14. Interface and application programming
Introduction to the theory stuff
Before deep diving into the interface and applications assignment, let's try to understand the terms a bit better. I think documenting tis is also going to help me in future when I return to this assignment for maybe whatever related work I might have to do in future.What are interfaces?
In software development, an interface refers to a set of rules or protocols that define how different software components or systems can communicate with each other. Interfaces act as a contract between different entities, specifying the methods, properties, and behaviors that can be accessed or implemented.Interfaces serve as a way to achieve abstraction, allowing developers to separate the implementation details from the external functionality of a system or component. By defining interfaces, developers can create modular and reusable code, as different components can interact with each other without relying on the underlying implementation.
What is Application Programming?
Application programming involves the development of software applications, which are programs designed to perform specific tasks or functions for end-users. Application programming focuses on writing code that defines the behavior, functionality, and user experience of an application.What is Processing?
Processing is an open-source programming language and development environment specifically designed for visual arts, creative coding, and multimedia projects. It is built on top of Java and provides a simplified syntax and an intuitive interface, making it accessible for artists, designers, and beginners to create interactive applications and visual experiences.Processing provides a simple and beginner-friendly environment to learn programming concepts while creating visually engaging applications. It has an active community, extensive documentation, and a vast collection of user-contributed libraries, examples, and tutorials
What is API?
In simple terms, we can say that an Application Program Interface (API) is code that allows two software programs to communicate with each other. An API is (usually) not a user interface. It provides software-to-software interaction, not user interactions. Although an API may provide a user interface widget, which an app can grab and display.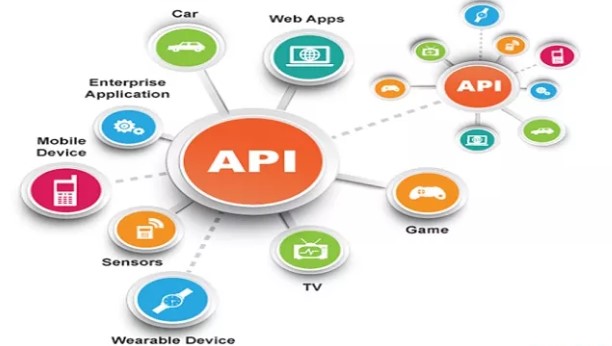
The assignment
In this assignment we need to interface our board with the Computer. i.e. we need to control our board with the computer by making a GUI which will be used to give command to the board via the USB Cable/USB to UART in my case.I have prepared a board which is same as the Arduino UNO Board because it has the same IC i.e. 328p, and does all the functionality of UNO. Below are the images of the final board
PNG image of the board file which is will be given to the moldela for the PCB fabrication.
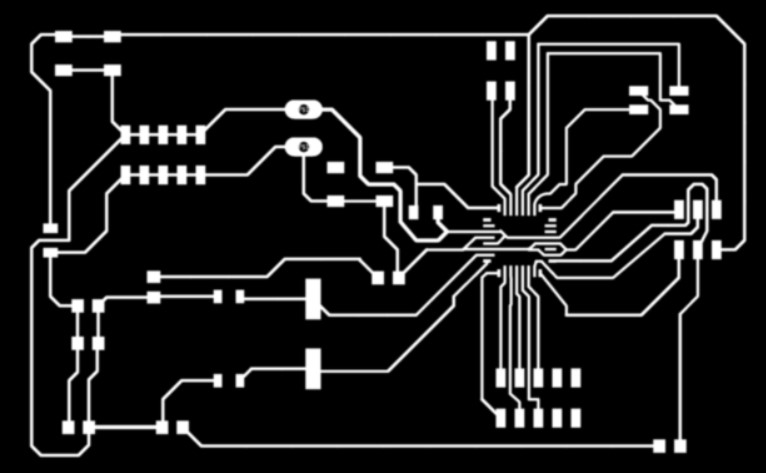
Schematic images of the board which was made in the Eagle software.
