Week 14
Interface and Application Programming
Group Assignment
1. Compare as many tool options as possible
Individual Assignment
1. Write an application that interfaces a user with an input &/or output device that you made
Graphic User Interface (GUI): According to Wikipedia, a GUI is a form of user interface that allows users to interact with electronic devices through graphical icons and audio indicator such as primary notation, instead of text-based user interfaces, typed command labels or text navigation. In today’s times, graphical user interfaces are used in many devices such as mobiles, MP3 players, gaming devices, etc. We can see GUI in almost all the electronic components we use in daily basis like oven, washing machine, smart phones, printing tokens in banks, etc. These make use easy to understand and input the required information without displaying all the process going behind to perform the task. The importance if GUI is to communicate with the user which and delivering the result to user by processing in a hidden background. This way the effectiveness of communication is better
Understanding Processing 3
Processing is a simple programming environment that was created to make it easier to develop visually oriented applications with an emphasis on animation and providing users with instant feedback through interaction. The developers wanted a means to “sketch” ideas in code. As its capabilities have expanded over the past decade, Processing has come to be used for more advanced production-level work in addition to its sketching role. Originally built as a domain-specific extension to Java targeted towards artists and designers, Processing has evolved into a full-blown design and prototyping tool used for large-scale installation work, motion graphics, and complex data visualization.
Processing is based on Java, but because program elements in Processing are fairly simple, you can learn to use it even if you don't know any Java. If you're familiar with Java, it's best to forget that Processing has anything to do with Java for a while, until you get the hang of how the API works.
The latest version of Processing can be downloaded at https://processing.org/download.
