9. Input Devices
Group Assignment
In our group work, we tested a thermistor and a photoresistor using simple circuits with an Arduino Uno. I had minimal prior knowledge on the following, so I had to put more time into learning it. We started with the thermistor, which decreases in resistance as temperature increases. Using the following code, we read values from 0 to 1024, corresponding to 0V to VCC. Then, we tested it by touching it with our fingers and placing it against a tub of ice cream.
Then, we swapped in a photoresistor, which behaves similarly where more light means lower resistance. The same code was tested by turning the lights on and off, covering it with our hands.
Research
For this weeks assignment, I connected a flame sensor to my board.
These types of sensors are used for short range fire detection.
How it works: Research has showed me that the flame sensor is sensitive to IR wavelength to 760nm ~ 1100nm light.
Analog Output (A0): Provides a real-time voltage signal corresponding to the thermal resistance.
Digital Output (D0): Outputs a high or low signal when the temperature crosses a set threshold, which can be adjusted using the onboard potentiometer.
My Work
Pins and Connections
The fire sensor has three wires, red, yellow, and black. The black wire was connected into a ground port, the red into a 3.3 volt port, and the yellow into the A0 port.
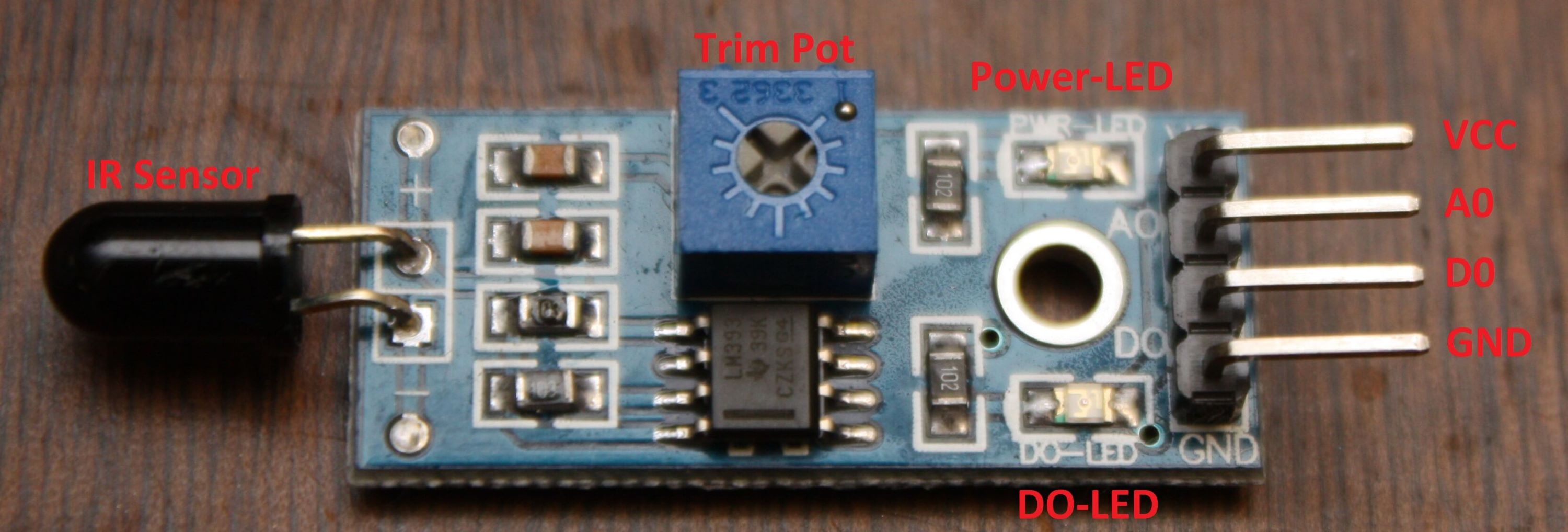
Code For Flame Sensor
I used a basic code for the flame sensor found on Arduino Project Hub. The code monitors an analog sensor to detect the presense and priximity of a fire. It then categorizes the fire as "Close", "Distant", or "No Fire" and prints that result. The following code also goes into depth on the functions of each portion of code. Arduino Modules - Flame Sensor
// lowest and highest sensor readings:
const int sensorMin = 0; // sensor minimum
const int sensorMax = 1024; // sensor maximum
void setup() {
// initialize serial communication @ 9600 baud:
Serial.begin(9600);
}
void loop() {
// read the sensor on analog A0:
int sensorReading = analogRead(A0);
// map the sensor range (four options):
// ex: 'long int map(long int, long int, long int, long int, long int)'
int range = map(sensorReading, sensorMin, sensorMax, 0, 3);
// range value:
switch (range) {
case 0: // A fire closer than 1.5 feet away.
Serial.println("** Close Fire **");
break;
case 1: // A fire between 1-3 feet away.
Serial.println("** Distant Fire **");
break;
case 2: // No fire detected.
Serial.println("No Fire");
break;
}
delay(1); // delay between reads
}
Working Fire Detector