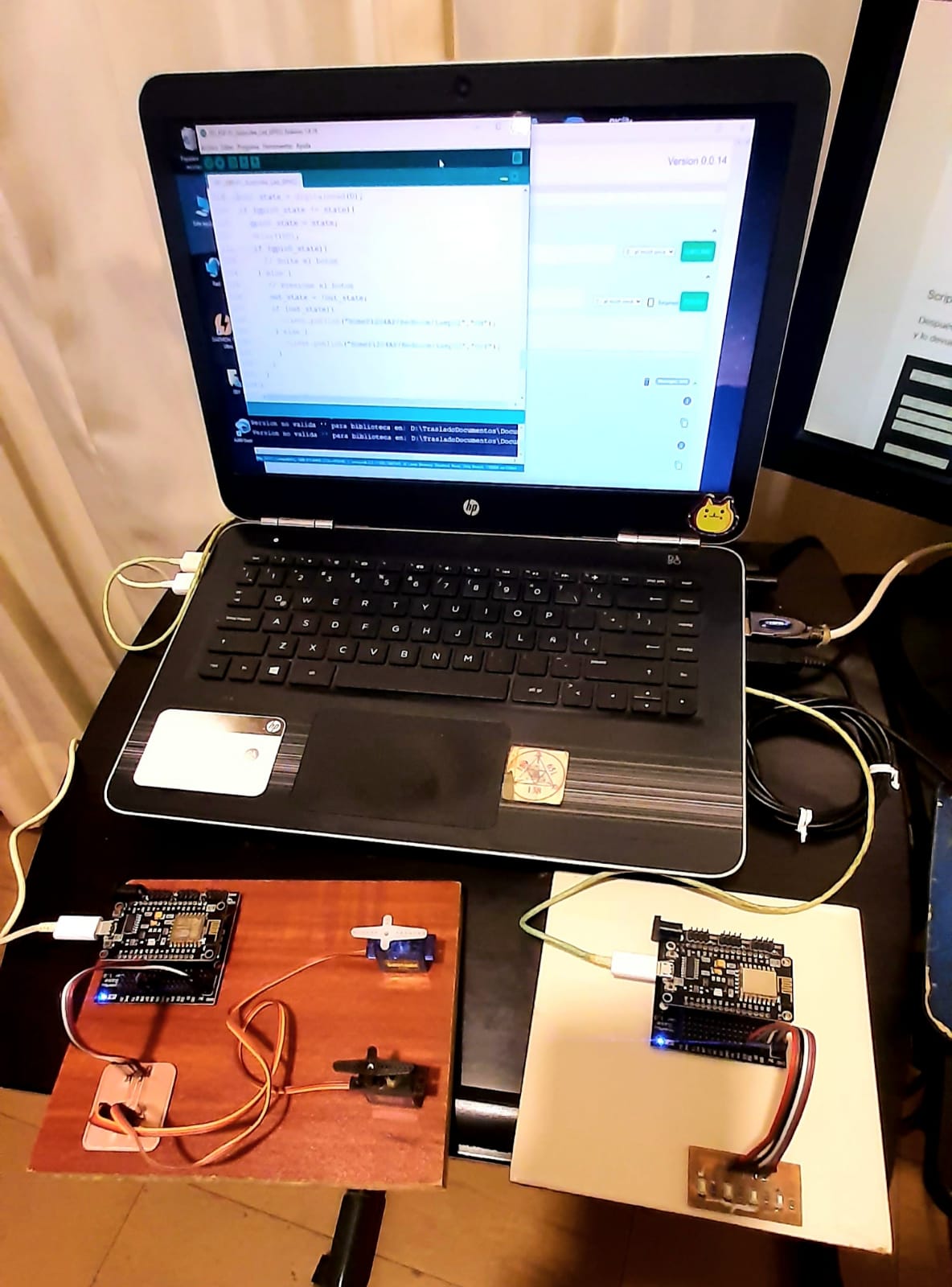
MQTTLens application¶
MQTT (Message Queue Telemetry Transport) is an OASIS standard for IoT connectivity. It is a publish/subscribe, extremely simple and lightweight messaging protocol, designed for constrained devices and low-bandwidth, high-latency or unreliable networks.
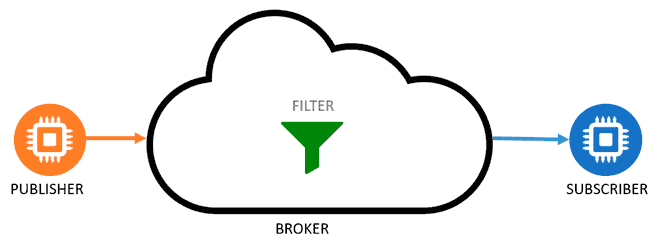
MQTTLens is a Google Chrome application, which connects to a MQTT broker and is able to subscribe and publish to MQTT topics.
