Make a Personal Site
Part of Week 1's Assignment
Environment, Constrains, Notes
The site is hosted at the fabcloud.io, which runs an instance of Gitlab, CI/CD possible, Static Site Generators like MkDocs, Hugo, Jekyll, etc are offered by default. Of course pure HTML is also an option. (See Neil's sites.)
While creating a site with Site Generators and Prefab Themes is of course perfectly valid, I would like to have full knowledge and understanding of the code that is used to generate the site.
Step 1: Make a (brutalist?) HTML page.
No HTML template, no CSS framework. Build from the ground up. I will allow myself modern CSS and also a modern font. The HTML will be mostly utilitarian, it might come across a bit brutal.
page.html
<!doctype html>
<html>
<head>
<title>FabAcademy 2021 - Georg Tremmel - FabLab Kamakura</title>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1" />
<link href="trembl.css" rel="stylesheet">
<link rel="stylesheet" href="https://use.typekit.net/mhw5cxw.css">
</head>
<body>
<header>
Navigation
</header>
Content
<footer>
Footer Navigation
</footer >
</body>
<html>
Option 1:
Now we have 2 options. Use Hugo, MkDocs, VuePress or a similar environment and create a minimal theme based on my HTML.
Option 2:
Don't use a site generator, use simple PHP to streamline site maintenance, and use wget
to generate static sites from PHP.
While I'd be happy to dive into site generators and make a custom theme, I'd like to stay minimal and not overly rely on frameworks to generate the site. Having said this, when using PHP, a local server is needed
Spiral Development: Sharing Headers & Footers
Wouldn't it be great to not always have to copy/paste the headers and footer? Let's make separate files for shared headers and footers. Move the header and footer section into their own PHP field, the page.php now looks like this:
<?php include('header.php'); ?>
Content
<?php include('footer.php'); ?>
We also need to crawl the site with wget
to save the pages and images - and to convert the absolute into relative links:
wget \
--recursive \
--page-requisites \
--no-parent \
--directory-prefix download \
--no-host-directories \
--adjust-extension \
--convert-links \
"http://127.0.0.1/fab/"
Condensed version:
wget -rpnp -nH -E -k "http://127.0.0.1/fab/"
Aside: Clean, nice Links in wget
wget
converts links like about
into about.html
. Which is ok-ish, but not really what we want. wget
has no option (if you know one, please tell me!)
<a href="about">About</a>
File: about.html NG
<a href="about/">About</a>
Folder: about, File: OK
Another Aside: wget
needs Content-Type Header
wget
needs this header
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
Otherwise the text looks like net.art, ca 1996
Now we can generate a static website - with nice, clean links - from the PHP files.
Spiral Development: Adding an optional Markdown Parser
Markdown simplifies writing documentations, I would like to have it as an option. There are a number of Client- and Server-side Markdown Parser out there. I would like to generate it on the server, and as we are in PHP, I will use Parsedown, a _simple, fast GitHub-flavoured Markdown Parser without dependencies. Which means we can just drop it in, feed it the text - and out comes Markdown.
include('includes/Parsedown.php');
$Parsedown = new Parsedown();
$text = "my markdown text";
echo $Parsedown->text($text);
Spiraling out of Control: Automatic Image Resizing and Management, Tags, Categories?
Now it's get a little be fuzzy. I could spend more and more time and add more and more features, but this is FabAcademy so I try to stand on the shoulders of giants - and not on their toes (©Neil Gershenfeld).
I integrated my custom code into one of the giants in the field of CMS (still not using any plugins or HTML templates/frameworks), wrote a shell script to generate the site with wget
, clean the link paths, and copy it to my local git lab directory.
