Network and Communication
Exploring ESP32 Wifi and bluetooth features
Intro.
Hello! So final project deadline is near so now as I plan every assignment need to be related to my final project. This is not complusion but neccessary step to complete my goal. I am using ESP32 in My project and wanted to making it IoT device. So here now I will explore more about ESP32, Wifi and internet.
Week Task:
Individual assignment:
design, build, and connect wired or wireless node(s) with network or bus addresses
Group assignment:
send a message between two projects
Group assignment: summary
Here in Group assignment we have done successful communication between two esp32 boards. We have send BME280 temperature and humidity sensor data from one esp32 board to other esp32 board using MAC address to know more...
Individual assignment:
Final Project Board - ESP32
...continued. I am using same board I made in output device week assignment there you will find all orginal design files and steps of making it
.jpg)
.jpg)
WiFi
Wi-Fi is a family of wireless network protocols, based on the IEEE 802.11 family of standards, which are commonly used for local area networking of devices and Internet access, allowing nearby digital devices to exchange data by radio waves. These are the most widely used computer networks in the world, used globally in home and small office networks to link desktop and laptop computers, tablet computers, smartphones, smart TVs, printers, and smart speakers together and to a wireless router to connect them to the Internet, and in wireless access points in public places like coffee shops, hotels, libraries and airports to provide the public Internet access for mobile devices.wikipedia
I refer article on randomnerdtutorials.com for setting up wifi communication with esp32 using WiFi.h library in arduino IDE. It also got nice explanation about how it work that I going to use here.
ESP32 Wi-Fi Modes
The ESP32 board can act as Wi-Fi Station, Access Point or both. To set the Wi-Fi mode, use WiFi.mode()
and set the desired mode as argument:
WiFi.mode(WIFI_STA) station mode: the ESP32 connects to an access point
WiFi.mode(WIFI_AP) access point mode: stations can connect to the ESP32
WiFi.mode(WIFI_STA_AP) access point and a station connected to another access point
Wi-Fi Station
From looking at picture I understand in this mode esp32 comminucate with router and Other devices communicate with esp32 through route
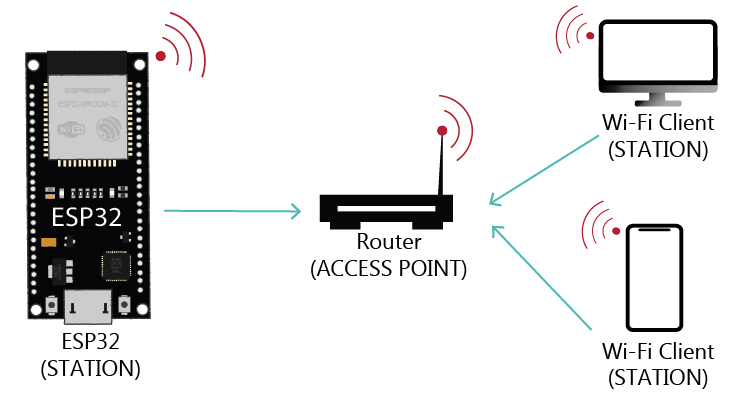
Access Point
Here esp32 and other devices comminucate directly with eachother. so here router dont exist and esp32 itself work as a Access Point
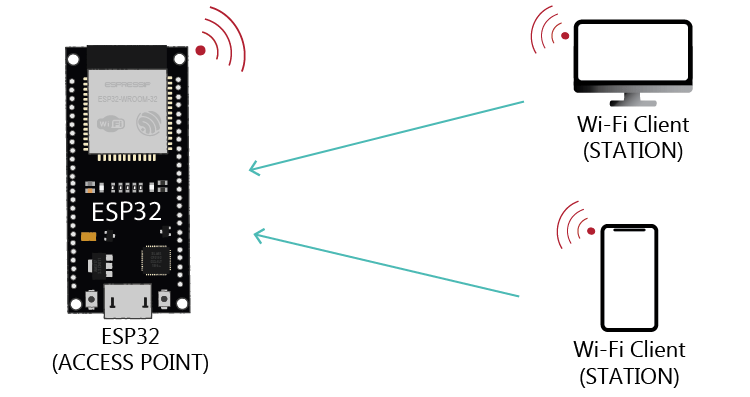
Scan Wi-Fi Networks
In this example esp32 will scan and display active WiFi around his range. Code are available in Arduino IDE, go to File > Examples > WiFi > WiFiScan.
. This will load the sketch for scanning Wifi. Upload it on esp32 board.
