ASSIGNMENT
Write an application that interfaces with an input &/or output device.
WHAT I DID
- I tried to use processing to read the pulses from the inputs device I implemented in my hello world board (class 06).
- My input device is a kind of mechanical switch, it means that it will transmit only two outputs: ON/OFF or 1/0.
So I have decide to investigate a bit deeper the potentiality of processing tried some code that doen't external data, a 'stand-alone' code.
ARDUINO & PROCESSING
I adjust the code for my hello world board so that the serial output is the number of impulses detected by the sensor.
Code:
#include <SoftwareSerial.h>
#define rxPin 0
#define txPin 1
int sw_pin = 3;
int buttonPushCounter = 0; // counter for the number of button presses
int buttonState = 0; // current state of the button
int lastButtonState = 0; // previous state of the button
SoftwareSerial serial(rxPin, txPin);
void setup() {
pinMode(sw_pin, INPUT);
pinMode(rxPin, INPUT);
pinMode(txPin, OUTPUT);
serial.begin(9600);
}
void loop(){
buttonState = analogRead(sw_pin);
if (buttonState == lastButtonState) {
if (buttonState == 1023) {
buttonPushCounter++;
serial.println(buttonPushCounter);
}
else {
}
}
lastButtonState = buttonState;
}
and on the serial monitor I can see the count (yeah, arduino can count):
I then wrote the processing file:
// Graphing sketch
import processing.serial.*;
Serial myPort; // The serial port
int xPos = 1; // horizontal position of the graph
void setup () {
// set the window size:
size(600, 300);
myPort=new Serial(this,"/dev/tty.usbserial-FTFXDZ4A",9600);
myPort.bufferUntil('\n');
background(0);
}
void draw () {
// everything happens in the serialEvent()
}
void serialEvent (Serial myPort) {
// get the ASCII string:
String inString = myPort.readStringUntil('\n');
if (inString != null) {
// trim off any whitespace:
inString = trim(inString);
// convert to an int and map to the screen height:
float inByte = float(inString);
inByte = map(inByte, 0, 1023, 0, height);
// draw the line:
//stroke(127,34,255); //viola
stroke(255);
line(xPos, height, xPos, height - inByte);
// at the edge of the screen, go back to the beginning:
if (xPos >= width) {
xPos = 0;
background(0);
}
else {
// increment the horizontal position:
xPos++;
}
}
}
It reads the data from the serial port, skipping the empty data and it draws a line with lenght proportional to the arduino output.
Code:
// Graphing sketch
import processing.serial.*;
Serial myPort; // The serial port
int xPos = 1; // horizontal position of the graph
void setup () {
// set the window size:
size(600, 300);
myPort=new Serial(this,"/dev/tty.usbserial-FTFXDZ4A",9600);
myPort.bufferUntil('\n');
background(0);
}
void draw () {
// everything happens in the serialEvent()
}
void serialEvent (Serial myPort) {
// get the ASCII string:
String inString = myPort.readStringUntil('\n');
if (inString != null) {
// trim off any whitespace:
inString = trim(inString);
// convert to an int and map to the screen height:
float inByte = float(inString);
inByte = map(inByte, 0, 1023, 0, height);
// draw the line:
//stroke(127,34,255); //viola
stroke(255);
line(xPos, height, xPos, height - inByte);
// at the edge of the screen, go back to the beginning:
if (xPos >= width) {
xPos = 0;
background(0);
}
else {
// increment the horizontal position:
xPos++;
}
}
}
Before to get a staight increase graph at first I got this result:
and the reson was very simple, I still had opened the Arduino serial monitor.So it's important to close the serial monitor to avoid data misunderstanding.
PROCESSING
I wanted to play with analog data, so I adopted a random generation command. With my code you create an array of 100 number between 0 and 99. Then I count how many times a number is catched and I visualize trought color on a bar graph.
Code:
void setup() {
size(700,700);
background(0);
smooth();
// lines number = aux_line-1
int aux_line = 3;
// I create aux_line-1 arrays of random number
for (int i = 1; i < aux_line; i++) {
int[] randoms = new int[500];
for (int j = 0; j < randoms.length; j++) {
int r = int(random(0,99));
randoms[j] = r; //fill the array
}
//calculatr the y position the the two lines
int aux = width/aux_line;
//barGraph function described below
barGraph(randoms, 10 + (i * aux));
};
};
// barGraph FUNCTION-----------------------------
void barGraph(int[] nums, float y) {
//Make a list of number counts
int[] counts = new int[100];
//Fill it with zeros
for (int i = 1; i < 100; i++) {
counts[i] = 0;
};
//Tally the counts
for (int i = 0; i < nums.length; i++) {
counts[nums[i]] ++;
};
//Draw the bar graph
for (int i = 0; i < counts.length; i++) {
colorMode(HSB);
fill(counts[i] * 30, 255, 255);
rect(i * 8, y, 8, -counts[i] * 10);
};
};
void draw() {
//This code happens once every frame.
};
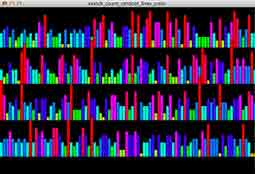
|