9. Input Devices
This week I read the data from an accelerometer I connected to the PCB I made last week via I2C. We also did a group assignment which you can find here.
To build the code, I searched for the accelerometer's datasheet. I used the MPU-6050 sensor, which can provide accelerometer data and a gyroscope.
I had little experience using this communication protocol, but I knew I had to find the address to connect with the accelerometer, find how to activate the sensor, and indicate the data I wanted to collect.
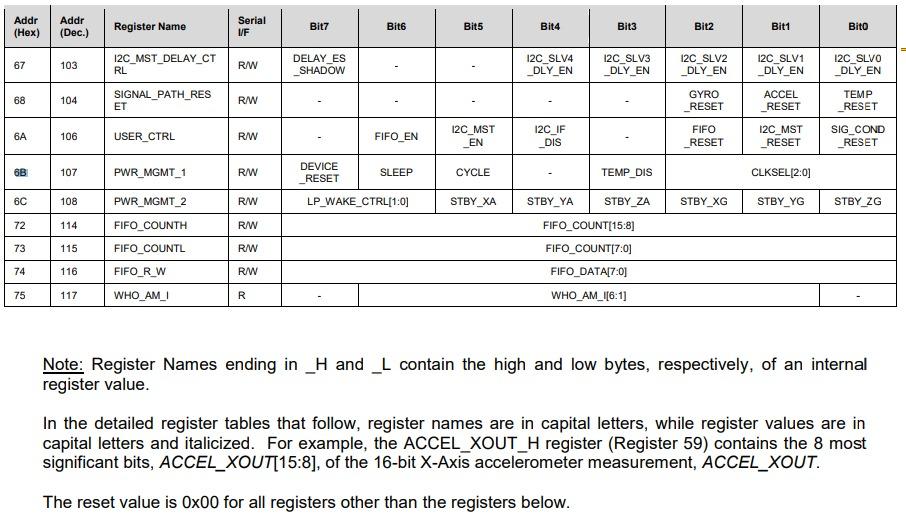
I also searched how to configure the sensor to read the accelerometer data:

Using as a guide the following code provided by my local instructor I built my code:
#include <Wire.h>
#define DS1307_ADDR 0x68 // I2C address of DS1307
void setup() {
Serial.begin(115200);
Serial.println("DS1307 RTC Test");
Wire.begin();
}
void loop() {
/* Read time from DS1307 */
Wire.beginTransmission(DS1307_ADDR); // Step 1: Start communication
Wire.write(0x00); // Step 2: Point to seconds register
Wire.endTransmission(false); // Step 3: Keep connection open
Wire.requestFrom(DS1307_ADDR, 3); // Step 4: Request 3 bytes (seconds, minutes, hours)
if (Wire.available() >= 3) { // Step 5: Check if data is available
byte segundos = dec(Wire.read()); // Step 6: Read seconds
byte minutos = dec(Wire.read()); // Step 7: Read minutes
byte horas = dec(Wire.read()); // Step 8: Read hours
// Print time in HH:MM:SS format
Serial.print(horas);
Serial.print(":");
Serial.print(minutos);
Serial.print(":");
Serial.println(segundos);
}
delay(1000); // Wait 1 second before next reading
}
// Convert BCD to decimal
byte dec(byte val) {
return (val / 16 * 10) + (val % 16);
}
I made this code to try to make a connection with the sensor:
#include <Wire.h>
const int MPU_ADDR = 0x68; // I2C address of MPU-6050
const int SDA_PIN = 21; // SDA pin (GPIO 21)
const int SCL_PIN = 22; // SCL pin (GPIO 22)
int16_t AcX, AcY, AcZ; // Variables to store accelerometer data
void setup() {
Serial.begin(115200);
Wire.begin(SDA_PIN, SCL_PIN); // Initialize I2C with specified pins
Wire.beginTransmission(MPU_ADDR);
Wire.write(0x6B); // PWR_MGMT_1 register
Wire.write(0x00); // Activate sensor
Wire.endTransmission(true);
}
void loop() {
// Read accelerometer data
Wire.beginTransmission(MPU_ADDR);
Wire.write(0x3B); // ACCEL_XOUT_H register
Wire.write(0x1C); // ACCEL_CONFIG
Wire.endTransmission(false);
Wire.requestFrom(MPU_ADDR, 6, true); // Request 6 registers (2 per axis)
// Read data and store in variables
AcX = Wire.read() << 8 | Wire.read(); // X-axis acceleration
AcY = Wire.read() << 8 | Wire.read(); // Y-axis acceleration
AcZ = Wire.read() << 8 | Wire.read(); // Z-axis acceleration
// Print the results
Serial.print("X: "); Serial.print(AcX); Serial.println(" m/s^2");
Serial.print("Y: "); Serial.print(AcY); Serial.println(" m/s^2");
Serial.print("Z: "); Serial.print(AcZ); Serial.println(" m/s^2");
delay(1000); // Wait 1 second before next reading
}
The hardware I2C pins for the ESP32 are GPIO 22 (SCL) and GPIO 21 (SDA), although I could use most of the other pins for I2C communication, I decided to use the default ones. I connected the SDA pin to the SDA pin of the accelerometer and the SCL pin to the SCL pin of the accelerometer. I also connected the VCC pin of the accelerometer to 3.3v and the GND pin to GND.