/*
* This sketch demonstrates how to scan WiFi networks.
* The API is almost the same as with the WiFi Shield library,
* the most obvious difference being the different file you need to include:
*/
#include "WiFi.h"
void setup()
{
Serial.begin(115200);
// Set WiFi to station mode and disconnect from an AP if it was previously connected
WiFi.mode(WIFI_STA);
WiFi.disconnect();
delay(100);
Serial.println("Setup done");
}
void loop()
{
Serial.println("scan start");
// WiFi.scanNetworks will return the number of networks found
int n = WiFi.scanNetworks();
Serial.println("scan done");
if (n == 0) {
Serial.println("no networks found");
} else {
Serial.print(n);
Serial.println(" networks found");
for (int i = 0; i < n; ++i) {
// Print SSID and RSSI for each network found
Serial.print(i + 1);
Serial.print(": ");
Serial.print(WiFi.SSID(i));
Serial.print(" (");
Serial.print(WiFi.RSSI(i));
Serial.print(")");
Serial.println((WiFi.encryptionType(i) == WIFI_AUTH_OPEN)?" ":"*");
delay(10);
}
}
Serial.println("");
// Wait a bit before scanning again
delay(5000);
}
Here I used given code to scan available Wifi network around me with my esp32 board. I made below video to show it.
Code work fine, it show my board working well.
One thing I noticed sometime board accept code without waiting for me to click button. Thats till now have not given any major problem and I think it maybe because button have be pressed already before and somehow board remember that and response back on that.
In video after Uploading code in my board I look on serial monitor, it showed all available Wifi around I double check on laptop wifi setting and both are same.
int n = WiFi.scanNetworks();
returns the number of networks found.
Serial.print(WiFi.SSID(i));
prints the SSID for a specific network
Serial.print(WiFi.RSSI(i));
returns the RSSI of that network. RSSI stands for Received Signal Strength Indicator. It is an estimated measure of power level that an RF client device is receiving from an access point or router.
Get WiFi Connection Strength
/*
Complete details at https://RandomNerdTutorials.com/esp32-useful-wi-fi-functions-arduino/
*/
#include <WiFi.h>
// Replace with your network credentials (STATION)
const char* ssid = "REPLACE_WITH_YOUR_SSID";
const char* password = "REPLACE_WITH_YOUR_PASSWORD";
void initWiFi() {
WiFi.mode(WIFI_STA);
WiFi.begin(ssid, password);
Serial.print("Connecting to WiFi ..");
while (WiFi.status() != WL_CONNECTED) {