TL;DR
The Shell Script, with comments:
#!/bin/zsh
# call script, when content changes
# define target and source directories, don't forget final '/'
TARGET="/Applications/XAMPP/htdocs/georg-tremmel/"
STATIC="/Applications/XAMPP/htdocs/fab/wp-content/static/fab/"
# clear STATIC
# .zsh, set 'setopt rmstarsilent' to allow silent rm *
rm -r ${STATIC}*
# generate static site with wget
#wget \
# --recursive \
# --page-requisites \
# --no-parent \
# --directory-prefix download \
# --no-host-directories \
# --adjust-extension \
# --convert-links \
# http://127.0.0.1/fab/
# (multi-line commend don't line spaces after \, so this is NG): wget \ # commend
# same as:
# wget \
# -r \
# -p \
# -np \
# -P download \
# -nH \
# -E \
# -k \
# http://127.0.0.1/fab/
# same as:
wget -rpnp -nH -E -k "http://127.0.0.1/fab/"
# Clean
# Change to directory, otherwise RE will also be replaces
cd $STATIC
LC_CTYPE=C && LANG=C find ./ -type f -exec sed -i '' -e 's///g' {} \;
# Clean Theme Path
mv ${STATIC}* $STATIC
LC_CTYPE=C && LANG=C find ./ -type f -exec sed -i '' -e 's/wp-content\/themes\/fab\///g' {} \;
# Clean Image Path
mv ${STATIC}images/ ${STATIC}images
LC_CTYPE=C && LANG=C find ./ -type f -exec sed -i '' -e 's/wp-content\/uploads/images/g' {} \;
rm -r ${STATIC}/wp-content/
# clear TARGET
# .zsh, set 'setopt rmstarsilent' to allow silent rm *
rm -r ${TARGET}*
# copy to target directory
#-a does not copy invisible files
cp -a $STATIC* $TARGET
cd $TARGET
# check target directory
ls -la
# check sizes of files
# as per suggestion of Neil at Git Recitation
du -sh *
cd $STATIC
# git push manually, why? push only major updates to the site
Great tip at the Recitation: use du -sh *
to check sizes before committing
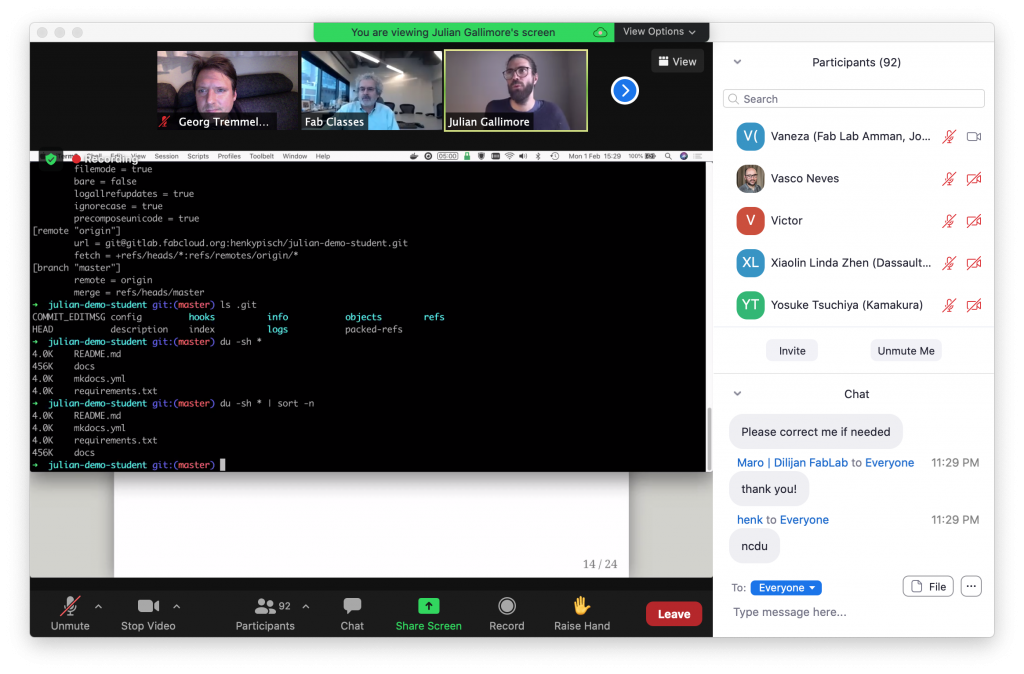
TODO
- Link to code & repo
- Update and evolve style during the FabAcademy