Welcome to Week 4
Embedded programming
Hello! this time, let’s learn about Embedded programming, for this week we have the following group and individual assignments:
- Group assignment:
- Individual assignment:
o Browse through the datasheet for your microcontroller. - ✅ Done
o Compare the performance and development workflows for other architectures. - ✅ Done
o Document your work to the group work page and reflect on your individual page what you learned - ✅ Done
o Write a program for a microcontroller development board to interact (with local input &/or output devices) and communicate (with remote wired or wireless devices) - ✅ Done
GROUP ASSIGNMENT
Greetings! Today, we'll delve into embedded programming, focusing on exploring the Seeeduino XIAO RP2040 microcontroller. Let's get started!
For the FAB ACADEMY 2024, we were advised to use the SEEED STUDIO XIAO RP2040, which will assist us in executing all our task programming as well as our final project. It's a highly potent microcontroller known for its versatility, enabling us to program effectively due to its outstanding features.
Seeeduino XIAO RP2040:
The Seeed XIAO RP2040 is a powerful microcontroller known for its versatility and features. Some of its key features include:
Small Form Factor | The XIAO RP2040 comes in a compact form factor, making it suitable for projects with space constraints or wearable applications |
---|---|
USB Connectivity | The microcontroller includes USB connectivity for programming and data transfer, enabling easy integration with computers and other devices. |
Programmability | It can be programmed using the Arduino IDE, MicroPython, or CircuitPython, providing flexibility and ease of development. |
Low Power Consumption | The RP2040 chip is designed for low power consumption, making it suitable for battery-powered applications. |
Integrated Peripherals | It features integrated peripherals such as SPI, I2C, UART, PWM, and ADC, expanding its capabilities for various projects |
Affordablen | The XIAO RP2040 is relatively affordable compared to other microcontrollers with similar capabilities, making it accessible to hobbyists, students, and professionals alike |
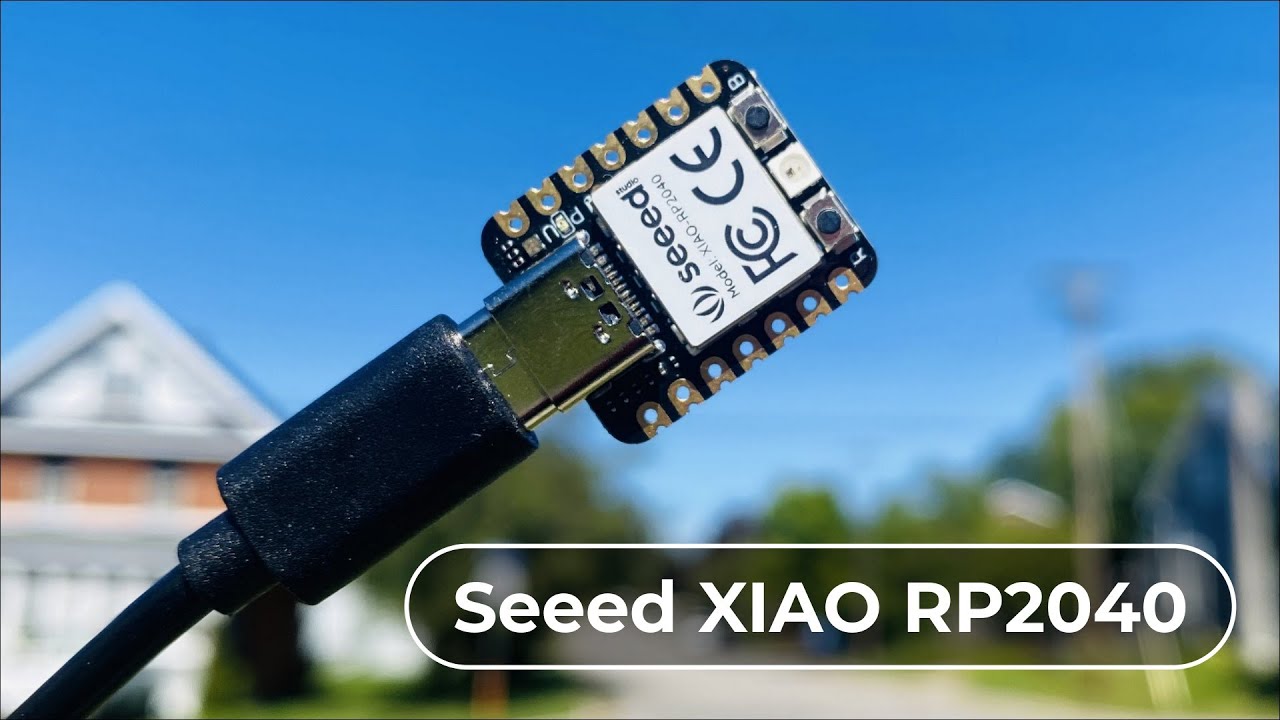
These features make the Seeed XIAO RP2040 a popular choice for a wide range of embedded projects and applications.
