Introduction
This week marks the beginning of Fabacademy and setting up the foundational website for documenting my Fab Academy journey. I’ve opted to reuse a Jekyll theme I previously crafted for my portfolio, which is both familiar and well-suited to the needs of this project.
Objectives
My objectives for the website are to streamline my documentation process but also to create a resource that is both useful and enjoyable for others:
- Streamline Creation: Simplify the process of blog and documentation creation.
- Free Hosting: Leverage free hosting services to minimize costs.
- Open-Source Tools: Utilize open-source tools for development and customization.
- Resource for Others: Design a site that serves as a helpful resource to the community.
- Tag Filtering: Implement a tagging system to organize and filter posts.
- User Experience: Ensure that the site is intuitive and enjoyable to use.
- Scalability and Modularity: Design the architecture to be scalable and modular to accommodate future expansions.
Jekyll
A robust static site generator that supports markup languages, facilitating the creation of modular and scalable websites. Particularly useful is its compatibility with GitHub Pages, which offers free hosting.
Muuri
A JavaScript library that enables dynamic grid layouts, enhancing the visual appeal and interactivity of the site.
Style: Neo-Brutalism
Design Principles
Following the Neo-Brutalist Design Guide on Behance, I aimed to embody the principles of this aesthetic through bold colors and structural elements.
Color Palette
- Orange:
#E95420
- Vibrant and energetic, capturing attention.
- Purple:
#4E00E0
- Deep and thought-provoking, ideal for highlights.
- Green:
#61DB0D
- Fresh and lively, evoking creativity.
- Teal:
#0DDBA5
- Calming yet dynamic, for balance.
- Yellow:
#DBD00D
- Bright and stimulating, ensuring visibility.
Process
- Setup and Configuration:
- Installed Jekyll using the official guide.
- Set up Git by downloading tools from git and GitHub Desktop.
- Used GitHub Desktop’s UI for cloning repositories and command line for detailed operations.
- Integration and Customization:
- Transferred files from my GitHub repository to my Fab Academy repository, ensuring not to copy the
.git
folder to avoid merge conflicts.
- Created layout templates and modular components for better design flexibility.
- Styling and Features:
- Applied Neo-Brutalist styling to CSS components based on the design guide.
- Enhanced interactivity with a code block copy button and an automatic gallery separate from the Muuri gallery.
- Development Cycle:
- Continuously tested, edited, and updated the site, using mosty using the Git commands:
1
2
3
4
| git pull // update from the remote repository
git add . // after your changes add all the changes it finds you can also use * instead of .
git commit -m "description of changes" // commit your changes locally
git push // push your local changes to the remote repository this will update the online site!
|
- This iterative process ensures that improvements are methodically documented and integrated.
this is the best diagram I found to explain git, you really just need to learn about 4 commands to use it :
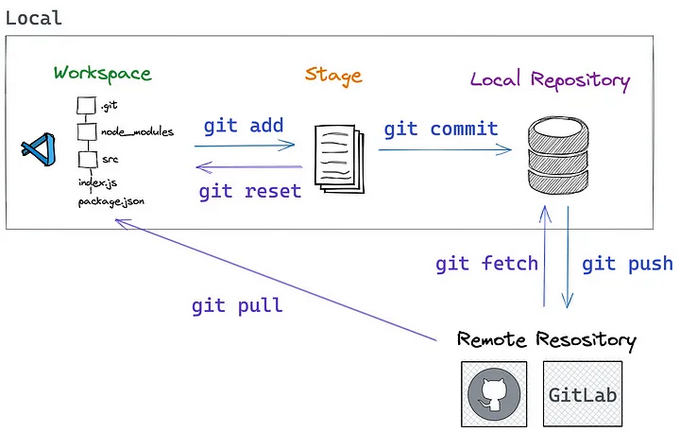
- File Structure
For my files I use the following structure:
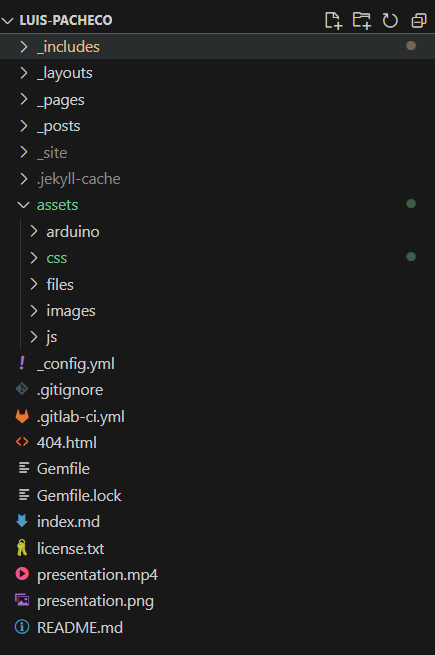
_includes/
: Directory for storing reusable components like headers and footers.
