Objectives
Group assignment: probe an input device's analog levels and digital signals
Individual assignment: measure something: add a sensor to a microcontroller board that you have designed and read it
Group assignment
In group assignment, we focused on analyzing input devices by probing their analog and digital signals using an oscilloscope. We tested the INMP441 MEMS microphone, which produces a digital signal, and the PS2 joystick module, which outputs an analog signal. The oscilloscope was used to measure and visualize voltage variations from both sensors.
Group assignment pageInput Devices
Sensors
Sensors are devices that detect and measure changes in their surrounding environment and convert them into electrical signals. These changes can be in the form of light, temperature, pressure, motion, humidity, sound, chemical composition, or other physical properties. Sensors play a crucial role in modern technology by enabling automated systems, data collection, and real-time monitoring.
As my final project consist of proximity sensor and digital clock I wanted to explore these sensors.
Proximity Sensor : A proximity sensor is a non-contact sensing device designed to detect the presence or absence of an object within its range without requiring physical interaction. These sensors operate by emitting electromagnetic fields, infrared signals, or sound waves and analyzing their reflections or disruptions caused by nearby objects. Their non-invasive nature makes them particularly useful for applications involving fragile, sensitive, or hazardous materials where direct contact could cause damage, contamination, or interference.
RTC module : An RTC (Real-Time Clock) module is an electronic component that maintains accurate time and date, even when the main power supply is unavailable, by using a built-in battery backup. It consists of an integrated circuit (IC) with a timer, a crystal oscillator for precise timekeeping, and a backup battery to ensure continuous operation. RTC modules are widely used in embedded systems, IoT devices, data loggers, and security applications like alarm systems and surveillance cameras, where reliable timekeeping is essential.
Designing the PCB
To design the PCB I used ATTINY 1614 microcontroller. The microcontroller needs an additional programmer. The design consist of a power LED and three connectors of which two are used for sensor modules.
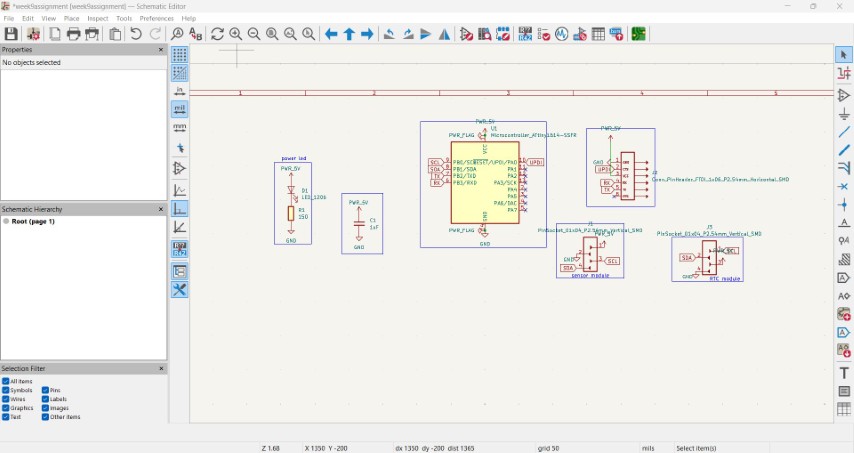
To check the connection use Ruleschecker tool
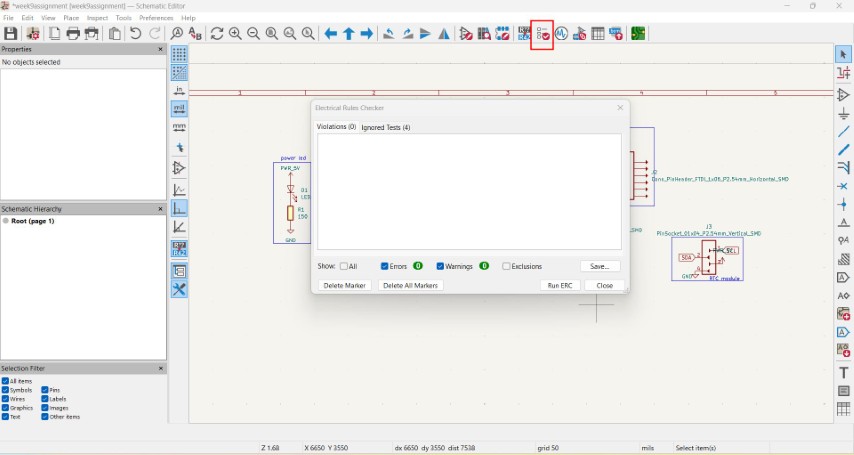
Open the schematic editor. We need to specify the design rules
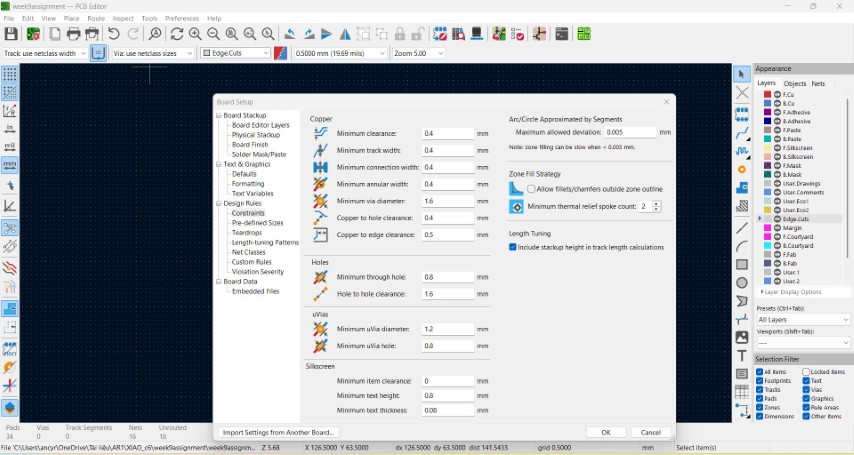
To trace route use the shortcut X or use the route tracks tool in the right panel. Click on edgecuts and using rectangle in tools option draw the outline. Rightclick on the outline and use shape modification option for reshaping outline. You can add text using text tool.
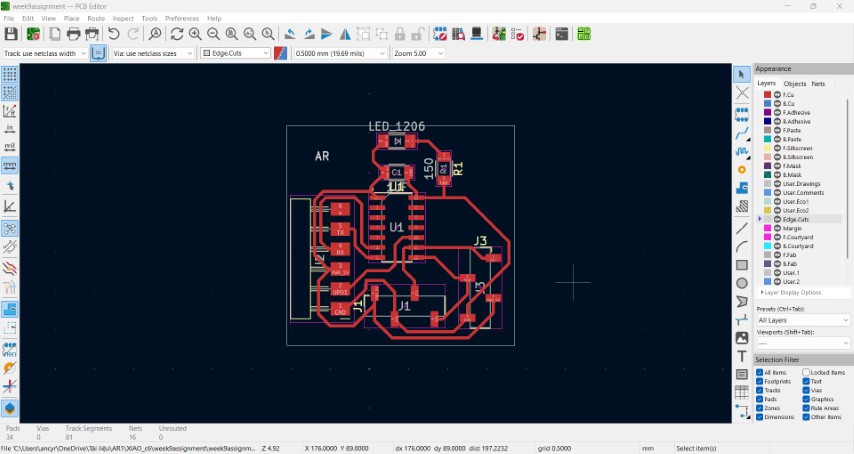
Check the traces using rules checker tool. from file -> Fabrication outputs -> gerbers , we get the gerber format. the gerber files are uploaded in gerber2PNG software to convert it to png format.
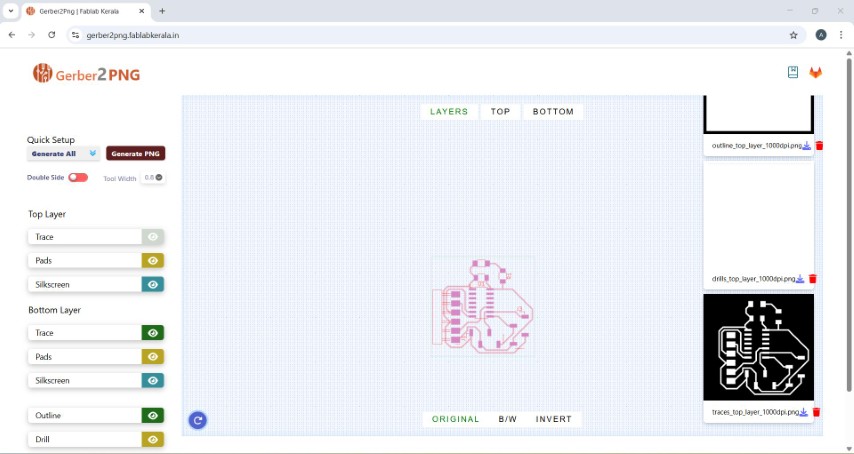
Milling the PCB
Open Mods CE. Upload the trace layer. I used 0.2mm 60 deg V bit. Millling was done in Modela MDX-20 3D Milling Machine. I set origin as x:6 and y:4. Set the zero position (X, Y, and Z-axis) manually. Adjust milling parameters like spindle speed, feed rate, and depth of cut based on the material and tool specifications.