After uploading this code into ESP32, I opened the Serial Monitor on the Arduino IDE to see if the communication was successful, but none of the axes showed a value. After doing some research, I found that I needed to add some resistors to the SDA and SCL pins and connect them to 3.3v. The recommended values were 4.7k ohm for both pins. I didn't have that exact value so I used 5k resistors. After making the connections I ran the code again and the following values appeared:
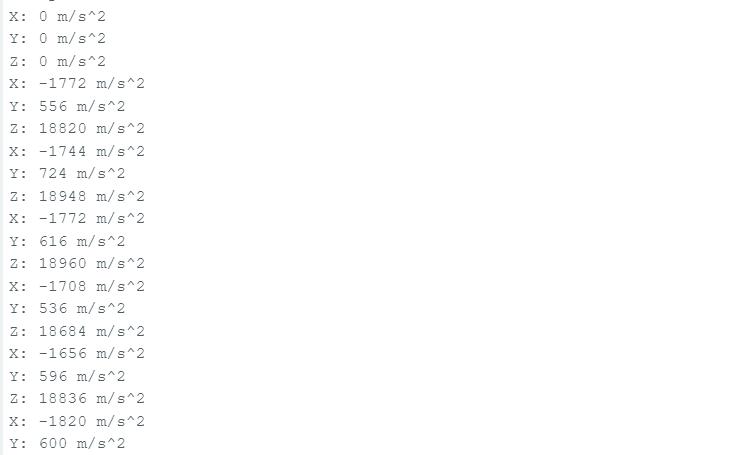
I knew the values were odd, on the z-axis it should show something like the Earth's gravity, so after reading more into the datasheet I found that I had to specify the scale range:
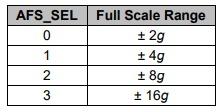
I used the 2g scale and although I did know I had to convert the information from the accelerometer I had to make some changes to convert the values correctly I asked an AI chatbot to help me with that part and here's what it gave to me:
// Convert to gravity (g) and then to m/s² (1g = 9.81 m/s²)
float g = 9.81; // Aceleración gravitacional
float range = 2.0; // Configured range (±2g)
float scale = range / 32768.0; // Scale for 16 bits
float AccX = AcX * scale * g;
float AccY = AcY * scale * g;
float AccZ = AcZ * scale * g;
And here's the final code:
#include <Wire.h>
const int MPU_ADDR = 0x68; //page 8
const int SDA_PIN = 21;
const int SCL_PIN = 22;
void setup() {
Serial.begin(115200);
Wire.begin(SDA_PIN, SCL_PIN);
// Start MPU-6050
Wire.beginTransmission(MPU_ADDR);
Wire.write(0x6B); // PWR_MGMT_1 page 8
Wire.write(0x00); // Activate sensor page8
Wire.endTransmission();
// Configurate acelerometer range (±2g)
