Objectives
Individual assignment: write an application that interfaces a user with an input &/or output device that you made
Group assignment: compare as many tool options as possible
Group assignment
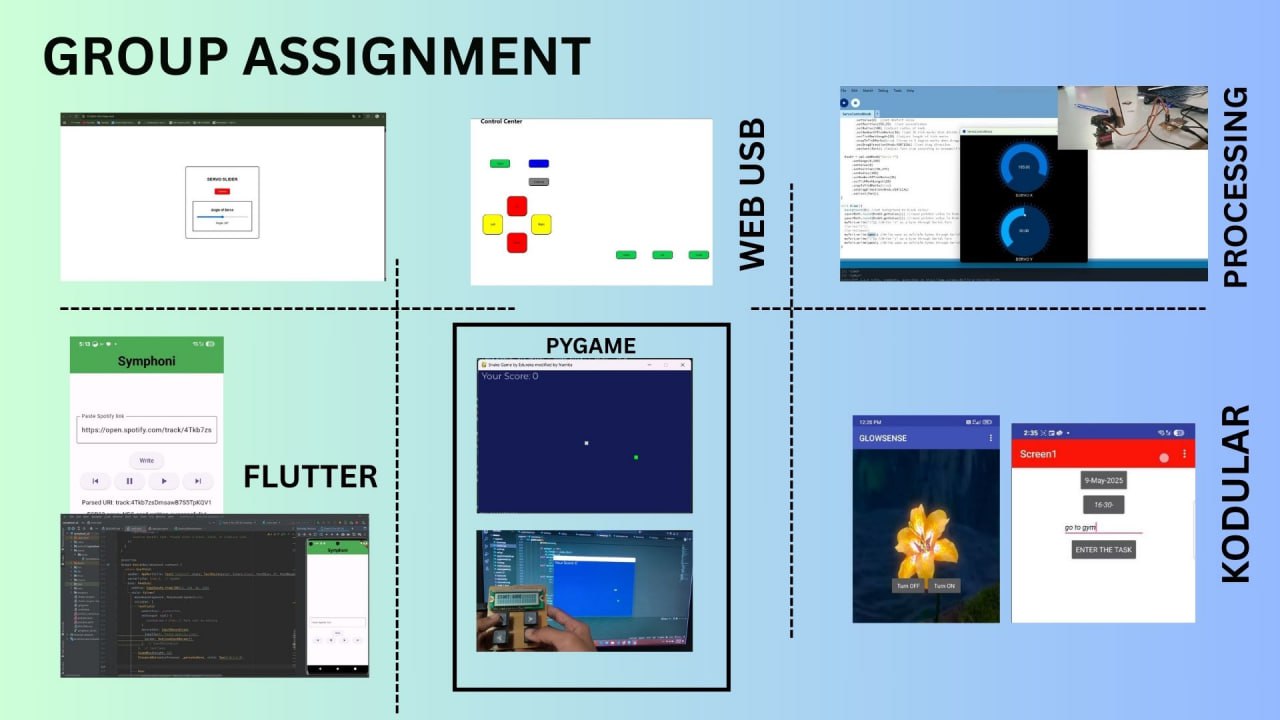
14. Interface and application programming
UI and its types
A User Interface (UI) is the medium through which a user interacts with a device, software application, or system. It includes all the controls and visual elements that allow users to:
- Input commands
- Manipulate or retrieve data
- Receive feedback
Graphical User Interface (GUI): Uses visual components like windows, icons, buttons, and menus. Users interact via mouse, keyboard, touchscreen, etc.
Command-Line Interface (CLI): A text-based interface where users type commands. Efficient for experienced users, especially in system administration and development.
Touchscreen Interface: Found in phones, tablets, kiosks, and more. Enables intuitive interaction through gestures like tapping, swiping, and pinching.
Voice User Interface (VUI) Allows users to interact using spoken commands. Examples: Siri, Alexa, Google Assistant.
Virtual and Augmented Reality Interfaces (VR/AR)
- VR: Immerses users in a fully digital environment.
- AR: Overlays digital content onto the real world. Interaction happens through headsets, hand-tracking, or controllers.
UI for interactive website
As a part of explaining interface, I tried the codes provided by the instructor.