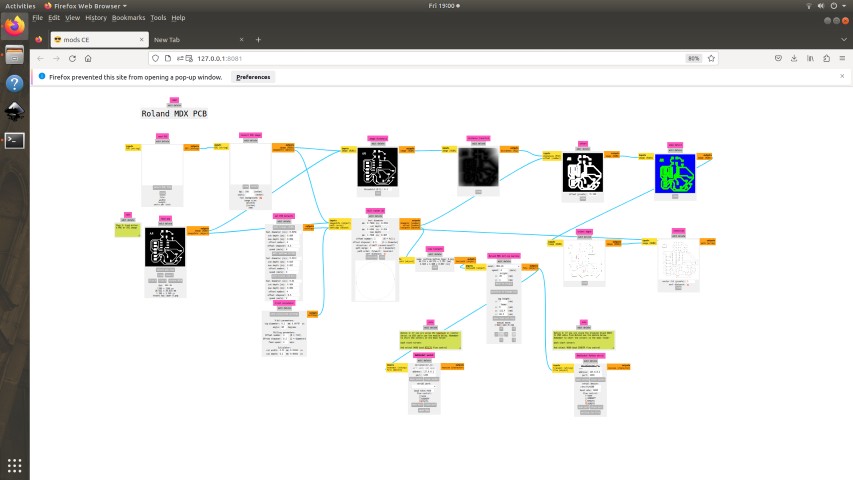
we can view the trace toolpath
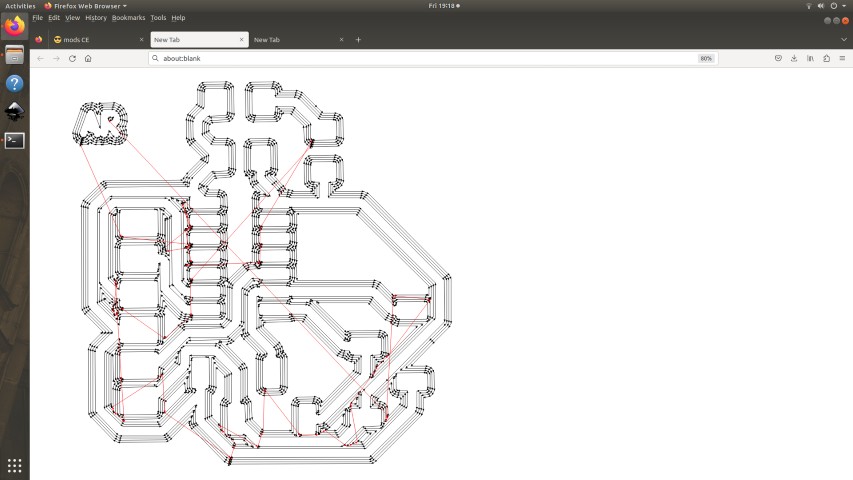
Stick the copper-clad board in a double sided tape
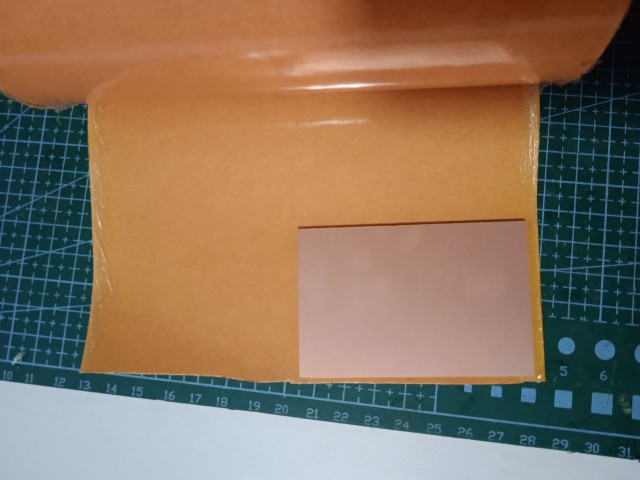
Secure the board in the bed. The double sided tape keeps the board firm in bed
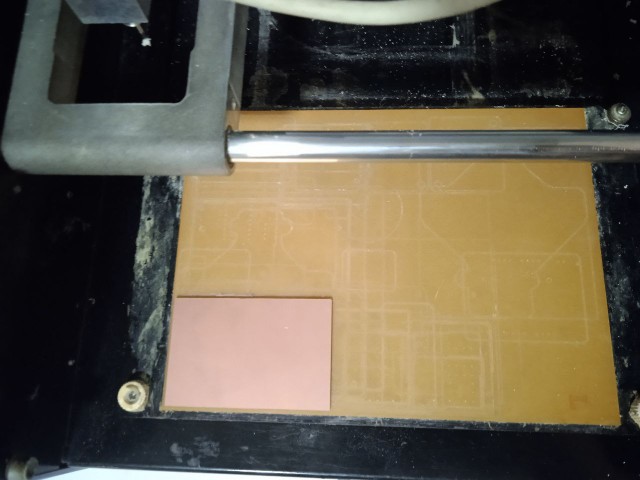
