11. Input devices¶
Group assignment:¶
-
Probe an input device(s)’s analog and digital signals
-
Document your work on the group work page and reflect on your individual page what you learned
To see our group assignment click here
Individual assignment:¶
- add a sensor to a microcontroller board that you have designed and read it.
FIRST PROJECT¶
SIM800L V1 GSM GPRS Module¶
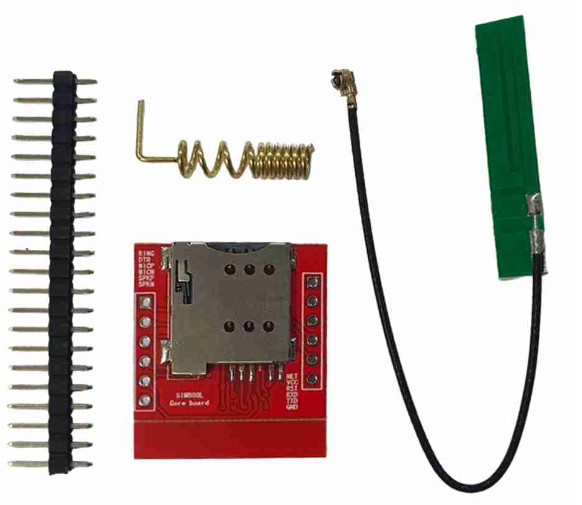
The SIM800L is a compact GSM/GPRS (Global System for Mobile Communications/General Packet Radio Service) module designed to enable electronic devices to connect to the cellular network for voice and data communications. It is often used in IoT (Internet of Things) projects, vehicle tracking, remote monitoring, and many other applications requiring cellular connectivity.
SIM800L module operation
The SIM800L module is a compact device enabling electronic devices to connect to the GSM/GPRS cellular network for voice and data communications. To operate, it requires a suitable power supply and a valid SIM card. Typically communicating via a UART interface with a microcontroller or host system, the module is initially configured using AT commands to establish the network connection and configure the necessary parameters. Once connected, it can be used to send SMS, make calls, receive GPRS data, etc. The SIM800L manages communications with the cellular network, optimizing data transmission and reception functions.
Communication with the SIM800L module is usually via a UART interface, where AT commands are sent from the host system or microcontroller to configure the module and perform various operations such as sending SMS messages or making calls. Responses from the module, indicating the success or failure of the commands, are received back and processed by the host system, enabling efficient control and use of the module’s functionality for applications such as remote monitoring, surveillance or communication in the Internet of Things (IoT).
Module pinout
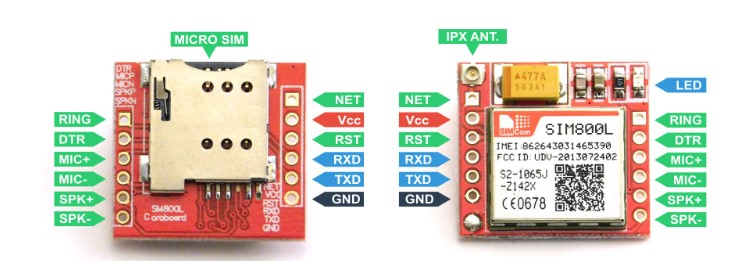
Module pinout
Referring to the following diagram, you should be able to wire the schematic.
