Interface and Application programming
Assignment:Write an application that interfaces with an input &/or output device.
For this week assignment its need to write a source code to interface input and output devices. Term interface is used inside computing to exchange information wheather it will analog or digital signals.Here I did Interfaced hello world board through arduino and controlled with mouse click.
Interface envirnoment:
For interfacing I used processing envirnoment along with arduino and hello world board.Processing is programming language having support with visual context.Using this envirnoment interfaced arduino and hello world board and operated LED bulb with mouse click.
Serial interface with Processing:
To interface arduino board with computer I used processing tool in that under sketch imported library 'serial'for serial interface. Defined port as 'my port'which shows used port.Defined window for mouse click and boud rate 9600 for communication.Inside draw defined high value when mouse is pressed and low when unpressed.
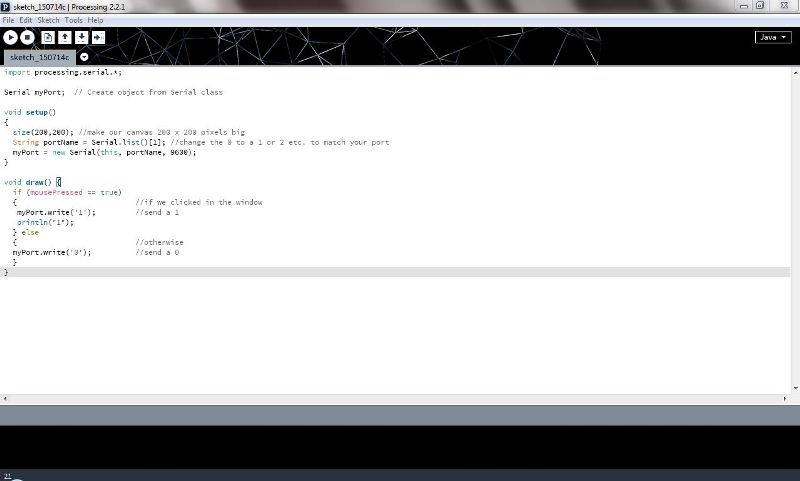
Interface programm and description:
import processing.serial.*;
Serial myPort; // Create object from Serial class
void setup()
{
size(200,200); //make our canvas 200 x 200 pixels big
String portName = Serial.list()[1]; //change the 0 to a 1 or 2 etc. to match your port
myPort = new Serial(this, portName, 9600);
}
void draw()
{
if (mousePressed == true)
{
//if we clicked in the window
myPort.write('1'); //send a 1
println("1");
}
else
{
//otherwise
myPort.write('0'); //send a 0
}
}
----------------------------------------------------------------------------------------------------------------
Arduino interface:
For arduino interfacing defined first variable to receive serial data and output pin 7 to send data to hellom world board.It will send data on this pin when serial data is available.
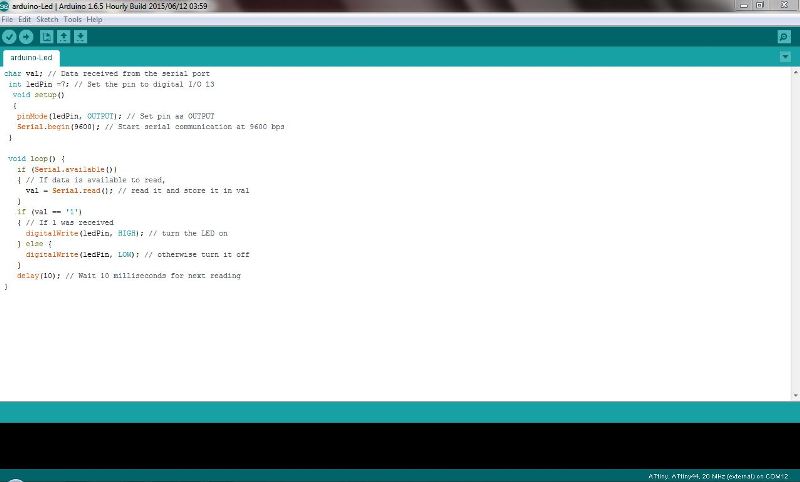
char val; // Data received from the serial port
int ledPin =7; // Set the pin to digital I/O 13
void setup()
{
pinMode(ledPin, OUTPUT); // Set pin as OUTPUT
Serial.begin(9600); // Start serial communication at 9600 bps
}
void loop()
{
if (Serial.available())
{
// If data is available to read,
val = Serial.read(); // read it and store it in val
}
if (val == '1')
{
// If 1 was received
digitalWrite(ledPin, HIGH); // turn the LED on
}
else
{
digitalWrite(ledPin, LOW); // otherwise turn it off
}
delay(10); // Wait 10 milliseconds for next reading
}
-----------------------------------------------------------------------------------------------------------
Hello world board:
Connected this hello world board to arduino with VCC ,GND, and DATA_in pin at PA0.Connected and defined PA3 for LED output .It glows at every mouse click.
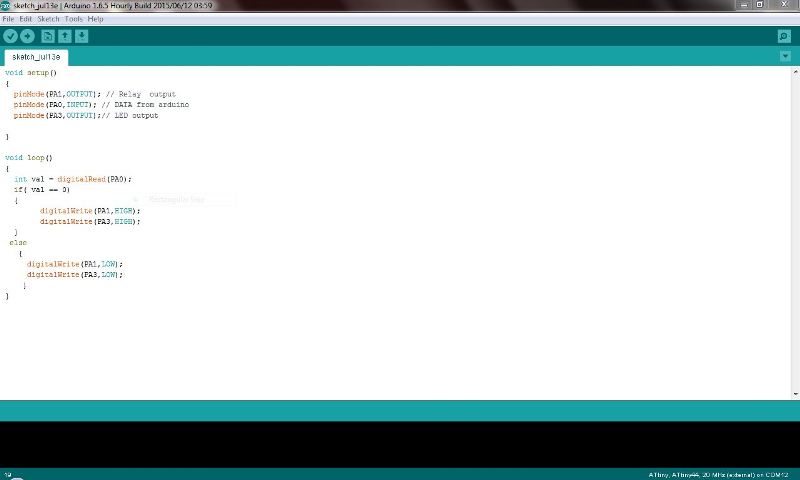
void setup()
{
pinMode(PA1,OUTPUT); // Relay output
pinMode(PA0,INPUT); // DATA from arduino
pinMode(PA3,OUTPUT);// LED output
}
void loop()
{
int val = digitalRead(PA0);
if( val == 0)
{
digitalWrite(PA1,HIGH);
digitalWrite(PA3,HIGH);
}
else
{
digitalWrite(PA1,LOW);
digitalWrite(PA3,LOW);
}
}
-------------------------------------------------------------------------------------
Setup and opeartion:
connected arduino board with computer through serial interface. Hello world is connected to arduino with VCC,GND and DATA_IN pin to see operation.
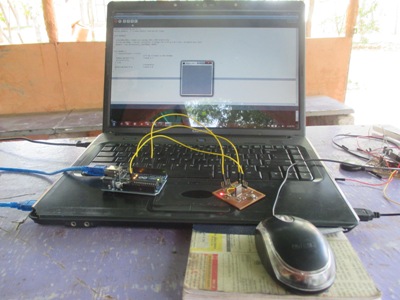
Go through following operational video :
Interface and applications operational video
Download files from here:
Interface source code files