Week 04 - Embedded Programming
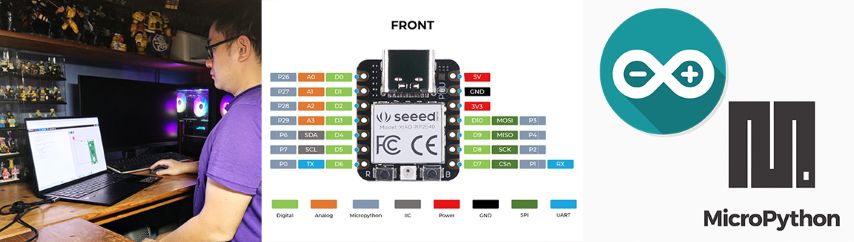
Group assignment:
• Demonstrate and compare the toolchains and development workflows for available embedded architectures
• Document your work to the group work page and reflect on your individual page what you learned
Individual assignment:
• Browse through the datasheet for your microcontroller
• Write a program for a microcontroller, and simulate its operation, to interact (with local input &/or output devices) and communicate (with remote wired or wireless connection)
Group assignment
For this week’s Group assignment, I teamed up with my fellow Fab Academy buddy Haw Ren to demonstrate and compare the toolchains and development workflows for available embedded architectures.
The documentation for our Group assignment can be found here.
Individual assignment
As I am totally new to this world of microcontrollers, I decided to get a bit of help from ChatGPT to decide which microcontroller to use.
Prompt: “Which microcontroller should I use to create an alarm clock with an LED display?”
ChatGPT generated the following table to compare some of the microcontrollers that were covered in this week's lecture.
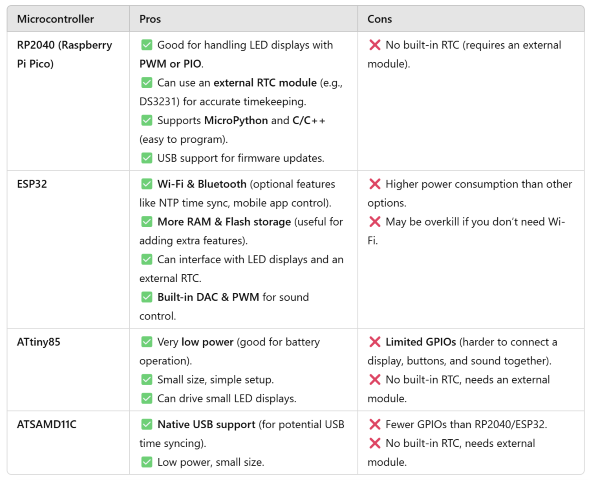
ChaGPT also provided the following recommendation:
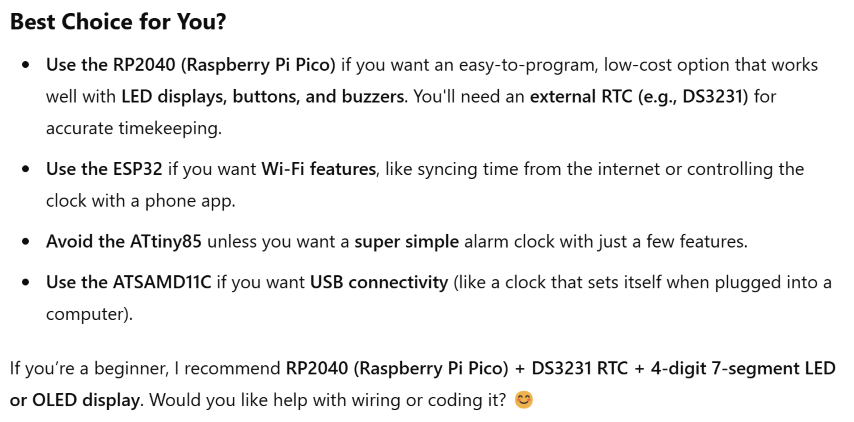
With this recommendation, I chose the RP2040.