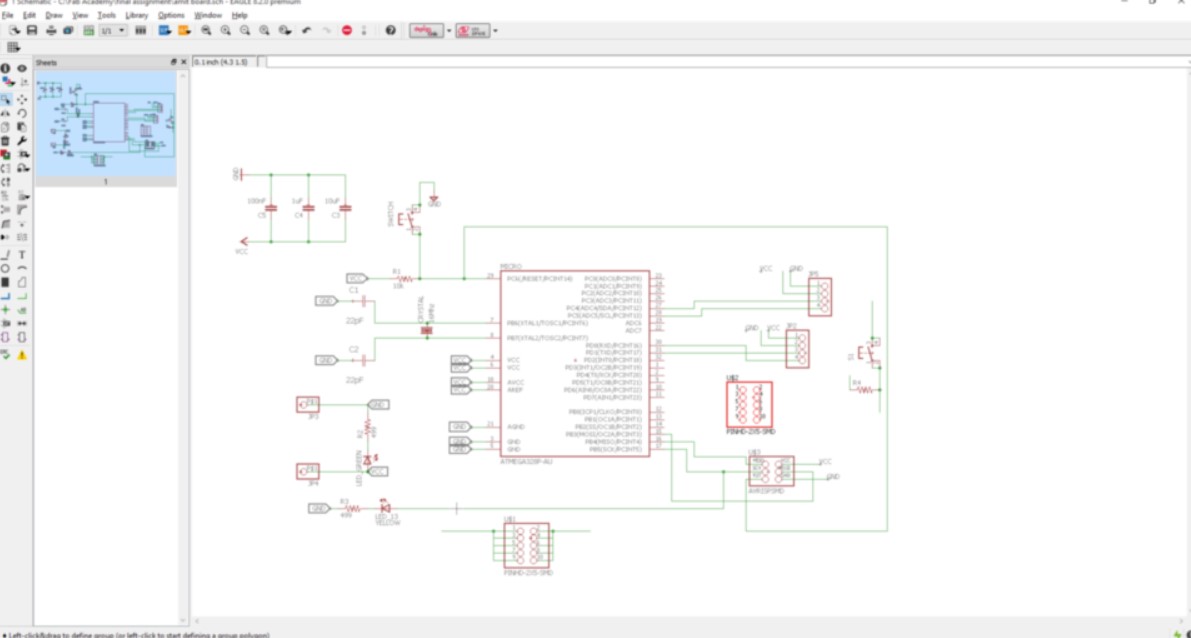
Board images of the final PCB
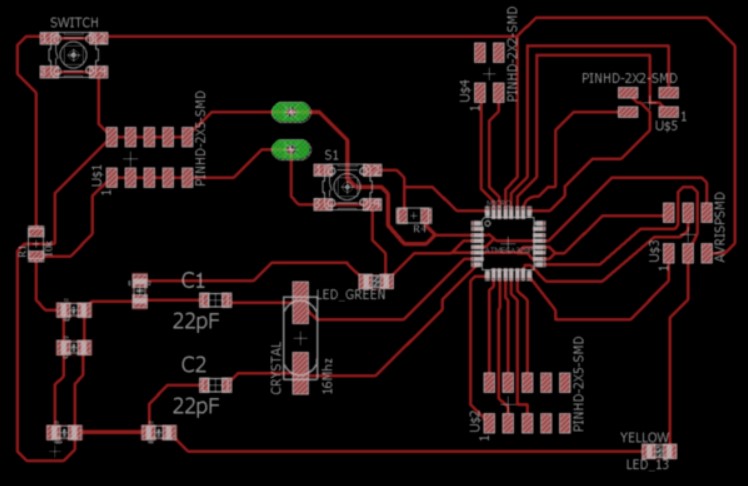
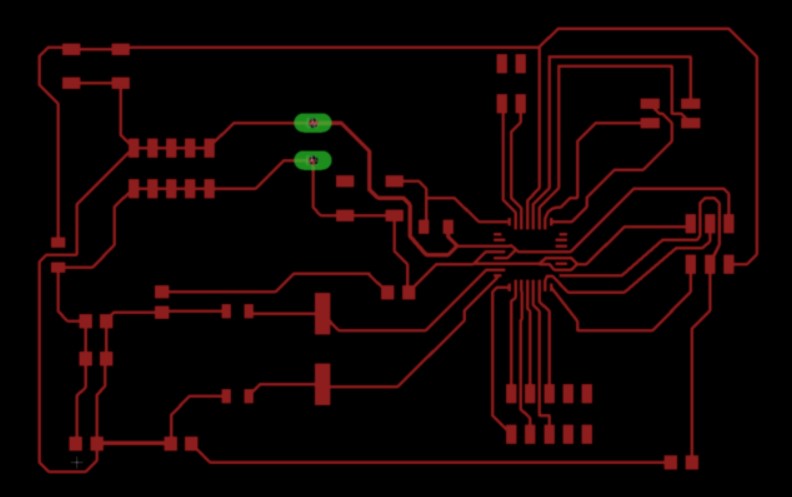
Image of the board after milled.
