06 Embedded Programming
This week's assignment is to browse through the datasheet for your microcontroller as well as compare the performance and development workflows for other architectures in the group assignment and write a program for a microcontroller development board to interact.
Group assignment: Embedded Programming
The documentation for our group assignment from the Barcelona node can be found here.
Lessons Learned
- Arduino is the go-to community, but the workflow takes a little longer since it needs to verify and than upload the code
- From an Arduino programmed board, you cannot read code - save your code!
- Micro-Phython is easier to debug (e.g. via Terminal), but slower because it's an interpreted language
- Micro-Phython has not yet a bunch of libraries to build on and noone in the Fablab Bcn is a real expert on the field
- Datasheets are incredibly long! - one of the most important pages is the Pin-out
First steps with Arduino
1. Recapture how the code is written
Being a complete newbie, I had to recapture our local classes and initially understand how the code is structured (again):
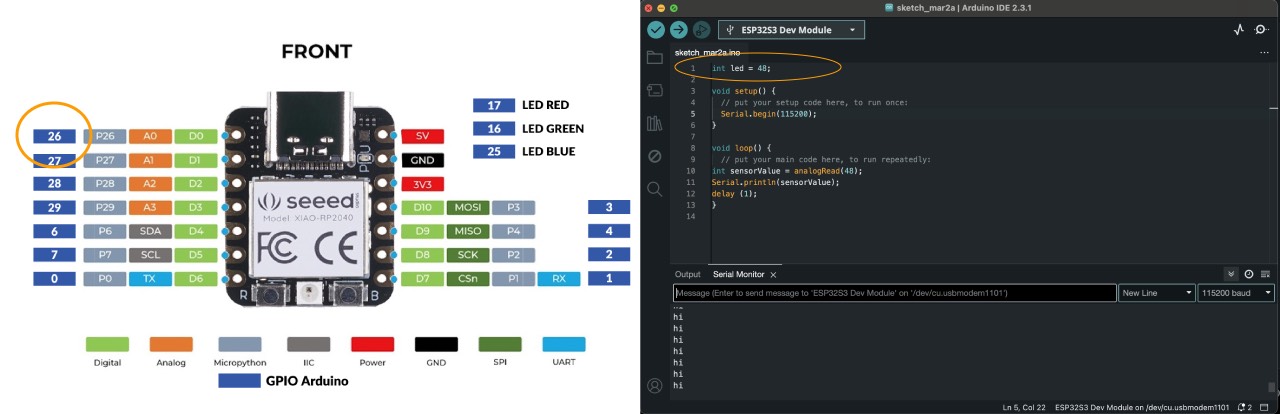
- top: definitions of pins, libraries, etc. = information the board needs to run the code and can be found in the datasheet (e.g. Pin-out)
- void setup () = code that runs everytime, once in the beginning
- void loop () = main code, that will run and restart
Secondly I needed some understanding of how the code is written:
- The code follows camelCase
- The Arduino page is a great cheatsheet on how you should write the code
- Arduino tells you, when you misspell something - love it!
- // plus text is a comment and will not affect your code
2. Get code running on my board(s)
On the first trials I experienced a lot of issues, when I uploaded code to both the Quentorres and the Barduino board we are using to learn in the Fablab Barcelona.
Debugging 1:1
- try different USB-A to USB-C converter cables or plug them differently to the board and your laptop
- try different USB ports on your laptop, initially my board was simply not showing up
- check if
Tools/USB CDC on Boot
is ENABLED, if disabled that will lead to errors - check if you are connected to the right version of the board, my laptop kept on defining the Barduino board as "ESP32-S3 Box" instead of "ESP32-S3 DevModule"
- reboot the board with pressing
B
while plugging the board to your laptop or pressingR
- restart the board with pressing
R
- if everything else fails try to restart your computer, that solved most of my first-day issues
Upload Code samples
I started uploading the code examples provided by our lab as well as some of the solutions of the Adventronics calender of Fablab BCN.
It worked on the boards, but simply uploading the code didn't help me get to the bottom of Arduino.
