9. Output devices¶
Group assignment:¶
-
Measure the power consumption of an output device.
-
Document your work to the group work page and reflect on your individual page what you learned
To see our group assignment click here
Individual assignment:¶
- Add an output device to a microcontroller board you’ve designed and program it to do something.
Hi! For this week, we’ve chosen a servomotor and DC motor with the board we designed for the mission.
Servomoteur¶
A servomotor is a device used in many systems to precisely control the position, speed or acceleration of a mechanism. It usually consists of an electric motor, a control circuit and a feedback mechanism.
Circuit diagram
Using the board we’ve designed, we’ve wired up our servomotor, and used a buzzer to produce a sound when the servomotor rotates.
TEST 1¶
We’ve written code in micropython using the Thonny editor introduced in Week 6.
Code
Library
Definition of the GPIO pin to which the servomotor is connected
PWM initialization for servomotor control
Conversion of angle to PWM pulse width (500 to 2500 µs for a range of 0 to 180 degrees)
pulse_width = int((angle / 180.0) * (2500 - 500) + 500)
servo_pwm.duty_u16(pulse_width * 65536 // 20000) # Conversion de la largeur d'impulsion en degrés
def play_train_sound():
# Jouer un son de train
buzzer_pin.on()
time.sleep_ms(100)
buzzer_pin.off()
time.sleep_ms(50)
while True:
# Faire tourner le servomoteur de 0 à 180 degrés
for angle in range(0, 181, 10):
set_angle(angle)
play_train_sound() # Jouer le son de train
time.sleep_ms(10) # Attendre 30 ms pour que le servomoteur atteigne la position
# Faire tourner le servomoteur de 180 à 0 degrés
for angle in range(180, -1, -10):
set_angle(angle)
play_train_sound() # Jouer le son de train
time.sleep_ms(10) # Attendre 30 ms pour que le servomoteur atteigne la position
TEST 1 Result
TEST 2¶
We’re going to program a DC motor using the board we’ve designed and a driver.
DC motor
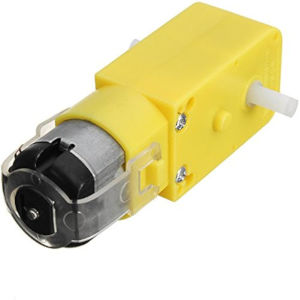
Module L298N
The L298N module is a popular double H-bridge motor controller used to control the speed and direction of DC motors and bipolar stepper motors.
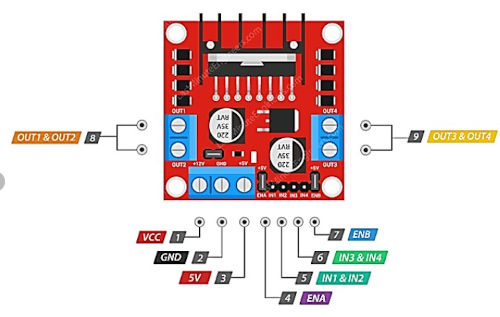
Circuit diagram
Code
Library
//*******************************************************************************//
// Association of the L298N inputs with the outputs used on our Arduino Uno //
//*******************************************************************************//
import machine
import utime
Pin definition
borneENA = machine.PWM(machine.Pin(26))
borneIN1 = machine.Pin(27, machine.Pin.OUT)
borneIN2 = machine.Pin(28, machine.Pin.OUT)
borneIN3 = machine.Pin(29, machine.Pin.OUT)
borneIN4 = machine.Pin(6, machine.Pin.OUT)
borneENB = machine.PWM(machine.Pin(5))
Direction of rotation
Direction of rotation setting function
def configurerSensDeRotationPontA(sensDeRotation):
if sensDeRotation == MARCHE_AVANT:
borneIN1.value(1)
borneIN2.value(0)
elif sensDeRotation == MARCHE_ARRIERE:
borneIN1.value(0)
borneIN2.value(1)
Motor speed change function
def changeVitesseMoteurPontA(nouvelleVitesse):
borneENA.duty_u16(nouvelleVitesse * 256) # Le RP2040 utilise une résolution de 16 bits pour le PWM
Output pin configuration
borneENA.freq(1000) # PWM signal frequency
borneENB.freq(1000)
borneIN1.value(0)
borneIN2.value(0)
borneIN3.value(0)
borneIN4.value(0)
Main loop
while True:
# MARCHE AVANT
configurerSensDeRotationPontA(MARCHE_AVANT)
for vitesse in range(vitesseMinimale, vitesseMaximale):
changeVitesseMoteurPontA(vitesse)
utime.sleep_ms(delaiChangementVitesse)
for vitesse in range(vitesseMaximale, vitesseMinimale, -1):
changeVitesseMoteurPontA(vitesse)
utime.sleep_ms(delaiChangementVitesse)
# Pause
changeVitesseMoteurPontA(0)
utime.sleep(1)
# MARCHE ARRIERE
configurerSensDeRotationPontA(MARCHE_ARRIERE)
for vitesse in range(vitesseMinimale, vitesseMaximale):
changeVitesseMoteurPontA(vitesse)
utime.sleep_ms(delaiChangementVitesse)
for vitesse in range(vitesseMaximale, vitesseMinimale, -1):
changeVitesseMoteurPontA(vitesse)
utime.sleep_ms(delaiChangementVitesse)
# Pause
changeVitesseMoteurPontA(0)
utime.sleep(1)
in reality, this is the type of motor we’re going to use for our robot’s navigation, so we’ve decided to write a program to see how it works.
this link will take you to a video of the robot being navigated by the motor
File source¶
End