The simplified pinout for the Seeed Studio XIAO RP2040 microcontroller is:
- 3V3: 3.3V power output
- Earth Earth
- A0-A7: analog input pins
- D0-D23: Digital I/O Pins
- TX, RX: UART communication pins
- SCL, SDA: I2C communication pins
- SCK, MISO, MOSI, SS: SPI communication pins
- DAC0, DAC1: digital-to-analog converter output pins
- PWM0-PWM6: pulse width modulation pins
- USB: USB communication and power input
- VBUS, VBAT: battery input pins
- SWDIO, SWCLK: serial cable debugging pins.
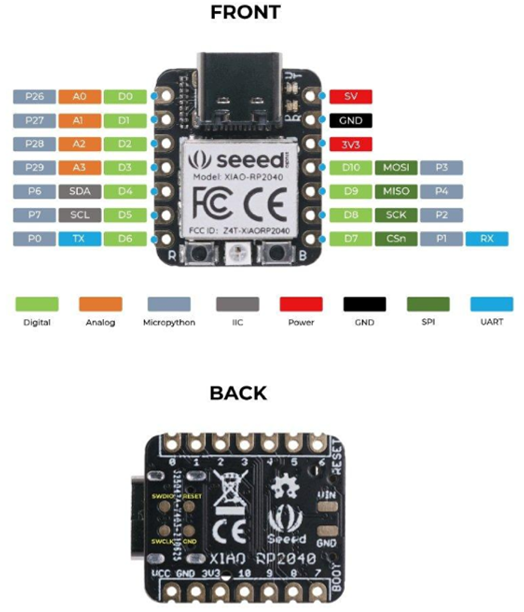
Conclusions
The Seeed Studio XIAO RP2040 is a versatile and powerful microcontroller that offers numerous features and capabilities. Its compact form factor, combined with the RP2040 microcontroller's dual-core ARM Cortex-M0+ processor, makes it suitable for a wide range of embedded projects and applications.
One of the key strengths of the XIAO RP2040 is its flexibility in programming, as it can be programmed using various development environments such as Arduino IDE, MicroPython, or CircuitPython. This flexibility makes it accessible to both beginners and experienced developers.
The XIAO RP2040 also offers a rich set of integrated peripherals, including GPIO pins, USB connectivity, analog inputs, UART, SPI, I2C, PWM, and DAC outputs, providing ample options for interfacing with sensors, actuators, and other external components.
Moreover, its low power consumption and affordability further enhance its appeal for projects requiring battery-powered operation or budget constraints.
In conclusion, the Seeed Studio XIAO RP2040 microcontroller stands out as a reliable and versatile choice for makers, hobbyists, students, and professionals looking to develop innovative embedded systems and IoT applications.
Well now let's look at other architectures and make a small comparison
AVR Microcontroller (ATmega328P, used in Arduino Uno):
Development: Uses Arduino IDE or platforms like PlatformIO. Code is written in C/C++ and compiled using AVR GCC. Programming is done via AVR programmers or the USB interface on the Arduino board. Performance Optimization: Memory and performance consumption are analyzed, code is optimized using AVR-specific techniques, and low-power techniques are implemented.
