Embedded Programming
On this week assignment I am going to write a program in to different lenguajes (Arduino-C++ and Python) for the PCB we made on our week 4 assignment. What I am going to code is a Morse translator using one button and one LED.
Programming the board
Before start coding, we have to setup the Xiao and the software we want to use.
Arduino (C++)
On week 4 I show you how to set everything up for the Arduino, once you did those steps follow these ones.
Python
To codify con python first we need to download a software with this lenguaje, in my case, Mu.
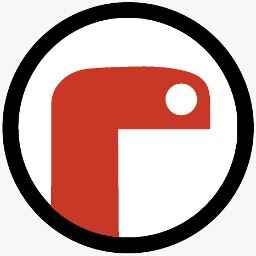
Segmented code and differences
On our group assignment we learn the differences when coding between C++ and Python, for example: variables, functions, even the way you comment.
Lets break apart the code so I can explain you how it works, also, while I was coding I saw a lot of differences too that I am going to share with you.
Functions, GPIO, delays/sleeps
On this parts of the code, I defined the funtcions dot and line. It works by using the delay/sleep, first the LED turns on and stays that way depending on the amount of delay I set, for the dot it was half of a second and for the line was a full second.
In general, the functions kinda work the same, in Python functions are defined using the def keyword and in C++ you must specify the return type explicitly.
The writing a pin is also kinda similar, the diffrences are how you write it, in C++ we use "HIGH" (LED ON) and "LOW" (LED OFF), and in Python use "True" (LED ON) and "False" (LED OFF), also you need to import the library "digitalio".
The timers are use to help you manage when and how often a part of the code runs. In C++ we used delay() and it works in milliseconds, in Python, first we need to import the library "time"; we used time.sleep() and it works in seconds. A tip in Python is to import it as this way: "from time import sleep", so each time you want to use a sleep you just need to write: "sleep()".
Printing and input reading
The purpouse of this part of the code is to let the user know what to do and get user input so we can "translate" it later.
To print something in the serial monitor in Arduino we use "Serial.println("")". To get user's input I used "Seiral.availabe()==0" with this the code won't do anything else until it receives a user input. To save this input, I used "Sentence = Serial.readStrings()" to save in the string "Sentence".
With Python is easer. With "sentence = input("")" you make the three task at the same time, printing, getting user's input and saving it.
As you can see Arduino requires more coding, something that was easil done in Python requires 4-5 more lines of coding in C++.
Loops (For) and Boolean Operators (AND, OR)
Finally, this part of the code will read each character of the sentence and compare it (using if - else if) until it match the letter. The process will be repeated until all the character were "translated".
The loop "for" kinda works the same. The difference is that in Python it is simpler
One thing to notice is that the Boolean Operators (AND, OR) between lenguajes is different. In Arduino we use "&&" as an and, and "||" as or. In Python we use it as it is "and" as and, and "or" as or.
Completed code
What I did was a Morse translator where you insert a sentence and using the monitor and a LED it wil translate it to you in Morse code. I made some differences between codes to know which one is one.
Arduino (C++)
In Arduino, I used the orange LED.
Python
In Python, I used the green LED and added a button to start the translation.
Now that both codes are finished, I would like to give my opinion in both Python and C. I learned C since the first year of university and It has not passed a lot of time since I started learning Python and I can say that I like more than C, the lenguaje is pretty easy to understand, the way you defined thing is easier. The only downside is that a lot slower than C, because Python reads the code your are writting on your computer and C sents the code to the microcontroller, maken it a lot faster than Py.