Browsing the RP2040 Datasheet
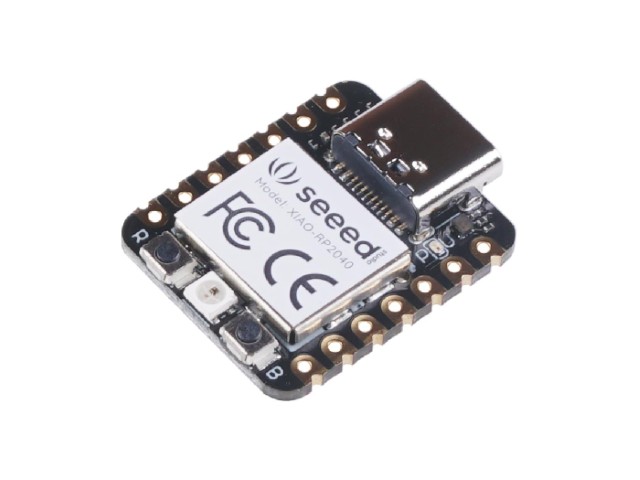
The datasheet for the RP2040 can be found here.
It is a 600 plus page beast and even if I read it cover to cover, I doubt I am going to understand or remember much of it. Fortunately, my instructor, Steven Chew helped me to focus on some of the more relevant sections.
I also referred to this Wiki as it has very good documentation for beginners (Home > XIAO > XIAO RP2040 > Getting Started with Seeed Studio XIAO RP2040).
While this documentation is much easier to read than the Datasheet, I still had trouble understanding some of the terms and features. Hence, I turned to ChatGPT again with the following:
Prompt: “The RP2040's datasheet is more than 600 pages long. Can you summarize and simplify it so that a person who is new to microcontrollers can understand its key features?”
My approach to understanding the RP20240 was to note the ChatCPT responses and then try to find the corresponding information in the datasheet (or the wiki). I found this to be a better way to learn about the RP2040, instead of trying to decipher the somewhat alien language of the datasheet.
Core Features according to ChatGPT (Compared with RP20240 Datasheet/Wiki)
1. Dual-Core Processor – It has two ARM Cortex-M0+ cores, meaning it can perform two tasks at the same time.
2. Fast Speed – Runs at 133 MHz by default but can be overclocked for more speed.
Datasheet/Wiki says:
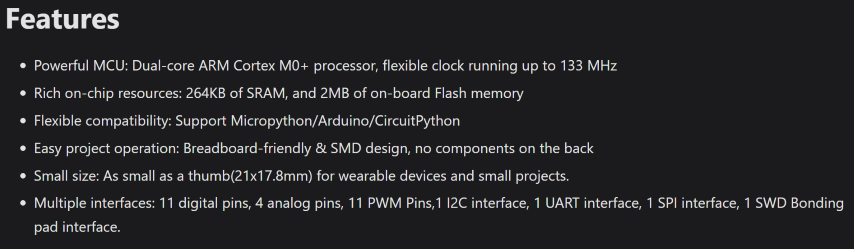
Yes, 1 and 2 are mentioned in the wiki under “Features”.
3. Low Power – Designed for efficient operation, making it great for battery-powered projects.
Datasheet/Wiki says:
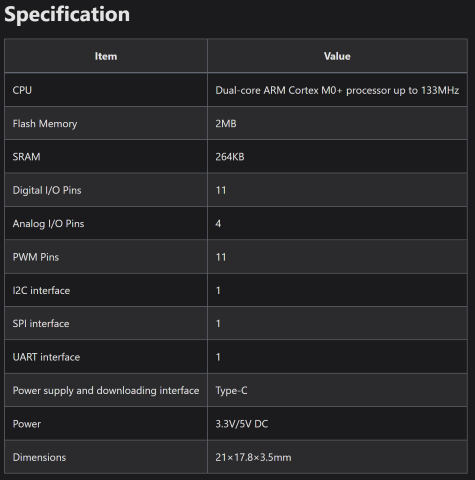
I’ll say this is correct too – The Specifications say that the power is 3.3V/5V DC.
Memory
4. 264 KB of SRAM – This is the fast memory used for running programs.
5. No Built-in Flash Storage – Unlike some microcontrollers, it doesn’t have built-in storage, but it can connect to external flash memory (usually 2MB or more on dev boards like the Pico).