Click send file option in mods to start engraving the trace
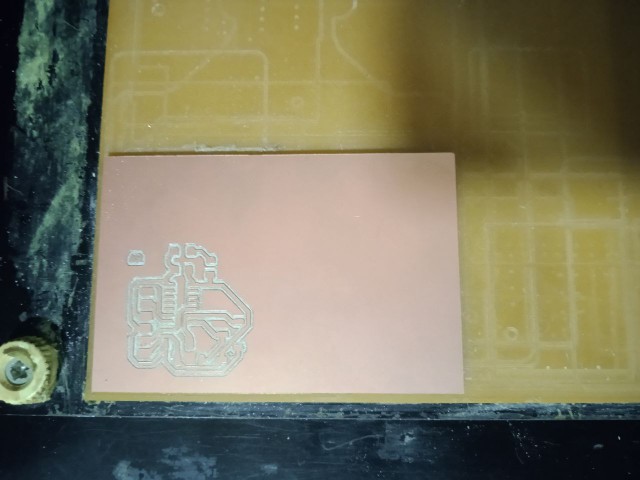
After engraving the trace, we have to cut the outline. The outline layer is oploaded in mods CE.We use 1/32" bit.
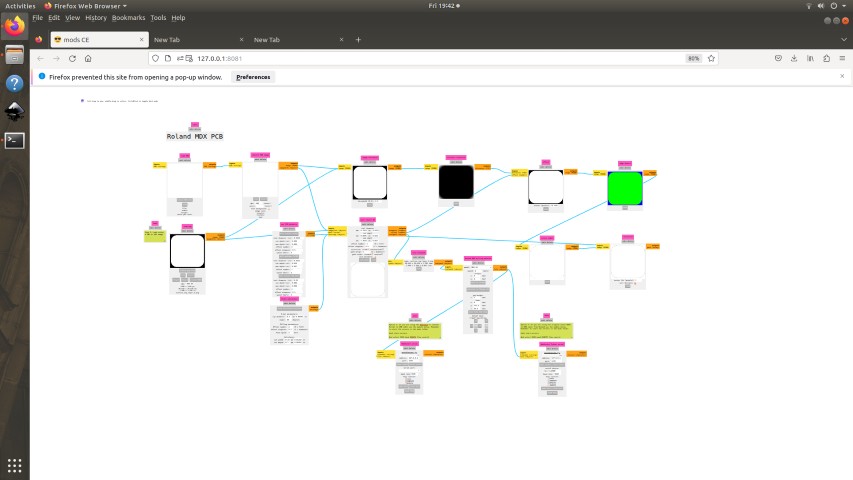
The toolpath is shown below.
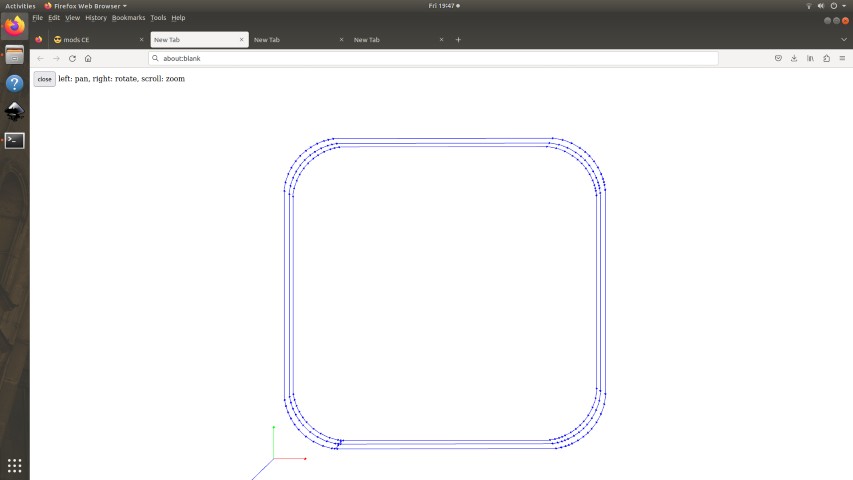
Using send file option initiate the cutting process.
