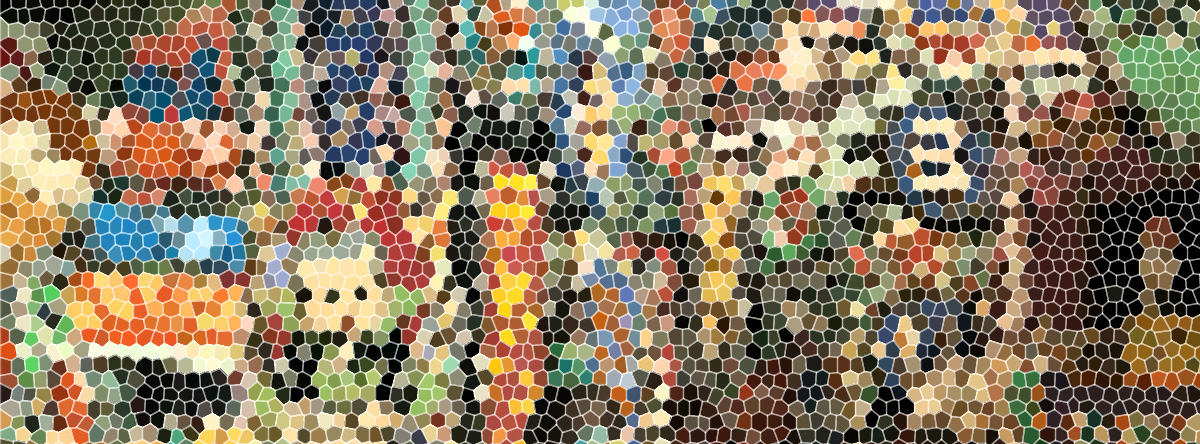
Embedded Programming
Program your board with C and Arduino
For this week assignment we will program the ATtiny44A (read the datasheet here) micro controller on your Hello Button + LED board that we made during the Electronics Design week using Neil's C code to echo back keyboard input.
To start you will need the FabISP programmed that was made in the Electronics production week , then open Arduino, in case you do not have it you can download it from its web page.
Next step is download Neil's C code and makefile
You will need open terminal and navigate to the location where you saved these files in order for the commands below to work. You must be in the same directory. Once you have everything ready proceed to connect the FabISP with your Hello Button + LED and both to the USB ports of your computer to provide energy and start programing the ATtiny44A micro controller.
1. Go to Arduino and run the ATtiny44A 20MHz
2. Chose the serial port: Tools > Seial port > // dev // tty.bluetooth-modern
3. Select the programator: Tools > Programator > USBtinyISP
4. Program your board > open terminal and navigate to where you saved the C code and makefile then type: sudo make -f hello.ftdi.44.echo.c.make program-usbtiny
5. Open the sample code and edit it (try to ad functions to your Hello Button + LED) : I changed the date and my name, also I tried with the code that when you provide energy to the board is going to turn the light automatically and when you press the button is going to turn it off. Also I added some moving to the light playing with the delays.
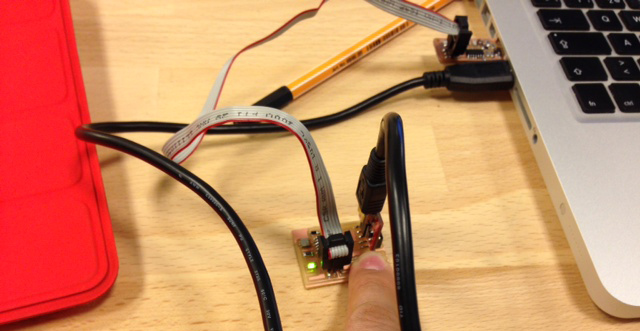
/* Attiny44a 3-pin LED + Pushbutton button turn on/off LED and runs the program that makes blink the LED created 2014 by Juan Esteban Vallejo (with help from Google and Andrew Leek) */ // constants won't change. // They're used here to set pin numbers: const int buttonPin = 3; // the number of the pushbutton pin const int ledPin = 7; // the number of the LED pin // initialize variables: int buttonState = 0; // variable for reading the pushbutton status void setup() { // initialize the LED pin as an output: pinMode(ledPin, OUTPUT); // initialize the pushbutton pin as an input: pinMode(buttonPin, INPUT); } void loop(){ // read the state of the pin the pushbutton is connected to: buttonState = digitalRead(buttonPin); // is the push button pressed? // if not pressed - the button state is HIGH // the pull up resistor the button / pin 3 makes the button state HIGH by default. if (buttonState == LOW) { // turn LED off (LED is off by default) digitalWrite(ledPin, LOW); } //otherwise..... // button is pressed else { // turn LED on: digitalWrite(ledPin, HIGH); delay(10); digitalWrite(ledPin, LOW); delay(10); } }
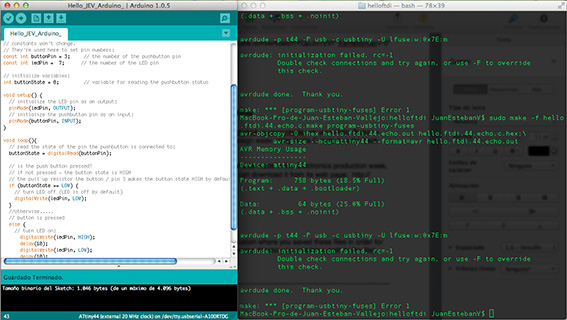