Appearance
Output Device
This week, I have designed and manufactured a new PCB board for testing the output device we choose. The output device we selected for testing a step motor. See a hero shot below.
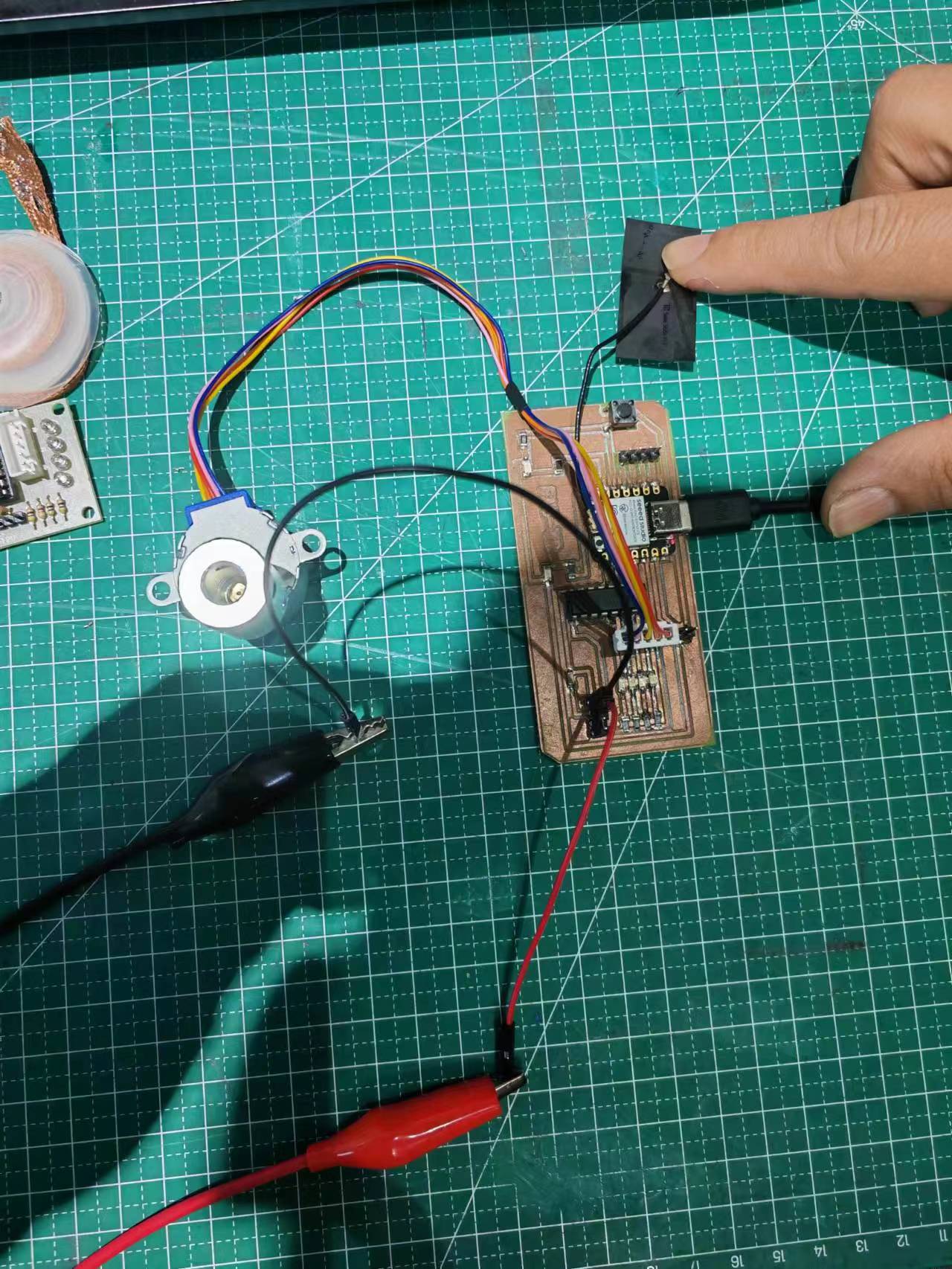
Group Work 2023 & 2025 - Test and measure an output device
Assignment: Measure the power consumption of an output device
Output Devices
Output devices transform digitized signals into a format that can be easily comprehended by the user. They serve as the opposite of input devices by presenting the processed data in a readable format to the user. In the context of a computer or microcontroller, output devices display or convey the information generated by the system. Examples of output devices include monitors, printers, speakers, and LEDs. These devices ensure that the results of data processing are accessible and understandable to users, allowing them to make informed decisions based on the output.
Step motor
The output device we choose to use for this week is a step motor 28BYJ-48. A stepper motor is an electric motor that moves in discrete steps. Each step is a fraction of a full rotation, and the motor's position can be controlled precisely without the need for feedback systems. This makes stepper motors highly useful in applications requiring precise positioning and repeatability such as robotics joints and arms, automotive, CNC Machines, etc.
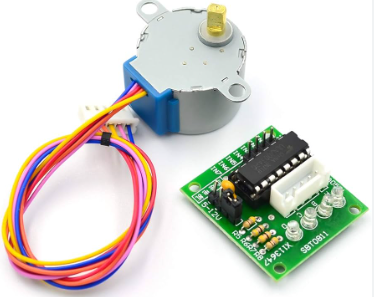
28BYJ-48 Stepper Motor
The 28BYJ-48 stepper motor is a widely used, cost-effective motor that is perfect for small projects and learning purposes. It operates on a 5V power supply and is commonly paired with a ULN2003 driver board for easy control with microcontrollers like Arduino. Here's a brief overview of its key features:
- Voltage: 5V DC
- Torque: 34.3 mN.m at around 15 RPM
- Steps per Revolution: 2048 steps (32 steps per full step, with a 64:1 gear reduction)
- Applications: Ideal for projects requiring precise positioning, such as controlling the vanes of an air conditioner unit or moving small mechanical parts.
