Electronics design
Design a development board to interact and communicate with an embedded
microcontroller.
Group assignment:
Group assignment
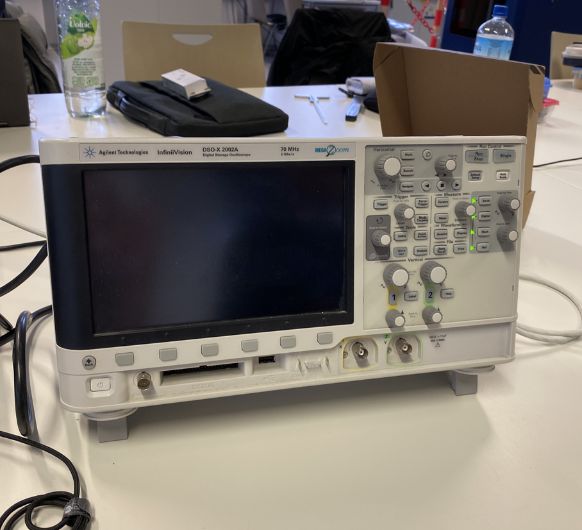
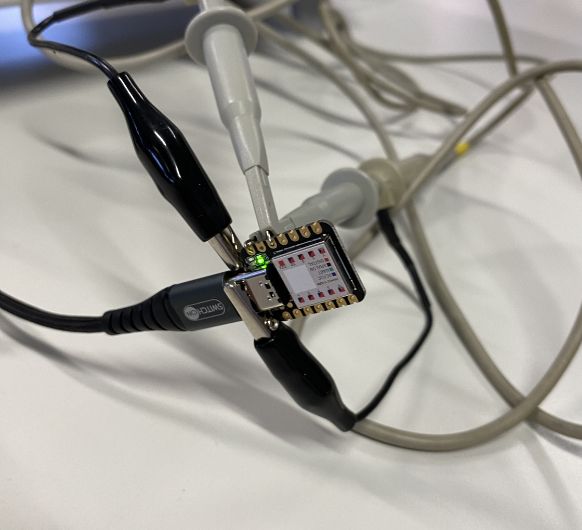
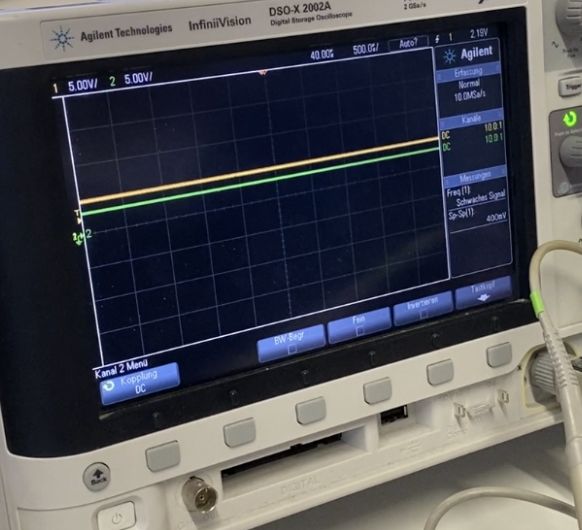
This version of the sketch is truly the simple blink sketch. It uses digitalWrite to write HIGH for 1 second and then LOW for 1 second to a pin:
// the setup function runs once when you press reset or power the board
void setup() {
// initialize digital pin LED_BUILTIN as an output.
pinMode(7, OUTPUT);
}
// the loop function runs over and over again forever
void loop() {
digitalWrite(7, HIGH); // turn the LED on (HIGH is the voltage level)
delay(1000); // wait for a second
digitalWrite(7, LOW); // turn the LED off by making the voltage LOW
delay(1000); // wait for a second
}
This sketch is slightly modified: It uses analogWrite to write an analog signal (number
between 0-255) to a pin:
// the setup function runs once when you press reset or power the board
void setup() {
// initialize digital pin LED_BUILTIN as an output.
pinMode(7, OUTPUT);
}
// the loop function runs over and over again forever
void loop() {
analogWrite(7, 128); // 128 is the pwm signal
}
Here, we're connecting the oscilloscope to pin number 7 (as
described in the sketch above) and to GND. You can see, that the oscilloscope measures
the HIGH and LOW very accurately. Jumping from 0V to 3V3 every second as described in
the code.
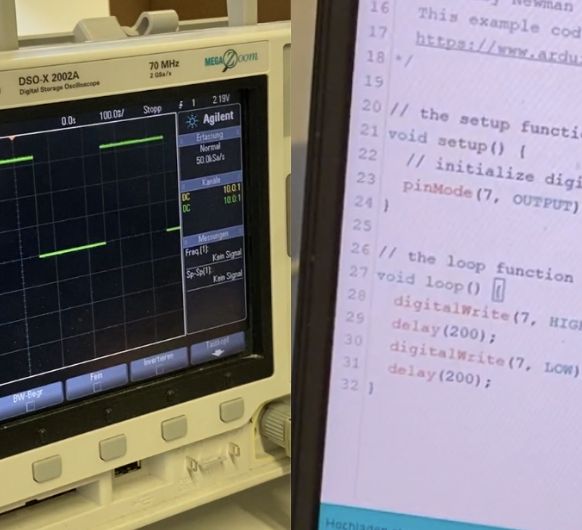
Trying to measure the same thing with the multimeter is very
inaccurate.