An important goal for the project was to make this type of programming accessible to a wider audience. For this reason, Processing is free to download, free to use, and open source. But projects developed using the Processing environment and core libraries can be used for any purpose. This model is identical to GCC, the GNU Compiler Collection. GCC and its associated libraries (e.g. libc) are open source under the GNU Public License (GPL), which stipulates that changes to the code must be made available. However, programs created with GCC (examples too numerous to mention) are not themselves required to be open source.
To know more about processing, you may click here
Individual Assignment
For this week's work, I wanted to do something using processing, as it is one software that always fascinated me. It is also very easy to use especially if you have some basic idea about the Java IDE.
I wanted to use the bunny board that I made in the previous week and use it to play songs with my keyboard and that was supposed to create patterns on the screen. I was very fascinated about the whole thing and my colleague Saheen helped me troubleshoot and gave me a better understanding of the whole thing.
For the project, what I essentially had to do, was to save sounds for different keys, make the computer communicate with the board to play different sounds through serial communication. I also wanted to make a visual outputs for each key, along with the sounds
For the board, I wanted to use the same board that I used for the previous assignment, as I wanted to experiment with different sounds too. I used the hello echo board from the earlier week and then used the bunny board from my previous week's work, to make it sing (make noise) according to my keys and give a visual output.
The connection was made such that, the hello echo board communicated with the bunny board getting me an output of sounds based on the key input that I give.
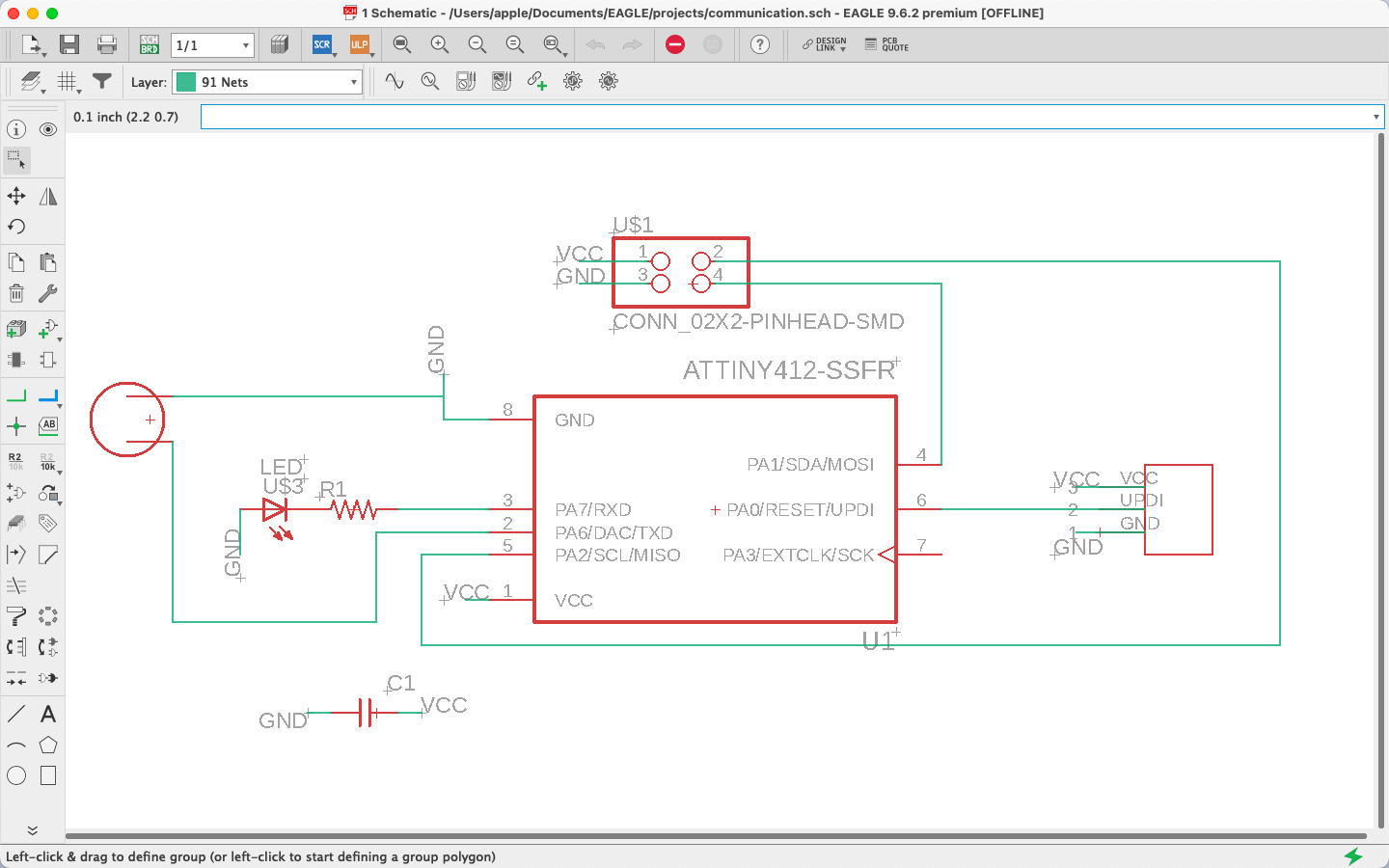
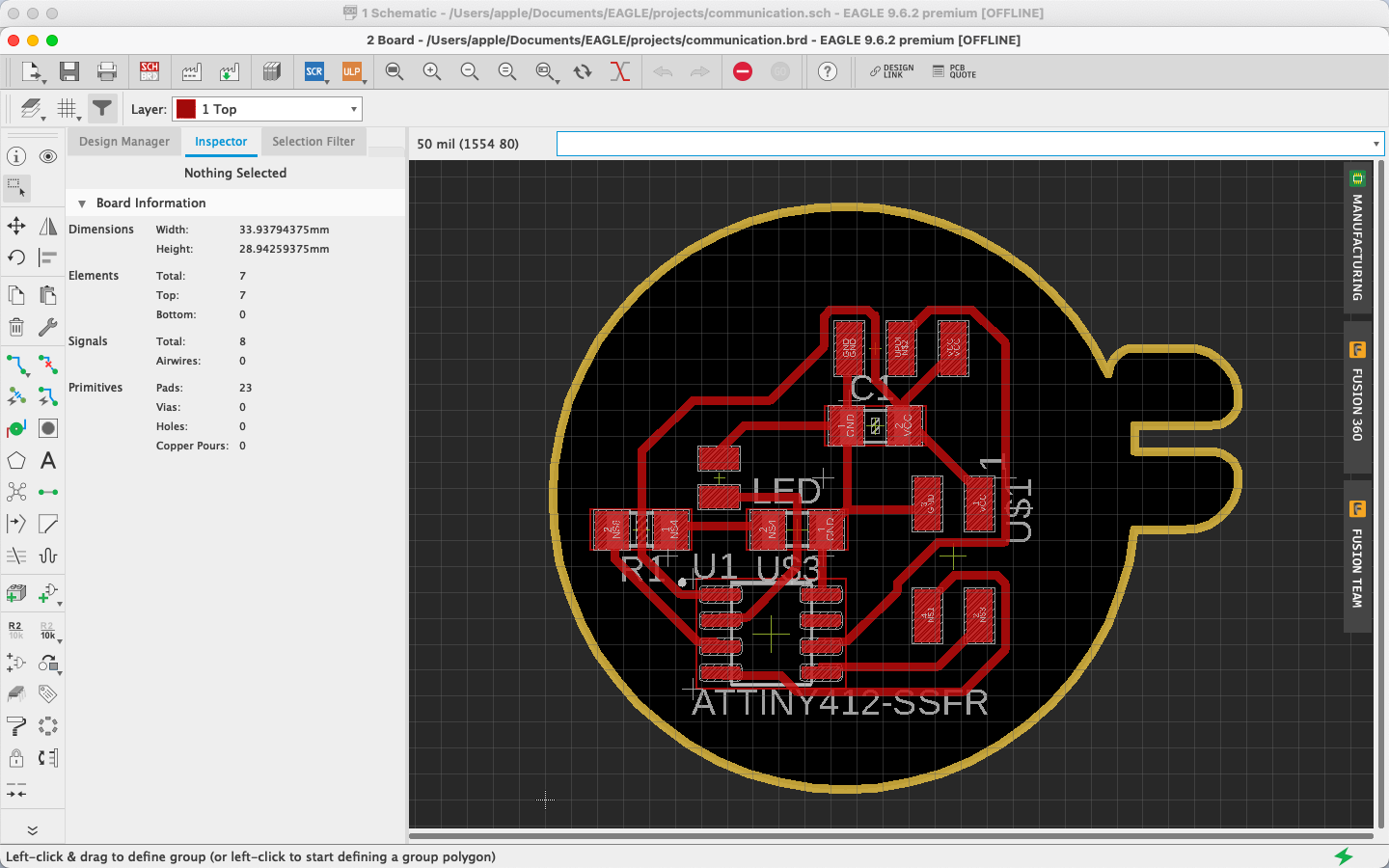
I first coded the part which would give me the visual output and also connected the ports to the respective keys
import processing.serial.*;
Serial myPort;
int rectWidth;
void setup()
{
size(640, 360);
noStroke();
background(0);
rectWidth = width/4;
printArray(Serial.list());
myPort = new Serial(this, Serial.list()[2], 9600);
}
fjsj
void draw()
{
// keep draw() here to continue looping while waiting for keys
}
void keyPressed()
{
int keyIndex = -1;
if (key >= 'A' && key <= 'Z') {