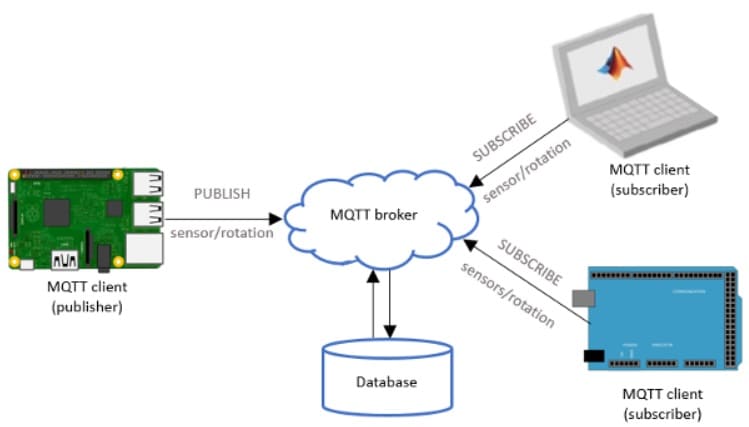
MQTTLens Application
ESP8266 NodeMcu Publisher¶
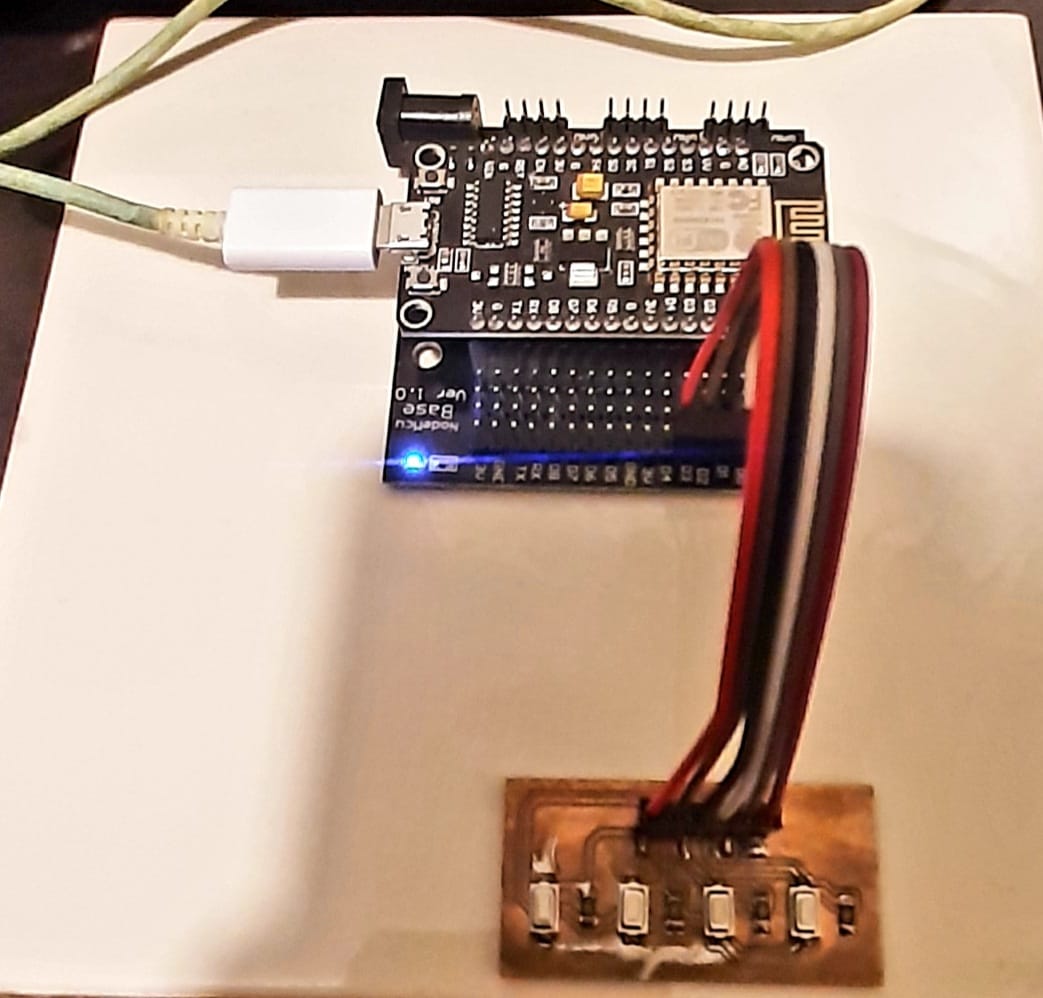
ESP8266 NodeMcu Publisher program code
#include <ESP8266WiFi.h>
#include <PubSubClient.h>
String ssid = "XXXX"; //Wifi name
String password = "iiiiiii"; //Wifi password
WiFiClient espClient;
PubSubClient client(espClient);
String mqtt_server = "test.mosquitto.org";
char mqtt_server_array[50];
char message_array[50];
char topic_array[50];
char inChar;
String inString = "",inTopic = "",inMessage = "";
bool actionMqtt, actionModule, flagMqtt = false;
// Process
bool gpio0_state1;
bool out_state1;
bool gpio0_state2;
bool out_state2;
void setup_wifi() {
delay(10);
// We start by connecting to a WiFi network
Serial.println();
Serial.print("Connecting to ");
Serial.println(ssid);
WiFi.mode(WIFI_STA);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println("");
Serial.println("WiFi connected");
Serial.println("IP address: ");
Serial.println(WiFi.localIP());
}
void callback(char* topic, byte* payload, unsigned int length) {
Serial.print(topic);
Serial.print(",");
for (int i = 0; i < length; i++) {
Serial.print((char)payload[i]);
}
Serial.println();
// Analisis de topico
String topico = String(topic);
if (topico=="HomeP1204AP/BedRoom/Lamp02"){
Serial.println("Si es igual topico");
String mensaje = String((char *)payload);
Serial.println(mensaje);
if (mensaje[1]=='N'){
Serial.println("Mensaje ON");
digitalWrite(4,HIGH);
out_state1=HIGH;
}
else if (mensaje[1]=='F'){
Serial.println("Mensaje OFF");
digitalWrite(4,LOW);
out_state1=LOW;
}
Serial.println("Salio");
}
}
void clientConnect() {
// Loop until we're reconnecte
while (!client.connected()) {
Serial.print("Attempting MQTT connection...");
// Create a random client ID
String clientId = "ESP8266Client-";
clientId += String(random(0xffff), HEX);
// Attempt to connect
if (client.connect(clientId.c_str())) {
Serial.println("connected");
// Once connected, publish an announcement...