Datasheet/Wiki says:
Referring to the Specifications in the wiki, this is correct. Note: For 5, the wiki Specifications simply say that the Flash Memory is 2MB without further elaboration.
Connectivity & I/O
6. Flexible Input/Output (I/O) Pins – It has 30 GPIO (General-Purpose Input/Output) pins, which can be used for LEDs, buttons, sensors, motors, etc.
7. Communication Interfaces – Supports I2C, SPI, UART, making it easy to connect to other devices like sensors and displays.
8. USB Support – It has USB 1.1, meaning it can act as a USB device (like a keyboard or mouse) or even a simple host (connect peripherals like flash drives).
Datasheet/Wiki says:
I was wondering about 6, because the wiki source says:
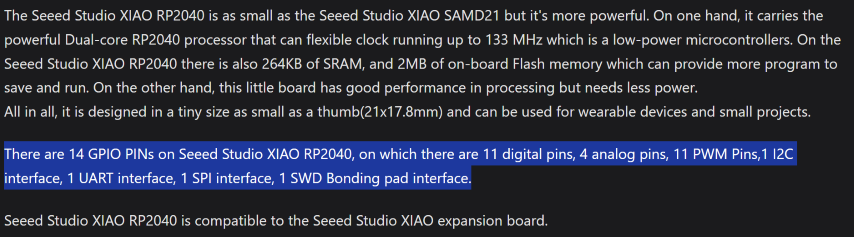
BUT… the Datasheet says:
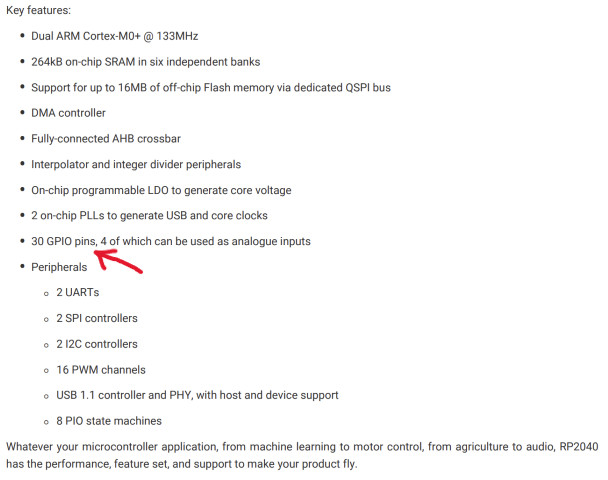
What is going on here? Looking at the pinout from the Datasheet, there are clearly 30 GPIO pins (Pin 0 to Pin 29)
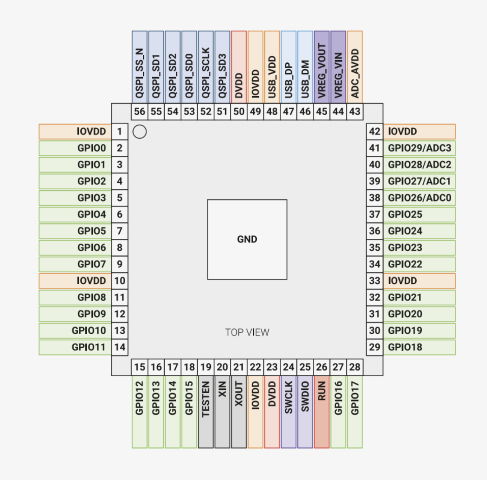
However, from the image below, we can see that there are 14 pins on the Seeed Xiao RP2040.