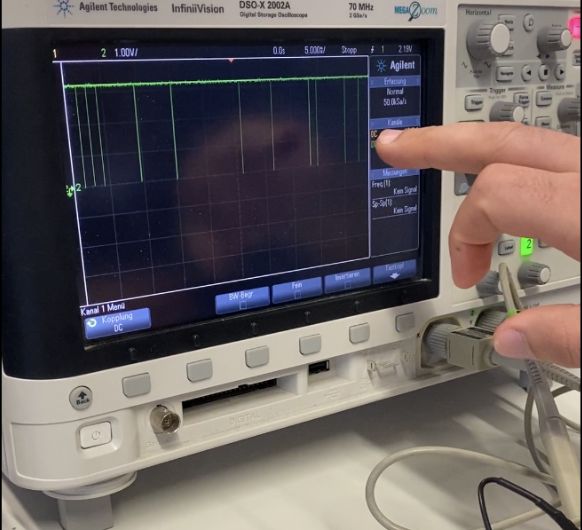
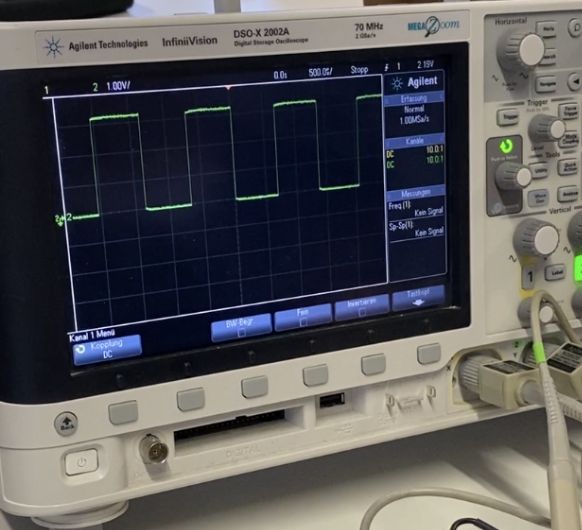
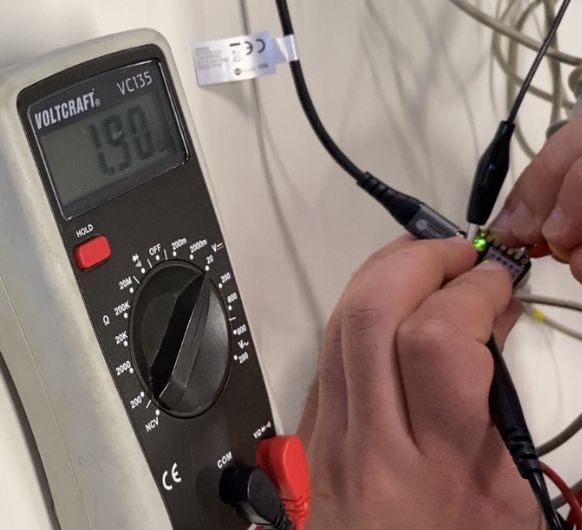
Board Features
I'm designing this PCB to work with my XIAO Seeduino SAMD21. To see its' features, go to my Embedded programming assignment. This SAMD21 can be programmed via USB and doesn't need pins for that. The following I/O-devices will have to connect to the board, since I want to use it for my final project:
