Week 14: Interface Application Programming
Interface Application Programming
Assignment activities: Group assignment: Compare as many tool options as possible If you want to explore the group Assignment Click Here 1. NODE RED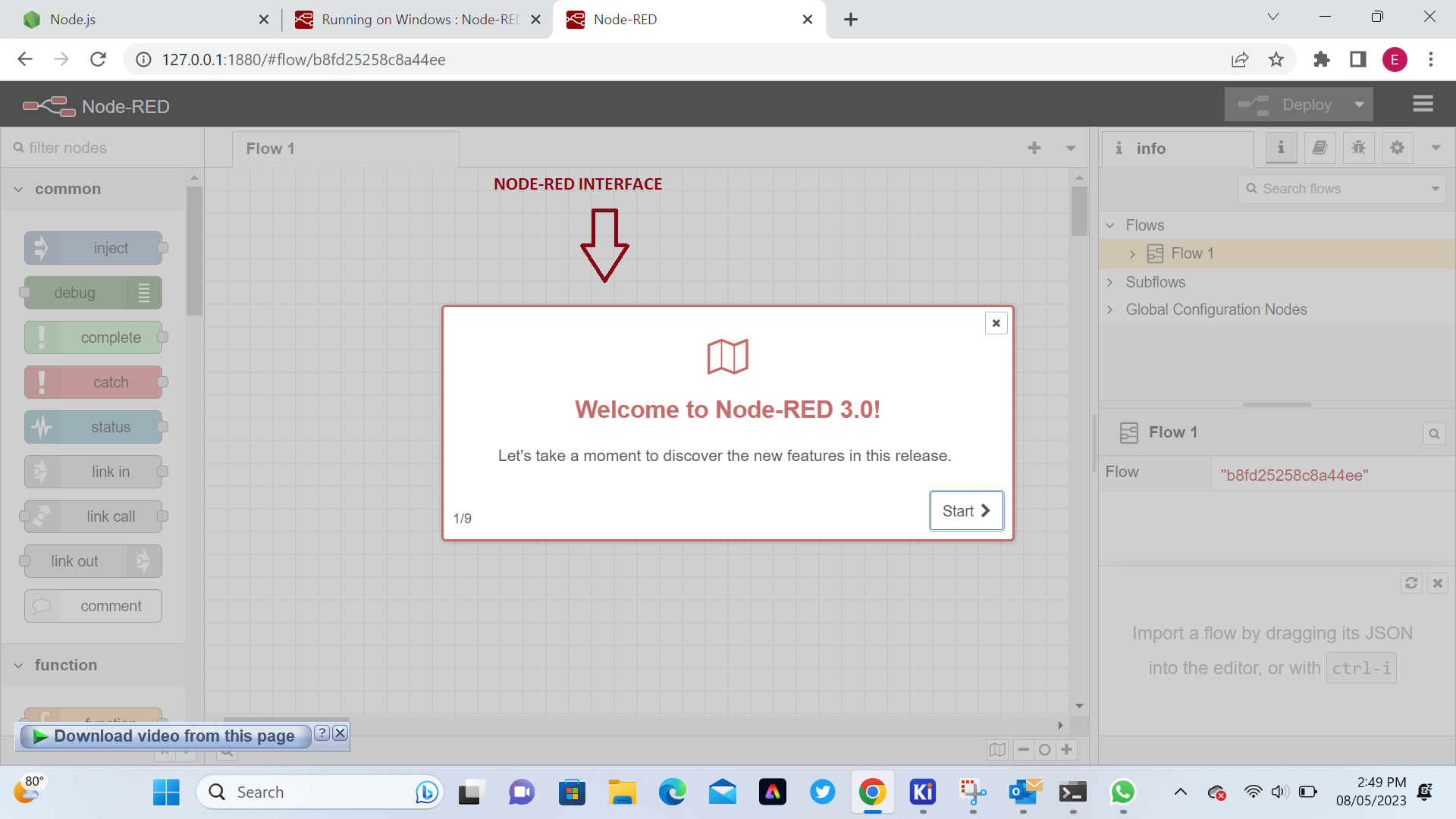
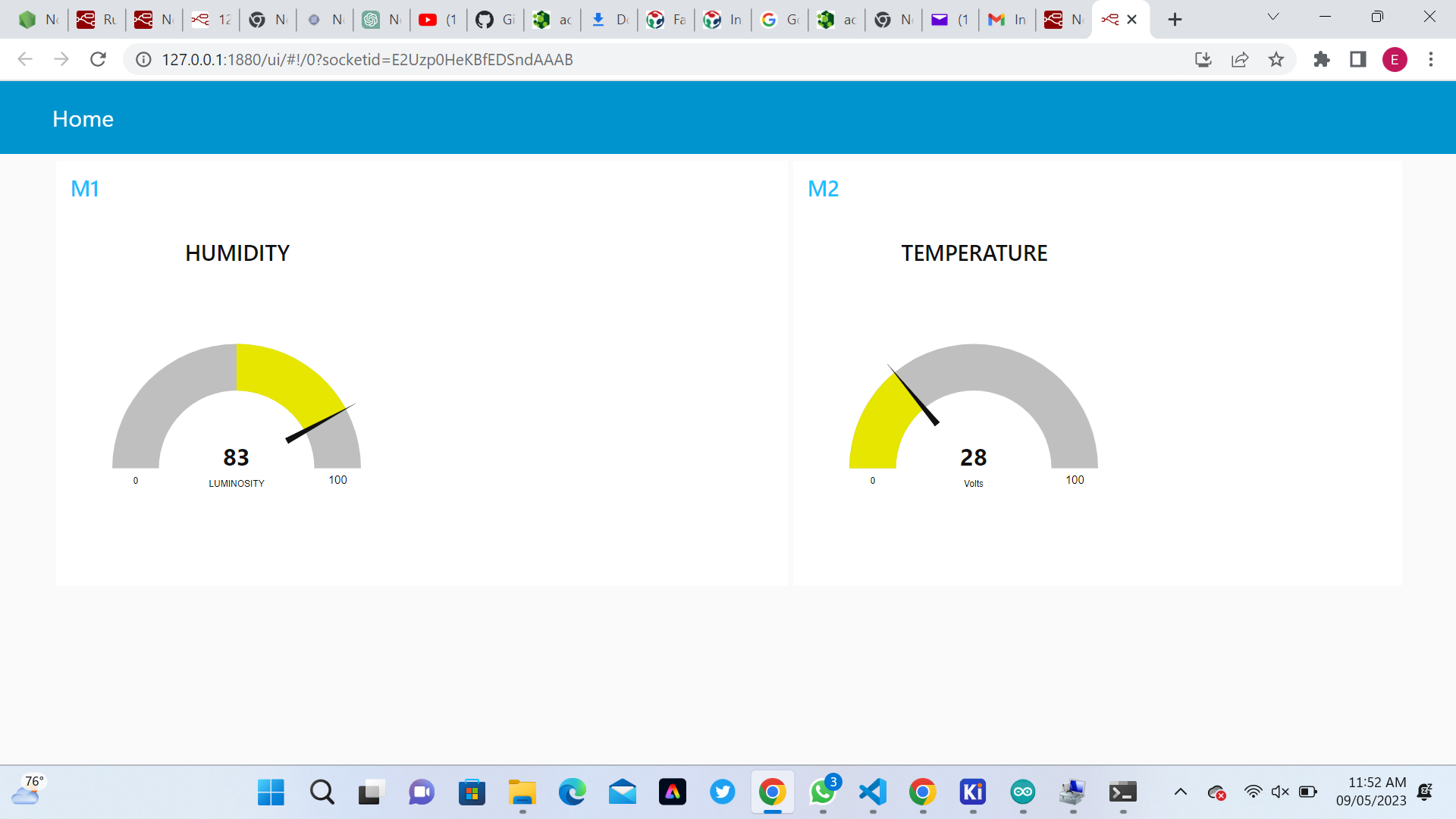
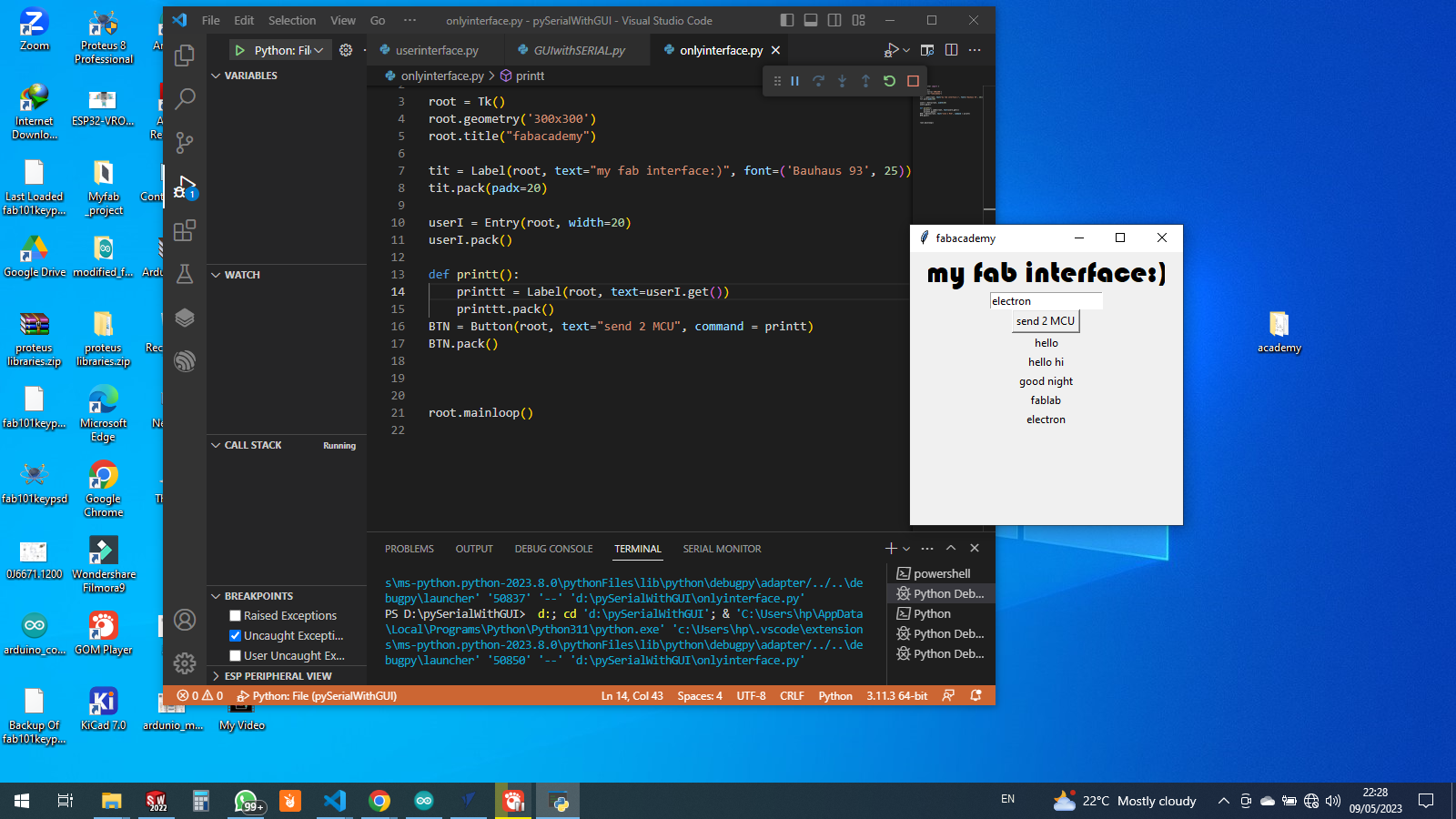
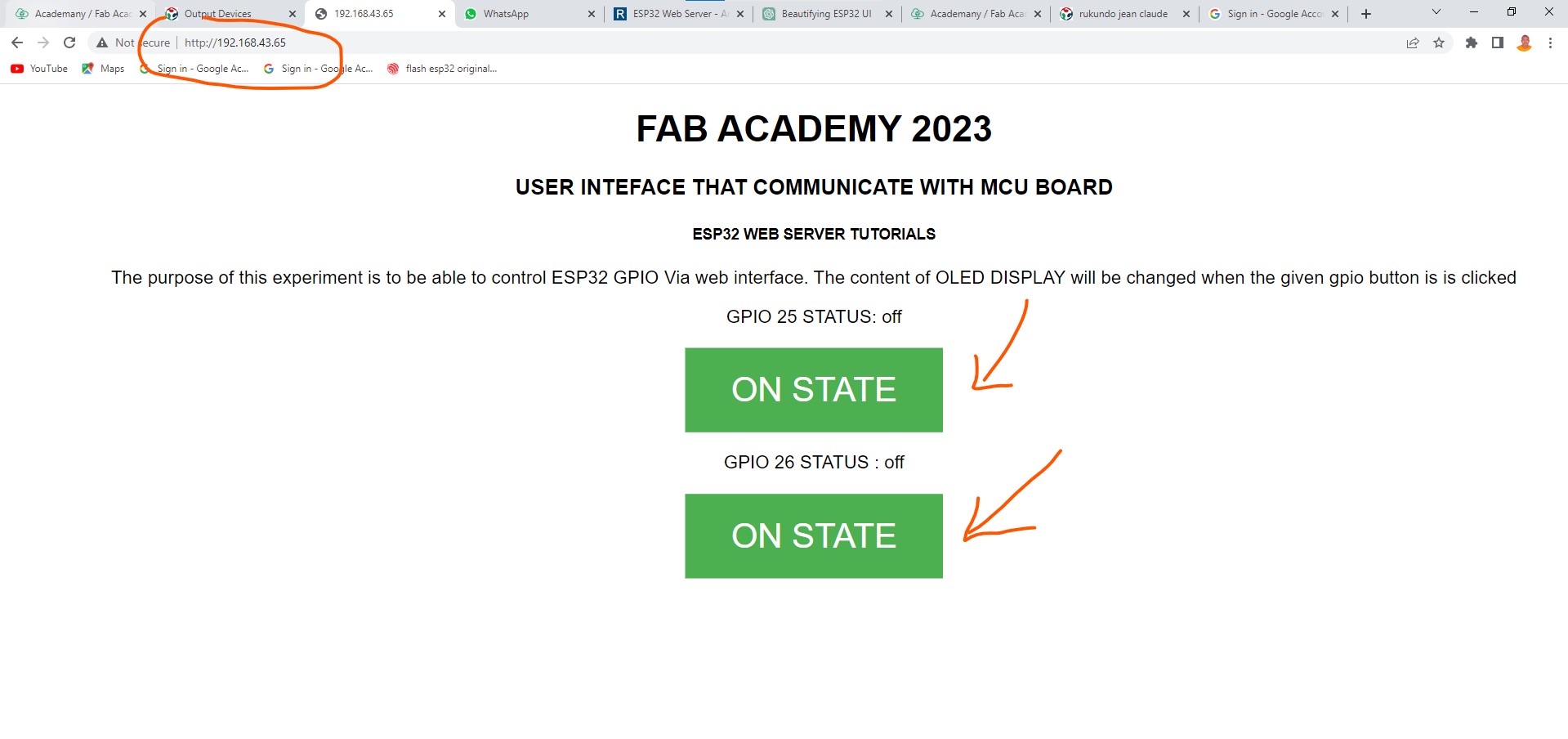