_layouts/
: Directory for storing layout templates for pages and posts.
_pages/
: Directory for storing standalone pages (e.g., about, contact).
_posts/
: Directory for storing your blog posts.
_site/
: Directory where the generated site files are stored (created by Jekyll, you don’t manually add to this).
.jekyll-cache/
: Directory for storing Jekyll’s cache files.
assets/
: Directory for storing assets like CSS, images, and JavaScript files.
arduino/
: Subdirectory for Arduino-related files.
css/
: Subdirectory for CSS files.
files/
: Subdirectory for miscellaneous files.
images/
: Subdirectory for image files.
js/
: Subdirectory for JavaScript files.
_config.yml
: Main configuration file for the Jekyll site.
.gitignore
: File specifying which files and directories to ignore in Git.
.gitlab-ci.yml
: GitLab CI configuration file.
404.html
: Custom 404 error page.
Gemfile
: File for specifying Ruby gem dependencies.
Gemfile.lock
: File for locking Ruby gem dependencies to specific versions.
index.md
: Markdown file for the homepage.
license.txt
: License file.
presentation.mp4
: MP4 video file for final presentation.
presentation.png
: PNG image file for final presentation.
README.md
: File for providing information about the template project.
- Custom Automations
To make it faster to edit this site i created a couple javascipt functions that automate generating entries the main site, code header with copy function, automatic gallery from image folder.
Generate blog post entry on main site
To generate a new UI element on the main website for each blog post it automatically looks at the _post folder for each file it looks at the thumbnail, title and caption variables to use them in the main site here is the code that does that, this is available on the main.md file, note that the following text requires the @ to be changed to % also each bracket should be double bracket, a } need to be changed to }} this is to avoid the code to run on this page:
1
2
3
4
5
6
7
8
9
10
11
12
| <div class="grid grid-1">{@ for post in site.posts @}
<a class="item project" data-category="{ post.categories | join: ' ' }" href="{ site.url }{ post.url }">
<div class="item-content">
<div class="container">
<img src="{ post.thumbnail }" alt="{ post.title }" class="image" style="width:100%">
<div class="middlev">
<div class="text vertical">{ post.caption }</div>
</div>
</div>
</div>
</a>{@ endfor @}
/div>
|
Code Header with copy button
for this i am using the following HTML on the _includes folder:
1
2
3
4
5
6
7
| <div class="code-header">
<button class="copy-code-button">
Copy code to clipboard
</button>
</div>
|
then before a code block just add the following line, note the % has been replaced with @ to avoid conflics on the site:
1
| {@ include codeHeader.html @}
|
Finally you need the copyCode.js file :
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
| const copyCodeButtons = document.querySelectorAll('.copy-code-button');
copyCodeButtons.forEach((copyCodeButton, index) => {
// This selects the specific 'rouge-code' class within the code block that the button is meant to copy.
// Assuming 'rouge-code' is nested within '.highlighter-rouge' and that is the correct structure.
const codeBlock = document.querySelectorAll('.highlighter-rouge .rouge-code')[index];
copyCodeButton.addEventListener('click', () => {
if (codeBlock) {
// Directly copy the text from 'rouge-code' class elements
const code = codeBlock.innerText;
window.navigator.clipboard.writeText(code);
// Provide visual feedback that the code has been copied
const originalText = copyCodeButton.innerText;
copyCodeButton.innerText = 'Copied!';
copyCodeButton.classList.add('copied');
// Reset the button text and remove the 'copied' class after 2 seconds
setTimeout(() => {
copyCodeButton.innerText = originalText;
copyCodeButton.classList.remove('copied');
}, 2000);
}
});
});
|
Image gallery from folder
The last automation allows you to make a folder with images and generates a gallery for you, this one is a bit more complex if you are intrested you need the image-gallery.html in your _includes folder and add the following line to automatically generate your gallery, as before the @ needs to be replaced for % to work:
1
| {@ include image-gallery.html folder="/assets/images/your-gallery-folder" @}
|
Finally in order for Jekyll to run on Gitlab you need to define gitlab pages automation, for this just copy the gitlab-ci.yml and paste it in your jekyll root folder.
File optimization and editing for web posting
In order to make a more interactive site I am using the following tools to optimize images, generate gifs ,etc.
- gifcam: Record gifs from your desktop, be careful with the softsonic site download it has bloatware! use the link here it takes you to the author site!.
- sharex: Screen capture that allows to optimize the captured files, open source.
- https://ezgif.com/: for some edits and optimizing when everything else failed.
Project Sketch
For a detailed visual representation of my final project, please visit the project diagram.