- 2x HC-SR04 ultrasonic distance sensor
- 1x peristaltic pump
Also needed:
- 1x MOSFET to drive the pump
- 1x button to test its' functionality
- 1x 5V regulator
- Resistors, Capacitors, LEDs etc
The board also includes a programmable button and an LED that turn on on receiving power (plus the needed capacitors and resistors).
Designing the PCB in KiCad
Library preparation:
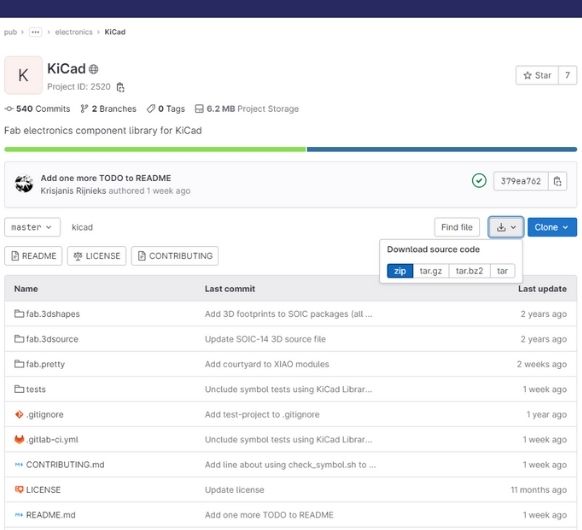
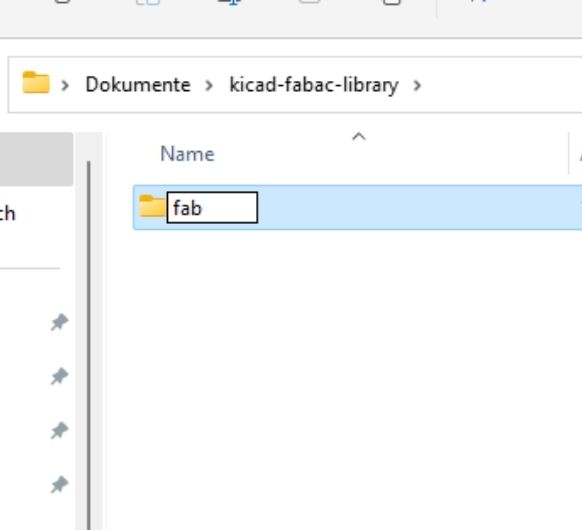
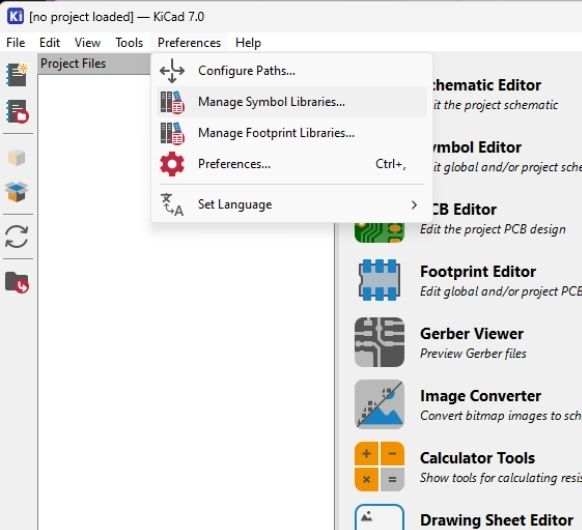
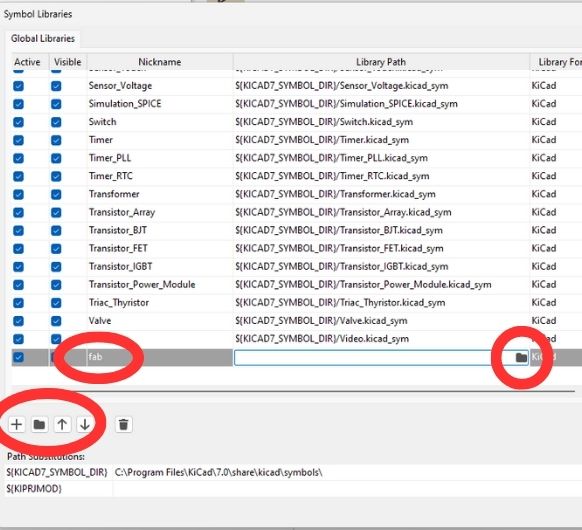
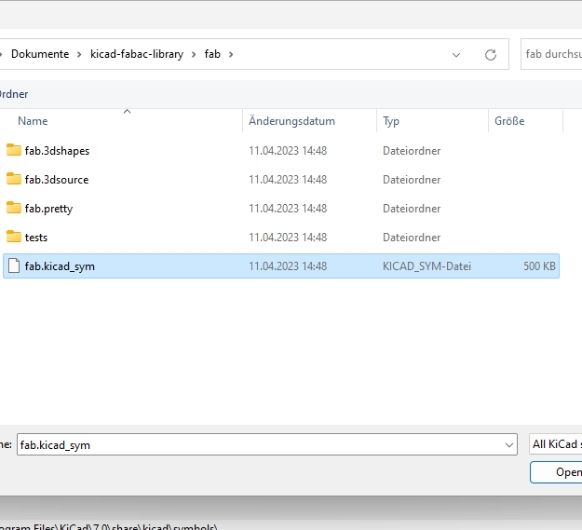
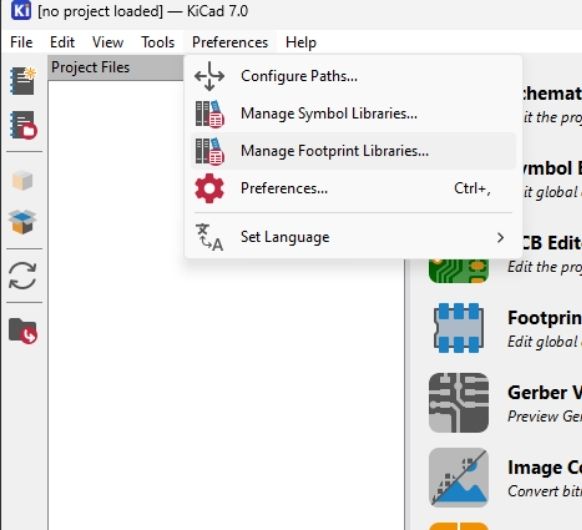
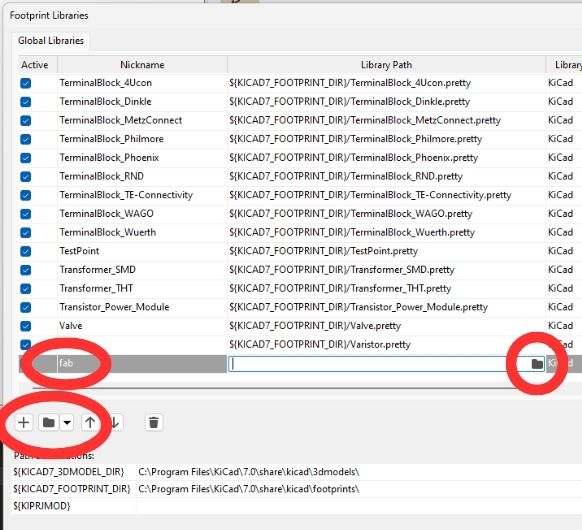
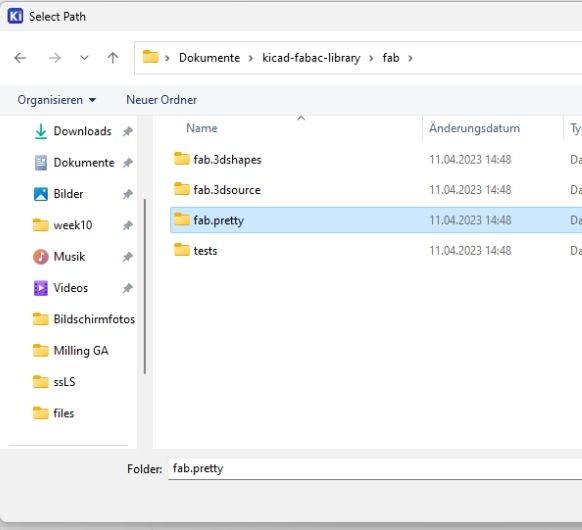
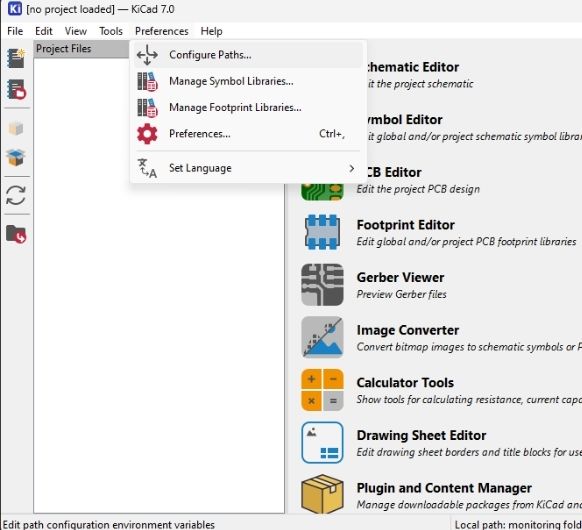
