Week 14 - Interface and application programming
SAMD21 capacitive touch sensors + Processing
For this weeks assignment I made a simple square in Processing that changes color while using my SAMD21 final board, values defined by my capacitive touch sensor pads.
In order to define the codes, I asked ChatGPT and Processing forums to resolve problems along the way. The only issued that I encountered was using "String" to translate better the values so it wasnt glitchy.
Below you will find a more detailed explanation of the codes:
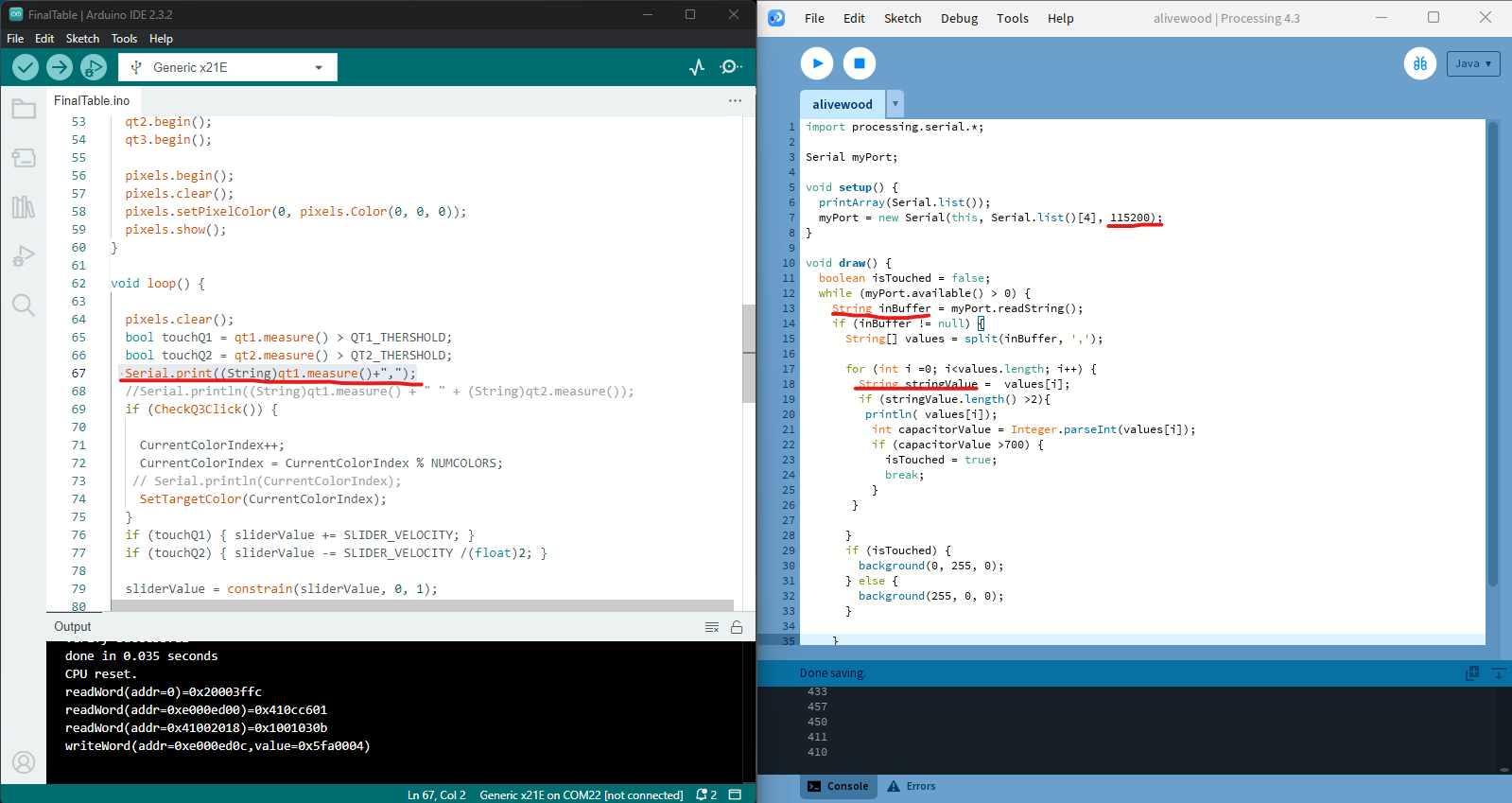
Arduino code
The left side of the image shows the Arduino code that is used to read values from my SAMD21 touch sensors (qt1) and send the measured values via serial communication. Key sections of the code:
qt1.begin();
: Initializes the touch sensors.pixels.begin();
: Initializes the LED strip.bool touchQ1 = qt1.measure() > QT1_THRESHOLD;
: Measures and checks if the first touch sensor exceeds the threshold.Serial.print((String)qt1.measure() + ",");
: Sends the measured value of the first touch sensor over serial in a comma-separated format.Processing Code
The right side of the image shows the Processing code that is used to receive and process the serial data sent from the Arduino. Key sections of the code:
import processing.serial.*;
: Imports the serial library for serial communication.Serial myPort;
: Declares a serial port object.void setup()
: Initializes the serial port for communication.
myPort = new Serial(this, Serial.list()[4], 115200);
: Opens the serial port at 115200 baud rate.void draw()
: Continuously reads and processes the incoming serial data.
String inBuffer = myPort.readString();
: Reads the incoming serial data into a string buffer.String[] values = split(inBuffer, ',');
: Splits the string buffer into an array of values based on commas.int capacitorValue = Integer.parseInt(values[i]);
: Converts the string values into integers.if (capacitorValue > 700) { isTouched = true; break; }
: Checks if the sensor value exceeds 700 and sets a flag.Final outcome
These codes demonstrate how to use Arduino and Processing together reading capacitive touch sensor values and visualizing a basic graphic on your laptop.
Group assignment
On the link below you can find the complete process of this week's group assignment. Click here
Resources
Josep Martà // Fab Lab Barcelona lead instructor
Files
Download the Arduino IDE and Processing codes here.