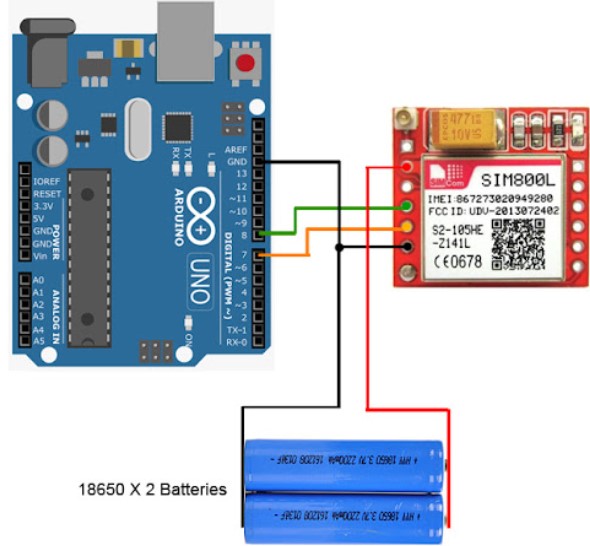
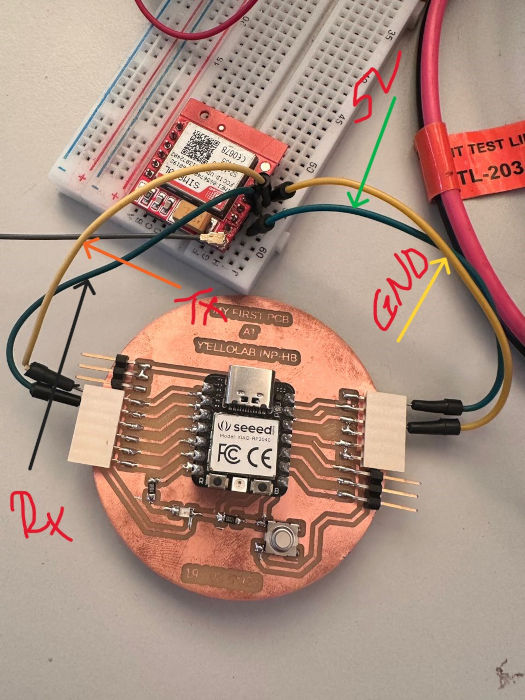
Arduino code - Sending an SMS
In this section, we’ll show you how to send an SMS using the SIM800L module. Here’s an example code:
Arduino IDE code image
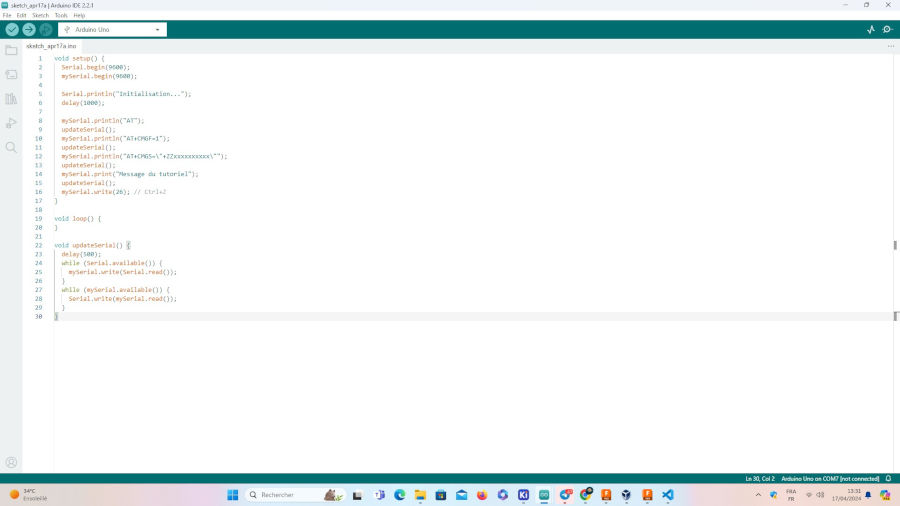
#include <SoftwareSerial.h>
SoftwareSerial mySerial(3, 2); // Tx, Rx
void setup() {
Serial.begin(9600);
mySerial.begin(9600);
Serial.println("Initialisation...");
delay(1000);
mySerial.println("AT");
updateSerial();
mySerial.println("AT+CMGF=1");
updateSerial();
mySerial.println("AT+CMGS=\"+ZZxxxxxxxxxx\"");
updateSerial();
mySerial.print("Message du tutoriel");
updateSerial();
mySerial.write(26); // Ctrl+Z
}
void loop() {
}
void updateSerial() {
delay(500);
while (Serial.available()) {
mySerial.write(Serial.read());
}
while (mySerial.available()) {
Serial.write(mySerial.read());
}
}
Code explanation
Once again, we use the SoftwareSerial library for communication.