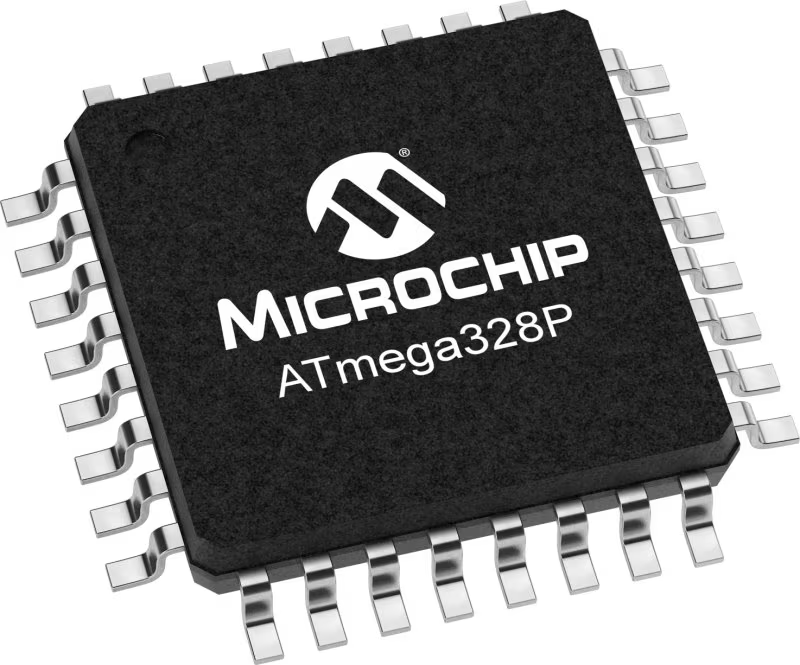
ARM Microcontroller (Xiao RP2040, based on Raspberry Pi Foundation's RP2040):
Development: Utilizes development tools like Arduino IDE or RP2040-specific environments. Code is written in C/C++ and compiled using ARM GCC or RP2040's official compiler. Performance Optimization: Bottlenecks are identified, code is optimized using ARM-specific instructions, hardware acceleration is utilized when possible, and power consumption is optimized using low-power modes.
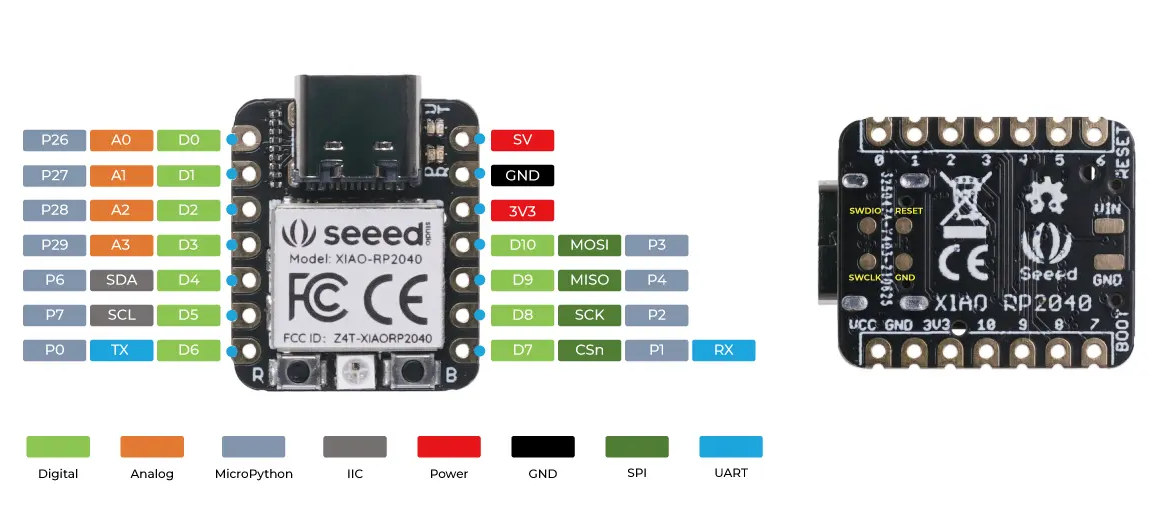
PIC Microcontroller (PIC16F877A, from Microchip Technology):
Development: Employs MPLAB X IDE with XC8 compiler. Code can be written in assembly or C. Programming is done using Microchip programmers like PICkit or MPLAB ICD. Performance Optimization: Performance is analyzed using Microchip-provided analysis tools, code is optimized using PIC-specific techniques, and low-power techniques are implemented.