Wire.beginTransmission(MPU_ADDR);
Wire.write(0x1C); // ACCEL_CONFIG page 15
Wire.write(0x00); // ±2g page 15
Wire.endTransmission();
}
void loop() {
// Read raw data
Wire.beginTransmission(MPU_ADDR);
Wire.write(0x3B); // ACCEL_XOUT_H
Wire.endTransmission(false);
Wire.requestFrom(MPU_ADDR, 6, true);
int16_t AcX = Wire.read() << 8 | Wire.read();
int16_t AcY = Wire.read() << 8 | Wire.read();
int16_t AcZ = Wire.read() << 8 | Wire.read();
// Convert to m/s² (±2g)
float AccX = AcX * (2.0 / 32768.0) * 9.81;
float AccY = AcY * (2.0 / 32768.0) * 9.81;
float AccZ = AcZ * (2.0 / 32768.0) * 9.81;
Serial.print("X: "); Serial.print(AccX); Serial.print(" m/s² | ");
Serial.print("Y: "); Serial.print(AccY); Serial.print(" m/s² | ");
Serial.print("Z: "); Serial.print(AccZ); Serial.println(" m/s²");
delay(500);
}
Here's a video of the code working:
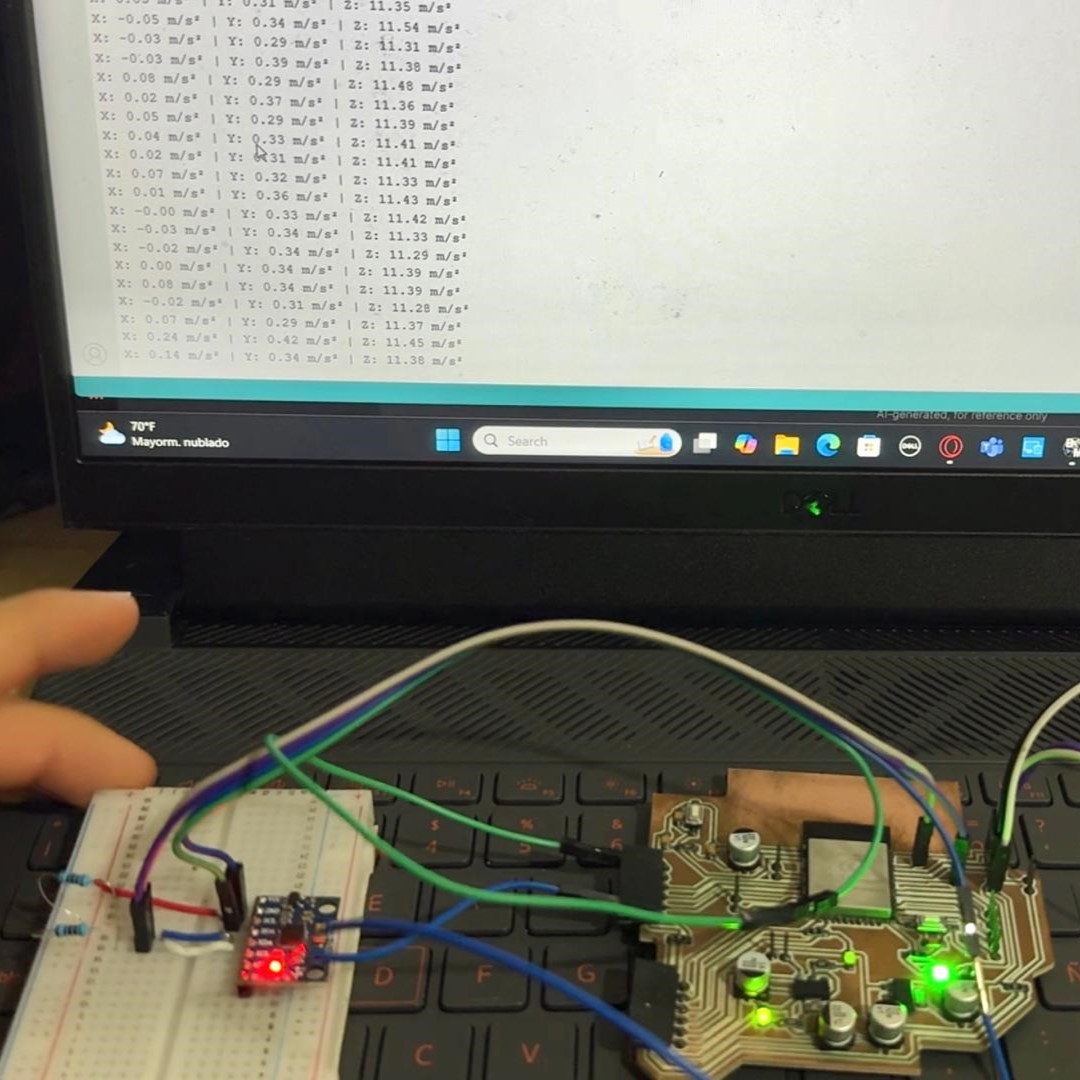
Summary
This week I learned how to obtain the data from the MPU-6050 accelerometer using I2C communication. The process involved:
- Identifying the correct I2C address (0x68)
- Adding pull-up resistors to the SDA and SCL lines (5kΩ in my case)
- Properly configuring the accelerometer range (±2g)
- Converting the raw sensor data to meaningful units (m/s²)
The most challenging part was understanding the data conversion process, but with help from the datasheet and some research, I was able to get accurate acceleration readings. I2C is a very useful communication protocol that allows us to connect multiple devices to a single bus, and it's widely used in embedded systems. I'm excited to continue exploring this protocol and using it in future projects.