In the setup() function, we send the AT commands needed to configure the SIM800L module in SMS mode and specify the destination phone number.
Next, we use mySerial.print() to send the message content and mySerial.write() to send the end-of-message character (Ctrl+Z).
The loop() function is empty, as we only want to send the SMS once.
normally, when you type the command AT in the search bar of the serial monitor, you should get the message OK.
SECOND PROJECT¶
Ultrasound sensor¶
Thanks to the ultrasonic sensor, we’re going to make a simple radar project. The sensor will read the data and display it in the serial monitor if there is an obstacle.
Referring to the following diagram, you should be able to wire the schematic.
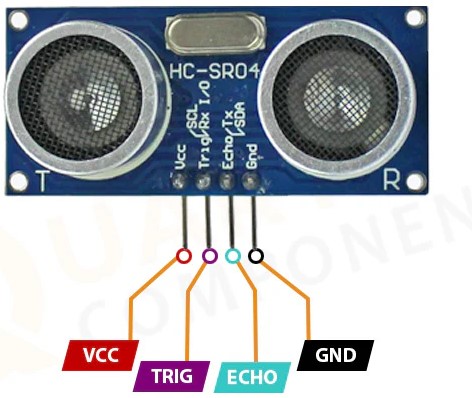
Ultrasound sensor operation
The ultrasonic sensor emits short, high-frequency sound pulses at regular intervals. These pulses travel through the air at the speed of sound. When they strike an object, they are reflected and return as an echo to the transducer.
from machine import Pin, time_pulse_us
import time
# Broches GPIO connectées au capteur à ultrasons
trigger_pin = Pin(27, Pin.OUT)
echo_pin = Pin(26, Pin.IN)
# Fonction pour mesurer la distance
def mesure_distance():
# Envoyer une impulsion de déclenchement au capteur
trigger_pin.value(0)
time.sleep_us(2)
trigger_pin.value(1)
time.sleep_us(10)
trigger_pin.value(0)
duration = time_pulse_us(echo_pin, 1, 30000) # Attendre jusqu'à 30 ms pour le signal de retour
# Convertir la durée en distance (en cm)
distance_cm = duration / 58 # Conversion en cm (343 m/s, vitesse du son dans l'air)
return distance_cm
# Boucle principale
while True:
distance = mesure_distance()
print("Distance:", distance, "cm")
time.sleep(1) # Attendre une seconde avant la prochaine lecture
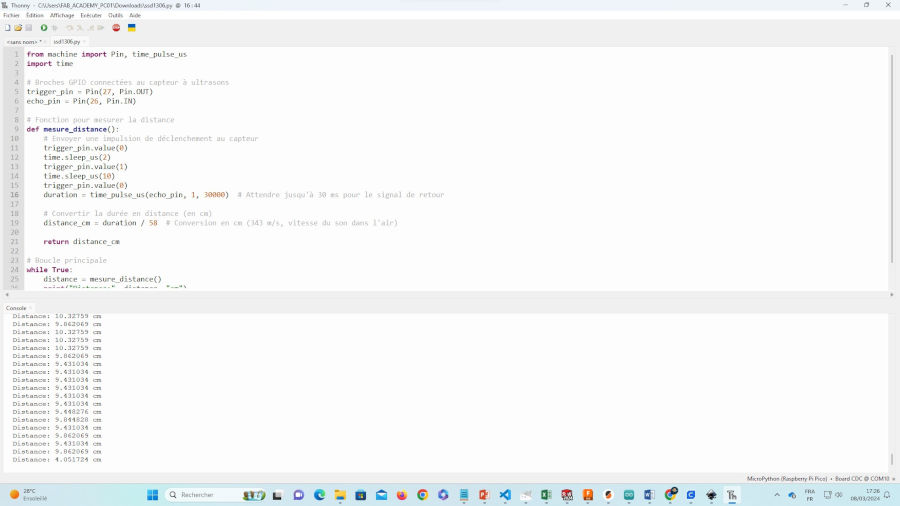
Observe the result by watching this video
Project files¶
End