Week 15 | Networking and Commnications
Fab Academy 2018 | Archive
SInce my final project has integration of 6 servo motors, I decided to make 6 slave boards and one master board in this networking and communication week.
Initially i made this master board having AtTiny 44 on it. My slave boards had AtTiny 45 on it. // design of original Master board.
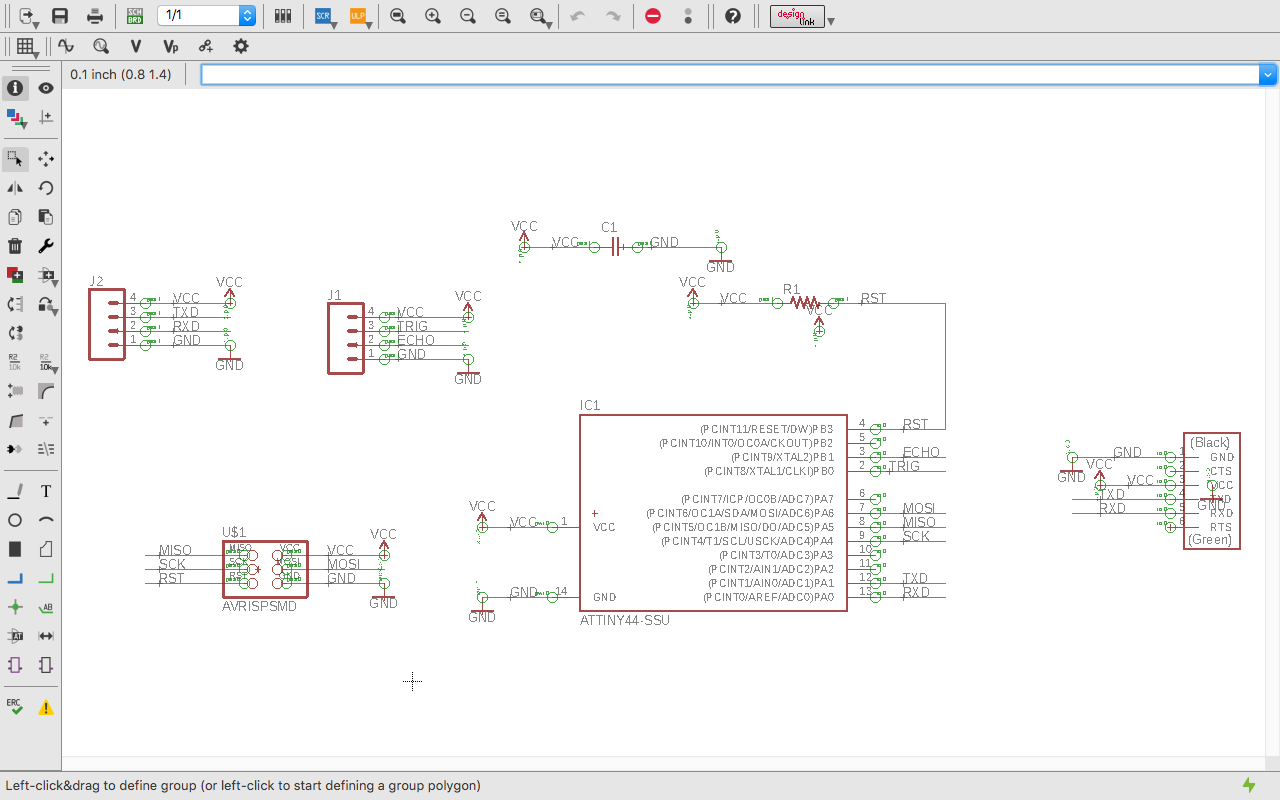
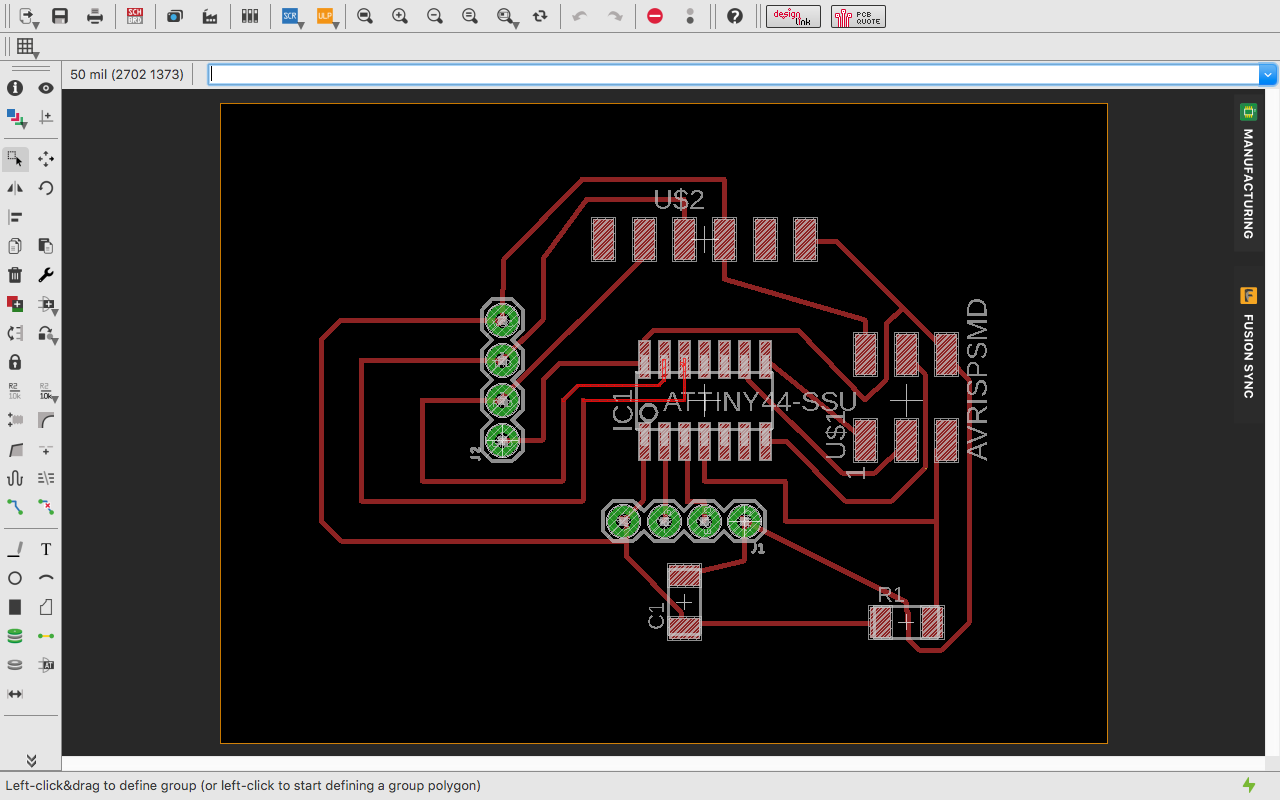
