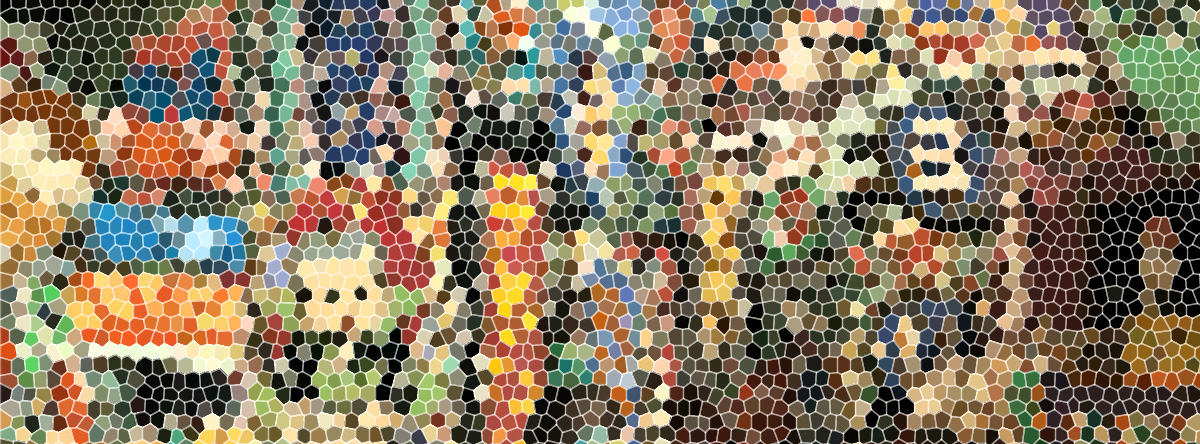
Interface and Application Programming
Code
/* interactive rgb concrete lamp interface and application by Juan Esteban Vallejo / 2014 (with help from Andrew Leek) */ import processing.serial.*; // The serial port: Serial myPort; int redRectX, redRectY; // Position of square button int greenRectX, greenRectY; // Position of square button int blueRectX, blueRectY; // Position of square button int rectSize = 200; // Diameter of rect int circleSize = 93; // Diameter of circle color redColor, greenColor, blueColor, baseColor; color currentColor; boolean redRectOver = false; boolean greenRectOver = false; boolean blueRectOver = false; void setup() { size(600, 200); redColor = color(255,0,0); greenColor = color(0,255,0); blueColor = color(0,0,255); baseColor = color(255); redRectX = 0; redRectY = 0; greenRectX = 200; greenRectY = 0; blueRectX = 400; blueRectY = 0; // List all the available serial ports: println(Serial.list()); // Open the port you are using at the rate you want: myPort = new Serial(this, "/dev/tty.usbserial-A100RTDG", 4800); } void draw() { update(mouseX, mouseY); background(baseColor); fill(redColor); rect(redRectX, redRectY, rectSize, rectSize); fill(greenColor); rect(greenRectX, greenRectY, rectSize, rectSize); fill(blueColor); rect(blueRectX, blueRectY, rectSize, rectSize); } void update(int x, int y) { if ( overRect(redRectX, redRectY, rectSize, rectSize) ) { redRectOver = true; greenRectOver = false; blueRectOver = false; } else if ( overRect(greenRectX, greenRectY, rectSize, rectSize) ) { redRectOver = false; greenRectOver = true; blueRectOver = false; } else if ( overRect(blueRectX, blueRectY, rectSize, rectSize) ) { redRectOver = false; greenRectOver = false; blueRectOver = true; } else { redRectOver = greenRectOver = blueRectOver = false; } } void mousePressed() { if (redRectOver) { //currentColor = circleColor; myPort.write("2,900"); } if (greenRectOver) { myPort.write("4,900"); } if (blueRectOver) { myPort.write("0,900"); } } boolean overRect(int x, int y, int width, int height) { if (mouseX >= x && mouseX <= x+width && mouseY >= y && mouseY <= y+height) { return true; } else { return false; } }