Weekly Summary
This weeks lecture by Neil gave a near-exhaustive tour of programming languages and interface software. This can be a purely software week, but as we are highly encouraged to make a new board every week, I will continue making my ESP32 dev board from last week.
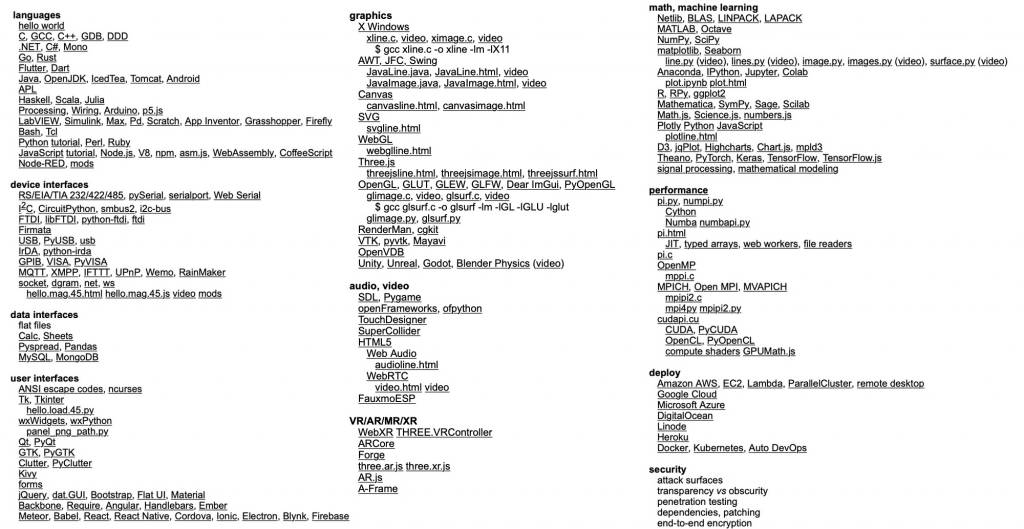
Assignments
Group Assignments
This weeks group assignment is to compare as many tool options as possible. I think we need to limit this somehow, as the full scope of the assignment is to compare all the tool options Neil mentioned in his lecture. For my part of the group assignment, I will make a comparison of browser-based Javascript UI tools, here is the line-up:
- Pure JS
- jQuery
- React, Redux, ReactRouter
- Vue, Vuex, Vue Router
- D3.js
- Firebase
For more details, see the Group Assignment Page.
Individual Assignment
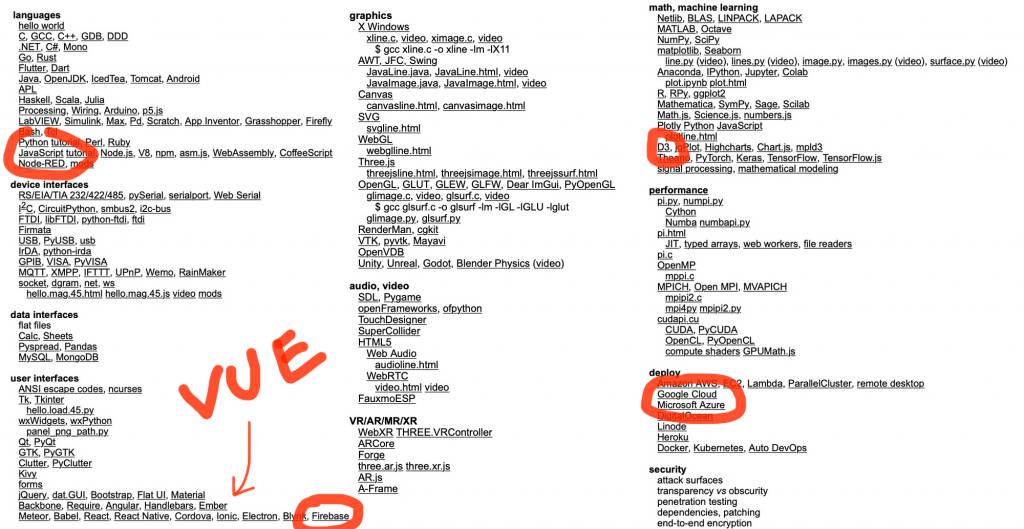
My individual assigment, write an application that interfaces a user with an input &/or output device that you made or interface a user with an input and/or output device.
