Two Projects Communication
Summary
This week, I worked on implementing I2C communication between two microcontrollers: a RP2040 (acting as the master) and an ESP32 (acting as the slave).
I configured the I2C communication, where the RP2040 sends messages to the ESP32, which processes the data and forwards it to another RP2040. Additionally, I integrated WiFi communication between the ESP32 units to enable communication over a network, allowing one ESP32 to receive data from the RP2040 via I2C, then transmit it over WiFi to another RP2040.
Throughout this process, I had to troubleshoot issues such as incorrect data type conversions, especially with Wire.write(), and used proper data formatting to ensure the communication worked correctly.
The group assignment
Page :
Work Process Detail
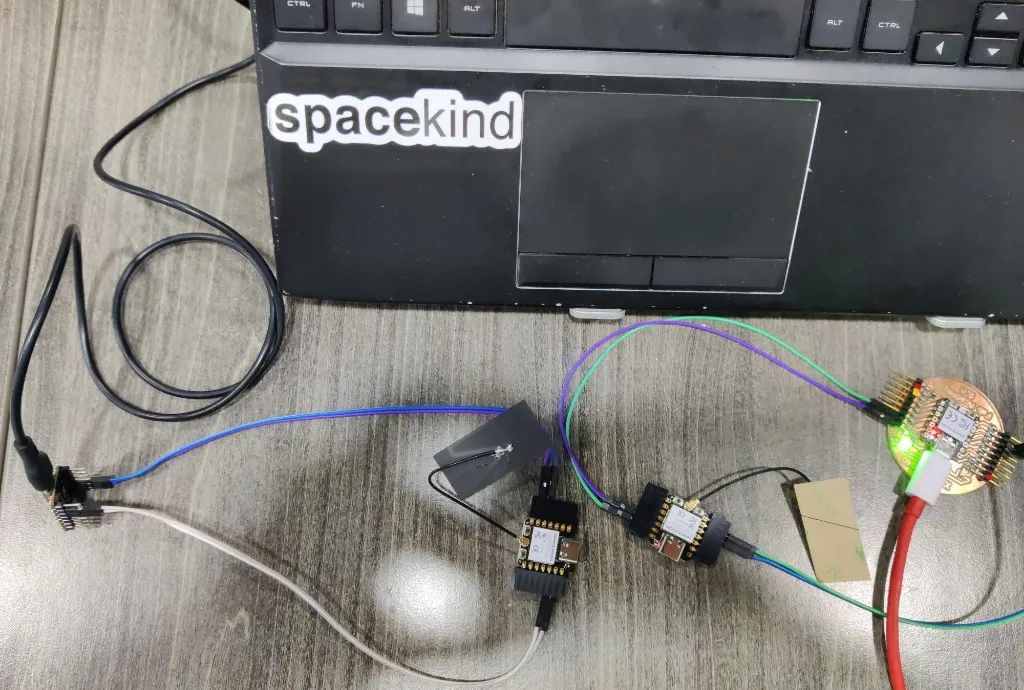
RP2040_1 --(I2C)--> ESP32_1 --(WiFi)--> ESP32_2 --(I2C)--> RP2040_2
Concept
- RP2040_1 (Master) → sends a message to ESP32_1 (Slave) over I2C
- ESP32_1 (WiFi Client) → sends that message over WiFi to ESP32_2 (WiFi Server)
- ESP32_2 (Master) → sends that message to RP2040_2 (Slave) over I2C
This is 100% possible 👍
Simple Flow
Step | Action |
1 | RP2040_1 sends text via I2C to ESP32_1 |
2 | ESP32_1 connects over WiFi to ESP32_2 and sends message |
3 | ESP32_2 receives WiFi message and sends it via I2C to RP2040_2 |
4 | RP2040_2 receives message and prints it |
Programming Hints
🟢 RP2040_1 (I2C Master)
Use Wire.h
library to send a string to ESP32_1.
#include <Wire.h>
void setup() {
Wire.begin();
}
void loop() {
const char* message = "Hello FAB25!";
Wire.beginTransmission(0x08); // Address of ESP32_1
Wire.write((const uint8_t*)message , strlen(message ));
Wire.endTransmission();
delay(5000);
}
🟢 ESP32_1 (I2C Slave + WiFi Client)
Use Wire.onReceive()
to get data from RP2040_1, then send via WiFi to ESP32_2.
#include <WiFi.h>
#include <Wire.h>
String i2cMessage = "";
void receiveI2C(int bytes) {
while (Wire.available()) {
i2cMessage += (char)Wire.read();
}
}
void setup() {
Wire.begin(0x08); // I2C Slave address
Wire.onReceive(receiveI2C);
WiFi.begin("ESP32_SERVER", "12345678");
while (WiFi.status() != WL_CONNECTED) delay(500);
}
void loop() {
if (i2cMessage.length() > 0) {
WiFiClient client;
if (client.connect("192.168.4.1", 80)) {
client.println(i2cMessage);
client.stop();
i2cMessage = ""; // Reset
}
}
🟢 ESP32_2 (WiFi Server + I2C Master)
Receive message over WiFi and send via I2C to RP2040_2.
#include <WiFi.h>
#include <Wire.h>
WiFiServer server(80);
void setup() {
Wire.begin();
WiFi.softAP("ESP32_SERVER", "12345678");
server.begin();
}
void loop() {
WiFiClient client = server.available();
if (client) {
String data = client.readStringUntil('\n');
Wire.beginTransmission(0x09); // Address of RP2040_2
Wire.write((const uint8_t*)data.c_str(), data.length());
Wire.endTransmission();
client.stop();
}
🟢 RP2040_2 (I2C Slave)
Just print the received message.
#include <Wire.h>
void receiveI2C(int bytes) {
while (Wire.available()) {
char c = Wire.read();
Serial.print(c);
}
}
void setup() {
Serial.begin(115200);
Wire.begin(0x09); // I2C Slave address
Wire.onReceive(receiveI2C);
}
void loop() {
delay(100);
}
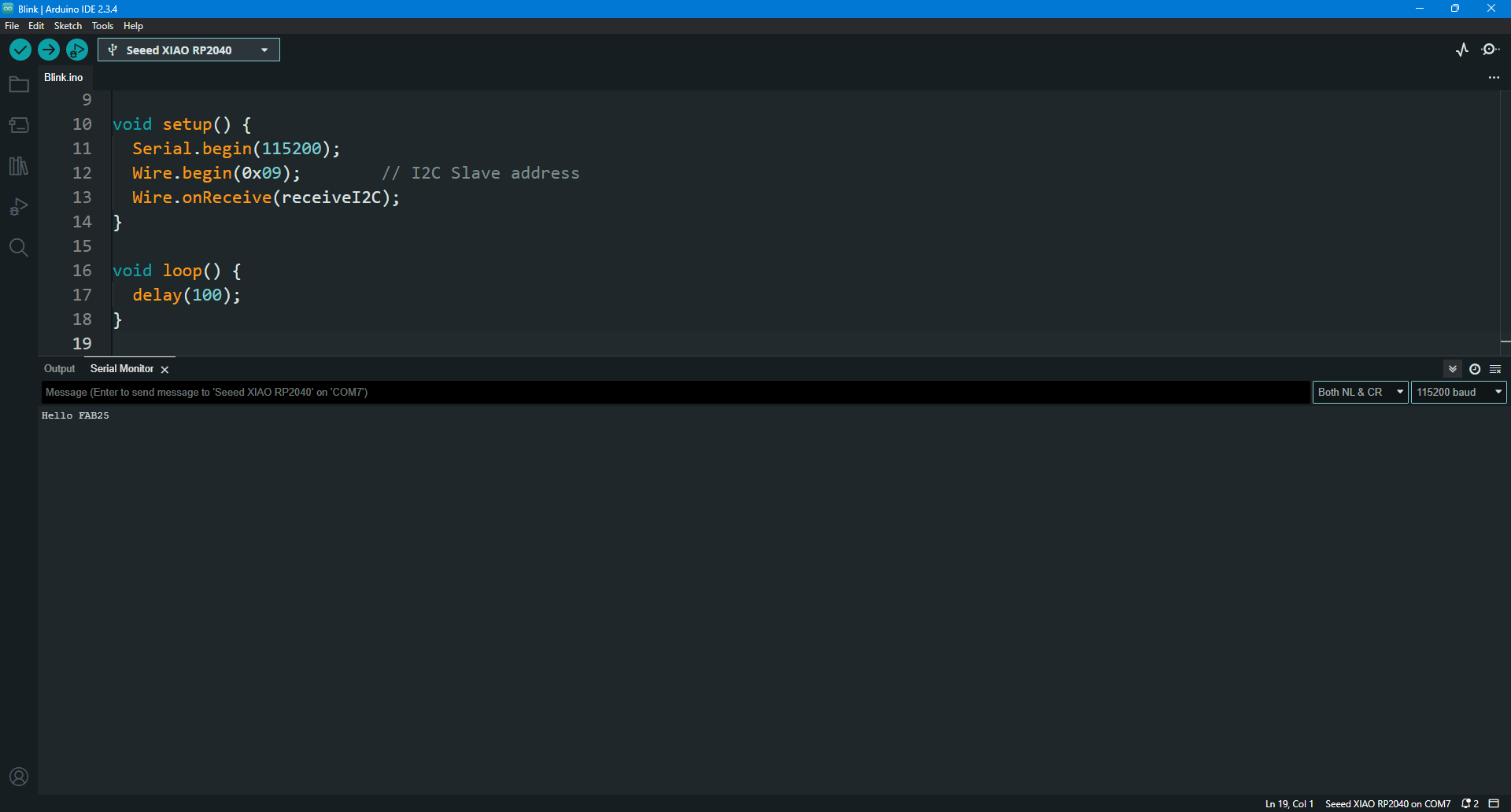
Learning Outcome
- I2C Communication Setup: Learned how to establish I2C communication between different microcontrollers (RP2040 & ESP32).
- Data Type Management: Gained experience handling data type conversions between different microcontrollers and communication protocols.
- WiFi Integration: Expanded knowledge of WiFi-based communication between devices and how it complements I2C.
- Debugging: Improved debugging skills when addressing issues related to data transfer and compatibility between devices.
- Practical Use of Communication Protocols: Deepened understanding of serial communication protocols (I2C & WiFi) in embedded systems and their integration for more complex setups.