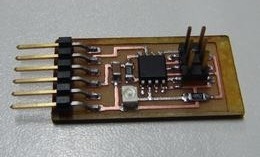
Introduction
For this assignment I used the Hello Light Board made at Input Devices Assignment. The program I did in Python, and execute in Windows 7 OS.
Programing
The idea of the program is to change the colour with the change of the light intensity. To made it more interesting will be the rainbow colors. With low light the colour will be Violet then with the increase of the light intensity will pass to blue, green, yellow, orange until red with brightness light. Also the windows will show the intensity of the light, then the RGB colour and the hexadesimal colour code.
The complete code of the program is below:
# # hello.light.45.color.py # receive and display light level with color # Author: Toshiro Tabuchi - FAB LAB LIMA # # Modified from: hello.light.45.py # Original Author: Neil Gershenfeld - CBA MIT 10/24/09 # # (c) Massachusetts Institute of Technology 2009 # Permission granted for experimental and personal use; # license for commercial sale available from MIT # from Tkinter import * import serial WINDOW = 300 # window size eps = 0.5 # filter time constant filter = 0.0 # filtered value def idle(parent,canvas): global filter, eps #--- idle routine ---# byte2 = 0 byte3 = 0 byte4 = 0 ser.flush() while 1: #--- find framing ---# byte1 = byte2 byte2 = byte3 byte3 = byte4 byte4 = ord(ser.read()) if ((byte1 == 1) & (byte2 == 2) & (byte3 == 3) & (byte4 == 4)): break low = ord(ser.read()) high = ord(ser.read()) value = 256*high + low filter = (1-eps)*filter + eps*value bw = int(filter/1024.0*255.0) colorBW = '#%02x%02x%02x' % (bw,bw,bw) colorRGB = '#%02x%02x%02x' % rainbow(int(filter),1024) x = int(.2*WINDOW + (.9-.2)*WINDOW*filter/1024.0) canvas.config(bg=colorRGB) canvas.itemconfigure("value",text="%.0f"%filter,fill=colorBW) canvas.itemconfigure("RGB",text=rainbow(int(filter),1024),fill=colorBW) canvas.itemconfigure("rgbhex",text=colorRGB,fill=colorBW) canvas.update() parent.after_idle(idle,parent,canvas) #--- Rainbow Color Function ---# def rainbow(val,rang): if val <= rang/5.0: col = (255,int(val/(rang/5.0)*255.0),0) elif val <= 2*rang/5.0: col = (int(255.0-(val-rang/5.0)/(rang/5.0)*255.0),255,0) elif val <= 3*rang/5.0: col = (0,255,int((val-2.0*rang/5.0)/(rang/5)*255.0)) elif val <= 4*rang/5.0: col = (0,int(255.0-(val-3.0*rang/5.0)/(rang/5.0)*255.0),255) else: col =(int((val-4.0*rang/5.0)/(rang/5.0)*255.0),0,255) return col #--- check command line arguments ---# if (len(sys.argv) != 2): print "command line: hello.light.45.py serial_port" sys.exit() port = sys.argv[1] #--- open serial port ---# ser = serial.Serial(port,9600) ser.setDTR() #--- set up GUI ---# root = Tk() root.title('hello.light.45.color.py (q to exit)') root.bind('q','exit') canvas = Canvas(root, width=WINDOW, height=WINDOW, background='white') canvas.create_text(.5*WINDOW,.4*WINDOW,text="...",font=("Helvetica", 14), tags="value",fill='Black') canvas.create_text(.5*WINDOW,.5*WINDOW,text="...",font=("Helvetica", 24), tags="RGB",fill='Grey') canvas.create_text(.5*WINDOW,.6*WINDOW,text="...",font=("Helvetica", 14), tags="rgbhex",fill='Grey') canvas.pack() #--- start idle loop ---# root.after(100,idle,root,canvas) root.mainloop()
* The function that generate the RGB code is call rainbow(val,rang)
. The val
argument is the value of the variable, and the rang
is the variable range. The variable value(val
) has to start from zero and increase to the value of rang
.
Testing
Now is time to test the program. The colours of the window will change with the light intensity.