█████ █████ ██████████ █████ █████ █████ █████ ███ ░░███ ░░███ ░░███░░░░░█ ░░███ ░░███ ░░███ ░░███ ░░░ ░███ ░███ █ ░███ █ ░ █████████████ ░███████ ██████ ███████ ███████ ██████ ███████ ████████ ████████ ██████ ███████ ████████ ██████ █████████████ █████████████ ████ ████████ ███████ ░███████████ ░██████ ░░███░░███░░███ ░███░░███ ███░░███ ███░░███ ███░░███ ███░░███ ███░░███ ░░███░░███░░███░░███ ███░░███ ███░░███░░███░░███ ░░░░░███ ░░███░░███░░███ ░░███░░███░░███ ░░███ ░░███░░███ ███░░███ ░░░░░░░███░█ ░███░░█ ░███ ░███ ░███ ░███ ░███░███████ ░███ ░███ ░███ ░███ ░███████ ░███ ░███ ░███ ░███ ░███ ░░░ ░███ ░███░███ ░███ ░███ ░░░ ███████ ░███ ░███ ░███ ░███ ░███ ░███ ░███ ░███ ░███ ░███ ░███ ░███░ ░███ ░ █ ░███ ░███ ░███ ░███ ░███░███░░░ ░███ ░███ ░███ ░███ ░███░░░ ░███ ░███ ░███ ░███ ░███ ░███ ░███░███ ░███ ░███ ███░░███ ░███ ░███ ░███ ░███ ░███ ░███ ░███ ░███ ░███ ░███ ░███ █████ ██ ██████████ █████░███ █████ ████████ ░░██████ ░░████████░░████████░░██████ ░░████████ ░███████ █████ ░░██████ ░░███████ █████ ░░████████ █████░███ █████ █████░███ █████ █████ ████ █████░░███████ ░░░░░ ░░ ░░░░░░░░░░ ░░░░░ ░░░ ░░░░░ ░░░░░░░░ ░░░░░░ ░░░░░░░░ ░░░░░░░░ ░░░░░░ ░░░░░░░░ ░███░░░ ░░░░░ ░░░░░░ ░░░░░███░░░░░ ░░░░░░░░ ░░░░░ ░░░ ░░░░░ ░░░░░ ░░░ ░░░░░ ░░░░░ ░░░░ ░░░░░ ░░░░░███ ░███ ███ ░███ ███ ░███ █████ ░░██████ ░░██████ ░░░░░ ░░░░░░ ░░░░░░
group assignments
in the group task, the programming of devices, using various platforms and tools. Environments such as Arduino, Python, and PlatformIO were explored.
Exploring from the basics within programming, from variable declaration to I2C and UART communications.
One of the key focuses was programming microcontrollers like ATtiny and ESP32, where I learned to optimize memory and resource usage to improve device performance. Communication protocols such as I2C and UART, essential for interaction between sensors and actuators, were also explored.
Grupal AssignmentsIntroduction to Microcontrollers
There are numerous microcontrollers available today, each with unique features. Which one ought to be used, then? In order to respond, we need to take into account a number of factors:
- Type of memory: What kind of memory is required, and how much does it have? (RAM, EEPROM, FLASH)
- Speed: Performance is impacted by processing speed.
- Power consumption: Crucial for applications that run on batteries.
- Connectivity: Does it allow Bluetooth, WiFi, or other forms of communication?
- Number of pins: Digital, analog, PWM, and other input/output choices are included.
- Dimensions: In small applications, size may be important.
- Interface: Protocols for communication such as SPI, UART, I²C, and EDP.
- Cost: Mass production budget considerations.
The microcontroller's datasheet has technical information for all of these features, with the exception of cost.
What is a Microcontroller?
An integrated circuit made specifically for electronic system control is called a microcontroller. It includes:
- The central processing unit, or CPU, processes data and carries out commands.
- RAM: Program-running temporary memory.
- User programs and firmware are stored in flash memory.
- Peripherals: Timers, ADC/DAC, and communication interfaces such as I²C, SPI, and UART.
- GPIOs: Sensors, actuators, and other devices are connected to general-purpose input/output pins.
Microcontrollers are more effective for embedded applications than microprocessors since they combine all required parts onto a single chip.
Types of Microcontrollers
Microcontrollers vary in architecture, impacting performance and capabilities:
- 8-bit MCUs: Low power, simple applications (e.g., ATmega328 in Arduino Uno).
- 16-bit MCUs: More processing power, used in industrial automation.
- 32-bit MCUs: High performance, often found in IoT and robotics (e.g., ESP32, STM32, RP2040).