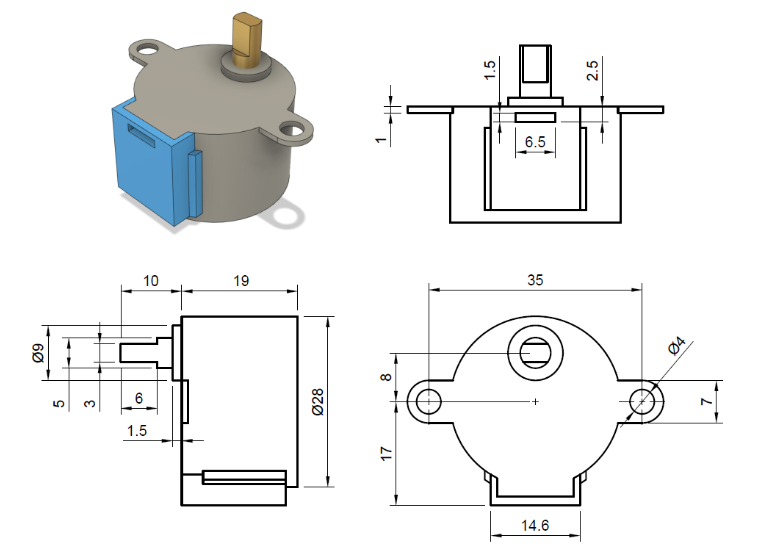
The 28BYJ-48 stepper motor has five wires: four for the coils (orange, pink, yellow, and blue) and one for the 5V power supply (red). By energizing the coils in a specific sequence, the motor rotates in precise steps, making it suitable for various DIY projects.
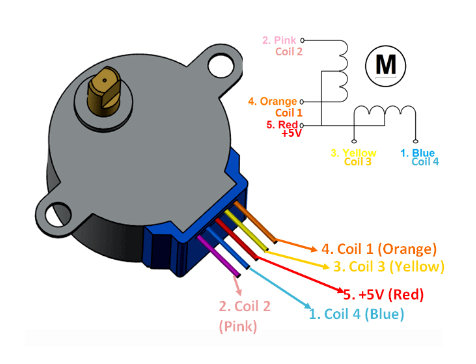
ULN2003 Stepper Motor Driver Board
Microcontrollers like Arduino typically output a maximum current of around 20-40 mA per I/O pin, which is insufficient to drive a stepper motor directly. So we need a motor driver to run the motor. The ULN2003 driver can handle higher currents and higher voltages, making it suitable for driving the coils of the stepper motor. It acts as a buffer between the microcontroller and the stepper motor, allowing the microcontroller to control the motor without being damaged by the higher currents required. Here's a brief overview of its key features:
- Voltage: 5V to 12V DC
- Current: Up to 500 mA per channel
- Control Interface: 4 input pins for stepper motor control
- Output Interface: 4 output pins connected to the stepper motor coils
- Indicators: Four LED indicators showing the activation of each coil
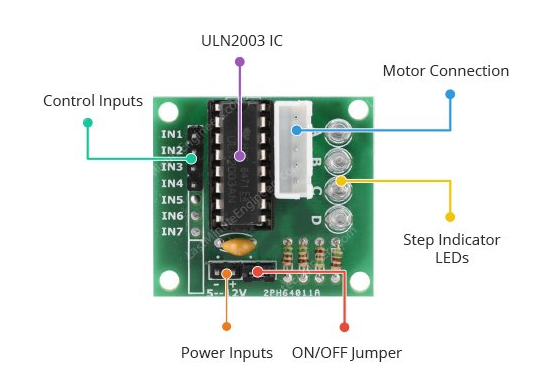
Wiring – Connecting 28BYJ-48 Stepper Motor and ULN2003 Driver Board to Arduino UNO
- The connections are straightforward. Begin by connecting an external 5V power supply to the ULN2003 driver. The stepper motor can be powered directly from the Arduino, but this is not recommended because the motor can generate electrical noise on its power supply lines, which may damage the Arduino.
- Connect the driver board’s IN1, IN2, IN3, and IN4 to Arduino digital pins 8, 9, 10, and 11, respectively. Then connect the stepper motor to the ULN2003 driver.
- Finally, make sure your circuit and Arduino share a common ground. Here is the illustration of circuit diagram.
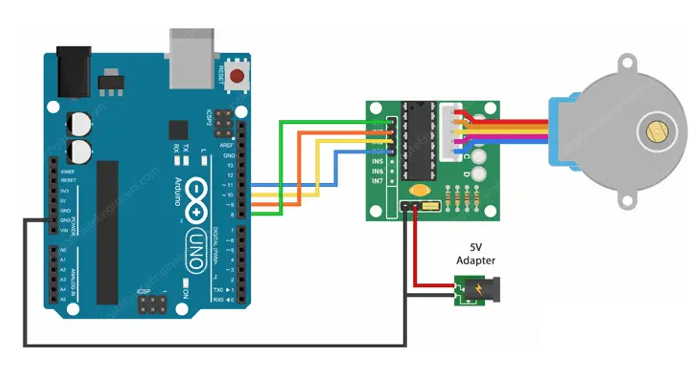
Code for control a 28BYJ-48 Stepper Motor
The code used for running the step motor is shown as below:
#include <Stepper.h>
//define Input pins of the Motor
#define OUTPUT1 7 // Connected to the Blue coloured wire
#define OUTPUT2 6 // Connected to the Pink coloured wire
#define OUTPUT3 5 // Connected to the Yellow coloured wire
#define OUTPUT4 4 // Connected to the Orange coloured wire
// Define the number of steps per rotation
const int stepsPerRotation = 2048; // 28BYJ-48 has 2048 steps per rotation in full step mode as given in data sheet
// Initialize the stepper motor with the sequence of control pins OUTPUT1, OUTPUT3, OUTPUT2, IN4
// OUTPUT1 and OUTPUT3 are connected to one coil and OUTPUT2 and OUTPUT4 are connected to one Coil
Stepper myStepper(stepsPerRotation, OUTPUT1, OUTPUT3, OUTPUT2, OUTPUT4);
void setup() {
// Set the speed of the motor in RPM (adjust as needed)
myStepper.setSpeed(15); // 15 RPM
}
void loop() {
// Rotate in One Direction and complete one complete rotation i.e 2048 steps
myStepper.step(stepsPerRotation);
delay(1000); // Delay between rotations
// For Rotation in opposite direction provide the variable to the parameter with negative Sign
myStepper.step(-stepsPerRotation);
delay(1000); // Delay between rotations
}
Overall, the code alternates the stepper motor's rotation direction, completing one full rotation in each direction with a 1-second delay between each rotation. This creates a back-and-forth rotational motion for the motor.
Measure the power output
We have used an UNI-T UTP1306S power supply to directly measure the power output.