Serial.print('.');
delay(1000);
}
Serial.println(WiFi.localIP());
}
void setup() {
Serial.begin(115200);
initWiFi();
Serial.print("RRSI: ");
Serial.println(WiFi.RSSI());
}
void loop() {
// put your main code here, to run repeatedly:
}
Above code is to find signal strength of a connect network. Here I connected it with my mobile. In below image it show negative number is to show strength. How big the number by ignoring negative sign, network is that much weak.
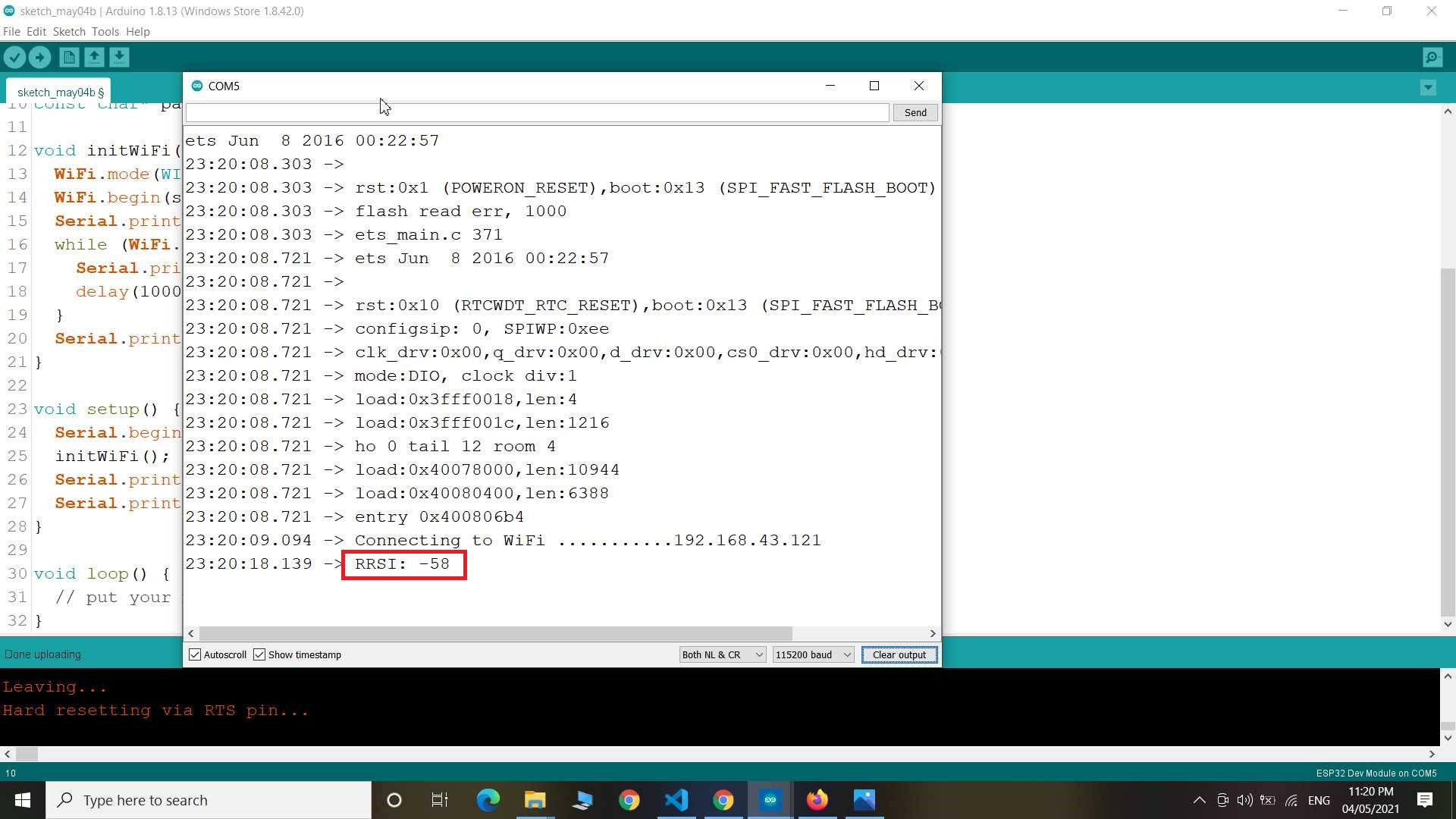
Reconnect to Wi-Fi Network After Lost Connection (Wi-Fi Events)
/*
Rui Santos
Complete project details at https://RandomNerdTutorials.com/solved-reconnect-esp32-to-wifi/
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files.
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
*/
#include <WiFi.h>
const char* ssid = "REPLACE_WITH_YOUR_SSID";
const char* password = "REPLACE_WITH_YOUR_PASSWORD";
void WiFiStationConnected(WiFiEvent_t event, WiFiEventInfo_t info){
Serial.println("Connected to AP successfully!");
}
void WiFiGotIP(WiFiEvent_t event, WiFiEventInfo_t info){
Serial.println("WiFi connected");
Serial.println("IP address: ");
Serial.println(WiFi.localIP());
}
void WiFiStationDisconnected(WiFiEvent_t event, WiFiEventInfo_t info){
Serial.println("Disconnected from WiFi access point");
Serial.print("WiFi lost connection. Reason: ");
Serial.println(info.disconnected.reason);
Serial.println("Trying to Reconnect");
WiFi.begin(ssid, password);
}
void setup(){
Serial.begin(115200);
// delete old config
WiFi.disconnect(true);
delay(1000);
WiFi.onEvent(WiFiStationConnected, SYSTEM_EVENT_STA_CONNECTED);
WiFi.onEvent(WiFiGotIP, SYSTEM_EVENT_STA_GOT_IP);
WiFi.onEvent(WiFiStationDisconnected, SYSTEM_EVENT_STA_DISCONNECTED);
/* Remove WiFi event
Serial.print("WiFi Event ID: ");
Serial.println(eventID);
WiFi.removeEvent(eventID);*/
WiFi.begin(ssid, password);
Serial.println();
Serial.println();
Serial.println("Wait for WiFi... ");
}
void loop(){
delay(1000);
}
After loading the code in my esp32 board. I check arduino ide serial monitor it start to show some text that it try to reconnect wifi.
Here I have used my mobile phone Hotshop which is off now and my esp32 is trying to connect it as show in first picture. Where in second picture it show connected to network and even give out IP address because here I turn on my Hotshop on and my esp32 device found it and got connected .