1.Button
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Button</title> </head> <body> button style="margin: auto;" id="press">Click me/button> <p id="para"> <script> const button = document.getElementById('press'); const para = document.getElementById('para'); button.addEventListener('click' , function(e){ para.textContent = para.textContent + 'Clicked'; document.getElementById('para').style.color = 'red' button.style.backgroundColor = button.style.backgroundColor === 'red' ? 'gray' : 'red'; });
2.Realtimeinput
<input type="text" id="myInput" name="myInput"> <div id="output"></div> <script> const inputElement = document.getElementById('myInput'); const outputElement = document.getElementById('output'); inputElement.addEventListener('input', function(event) { const inputValue = event.target.value; outputElement.textContent = 'My name is ' + inputValue; //const input = (e.target.Value).split(""); if (inputValue.length>8){ outputElement.style.color = 'red' }else if(inputValue.length>4){ outputElement.style.color = 'blue'; }else{ outputElement.style.color = 'green' } }); </script>
KODULAR
Kodular is a free, drag-and-drop platform that enables users to create Android applications without writing traditional code. It features a visual interface where users can design apps by assembling components like buttons, sensors, and layouts, and it employs a block-based programming system based on Googleβs Blockly, similar to MIT App Inventor and Scratch. This approach allows beginners and non-programmers to build functional Android apps using visual logic blocks instead of text-based code. While Kodular itself operates through these visual blocks, the apps created with it are ultimately compiled into Java code for Android. To facilitate real-time testing and debugging, Kodular provides a companion app called Kodular Companion. This