keyIndex = key - 'A';
} else if (key >= 'a' && key <= 'z') {
keyIndex = key - 'a';
}
if (keyIndex == -1) {
// If it's not a letter key, clear the screen
background(0);
} else {
// It's a letter key, fill a rectangle
fill(millis() % 255);
float x = map(keyIndex, 0, 25, 0, width - rectWidth);
rect(x, 0, rectWidth, height);
}
switch(key) {
case 'a': myPort.write(97); break;
case 'b': myPort.write(98); break;
case 'c': myPort.write(99); break;
case 'd': myPort.write(100); break;
case 'e': myPort.write(101); break;
case 'f': myPort.write(102); break;
case 'g': myPort.write(103); break;
case 'h': myPort.write(104); break;
case 'i': myPort.write(105); break;
case 'j': myPort.write(106); break;
case 'k': myPort.write(107); break;
case 'l': myPort.write(108); break;
case 'm': myPort.write(109); break;
case 'n': myPort.write(110); break;
case 'o': myPort.write(111); break;
case 'p': myPort.write(112); break;
case 'q': myPort.write(113); break;
case 'r': myPort.write(114); break;
case 's': myPort.write(115); break;
case 't': myPort.write(116); break;
case 'u': myPort.write(117); break;
case 'v': myPort.write(118); break;
case 'w': myPort.write(119); break;
case 'x': myPort.write(120); break;
case 'y': myPort.write(121); break;
case 'z': myPort.write(122); break;
}
}
I then took some help from my colleague who helped me understand how making tunes works. I then uploaded the code required to make and save the notes to the board. I also set up the serial communication there and uploaded it to the board.
int speakerPin = 0;
char c;
int ton =0;
int tones[] = { 1136, 1014, 1915, 1700, 1519, 1432, 1275 };
int tempo = 300;
void setup() {
Serial.swap(1);
Serial.begin(9600);
pinMode(speakerPin, OUTPUT);
}
void loop() {
while (Serial.available() > 0) {
c= Serial.read();
ton = c-96;
// Serial.println(ton);
// Serial.println(c);
playTone(tones[ton-1], tempo);
}
}
void playTone(int tone, int duration) {
for (long i = 0; i < duration * 1000L; i += tone * 2) {
digitalWrite(speakerPin, HIGH);
delayMicroseconds(tone);
digitalWrite(speakerPin, LOW);
delayMicroseconds(tone);
}
}
I then put both together and ran the program. I opened the output window, and it wa working exactly how i wanted!
VOILA! That would be the end of this week's individual assignment
Files
You can download the ino file of it from here
You can download the pde file of it from here