II.Individual assignment:
What I did in this week: In this week we make an interface which display Temperature and Humidity by using Node-RED to control the ESP32 with a Serial Coomunication and DHT11 temperature and humidity sensor module. I.We started by Designing the PCB and built our own board By using a microcontroller board that you have designed in Week 9 Output_Deviceswe use ESP23 Microcontroller,for Temperature and Humidity by using Node-RED to control the ESP32 with a Serial Coomunication and DHT11 temperature and humidity sensor module here is ESP23 Datasheet What is ESP 32? ESP32 is a powerful microcontroller developed by Espressif Systems. It is a dual-core, 32-bit processor with built-in Wi-Fi and Bluetooth connectivity. The ESP32 is designed for a wide range of applications such as IoT (Internet of Things) devices, home automation, industrial automation, smart appliances, and more. It has a clock speed of up to 240 MHz, 520 KB of RAM, and 4 MB of flash memory for program storage. Additionally, it has a variety of peripheral interfaces including SPI, I2C, UART, and ADC, making it easy to connect to sensors, displays, and other devices. The ESP32 can be programmed using a variety of programming languages including C++, Python, and MicroPython, making it a popular choice for both hobbyists and professional developers. Here is Schematic Used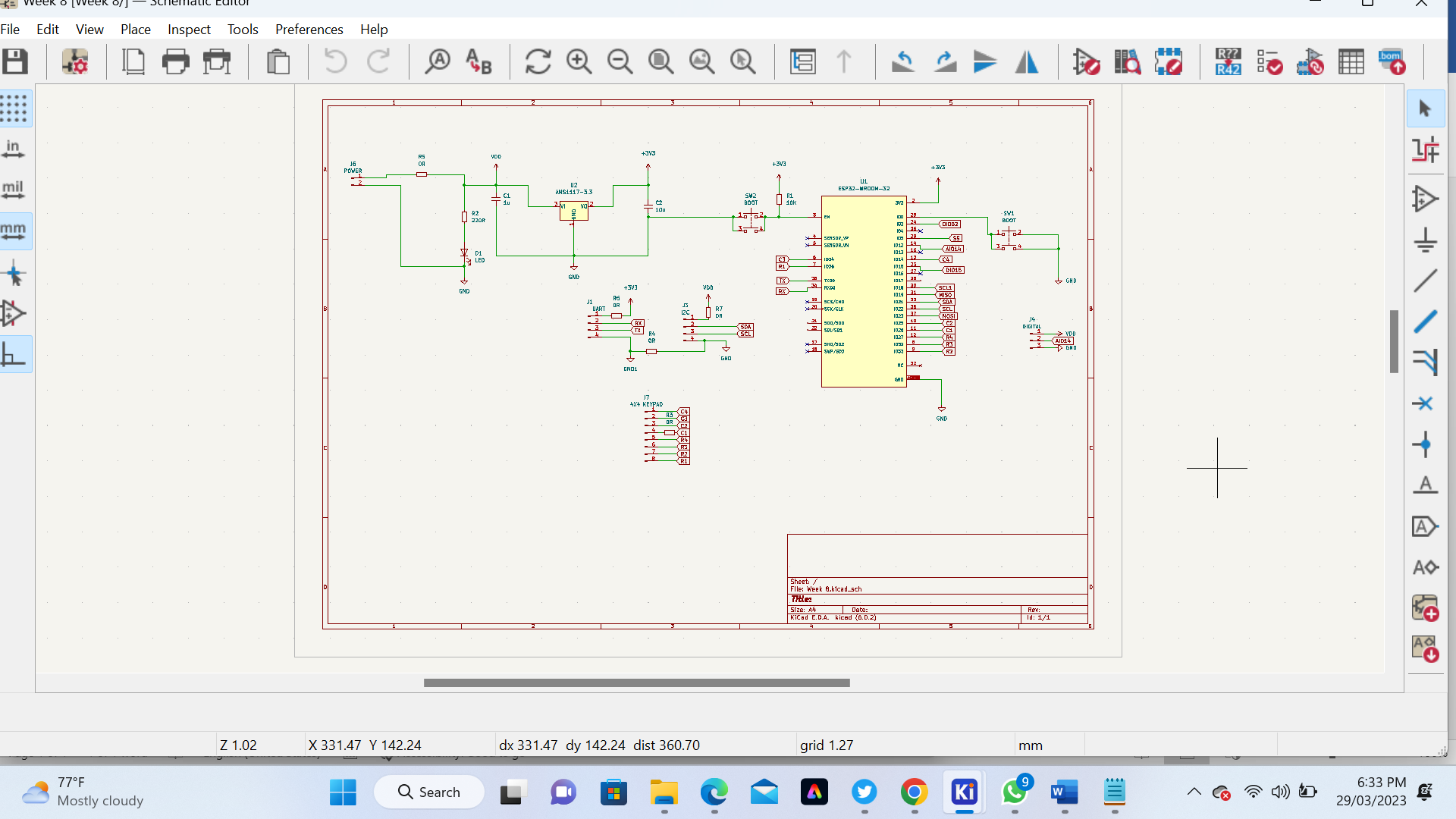
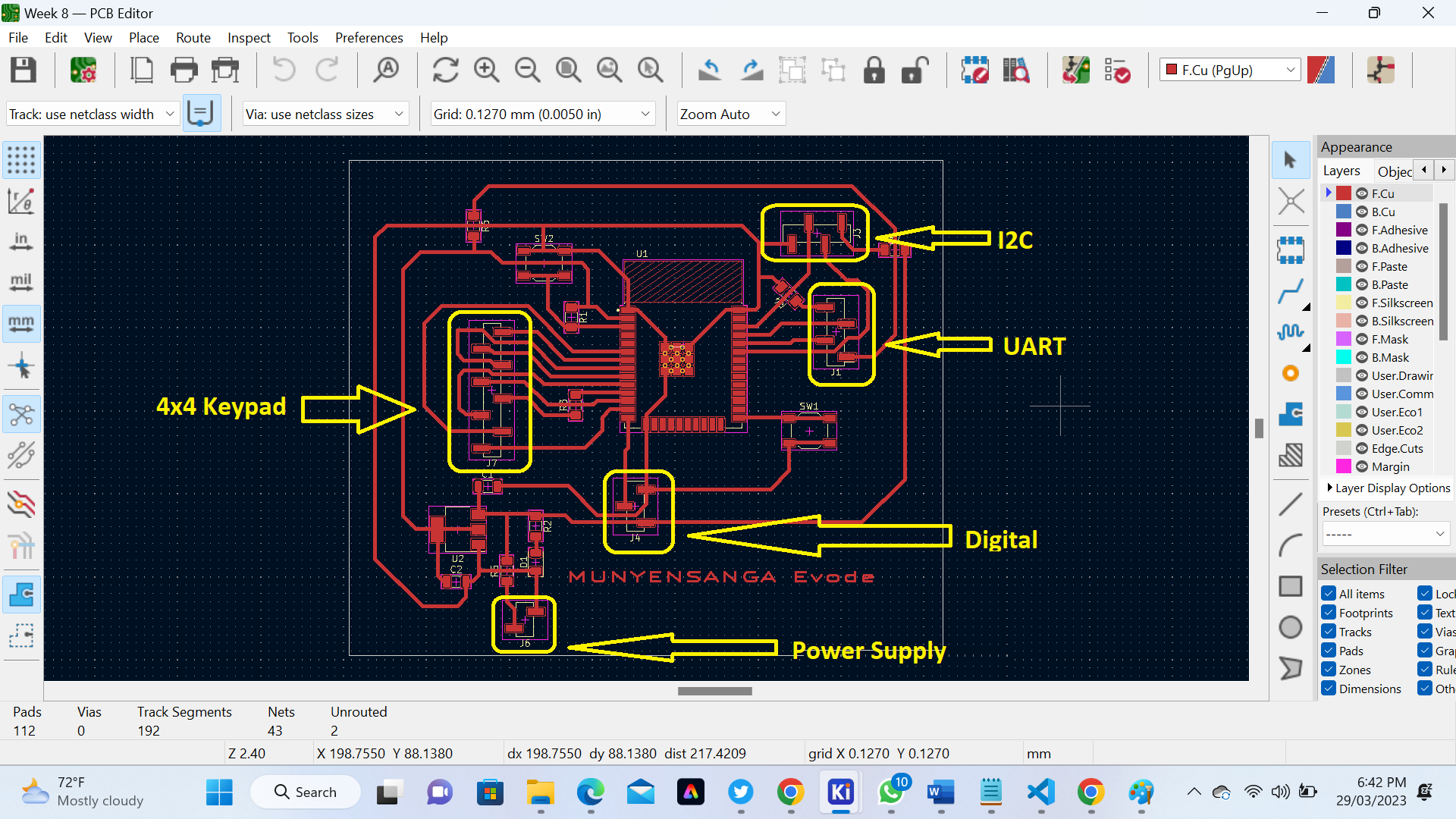