client.publish("HomeP1204AP", "hello world");
client.subscribe("HomeP1204AP/#");
} else {
Serial.print("failed, rc=");
Serial.print(client.state());
Serial.println(" try again in 5 seconds");
// Wait 5 seconds before retrying
delay(5000);
}
}
}
void setup() {
Serial.begin(115200);
setup_wifi();
mqtt_server.toCharArray( mqtt_server_array, 100 );
client.setServer(mqtt_server_array, 1883);
client.setCallback(callback);
// Process
pinMode(0,INPUT);
gpio0_state1 = digitalRead(0);
pinMode(4,OUTPUT);
digitalWrite(4,LOW);
out_state1 = LOW;
}
void loop() {
if (!client.connected()) {
clientConnect();
} else {
client.loop();
if (flagMqtt) {
flagMqtt = false;
inMessage.toCharArray( message_array, 100 );
inTopic.toCharArray( topic_array, 100 );
client.publish(topic_array, message_array);
}
}
bool statep1 = !digitalRead(16);
if (gpio0_state1 != statep1){
gpio0_state1 = statep1;
delay(100);
if (gpio0_state1){
// Solte el boton
} else {
// Presione el boton
out_state1 = !out_state1;
if (out_state1){
client.publish("HomeP1204AP/Servo01","ON");
} else {
client.publish("HomeP1204AP/Servo01","OFF");
}
}
}
bool statep2 = !digitalRead(5);
if (gpio0_state2 != statep2){
gpio0_state2 = statep2;
delay(100);
if (gpio0_state2){
// Solte el boton
} else {
// Presione el boton
out_state2 = !out_state2;
if (out_state2){
client.publish("HomeP1204AP/Servo02","ON");
} else {
client.publish("HomeP1204AP/Servo02","OFF");
}
}
}
}
void serialEvent() {
while (Serial.available()) {
inChar = (char)Serial.read();
switch (inChar){
case '\r':
inMessage = inString;
break;
case '\n':
inString = "";
flagMqtt = true;
break;
case ',':
inTopic = inString;
inString = "";
break;
default:
inString += inChar;
break;
}
}
}
ESP8266 NodeMcu Subscriber¶
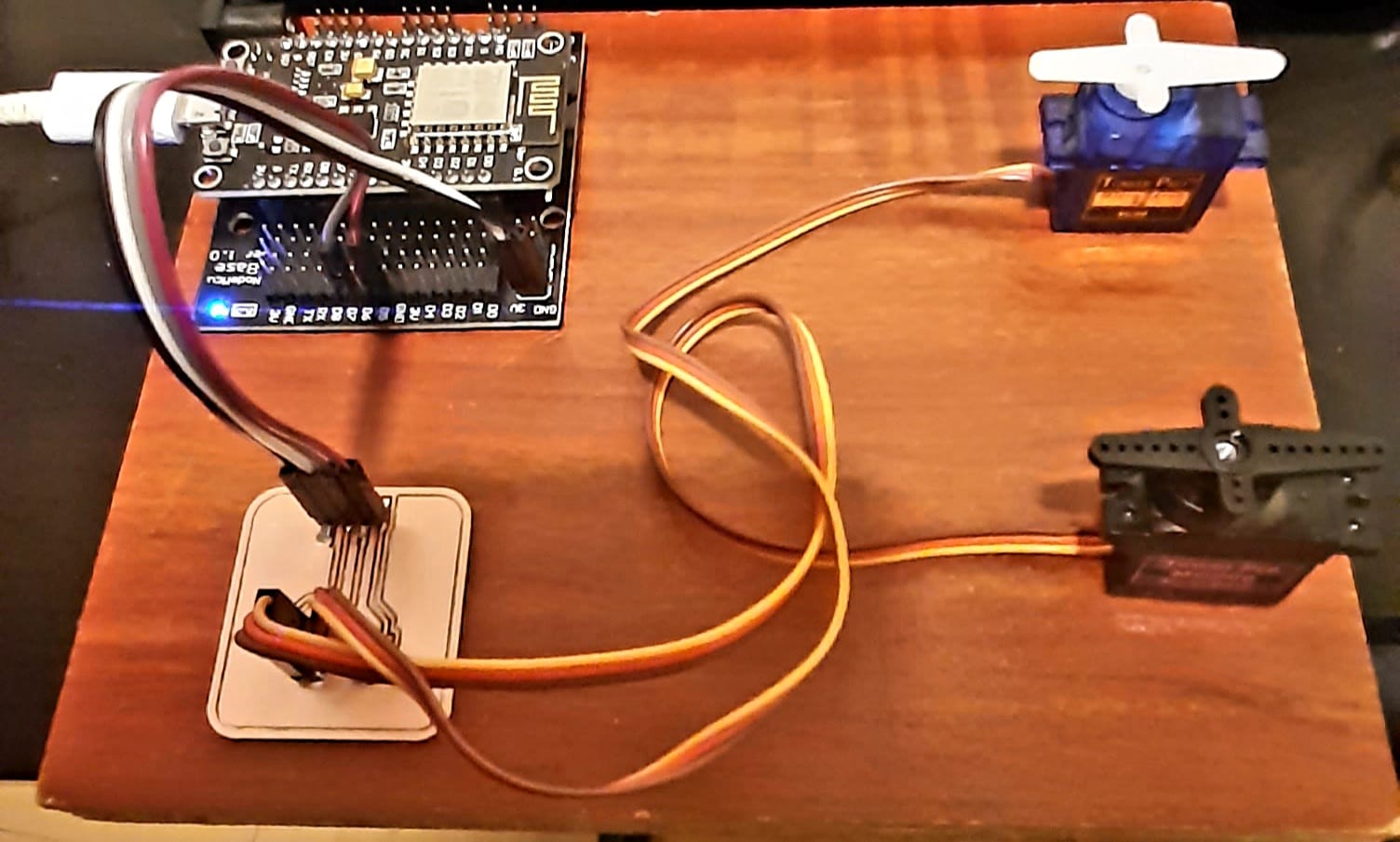
ESP8266 NodeMcu Subscriber program code
#include <ESP8266WiFi.h>
#include <PubSubClient.h>
#include <Servo.h>
String ssid = "XXXX";
String password = "iiiiiiii";
WiFiClient espClient;
PubSubClient client(espClient);
String mqtt_server = "test.mosquitto.org";
char mqtt_server_array[50];
char message_array[50];
char topic_array[50];
char inChar;
String inString = "",inTopic = "",inMessage = "";
bool actionMqtt, actionModule, flagMqtt = false;
// Process
bool gpio0_state;
bool out_state;
int servo01_pin = 14;
Servo myservo01;
int angle01 = 0;
int servo02_pin = 13;
Servo myservo02;
int angle02 = 0;
void setup_wifi() {
delay(10);
// We start by connecting to a WiFi network
Serial.println();
Serial.print("Connecting to ");
Serial.println(ssid);
WiFi.mode(WIFI_STA);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println("");
Serial.println("WiFi connected");
Serial.println("IP address: ");
Serial.println(WiFi.localIP());
}
void callback(char* topic, byte* payload, unsigned int length) {
Serial.print(topic);
Serial.print(",");
for (int i = 0; i < length; i++) {
Serial.print((char)payload[i]);
}
Serial.println();
// Analisis de topico
String topico = String(topic);
if (topico=="HomeP1204AP/Servo01"){