- Build a UI
- Visualise it
- Extra Credit: Sonify it
- Extra-extra Credit: 3D it
- Extra-extra-extra Credit: VR/AR it
I am leaving 3D, VR, AR out of it, as they have no place in information visualizing, unless the source data is in 3D. Otherwise stick to 2D.
Assignment Work Plan
-
Design, make, mill, stuff, test a Dev Board
-
Connect I2C Temp Sensor and ToF Sensor
-
Sync/Broadcast data with MQTT and collect/distribute with Firebase Realtime Database
-
Friday: Design my version of the DevBoard
-
Saturday: Lab Day. Mill & Stuff the Dev Board
-
Sunday: Programming and Hardware Debugging
-
Tuesday: FirebaseVue & D3
-
Wednesday: More Firebase, Vue & D3, Final Updates
The Group Assignment is to compare as many tool options as possible, and it feed into the individual assignment of making an I/O UI App. As Neil basically went through the whole history of computing in his lecture, comparing the tools could be a life-long endeavour.
I will focus on concepts and languages that A, interest me, and B, are feasibile to finish within the allocated time-frame.
A: Interesting...
- Haskell
- Erlang
- MaxMSP ("Max"), Pure Data, ("Pd")
- TouchDesigner
- Google Firebase
- MQTT
- TensorFlow, TensorFlow.js
- D3.js
- Vue, React, Svelte
B: Will use in Final Project
- Syncing Data from ESP32 to Cloud
- Visualising Data in the Browser
C = A ∪ B
- Google Firebase,
- MQTT?
- Vue
- D3.js
Update from this Week's Global Open Time
- Comparing only software is not really in the spirit of the group assignment.
- Also connect a board and compare software tools to create different UI and UI approaches to it. (See Adrian Torres' 2020 Site)
Comparing in-browers update and management of data
In the Bronze Age of JS, shortly after the Stone Age of mutual incomprehensible JS dialects, jQuery became the too in interact programmatically with the DOM and ferry data between the browser and the server. AJAX and jQuery were a bit step forward, one problem that emergent was that of keeping data in sync - not only within the browser, but also within and across servers.
While it is possible it jQuery, anyone who ever tries it, will not try it ever again. React (which was interestingly modelled after PHP as a sort of templating tool) started to solve this, and with the introduction of stores like Flux and Redux an ecosystem started to develop.
Vue is a more/less opinionated implementation of the same idea, without the idealogical baggage of Facebook and without the non-elegance of yet another templating language that is JSX. JSX is used for writing HTML in JS. If you want to do that, React is for you, if you want to keep your HTML and JS nicely and cleanly separated, have a look at Vue. Svelte is made for speeed, unlike React and Vue it manipulated the DOM directly.
D3 in an interesting outlines, while mainly used for data visualization, D3 actually stands for Data Driven Documents, the power - and the most difficult part of D3 to get your head around is the General Update Pattern.
Google Firebase
What is Firebase? What is it used for?
Firebase is a real-time database that solves the problem of sharing and updating data across devices.
https://console.firebase.google.com/
I am following the Getting Started for Web Tutorial to set up a test installation of Firebase. They also have Quick Start Samples to get up and running, I am choosing the Quickstart for Realtime Database for JS to get started.
The Quickstart Repo has 23 examples for authentication (another key feature of Firebase) and one example for a Realtime Database
MQTT
What is MQTT? What is it used for? And how does it differ from Firebase?
MQTT is a open-source aggregator that allows for the publishing/subscription of data. Usually it is run on a local server, to collect data from sensor and make it available and consumable.