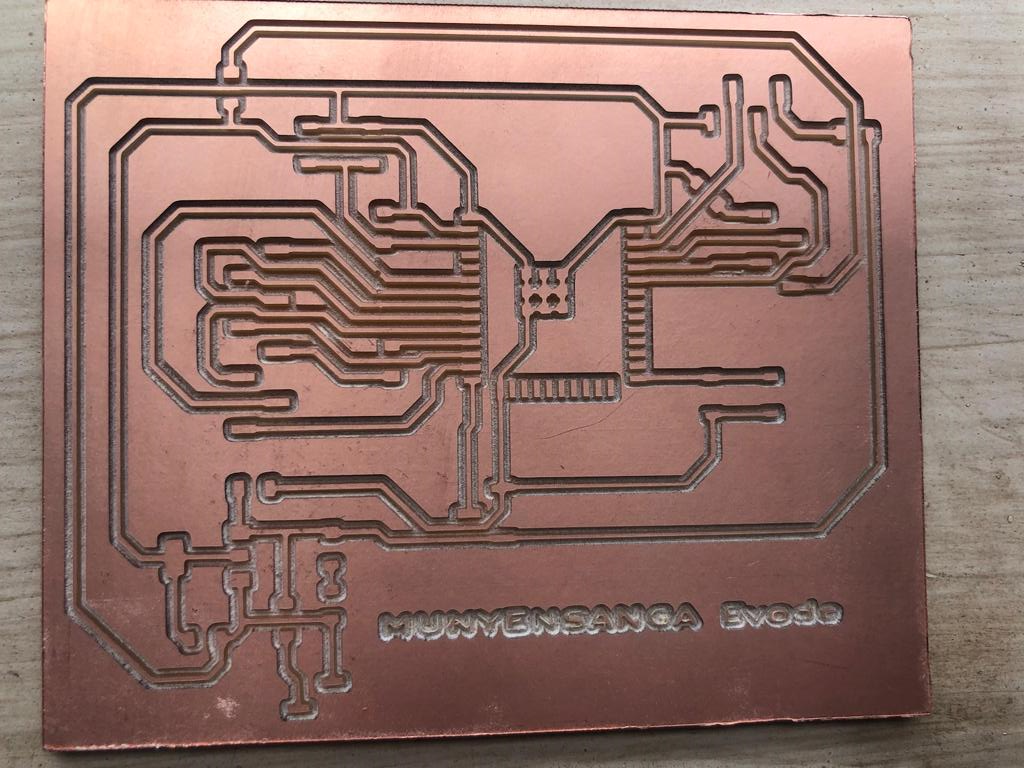
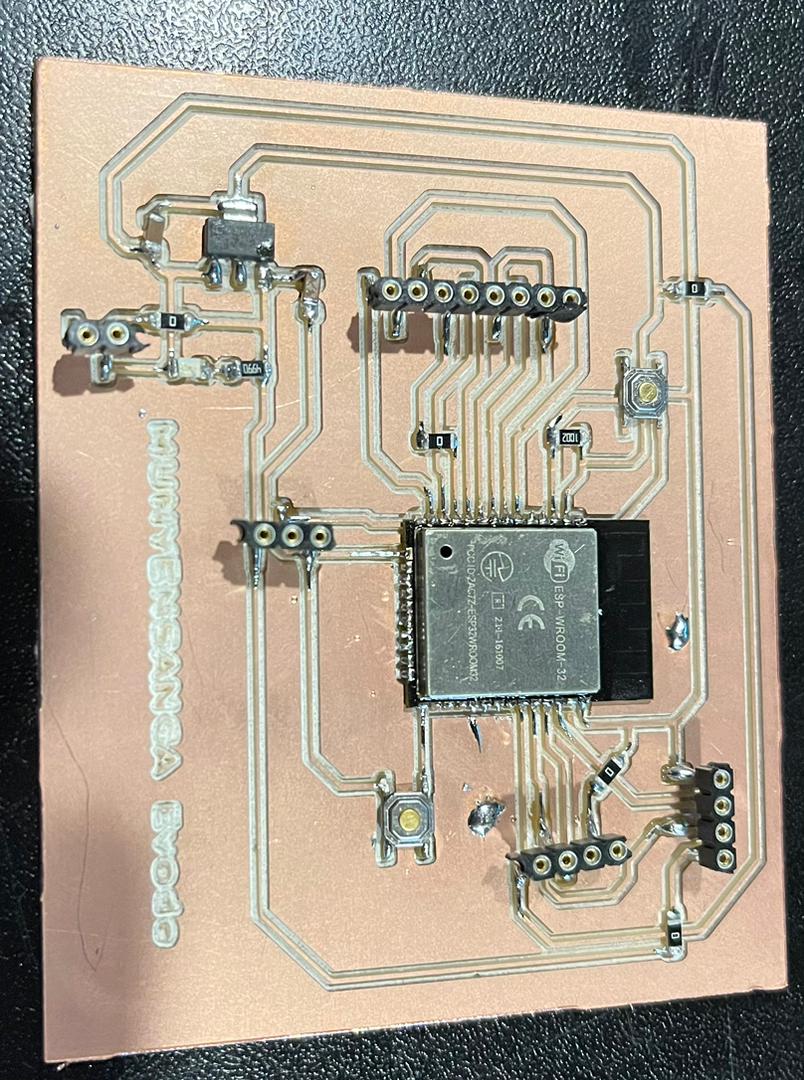
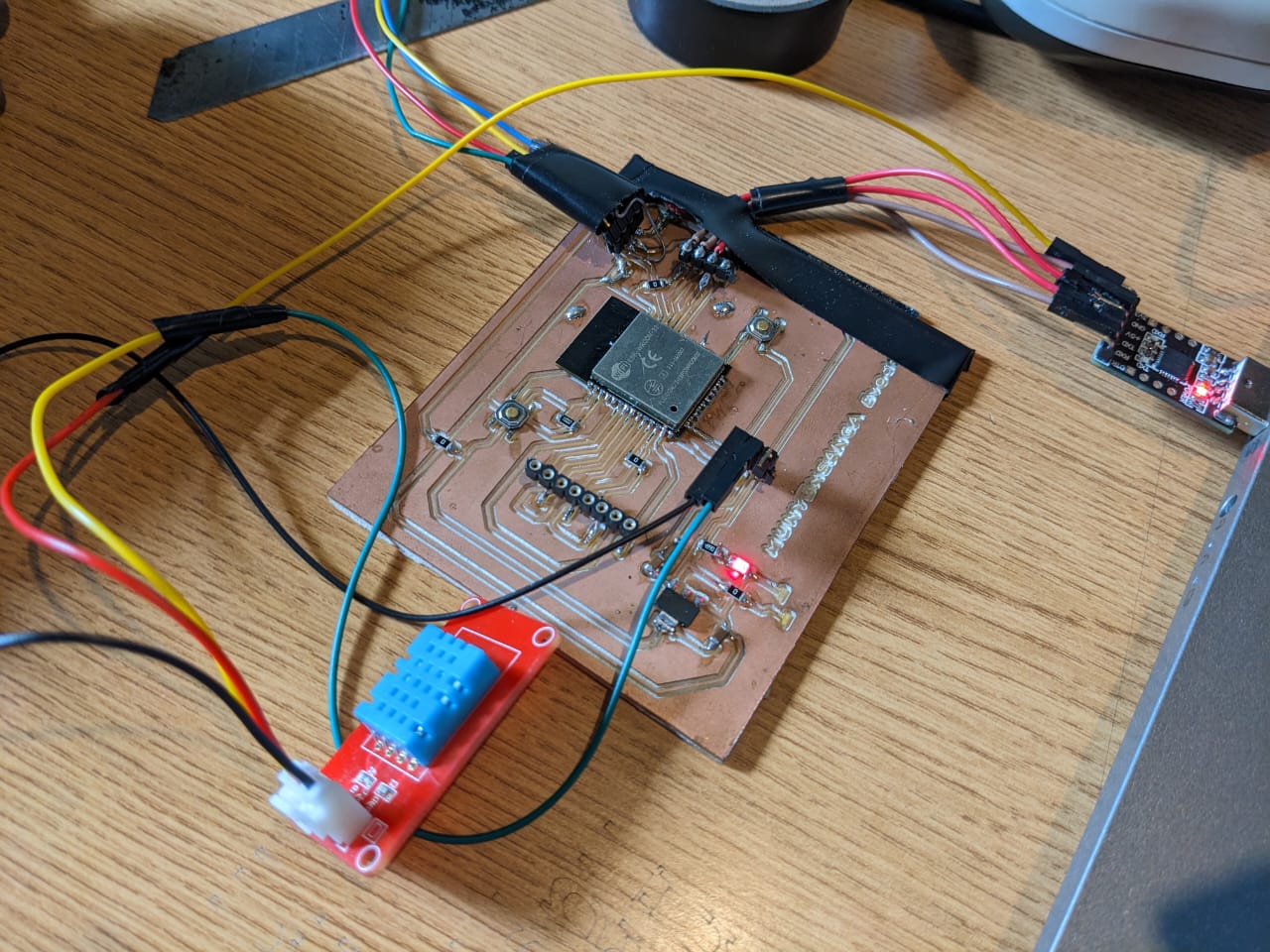
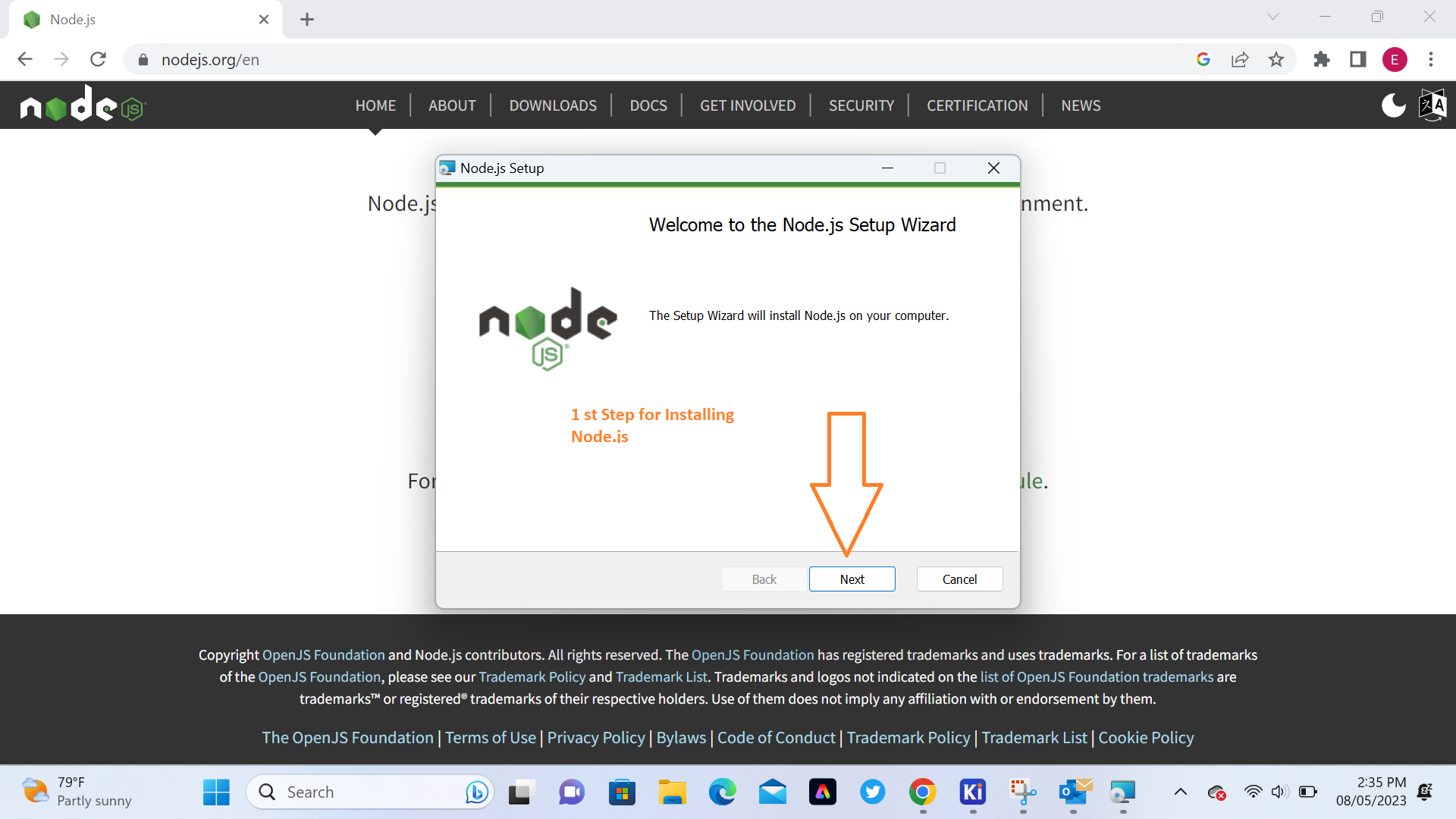
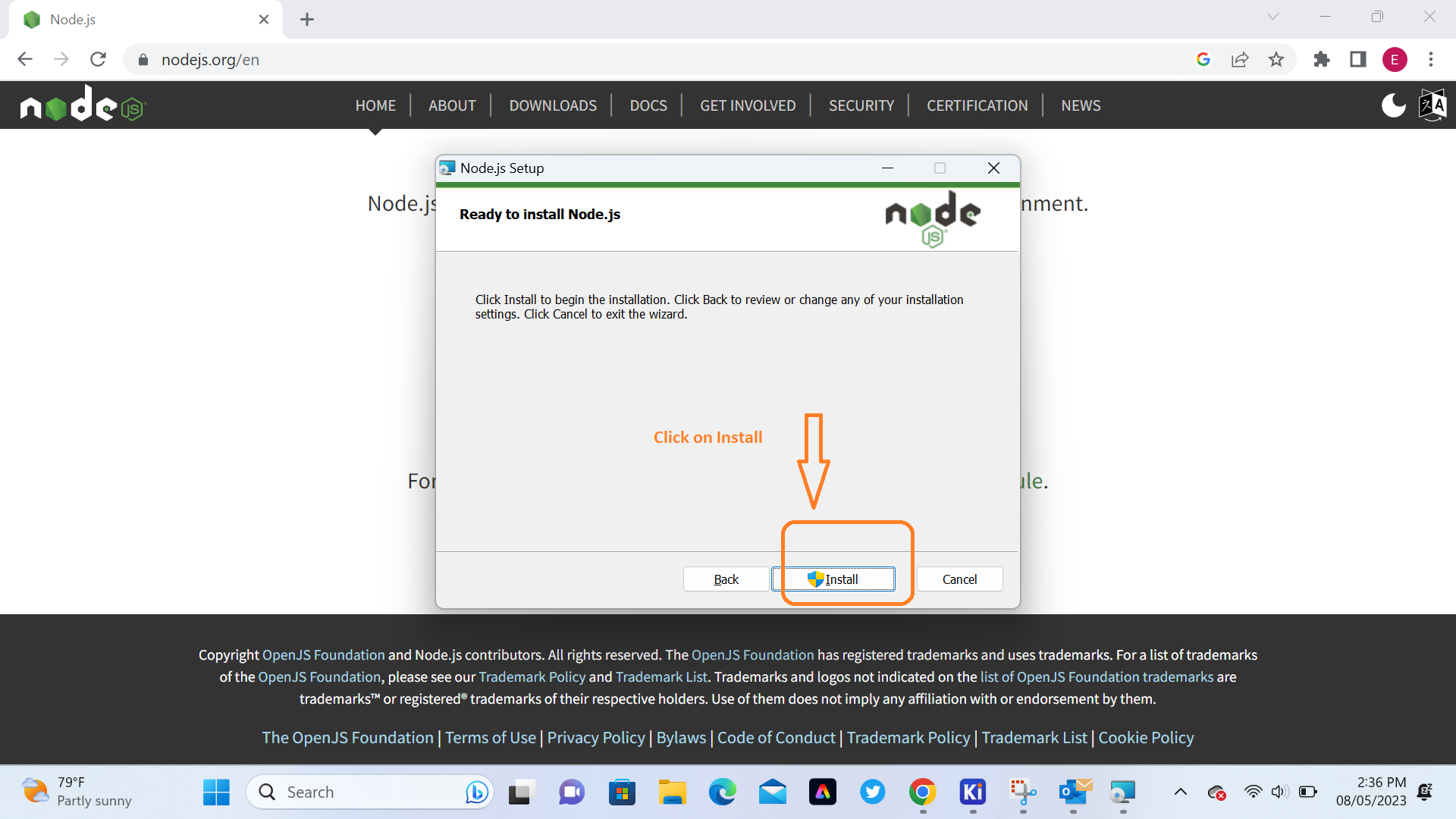
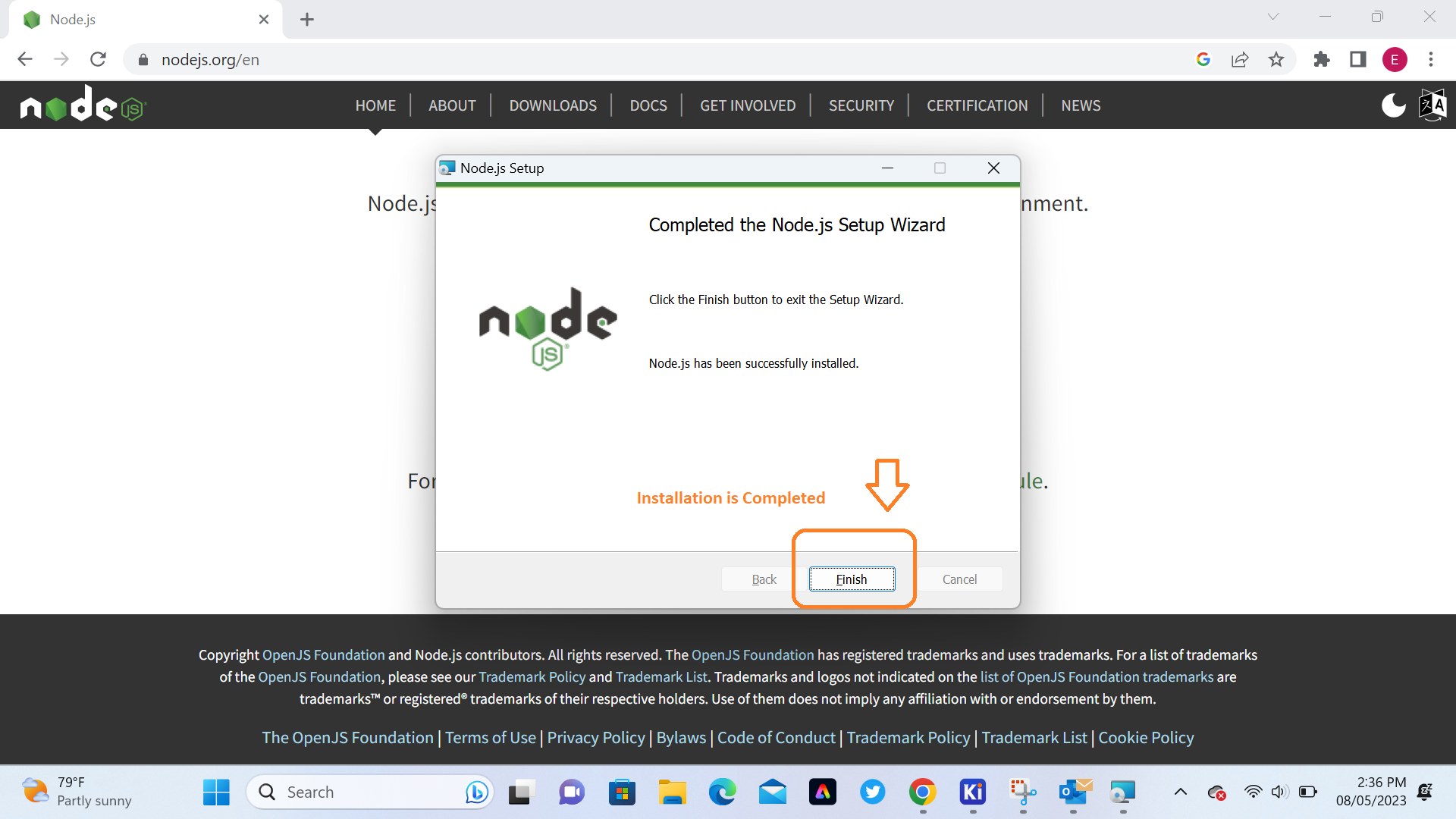
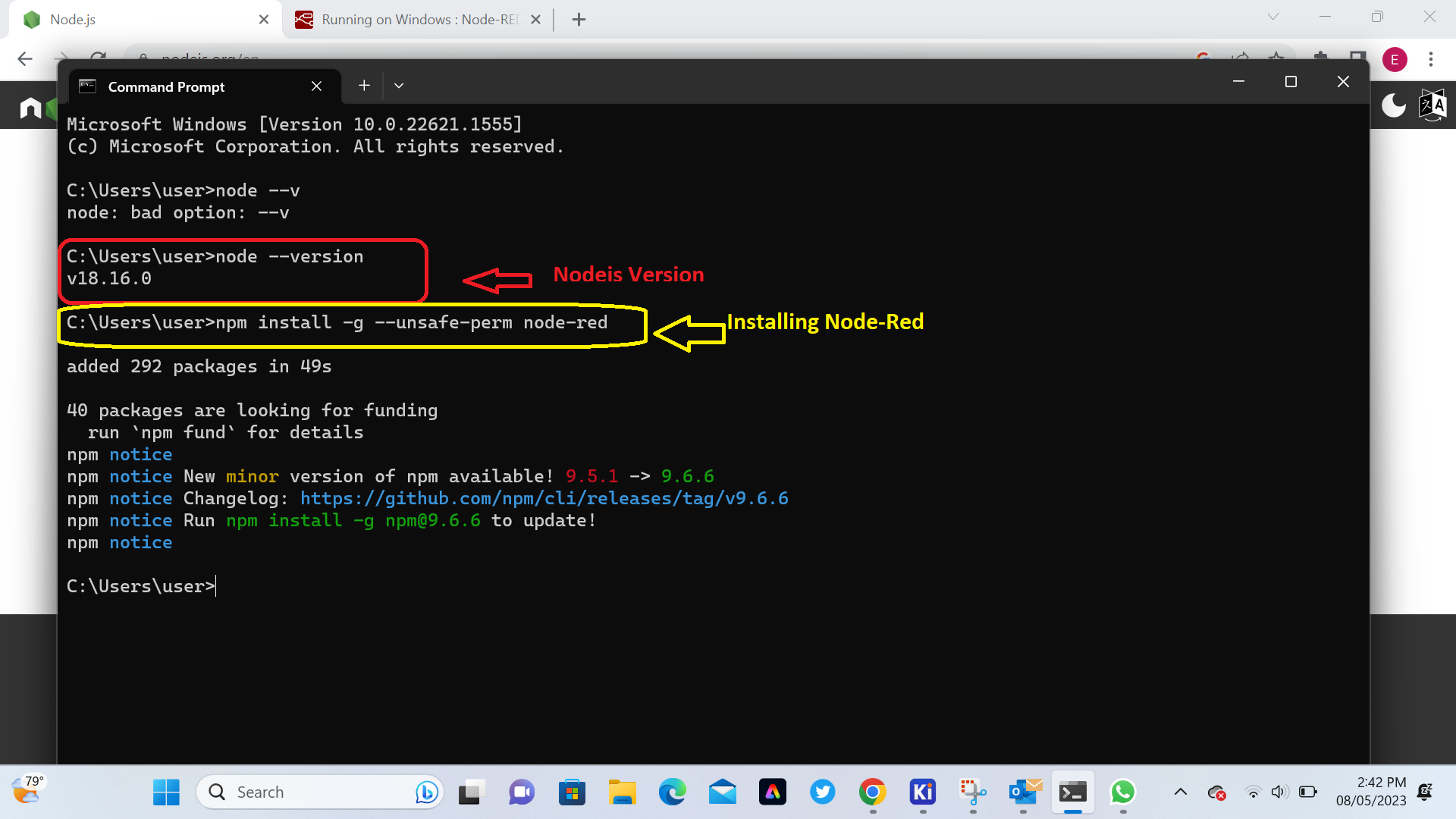
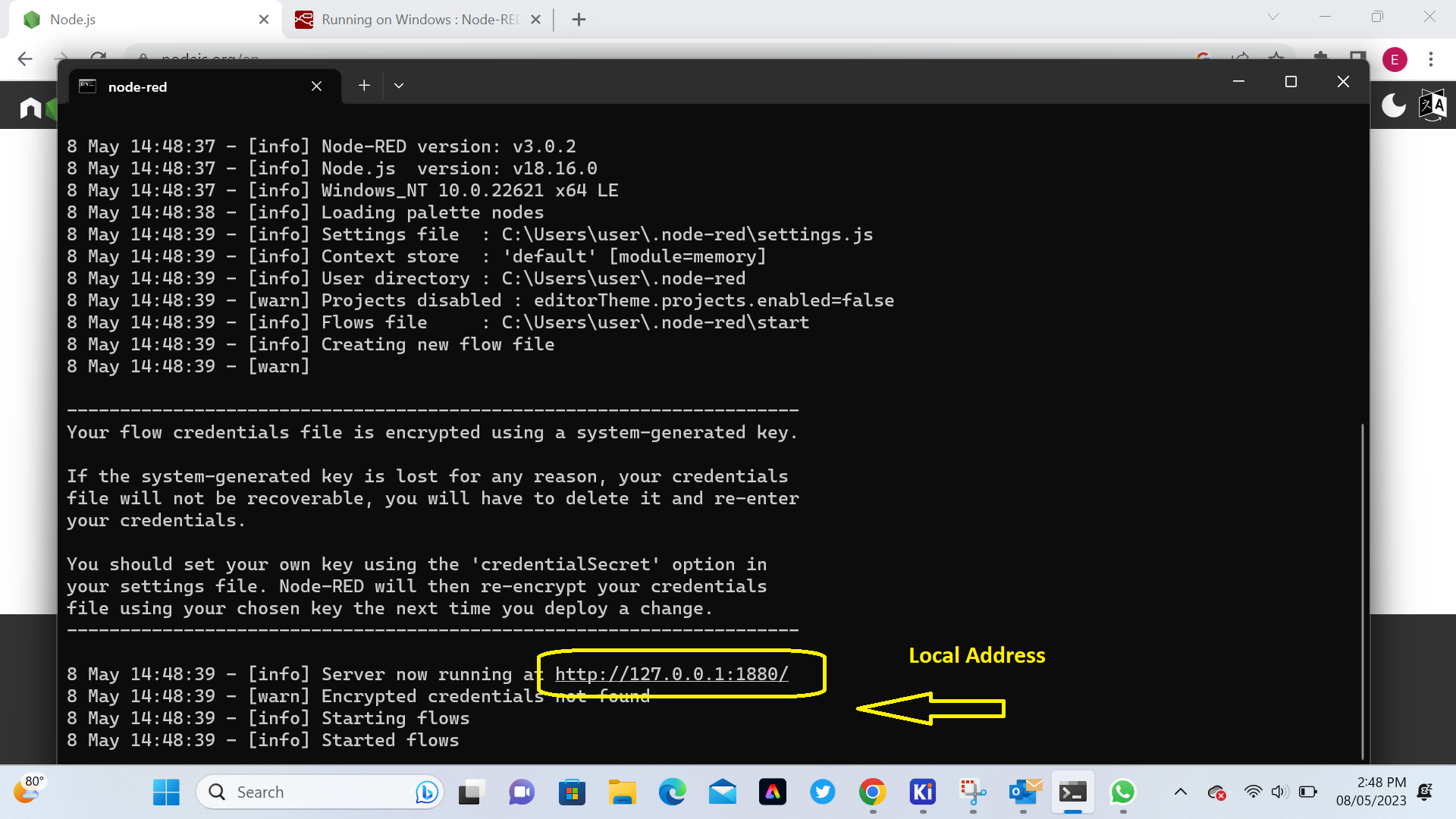
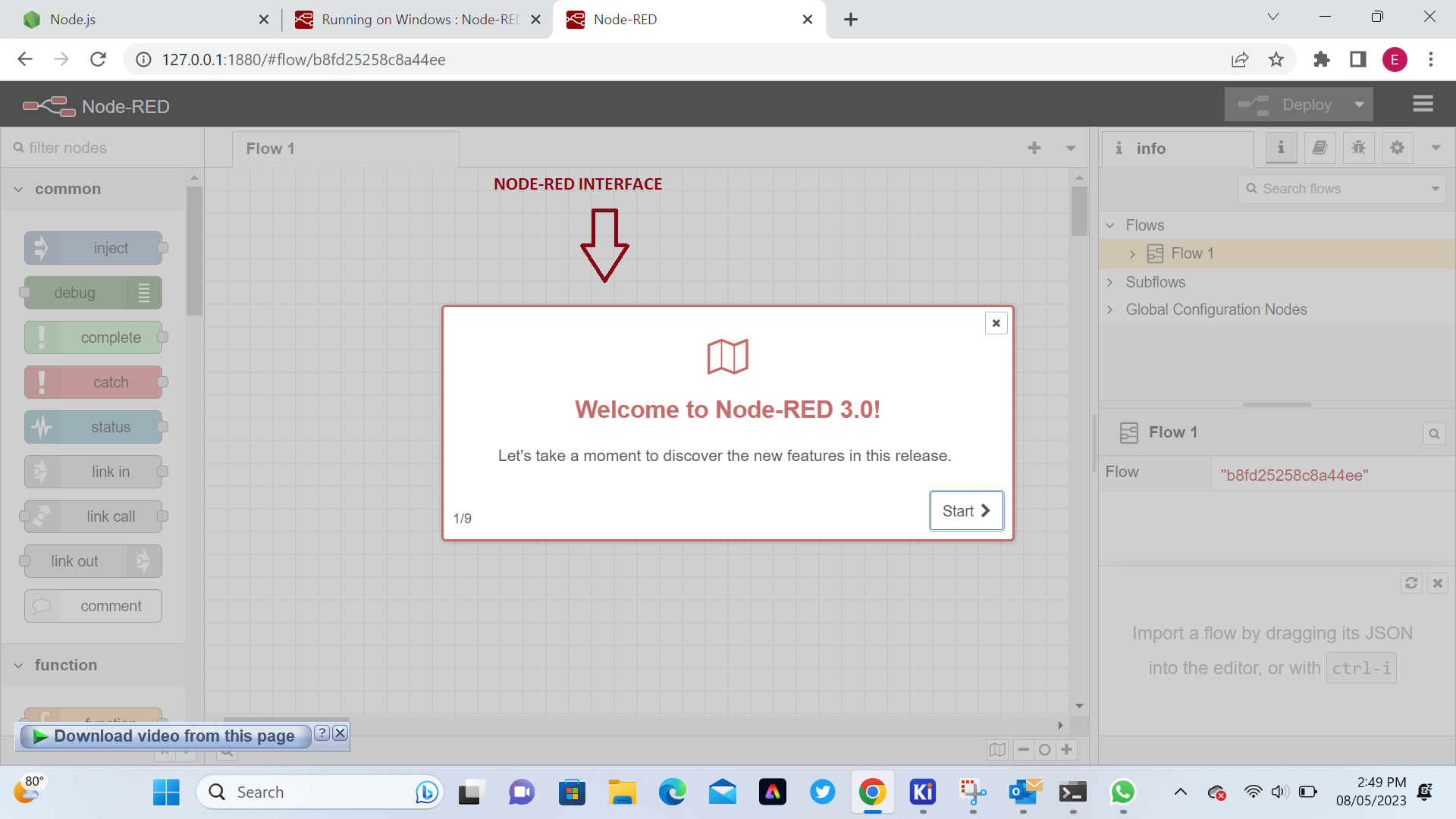