Serial.println("Si es igual topico");
String mensaje = String((char *)payload);
Serial.println(mensaje);
if (mensaje[1]=='N'){
Serial.println("Mensaje ON");
// digitalWrite(4,HIGH);
// out_state=HIGH;
for(angle01 = 0; angle01 < 180; angle01 += 1) {
myservo01.write(angle01);
delay(10);
}
} else if (mensaje[1]=='F'){
Serial.println("Mensaje OFF");
// digitalWrite(4,LOW);
// out_state=LOW;
for(angle01 = 180; angle01 >= 1; angle01 -= 1) {
myservo01.write(angle01);
delay(10);
}
}
Serial.println("Salio");
}
if (topico=="HomeP1204AP/Servo02"){
Serial.println("Si es igual topico");
String mensaje = String((char *)payload);
Serial.println(mensaje);
if (mensaje[1]=='N'){
Serial.println("Mensaje ON");
for(angle02 = 0; angle02 < 180; angle02 += 1) {
myservo02.write(angle02);
delay(10);
}
} else if (mensaje[1]=='F'){
Serial.println("Mensaje OFF");
for(angle02 = 180; angle02 >= 1; angle02 -= 1) {
myservo02.write(angle02);
delay(10);
}
}
Serial.println("Salio");
}
}
void clientConnect() {
// Loop until we're reconnected
while (!client.connected()) {
Serial.print("Attempting MQTT connection...");
// Create a random client ID
String clientId = "ESP8266Client-";
clientId += String(random(0xffff), HEX);
// Attempt to connect
if (client.connect(clientId.c_str())) {
Serial.println("connected");
// Once connected, publish an announcement...
client.publish("HomeP1204AP", "hello world");
client.subscribe("HomeP1204AP/#");
} else {
Serial.print("failed, rc=");
Serial.print(client.state());
Serial.println(" try again in 5 seconds");
// Wait 5 seconds before retrying
delay(5000);
}
}
}
void setup() {
Serial.begin(115200);
setup_wifi();
mqtt_server.toCharArray( mqtt_server_array, 100 );
client.setServer(mqtt_server_array, 1883);
client.setCallback(callback);
// Process
pinMode(0,INPUT);
gpio0_state = digitalRead(0);
// pinMode(4,OUTPUT);
// digitalWrite(4,LOW);
// out_state = LOW;
myservo01.attach(servo01_pin);
myservo02.attach(servo02_pin);
myservo01.write(angle01);
myservo02.write(angle02);
}
void loop() {
if (!client.connected()) {
clientConnect();
} else {
client.loop();
if (flagMqtt) {
flagMqtt = false;
inMessage.toCharArray( message_array, 100 );
inTopic.toCharArray( topic_array, 100 );
client.publish(topic_array, message_array);
}
}
bool state = digitalRead(0);
if (gpio0_state != state){
gpio0_state = state;
delay(100);
if (gpio0_state){
// Solte el boton
} else {
// Presione el boton
out_state = !out_state;
if (out_state){
client.publish("HomeP1204AP/BedRoom/Lamp02","ON");
} else {
client.publish("HomeP1204AP/BedRoom/Lamp02","OFF");
}
}
}
}
void serialEvent() {
while (Serial.available()) {
inChar = (char)Serial.read();
switch (inChar){
case '\r':
inMessage = inString;
break;
case '\n':
inString = "";
flagMqtt = true;
break;
case ',':
inTopic = inString;
inString = "";
break;
default:
inString += inChar;
break;
}
}
}
Group assignment:¶
Send a message between two projects¶
Now, we control the conveyor belt (Mecanical Design, Machine Design) manually through a bluetooth wireless network.