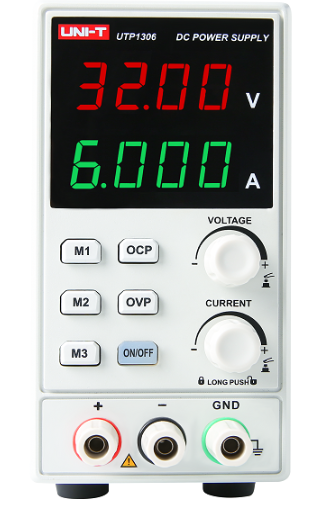
The UTP1306S power supply is an economical switching DC power supply with a single output.
- Rated output voltage 0~32V
- Rated output current 0~6A
- Max Output Power 192W
- Voltage/current display resolution: 10mV/1mA
- Four-digit voltage and current display
- Overvoltage/overcurrent/overtemperature protection
- Three groups of one-button call memory values
- Can be set Flow/overvoltage protection value
- Independent output switch
- Operation button can be locked
The power supply result have shown as below while the motor is running stable, with
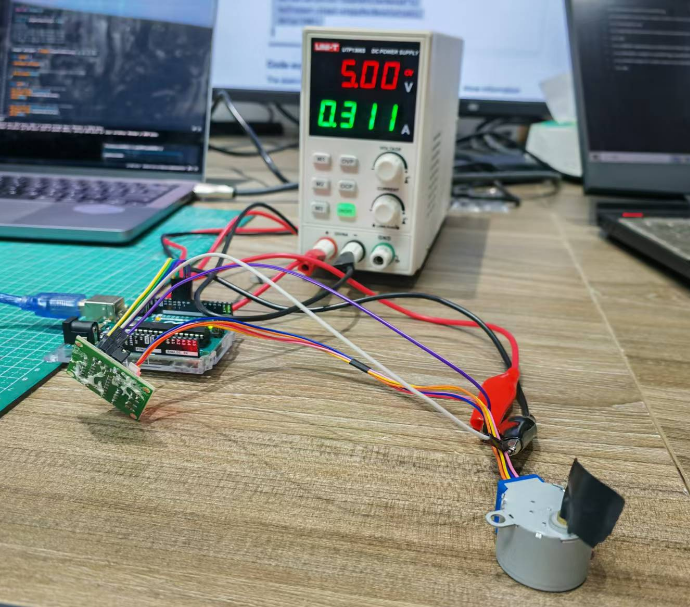
For the average 0.311 amperes with a constant voltage of 5, then we have the overall power output calculated as: P =I * V =0.311 X 5 =1.555 watt The resistance can be calculated as: R =V / I= 16.07 ohm
Seeed Xiao ESP32 board design for using the step motor
Based on the test presented, I have further designed a PCB using Seeed XIAO ESP32 to run the step motor. The detail schematic design is shown as pictures below.
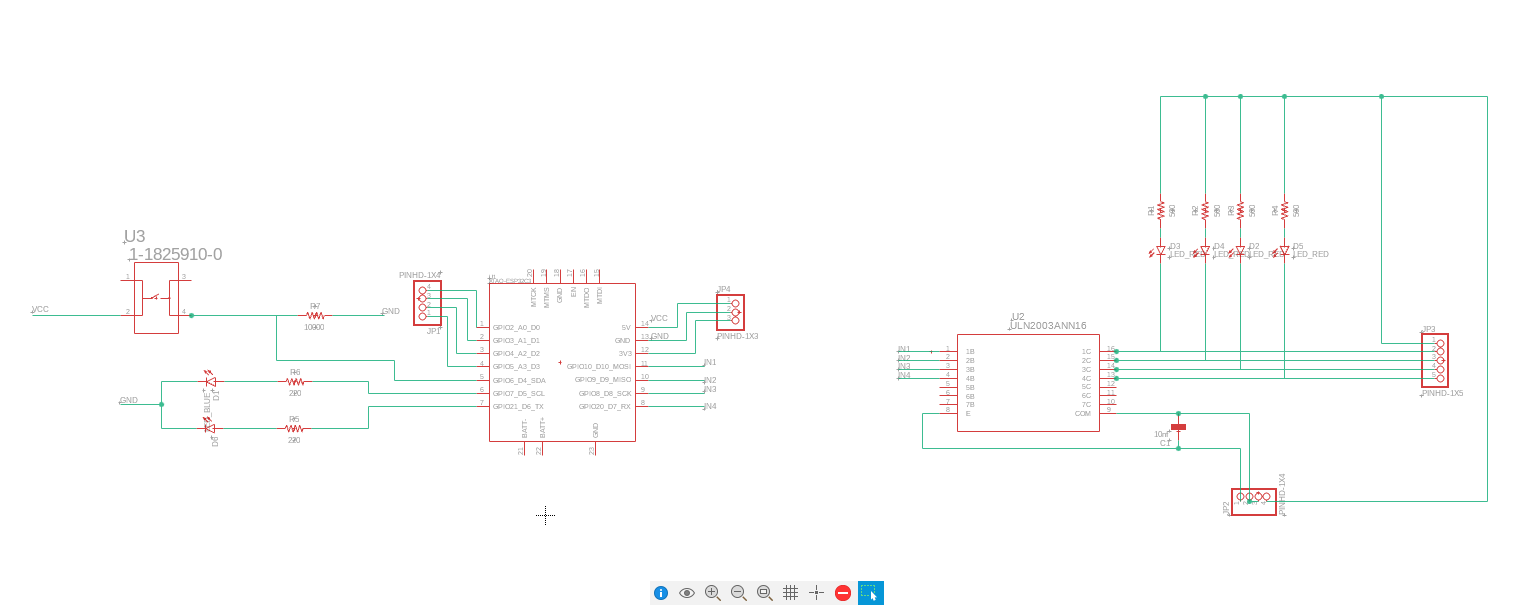
The board routing is provided.
