Embedded Programming
To do:
Group assignment: demonstrate and compare the toolchains and development workflows for available embedded architectures
Arduino IDE
We carried out the following steps:
- The XIAO RP2040 to Arduino
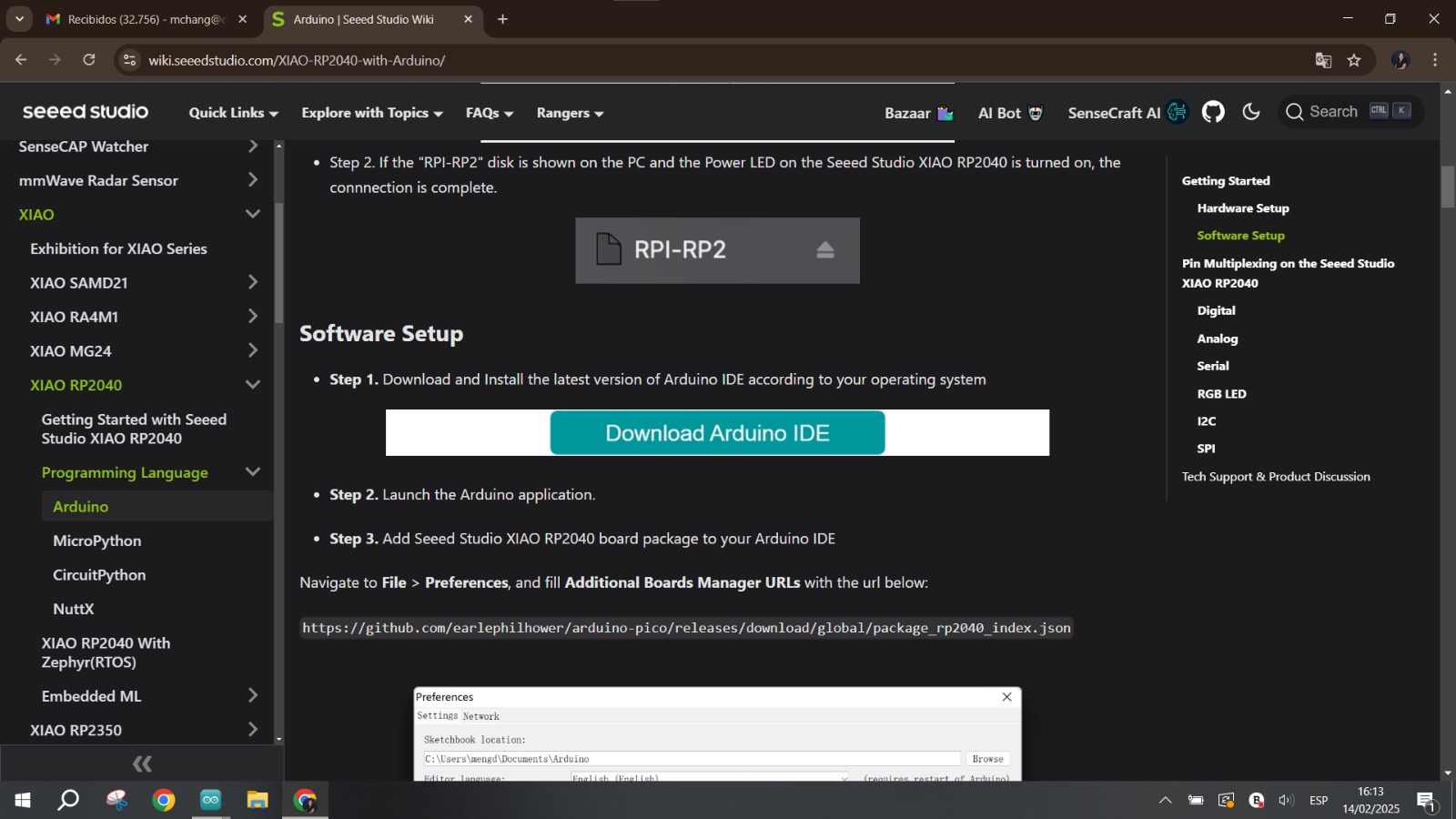
https://files.seeedstudio.com/arduino/package_seeeduino_boards_index.json
We copied the following path into the Arduino IDE:
File/Preferences/Additional Boards Manager URLs
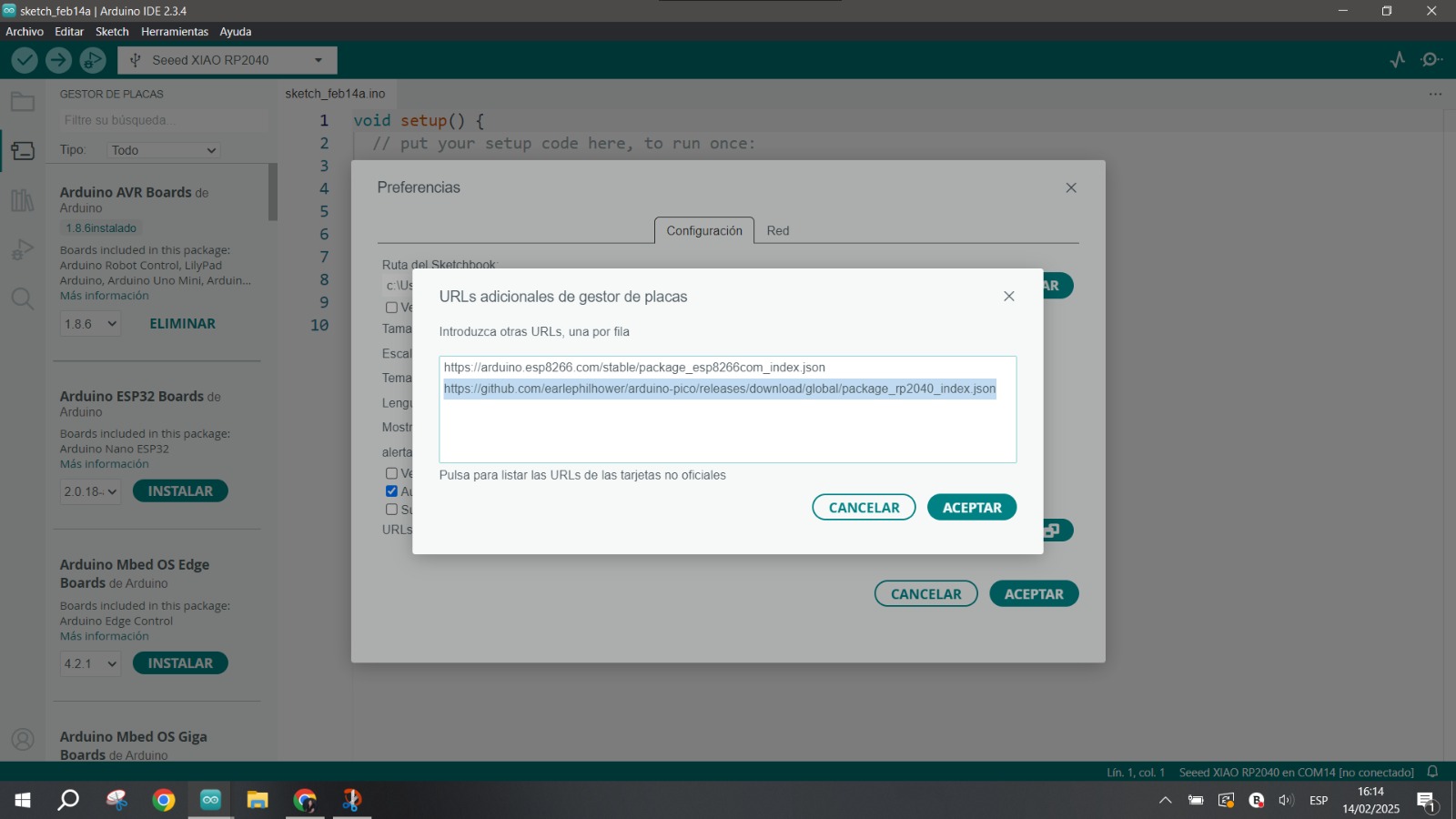
Turning an LED light on and off with a switch
- We generated the code (botton_Led.ino).