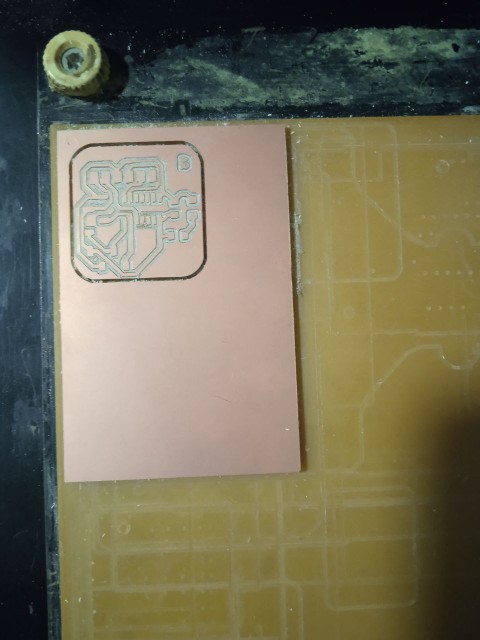
Use a vaccumcleaner to remove dust and debris. Use a scraper to lift the board.And here is the final result.
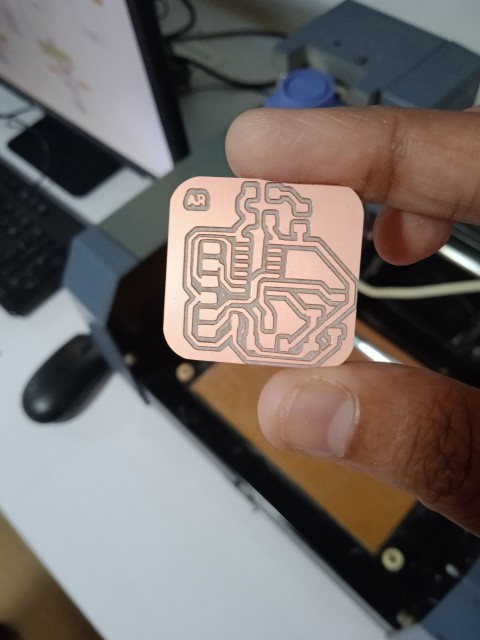
Mistakes
Initially the tool depth wasn't enough to engrave properly.So I had to stop the process. I used the down button in pannel key to make sure the engrave is in right depth.
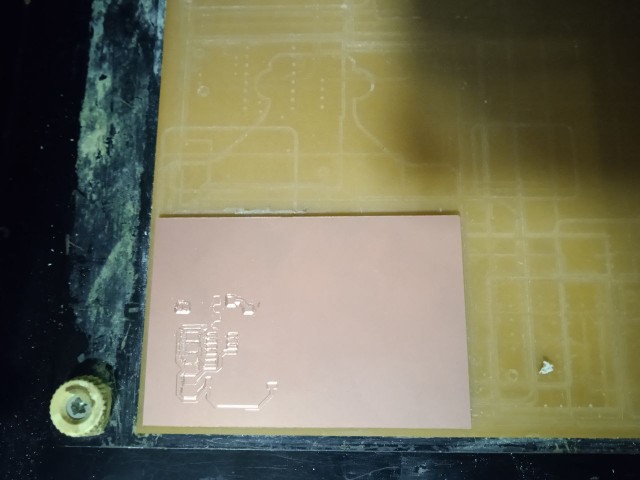
Assembling the PCB
Interactive HTML BOM is generated in KiCad and the components were taken from inventory.