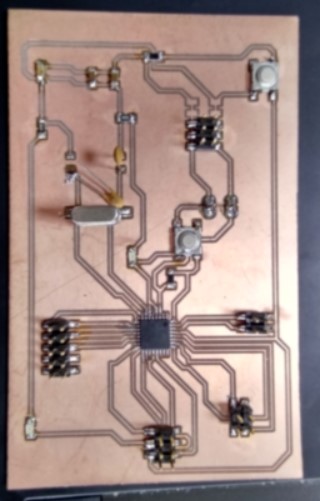
Connection diagram in simple terms (Using USB to UART converter)
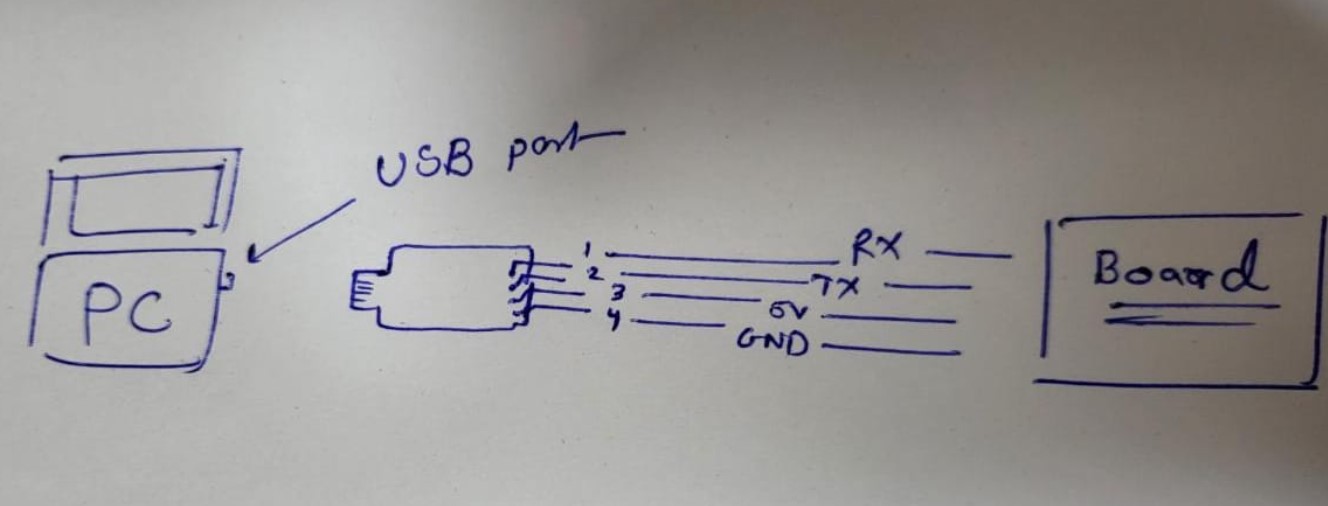
Now for the assignment, once the board is ready and the connection is set with the electronics, I have used the processing as interfacing program between the board and PC.
What exactly is happening in the program? and what processes are running?
I wrote a code in which when we hover our cursor on the buttons of the dialog box, the LED will turn ON and OFF. The processing is communicating with my board through serial communication, which is done by USB to UART converter, the processing is communicating through COMPORT. When processing needs to turn ON the LED, it sends "port.write('1')" to my board, and to turn OFF it sends "port.write('0')". At the receiving end my board reads command by "Serial.read()" function.