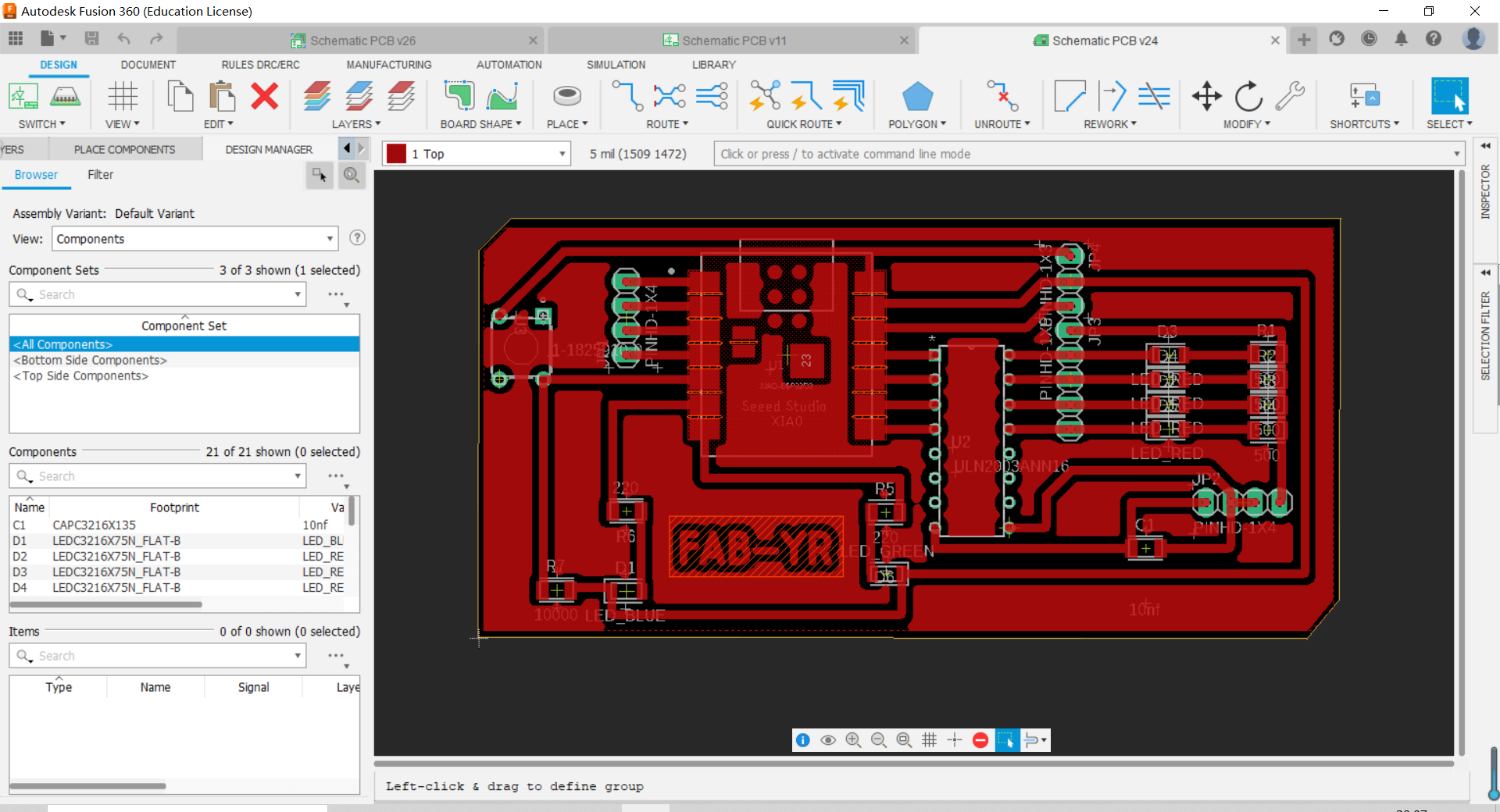
Push to get 3D version of the board.
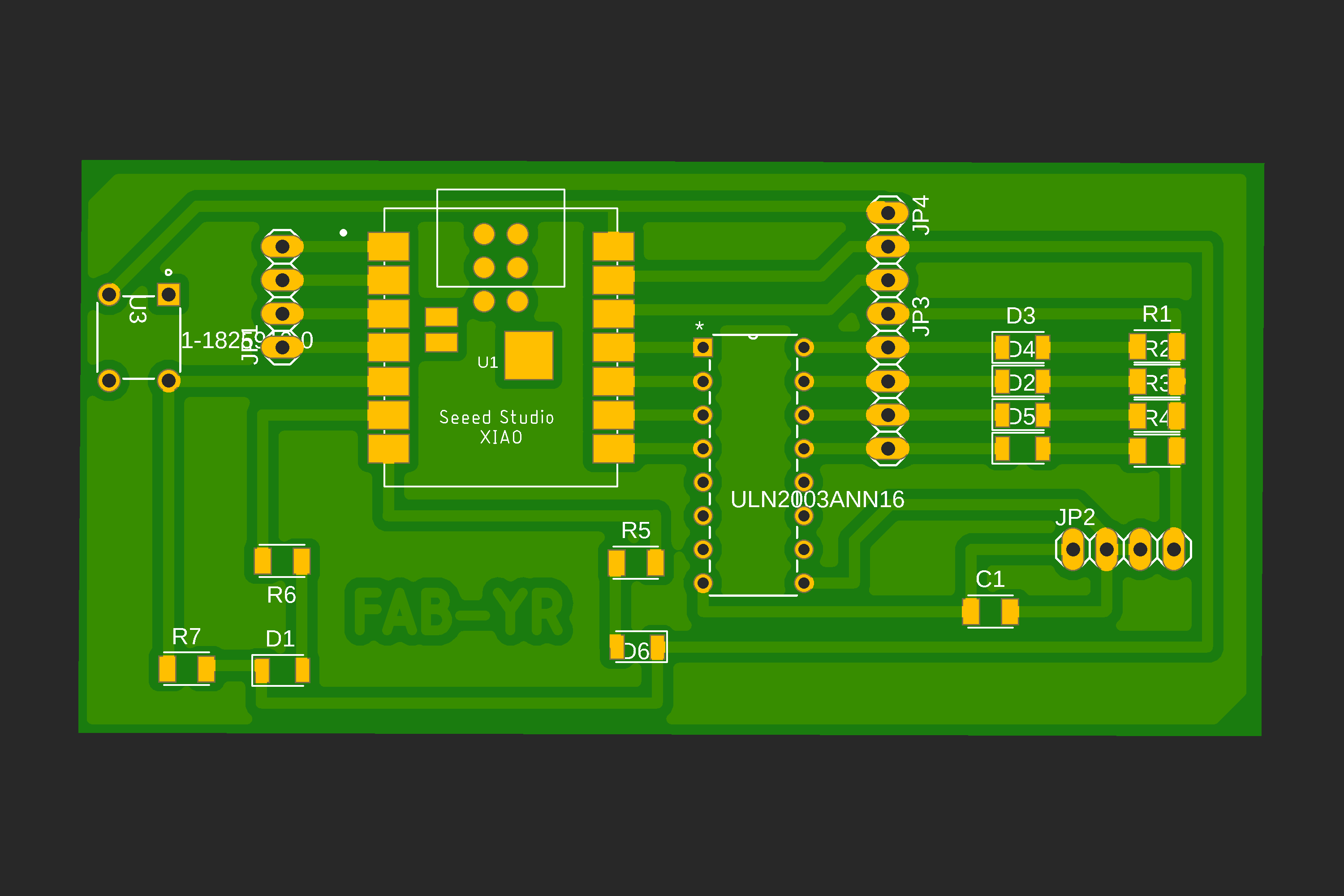
Then BOM list for manufacturing can be obtained.