Popular Microcontrollers and Their Features
Microcontroller | Bits | Speed | Memory | Connectivity |
---|---|---|---|---|
ATmega328 (Arduino Uno) | 8-bit | 16 MHz | 2KB RAM, 32KB Flash | No built-in connectivity |
ESP32 | 32-bit | 240 MHz | 520KB RAM, 4MB Flash | WiFi, Bluetooth |
STM32 | 32-bit | Up to 480 MHz | Varies | SPI, I²C, UART |
RP2040 | 32-bit | 133 MHz | 264KB RAM, 2MB Flash | No built-in connectivity |
The Basics for Learning to Program
Programming is the procedure of writing and structuring instructions for a computer to carry out specific tasks. These guidelines have been written in a programming language, which converts the code into a format that the machine can understand and execute.
Programming is based on three fundamental principles:
- Input: The computer receives data from the user or from sensors.
- Processing: Calculations, decisions, and operations are made with the data.
- Output: A result is generated, such as displaying information on the screen or activating a device.
Language Overview
Language | Creator and Year | Main Purpose | Problems it solves | Unique Features | Advantages | Disadvantages |
---|---|---|---|---|---|---|
Python | Guido van Rossum, 1991 | Web development, AI, data science, automation | Simplify development with readable code | Interpreted, dynamic typing, extensive standard library | Easy to learn, versatile, extensive community | Less efficient than C/C++, high memory consumption |
C | Dennis Ritchie, 1972 | OS development, low-level programming | Delivers hardware efficiency and control | Mid-level language, memory access with pointers | Fast, efficient, used in critical systems | Difficult to learn, prone to memory errors |
C++ | Bjarne Stroustrup, 1983 | High-performance applications, video games, embedded systems | Extend C with OOP and generic programming | Support for OOP, more control over resources | High performance, flexible memory management | High complexity, difficult to debug errors |
Assembly | Various (1940-1950) | Low-level programming, direct hardware control | Maximize hardware performance and efficiency | Lower-level, architecture-dependent language | Maximum control, efficiency on specific hardware | Complex, processor dependent |
Java | James Gosling, 1995 | Business applications, mobile, web | Portability to multiple platforms with the JVM | "Write once, run anywhere" (WORA) | Portability, strong community, automatic memory management | High resource consumption, speed lower than C++ |
JavaScript | Brendan Eich, 1995 | Frontend and backend web development | Makes web pages dynamic | Executed in browser, asynchrony with event loop | Versatile, run in a browser without additional installation | Browser dependency, security issues |
Rust | Mozilla Foundation, 2010 | Security and performance in secure software | Prevents memory errors | Focus on security without garbage collector | Memory security without losing performance, high efficiency | Steep learning curve, slow builds |
Key Programming Concepts
0. Libraries
Libraries are collections of functions and definitions that allow programmers to reuse code, simplifying complex tasks. In Arduino, they are files that facilitate interaction with hardware.
1. Common Syntax Rules
Syntax rules in programming dictate how to write code correctly. They include reserved keywords, declaration of variables with type and name, delimiters to separate statements, and the use of indentation, quotes, and comments. They also address operators and control structures to manage program flow.
Variables:
In Python, variables are declared dynamically, while in C/C++ they require a static declaration. Python allows changing the type of variables, while C/C++ prohibits it without explicit conversion.
2. Control Structures
Control structures manage the flow of code execution. Conditionals (if, else) and loops (for, while) are essential for making decisions and repeating actions. Although Python does not have a native switch statement, it can be simulated with control structures.
3. Functions
Functions are reusable blocks of code that perform specific tasks, allowing you to modularize and simplify your code. They facilitate debugging and maintenance, improving collaboration and program scalability.
4. Comments
Comments are annotations in the code that do not affect its execution, helping other programmers better understand what each block of code does.
5. Initialization of a Pin
Initializing a pin involves configuring a microcontroller pin as an input or output, allowing the reading of sensors or the control of devices.
6. Reading a Pin
Reading a pin means getting its value set as input. On Arduino, this is done with digitalRead()
and analogRead()
.
7. Writing to a Pin
Writing to a pin involves sending signals to a pin configured as an output, allowing control of devices such as LEDs.
8. Analog Reading with ADC
Analog reading refers to obtaining values from continuous signals. An analog to digital converter (ADC) is used to convert the analog signal into a processable digital value.
9. Timers
Timers in microcontrollers manage the frequency of execution of parts of the code, useful for tasks such as flashing an LED or regular sensor readings.
10. Printing
Console printing displays information or results from a program, using functions such as print()
in Python or Serial.println()
in C/C++.
11. Pulse Width Modulation (PWM)
PWM converts digital signals to analog, controlling devices such as servomotors through the duration of the pulses sent.
12. Interruptions
Interrupts allow a microcontroller to interrupt its current execution to execute a specific function by detecting events such as changes in signals.