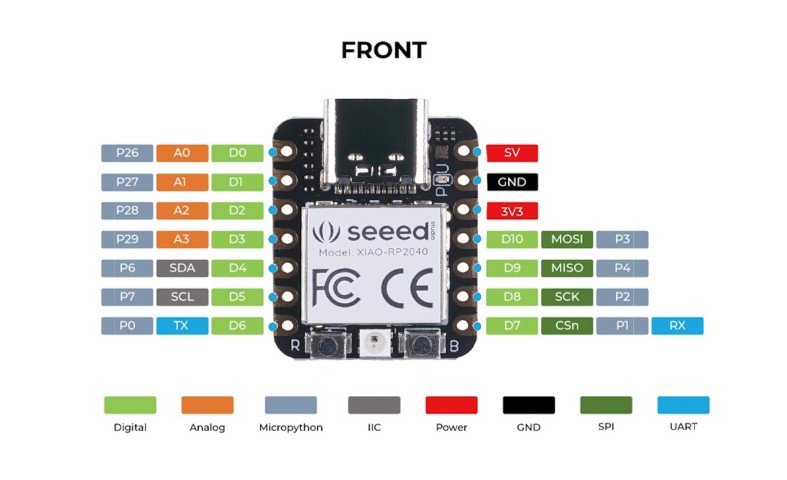
Stumped. I asked Chat GPT again.
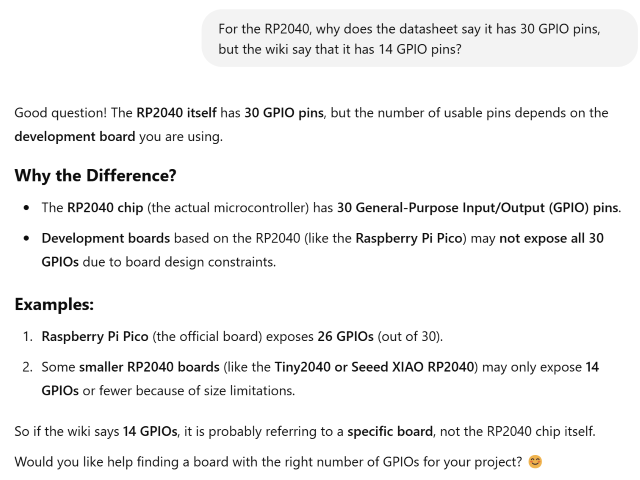
I learnt that there is a difference between the RP2040 chip and the development board, and the number of usable pins is due to the board constraints.
For 7, these are correct, since both the Features and the Specifications mention it.
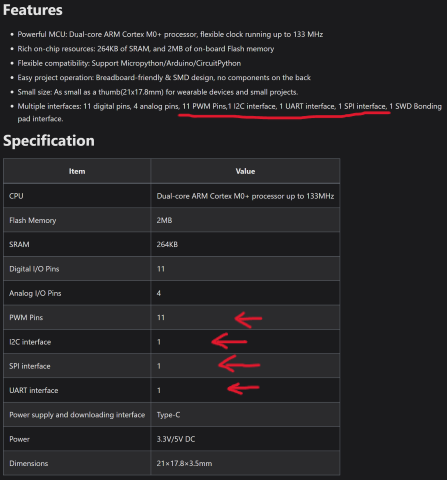
8 can be found in the Datasheet’s introduction under Key Features.
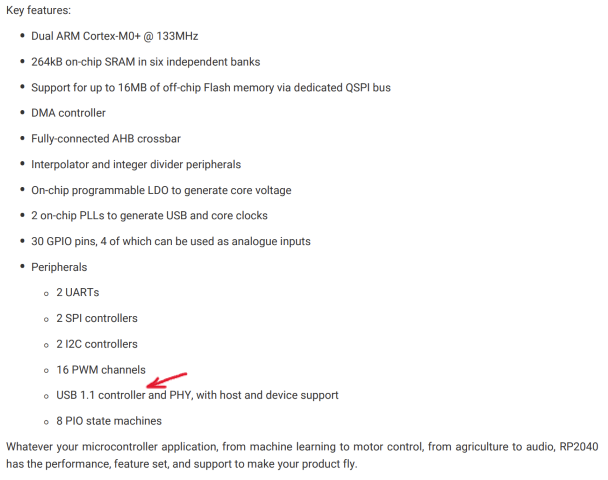
Special Features
9. Programmable I/O (PIO) – A unique feature that allows you to create custom hardware interfaces using software, making it very flexible for things like video output, complex communication protocols, or even generating sound.
