9. Output Devices
This week, it's all about connecting outputs to the pcb's we made last week.
LCD
I thought it could be fun to connect a LCD to the board that i made in week 8.
Unfortunately, I did not have any traces for the pins i needed but managed to "jump" with 2 additional wires to the pins that were needed.
LCD stands for Liquid Crystal Display.
I've asked chatgpt to explain why we need i2c:
In I2C communication, two pins are used: SDA (Serial Data Line) and SCL (Serial Clock Line). These pins facilitate bidirectional data transfer between the microcontroller (such as ATtiny) and the peripheral devices (such as the LCD module). The SDA line carries the actual data being transmitted, while the SCL line provides the clock signal for synchronizing the data transfer between devices. I2C is utilized with the ATtiny microcontroller and LCD module due to its ability to communicate using only two pins, conserving GPIO resources. Its support for multiple devices on the same bus allows for efficient control of multiple LCDs or other peripherals. I2C's standardized protocol ensures compatibility and ease of integration across different components, while library support simplifies programming and interfacing with the LCD. Overall, I2C offers a streamlined and efficient solution for connecting and controlling LCD displays in ATtiny-based projects.
Programming
I'm using arduino and the Seed Xiao board we made in week 4 to program this board.
As i'm using the ATtiny 1614, it's good to have the pinout sheet next to me. Here the SDA is connected to pinnumber 6 and SCL is connected to pinnumber 7.
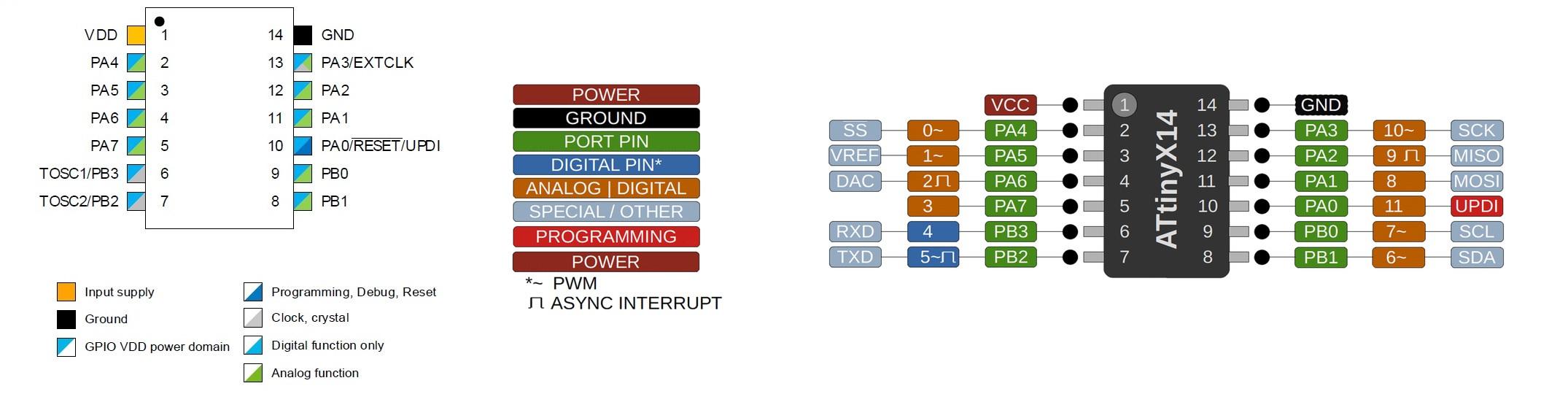
This is the code I used in arduino to have the LCD displaying jokes.
To see the next line of the joke, the button needs to be pressed.
#include "Wire.h" // Library for I2C communication #include "LiquidCrystal_I2C.h" // Library for LCD #define BUTTON_PIN 10 // Pin connected to the button LiquidCrystal_I2C lcd = LiquidCrystal_I2C(0x27, 16, 2); // Change to (0x27,20,4) for 20x4 LCD. const int numJokes = 4; // Number of jokes const char* jokes[numJokes][5] = { {"hello.", "my name is mahe", "these are my jokes", "hihi"}, {"Knock, knock.", "Who's there?", "Lettuce.", "Lettuce who?", "Lettuce in, it's cold out here!"}, {"Knock, knock.", "Who's there?", "Who.", "Who who?", "I didn't know you were an owl!"}, {"Knock, knock.", "Who's there?", "Cow says.", "Cow says who?", "No silly, cow says moo!"}, }; int currentJoke = 0; int currentLine = 0; void setup() { // Initiate the LCD: lcd.init(); lcd.backlight(); pinMode(BUTTON_PIN, INPUT); displayJokeLine(); } void loop() { // Print the current joke line on the LCD //displayJokeLine(); // Check if the button is pressed if (digitalRead(BUTTON_PIN) == LOW) { // Button is pressed, move to the next joke line displayJokeLine(); currentLine++; if (currentLine >= 5) { // Reached the end of the joke, move to the next joke currentLine = 0; currentJoke++; if (currentJoke >= numJokes) { currentJoke = 0; // Wrap around to the first joke } } delay(500); // Debounce delay } } void displayJokeLine() { lcd.clear(); // Clear the LCD before printing the new line // Set the cursor to the beginning of the first line lcd.setCursor(0, 0); // Print the current joke line lcd.print(jokes[currentJoke][currentLine]); // If the text length is greater than the number of columns on the LCD if (strlen(jokes[currentJoke][currentLine]) > 16) { // Move to the beginning of the second line lcd.setCursor(0, 1); // Print the rest of the text starting from the next character lcd.print(jokes[currentJoke][currentLine] + 16); } }