- We assembled our circuit.
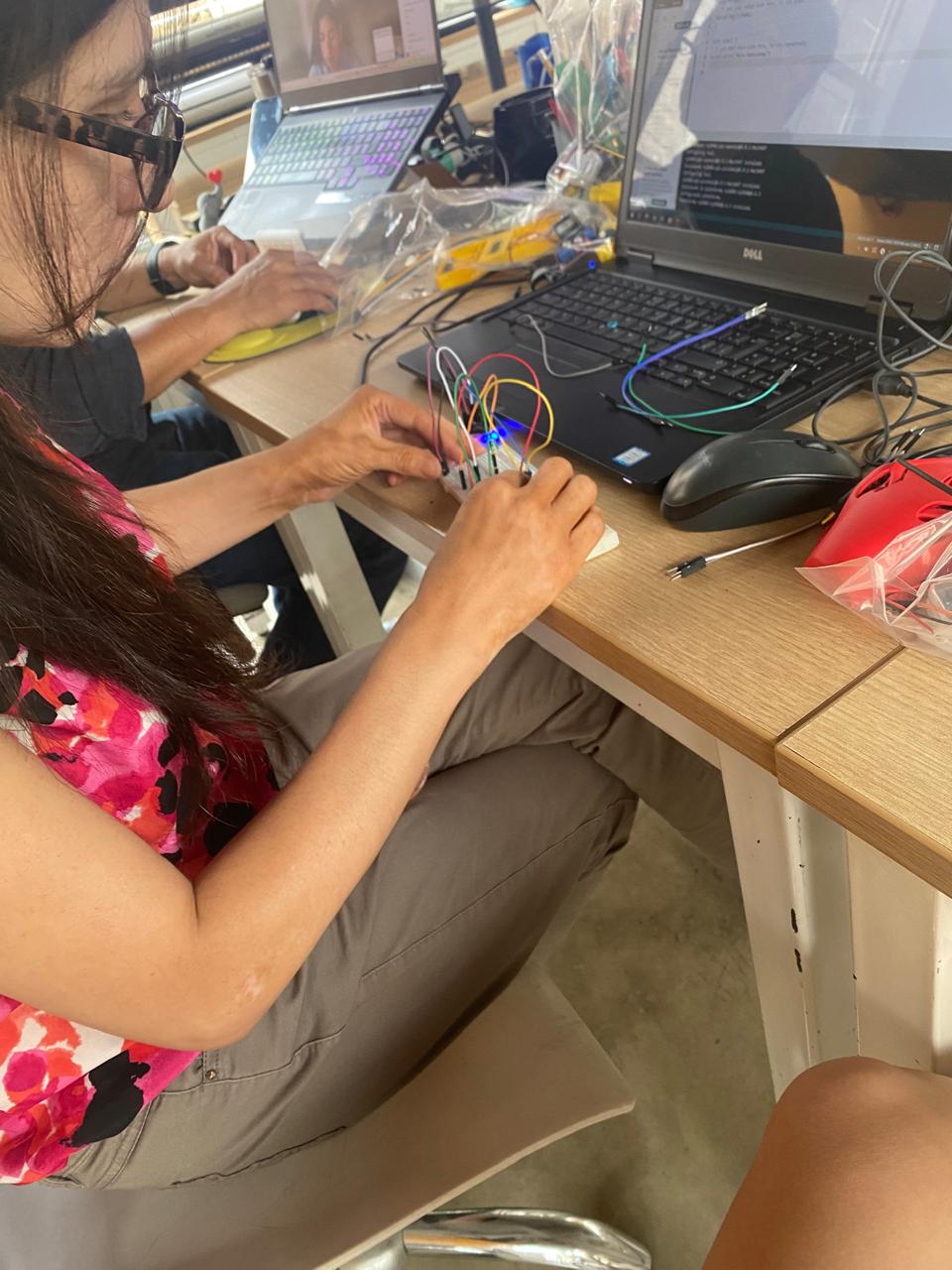
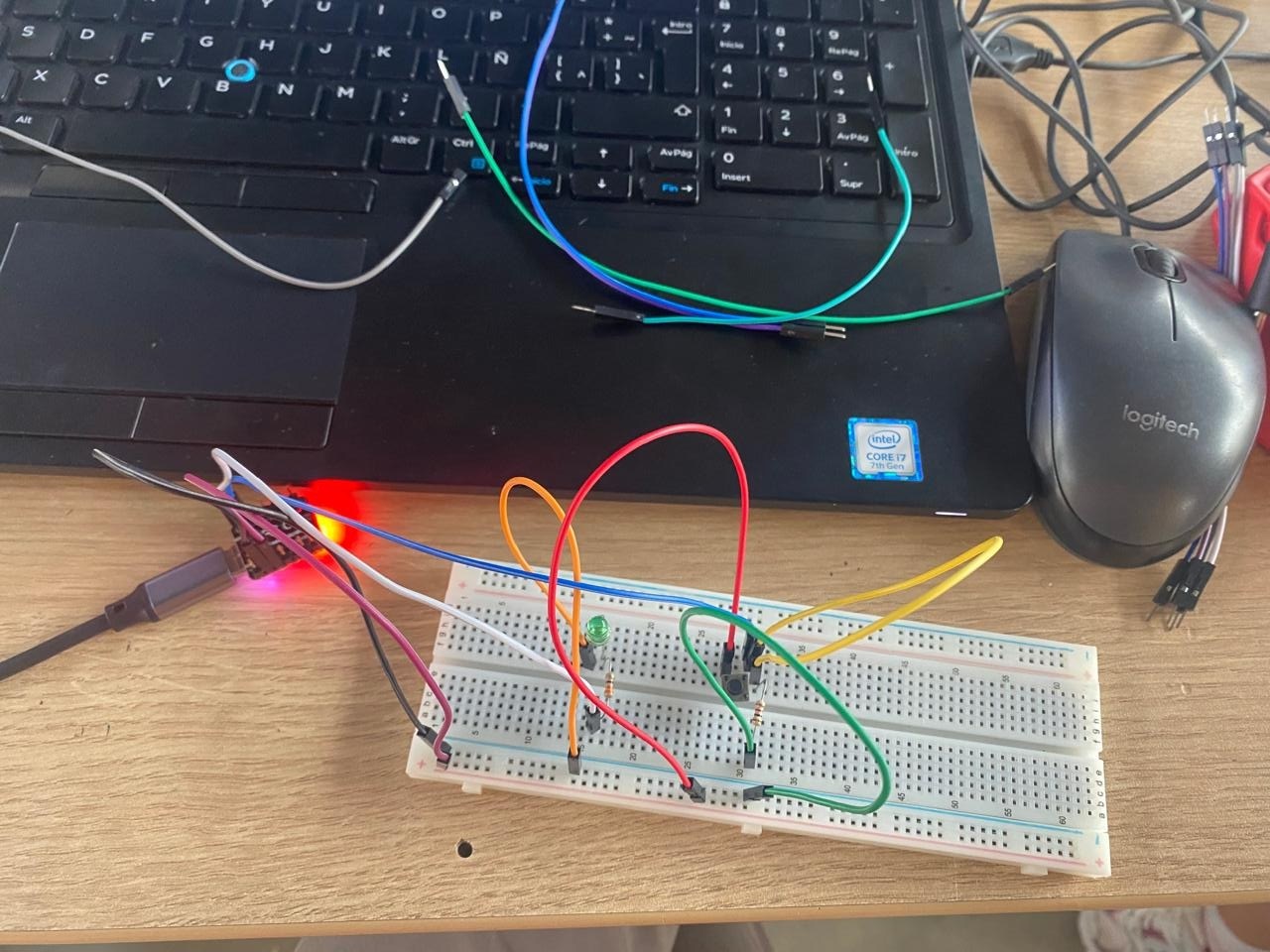
We ran the program, and the LED light turned on.