Firebase vs MQTT
Firebase and MQTT serve similar but different purposes, Firebase grew from being a real-time database into an eco-system with additional support of authentication, cloud storage, simple hosting, cloud function and machine learning.
Firebase starts as a free tier, but depending on the amount of connections and the success of your app, a fee needs to be paid.
The choice of Firebase or MQTT depends on the scale and the scope of your project. Ideally a ESP32 with Firebase and connected via WiFi would do the trick.
Installing Vue
Vue.js bills itself as a progressive, approachable, versatile and performant JS frame work.
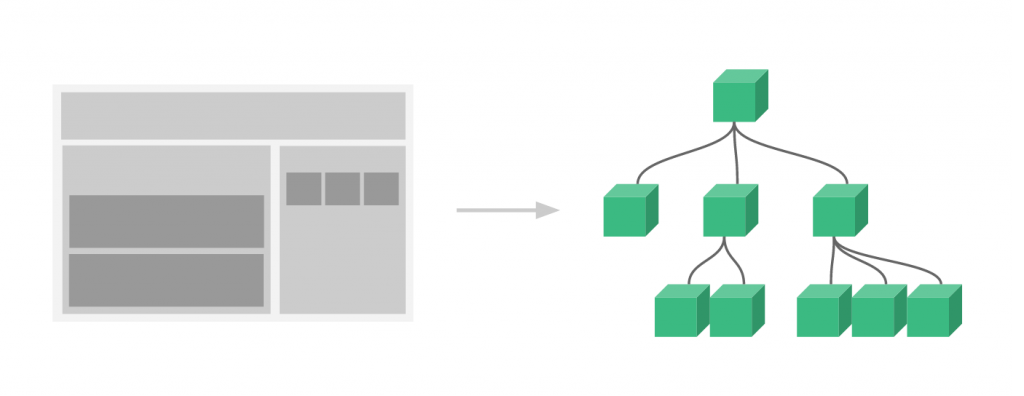
Getting the Vue CLI
Install tooling for Vue JS
npm install -g @vue/cli
Checking the installed version:
vue --version
@vue/cli 4.5.13
I choose to make a rather minimal Vue Project, without Routing (vue-router) but with a global store (vuex).
vue create georg-fab21-ui
Vue CLI v4.5.13
? Please pick a preset: (Use arrow keys)
❯ Default ([Vue 2] babel, eslint)
Default (Vue 3) ([Vue 3] babel, eslint)
Manually select features
Choose the default Vue 2 settings, prepares an empty project.
cd georg-fab21-ui
npm run serve
DONE Compiled successfully in 2136ms 4:20:32 PM
App running at:
- Local: http://localhost:8080/
- Network: http://10.0.1.15:8080/
Note that the development build is not optimized.
To create a production build, run npm run build.