// design of Slave boards.
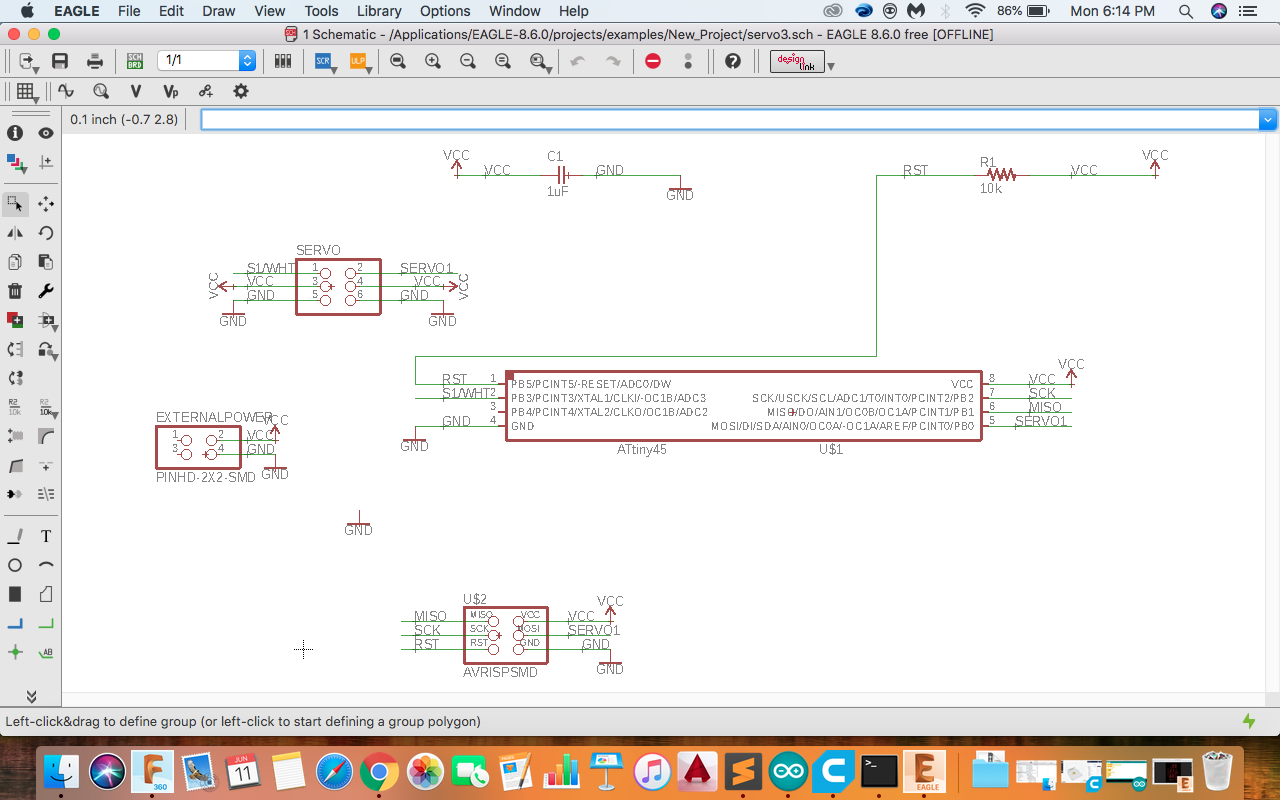
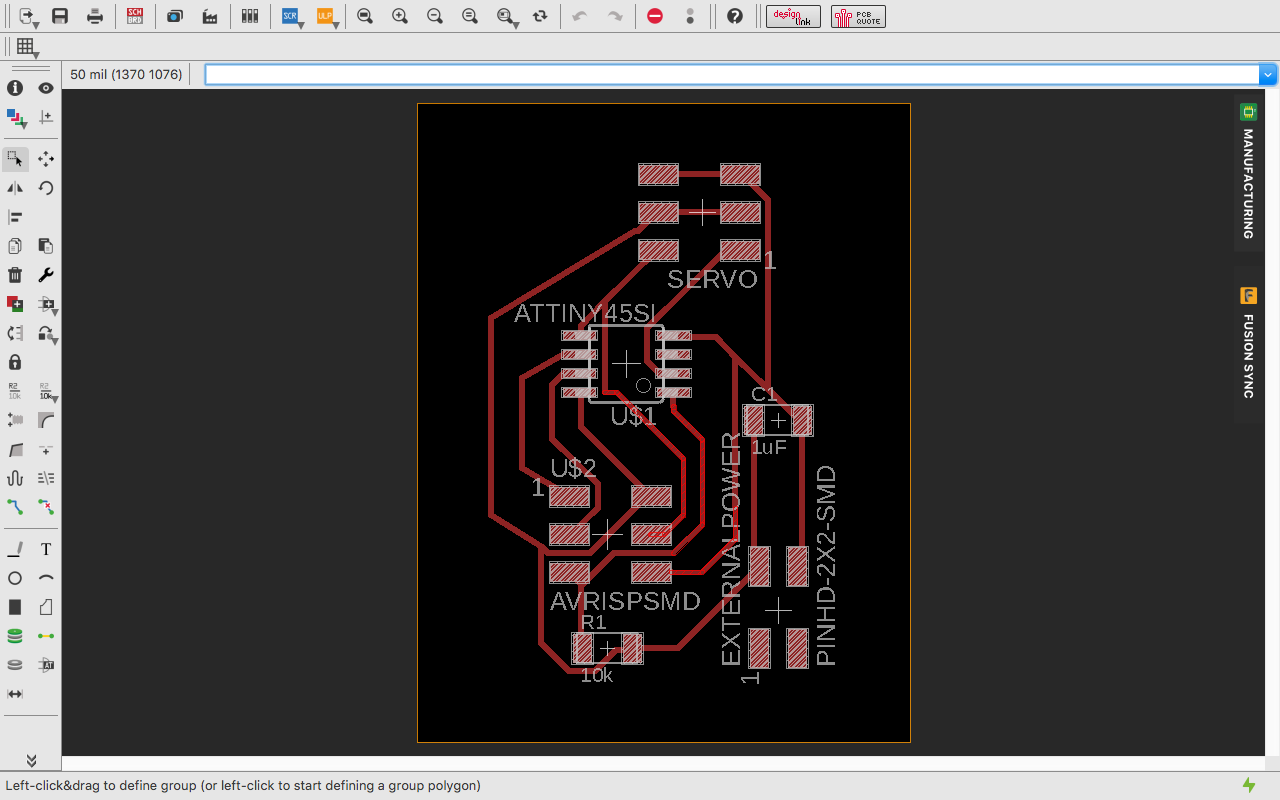
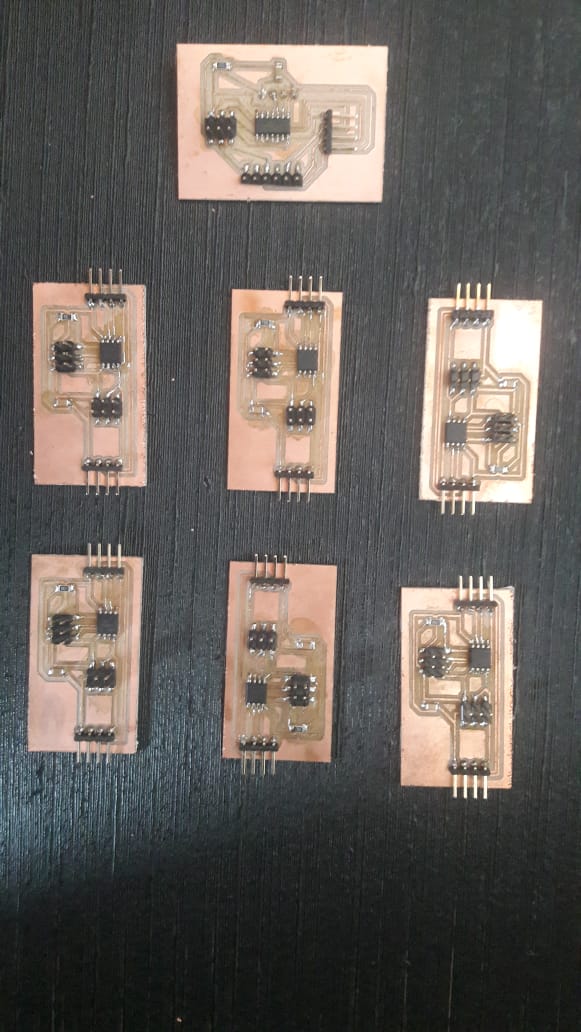
Master board had two pin outs for communicating with ultrasonic sensor with echo and trig pin.
One Issue came when, i programmed master board to communicate with slaves via Software Serial. There was some power decay somewhere which is causing low signals passed through software serial to slave boards. So slave boards were not able to read data from serial.
(Surprisingly I tested Master output with arduino via sending Serial data to arduino, and arduino was able to understand the software signals. Also If we keep arduino as master, Signals sent from arduino were successfully be catched by slave boards. So there was some mistake which i was not able to figure out.)
So to get out from this frustration, I changed my plan of ultrasonic, and milled another board which had similar design as slave board ad had AtTiny 45 on it, I called it new master.
As you can see in the following image, I connected the new master board to 3 slave boards.
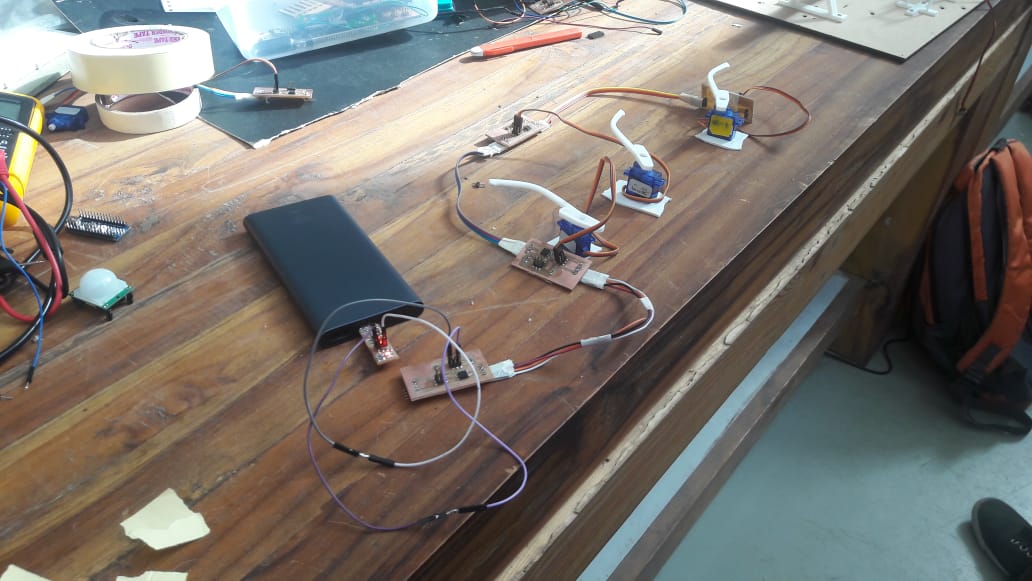
I assigned each slave board an alphabet starting from A. I programmed each slave board to listen character of its own alphabet (both capital and small, indicating which direction the servo should rotate).
Following is the code of slave board.
#include
int rxPin = 3;
int txPin = 4;
char chr;
SoftwareSerial myserial(rxPin, txPin);
void setup()
{
pinMode(rxPin,INPUT);
pinMode(txPin,OUTPUT);
pinMode(0, OUTPUT);
myserial.begin(9600);
}
void loop()
{
char chr = myserial.read();
if (chr == 'f') {
for (int i = 0; i < 50; ++i) {
digitalWrite(0, HIGH);
delayMicroseconds(2000);
digitalWrite(0, LOW);
delayMicroseconds(18000);
}
}
else if(chr == 'F') {
for (int i = 0; i < 50; ++i) {
digitalWrite(0, HIGH);
delayMicroseconds(1000);
digitalWrite(0, LOW);
delayMicroseconds(19000);
}
}
}
The new master board was programmed to send alphabets as test signals to the connected slave boards via software serial communication. Whichever slave has ability to read that alphabet will move the servo in one direction and then to the reverse.
You can understand the static signals I passed from following code.
#include
SoftwareSerial ms(4,3); // rx , tx
void setup()
{
pinMode(4,INPUT);
pinMode(3,OUTPUT);
pinMode(0,INPUT);
pinMode(1,INPUT);
ms.begin(9600);
}
void loop()
{
ms.write('b');
ms.write('B');
delay(2000);
ms.write('c');
ms.write('C');
delay(2000);
ms.write('d');
ms.write('D');
delay(2000);
}
Here is the full working video.
Updated Code
I made corrections as guided by my Global Evaluator.Here in this code the slave also interacts with master board. Here the master board waits for the response of the board. When the first board responds, then the led blinks once and when the second board sends response the led will blink twice. The response from the slave board indicates that the motor has moved.
Code for master
#include <SoftwareSerial.h>
SoftwareSerial ms(4,3); // rx , tx
void setup(){
pinMode(4,INPUT);
pinMode(3,OUTPUT);
pinMode(2,OUTPUT);
ms.begin(9600);
}
//function to blink LED n number of times
void BlinkTimes(int n){
for( int p = 0; p < n; p++){
digitalWrite( 2, HIGH);
delay(300);
digitalWrite( 2, LOW);
delay(150);
}
}
//function which waits for slave's acknowledgement.
void waitForAck(){
//wait until we get ack from slave that motor has finished Moving
while( !ms.available() );
char ack = ms.read();
if( ack == '1' ){
//slave 1 replied, Blink 1 time
BlinkTimes( 1 );
}
else if( ack == '2' ){
//slave 2 replied, Blink 2 times
BlinkTimes( 2 );
}
}
void loop()
{
ms.write('c');
ms.write('C');
//wait for ack form slve
waitForAck();
delay(3000);
ms.write('x');
ms.write('X');
//wait for ack form slve
waitForAck();
delay(3000);
}
Code for slave
#include
int rxPin = 3;
int txPin = 4;
char chr;
SoftwareSerial myserial(rxPin, txPin);
void setup()
{
pinMode(rxPin,INPUT);
pinMode(txPin,OUTPUT);
pinMode(0, OUTPUT);
myserial.begin(9600);
}
void loop()
{
char chr = myserial.read();
if (chr == 'y') {
for (int i = 0; i < 50; ++i) {
digitalWrite(0, HIGH);
delayMicroseconds(2000);
digitalWrite(0, LOW);
delayMicroseconds(18000);
}
}
else if(chr == 'Y') {
for (int i = 0; i < 50; ++i) {
digitalWrite(0, HIGH);
delayMicroseconds(1000);
digitalWrite(0, LOW);
delayMicroseconds(19000);
}
// send ack to master that "back to zero" is called
// indicating motor has moved in both direction
myserial.write('3');
// Sending 1 will be reflacted as blink LED in master 1 times
}
}