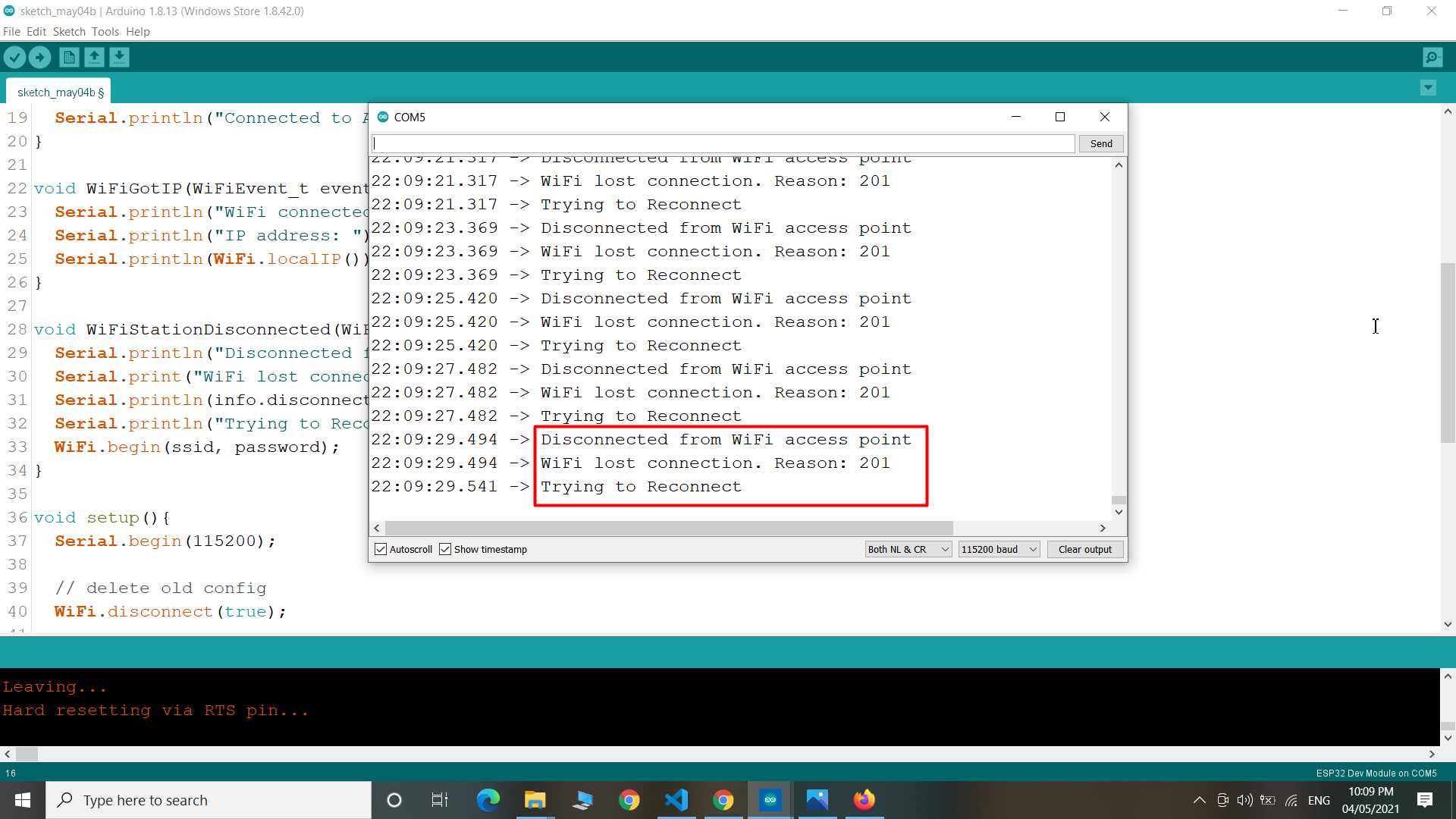
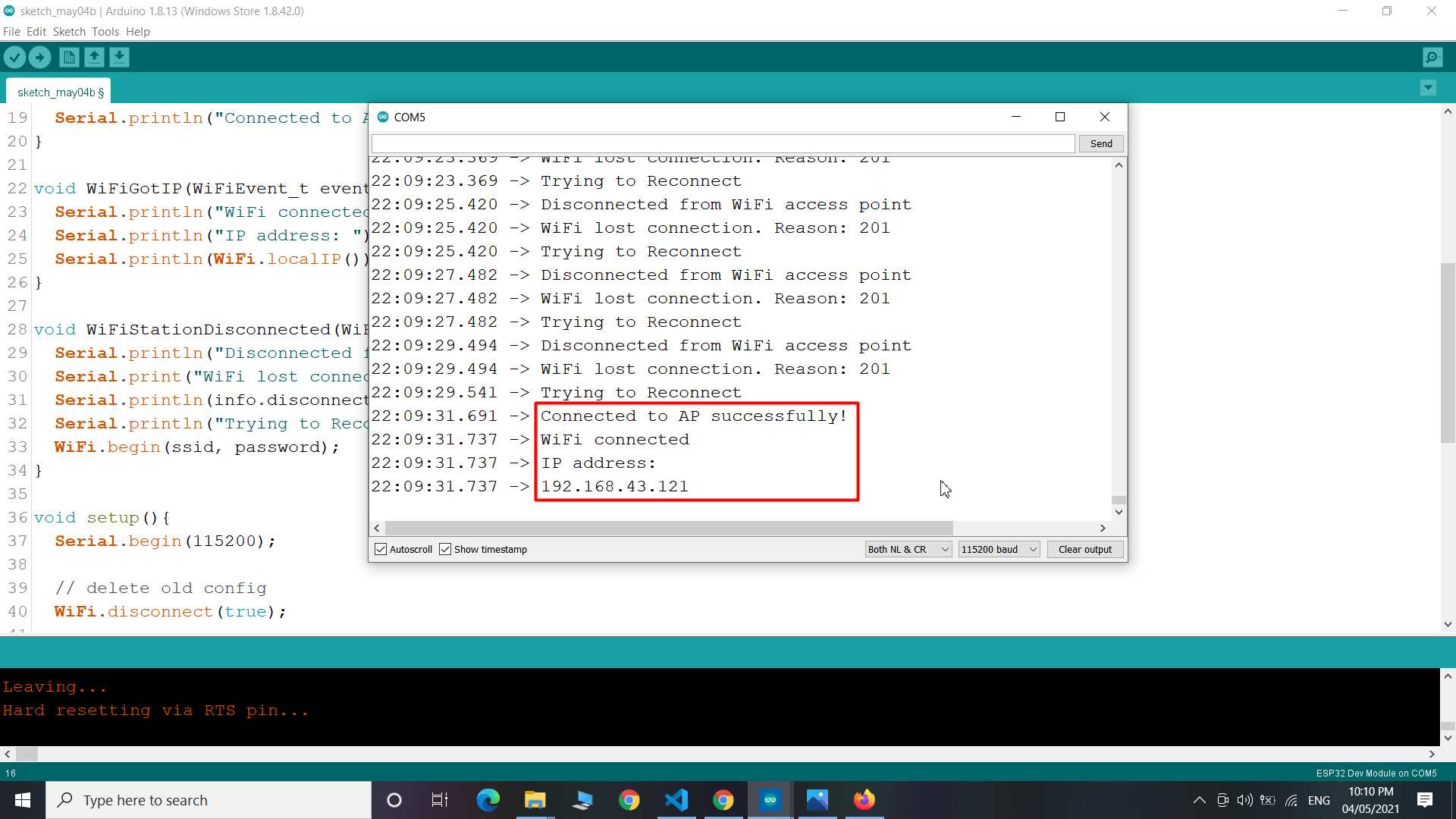
Then to check I again disconnect my phone and it started to show reconnecting msg.
Then I again turn on my mobile Hotshop and It got connected as quick as possible compare to trying to connect it back manually. This is good because Unkowningly if it get disconnect due to any reason it will auto connect to network when it get available.