Connecting an OLED display and a light with a sensor
For this example, using the XIAO2040 microcontroller, we will use an OLED screen and an FC-51 infrared sensor. Our goal is to recreate a scene where the display changes based on the sensor's detection.
Specifically, we have recreated a scene from The Powerpuff Girls: when the sensor detects a signal, the screen will show Bubbles answering the phone (HI). When no signal is detected, the screen will display her hanging up (BYE).
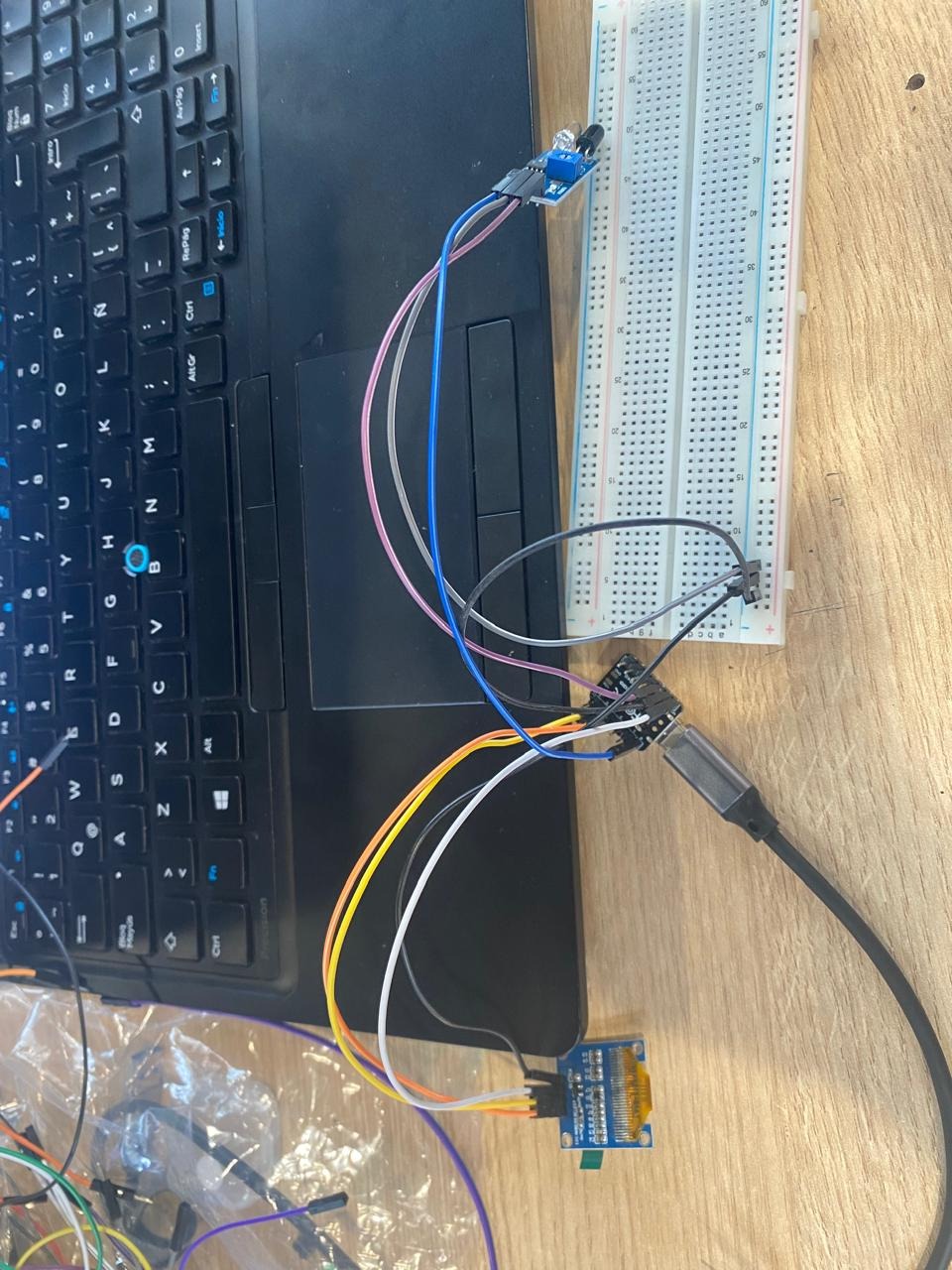
Steps to Prepare Images and Display them on the OLED.
