We took two arduino unos, and connected them together with the TX pin of one going to the RX pin of the other and vice versa.
Week 13: Networking and communications.
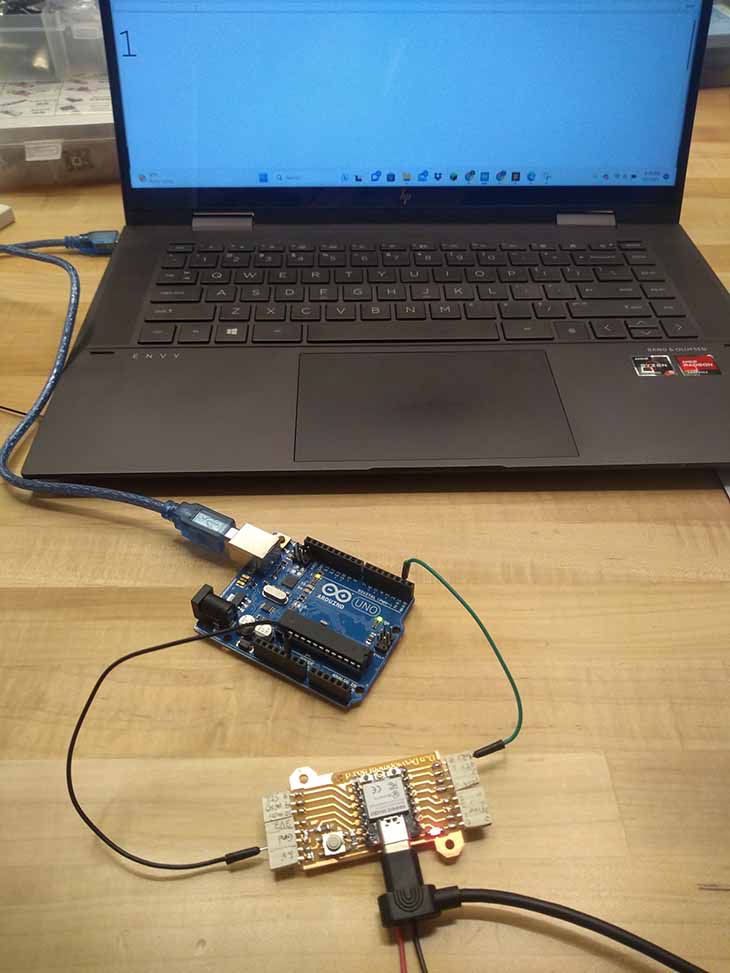
This week, I established UART communication between an arduino uno and an ESP32 C3. I also connected my ESP32 C3 to my phone via bluetooth. I worked in a group to do both of these things as well, though the individual work involved integrating both systems into one code.
We never got around to finishing the group documentation, so I’ve put together a summary of what we did here:
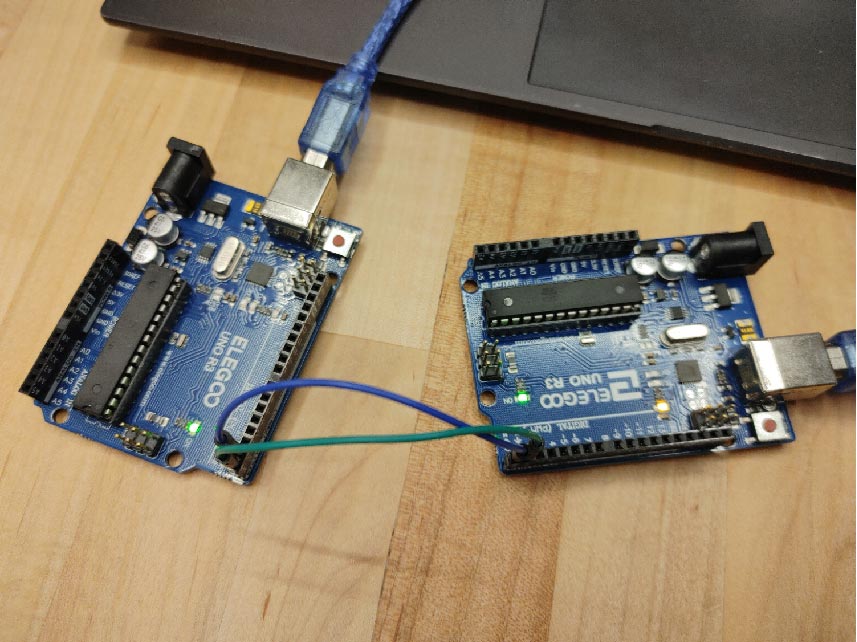
We then wrote a simple code to send messages between them using serial communication:
#include <SoftwareSerial.h> #define RXPIN 2 #define TXPIN 3 SoftwareSerial Serial2(RXPIN,TXPIN); void setup() { Serial.begin(9600); Serial2.begin(9600); pinMode(2,OUTPUT); } void loop() { int input = 0; if (Serial.available() > 0) { input = Serial.read(); Serial2.write(input); } if (Serial2.available() > 0) { input = Serial2.read(); Serial.write("Message Received: "); Serial.write(input); } }
With this code, messages sent from one serial monitor would be outputted from the other.
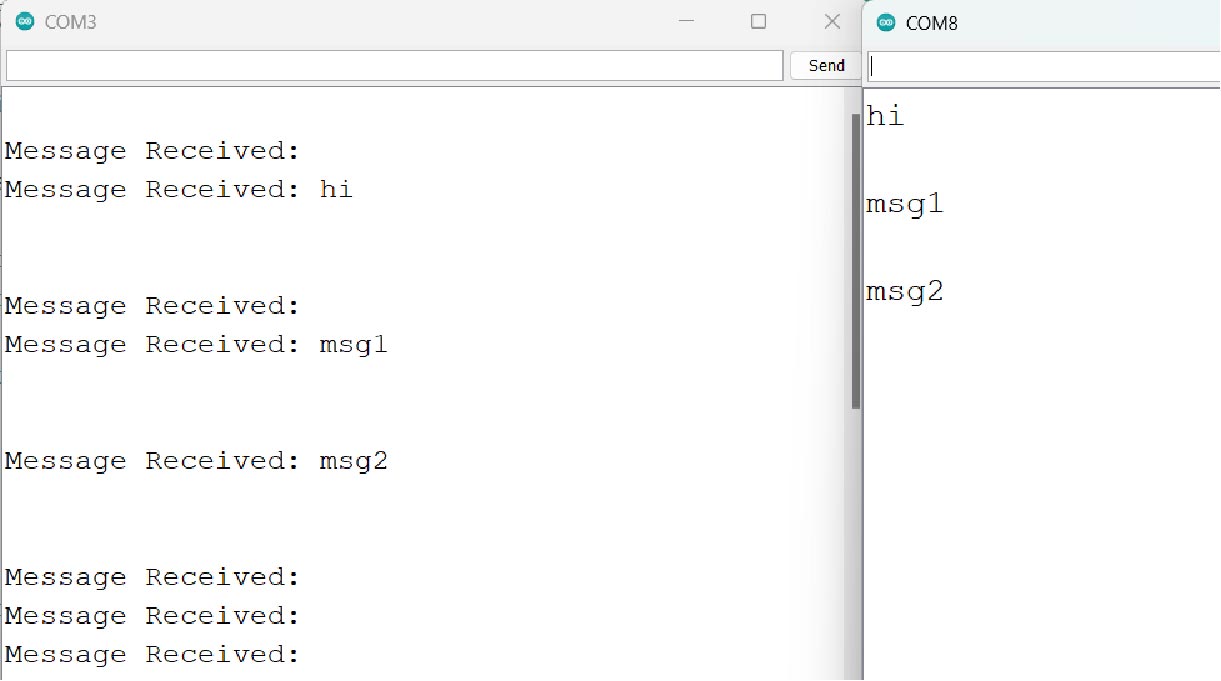
We also used lightblue to send messages to an ESP32, though I don’t have any images of that. I documented my own use of lightblue for the individual assignment. In the group work I learned how to use UART communication and bluetooth connections. I have never programmed any sort of communication protocol like this before, so it helped me figure out how to do the individual work.