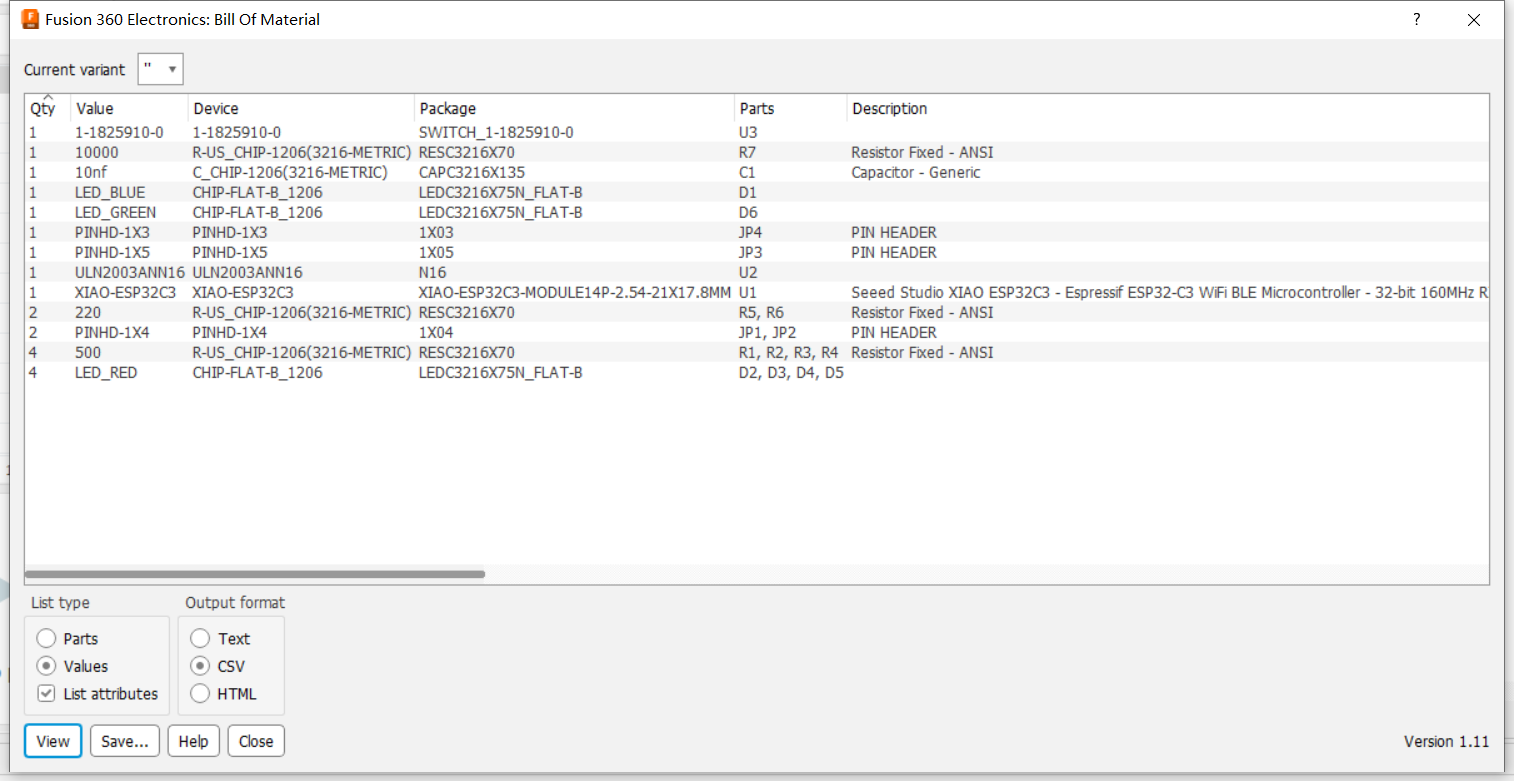
Manufacturing of the board
Using our ROLAND to machine the board.