.jpeg)
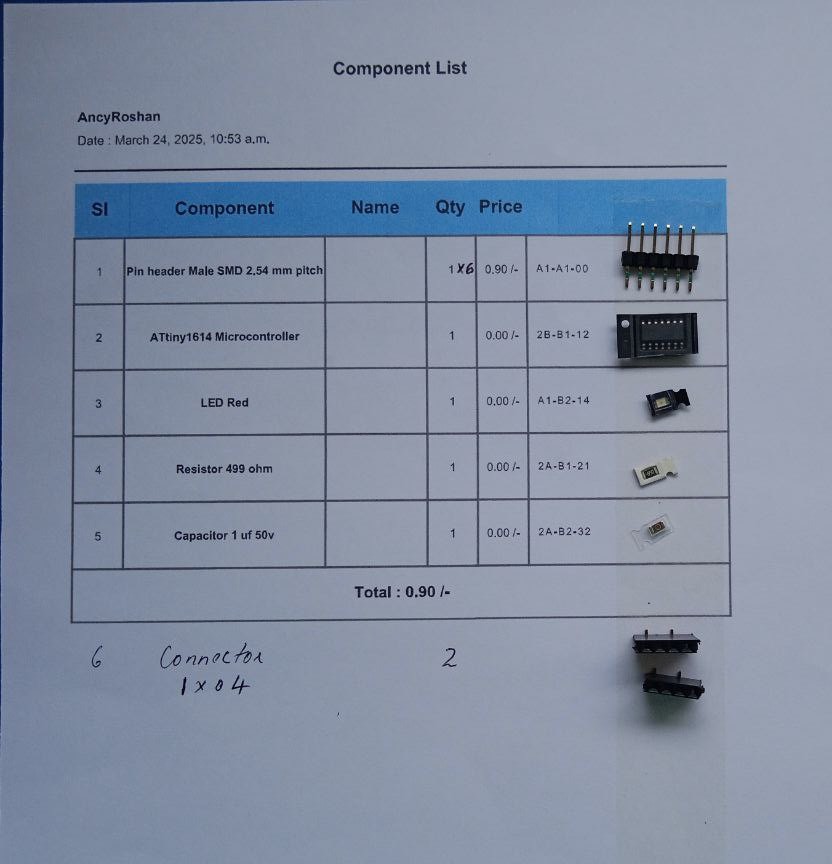
ATTINY 1614 Pin out diagram
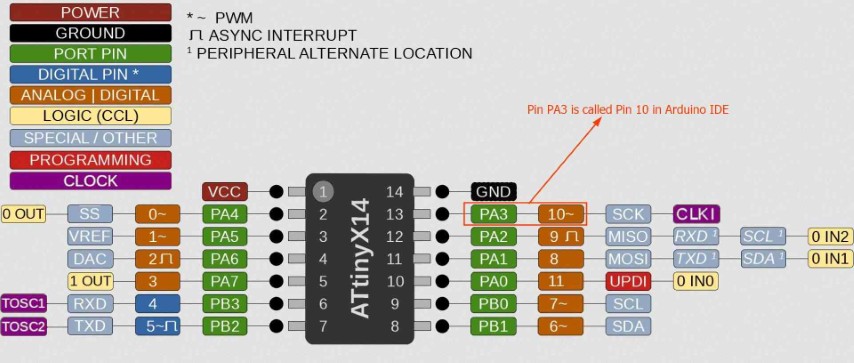
VL53L0X module
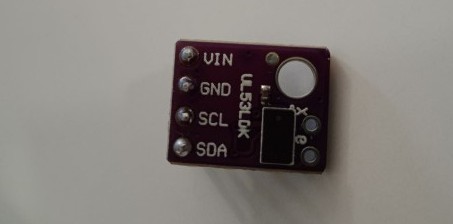
The VL53L0X contains a 940 nm infrared (IR) vertical-cavity surface-emitting laser (VCSEL) that emits a short pulse of laser light. This laser pulse travels toward the target object. When the laser pulse hits an object, it gets reflected back toward the sensor. The Single Photon Avalanche Diode (SPAD) array in the VL53L0X detects the returning photons. The SPAD is highly sensitive and can detect even a single photon of light. The sensor measures the time delay between emitting the laser pulse and detecting the reflected signal.
RTC module
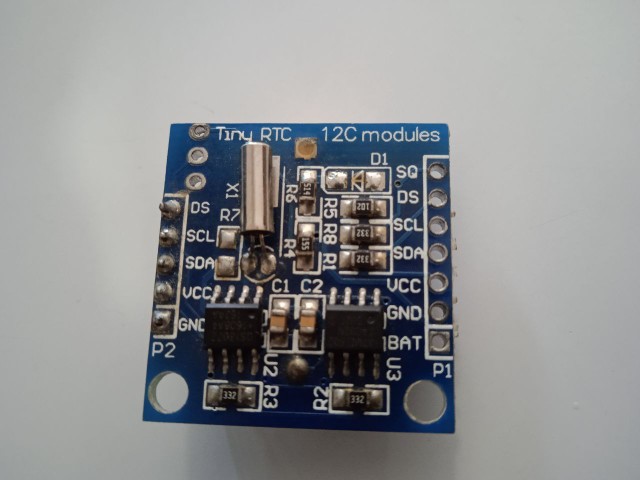
The working principle of a Real-Time Clock (RTC) module is based on a crystal oscillator and a battery backup to maintain accurate timekeeping independently of the main power supply. The module uses a quartz crystal oscillator, which provides a stable frequency that is divided down to generate precise one-second time intervals. An internal counter keeps track of seconds, minutes, hours, days, months, and years, with some models also handling leap year adjustments. When the main power is available, the RTC operates normally and synchronizes with the system via an I²C interface. If power is lost, a small backup battery ensures continuous operation, preventing time resets.