ESP32 C3 bug
Part way through troubleshooting another problem, I found that I could no longer upload code to my esp32 c3. Uploading to it gave the error “no serial data received”. I tried uploading to a different board of the same model, and it worked fine, then went back and tried it again on my board, and it showed the error again, then I tried it again on the other board and now it gives the same error on that board as well. I held down the GPIO9 and pressed the reset button to bootload the board. This seems to have temporarily solved the problem. It gave the error several more times, and then stopped happening on its own. The reset continued to work to fix the error.
UART communication
code [ Download ]
I had some difficulty at first because the arduino and the esp32 cannot be uploaded to while they are both plugged in. Once I realized this I was able to establish some simple communication quickly. I programmed the arduino to send whatever message I imputed into the serial monitor, and I programmed the ESP32 to toggle the LED on the board when it received the number 1.
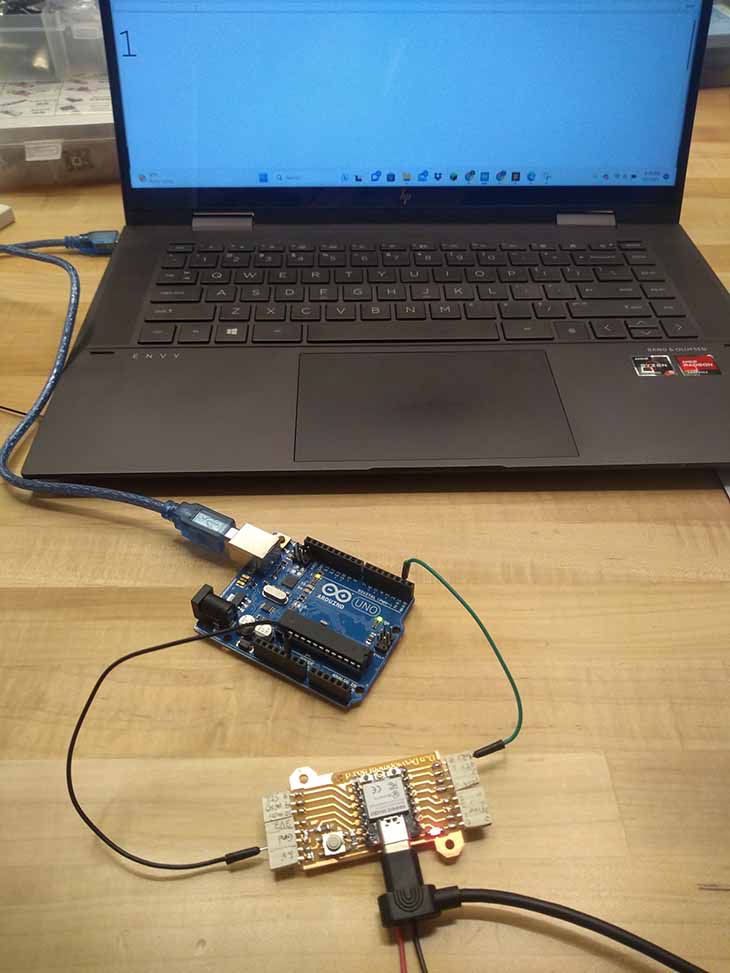
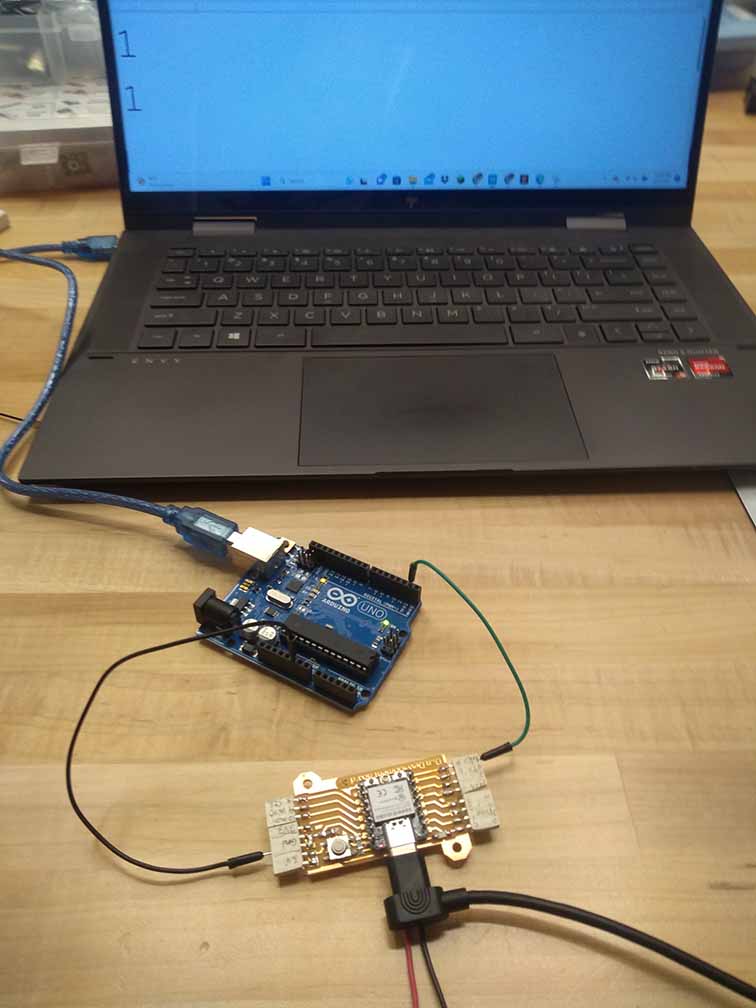
Bluetooth
Code [ Download ]
I used Lightblue to connect to the ESP32 and send messages to it. If you search for your board and connect to it on Lightblue you can send messages to the board from your device.
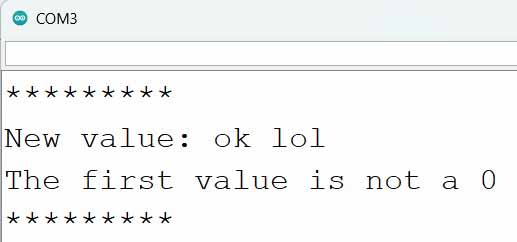
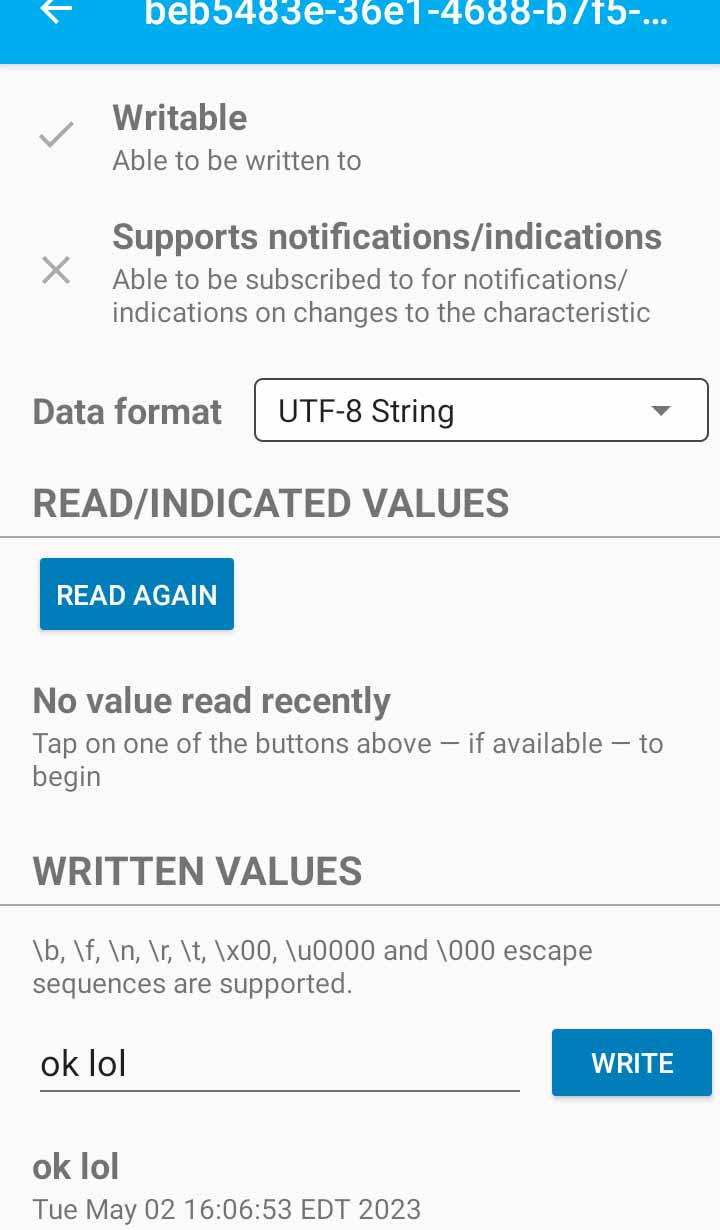
Combined Bluetooth and UART program
Code [ Download ]
I combined the UART and Bluetooth communication code into one program so that the LED can be controlled by my phone or the arduino through my Laptop. A 1 from the serial monitor of the arduino or from the bluetooth connection turns the LED on, a 2 from the serial monitor or a 0 from the bluetooth connection turns it off. The reason it’s 2 to turn the light off with the serial monitor is because the serial monitor interprets the absence of a number as 0 for Serial.parseInt().
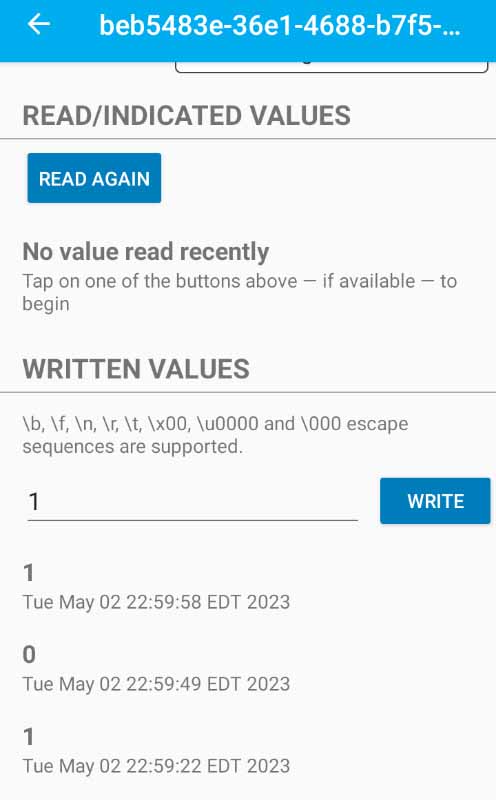
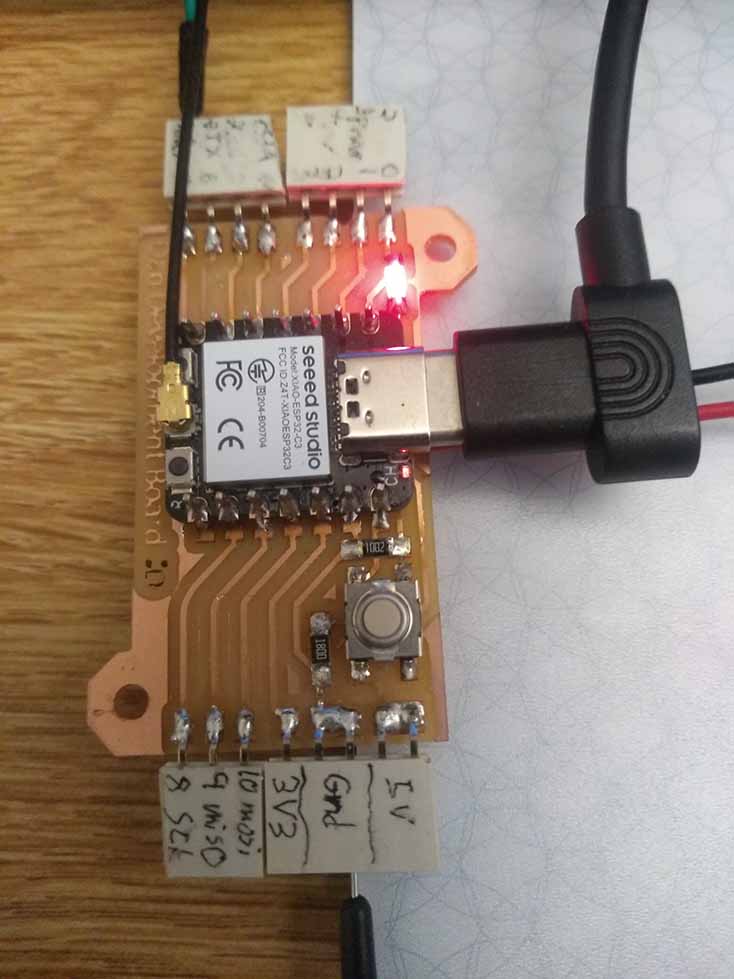
Here is the code for the Bluetooth and UART communication:
#include#include #include #include HardwareSerial Serial2(1); bool light = 0; #define SERVICE_UUID "4fafc201-1fb5-459e-8fcc-c5c9c331914b" #define CHARACTERISTIC_UUID "beb5483e-36e1-4688-b7f5-ea07361b26a8" #define RXp2 20 #define TXp2 21 //defining receiving and transmitting pins for UART communication. class MyCallbacks: public BLECharacteristicCallbacks { void onWrite(BLECharacteristic *pCharacteristic) { //whenever a message is received from bluetoooth std::string value = pCharacteristic->getValue(); //set the message received to a string variable if (value.length() > 0) { //if the message is at least 1 character long if (value[0] - 48 == 0) //and the first character is 0 light = 0; // set the light to off else if (value[0] - 48 == 1) //otherwise if the first character is 1 light = 1; // set the light to on } } }; void setup() { Serial2.begin(9600, SERIAL_8N1, RXp2, TXp2); // open a serial monitor to listen to the arduino pinMode(2,OUTPUT); // set the pin with the led to output BLEDevice::init("DanDevBoard"); // name the bluetooth device so I can find it easily BLEServer *pServer = BLEDevice::createServer(); // create a bluetooth server named pServer BLEService *pService = pServer->createService(SERVICE_UUID); // create a bluetooth service called pService BLECharacteristic *pCharacteristic = pService->createCharacteristic( //add a charactaristic that says that CHARACTERISTIC_UUID, //the device can read and write BLECharacteristic::PROPERTY_READ | BLECharacteristic::PROPERTY_WRITE ); pCharacteristic->setCallbacks(new MyCallbacks()); //sets the callback operation to an instance of the MyCallbacks class pCharacteristic->setValue("Hello World"); pService->start(); BLEAdvertising *pAdvertising = pServer->getAdvertising(); pAdvertising->start(); //starts broadcasting it's existance to the universe } void loop() { // put your main code here, to run repeatedly: switch(Serial2.parseInt()) { //reads the serial monitor and grabs the first integer it can find case 1: //if the monitor had integer 1 light = 1; //set light to on break; case 2: //if the monitor had integer 2 light = 0; //set light to off break; default: //if it was some other number break; //do nothing } if(light){ //if the light should be on digitalWrite(2,HIGH); //turn it on }else{ // otherwise turn it off digitalWrite(2,LOW); } }