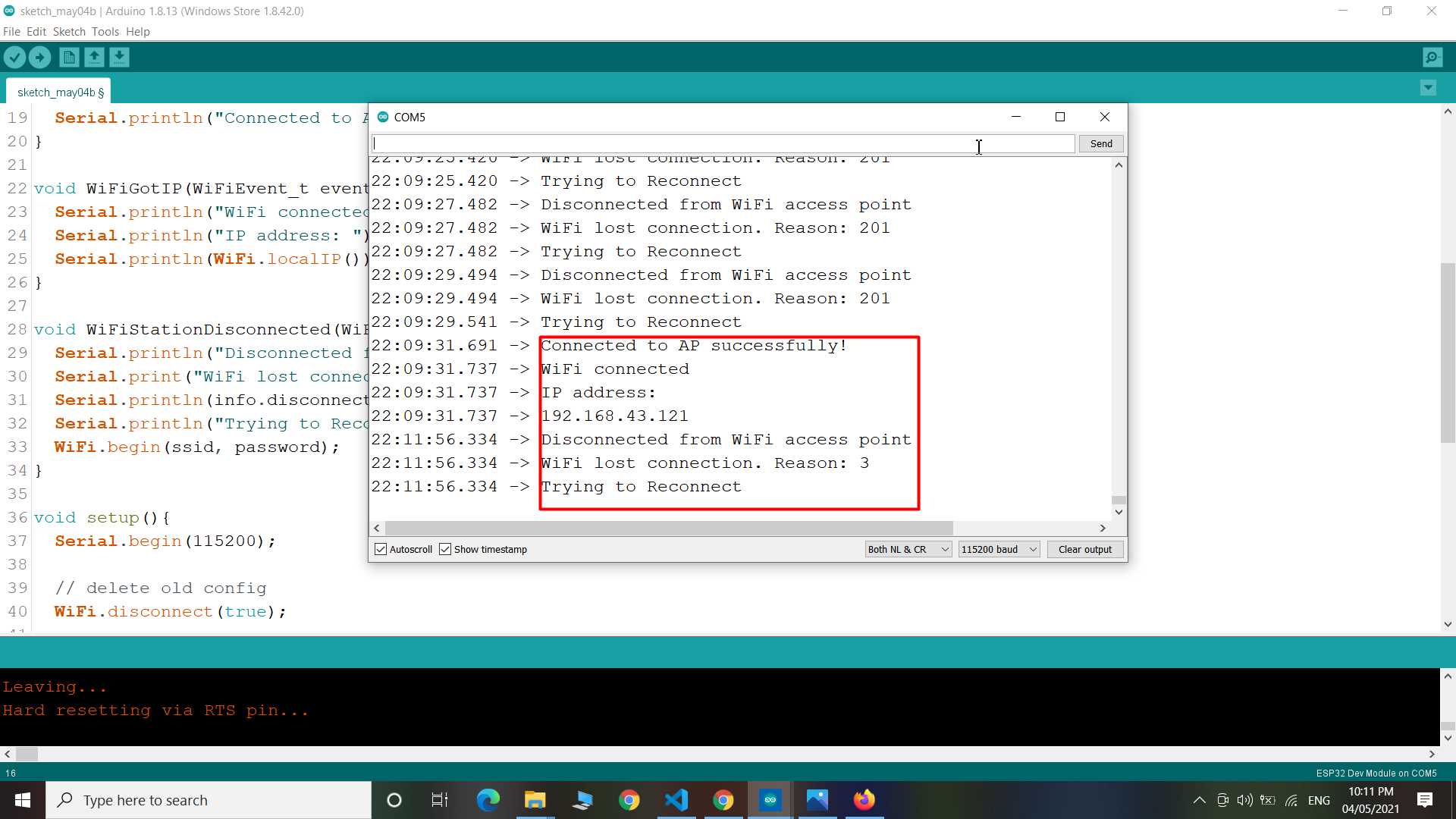
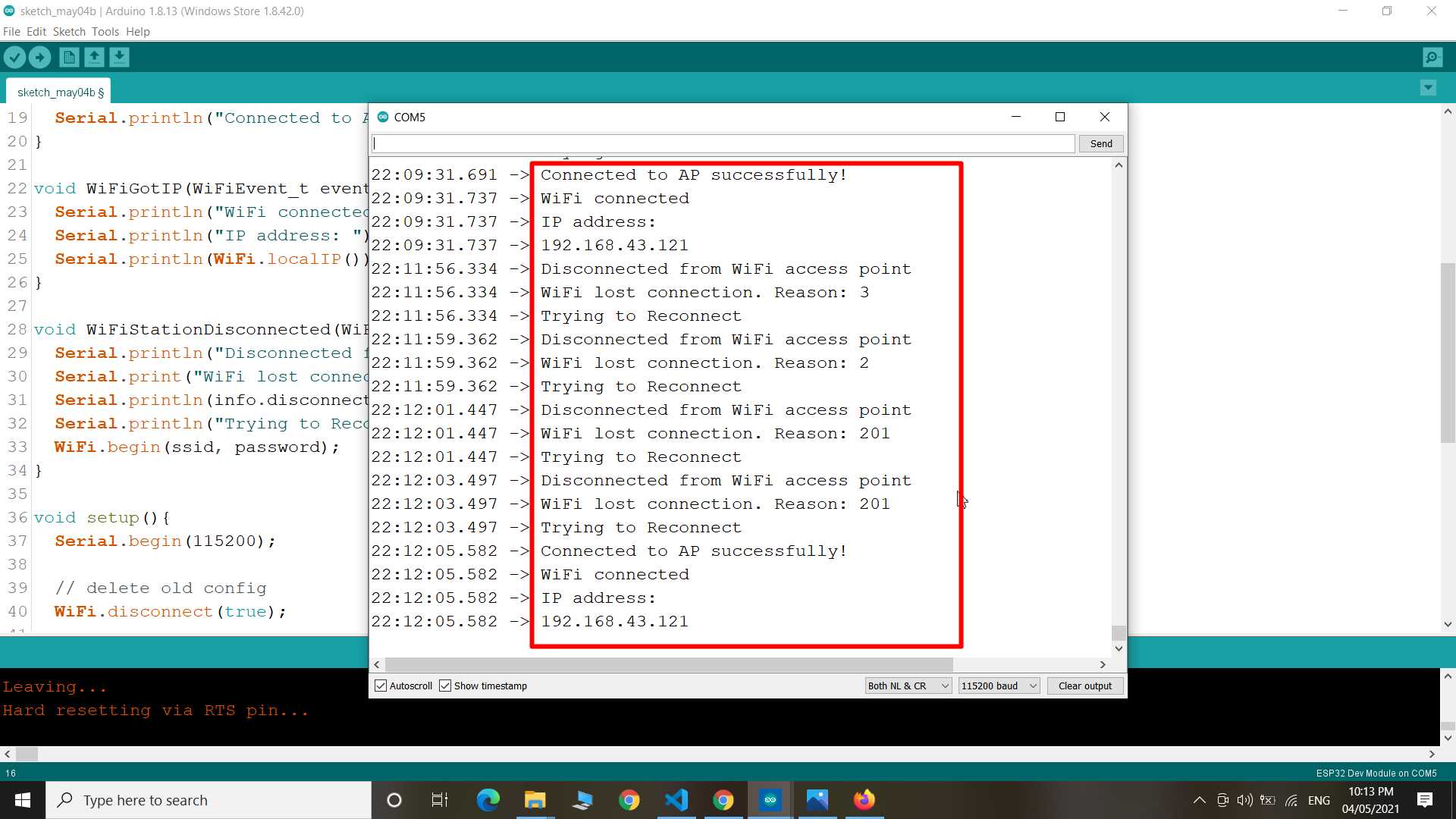
ESP32 Bluetooth Comminucation
Bluetooth is a short-range wireless technology standard used for exchanging data between fixed and mobile devices over short distances using UHF radio waves in the ISM bands, from 2.402 GHz to 2.48 GHz, and building personal area networks (PANs). It was originally conceived as a wireless alternative to RS-232 data cables. wikipedia
Some tutorial I found on randomnerdtutorials.com and followed that with my board.
Here I have perform example for Bluetooth Comminucation between my smartphone and board + laptop
I have not connected any kind of display so I using computer serial monitor in arduino ide to see and to send text. So here phone and board are comminucating through bluetooth protocol which wireless and my esp32 board and my laptop is Communicating through serial communication using usb to serial converter that I made in my electronic production week.
This code I uploaded in esp32 board
//This example code is in the Public Domain (or CC0 licensed, at your option.)
//By Evandro Copercini - 2018
//
//This example creates a bridge between Serial and Classical Bluetooth (SPP)
//and also demonstrate that SerialBT have the same functionalities of a normal Serial
#include "BluetoothSerial.h"
#if !defined(CONFIG_BT_ENABLED) || !defined(CONFIG_BLUEDROID_ENABLED)
#error Bluetooth is not enabled! Please run `make menuconfig` to and enable it
#endif
BluetoothSerial SerialBT;
void setup() {
Serial.begin(115200);
SerialBT.begin("ESP32Bth"); //Bluetooth device name
Serial.println("The device started, now you can pair it with bluetooth!");
}
void loop() {
if (Serial.available()) {
SerialBT.write(Serial.read());
}
if (SerialBT.available()) {
Serial.write(SerialBT.read());
}
delay(20);
}
SerialBT.begin("ESP32Bth"); //Bluetooth device name
this syntax is to give name to your esp32 bluetooth.