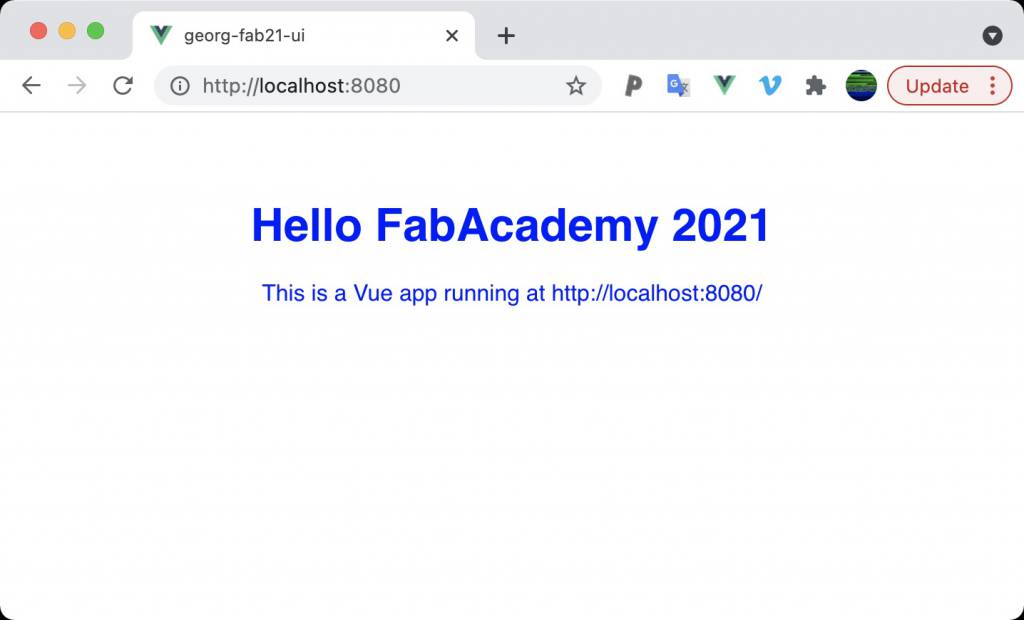
Adding Vuex and Firebase
Adding vuex, vuefire, vuex-firebase bindings and firebase
npm install vuex vuexfire vuefire firebase --save
The dependencies
in the package.json
now look like this:
"dependencies": {
"core-js": "^3.6.5",
"firebase": "^8.5.0",
"vue": "^2.6.11",
"vuefire": "^2.2.5",
"vuex": "^3.6.2",
"vuexfire": "^3.2.5"
},
A Simple App
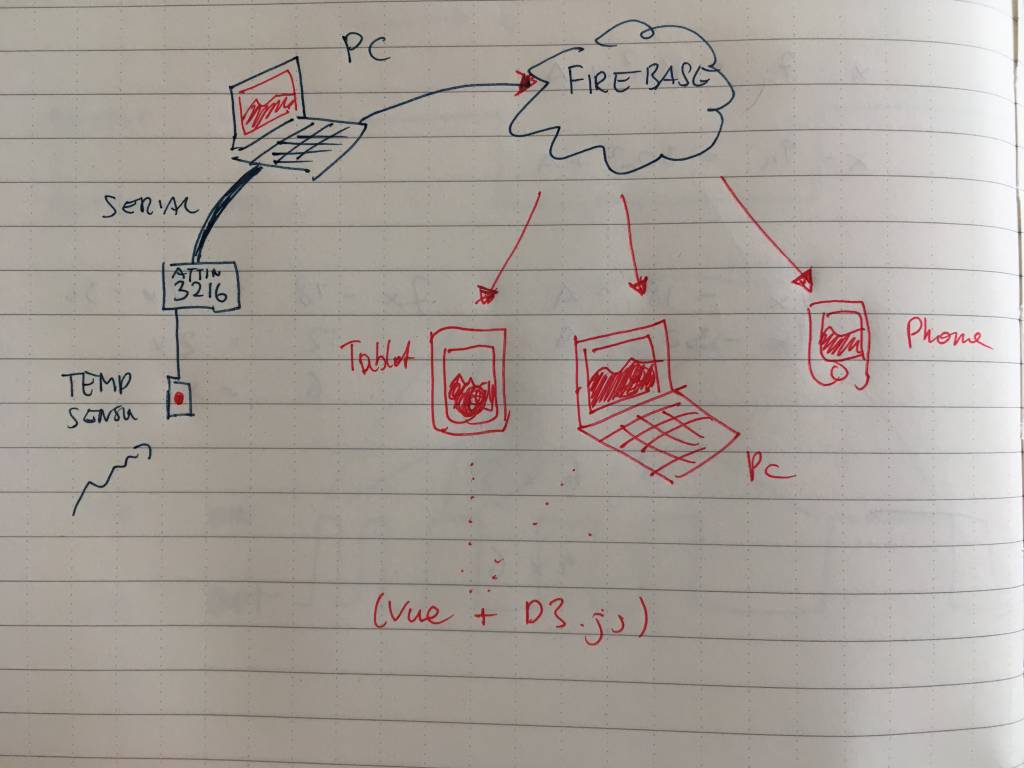
For testing, I made a simple app that shows the temperature - and not much else. Behind the scenes, the temp field is connected to the vuex store, being kept in sync across the app.