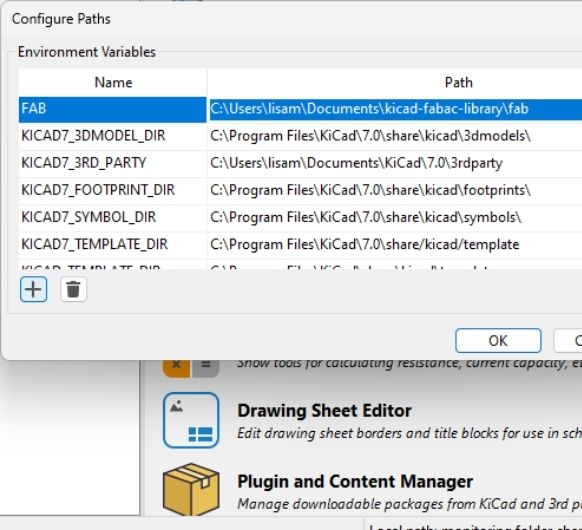
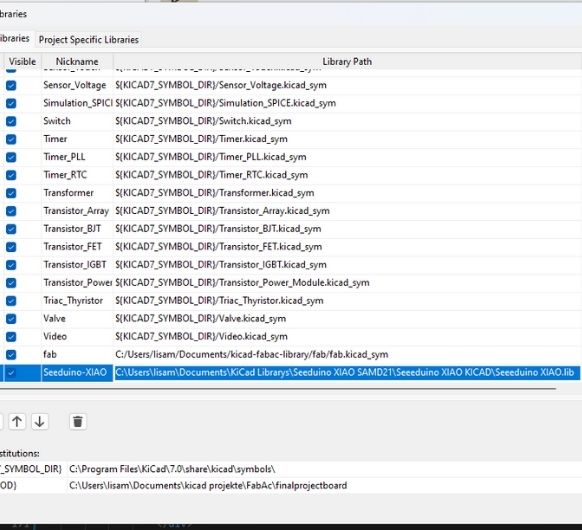
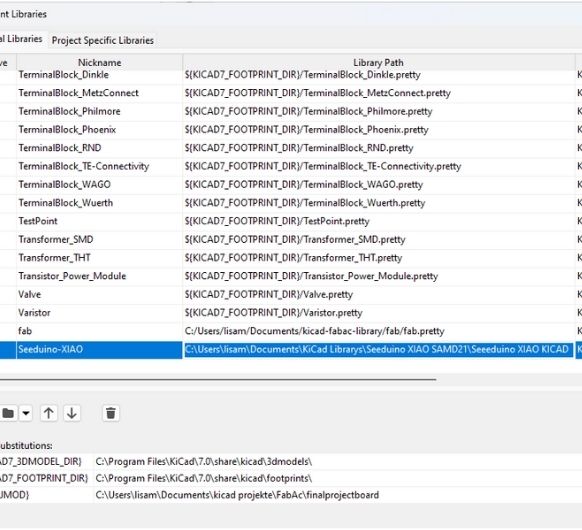
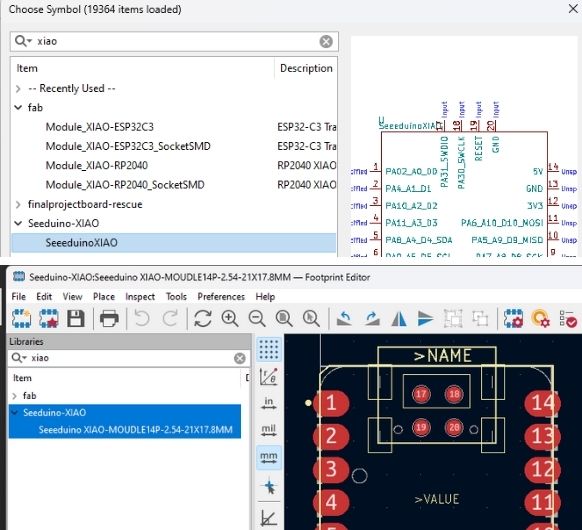
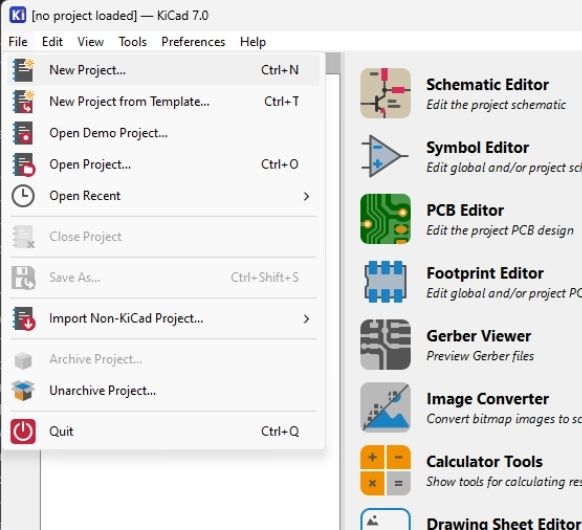
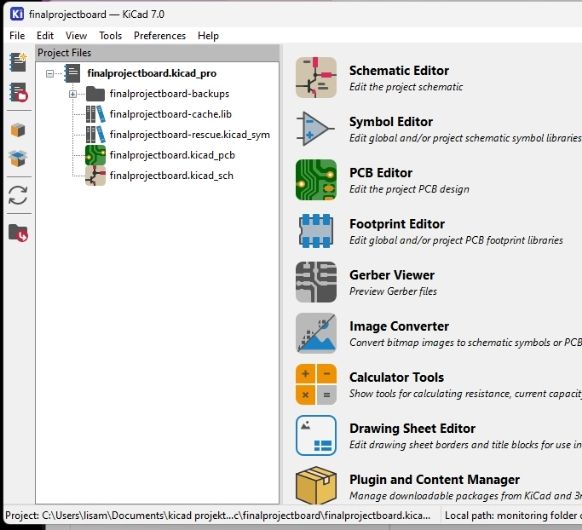
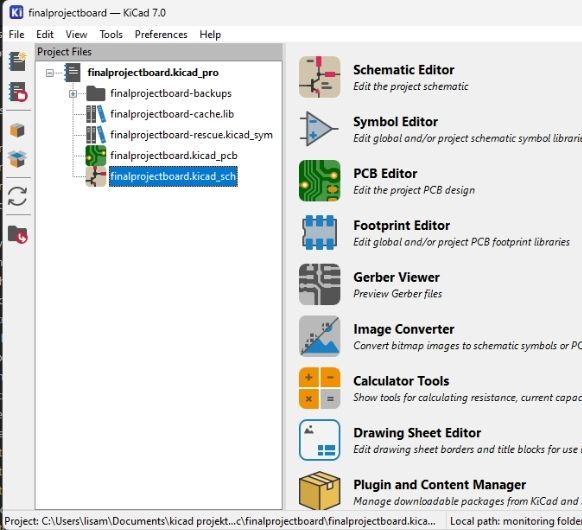
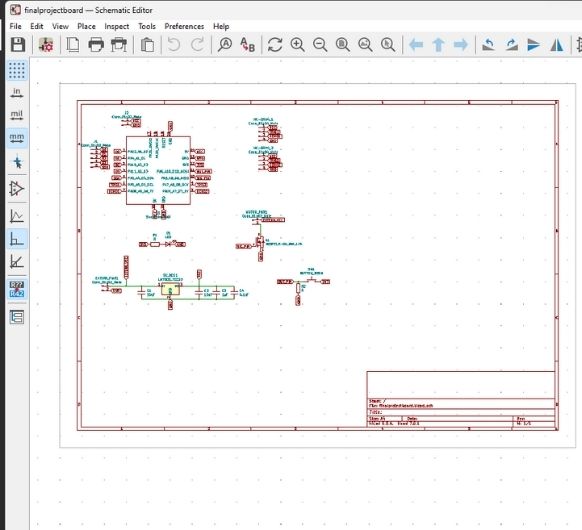
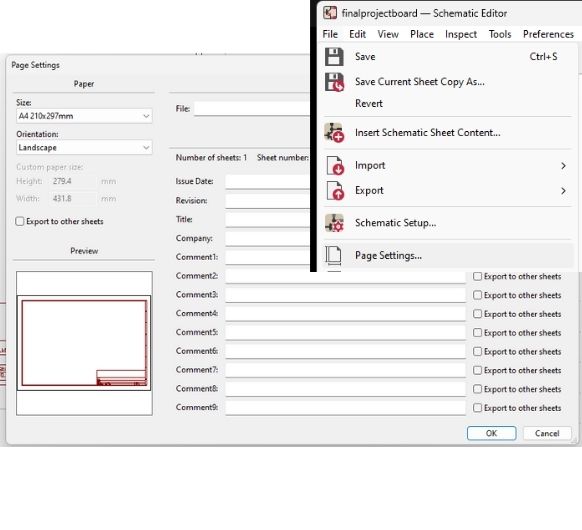