- 1. Find suitable images
- It is recommended to use black-and-white images to simplify conversion and improve compatibility with the OLED screen.
- 2. Resize the image
- Adjust the image dimensions to fit the OLED screen resolution, ensuring that details remain visible. Paint or any image editing software can be used.
- 3. Convert to BMP format
- Save the image as a monochrome BMP file to facilitate conversion to hexadecimal code.
- 4. Generate hexadecimal code
- Use the image2cpp tool to convert the BMP image into a hexadecimal number matrix that the microcontroller can interpret. These are the settings that have been modified; the rest remain at their default values.
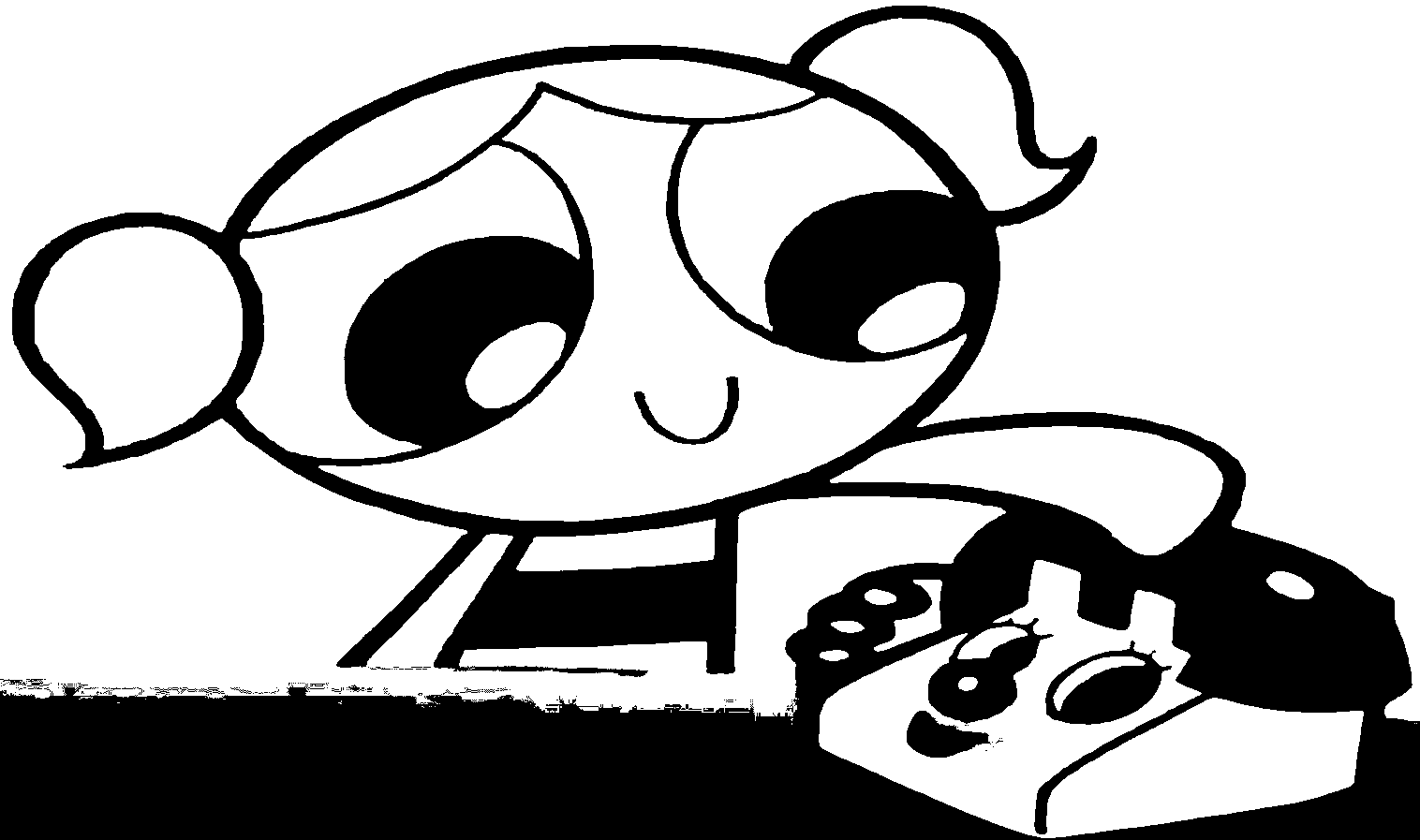
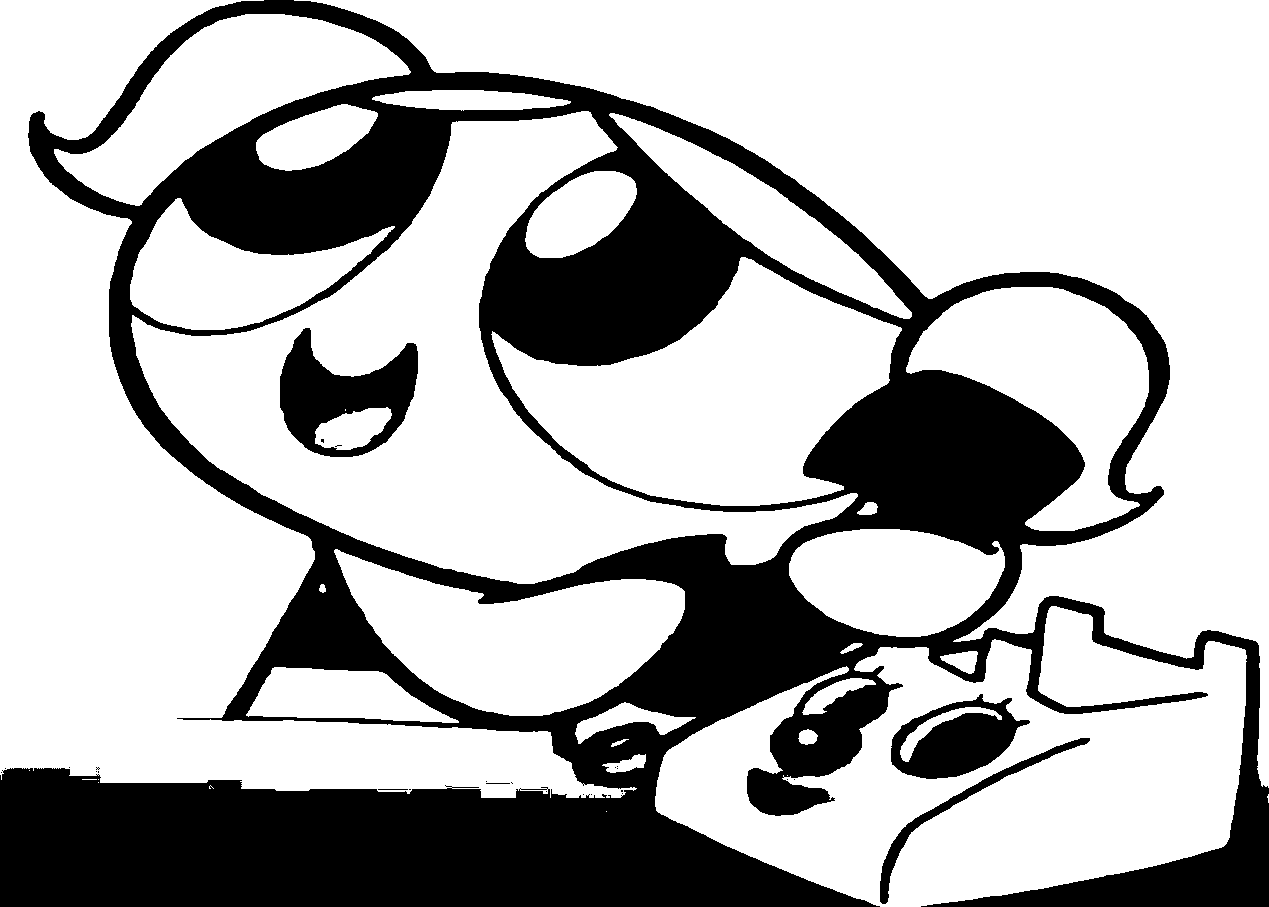
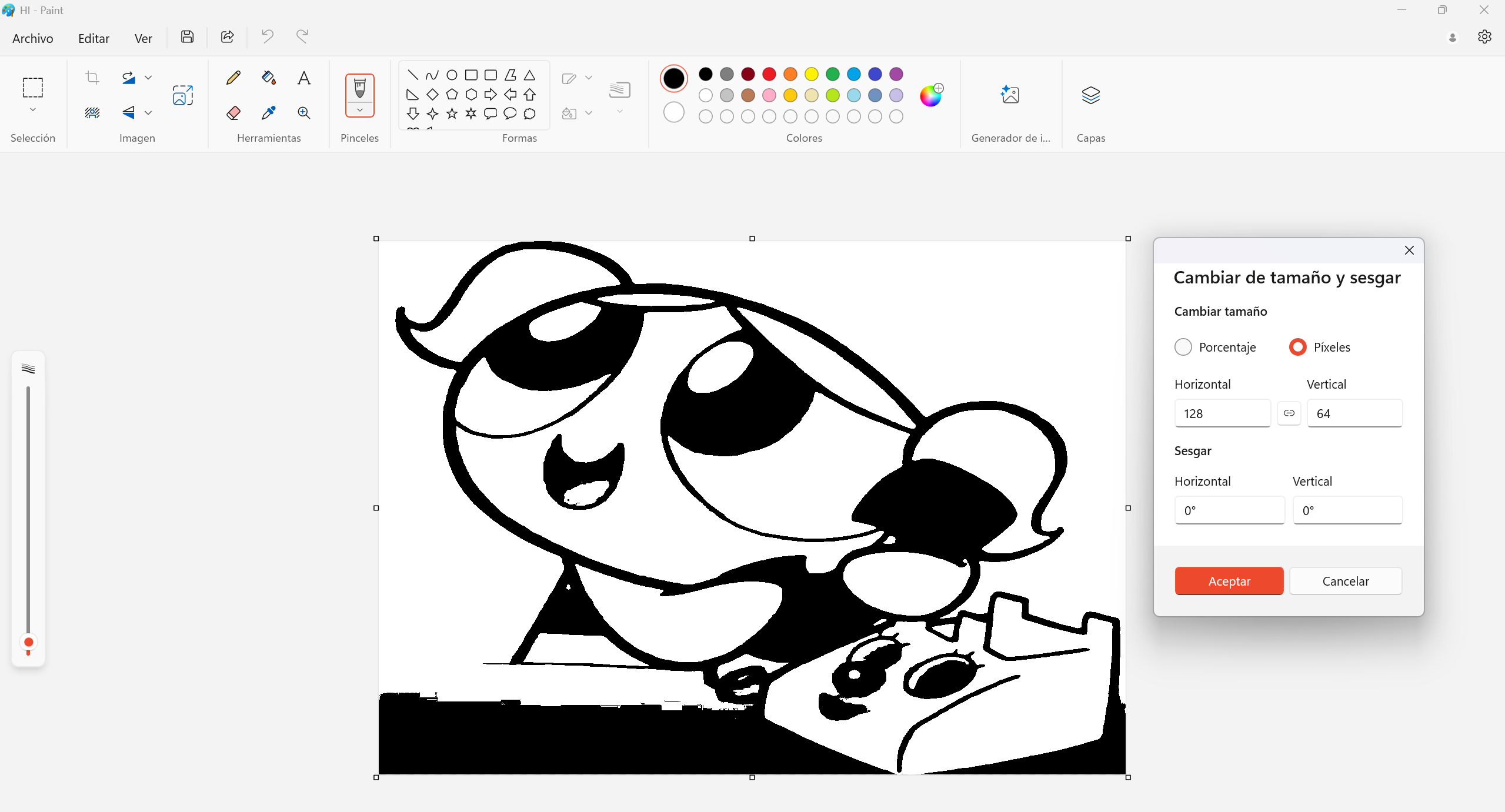
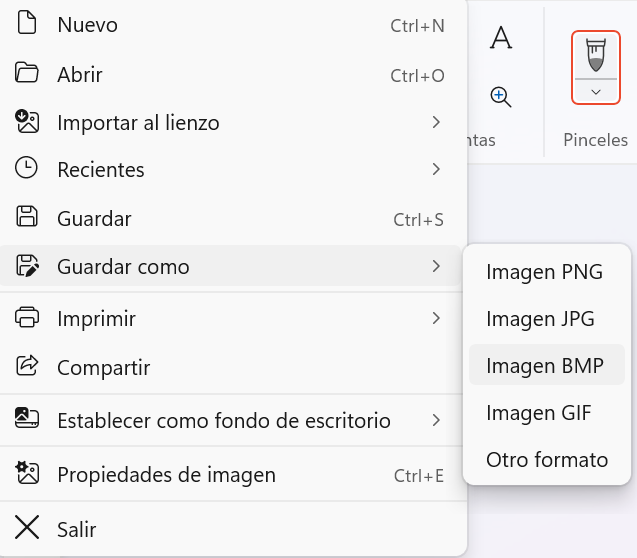
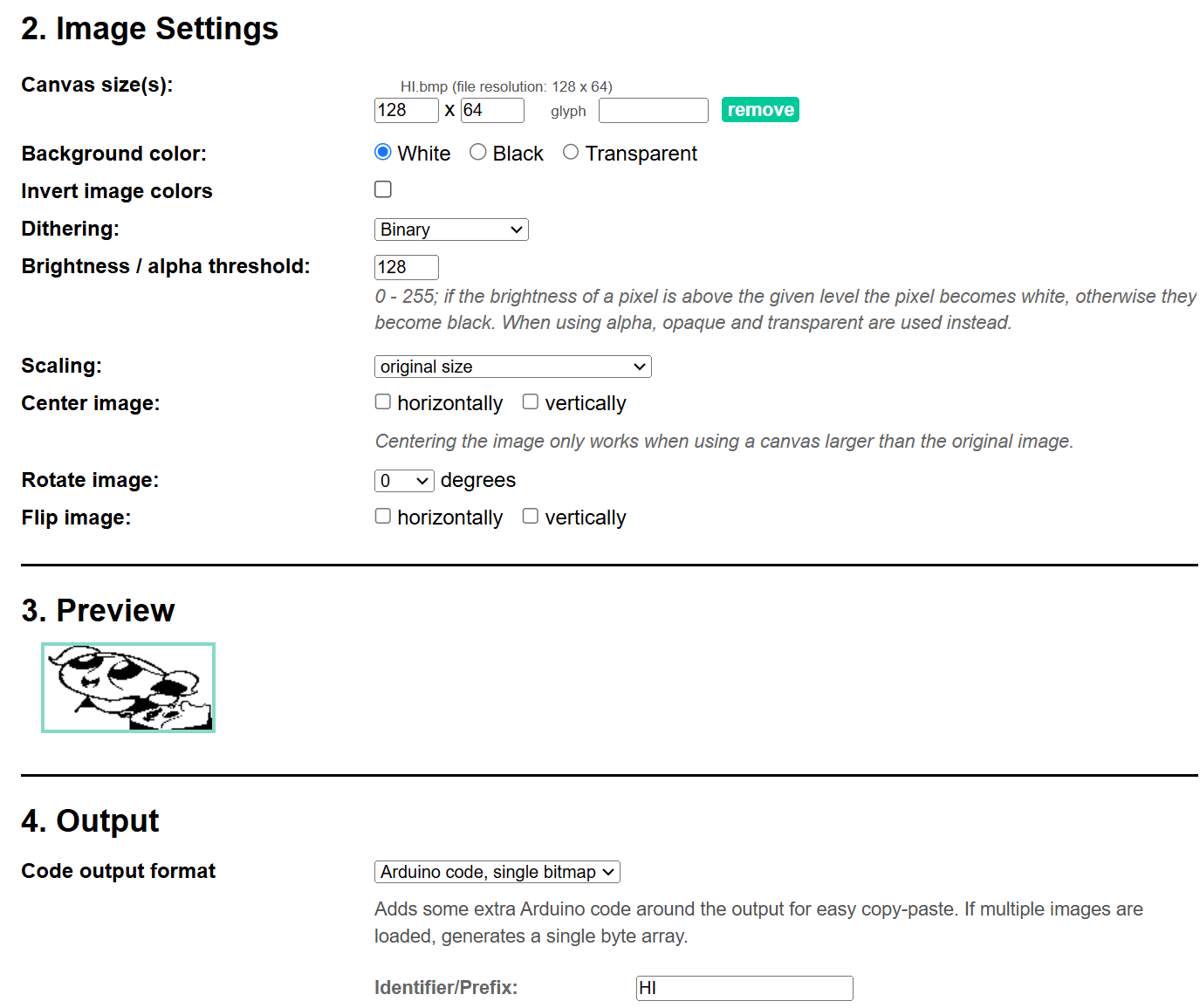
Then, the code is generated.
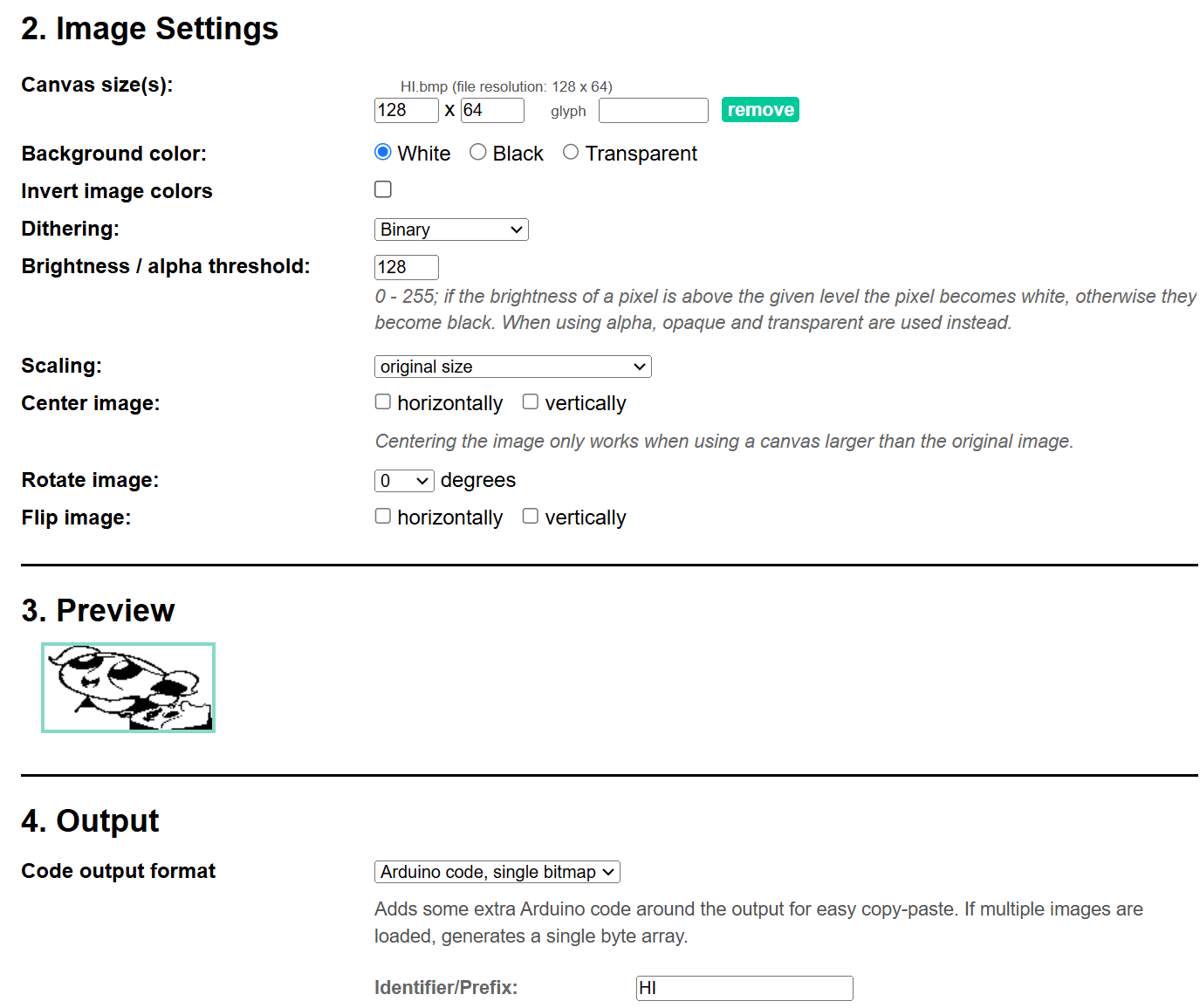
Steps to Implement in Arduino IDE
- 1. Install the necessary libraries
- Install the required libraries to use the OLED screen (e.g., Adafruit GFX, Adafruit SSD1306).