Next step: keeping in-sync across browsers and networks.
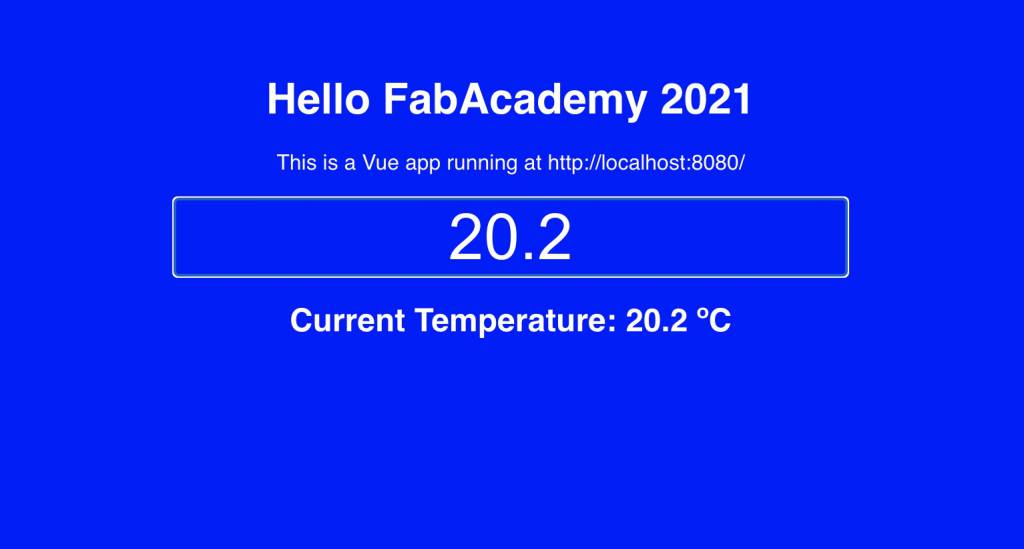
Realtime DB, Cloud Firestore or Cloud Messaging?
The Realtime DB and Cloud Firestore are slightly different solutions for the same problem. I looks like the Cloud Firestore is an updated, more feature-rich version of the Realtime DB. A comparision between the Realtime DB and Cloud Firestore. And more details about Cloud Messaging*Thank you, Homma-san for the suggestion..
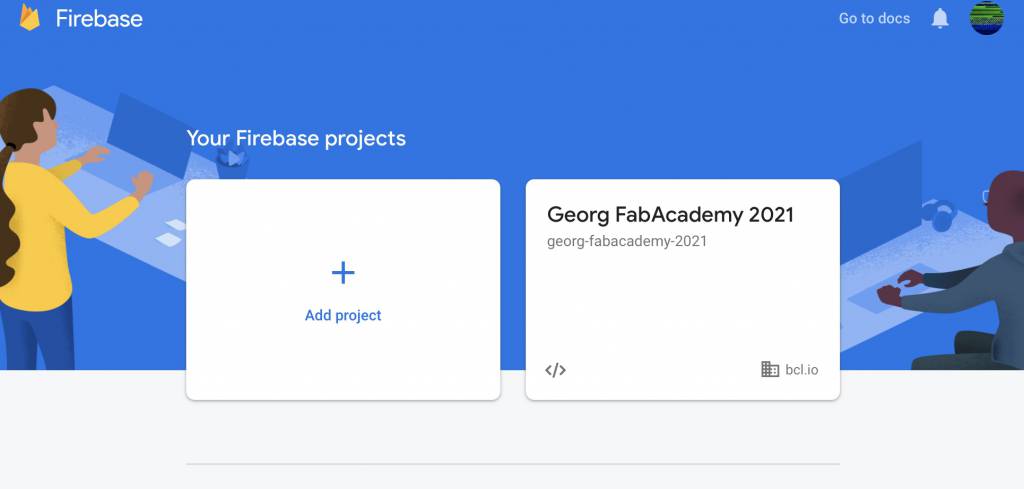
Connecting to the Firebase Realtime Database
Route
- i2C Temp & OLED
- ATTiny
- WebSerial
- Browser A: send to Firebase
- Browser B: receive with Firebase, show with Vue & D3
UI Demo
Temp & D3
I created a UI Application, visualizing the data generated from my ATTiny 3216 board and the Temperature Breakout Board. This UI Demo is a simple time-series display of the temperature data.