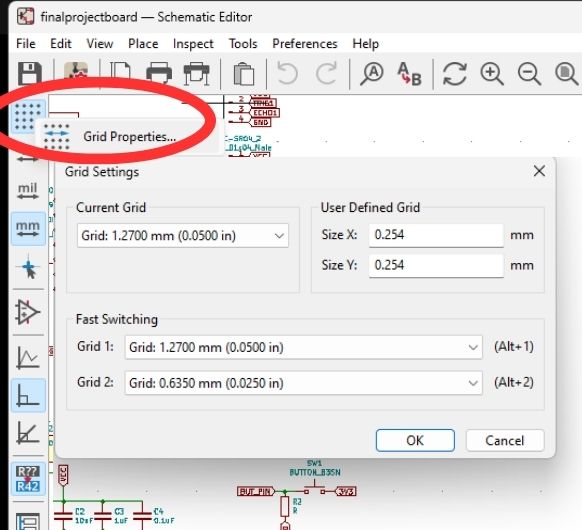
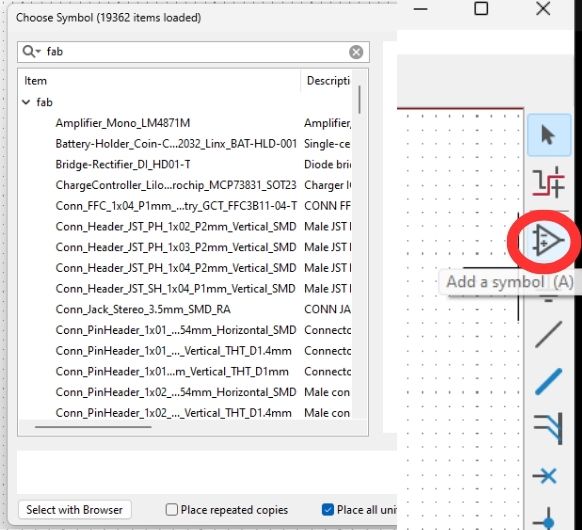
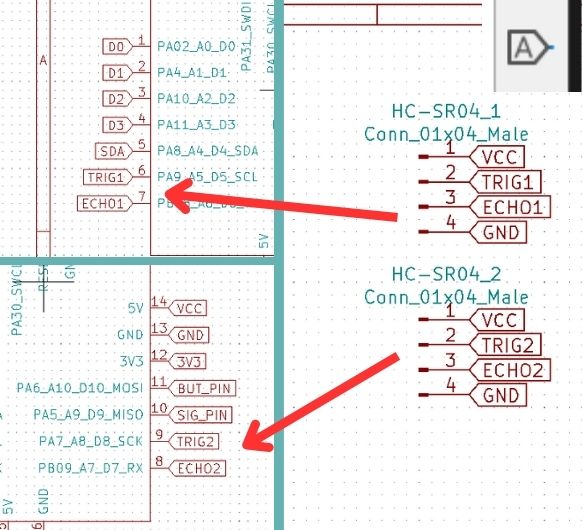
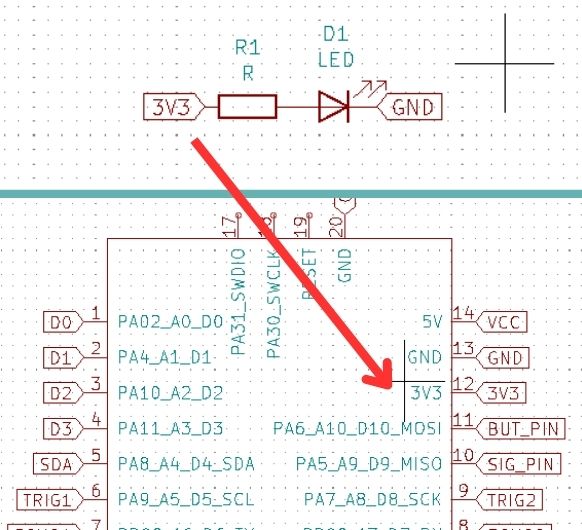
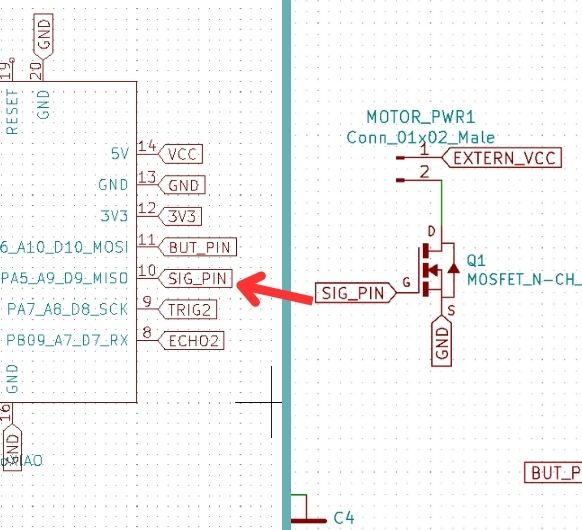
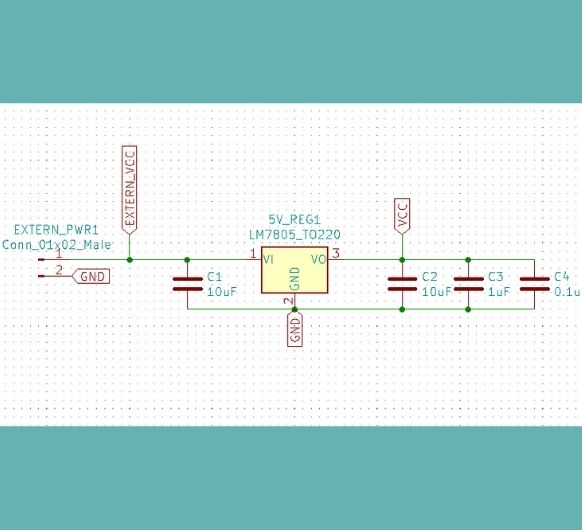
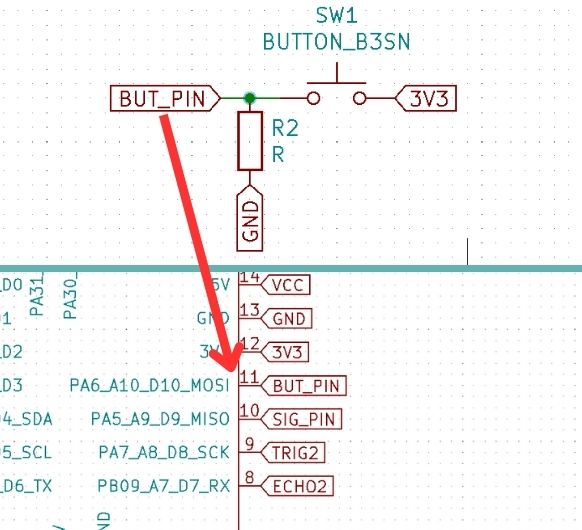
What to do after you added all of your components: From logic to layout
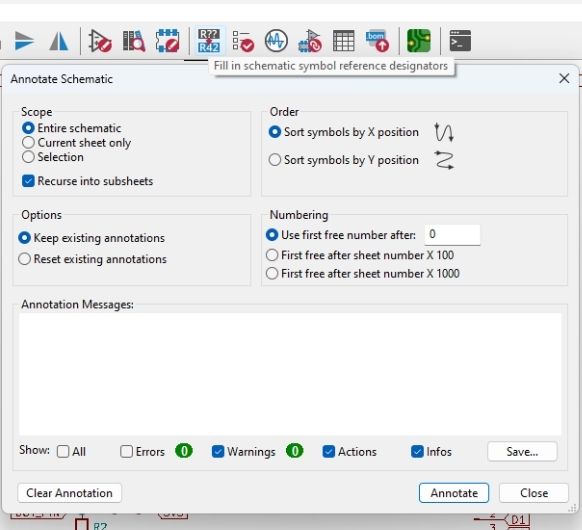