Using a XIAO RP2040
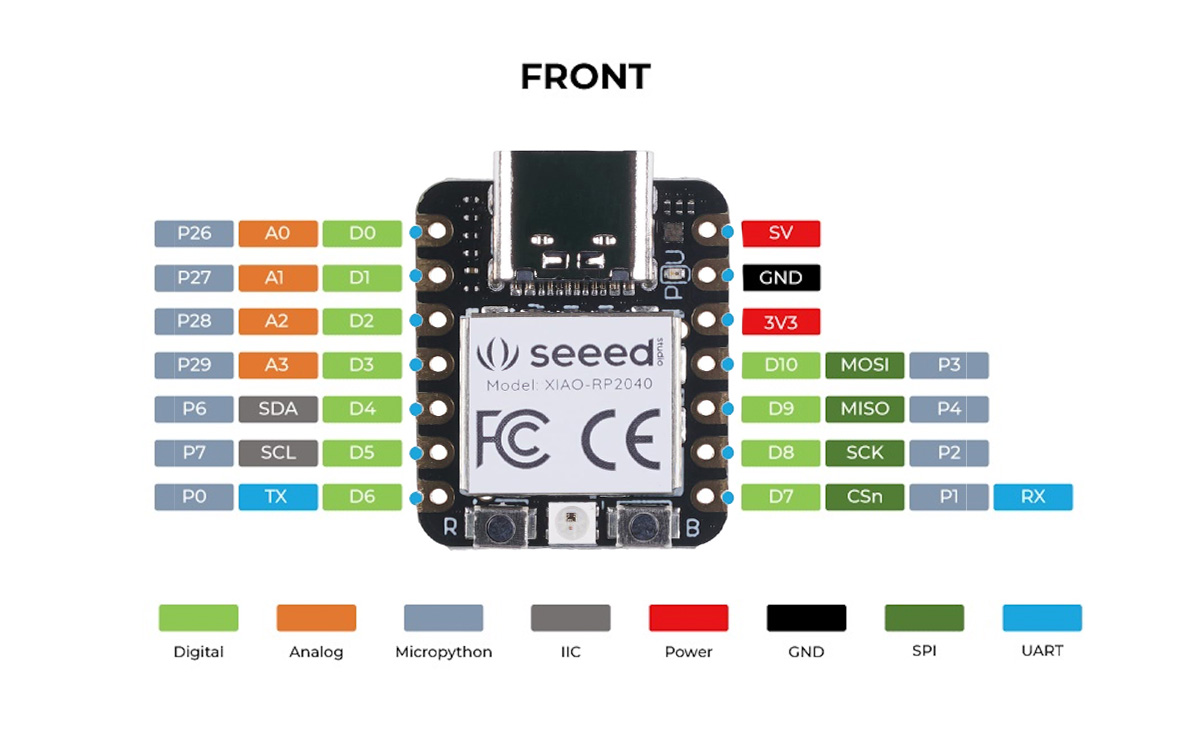
Seeed Studio's XIAO RP2040 board features an RP2040 dual-core processor, 264KB of SRAM memory, and 2MB of built-in Flash memory. It has an adaptive clock that can operate up to 133 MHz, making it ideal for portable devices and small initiatives. The board features 14 GPIO pins, including 11 digital, 4 analog, 11 PWM, I2C, 1 UART, 1 SPI, and 1 SWD bonding pad interface.
rp2040_datasheetSpecification
Item | Value |
---|---|
CPU | Dual-core ARM Cortex M0+ processor up to 133MHz |
Flash Memory | 2MB |
SRAM | 264KB |
Digital I/O Pins | 11 |
Analog I/O Pins | 4 |
PWM Pins | 11 |
I2C interface | 1 |
SPI interface | 1 |
UART interface | 1 |
Power supply and downloading interface | Type-C |
Power | 3.3V/5V DC |
Dimensions | 21×17.8×3.5mm |
For more information about this microprocessor: https://wiki.seeedstudio.com/XIAO-RP2040/
In addition to that microcontroller, I chose to incorporate a color sensor, since I want to incorporate it into my robot. An example of how it would work would be to pick up something that is a different color than the floor, like a red sock.
Why this?
I want my final project to have the ability to grab objects and "carry" them, so I think a good start would be to recognize the color of things and distinguish them from the ground.
TCS34725
The TCS34725 is an RGB color sensor equipped with an anti-infrared filter and a programmable gain amplifier. It operates by identifying the intensity of red, green, blue, and white light (without a color filter) and transforming it into digital signals that are understandable by a microcontroller.
Main features:
- Integrated IR blocking filter: Minimizes interference caused by infrared light, increasing the accuracy of color identification.
- Large dynamic spectrum: It is appropriate for different light conditions, from environments with low light to those with high light intensity.
- I2C System: Promotes communication with microcontrollers and other devices, which facilitates its integration into different projects.