3. Understanding the basic structure of Arduino
I started following the Basic Arduino built-in-examples and mapping out a cheatsheet for myself about Arduino and use the language reference done by Arduino. Most of the examples I tried out with the Quentorres, the Barduino and/or the Elegoo UNO R3.
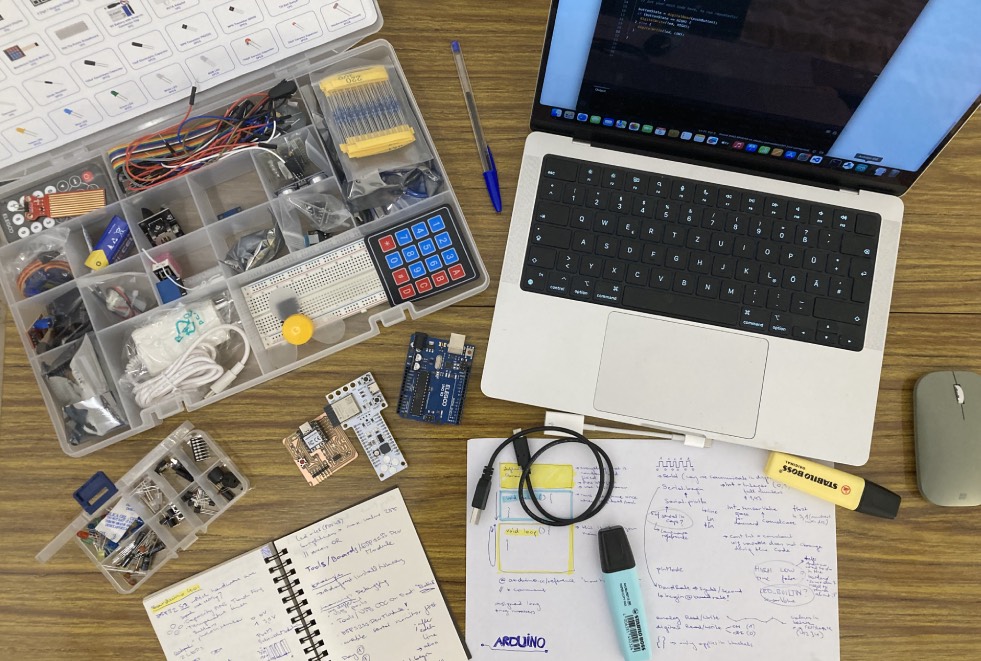
- Analog Read Serial: initially I got the wrong PIN on the Quentorres, lesson learned to check for analog output again on the Pinout (also I thought I had burnt my laptop)
- Blink: I had to resolder one LED, since checking with the multimeter revealed that current was flowing, and the program was running on the other LEDs
- Digital Read:keep in mind the baud rate for the different boards!
- Fade LED: keep in mind how the comparison operators are written
- Read Analog Voltage: use Serial to understand what datarange your component is sending
Generally speaking the Pinout is your best friend and you always wanna keep an eye on your code ending with;
. Additionally some case examples (e.g. LED_BUILTIN
or Serial.begin
) are simly exceptions
you just need to accept that it does not follow the rules. Personally being a very visual person it was much easier to understand what is happening if you need to plug in components on the breadboard.
Thanks
4. Write and Wire on my own: Blink with Button
First declare where your components are located and that they can have different states. It's not necessary to declare here which states they have. Secondly define which component is the INPUT and OUTPUT in the void setup. In the void loop define what is happening: ButtonState needs to "digitally read" the Button, if that is high "digitally write" the LED state to high else "digitally write" the LED state to low.
int led = 28;
int pushButton = 27;
int buttonState;
int ledState;
void setup() {
// put your setup code here, to run once:
pinMode(pushButton, INPUT);
pinMode(led, OUTPUT);
}
void loop() {
// put your main code here, to run repeatedly:
buttonState = digitalRead(pushButton);
if (buttonState == HIGH) {
digitalWrite(led, HIGH);
} else {
digitalWrite(led, LOW);
}
}
Lessons Learned
- it's great to learn from others - it's hard to simply learn from tutorials
- there is much much more to learn - a whole box of joy to explore
- for me it's simpler to see the physical hardware and the wires to understand the underlying process
- keep your pin-out always close!