10. Timers & PWMs – Useful for precise timing, LED dimming, motor control, and more.
11. Analog Inputs (ADC) – 3 input pins can read analog signals (e.g., from temperature or light sensors).
Datasheet/Wiki says:
For 9, the Datasheet has an entire chapter on PIO, most of it I cannot understand, and so I turn to other sources… I found this YouTube video from Raspberry Pi a little more useful in helping me to understand PIO.
I learn that PIO (Programmable I/O) on the RP2040 is like a mini customizable hardware helper that can handle complex tasks independently, without using the main processor.
10 and 11 can be confirmed as mentioned in the wiki specifications.
Software & Programming
12. MicroPython & C/C++ Support – You can program it using MicroPython (easy for beginners) or C/C++ (more control and efficiency).
13. Drag-and-Drop Programming – You can program it simply by dragging a file onto it like a USB drive.
Datasheet/Wiki says:
Wiki has detailed guides for both programming in Arduino and MicroPython so 12 is correct.
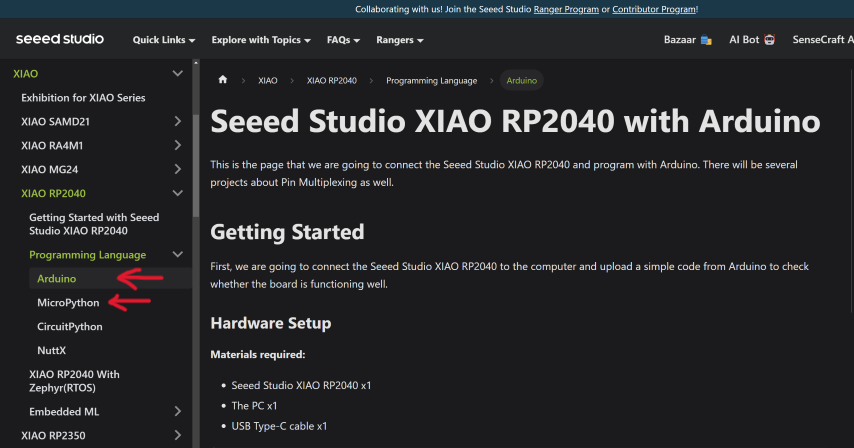
For 13, I do not know yet at this point, but I suppose I will know when I start programming the RP2040.
Writing a program for the RP2040, simulating its operation, to interact and communicate
For this part, I will attempt to write a simple program for the RP2040 using a simulator in Wokwi that blinks an LED on and off. When the LED is blinking on and off, the state of the LED will be communicated to a terminal.
I will try this using two different languages:
1. Arduino
2. MicroPython
Arduino
Go to the Wokwi website and select the Pi Pico.
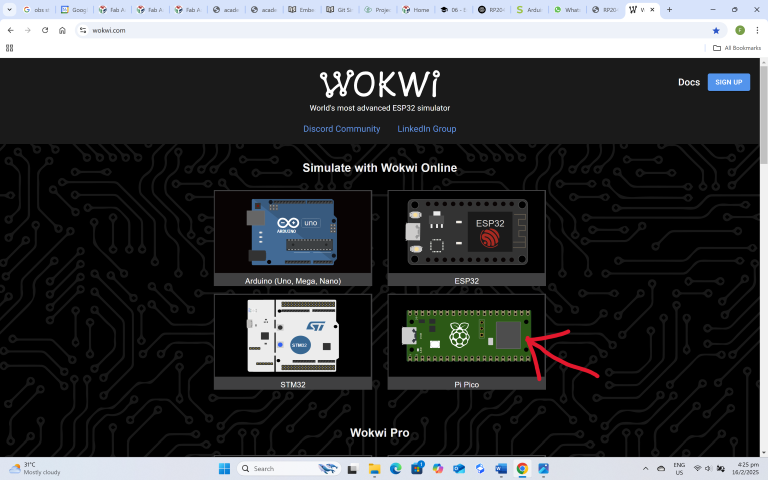
Select a starter template.
