Networking and Communications
This week, we did some experiments on communicating between boards via TTL protocal.
Documented by Darren Chan
Experiment1 - USB-TTL -> HelloBoard
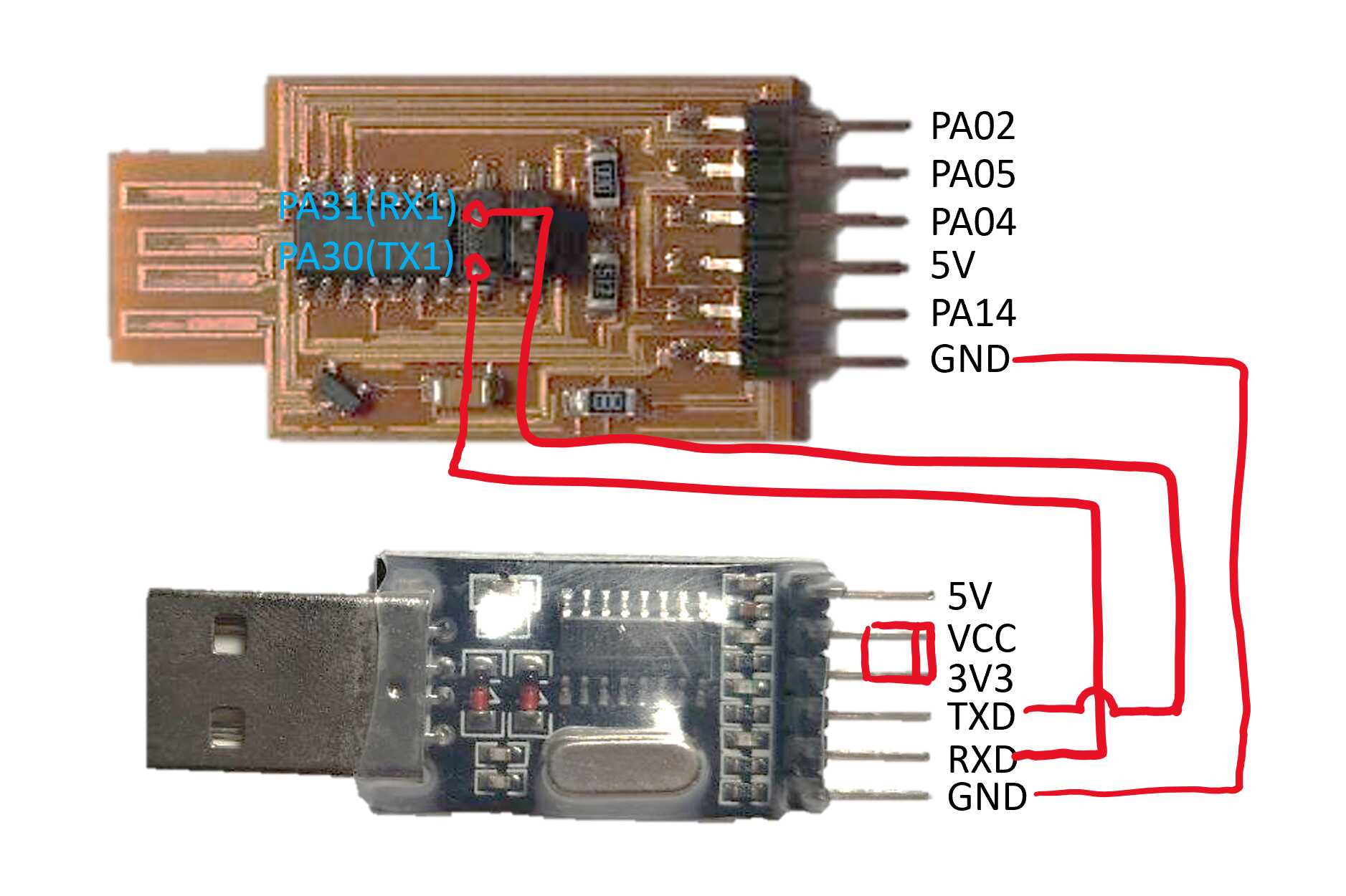
C++ |
---|
| void setup() {
Serial.begin(9600);
Serial1.begin(9600);
}
void loop() {
Serial.println("Hello Serial");
Serial1.println("Hello Serial1");
delay(1000);
}
|
Experiment2 - HelloBoard -> USB-TTL
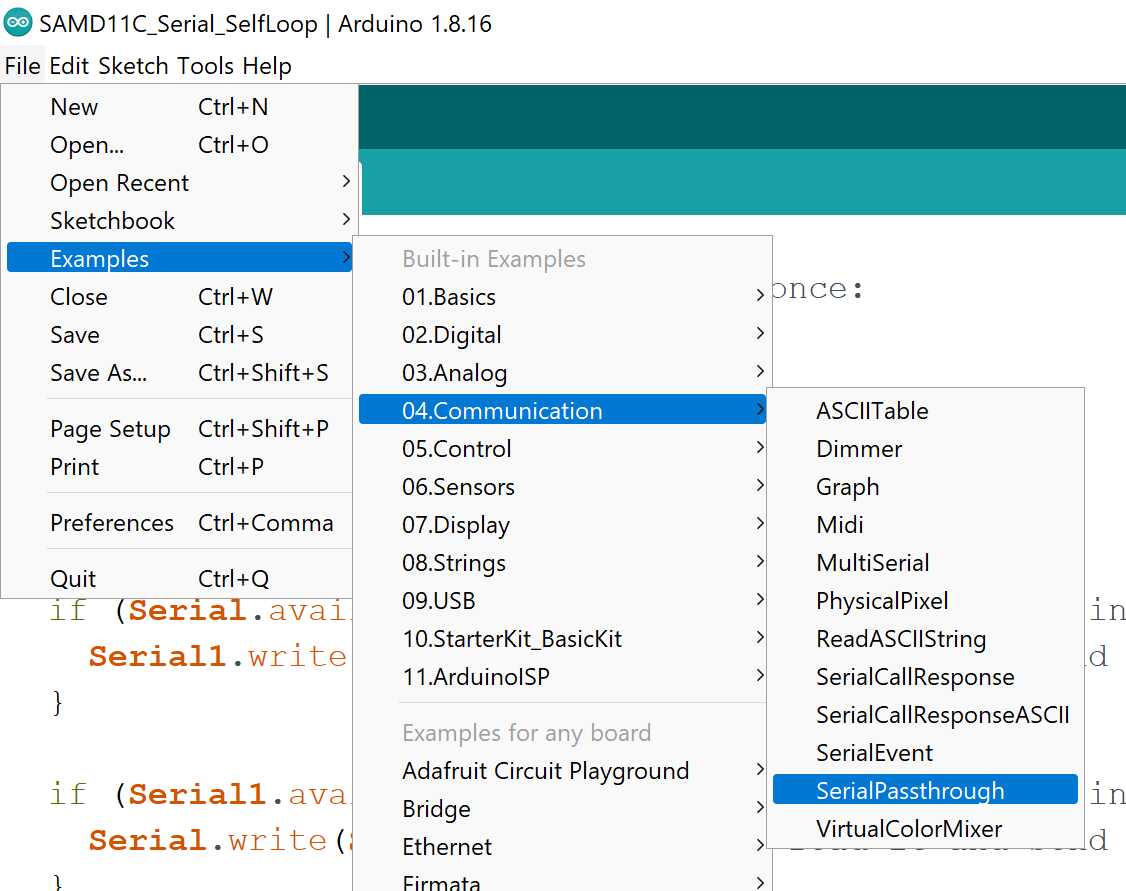
C++ |
---|
| void setup() {