- 2. Import the generated hexadecimal code
- Copy the hexadecimal matrix generated by image2cpp into the Arduino code.
- 3. Adapt the code for the project
- Insert the image codes generated by image2cpp.
- 4. Upload the code to the microcontroller
- Connect the microcontroller and upload the code via Arduino IDE.
- 5. Test the OLED display
- Verify that the images appear correctly when the sensor is triggered.
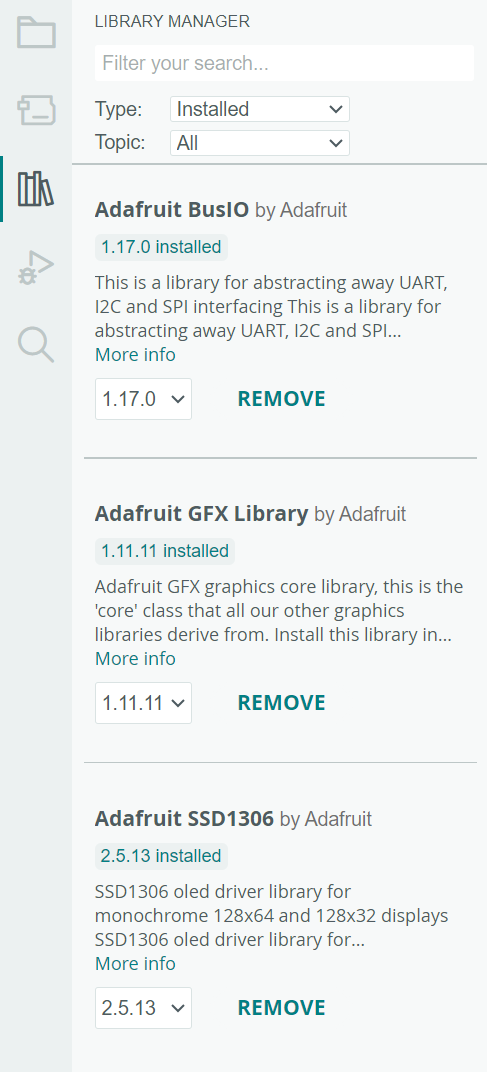

#include
#include
#include
#define SCREEN_WIDTH 128
#define SCREEN_HEIGHT 64
#define sensor D0
Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, -1);
// HI
const unsigned char HI [] PROGMEM = {
// 'HI, 128x64px
0xff, 0xff, 0xfc, 0x00, 0x3f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xe3, 0xff, 0x03, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0x8f, 0xff, 0xf8, 0x7f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xfe, 0x3f, 0xff, 0xfe, 0x3f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xfc, 0x7f, 0xff, 0xff, 0x9f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xf9, 0xff, 0xff, 0xfc, 0x00, 0x01, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xf3, 0xff, 0xff, 0x00, 0xff, 0xe0, 0x07, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xe3, 0xff, 0xf0, 0x30, 0xff, 0xff, 0x00, 0x3f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xe7, 0xc7, 0xff, 0x83, 0xfc, 0x00, 0x03, 0xf3, 0x07, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xe3, 0x9f, 0xfe, 0x1f, 0xf8, 0x07, 0xff, 0xf9, 0xf0, 0x7f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xe0, 0x3f, 0xf0, 0x3f, 0xe0, 0x07, 0xff, 0xfc, 0x7e, 0x1f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xf3, 0xff, 0xc0, 0x1f, 0x80, 0x07, 0xff, 0xe0, 0x3f, 0xc3, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xf8, 0xff, 0x80, 0x00, 0x00, 0x0f, 0xff, 0xbe, 0x0f, 0xf0, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xfc, 0x3e, 0x00, 0x00, 0x00, 0x0f, 0xfe, 0xff, 0x07, 0xfc, 0x3f, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0x00, 0x60, 0x00, 0x00, 0x1f, 0xf9, 0xff, 0x01, 0xff, 0x0f, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xf8, 0xe0, 0x00, 0x00, 0x3f, 0xf3, 0xfe, 0x00, 0x7f, 0xc7, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xf9, 0xf8, 0x00, 0x02, 0x7f, 0xe7, 0xf8, 0x00, 0x3f, 0xf1, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xf3, 0xff, 0x00, 0x3d, 0xff, 0xc7, 0xe0, 0x00, 0x07, 0xfc, 0x7f, 0xff, 0xff, 0xff, 0xff,
0xff, 0xf3, 0xff, 0xff, 0xf3, 0xff, 0xc0, 0x00, 0x00, 0x11, 0xfe, 0x3f, 0xff, 0xff, 0xff, 0xff,
0xff, 0xe7, 0xff, 0xff, 0xcf, 0xff, 0x80, 0x00, 0x00, 0x3e, 0x7f, 0x9f, 0xe0, 0x07, 0xff, 0xff,
0xff, 0xe7, 0xff, 0xff, 0xbf, 0xff, 0x80, 0x00, 0x00, 0x7f, 0x8f, 0xc7, 0x0f, 0xe0, 0xff, 0xff,
0xff, 0xe3, 0xff, 0xff, 0xff, 0xff, 0x00, 0x00, 0x00, 0xff, 0xf0, 0xe0, 0xff, 0xfe, 0x3f, 0xff,
0xff, 0xe1, 0xff, 0xff, 0xff, 0xff, 0x00, 0x00, 0x01, 0xff, 0xff, 0x01, 0xff, 0xff, 0x8f, 0xff,
0xff, 0xe7, 0xff, 0xfd, 0xff, 0xff, 0x00, 0x00, 0x07, 0xff, 0xff, 0xe7, 0xff, 0xff, 0xc7, 0xff,
0xff, 0xe3, 0xff, 0xf9, 0xff, 0xbf, 0xb0, 0x00, 0x1f, 0xff, 0xff, 0xcf, 0xff, 0xff, 0xe3, 0xff,
0xff, 0xe3, 0xff, 0xf8, 0xfe, 0x3f, 0x9e, 0x01, 0xff, 0xff, 0xff, 0xcf, 0xff, 0xff, 0xf3, 0xff,
0xff, 0xf1, 0xff, 0xf0, 0x30, 0x3f, 0xdf, 0xff, 0xff, 0xff, 0xff, 0xc0, 0x7f, 0xff, 0xf3, 0xff,
0xff, 0xf1, 0xff, 0xf0, 0x00, 0x3f, 0xcf, 0xff, 0xff, 0xff, 0xff, 0x00, 0x0f, 0xff, 0xf3, 0xff,
0xff, 0xf8, 0xff, 0xf0, 0x00, 0x7f, 0xe7, 0xff, 0xff, 0xff, 0xfc, 0x00, 0x01, 0xff, 0xe7, 0xff,
0xff, 0xfc, 0x7f, 0xf8, 0x3e, 0x7f, 0xf3, 0xff, 0xff, 0xff, 0xf8, 0x00, 0x00, 0x7f, 0xef, 0xff,
0xff, 0xfe, 0x3f, 0xf8, 0xfc, 0xff, 0xfd, 0xff, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x1f, 0xcf, 0xff,
0xff, 0xff, 0x0f, 0xfe, 0x7b, 0xff, 0xfe, 0x7f, 0xff, 0xff, 0xc0, 0x00, 0x00, 0x07, 0x9f, 0xff,
0xff, 0xff, 0xc7, 0xff, 0xff, 0xff, 0xff, 0x9f, 0xff, 0xff, 0x80, 0x00, 0x00, 0x07, 0x3f, 0xff,
0xff, 0xff, 0xf1, 0xff, 0xff, 0xff, 0xff, 0xef, 0xff, 0xff, 0x80, 0x00, 0x00, 0x07, 0x7f, 0xff,
0xff, 0xff, 0xfc, 0x3f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xf0, 0x00, 0x00, 0x0f, 0x37, 0xff,
0xff, 0xff, 0xff, 0x0f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xfd, 0x00, 0x00, 0x3f, 0xc7, 0xff,
0xff, 0xff, 0xff, 0xe1, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xf0, 0x00, 0x00, 0xff, 0x1f, 0xff,
0xff, 0xff, 0xff, 0xfc, 0x3f, 0xff, 0xff, 0xff, 0xff, 0xff, 0x07, 0xf0, 0x00, 0x00, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0x03, 0xff, 0xff, 0xff, 0x80, 0x7e, 0x7f, 0xff, 0xc1, 0x0f, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0x30, 0x3f, 0xff, 0xf0, 0x00, 0x7c, 0xff, 0xff, 0xf9, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xfe, 0x1f, 0x80, 0xff, 0x80, 0x00, 0x00, 0xff, 0xff, 0xf9, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xfc, 0x1f, 0xfc, 0x00, 0x3f, 0xf8, 0x00, 0xff, 0xff, 0xf3, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xf8, 0x0f, 0xff, 0x9f, 0xff, 0xf8, 0x00, 0x3f, 0xff, 0xe3, 0x83, 0xff, 0xff,
0xff, 0xff, 0xff, 0xf0, 0x07, 0xff, 0xff, 0xff, 0xf8, 0x00, 0x0f, 0xff, 0xcf, 0x3d, 0xff, 0xff,
0xff, 0xff, 0xff, 0xf0, 0x03, 0xff, 0xff, 0xff, 0xf0, 0x00, 0x01, 0xfe, 0x37, 0x7c, 0xff, 0xdf,
0xff, 0xff, 0xff, 0xe0, 0x01, 0xff, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x0c, 0x7e, 0x1f, 0xa1,
0xff, 0xff, 0xff, 0xc0, 0x00, 0xff, 0xff, 0xff, 0x80, 0x00, 0x01, 0xfc, 0xcf, 0x7f, 0xe0, 0x7d,
0xff, 0xff, 0xff, 0x9f, 0xfe, 0x3f, 0xff, 0xff, 0x00, 0x00, 0x03, 0x7f, 0x5f, 0x7f, 0xff, 0xfd,
0xff, 0xff, 0xff, 0x3f, 0xff, 0x0f, 0xff, 0xfc, 0x00, 0x00, 0xde, 0x3f, 0xff, 0x7f, 0xff, 0xfd,
0xff, 0xff, 0xfe, 0x3f, 0xff, 0xe3, 0xff, 0xe0, 0x00, 0x07, 0xf0, 0x3f, 0xff, 0x9f, 0xff, 0xfd,
0xff, 0xff, 0xfe, 0x7f, 0xff, 0xf8, 0x00, 0x0f, 0xf0, 0x3f, 0x00, 0x7f, 0x03, 0xff, 0xff, 0xfd,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x80, 0x38, 0x31, 0xfc, 0x01, 0xec, 0x01, 0xff, 0xff, 0xf9,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x00, 0x07, 0xfc, 0x41, 0xd8, 0x03, 0xff, 0xff, 0xf9,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x81, 0x9f, 0xfc, 0x03, 0xd0, 0x0f, 0x7f, 0xff, 0xf9,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xc2, 0x1f, 0xf4, 0x0f, 0xe0, 0x79, 0xff, 0xff, 0xf9,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xbf, 0xe0, 0x7f, 0xff, 0xcf, 0xff, 0xff, 0xf8,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xbf, 0xf0, 0x0f, 0xfe, 0x7f, 0xff, 0xff, 0xf8,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x9f, 0xfc, 0xff, 0xf9, 0xff, 0xff, 0xff, 0xf0,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x83, 0xff, 0xff, 0xe7, 0xff, 0xff, 0xff, 0xf0,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x80, 0x7f, 0xff, 0xdf, 0xff, 0xff, 0xff, 0xf0,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x80, 0x0f, 0xff, 0x9f, 0xff, 0xff, 0xff, 0x00,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x80, 0x01, 0xff, 0xbf, 0xff, 0xff, 0xc0, 0x00,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x80, 0x00, 0x1f, 0xbf, 0xff, 0xe0, 0x00, 0x00,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x80, 0x00, 0x01, 0xff, 0x80, 0x00, 0x00, 0x00
};
// BYE
const unsigned char BYE [] PROGMEM = {
// 'BYE, 128x64px
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x0f, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xf0, 0x40, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xc7, 0xfe, 0x3f, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xf0, 0x03, 0xff, 0x1f, 0xff, 0x9f, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xf8, 0x00, 0x00, 0x06, 0x7f, 0xff, 0xcf, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0x03, 0xff, 0xff, 0xe0, 0x7f, 0xff, 0xef, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xf8, 0x7f, 0x3f, 0xff, 0xff, 0x0f, 0xff, 0xe7, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xc3, 0xff, 0x67, 0xff, 0xff, 0xa1, 0xff, 0xe7, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0x1f, 0xfe, 0xfc, 0x7f, 0xff, 0xf8, 0x7f, 0xf7, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xfc, 0x7f, 0xf9, 0xff, 0xfc, 0xff, 0xff, 0x3f, 0xf7, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xf1, 0xff, 0xf3, 0xff, 0xfd, 0xff, 0xff, 0x8f, 0xf7, 0xdf, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xc7, 0xff, 0xce, 0xff, 0xfb, 0xff, 0xff, 0xc7, 0xf3, 0x9f, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0x8f, 0xff, 0xbf, 0x7f, 0xfb, 0xff, 0xff, 0xf3, 0xf8, 0x3f, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0x3f, 0xfe, 0xff, 0x9f, 0xf7, 0xff, 0xff, 0xf9, 0xff, 0x3f, 0xff, 0xff, 0xff,
0xff, 0xff, 0xfc, 0x7f, 0xf3, 0xff, 0xdf, 0xf7, 0xff, 0xff, 0x18, 0xfe, 0x7f, 0xff, 0xff, 0xff,
0xff, 0xff, 0xfc, 0xff, 0x9f, 0xff, 0xef, 0xf7, 0xff, 0xe0, 0x00, 0x70, 0xff, 0xff, 0xff, 0xff,
0xff, 0x80, 0xf9, 0xf9, 0xff, 0xff, 0xe7, 0xf7, 0xff, 0x80, 0x00, 0x03, 0xff, 0xff, 0xff, 0xff,
0xfc, 0x1e, 0x11, 0xbf, 0xff, 0xff, 0xf7, 0xf7, 0xfe, 0x00, 0x00, 0x3f, 0xff, 0xff, 0xff, 0xff,
0xf1, 0xff, 0xc3, 0xff, 0xff, 0x80, 0x77, 0xfb, 0xfc, 0x00, 0x00, 0x3f, 0xff, 0xff, 0xff, 0xff,
0xe3, 0xff, 0xf3, 0xff, 0xfc, 0x00, 0x07, 0xfb, 0xfc, 0x00, 0x00, 0x3f, 0xff, 0xff, 0xff, 0xff,
0xc7, 0xff, 0xf9, 0xff, 0xf0, 0x00, 0x07, 0xfd, 0xf8, 0x00, 0x00, 0x3f, 0xff, 0xff, 0xff, 0xff,
0xcf, 0xff, 0xf9, 0xff, 0xe0, 0x00, 0x03, 0xfe, 0xf8, 0x00, 0x08, 0x3f, 0xff, 0xff, 0xff, 0xff,
0x9f, 0xff, 0xfc, 0xff, 0x80, 0x00, 0x03, 0xff, 0x78, 0x00, 0x7e, 0x3f, 0xff, 0xff, 0xff, 0xff,
0x9f, 0xff, 0xfc, 0xff, 0x80, 0x00, 0x07, 0xff, 0xb8, 0x01, 0xfe, 0x3f, 0xff, 0xff, 0xff, 0xff,