app allows developers to instantly preview and interact with their projects on an Android device without the need to compile and install the APK each time. By scanning a QR code or entering a code generated in the Kodular creator interface, users can connect their device to the project and see live updates as they modify their app, making the development process more efficient and user-friendly.
To use kodular, I signed in to kodular. I didn't had an idea about how to use Kodular. So I referred Fabacadamey 2024 student Ansu Thomas's documentation.
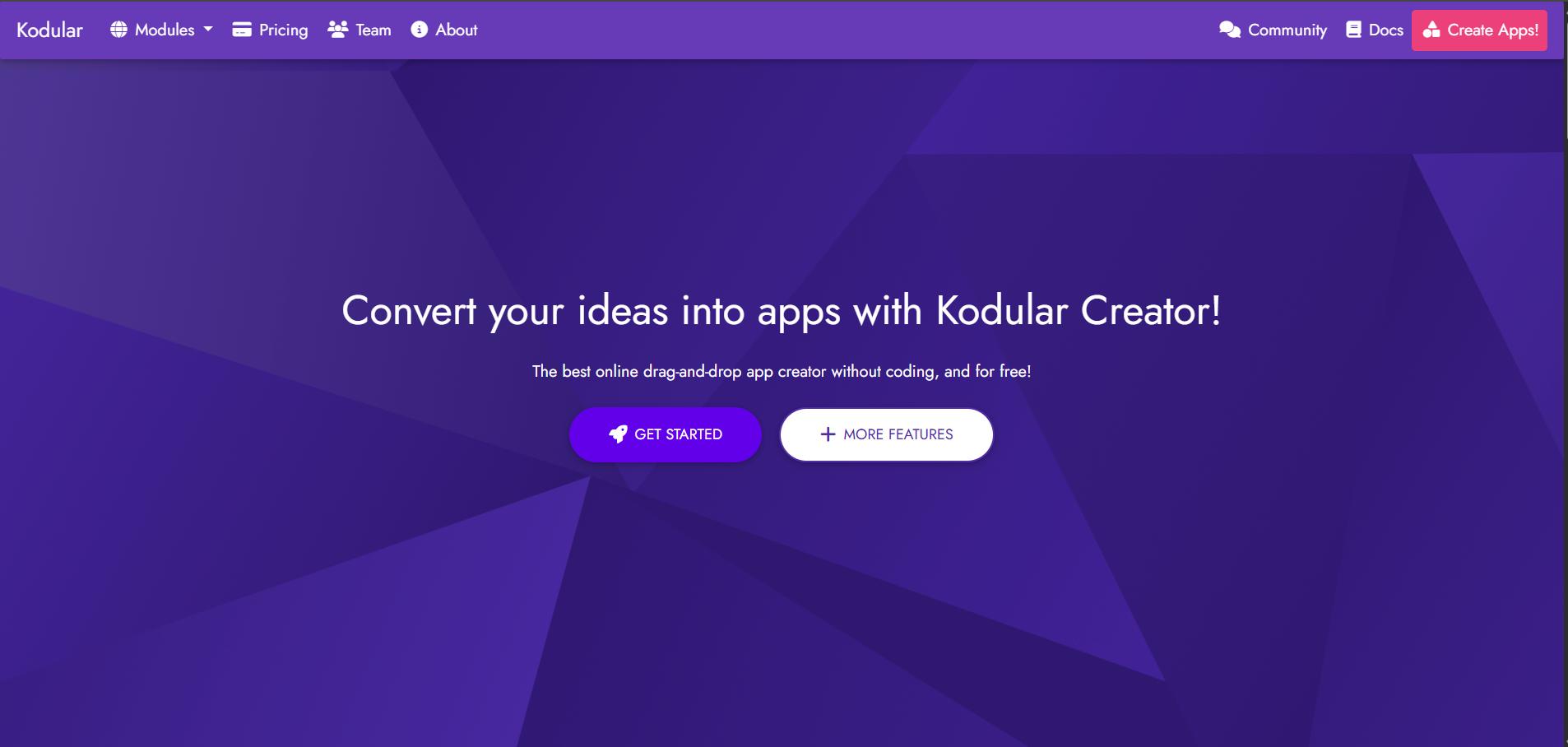
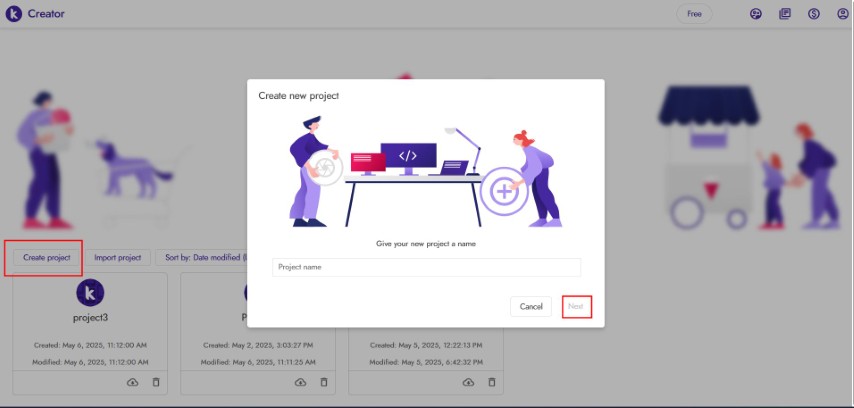
After creating project a new window opens. Kodular Creator has two main views:
- Designer: Drag and drop components (buttons, labels, sensors, etc.).
- Blocks: Create logic using visual blocks (like Scratch or Blockly).
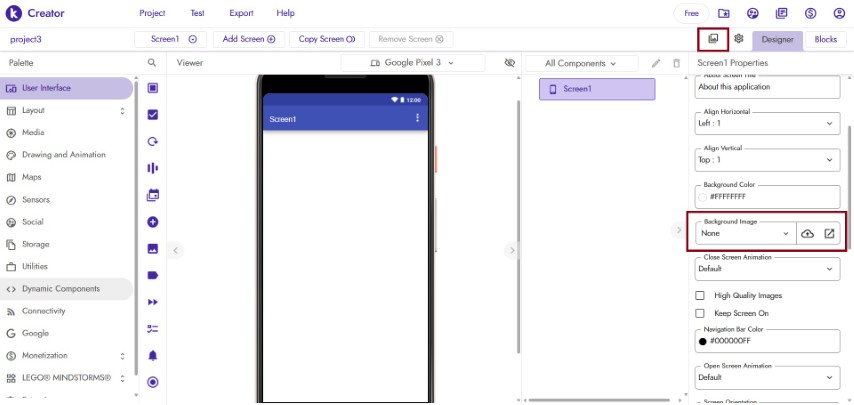
I wanted to add a background image for my app. So i downloaded an, image . From the image icon The image is uploded. The changes were made in the background image option.
I dragged the horizontal arrangement from the layout. From properties panel I aligned it in the center. Then I draged button From user interface, and placed it inside the horizontal arrangement.I named button 1 as "Turn on" and button 2 as "turn off". I also draged Web from connectivity. Components like Buttons, Labels, Text Boxes etc..can be dragged from the Palette to the Viewer. Non-visible components like Bluetooth, Sensors, or Notifiers are avilable in the bottom of the palette. We can customize properties (text, color, size, etc.) using the Properties panel.
Then I switched to blocks tab. The block tab consists of event blocks, in the left panel. Drag event blocks and add logic.I copied the IP address Of XIAO board from the code and pasted it in the block.
