Programming
Calculate the angle between the two sensors
I connected the 2 Ultrasonic Sensor with the two ends of the Breadboard and prepared the code that I will use to find out where the two sensors will intersect, where it will be the zero point that I will take. That is between the two ends of the Breadboard, and when the sensors no longer have the ability to capture anyone’s movement or the area between the two sensors is no longer able to capture anything through it, I considered that this is the point I am looking for, and the following image shows the location of the two sensors in addition to how to connect them, I used the Serial option Monitior to find out the angle that will separate them, in the end I got the angle 40 with a radius of 10 cm, and I designed my project and placed the sensors based on these data.
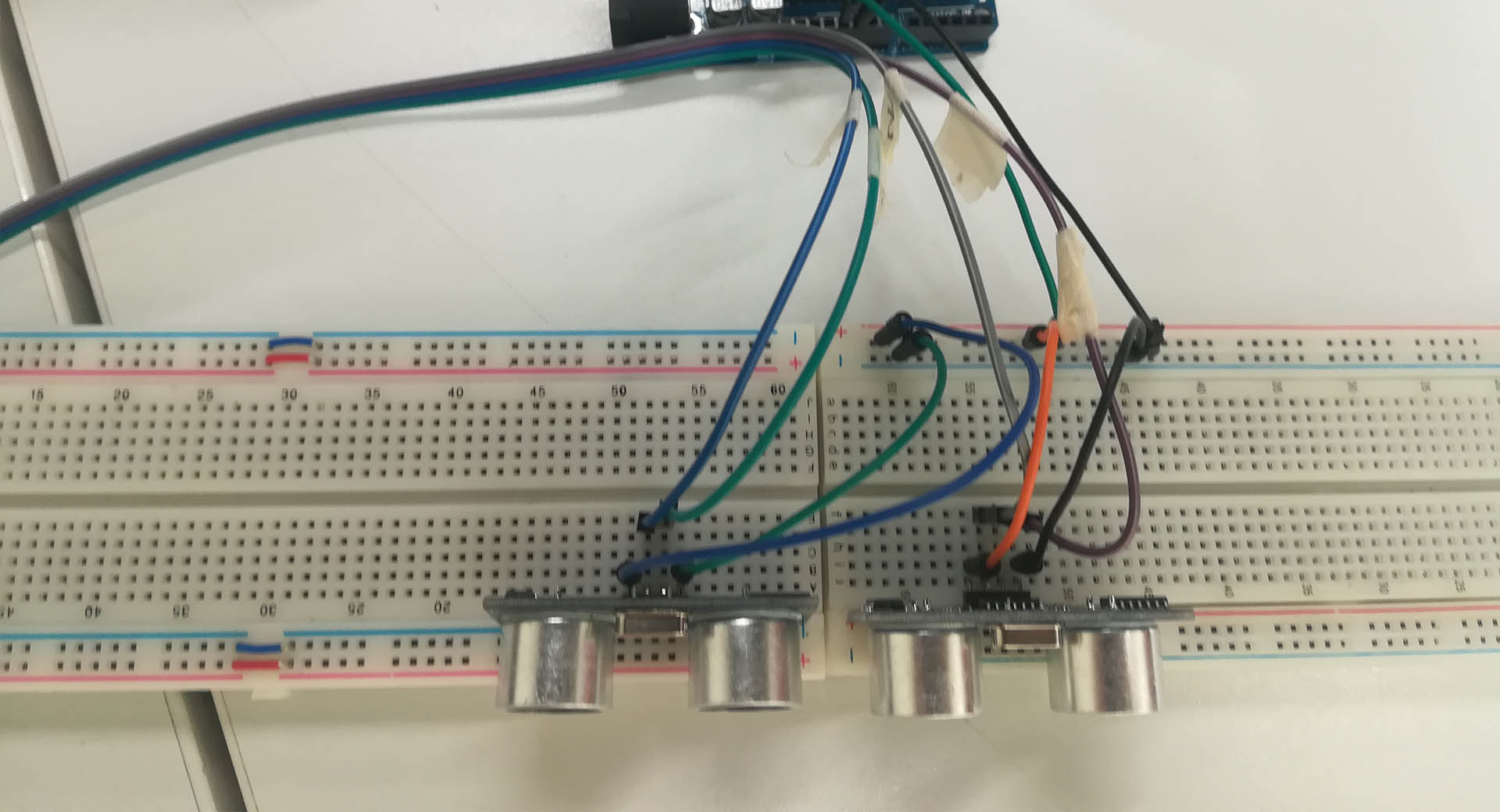
Going to the programming phase and making sure that the electronic board that I designed is working, I used the Arduino IDE software.
The image above shows the success of the operation as required "Done burning bootloader".
Code Explanation
#include <Servo.h>
Servo myservo;
const int echopin1 = 17;
const int trigpin1 = 18;
const int echopin2 = 7;
const int trigpin2 = 6;
const int servo = PC5;
long Rightduration, Leftduration, Rightinch, Leftinch;
int threshold = 10;
int angle = 80;
void setup()
{
myservo.attach(19);
}
void loop()
{
pinMode(trigpin1, OUTPUT);
digitalWrite(trigpin1, LOW);
delayMicroseconds(3);
digitalWrite(trigpin1, HIGH);
delayMicroseconds(5);
digitalWrite(trigpin1, LOW);
Rightduration = pulseIn(echopin1, HIGH);
pinMode(trigpin2, OUTPUT);
digitalWrite(trigpin2, LOW);
delayMicroseconds(3);
digitalWrite(trigpin2, HIGH);
delayMicroseconds(5);
digitalWrite(trigpin2, LOW);
Leftduration = pulseIn(echopin2, HIGH);
Rightinch = microsecondsToInches(Rightduration);
Leftinch = microsecondsToInches(Leftduration);
follow();
}
long microsecondsToInches(long microseconds)
{
return microseconds / 74 / 2;
}
void follow()
{
if (Leftinch <= threshold || Rightinch <= threshold)
{
if (Leftinch + 2 < Rightinch)
{
angle = angle - 2;
}
if (Rightinch + 2 < Leftinch)
{
angle = angle + 2;
}
}
if (angle > 160)
{
angle = 160;
}
if (angle < 0)
{
angle = 0;
}
myservo.write(angle);
}
void follow() Conditions For The Ffollow Command
Files - Open Source
Arduino Code