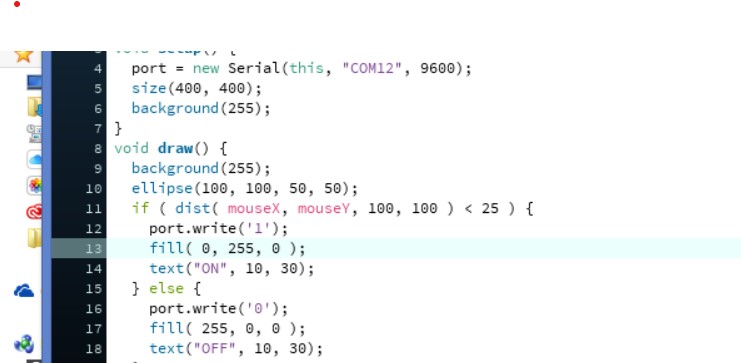
Below is the image of the Processing IDE
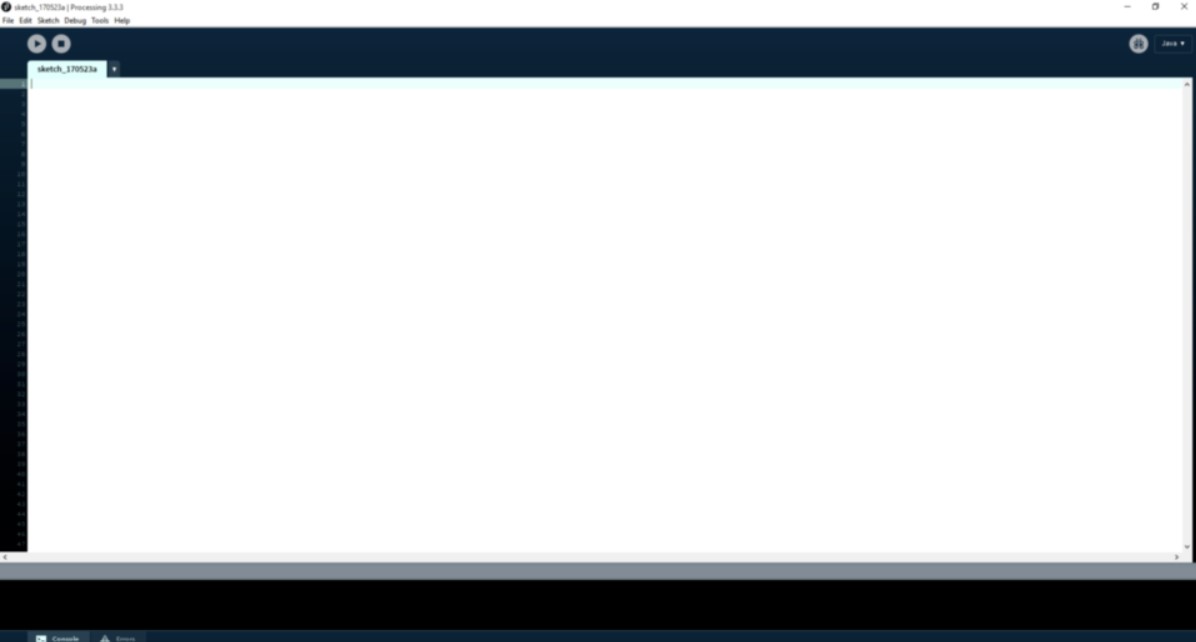
Working between progessing and Arduin IDE
I made a GUI with the help of processing which can turn ON and OFF the led which is connected to digital pin 13 on the board when we hover the mouse button on the virtual button. Below are the images of the processing program.
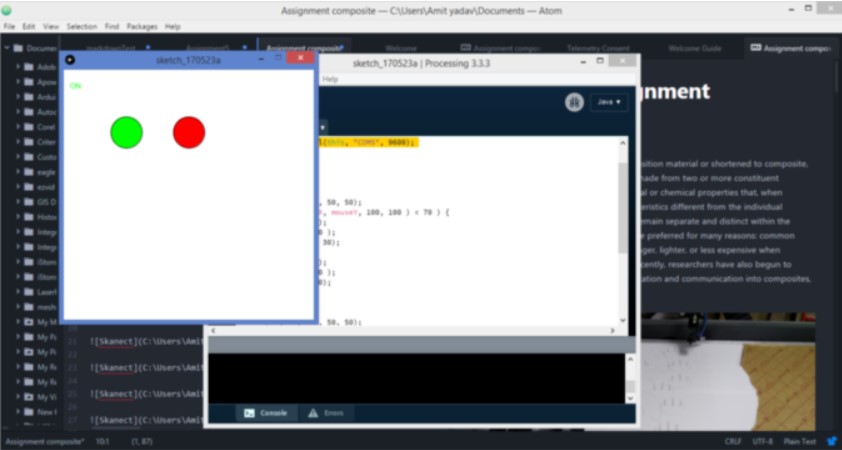
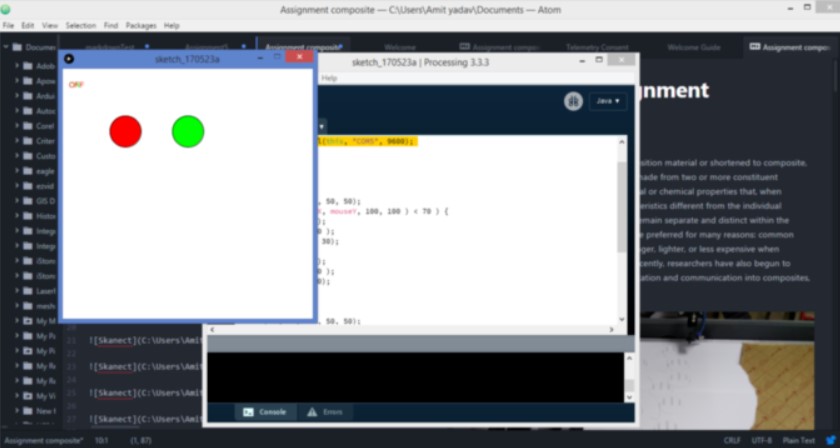
I have used the Arduino IDE for programming the board. In the code, the led which is on pin 13 on the board will be ON when the mouse is hovering on the first button and will be OFF when the mouse is hovering on the second button.