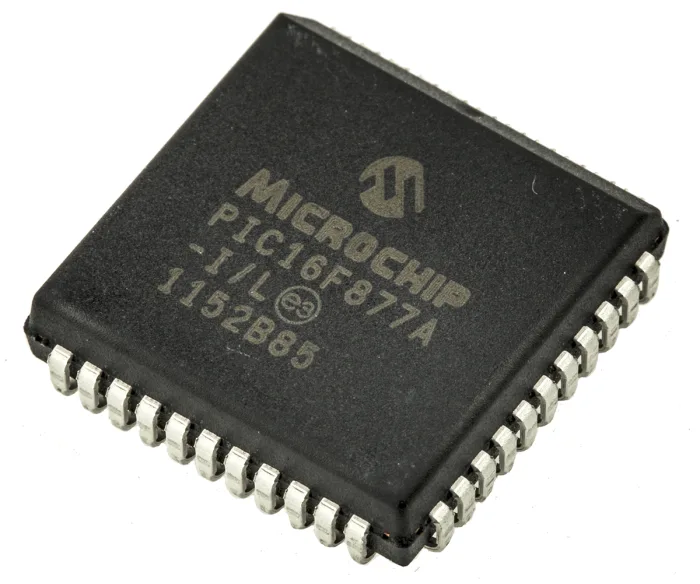
AVR Microcontroller (ATtiny85, from Microchip Technology):
Development: Uses tools like Arduino IDE or PlatformIO. Code is written in C/C++ and compiled with AVR GCC. Programming is done with compatible AVR programmers or the ISP interface on some boards. Performance Optimization: Memory and performance consumption are analyzed, code is optimized using AVR-specific techniques, and low-power techniques are implemented as per application requirements.
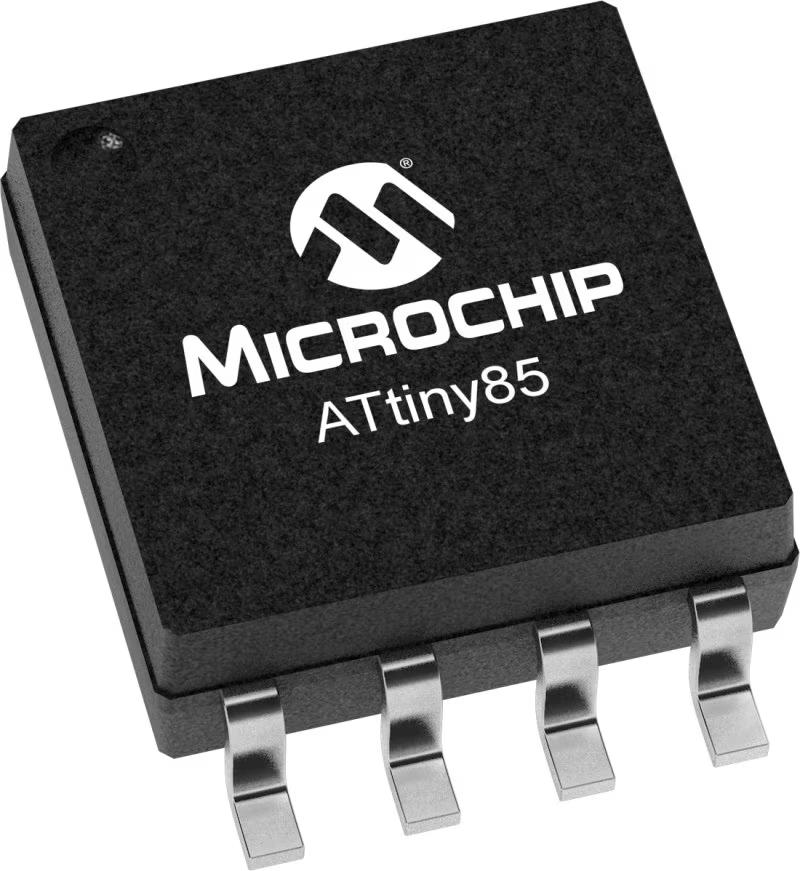
We already know about the SEEED XIAO RP2040, now let's start doing some programming exercises, let's get to work.