- 16-bit Analogue-Digital Converters (ADC): They offer high resolution in color measurements.
Common uses:
- Backlight regulation in monitors: Dynamic modification of the backlight depending on the surrounding light conditions.
- Evaluation of color temperature: Establishment of the color temperature of light resources for lighting uses.
Programming with a XIAO RP2040
Push Button to Blink LED
Hardware Connection:
Component | Connection | Description |
---|---|---|
Seeed XIAO RP2040 | - | Main microcontroller |
Push Button | - One end to the pin D0 of the XIAO RP2040 - Another end to 3.3V |
Input button to control the LED |
Resistance 10kΩ | - One end to the pin D0 - Another end to GND |
Avoid floating states (pull-down) |
Internal LED | - Connected to pin 25 of the XIAO RP2040 | No external wiring required |
const int buttonPin = D0; // the number of the pushbutton pin
const int ledPin = 25; // the number of the LED pin
// const indicates that a variable or object cannot be modified
int buttonState = 0; // variable for reading the pushbutton status
void setup() {
// initialize the LED pin as an output:
pinMode(ledPin, OUTPUT);
// initialize the pushbutton pin as an input:
pinMode(buttonPin, INPUT);
}
void loop() {
// read the state of the pushbutton value:
buttonState = digitalRead(buttonPin);
if (buttonState == HIGH) {
// turn LED on:
digitalWrite(ledPin, HIGH);
} else {
digitalWrite(ledPin, LOW);
}
}
Color Sensor
Hardware Connection:
The TCS34725 uses I2C communication (referring to the abbreviation for Inter-IC, a type of bus created by Philips Semiconductors in the early 1980s, used to join integrated circuits), so you must connect its pins to the I2C pins of the XIAO RP2040.
TCS34725 | XIAO RP2040 | Description |
---|---|---|
VIN | 3V3 | Power (3.3V) |
GND | GND | - |
SDA | GP6 | I2C data |
SCL | GP7 | I2C clock |
INT | (Optional) | Interrupt (you can leave it unconnected) |
LED | (Optional) | Integrated LED control |
Arduino Code (C++)
Here we use Arduino and add the library to the code Adafruit_TCS34725
from the Library Manager.
Code:
1. Included Libraries
#include // Library for I2C communication
#include "Adafruit_TCS34725.h" // Sensor-specific library
Wire.h
: It is the standard Arduino library for managing I2C devices.
Adafruit_TCS34725.h
: Adafruit library that facilitates communication with the TCS34725 sensor.
2. Creating the Sensor Object
Adafruit_TCS34725 tcs = Adafruit_TCS34725(TCS34725_INTEGRATIONTIME_700MS, TCS34725_GAIN_4X);
Here we create an object called tcs
to communicate with the sensor. Two important parameters are configured:
- Integration time:
TCS34725_INTEGRATIONTIME_700MS
- Controls how long the sensor captures light before sending data. A higher value improves accuracy in dark environments. - Gain:
TCS34725_GAIN_4X
- Increases sensor sensitivity. Options: 1X, 4X, 16X, 60X (higher values capture more light).
3. Initial Setup setup()
void setup() {
Serial.begin(115200);
Wire.setSDA(6); // GP6 on XIAO RP2040
Wire.setSCL(7); // GP7 on XIAO RP2040
Wire.begin();
Serial.begin(115200);
→ Starts serial communication at 115200 baud.
Wire.setSDA(6);
→ Configure GP6 as the SDA line.
Wire.setSCL(7);
→ Configure GP7 as the SCL line.
Wire.begin();
→ Starts I2C communication.
Sensor Check
if (!tcs.begin()) {
Serial.println("TCS34725 sensor not found.");
while (1);
}
tcs.begin()
tries to communicate with the sensor. If it can't find it, it prints the message "TCS34725 sensor not found." and enters an infinite loop (while(1)
) to stop the program.
4. Reading Data in loop()
void loop() {
uint16_t r, g, b, c;
tcs.getRawData(&r, &g, &b, &c);
Four variables r
, g
, b
, and c
of type uint16_t
(values from 0 to 65535) are declared.
tcs.getRawData(&r, &g, &b, &c);
gets the raw values of the Red, Green, Blue, and Clear Light channels.
5. Show Data in Serial
Serial.print("R: "); Serial.print(r);
Serial.print(" G: "); Serial.print(g);
Serial.print(" B: "); Serial.print(b);
Serial.print(" C: "); Serial.println(c);
This displays the values R, G, B, and C on the Arduino Serial Monitor.
"C" is the total amount of light detected.
6. Pause Before Next Reading
delay(1000);
delay(1000);
→ Wait 1 second before taking another reading.
Final Notes:
The XIAO RP2040 works with 3.3V, so the TCS34725 should also run at 3.3V to avoid damaging the board.