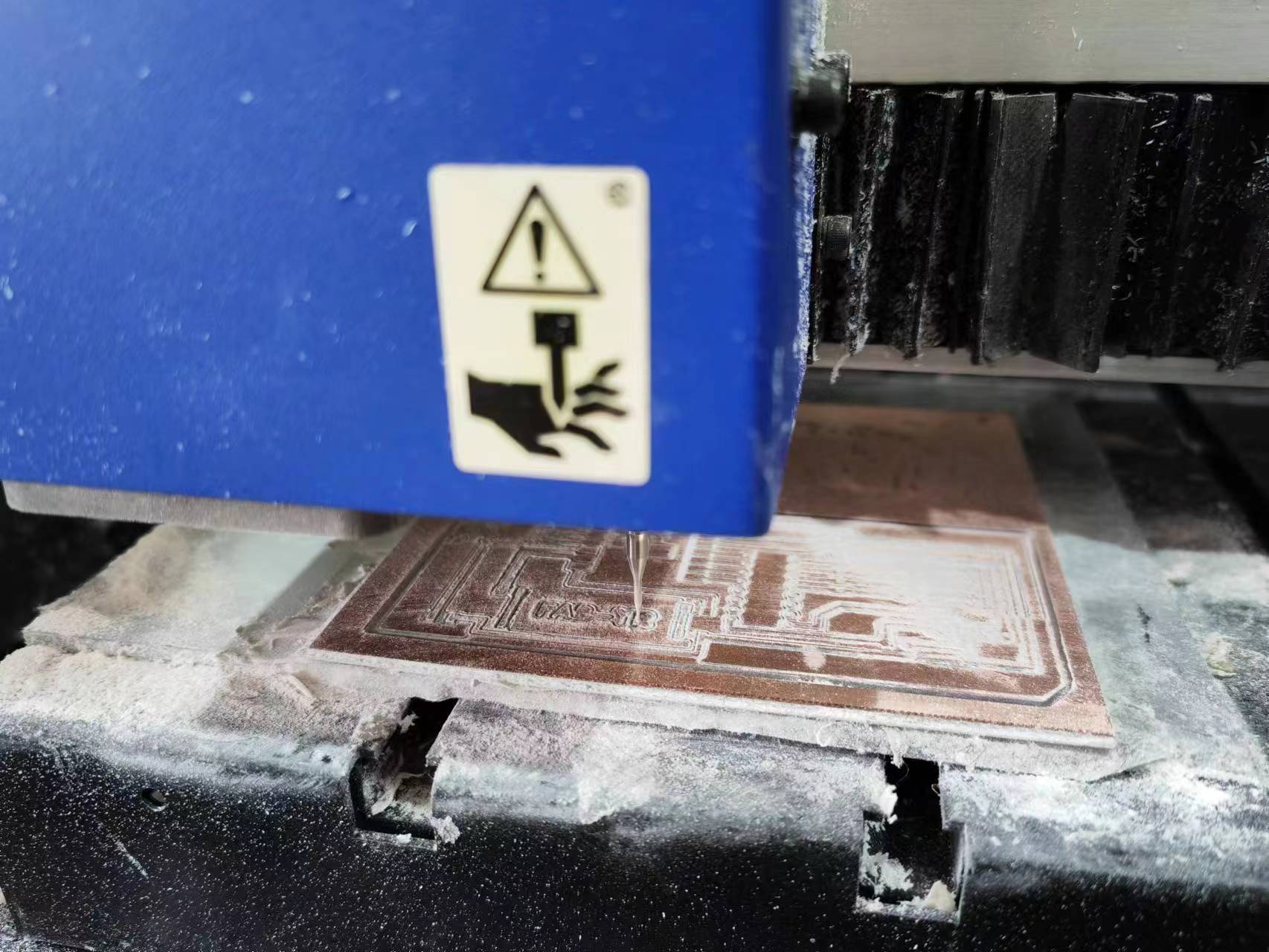
After machining.
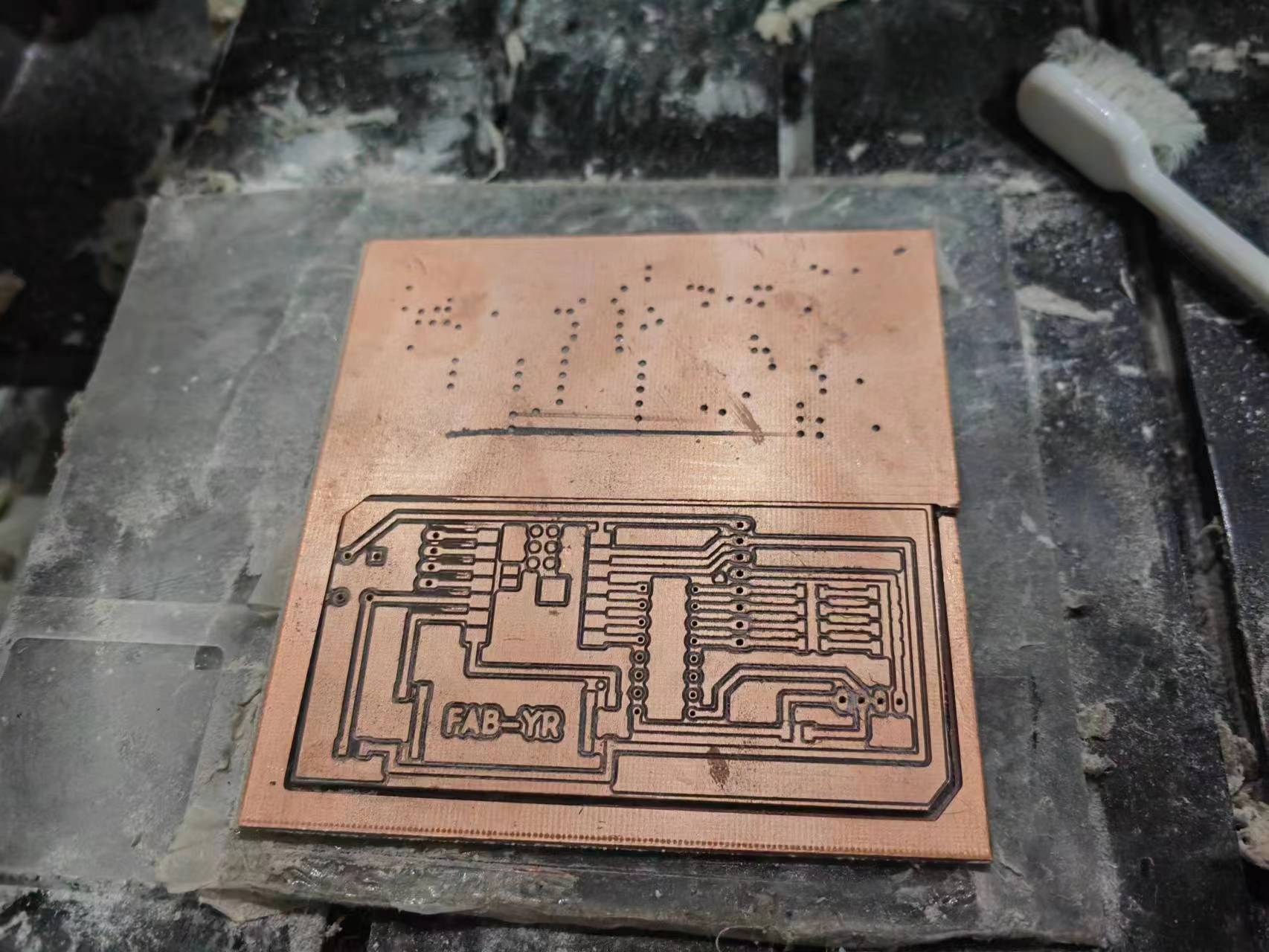
The electronic components used in soldering process is documented.