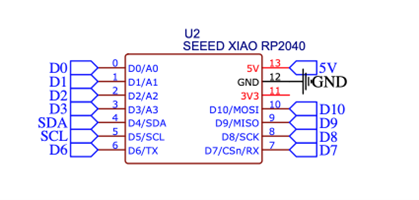
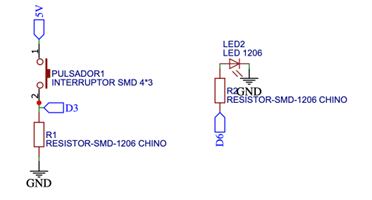
This is part of my circuit from my previously manufactured seeed xiao shield, place an LED on my shield, which is connected to pin D6. This is the one we will use for the first programming exercise.
Prior to uploading the code, we need to establish communication between our microcontroller and our PC. To do so, we should follow these steps:
1. Visit the Seeed Studio webpage.
2. Install the Seeeduino libraries for Raspberry Pi Pico/RP2040.
The information available on the Seeed Studio webpage is comprehensive and detailed. However, for clarity and convenience, I will provide a summary of the installation steps. (It's always advisable to maintain clear information by attributing authorship and the information source.)
Once the library is installed, we can proceed to program our Seeed XIAO.
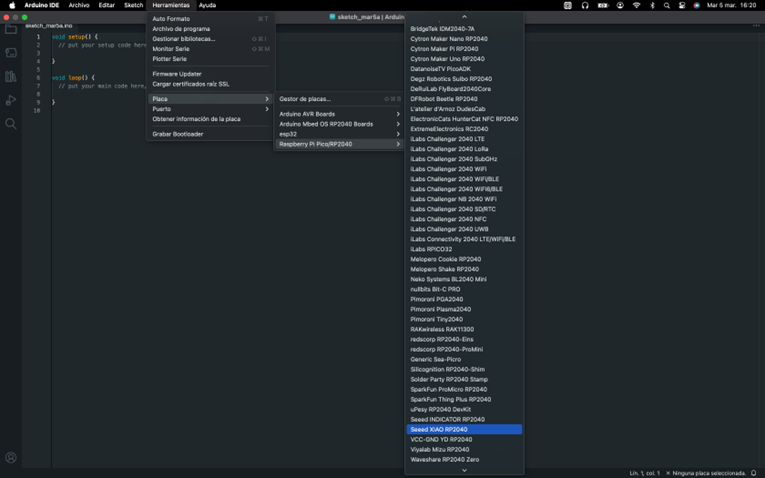
I made a code to test our Seeed XIAO:
int LED = D6;
void setup() {
pinMode(LED, OUTPUT);
}
void loop() {
digitalWrite(LED, HIGH);
delay(1000);
digitalWrite(LED, LOW);
delay(1000);
}
We can see that the LED of pin D6 is FLASHING as per the data mentioned in the program.
INDIVIDUAL ASSIGNMENT
It was great but it was simple, let's go up a little bit this time let's do some programming but getting readings for it we will use an ultrasound sensor.