The components are soldered into the PCB
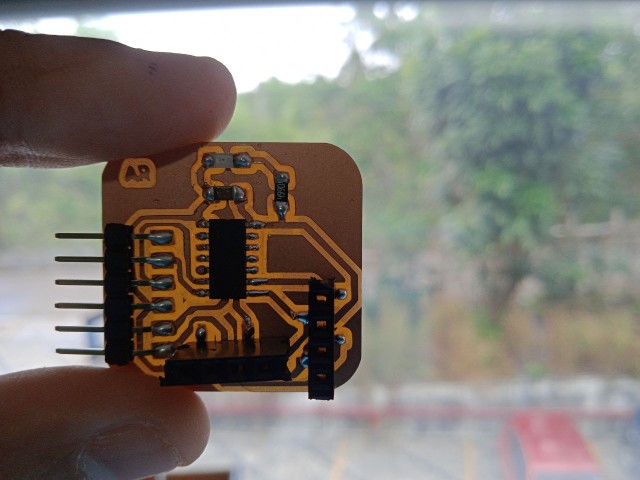
Inspection
To check the connection I checked the PCB under a microscope and checked the connections with multimeter.
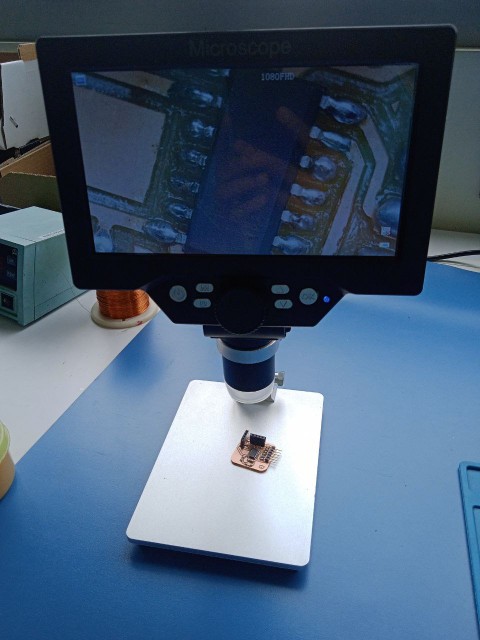
Mistakes
I lost an LED while soldering. The components are very small and need careful handling.
programmer
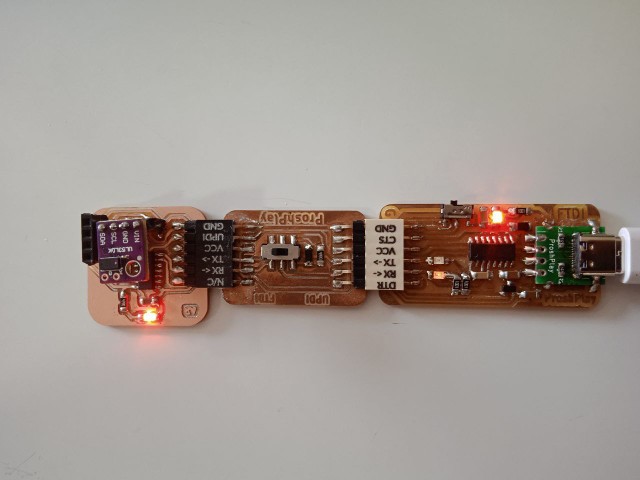
I used the programmer that was avilable in the lab made by Saheen Palayi. The links are given below. The programmer consists of FTDI board and FTDI to UPDI converter. It is then connected to computer with a USB port.
FT232l FTDI ProgramerCH340 typr C Programmer
UPDI Converter
Programming
VL53L0X Sensor module
To program in Arduino IDE I followed the steps in group assignment, week 4
week4 groupassignmentChoose the correct programmer and the chip to ATtiny 1614. I uploaded an example program and it worked.
I used chatgpt for code reference. Prompt:write a program for VL53L0X with ATTINY 1614.