This network allows a Master - Slave configuration
Marter - Slave Network Schematic
Components to implement the network
We need two HC-05 bluetooth, these devices allow master and slave configurations. We also need two microcontrollers, the first is the one developed for all our projects and the second is an Arduino UNO
Bluetooth HC-05.
We must make the respective master and slave configurations, for this we do not support the usb to TTL converter, the connection is as follows:
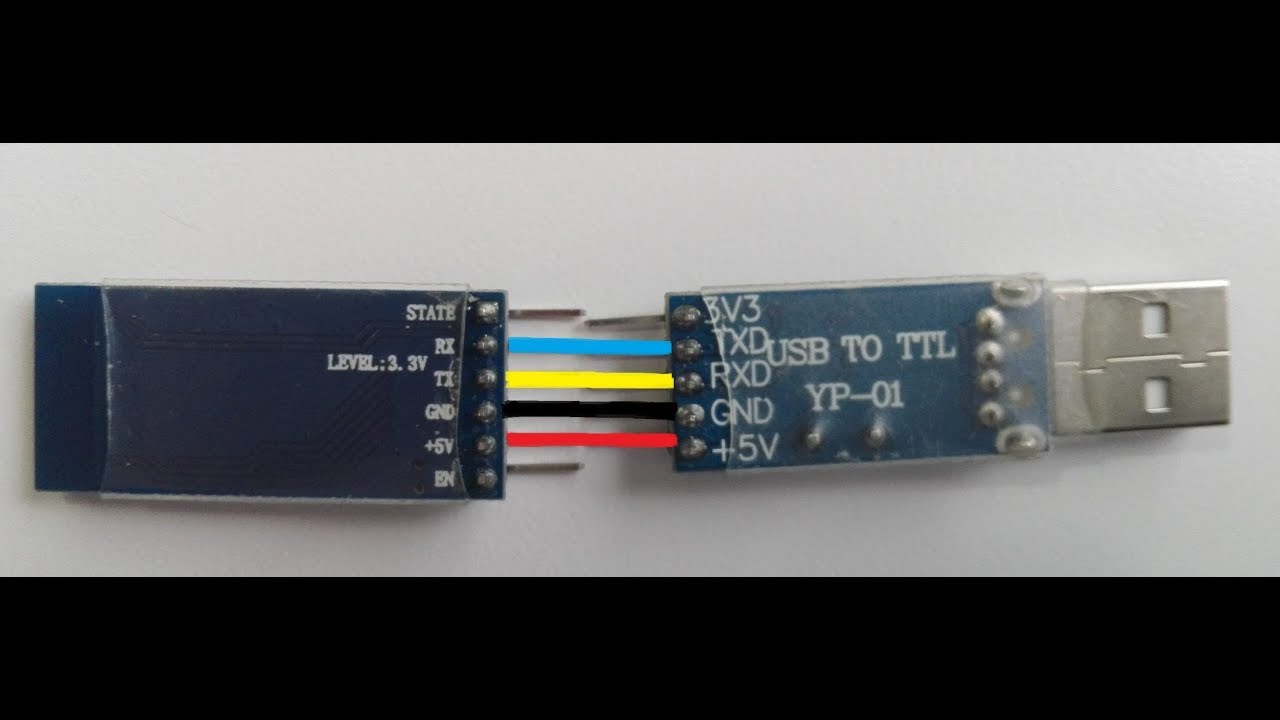
Bluetooth HC-05 and USB to TTL Adapter.
Now, using AT commands and using the Serial Monitor of the Arduino IDE as a message interface we proceed to configure the work role of each device, one as master and the other as slave, we will also configure the data transfer speed of 9600 kbps to 38400 kbps in both. Next, we show the process for the MASTER
First, To enter the configuration mode we must keep the Bluetooth HC-05 button pressed, while we connect the usb - TTL adapter.