- Y is temperature
- X is time
I added an interactive overview track, that allows the user to zoom+focus on certain regions of interest. The focus area is expandable and moveable by dragging, the detailed view of the temperature data will update accordingly.
Live UI Demo: D3 Temperature Demo
Video from UI Demo:
Firecloud
Syncing the temperature data across browsers.
Live UI Demo: Firecloud Temperature Demo
Video from UI Demo:
Conclusion
Vue, D3 and Firebase all have a slightly steep learning curve, but they can be made to work nicely together. D3 provides the Visualisation Components and APIs, The Vue Ecosystems handles App complexity, and Firebase syncs data effortlessly. The next steps are to integrate the system with a ESP32-based board, making use of the built-in WiFi communication abilities of the chip.
Repository
The code for the Firebase+Vue+D3 App can be found in the FabCloud:
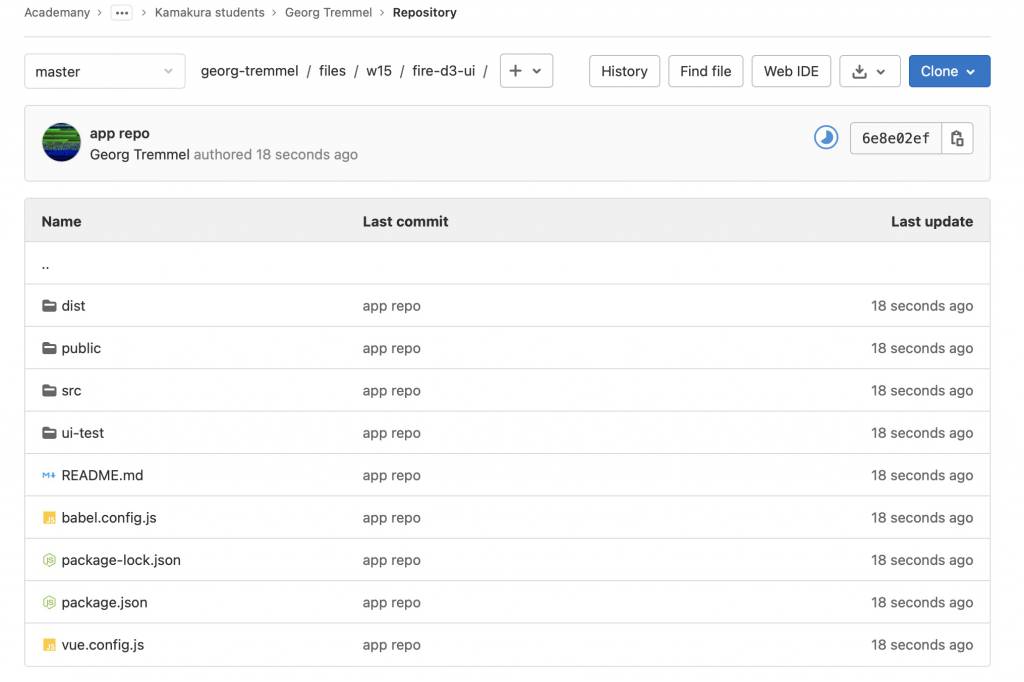