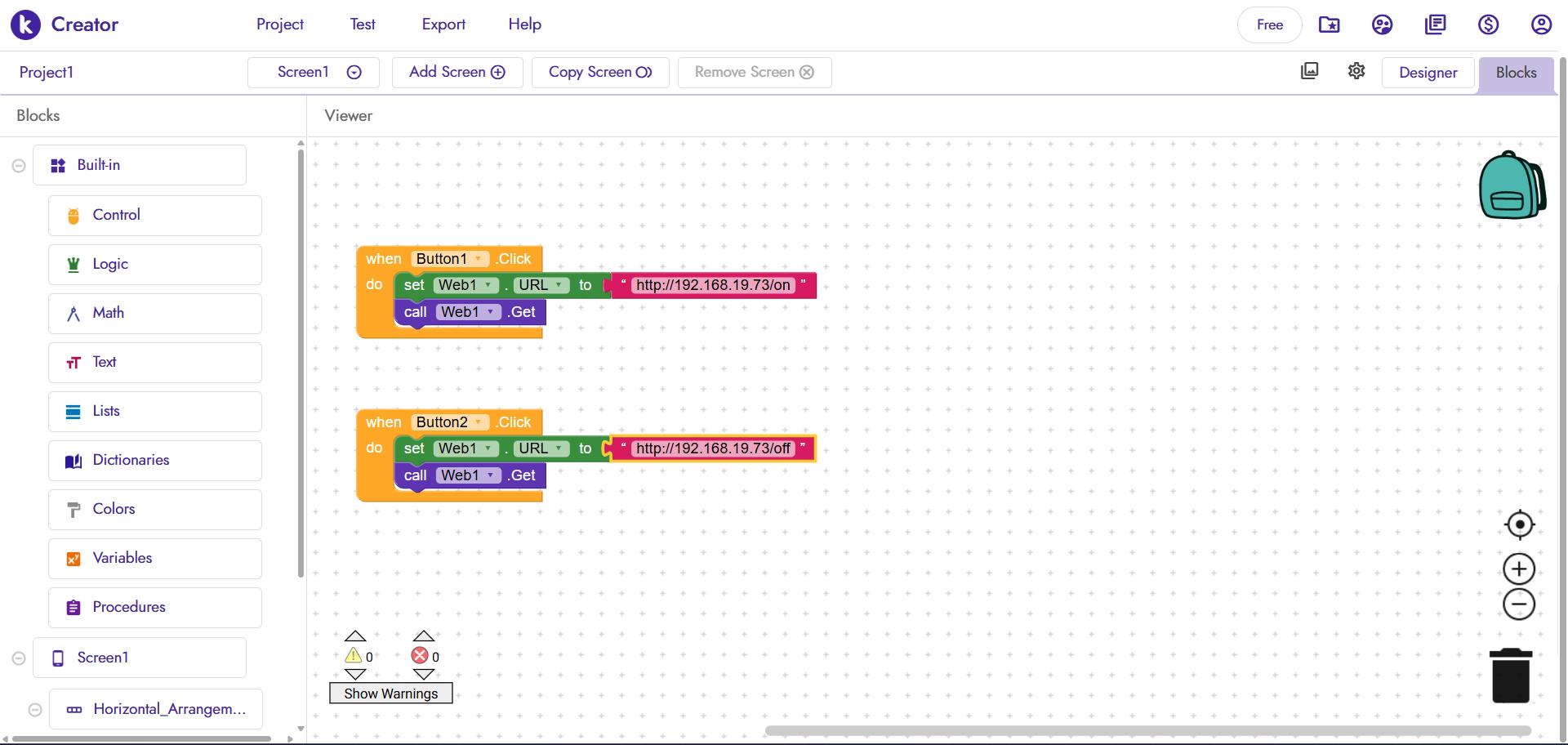
I needed to upload the code in xiao Esp32 C6. To get the code , I used chatgpt.
The code is given below
#include <WiFi.h> #include <WebServer.h> #define LED_PIN 18 // Define your LED pin // Replace with your network credentials const char* ssid = "yourSSID"; const char* password = "Yourpassword"; WebServer server(80); void handleRoot() { String html = "<html><head><title>ESP32-C6 LED Control</title></head><body>"; html += "<h1>ESP32-C6 LED Control"; html += "<p><a href=\"/on\"><button>Turn ON</button></a></p>"; html += "<p><a href=\"/off\"><button>Turn OFF</button></a></p>"; html += "</body>