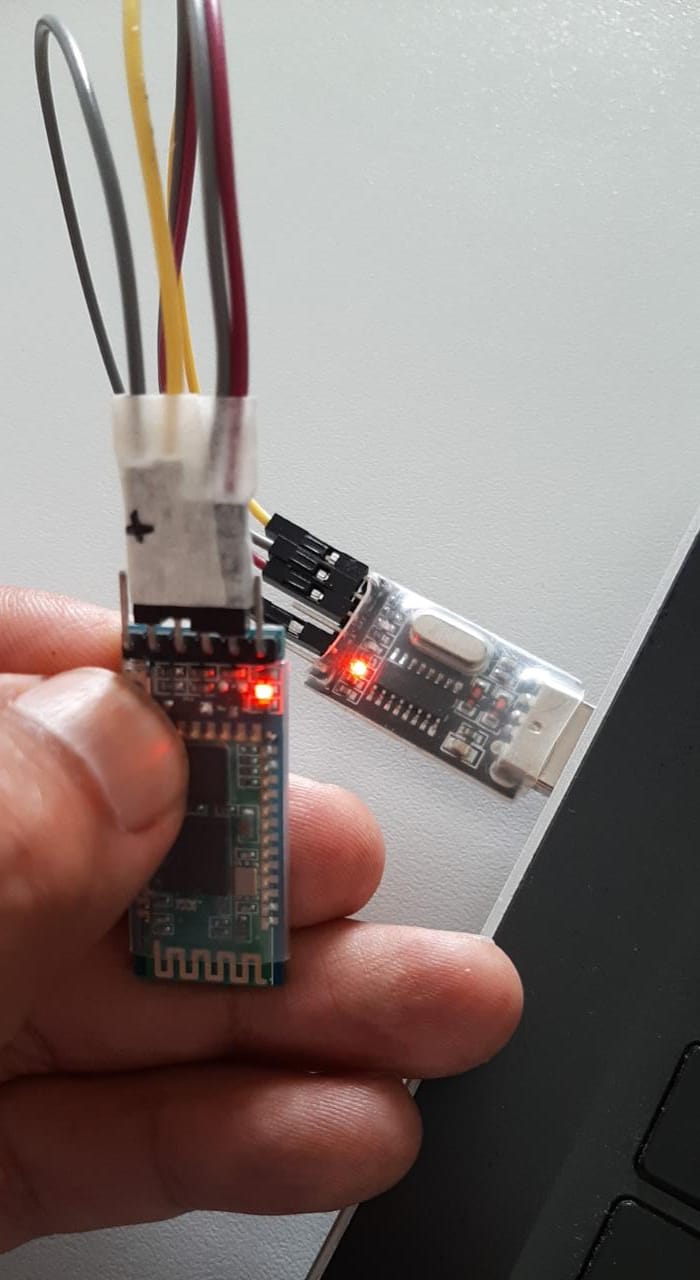
Push button pressed while USB to TTL adapter is connected.
Next, we use the Arduino IDE’s serial monitor to send the AT commands, we will use the following AT commands to query and configure Bluetooth:
Consulting parameters AT, When the response is OK, the communication is successful AT+UART, Displays the data transfer settings AT+ROLE, Indicates if it is in the role of master or slave
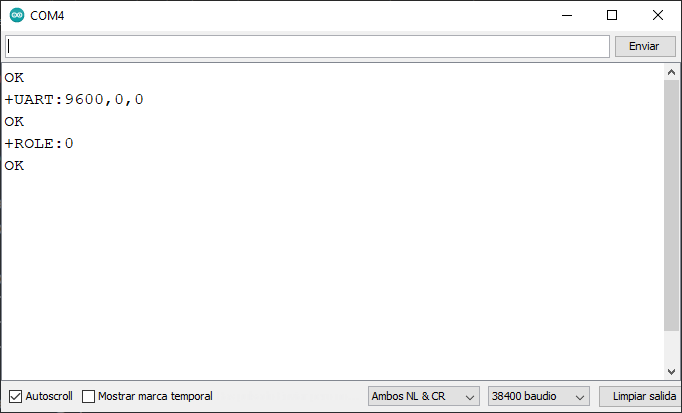
Cconsultation of the current parameters of the bluetooth HC-05.
Setting parameters AT, When the response is OK, the communication is successful AT+UART=38400,0,0 AT+ROLE=1
Consultation of the parameters after making the desired configuration of the bluetooth HC-05.
Once the bluetooth is configured, we connect them to their respective microcontrollers, the master to the Arduino UNO and the slave to our universal board.
Arduino UNO and Bluetooth HC-05 Maestro schematic.
Universal Board and Bluetooth HC-05 Slave schematic.
Finally, we connect them with the entire structure of the conveyor belt and the manual control buttons.
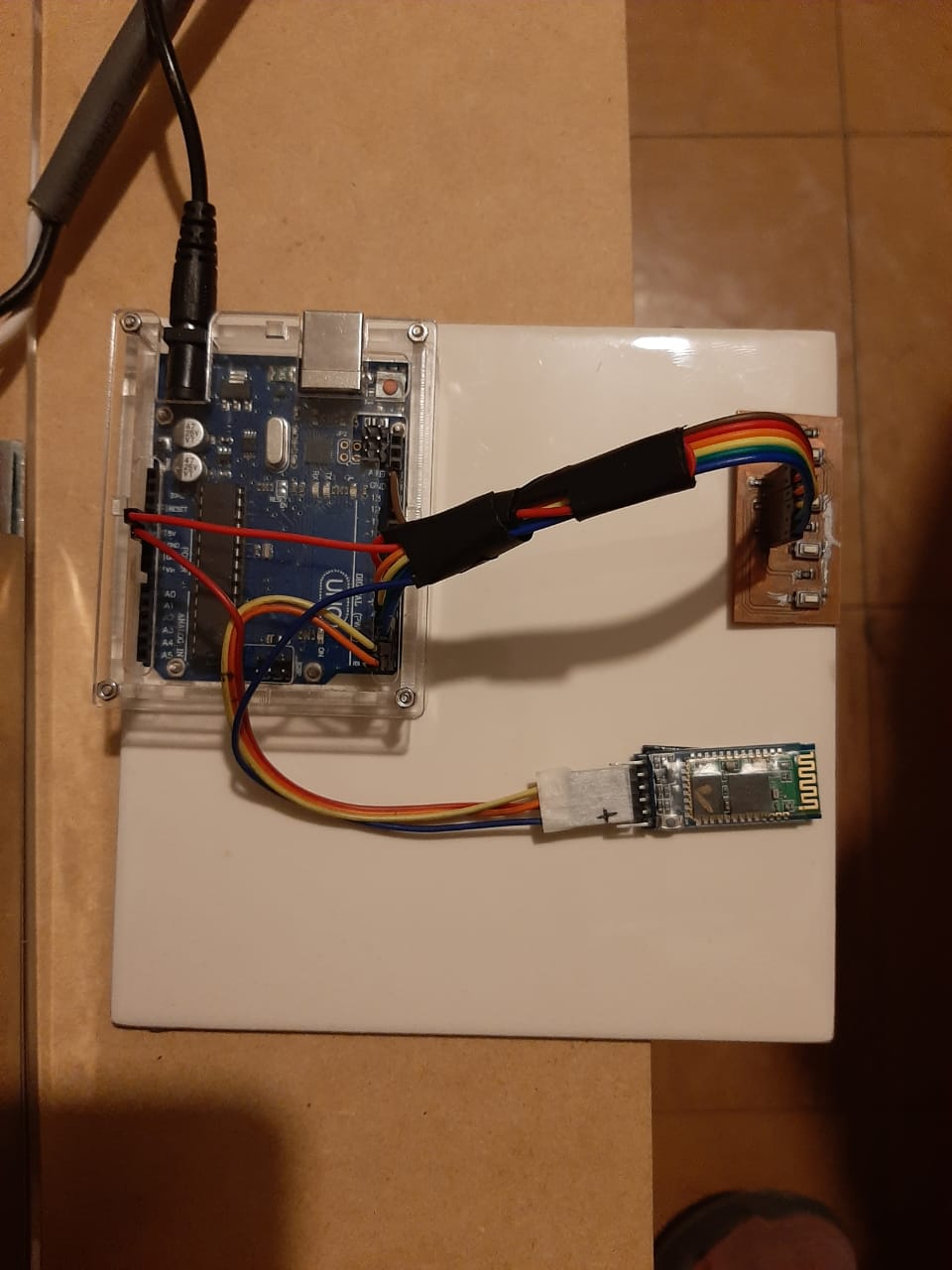
Arduino UNO with Bluetooth HC-05 Maestro and control buttons.
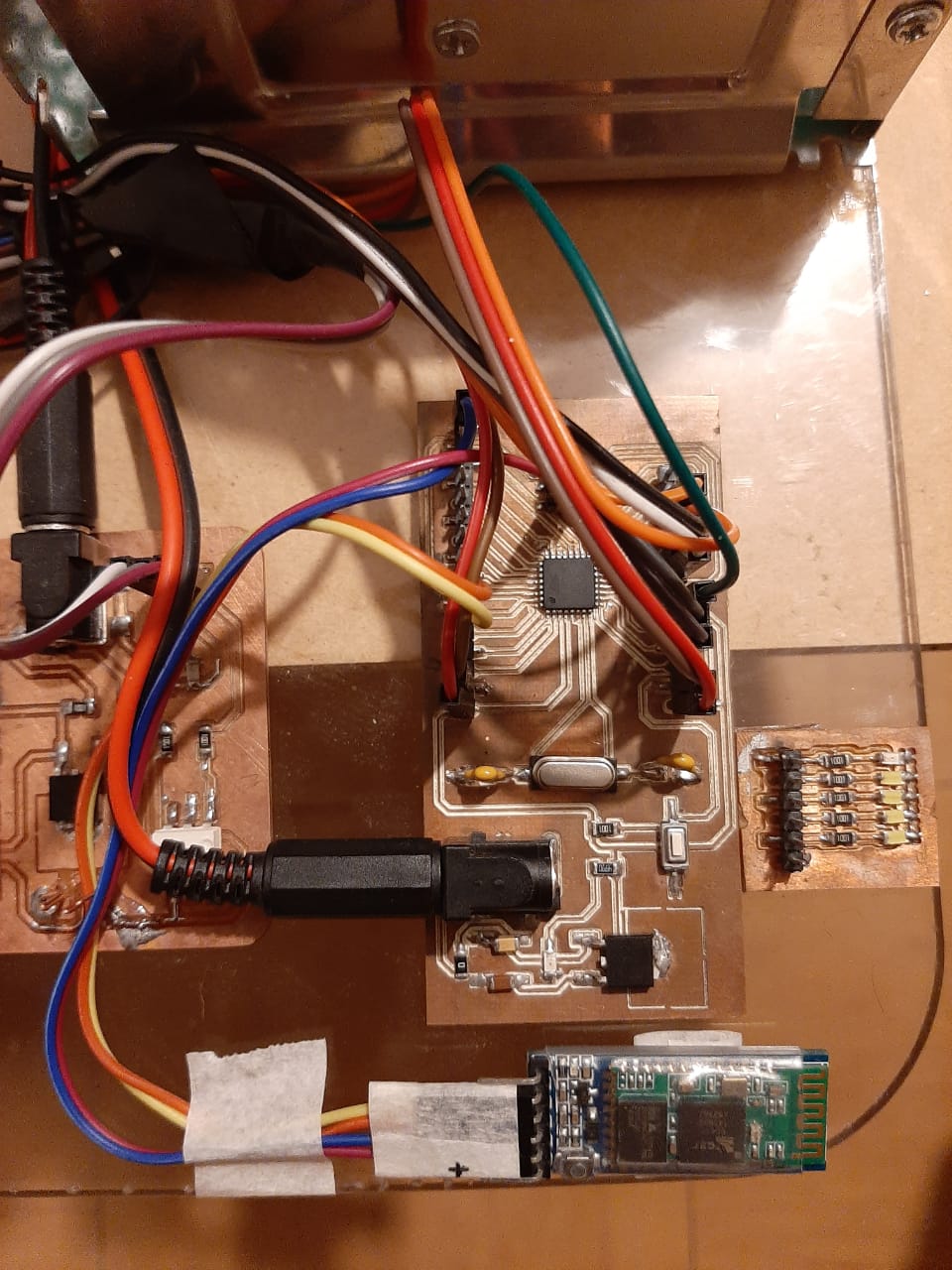
Universal Board with Bluetooth HC-05 Slave ans conveyor belt.
In the following video we show the control of the conveyor belt through the bluetooth wireless network
Hand operation of Conveyor belt through bluetooth Network Master - Slave
Code of the emitter node, master.
int ButtonStepForward = 2;
int ButtonStepReverse = 3;
int ButtonServoMetal = 4;
int ButtonServoNoMetal = 5;
void setup() {
Serial.begin(38400);
pinMode(ButtonStepForward,INPUT);
pinMode(ButtonStepReverse,INPUT);
pinMode(ButtonServoMetal,INPUT);
pinMode(ButtonServoNoMetal,INPUT);
}
void loop() {
if(!digitalRead(ButtonStepForward)){
delay(100);
Serial.print('a');
} else if(!digitalRead(ButtonStepReverse)){
delay(100);
Serial.print('b');
} else if(digitalRead(ButtonServoMetal)){
delay(250);
Serial.print('c');
} else if(digitalRead(ButtonServoNoMetal)){
delay(250);
Serial.print('d');
}
}
Receiver node code, slave
#include <Servo.h>
Servo servoMetal;
Servo servoNoMetal;
int ServoMetal = 10;
int ServoNoMetal = 11;
int pos01 = 90;
int pos02 = 90;
int Coil01 = 6;
int Coil02 = 7;
int Coil03 = 8;
int Coil04 = 9;
int espera = 20;
char data;
void setup() {
Serial.begin(38400);
pinMode(Coil01,OUTPUT);
pinMode(Coil02,OUTPUT);
pinMode(Coil03,OUTPUT);
pinMode(Coil04,OUTPUT);
servoMetal.attach(ServoMetal);
servoNoMetal.attach(ServoNoMetal);
servoMetal.write(pos01);
servoNoMetal.write(pos02);
}
void loop() {
if (Serial.available()>0){
data = Serial.read();
if (data == 'a'){
digitalWrite(Coil01,LOW);
digitalWrite(Coil02,HIGH);
digitalWrite(Coil03,HIGH);
digitalWrite(Coil04,LOW);
delay(espera);
digitalWrite(Coil01,LOW);
digitalWrite(Coil02,LOW);
digitalWrite(Coil03,HIGH);
digitalWrite(Coil04,HIGH);
delay(espera);
digitalWrite(Coil01,HIGH);
digitalWrite(Coil02,LOW);
digitalWrite(Coil03,LOW);
digitalWrite(Coil04,HIGH);
delay(espera);
digitalWrite(Coil01,HIGH);
digitalWrite(Coil02,HIGH);
digitalWrite(Coil03,LOW);
digitalWrite(Coil04,LOW);
delay(espera);
data == '0';
}
if (data == 'b'){
digitalWrite(Coil01,HIGH);
digitalWrite(Coil02,HIGH);
digitalWrite(Coil03,LOW);
digitalWrite(Coil04,LOW);
delay(espera);
digitalWrite(Coil01,HIGH);
digitalWrite(Coil02,LOW);
digitalWrite(Coil03,LOW);
digitalWrite(Coil04,HIGH);
delay(espera);
digitalWrite(Coil01,LOW);
digitalWrite(Coil02,LOW);
digitalWrite(Coil03,HIGH);
digitalWrite(Coil04,HIGH);
delay(espera);
digitalWrite(Coil01,LOW);
digitalWrite(Coil02,HIGH);
digitalWrite(Coil03,HIGH);
digitalWrite(Coil04,LOW);
delay(espera);
data == '0';
}
if (data == 'c'){
for (pos01 = 90; pos01 >= 0; pos01 -= 1) {
servoMetal.write(pos01);
delay(15);
}
for (pos01 = 0; pos01 <= 90; pos01 += 1) {
servoMetal.write(pos01);
delay(15);
}
data == '0';
}
if (data == 'd'){
for (pos02 = 80; pos02 >= 0; pos02 -= 1) {
servoNoMetal.write(pos02);
delay(15);
}
for (pos02 = 0; pos02 <= 80; pos02 += 1) {
servoNoMetal.write(pos02);
delay(15);
}
data == '0';
}
data == '0'
}
}