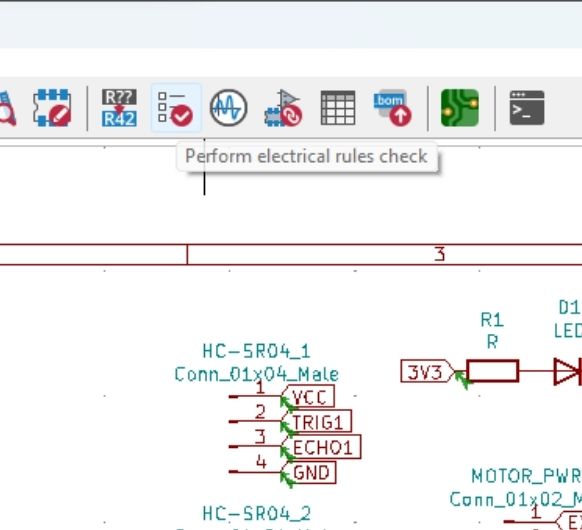
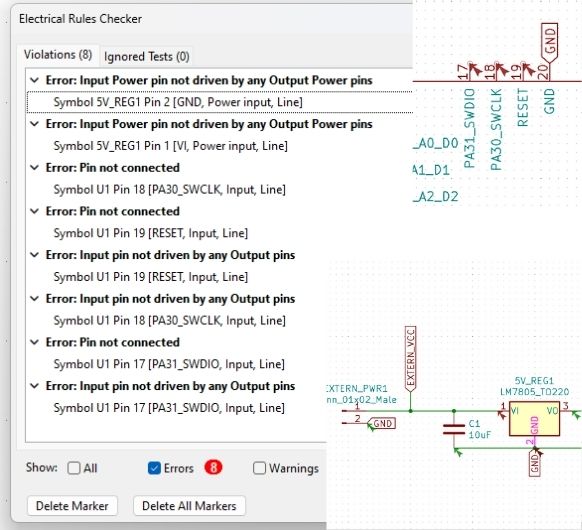
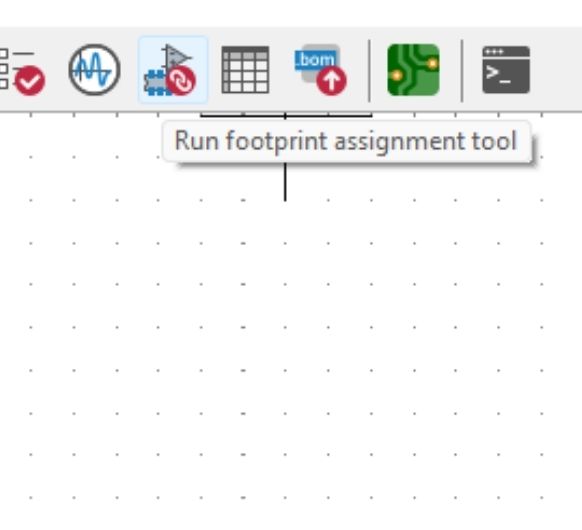
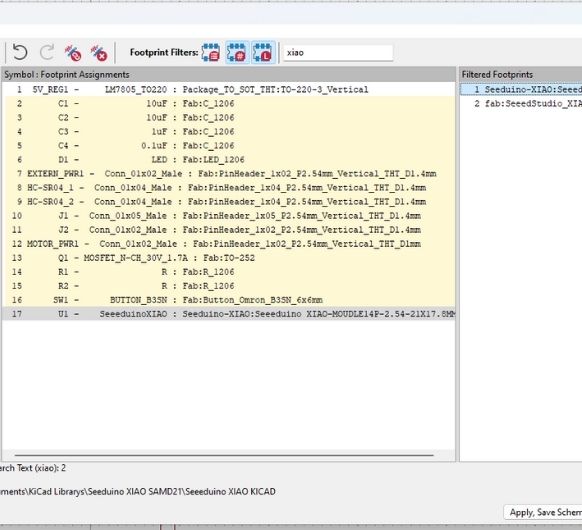
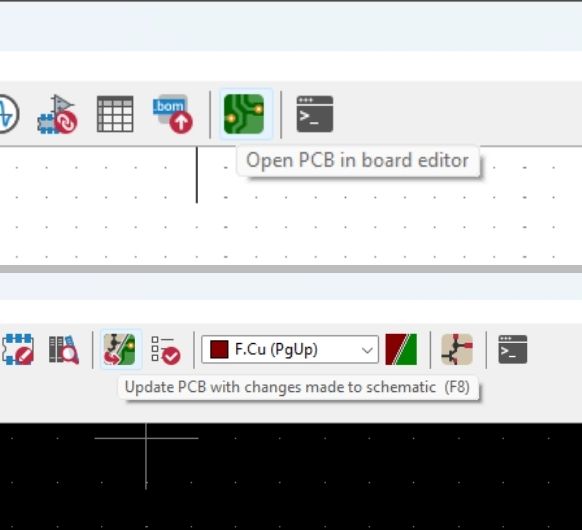
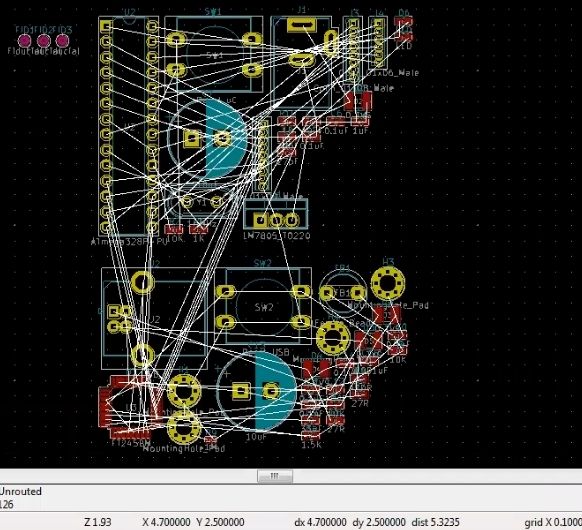
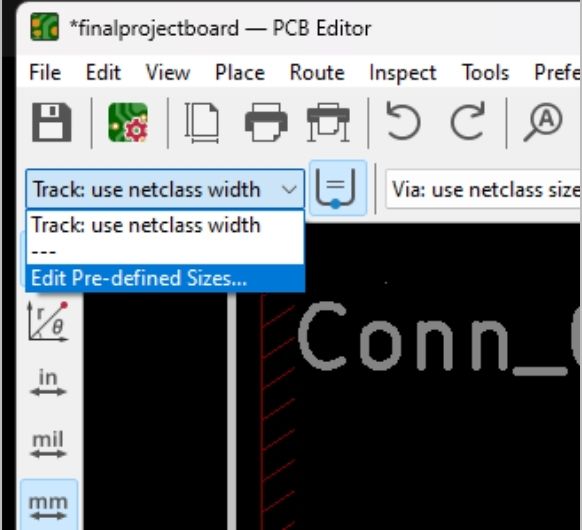
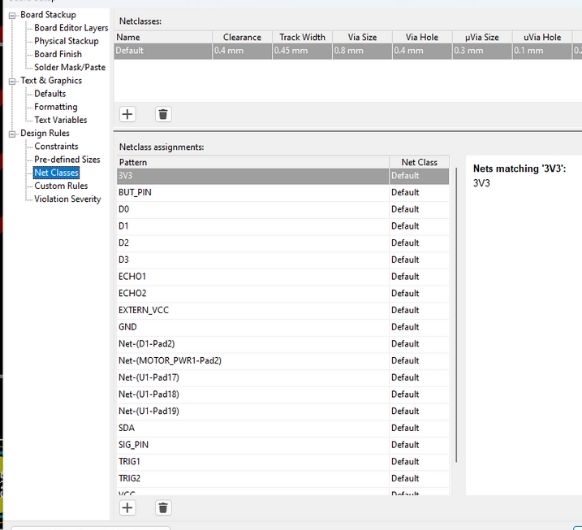
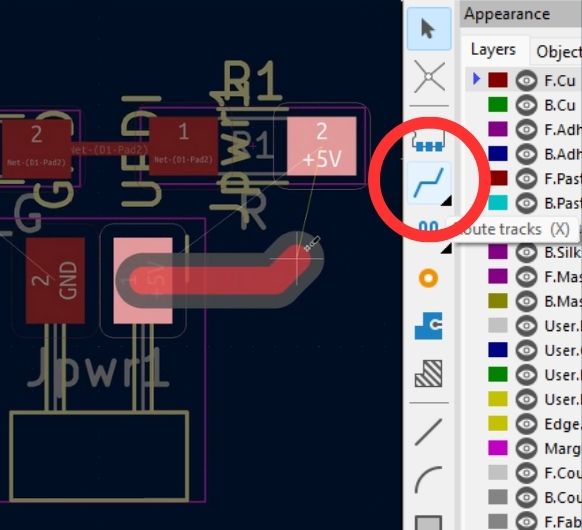