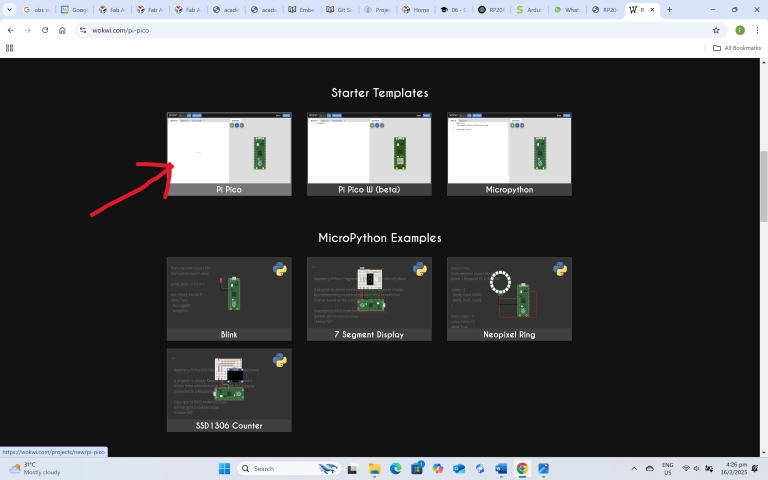
Click the “+” button to add an LED and a resistor to the simulator. You can change the color of the LED, as well as the resistor value. In this case, I have set the LED color to pink, and the resistor value to 200 ohms.
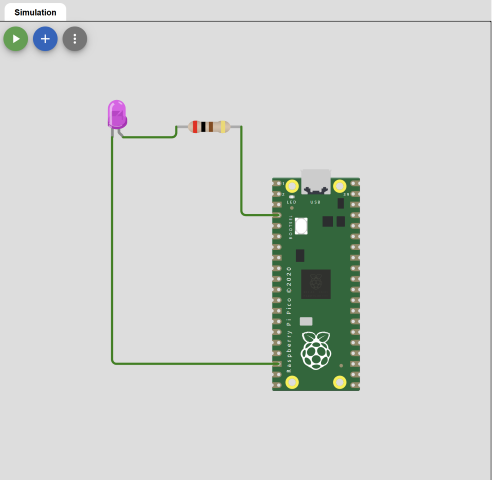
Connect the components to the Pi Pico. You can hover over the pins to see which pins you are connecting to. In my case, I used GP2 and GND.4.
Once I have the components connected, I can begin coding.
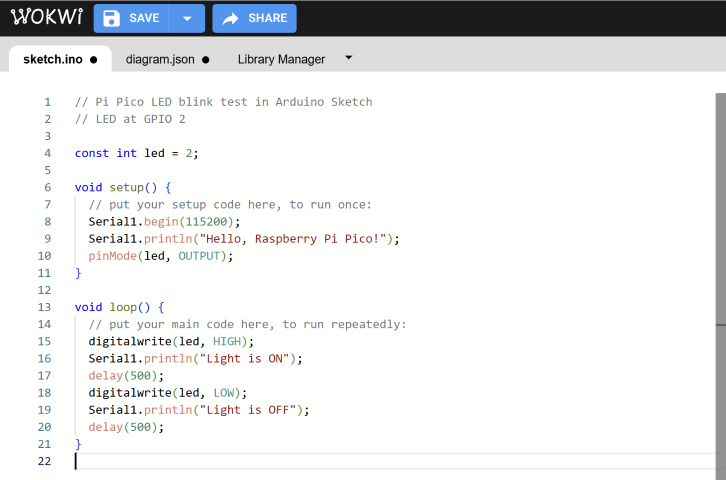
What the Code Does
1. Initializes Serial1 communication.
2. Prints "Hello, Raspberry Pi Pico!" once.
3. Sets GPIO 2 as an output.
4. In the loop:
o Turns the LED ON for 0.5 seconds.
o Prints "Light is ON" to the serial monitor.
o Turns the LED OFF for 0.5 seconds. o Prints "Light is OFF" to the serial monitor.
o Repeats indefinitely.
Error compiling
I encountered this error when compiling.