Serial.begin(9600);
Serial1.begin(9600);
}
void loop() {
// put your main code here, to run repeatedly:
if (Serial.available()) { // If anything comes in Serial (USB),
Serial1.write(Serial.read()); // read it and send it out Serial1 (pins 0 & 1)
}
if (Serial1.available()) { // If anything comes in Serial1 (pins 0 & 1)
Serial.write(Serial1.read()); // read it and send it out Serial (USB)
}
}
|
Experiment3 - HelloBoard <-> Arduino MEGA 2560
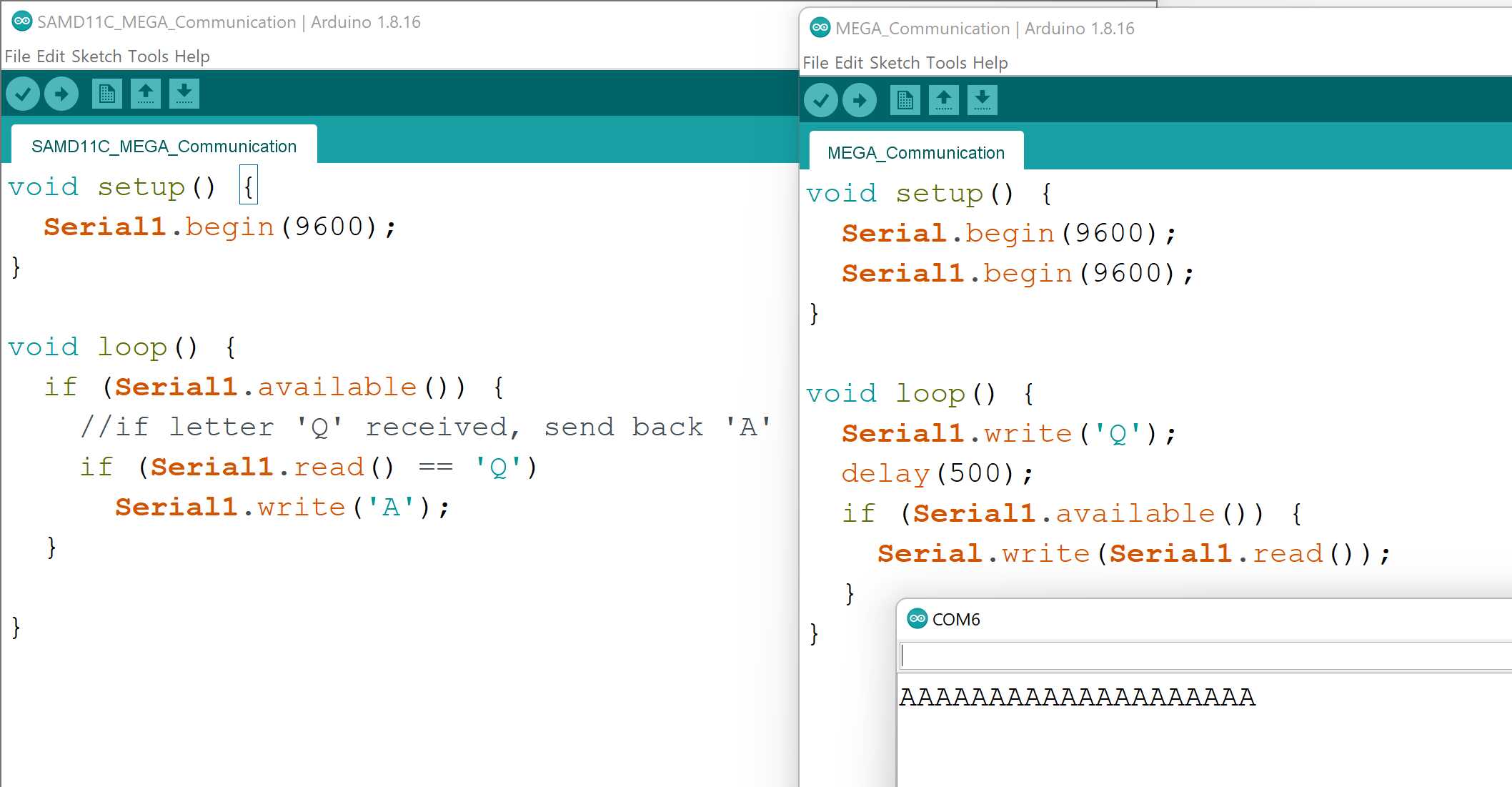
C++ |
---|
| void setup() {
Serial1.begin(9600);
}
void loop() {
if (Serial1.available()) {
//if letter 'Q' received, send back 'A'
if (Serial1.read() == 'Q')
Serial1.write('A');
}
}
|
C++ |
---|
| void setup() {
Serial.begin(9600);
Serial1.begin(9600);
}
void loop() {
Serial1.write('Q');
delay(500);
if (Serial1.available()) {
Serial.write(Serial1.read());
}
}
|
Downloads
SAMD11C_Serial_Test.zip
Communication_Test_MEGA.zip
SAMD11C_Serial_SelfLoop.zip
Communication_Test_SAMD11C.zip
Last update:
May 3, 2022