#include <Wire.h> // Use Wire.h for ATtiny1614 I2C #include <VL53L0X.h> // Pololu VL53L0X Library VL53L0X sensor; // Declare sensor object void setup() { Wire.begin(); // Initialize hardware I2C for ATtiny1614 Serial.begin(9600); // Use SoftwareSerial if needed if (!sensor.init()) { // Initialize VL53L0X Serial.println("VL53L0X Init Failed!"); while (1); } sensor.setTimeout(500); sensor.startContinuous(); } void loop() { uint16_t distance = sensor.readRangeContinuousMillimeters(); if (sensor.timeoutOccurred()) { Serial.println("Timeout!"); } else { Serial.print("Distance: "); Serial.print(distance/10); Serial.println(" cm"); } delay(100); }
And the result
I wanted to add a programmable LED. The 13 th pin of microcontroller was used for the purpose. The pin is called pin 10 in arduino. I tried the blink program given in the examples and it worked.My idea was to vary the intensity of the brightness in led according to distance. The program I used is given below
#include <Wire.h> #include <VL53L0X.h> VL53L0X sensor; int LED = 10; void setup() { Wire.begin(); Serial.begin(9600); pinMode(LED, OUTPUT); if (!sensor.init()) { Serial.println("VL53L0X Init Failed!"); while (1); } sensor.setTimeout(500); sensor.startContinuous(); } void loop() { uint16_t distance = sensor.readRangeContinuousMillimeters(); if (sensor.timeoutOccurred()) { Serial.println("Timeout!"); } else { Serial.print("Distance: "); Serial.print(distance / 10); Serial.println(" cm"); } delay(100); if (distance/10 <20) { int brightness = map(distance / 10, 20, 0, 0, 255); brightness = constrain(brightness, 0, 255); analogWrite(LED, brightness); } else { analogWrite(LED, 0); } }
RTC module
I followed a tutorial to program RTC module. The program is given below
#include "Arduino.h" #include "uRTCLib.h" // uRTCLib rtc; uRTCLib rtc(0x68); char daysOfTheWeek[7][12] = {"Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday"}; void setup() { Serial.begin(9600); delay(3000); // wait for console opening URTCLIB_WIRE.begin(); // Comment out below line once you set the date & time. // Following line sets the RTC with an explicit date & time // for example to set January 13 2022 at 12:56 you would call: rtc.set(0, 56, 12, 5, 13, 1, 22); // rtc.set(second, minute, hour, dayOfWeek, dayOfMonth, month, year) // set day of week (1=Sunday, 7=Saturday) } void loop() { rtc.refresh(); Serial.print("Current Date & Time: "); Serial.print(rtc.year()); Serial.print('/'); Serial.print(rtc.month()); Serial.print('/'); Serial.print(rtc.day()); Serial.print(" ("); Serial.print(daysOfTheWeek[rtc.dayOfWeek()-1]); Serial.print(") "); Serial.print(rtc.hour()); Serial.print(':'); Serial.print(rtc.minute()); Serial.print(':'); Serial.println(rtc.second()); delay(1000); }
Files
design filesConclusion
This week, my assignment covered PCB design, milling, assembly, and programming using the ATTINY 1614 microcontroller. By integrating a proximity sensor and an RTC module, I gained a deeper understanding of how embedded systems function in real-world applications. Throughout the process, I encountered challenges, such as ensuring proper milling depth and soldering tiny surface-mount components, which required careful adjustments and troubleshooting. Working with these sensors gave me an insight into my final project
Hero shot
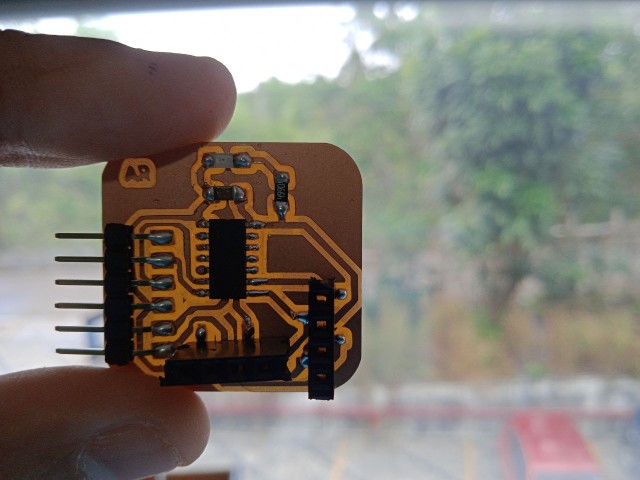