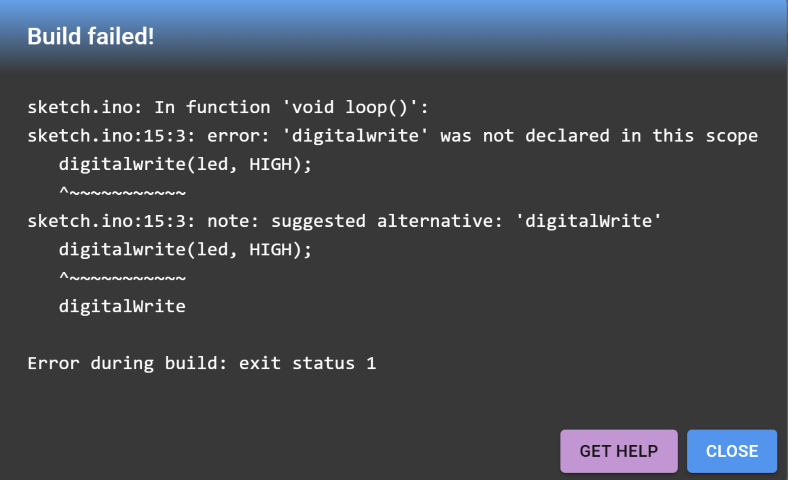
It turns out that this error is just that the “W” in digitalWrite should be in uppercase. Once I corrected this mistake, the program runs correctly.
Link to project: https://wokwi.com/projects/423035598722725889
MicroPython
The process for MicroPython is similar to the process for the Arduino. Here are the steps
Go to the Wokwi website and select the Pi Pico Select a starter template. This time, I chose the MicroPython starter template.
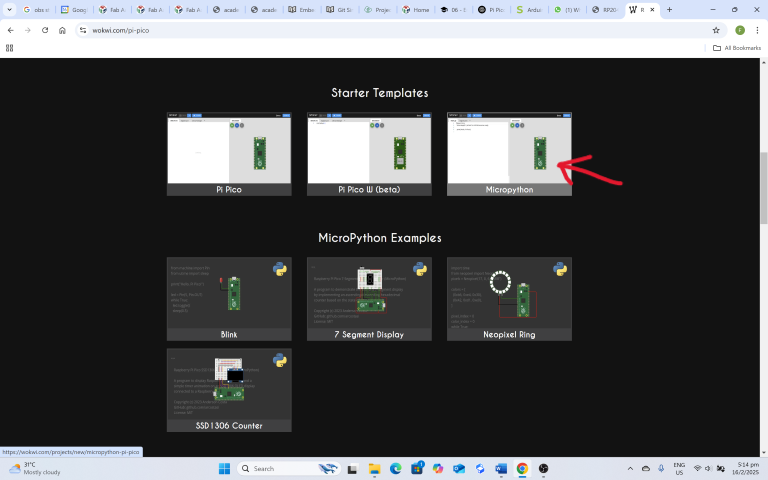
Click the “+” button to add an LED and a resistor to the simulator. Like before, I have set the LED color to pink, and the resistor value to 200 ohms. Connect the components to the Pi Pico. I used GP2 and GND.4.
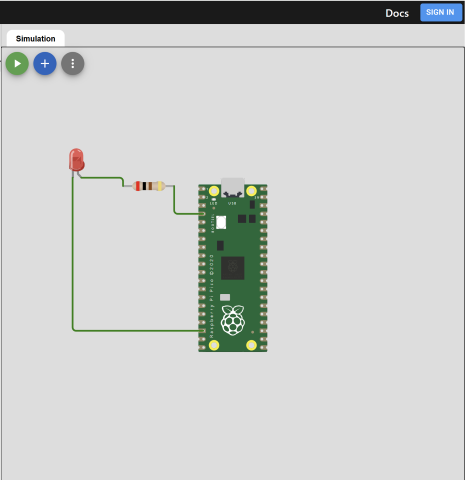
Once I have the components connected, I begin coding and write the following code:
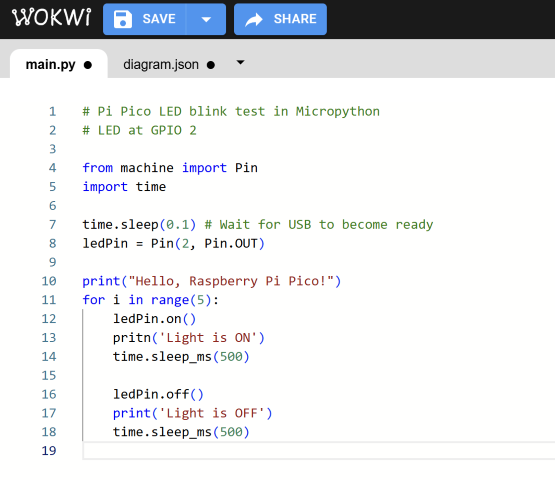
What the Code Does
1. Initializes GPIO 2 as an output.
2. Prints "Hello, Raspberry Pi Pico!".
3. Blinks the LED ON and OFF for 5 cycles, each lasting 500ms.
4. Stops execution after 5 blinks.
Error compiling
I made a small typo error, which tells me I need to be careful when coding.
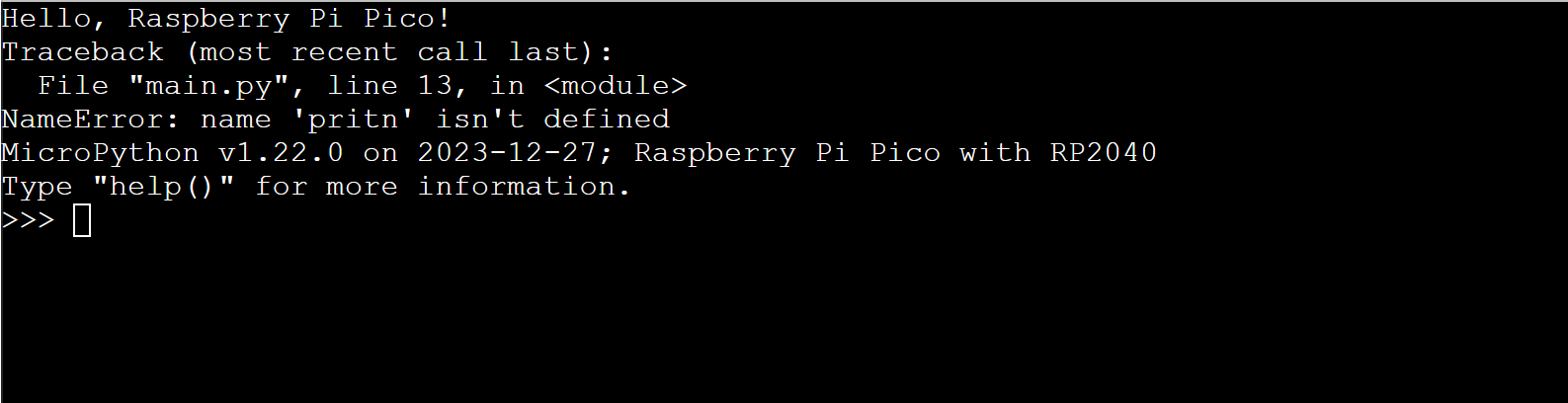
Once corrected, the program runs correctly.
Link to project: https://wokwi.com/projects/423037229034180609
Comparing the Arduino sketch and MicroPython codes
With help from ChatGPT, I compared the code from Arduino sketch and the code from MicroPython.
Feature | Arduino C++ | MicroPython |
GPIO Setup | pinMode(led, OUTPUT); | Pin(2, Pin.OUT) |
Turning LED ON/OFF | digitalWrite(led, HIGH/LOW); | ledPin.on()/off() |
Delay | delay(500); | time.sleep_ms(500) |
Serial Output | Serial1.println("Light is ON"); | print("Light is ON") |
Looping | loop() (runs indefinitely) | for i in range(5): (runs 5 times and stops) |
Apart from the differences in how the codes are written, the functions are almost identical. I learnt that I can even make the loop indefinite for the MicroPython (replace for i in range(5): with while True:)
Closing Thoughts
As I am totally new to this topic, I found that ChatGPT was really useful in helping me to understand datasheets and how code works. ChatGPT excels at expressing ideas in “non-engineer” language, which helps me understand this topic better. For now, I doubt I can start writing code without referring to any references, but it takes time to learn any language, programming language included.
Program Files
This concludes the documentation for my individual assignment for this week.