WEEK15 - Interface & Application Programming
This week is about learning to build interfaces. It's a hard week for me. I'm not good a coding, and also i struggle to see how it relates to my final project.
WEEK FIFTEEN ASSIGNMENT
Assignments | Completed |
---|---|
GROUP | |
- Compare as many tool options as possible | GROUP ASSIGNMENT PAGE |
INDIVIDUAL | |
- Write an application that interfaces a user with an input &/or output device that you made | - done |
HERO-SHOTS
GROUP ASSIGNMENT
forthcoming
THOUGHS, EXPLORATION AND IDEAS
through out Neil's lecture it became very clear that this week would be easy for those who already have strong background in coding and a nightmare for those like me who deeply struggled with code already.
Based on the lecture I'm most keenly interested in the following things:
-
Ncurses, which Neil had mentioned to me way in the beginning in relation to my final project and which I kept wanting to try but just couldn't properly figure out.
-
Stable Diffusion
-
Touch designer
-
Processing
-
js5
-
Unity
etc etc etc
WHAT IS INTERFACE?
The process here was to create a computer interface or app which would be able to interact directly with board etc.
TIME MANAGEMENT
As we are heading further into final project. I really need to get a grip on time management. While there are many thing I wanted to learn and figure out with interfaces... I need to make a decision to focus on Processing
PROCESSING
FINDING OUT YOUR PORT
Before starting the code for processing. I had to find out my port.
import processing.serial.*;
Serial myPort;
printArray(Serial.list());
//γγγ§γ·γͺγ’γ«ιδΏ‘ιε§
myPort = new Serial(this, Serial.list()[0], 9600);
myPort.write(65);
BUTTON: ARDUINO SIDE
Once I knew my port, it got time to figure out some coding. I started with just some example code for a blinking-square which would blink after I would press the button on my board.
// Wiring / Arduino Code
// Code for sensing a switch status and writing the value to the serial port.
int switchPin = 7; // Switch connected to pin 4
// variables will change:
int buttonState = 1; // variable for reading the pushbutton status
void setup() {
pinMode(switchPin, INPUT); // Set pin 0 as an input
Serial.begin(9600); // Start serial communication at 9600 bps
}
void loop() {
if (digitalRead(switchPin) == HIGH) { // If switch is ON,
Serial.print(1); // send 1 to Processing
Serial.write(1); // send 1 to Processing
} else { // If the switch is not ON,
Serial.print((byte) 0x00); // send 0 to Processing
Serial.write((byte) 0x00); // send 0 to Processing
}
delay(50); // Wait 100 milliseconds
}
BUTTON: PROCESSING SIDE
/**
* Simple Read
*
* Read data from the serial port and change the color of a rectangle
* when a switch connected to a Wiring or Arduino board is pressed and released.
* This example works with the Wiring / Arduino program that follows below.
*/
import processing.serial.*;
Serial myPort; // Create object from Serial class
int val; // Data received from the serial port
void setup()
{
size(200, 200);
// I know that the first port in the serial list on my mac
// is always my FTDI adaptor, so I open Serial.list()[0].
// On Windows machines, this generally opens COM1.
// Open whatever port is the one you're using.
String portName = Serial.list()[4];
myPort = new Serial(this, portName, 9600);
}
void draw()
{
if ( myPort.available() > 0) { // If data is available,
val = myPort.read(); // read it and store it in val
}
background(255); // Set background to white
if (val == 0) { // If the serial value is 0,
fill(0); // set fill to black
}
else { // If the serial value is not 0,
fill(204); // set fill to light gray
}
rect(50, 50, 100, 100);
}
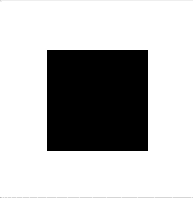
LIGHT 2 PROCESSING: ARDUINO
Once the button worked, I wanted to keep interacting with the noise aswell as the light level inputs.
This feels alot like early two-thousands audio visuals that I'd use alot when I was a kid. So in someways its like looking behind some childhood magic.
int light_sensor = 8;
const int spPin = 5;
void setup() {
Serial.begin(9600); //begin Serial Communication
pinMode(spPin,OUTPUT);
}
void loop() {
int raw_light = analogRead(light_sensor); // read the raw value from light_sensor pin (A3)
int light = map(raw_light, 0, 1023, 0, 360); // map the value from 0, 1023 to 0, 100
//Serial.print("Light level: ");
//Serial.println(light); // print the light value in Serial Monitor
Serial.write(light); // send 1 to Processing
//tone(spPin, light);
tone(spPin, light);
delay(50); // add a delay to only read and print every 1 second
}
LIGHT 2 PROCESSING: PROCESSING SIDE:
import processing.serial.*;
Serial myPort;
int dim;
int light;
void setup() {
size(640, 360);
dim = width/2;
background(0);
colorMode(HSB, 360, 100, 100);
noStroke();
ellipseMode(RADIUS);
String portName = Serial.list()[4];
myPort = new Serial(this, portName, 9600);
}
void draw() {
if (myPort.available() > 0) {
light = myPort.read();
println(light);
//light = map(light, 0, 255, 0, 360);
}
background(0);
for (int x = 0; x <= width; x+=dim) {
drawGradient(x, height/2);
}
}
void drawGradient(float x, float y) {
int radius = dim/2;
float h = light;
for (int r = radius; r > 0; --r) {
fill(h, 90, 90);
ellipse(x, y, r, r);
h = (h + 1) % 360;
}
}
BOOM
soo yeah.. it works. but I wanna do more but honestly, i'm kinda stressed and paralysed by final project so i keep falling behind my own schedule everyday....
FILES
Button2squareblink: Arduiono: sketch_button2processing.zip
Processing: sketch_button2processing.zip
Lightsensor2gradient links: