Embedded Programming
- Group: Compare the performance and development workflows for other architectures.
- Individual assignment: Read a microcontroller data sheet.
- Individual assignment: Program your board to do something, with as many different programming languages and programming environments as possible.
Group Assignment
Detail of each student work can be found at the link.
- Iván Callupe
- Carlos Nina
- Diego Santa Cruz
Microcontroller
Microcontroller is a integrated group of a microprocessor, memory, IO (Input/Output) and other peripherals on one single chip. They come with different data bus sizes: 4, 8, 16 and 32 bits.
Architectures Classification
Memory classification:
-
Von-Neuman Architecture: program and data are in the same memory, instructions and data are fetched over the same data bus. Two fetches are needed to execute an instruction (one to get the instruction, one to get (or put) the data).
-
Harvard Architecture: program and data are in separate memories, data bus and instruction bus are separate, can be operated at the same time. Next instruction is fetched while current instruction operates on the data bus. This pre-fetching speeds up processing by a factor of two (except when branching).
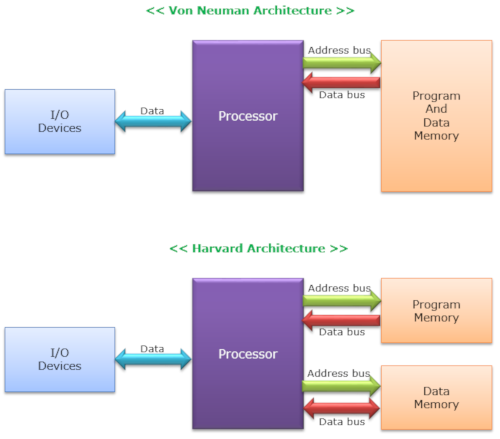
Memory classification:
-
CISC: Complex Instruction Set Computer - often have over 80 instructions in the instruction set.
-
Reduced Instruction Set Computer - Fewer instructions, better performance, smaller chip, lower power consumption.
Popular Microcontrollers
Common MCUs include the Intel MCS-51, often referred to as an 8051 microcontroller, which was first developed in 1985; the AVR microcontroller developed by Atmel in 1996; the programmable interface controller (PIC) from Microchip Technology; and various licensed ARM microcontrollers. A number of companies manufacture and sell microcontrollers, including NXP Semiconductor, Renesas Electronics, Silicon Labs and Texas Instruments.
Individual Assignment
In this assigment I will be using the Electronics Design week board I did. This is an attiny45 micro from AVR. I found the micro datasheet at this link.
Reading a micro might be a long and messy task. I used Sparkfun Electronics guide "How to read a Datasheet" (link here), which summaries the most important stuff when not having experience with electronics.
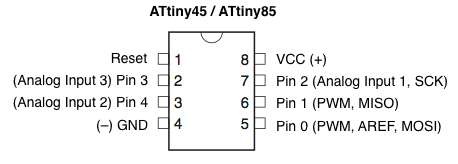
According to the guide, I will be looking for: summary, specifications, pinout, absolute ratings, recommended operation conditions and example schematics.
Attiny 45 it's a 8 pin microcontroller. Taking out VCC and GROUND pins, the micro have 6 I/O pins from which RESET is usually left alone. Works with 2.7 to 5.5 Volts.
It does not have hardware serial comm, which might be solved with a software based one. Could be hard coded directly or "SoftwareSerial" library could be used.
The micro have 4K storage, meaning it have really short space. The lighter the code, the better.
Arduino
I will be coding to the Week7 board with Arduino languaje and C to blink the LED on Pin 2. To program the micro, an arduino as ISP will be used.
Using the Arduino IDE, we load the blink example. From "File" menu >> Examples >> Basics >> Blink. This code needs to be prepared to match the board pinout.
Week 7 board have it's LED on Pin2, so I need to change the code from LED_BUILTIN to pin 2. I created a variable to storage the pin number and labeled "ledpin". The last part of the code is the standard blink part which turns light on/off with digitalWrite() function.
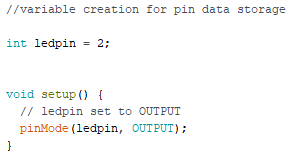
Now I load it to the board and see the code takes 942 bytes of memory from the 4096 bytes available (22%).
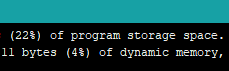
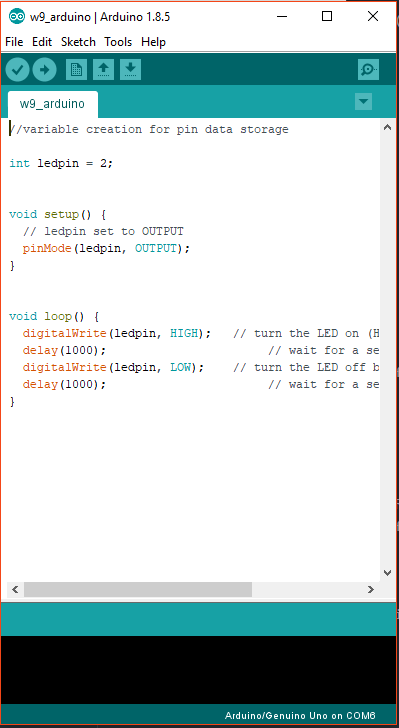
C Language
Arduino language is just a variation of C++. Programming with C directly is similar but looks weird for someone who does not know about registers.
Using Arduino IDE, the first part of the code is to set CPU speed. Attiny45 runs usually at 8MHz, so speed is defined as a whole number. Also it is important to include avr library since we are working with an attiny which is an avr based microcontroller.
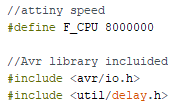
The main function (void function which loops infinitely) contains the code to set all PORTB pins. PORTB stands as a "group" name for those pins. Attiny45 "PB" pins are physical pin 1 (PB5), 2 (PB3), 3 (PB4), 5 (PB0), 6 (PB1) and 7 (PB2). Physical pins 4 and 8 are for GROUND and VCC.
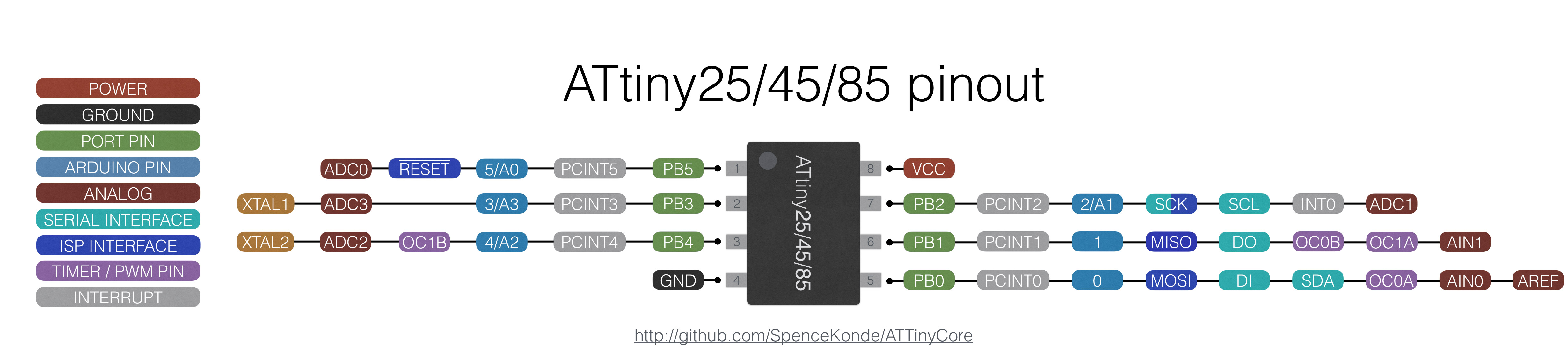
A "For" function with no condition runs the snippet with toggle function for light on/off the LED while delaying 1 second between actions.
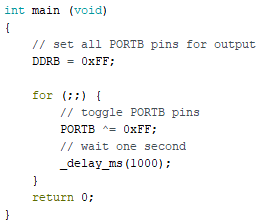
Finally when loading the code, an amazing low memory usage shows why using c is efficient.

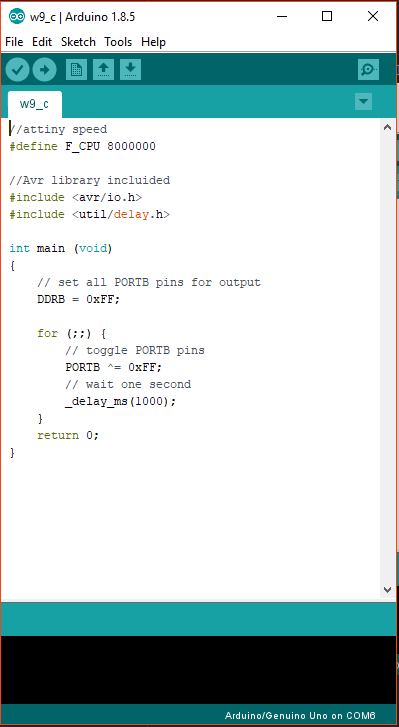
Check my week 7 work to get the Arduino code for this: