11. Input Devices
The assignment this week is to measure something by adding a sensor to a microcontroller board that we have designed and read the information comming from it. As a group we had to probe an input device's analog levels and digital signals.
Input Device and Arduino Coding
The input device that I have chosen for this week is a simple joystick. A joystick is able to measure directional movement accross X and Y axies using analonge potentiometers. Using this information I decided to have four lights light up in the corresponding directions that the joystick is pushed in when the connecting pins are pointing to the left. To test if this would work I first started writing the code for use in an Arduino Uno. Encountering a few problems where only some lights would turn on and some of the directions were incorrect, my lab teacher helped me troubleshoot the code. We found that two of the LEDs were actually broken, some of the variables were missnamed, and that the ranges were origonally not wide enough for the the directions to be reasonably sensed. We settled on the arduino code:
void setup() { // put your setup code here, to run once: pinMode(3, OUTPUT); pinMode(5, OUTPUT); pinMode(6, OUTPUT); pinMode(9, OUTPUT); Serial.begin(9600); } void loop() { // put your main code here, to run repeatedly: int xValue = analogRead(A1); int yValue = analogRead(A0); Serial.print("X: "); Serial.println(xValue); Serial.print("Y: "); Serial.println(yValue); //xValue <= 490 && xValue >= 530 && yValue <= 490 && yValue >= 530 is the center if(xValue <= 490 && xValue >= 0){analogWrite(3, 255);} else{analogWrite(3, 0);} if(xValue <= 1023 && xValue >= 530){analogWrite(5, 255);} else{analogWrite(5, 0);} if(yValue <= 1023 && yValue >= 530){analogWrite(6, 255);} else{analogWrite(6, 0);} if(yValue <= 490 && yValue >= 0){analogWrite(9, 255);} else{analogWrite(9, 0);} //analogWrite }Then I uploaded the corrected code into the Arduino and got this for the resting state.
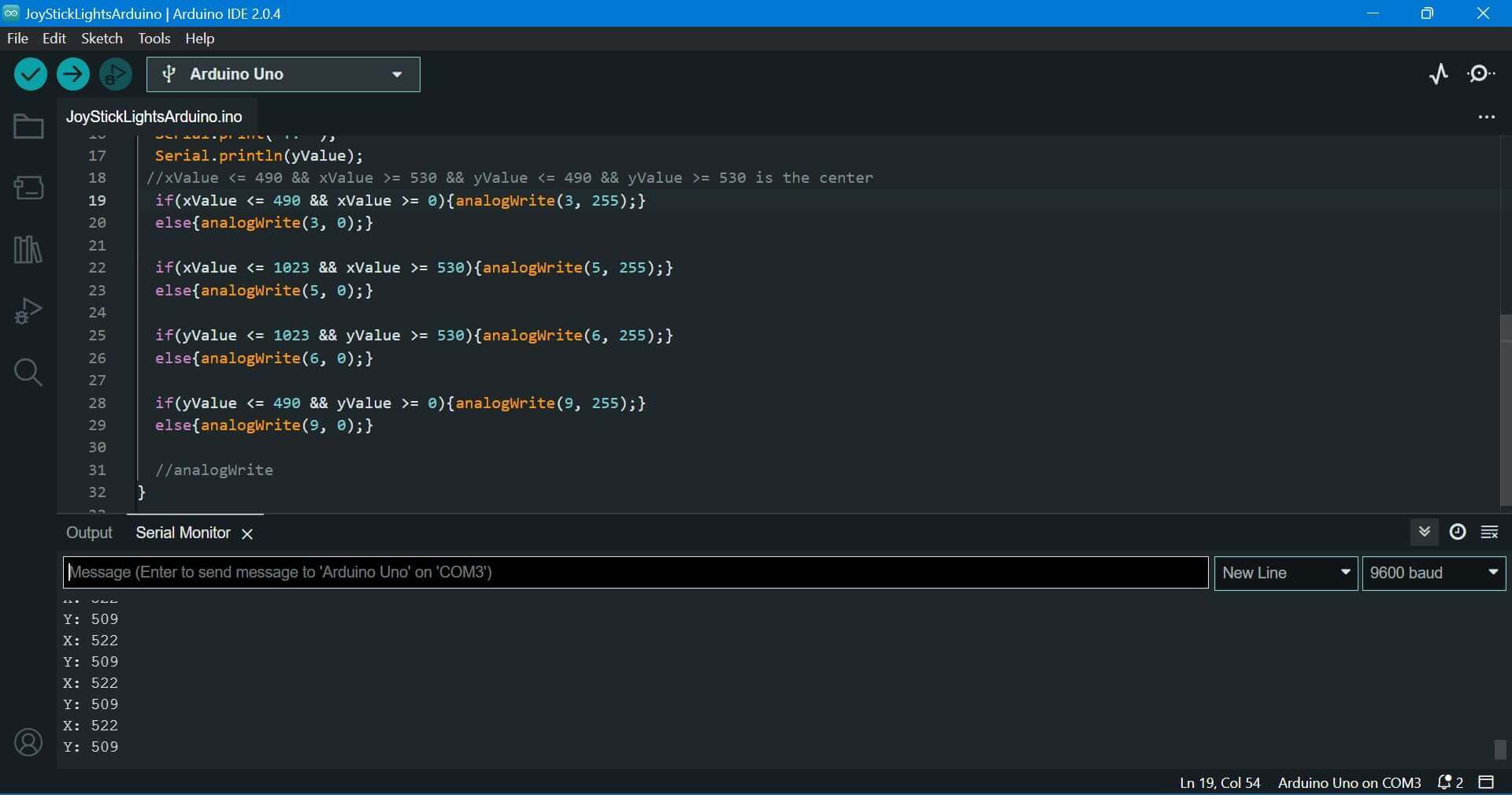
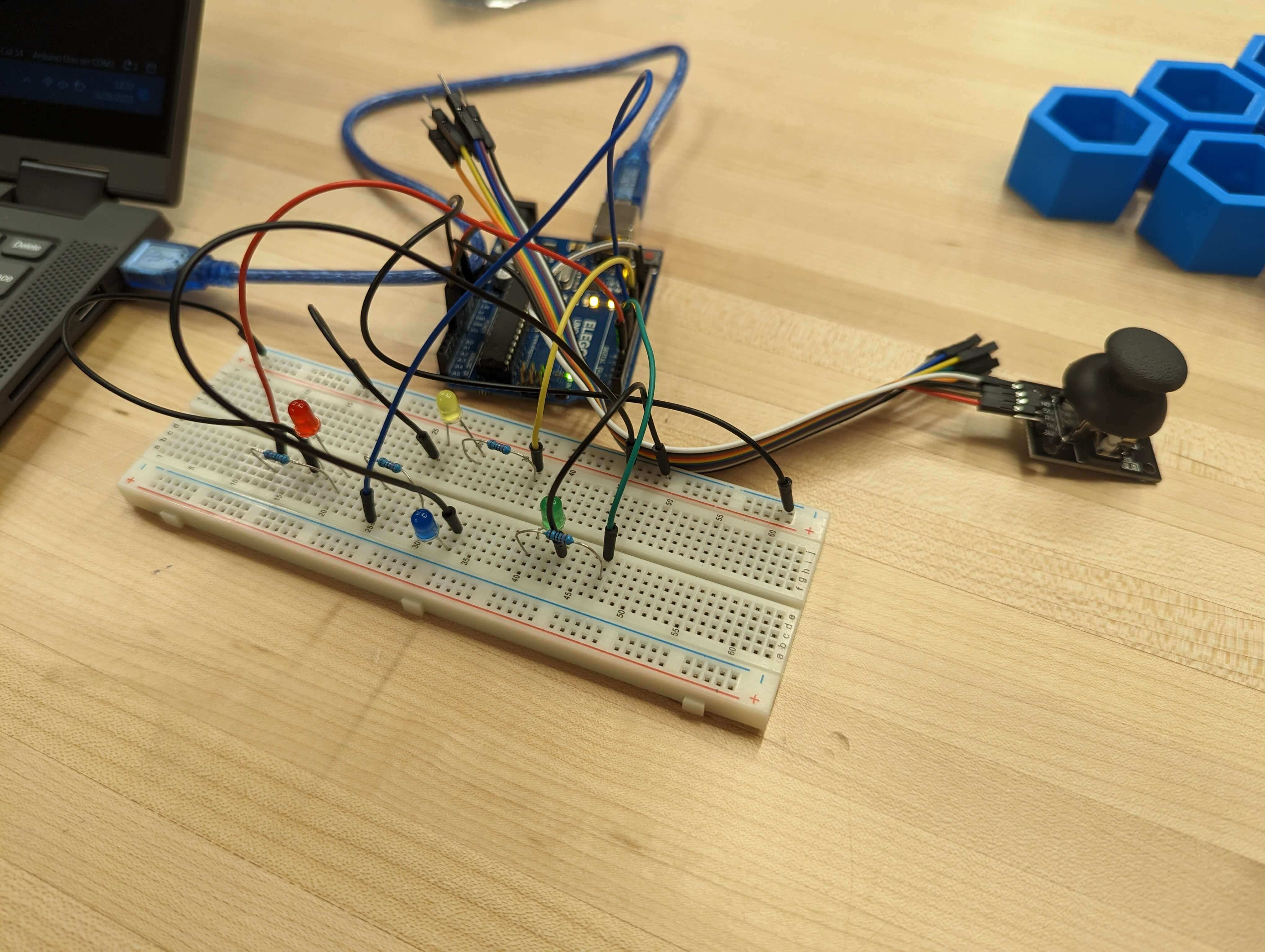
Next, I tested up, down, left, and right on the joystick and checked the lights and serial monitor to make sure everything was working in the Arduino.
Up:
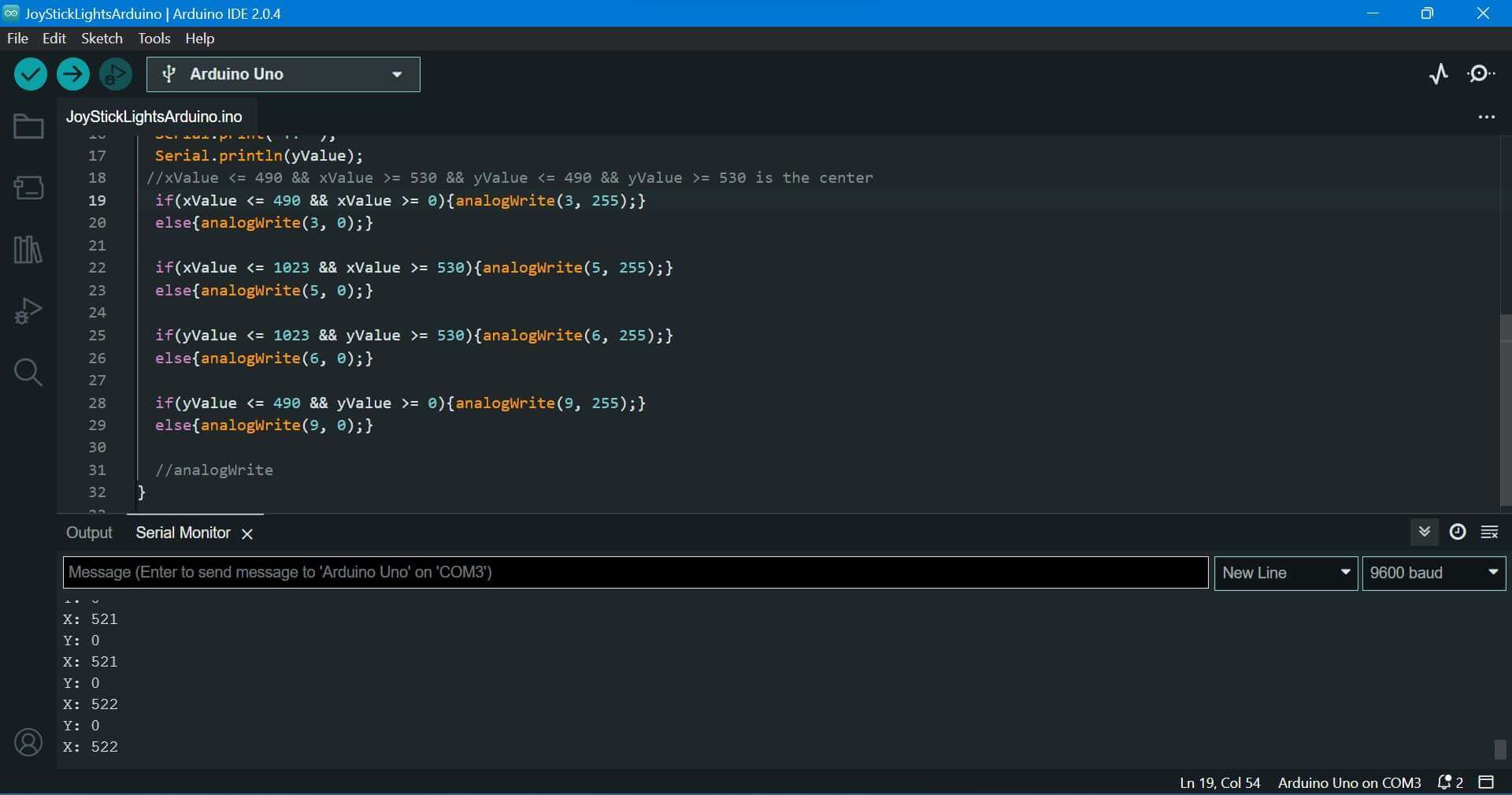
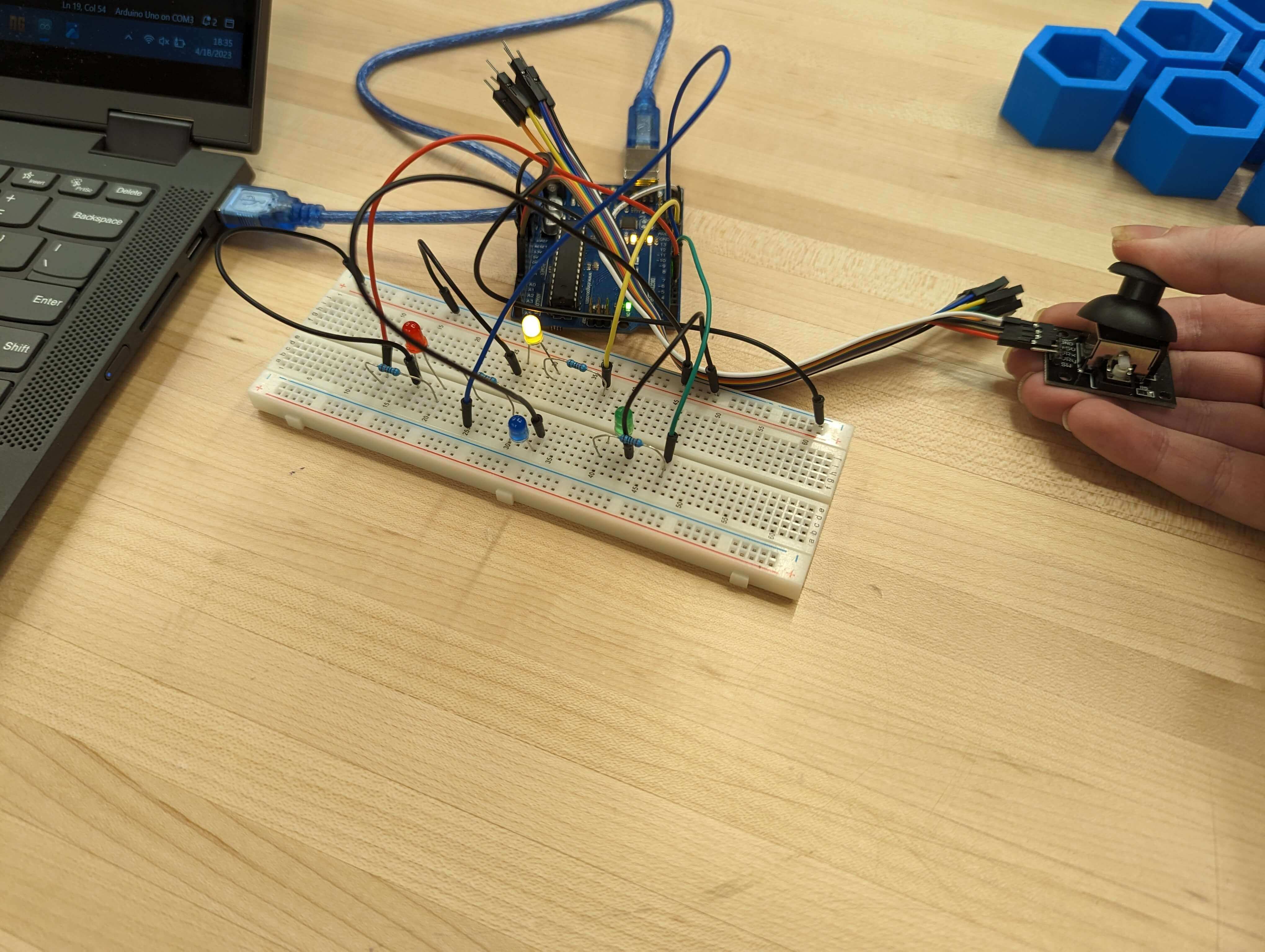
Down:
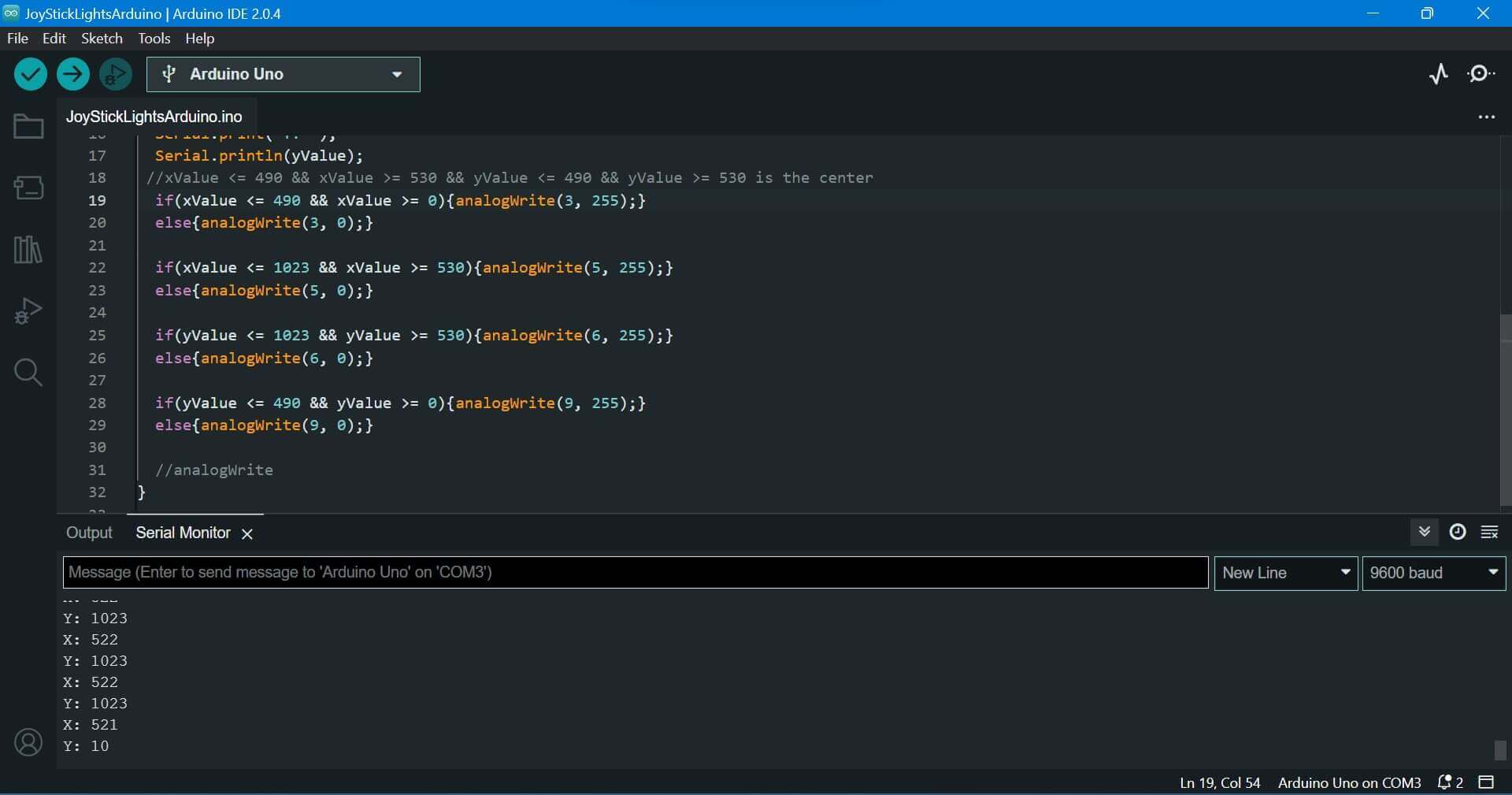
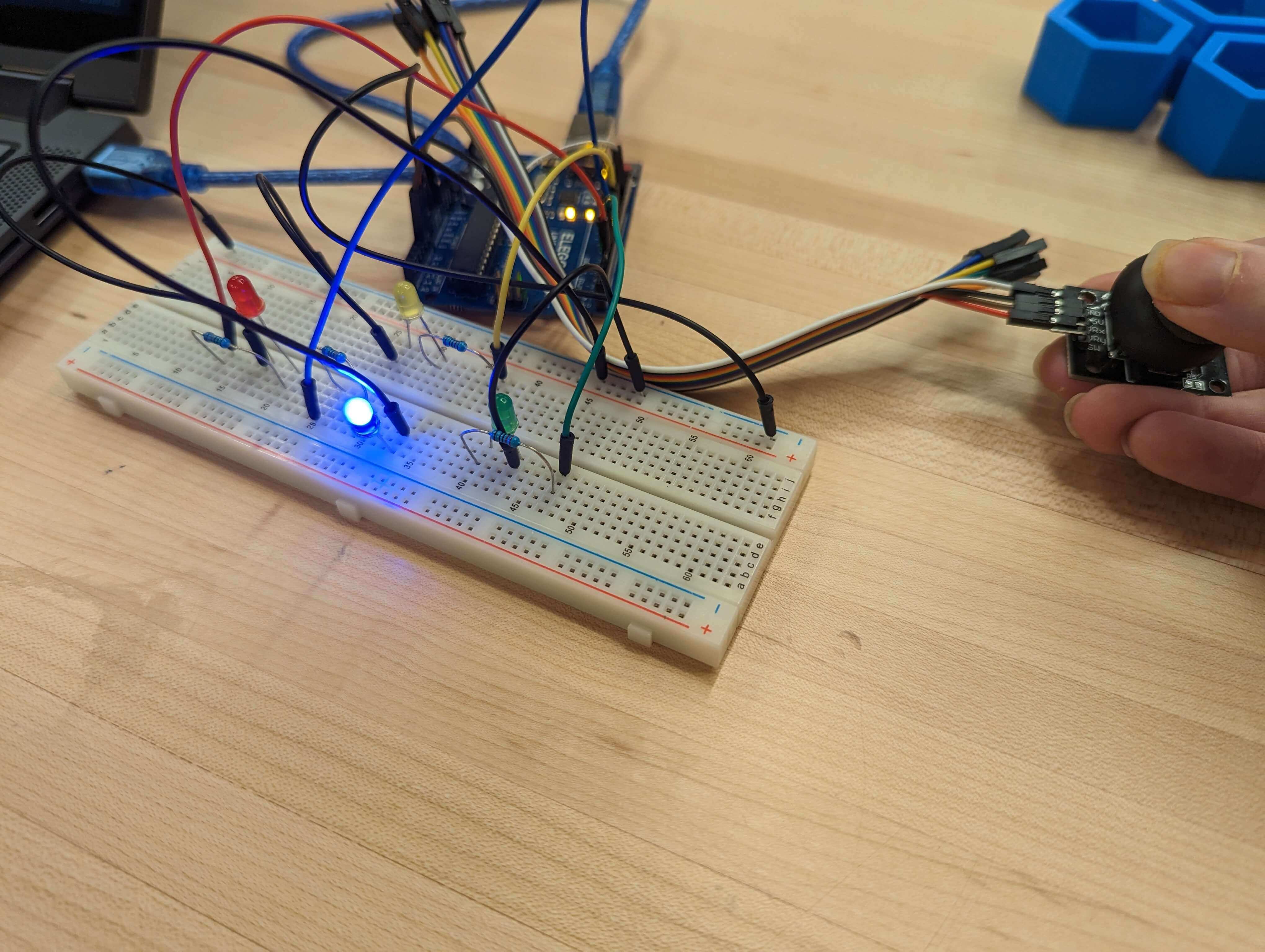
Left:
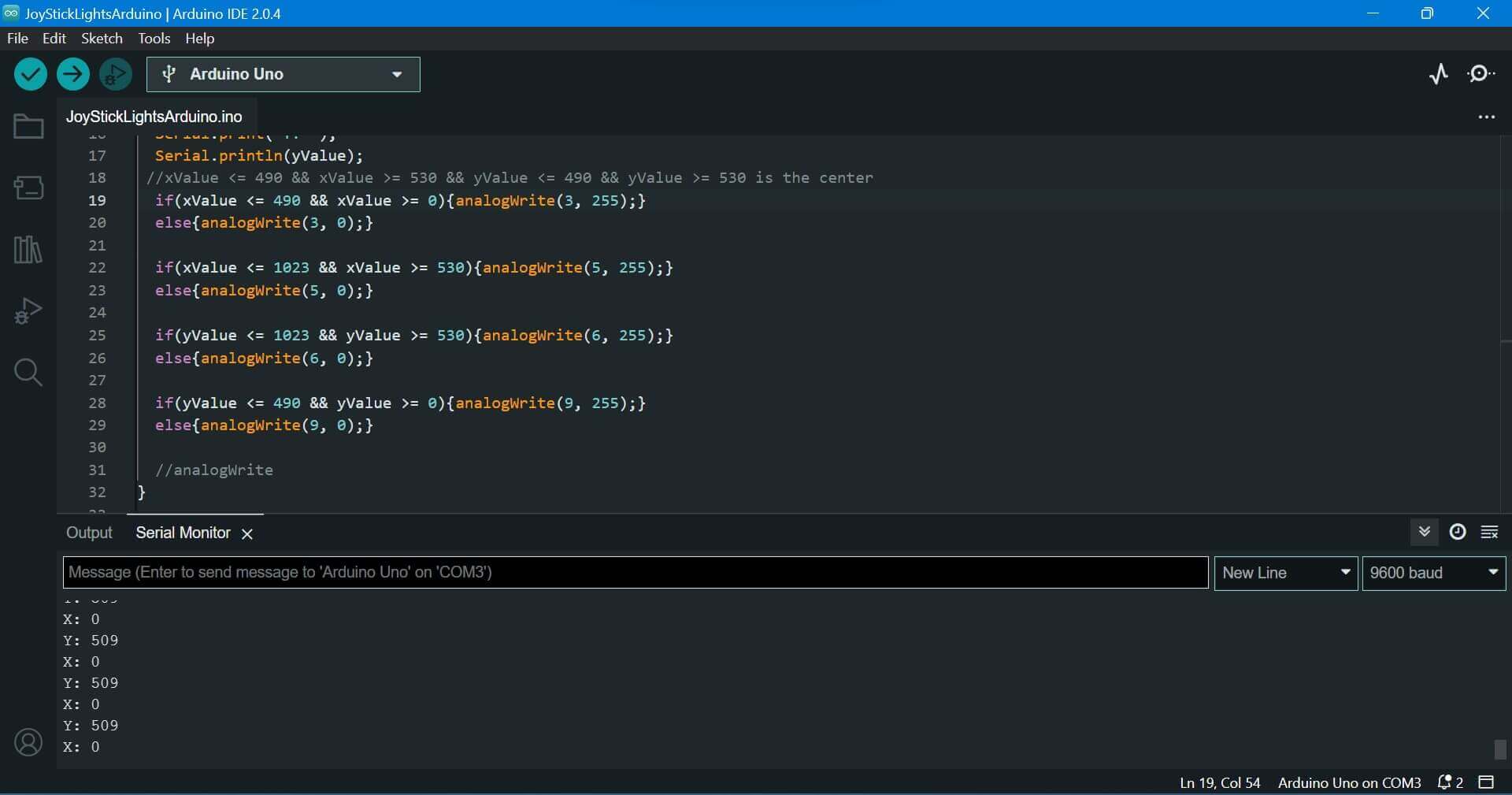
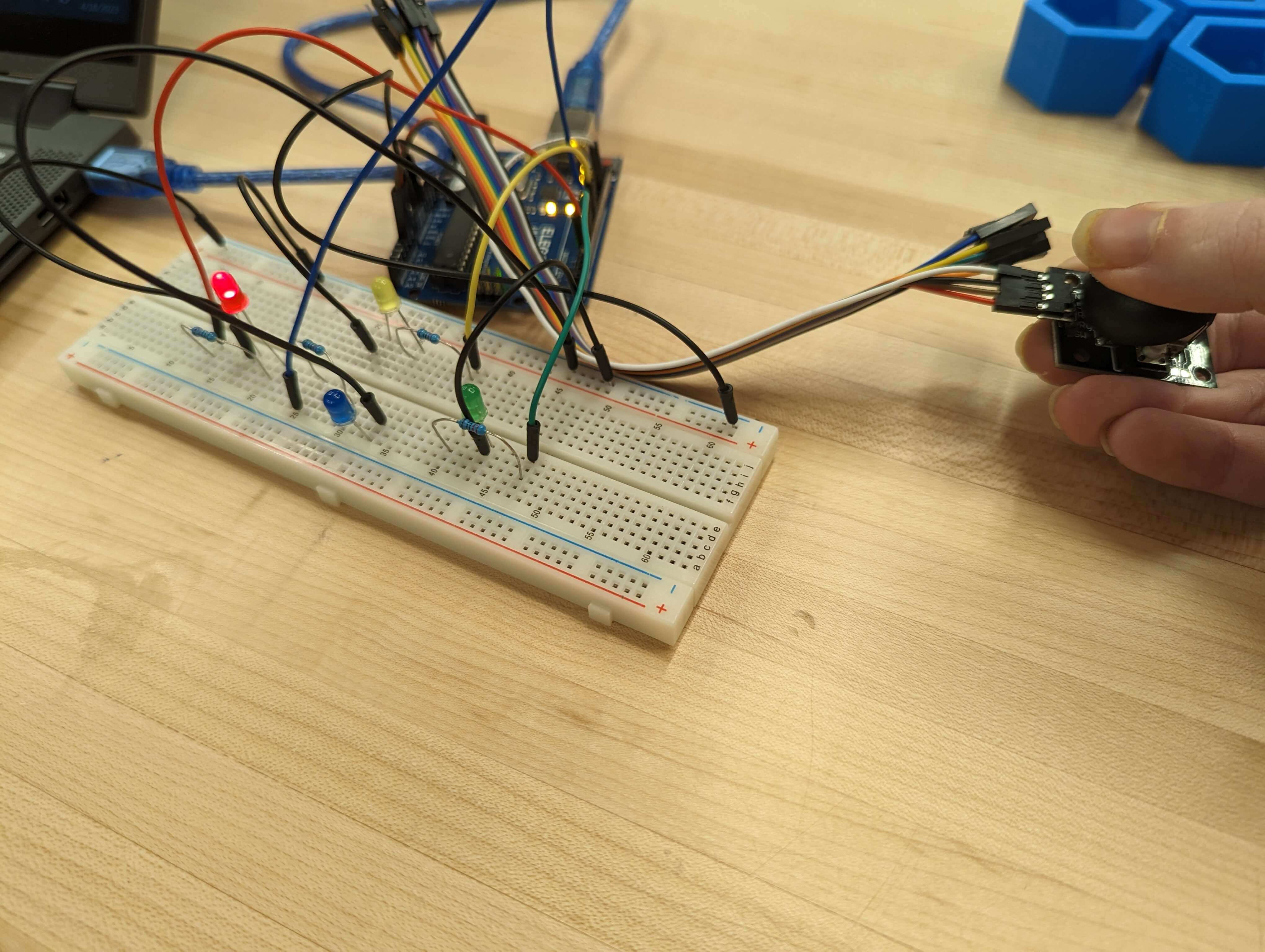
Right:
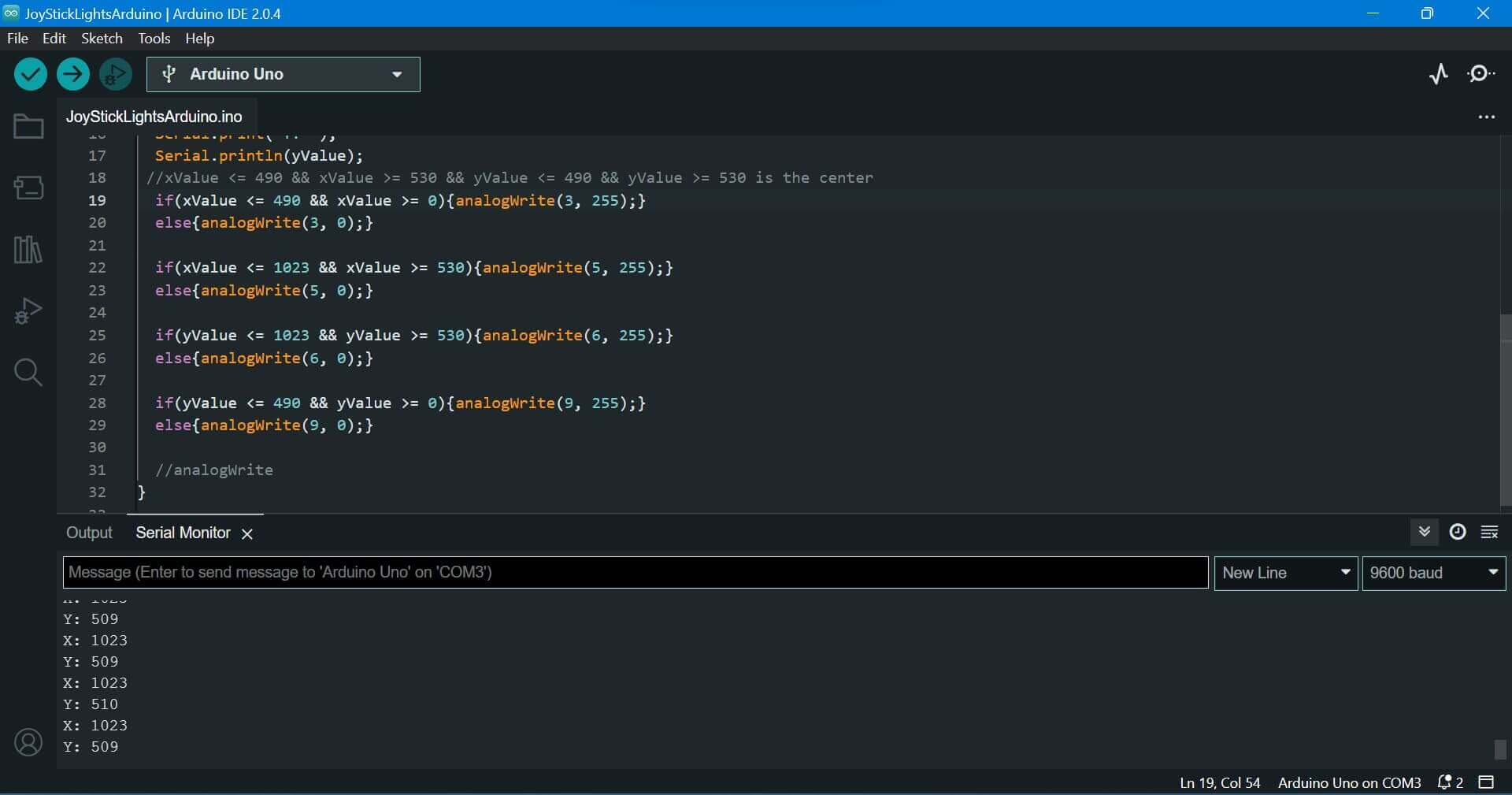
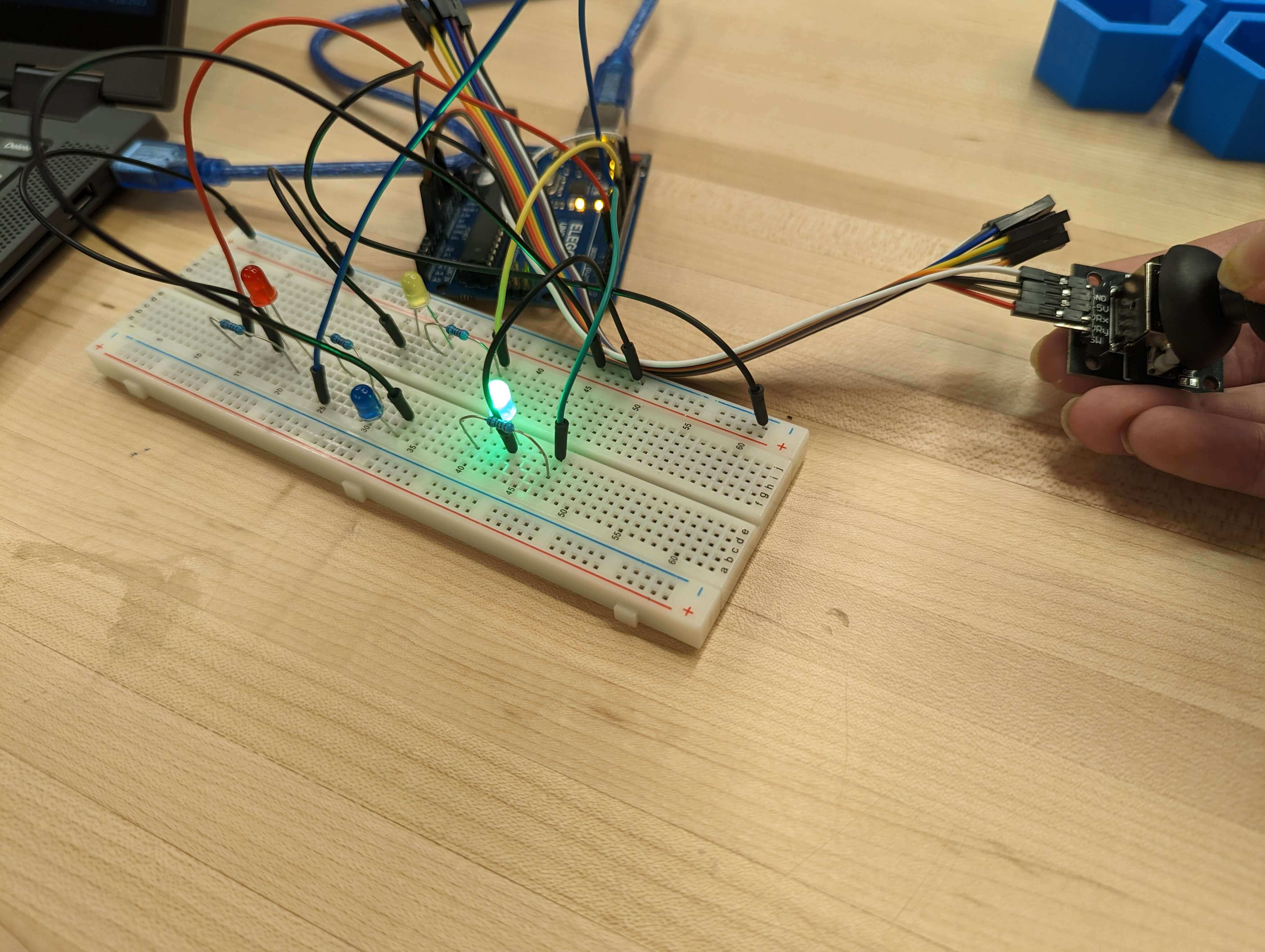
Personal Board Coding