0x9f, 0xff, 0xfc, 0xff, 0x00, 0x00, 0x07, 0xff, 0xc8, 0x03, 0xfc, 0x7f, 0xff, 0xff, 0xff, 0xff,
0x9f, 0xff, 0xfc, 0xfe, 0x00, 0x03, 0xef, 0xff, 0xf0, 0x07, 0xf8, 0x7f, 0xff, 0xff, 0xff, 0xff,
0x9f, 0xff, 0xfc, 0xfe, 0x00, 0x0f, 0xef, 0xff, 0xfe, 0x03, 0xce, 0x7f, 0xff, 0xff, 0xff, 0xff,
0xcf, 0xff, 0xf9, 0xfe, 0x00, 0x1f, 0xdf, 0xff, 0xff, 0xe0, 0xfc, 0xff, 0xff, 0xff, 0xff, 0xff,
0xe7, 0xff, 0xf9, 0xfe, 0x00, 0x3f, 0xbf, 0xff, 0xff, 0xff, 0xf9, 0xff, 0xff, 0xff, 0xff, 0xff,
0xf3, 0xff, 0xf3, 0xfe, 0x00, 0x3f, 0x7f, 0xff, 0x9f, 0xff, 0xf3, 0xff, 0xff, 0xff, 0xff, 0xff,
0xf9, 0xff, 0xe7, 0xfe, 0x00, 0x1e, 0xff, 0xbf, 0xdf, 0xff, 0xe7, 0xff, 0xff, 0xff, 0xff, 0xff,
0xfc, 0xff, 0xc3, 0xff, 0x00, 0x01, 0xff, 0xdf, 0xbf, 0xff, 0x8f, 0xe0, 0x0f, 0xff, 0xff, 0xff,
0xfe, 0x7f, 0x19, 0xff, 0x80, 0x07, 0xff, 0xef, 0xbf, 0xff, 0x00, 0x00, 0x00, 0x3f, 0xff, 0xff,
0xff, 0x78, 0x7c, 0xff, 0xf0, 0x3f, 0xff, 0xf7, 0x7f, 0xfc, 0x0f, 0xff, 0xff, 0x87, 0xff, 0xff,
0xfe, 0x03, 0xff, 0x1f, 0xf9, 0xff, 0xff, 0xff, 0xff, 0xf1, 0xff, 0xff, 0xff, 0xf1, 0xff, 0xff,
0xfe, 0x7f, 0xff, 0xc3, 0xff, 0xff, 0xff, 0xff, 0xff, 0xc7, 0xff, 0xff, 0xff, 0xfc, 0xff, 0xff,
0xff, 0xff, 0xff, 0xf1, 0xff, 0xff, 0xff, 0xff, 0xfe, 0x0f, 0xff, 0xff, 0xff, 0xfc, 0xff, 0xff,
0xff, 0xff, 0xff, 0xfc, 0x3f, 0xff, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x1f, 0xff, 0xfc, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0x81, 0xff, 0xff, 0xfe, 0x07, 0xff, 0xff, 0x01, 0xff, 0xfc, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xf8, 0x03, 0xfc, 0x00, 0x1f, 0xff, 0xff, 0xc0, 0x3f, 0xf9, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xc0, 0x00, 0x3f, 0x1f, 0xff, 0xff, 0x00, 0x0f, 0xf0, 0x7f, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0x9c, 0xff, 0xff, 0x3f, 0xff, 0xfe, 0x00, 0x07, 0xc0, 0x0f, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0x39, 0xff, 0xff, 0x3f, 0xff, 0xfc, 0x00, 0x00, 0x00, 0x00, 0xff,
0xff, 0xff, 0xff, 0xff, 0xfe, 0x71, 0xc0, 0x00, 0x3f, 0xfe, 0x00, 0x07, 0x80, 0x00, 0x00, 0x3f,
0xff, 0xff, 0xff, 0xff, 0xfc, 0xf0, 0x00, 0x00, 0x3f, 0xf0, 0x18, 0x07, 0x80, 0x00, 0x3c, 0x1f,
0xff, 0xff, 0xff, 0xff, 0xf1, 0xe0, 0x00, 0x00, 0x3f, 0xe3, 0x08, 0x07, 0x83, 0x80, 0x0c, 0x07,
0xff, 0xff, 0xff, 0xff, 0xe3, 0xc0, 0x00, 0x00, 0x3f, 0x80, 0x18, 0x0f, 0x83, 0xc0, 0x00, 0x03,
0xff, 0xff, 0xff, 0xff, 0xcf, 0x80, 0x00, 0x00, 0x3f, 0x98, 0x78, 0x3f, 0xe3, 0xc0, 0x00, 0x03,
0xff, 0xff, 0xff, 0xff, 0x9f, 0x00, 0x00, 0x00, 0x3e, 0x00, 0xe0, 0xf3, 0xff, 0xc0, 0x00, 0x03,
0xff, 0xff, 0xff, 0xfe, 0x3f, 0x3f, 0xff, 0xff, 0x3e, 0x00, 0x0f, 0xc3, 0xff, 0xe0, 0x00, 0x01,
0xff, 0xff, 0xff, 0xfe, 0x7e, 0x7f, 0xff, 0xff, 0x3f, 0x00, 0x78, 0x07, 0xfc, 0xe0, 0x00, 0x01,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x83, 0xf0, 0x0e, 0xe0, 0x40, 0x00, 0x01,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x8f, 0xf1, 0x0d, 0x80, 0xe0, 0x00, 0x01,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xbf, 0xf0, 0x1d, 0x01, 0xee, 0x00, 0x07,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xbf, 0xc8, 0x3e, 0x03, 0xbf, 0xff, 0xe7,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xbf, 0xc3, 0xff, 0xfc, 0xff, 0xff, 0xe0,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xbf, 0xc0, 0x7f, 0xf3, 0xff, 0xff, 0xe0,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x9f, 0xe3, 0xff, 0xcf, 0xff, 0xff, 0xc0,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x83, 0xff, 0xff, 0x3f, 0xff, 0xff, 0xc0,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x80, 0xff, 0xfc, 0xff, 0xff, 0xff, 0xc0,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x80, 0x1f, 0xf9, 0xff, 0xff, 0xff, 0x00,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x80, 0x03, 0xfb, 0xff, 0xff, 0xc0, 0x00,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x80, 0x00, 0x7b, 0xff, 0xe0, 0x00, 0x00,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x80, 0x00, 0x03, 0x80, 0x00, 0x00, 0x00
};
void setup() {
pinMode(sensor, INPUT);
if (!display.begin(SSD1306_SWITCHCAPVCC, 0x3C)) {
Serial.println(F("SSD1306 no encontrado"));
for (;;);
}
display.clearDisplay();
}
void loop() {
int sensorState = digitalRead(sensor); // Lee el estado del sensor
display.clearDisplay();
if (sensorState == HIGH) {
display.drawBitmap(0, 0, BYE, SCREEN_WIDTH, SCREEN_HEIGHT, 1);
} else {
display.drawBitmap(0, 0, HI, SCREEN_WIDTH, SCREEN_HEIGHT, 1);
}
display.display(); // Actualiza la pantalla
delay(100);
}
Thonny
We specified that the Interpreter would be MicroPython
We connected the device and verified that we had the RP1-RP2.
We located "Raspberry" at the bottom.
We began programming and tested the following code to turn an LED light on and off with varying intensity:
FILES
Students
Continuing Students
New Students
Instructors