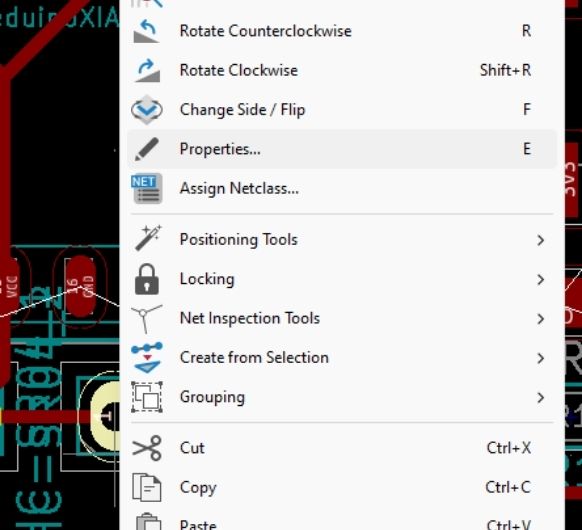
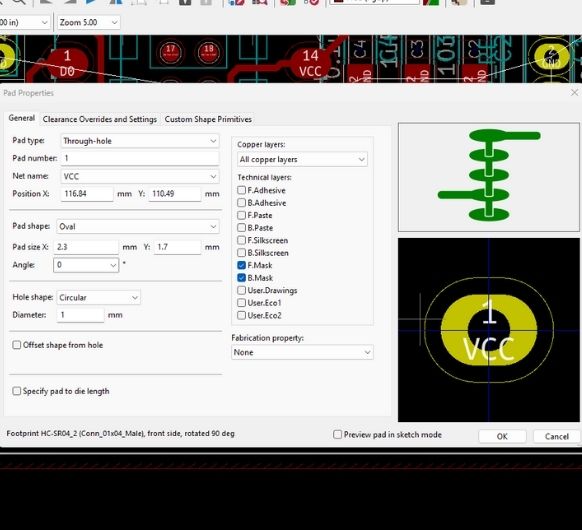
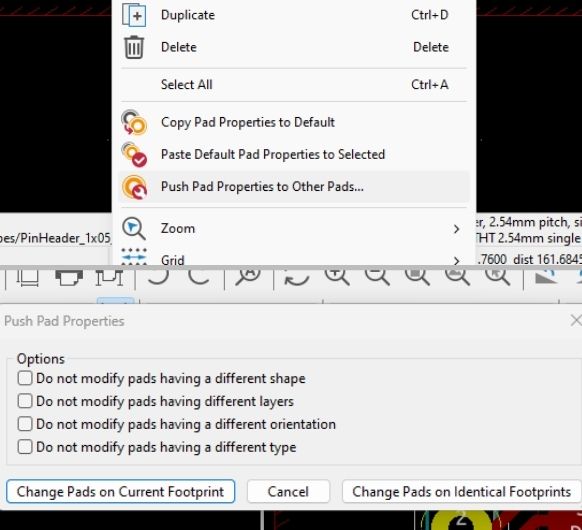
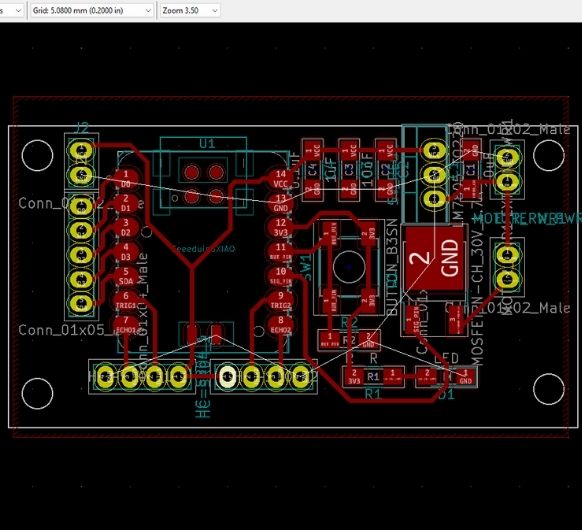
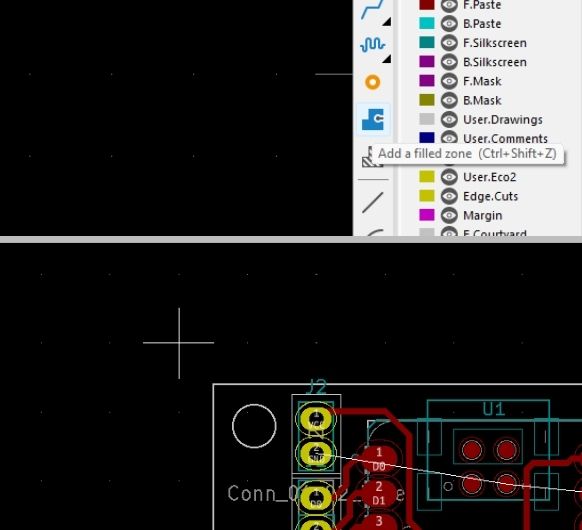
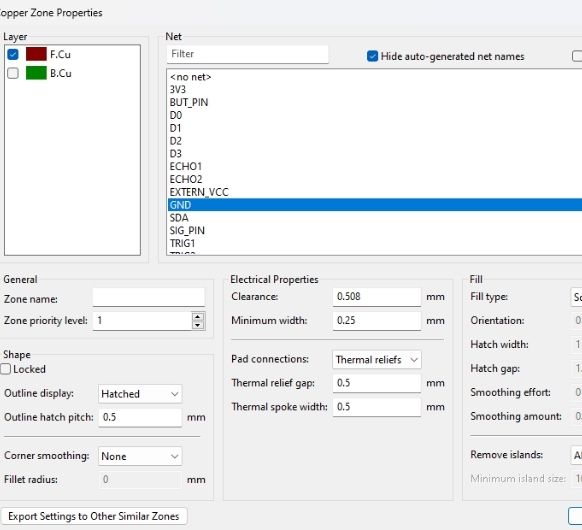
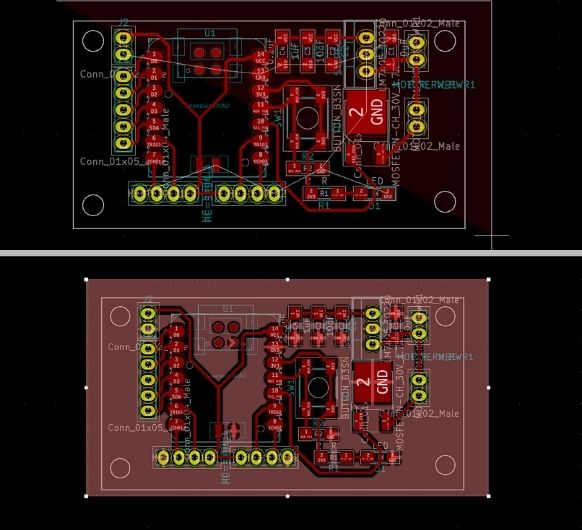
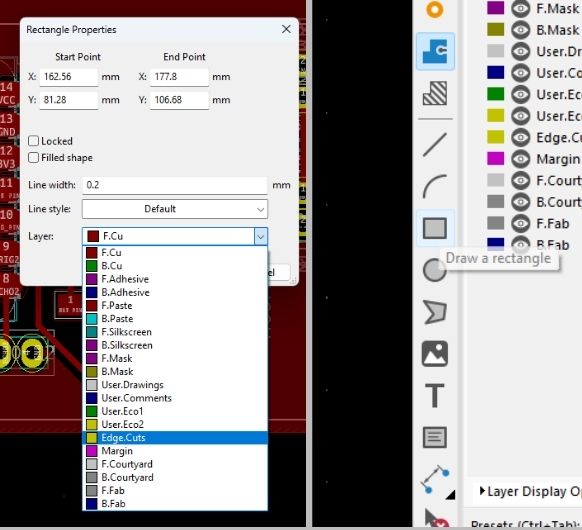
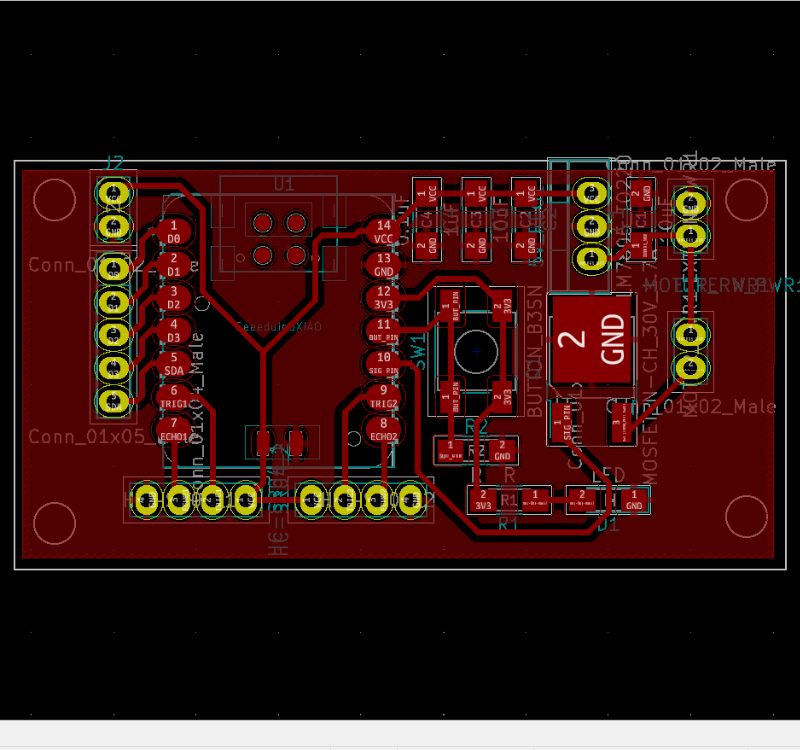
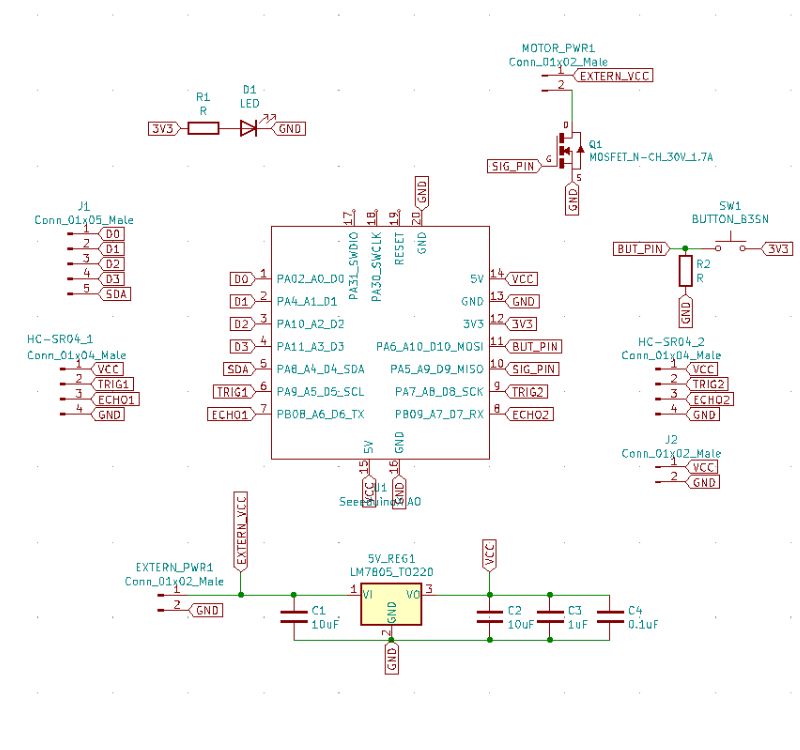