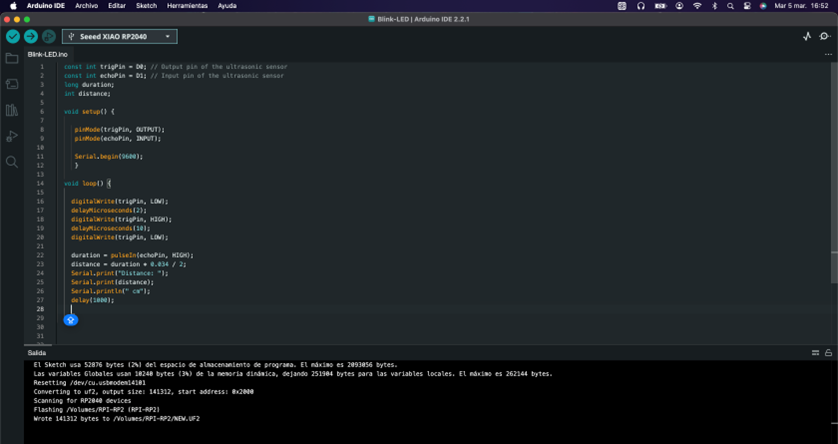
I made a code to test our Seeed XIAO:
const int trigPin = D0; // Output pin of the ultrasonic sensor
const int echoPin = D1; // Input pin of the ultrasonic sensor
long duration;
int distance;
void setup() {
pinMode(trigPin, OUTPUT);
pinMode(echoPin, INPUT);
Serial.begin(9600);
}
void loop() {
digitalWrite(trigPin, LOW);
delayMicroseconds(2);
digitalWrite(trigPin, HIGH);
delayMicroseconds(10);
digitalWrite(trigPin, LOW);
duration = pulseIn(echoPin, HIGH);
distance = duration * 0.034 / 2;
Serial.print("Distance: ");
Serial.print(distance);
Serial.println(" cm");
delay(1000);
}
It was good, we learned to obtain distance readings through this sensor, it is time to draw power through our shield and apply the output with a servomotor.
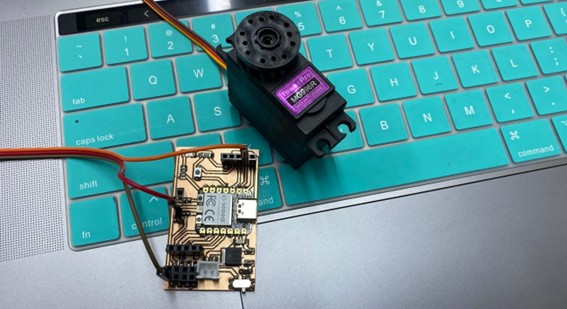
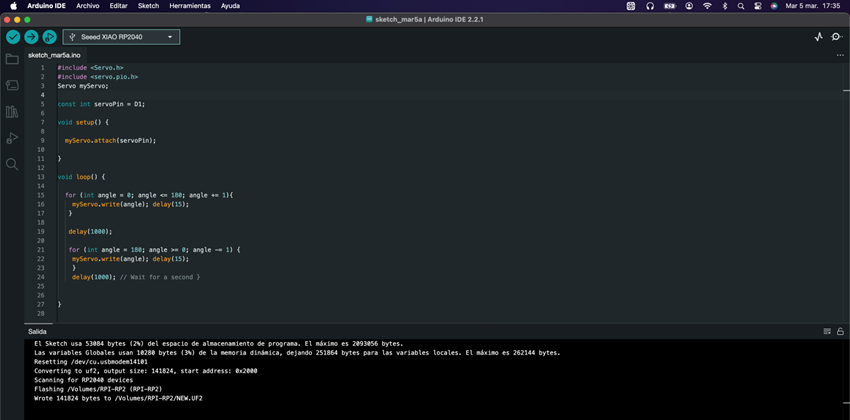
I made a code to test our Seeed XIAO:
#include
#include
Servo myServo;
const int servoPin = D1;
void setup() {
myServo.attach(servoPin);
}
void loop() {
for (int angle = 0; angle <= 180; angle += 1){
myServo.write(angle); delay(15);
}
delay(1000);
for (int angle = 180; angle >= 0; angle -= 1) {
myServo.write(angle); delay(15);
}
delay(1000); // Wait for a second }
Learning
Well we finished the assignment and we definitely did not take away important learnings:
o The comparison highlights the importance of familiarization with a variety of microcontrollers, as well as the need to learn how to optimize performance, adapt to different development environments, and select the right platform for each project.
o The Xiao RP2040 offers a combination of power, flexibility, ease of use, and affordable price that makes it an excellent choice for a wide range of embedded development projects.
That`s All my friends, see you later.
See you next week.