Here I used random app for serial bluetooth Comminucation in Smartphone from google playstore to send and receive text through bluetooth device. I checked it with HC-05 bluetooth module and worked find there. It detected my esp32 board bluetooth so try if it work and it worked same like with HC-05. I send
.png)
.png)
.png)
Here on other side to the desktop it showed that esp32 board received msg and push forward to serial comminucation on desktop which we see in arduino ide serial monitor.
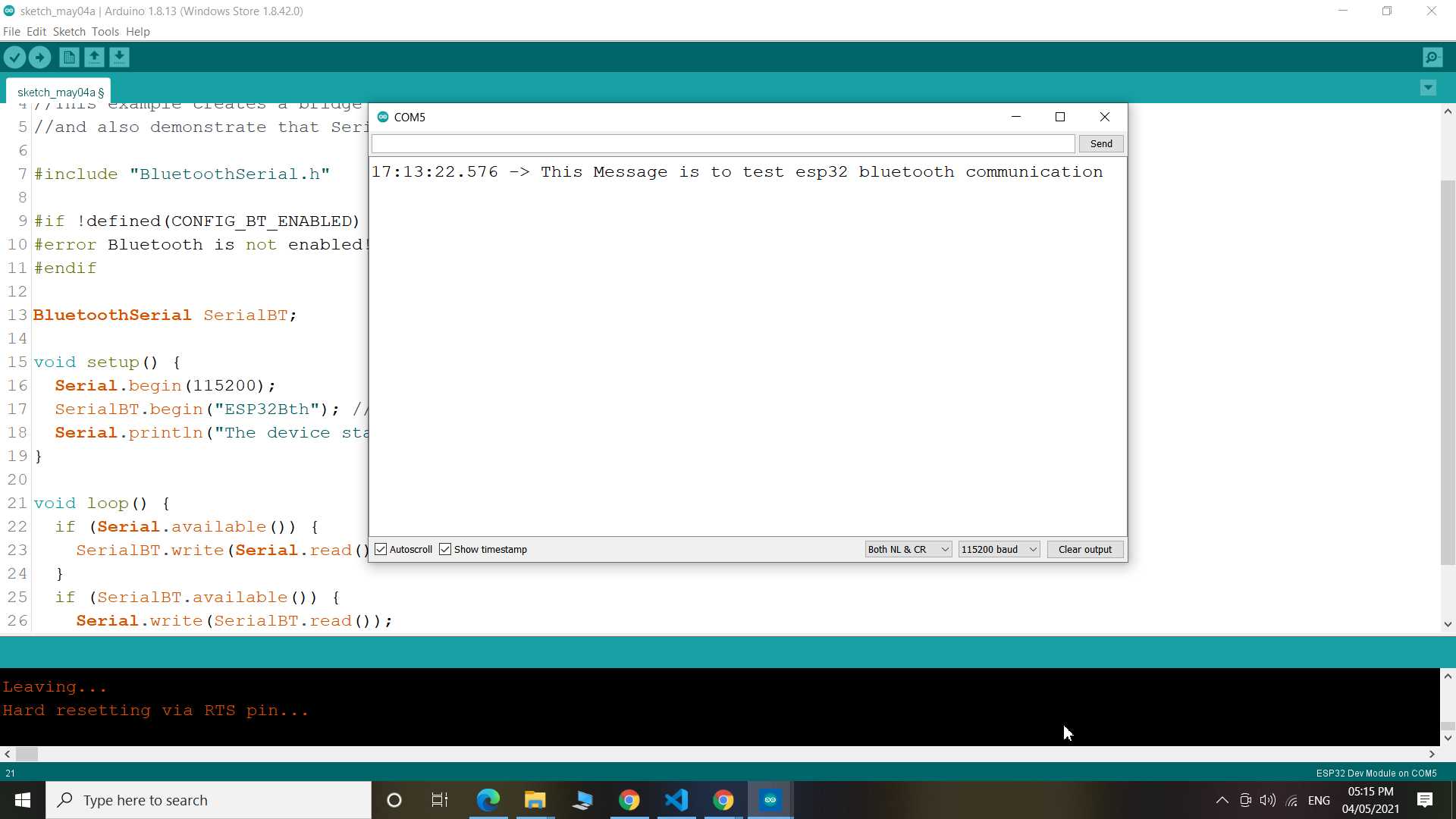
Learning Outcomes:
ESP32 is really multi application chip very useful for project needed wifi or bluetooth or both comminucation. It have those feature embedded in it . I here have tried this all feature because I wanted to learn it and implement it in my final project.