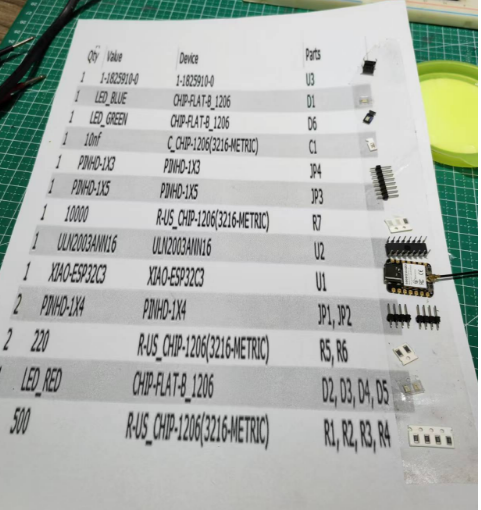
The board is perfectly produced.
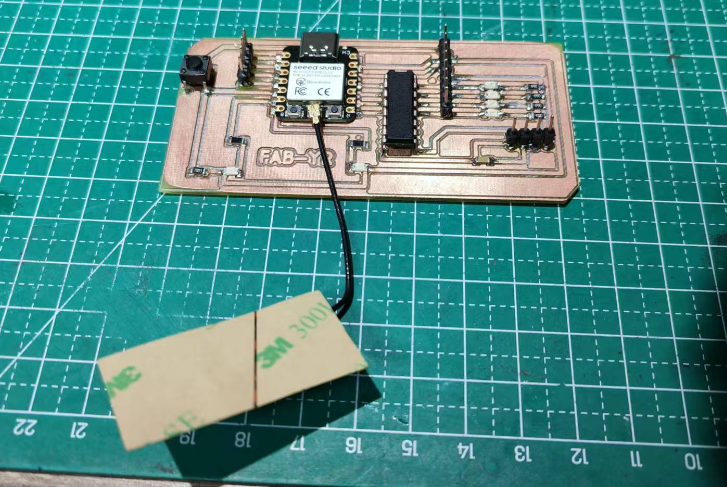
Testing in progress
The code used for testing the motor is generally the same, only added two LED light to indicate the turning direction of the motor.
#include <Stepper.h>
// The number of steps per full rotation of the output shaft.
// 32 motor steps * 64:1 gear ratio = 2048 steps per rotation.
#define STEPS 2048
// Create an instance of the stepper class, specifying the
// number of steps per rotation and the pins it's attached to.
// NOTE D8 and D9 are swapped!
Stepper stepper(STEPS, D10, D8, D9, D7);
// Define LED pins
const int ledCW = D6;
const int ledCCW = D5;
void setup() {
// Max speed without skipping seems to be about 19rpm
// under no load; using 15 to be safe.
stepper.setSpeed(15);
// Initialize LED pins as outputs
pinMode(ledCW, OUTPUT);
pinMode(ledCCW, OUTPUT);
}
void loop() {
// One full CW rotation (5 seconds)
digitalWrite(ledCW, HIGH); // Turn on CW LED
stepper.step(STEPS);
digitalWrite(ledCW, LOW); // Turn off CW LED
delay(5000); // Wait 5 seconds
// One full CCW rotation (5 seconds)
digitalWrite(ledCCW, HIGH); // Turn on CCW LED
stepper.step(-STEPS);
digitalWrite(ledCCW, LOW); // Turn off CCW LED
delay(5000); // Wait 5 seconds
}
The wire connection is shown in the figure below.
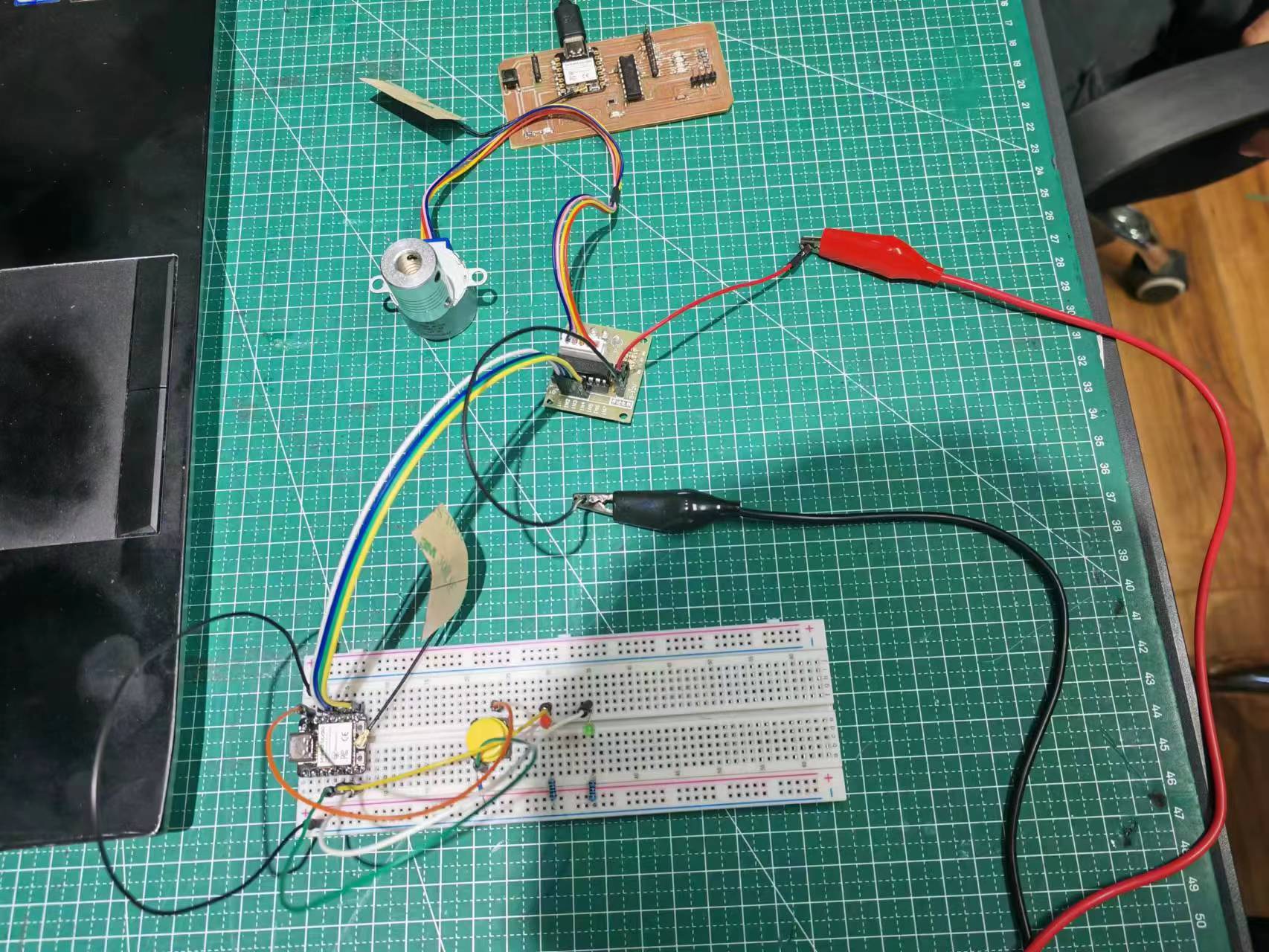
The blue led is lighted when step motor run clockwise.