There were a few problems that I encountered durring the week and needed help from my teacher to discover and fix the first one. The first issue was one with my board that made me think I had to either re-solder a new ESP onto it or fully redesign and mill my board. It had turned out however that the ESP was passivly running a program that didn't allow for the serial monitor to output information and was possibly even hindering my ability to upload any new code to it. We were eventually able to reset the board and upload my code to it so that it could read in information from the joystick. When looking at the serial moniter my teacher and I discovered that not only had the number range jump from the normal 0-1023 to 0-4095 but also that it had drifted almost to the max on both the X and Y axies. We do not know why the range was different other than the ESP and Arduino being made and coded differently. As for the drift I can only assume faulty code on either my or the ESP's end or that the joystick had broke between the tests done on the different boards. Sadly I couldn't make the lights light up correctly but I was able to document these results and serial monitor ouputs.
Center/Resting:
Up:
Down:
Left:
Right:
Group Work:
For the lab this week we tested how a thermoresistor and photoresitor works and how ther could be used in a circuit.
Thermoresistors when they are at room tempurature they have a resistance of 9.17k, when under a cup of cold water it has a resistance of 12.6k, when under a wrapped icecream sandwich it has a resistance of 16.0k, when held it had a resistance of 6.3k. This means that as the tempurature decreases the resistance incrases and vice versa.
Photoresistors when in the presence of ambient light have a resistance of 1.5k, when it is covered/in complete darkness the resistance is 10k, when it has a flashlight shone directly on it the resistor has a resistance of 0.15k. This means that as it gets darker the resistance increases.
The biggest difference between the two seems to be their max and min resistances as the thermoresistor is much more resistant right off the bat and may even have a greater range than the photoresistor.
Useful links
None for this week.