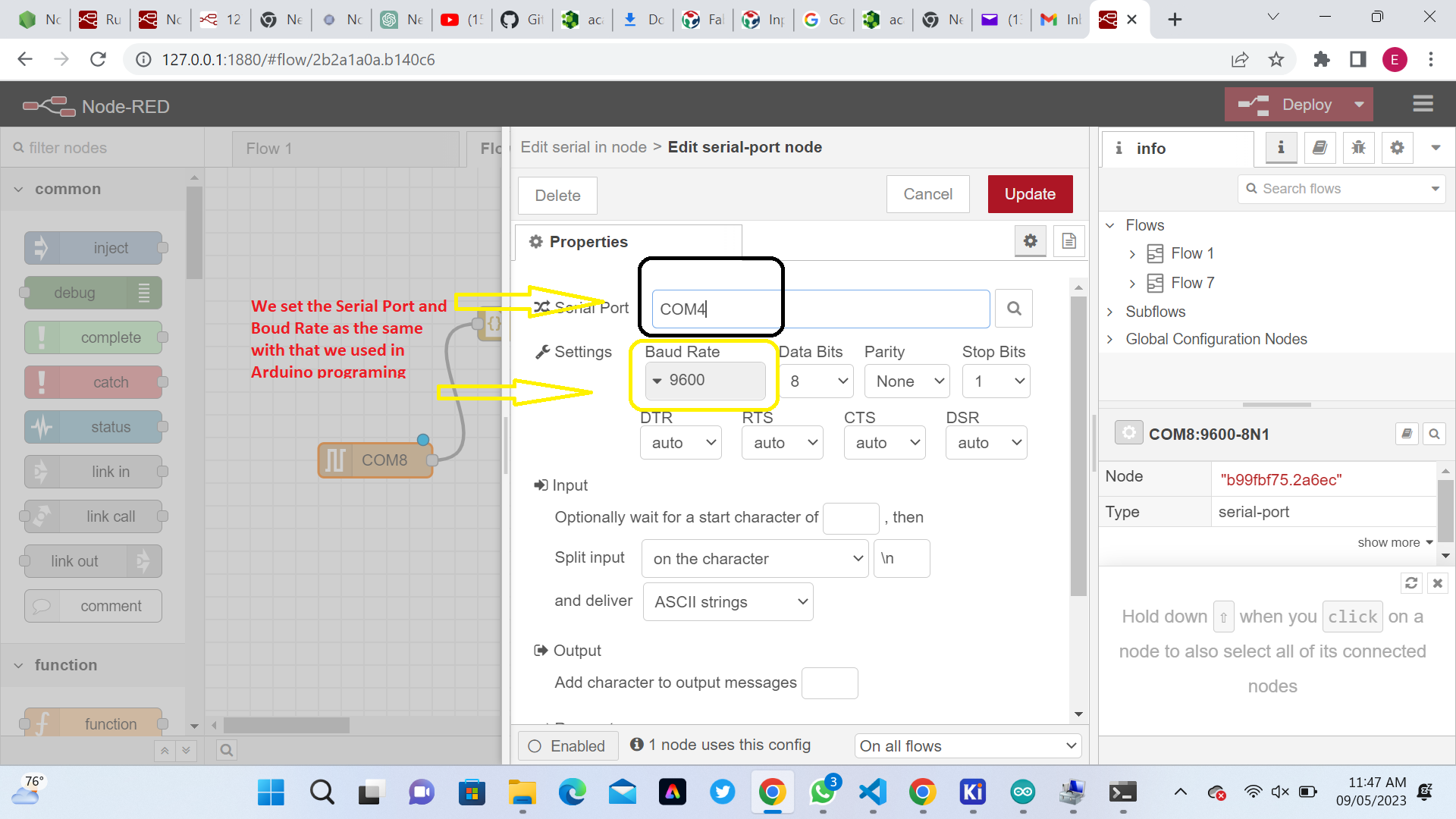
.png)
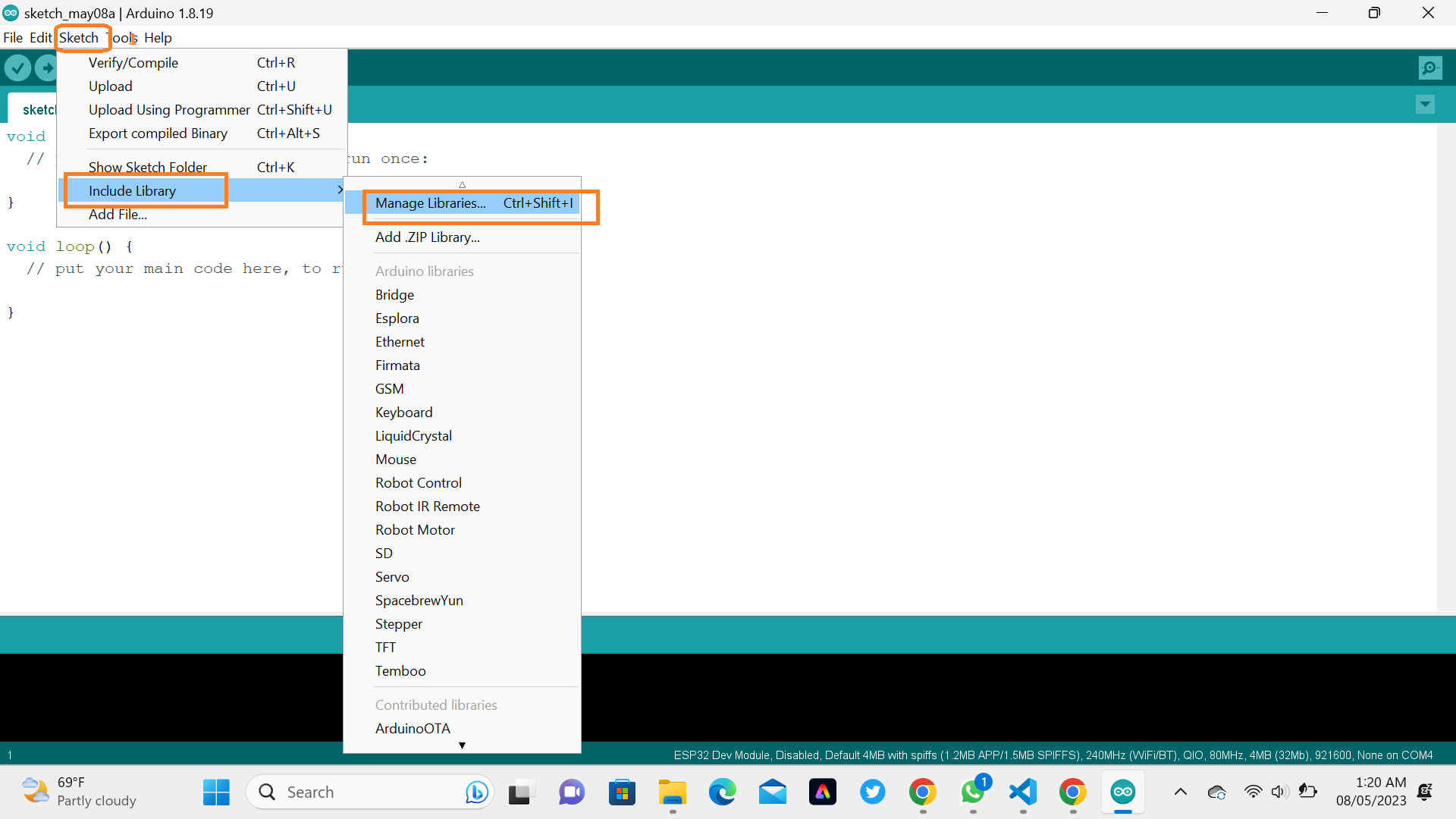
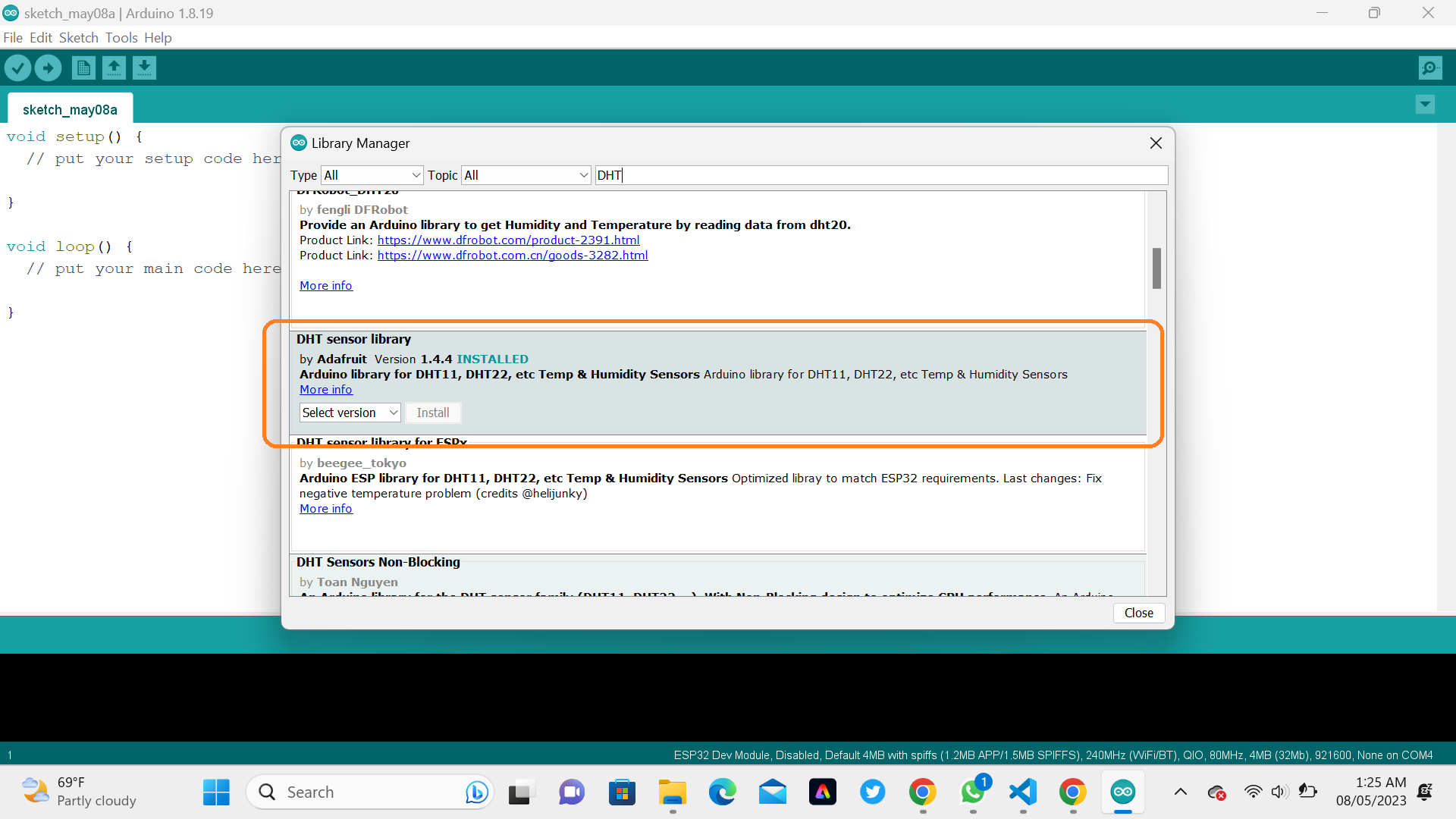
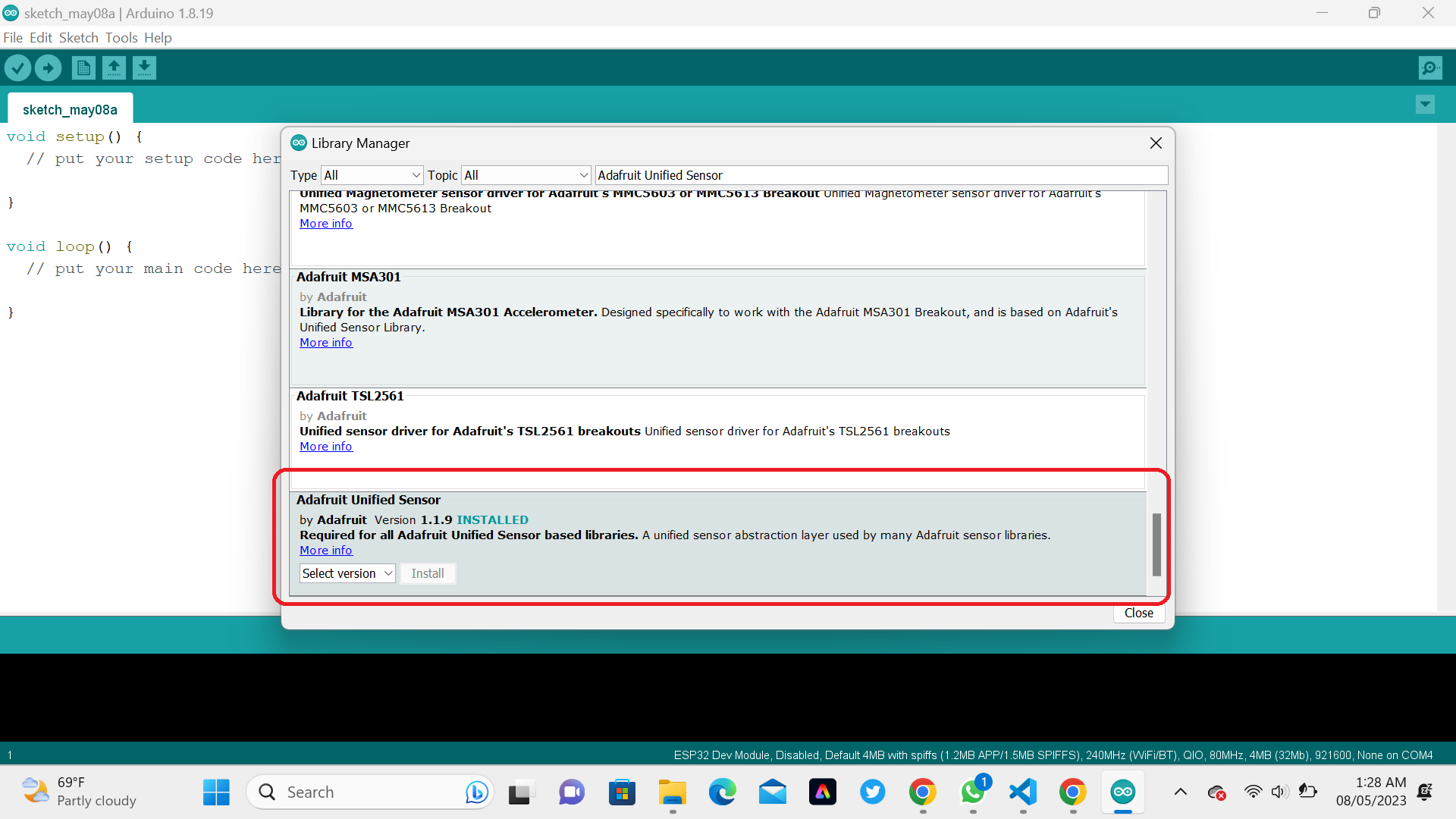
#include "DHT.h"
#define DHTPIN 12
#define DHTTYPE DHT11
DHT dht(DHTPIN, DHTTYPE);
void setup() {
Serial.begin(9600);
dht.begin(); // initialize the sensor
}
void loop() {
// wait a few seconds between measurements.