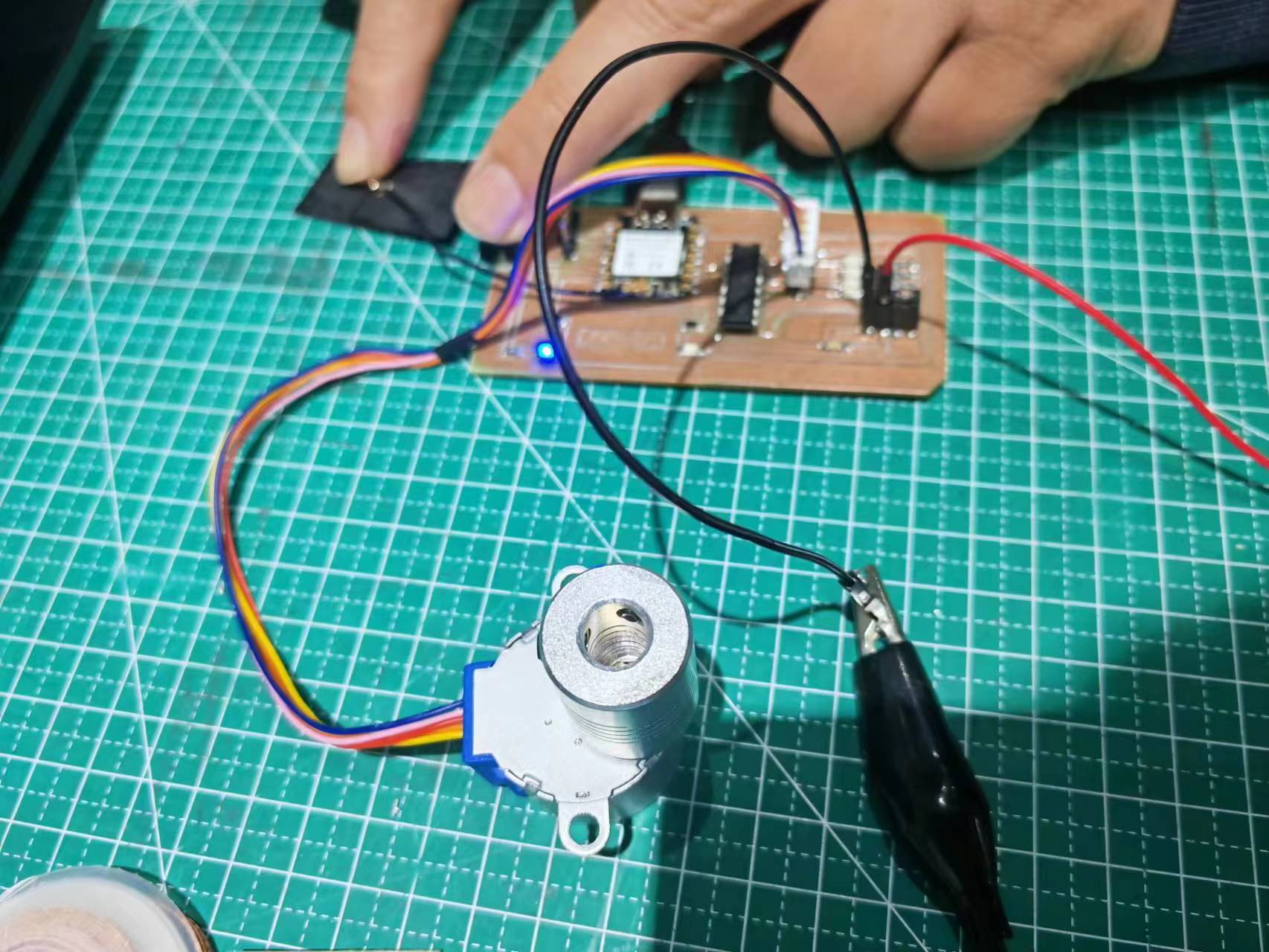
The green led is lighted when step motor run counter-clockwise.
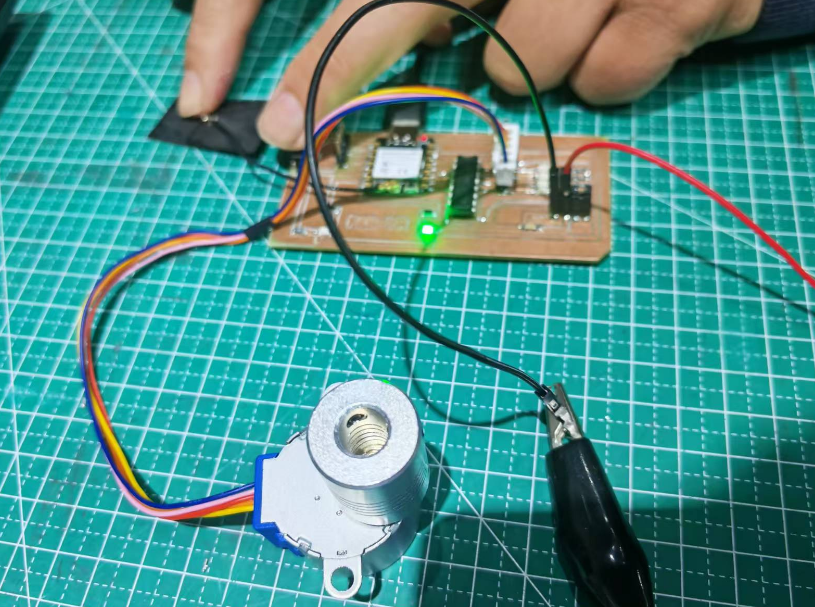
Reference
Download my board design here: https://a360.co/3CzHrP8. The design file can also be downloaded from my repo: https://gitlab.fabcloud.org/academany/fabacademy/2023/labs/ningbo/students/yaorun-zhang/-/tree/main/docs/assignments/week10?ref_type=heads.