delay(2000);
// read humidity
float humi = dht.readHumidity();
// read temperature as Celsius
float tempC = dht.readTemperature();
// read temperature as Fahrenheit
float tempF = dht.readTemperature(true);
// check if any reads failed
if (isnan(humi) || isnan(tempC) || isnan(tempF)) {
Serial.println("Failed to read from DHT sensor!");
} else {
Serial.print("{\"temperature\":");
Serial.print(tempC);
Serial.print(",\"humidity\":");
Serial.print(humi);
Serial.println("}");
}
}
Here we are uploading the above Arduino code in ESP32 Microcontroller
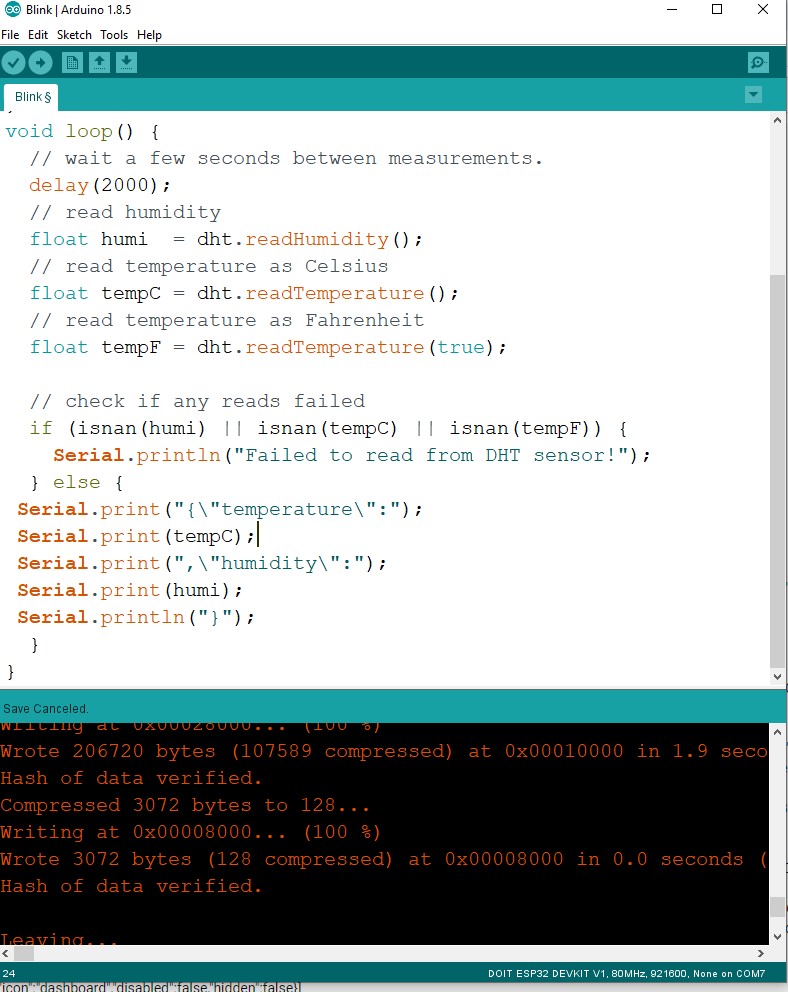
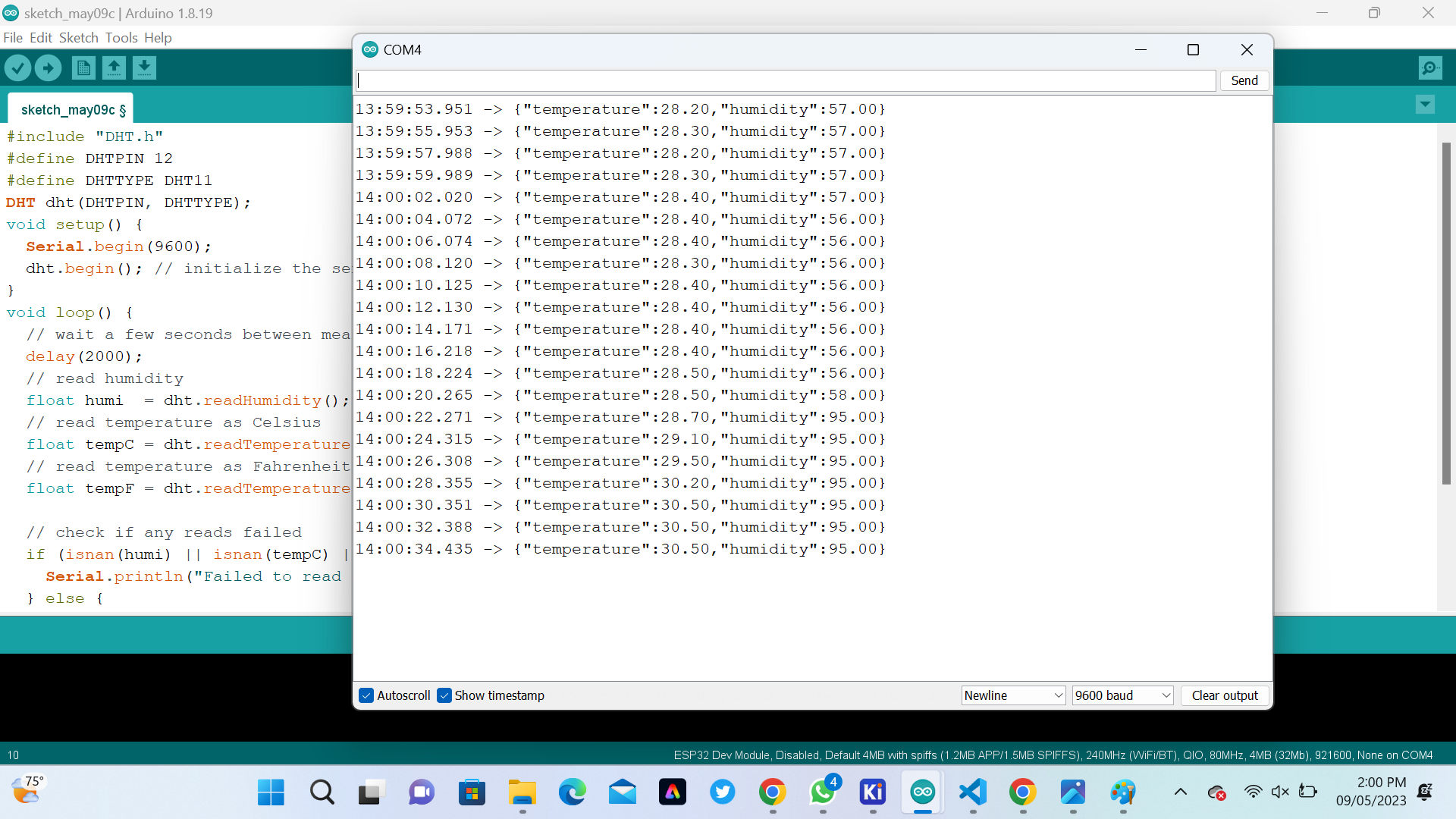
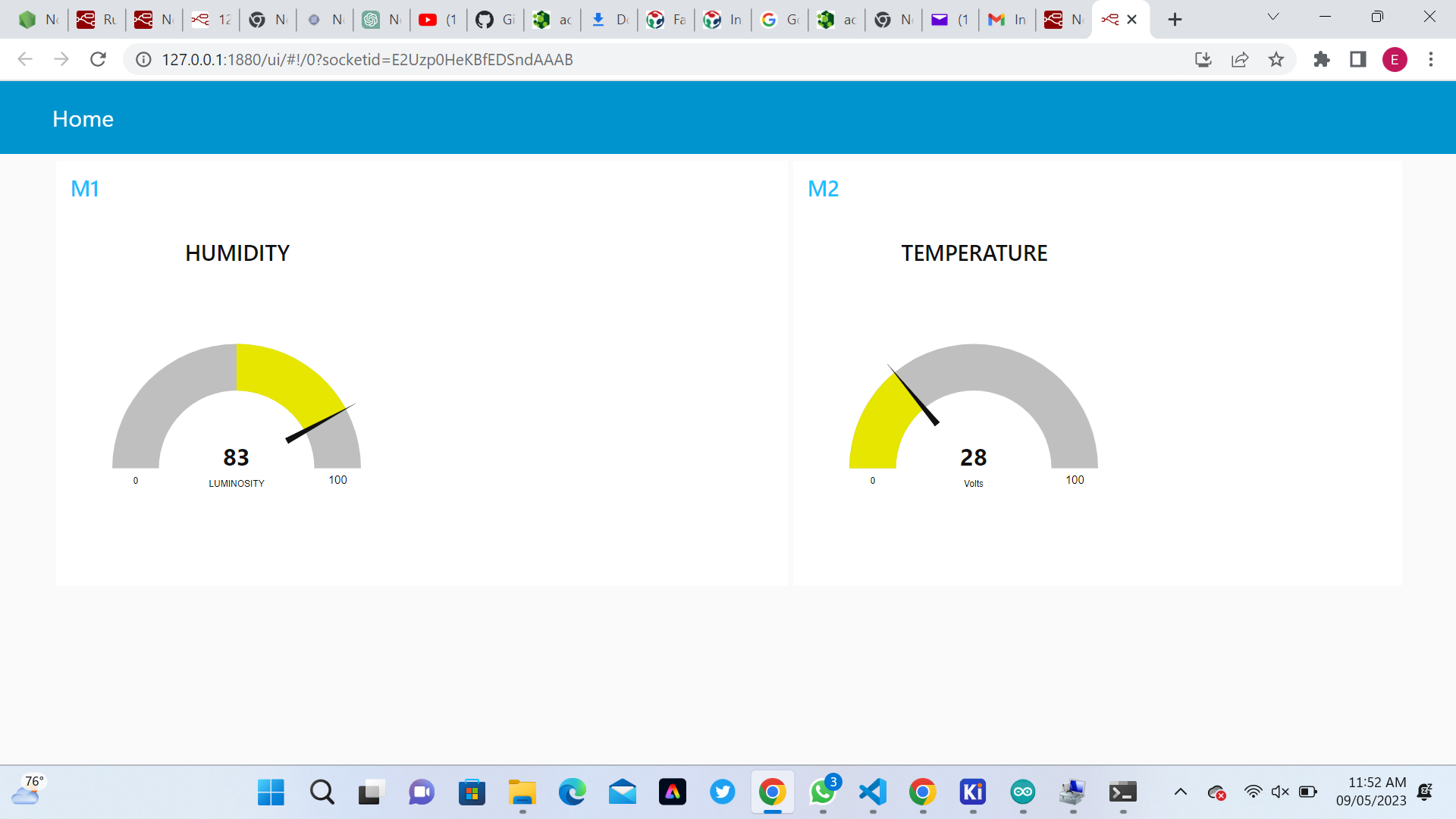
If you want to learn about my Programming kindly click here for download my files