I used USB to serial converter to upload the Arduino code to the board and to get me the port address so that I can connect it with the processing for run the program.
The program flow is as follows:
1. When we hover on one of the buttons of the processing program, the LED will glow on the board.
2. When we on the hover on the other button of the processing program the LED will glow off.
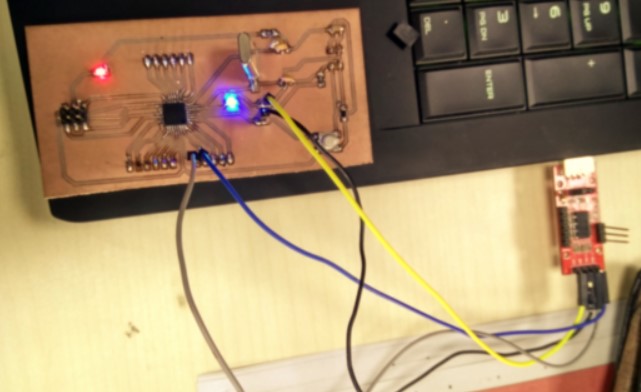
There is the final output video: when I am hovering the mouse on the between the two buttons the led is glowing on the board.
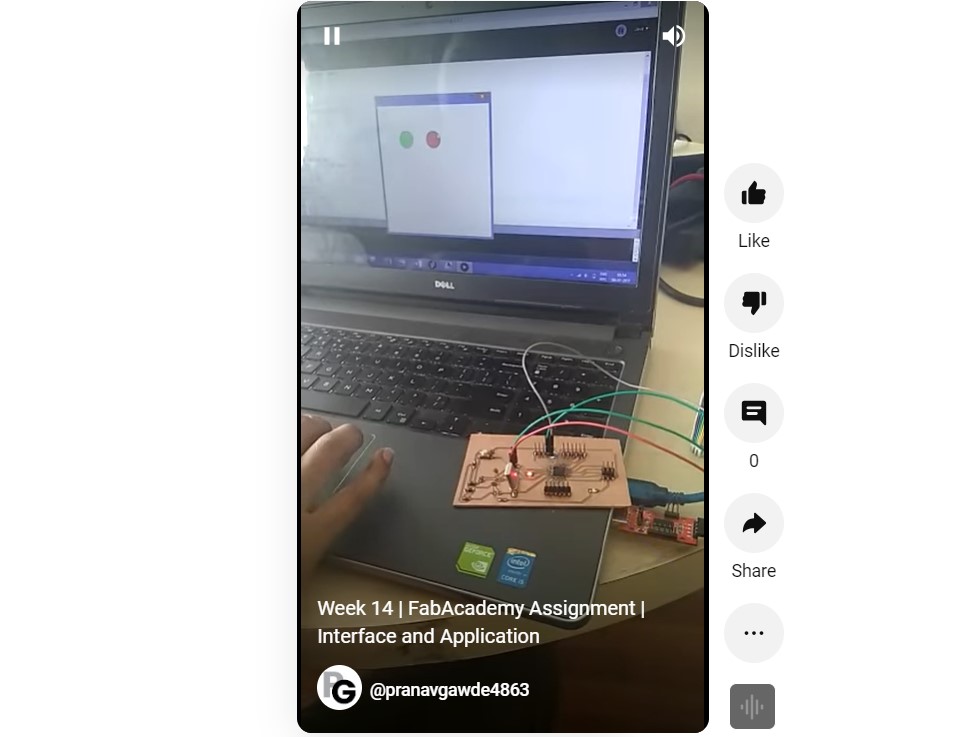
Processing (.pde Code)
import processing.serial.*;
Serial port;
void setup() {
port = new Serial(this, "COM5", 9600);
size(400, 400);
background(255);
}
void draw() {
background(255);
ellipse(100, 100, 50, 50);
if ( dist( mouseX, mouseY, 100, 100 ) < 25 ) {
port.write('1');
fill( 0, 255, 0 );m
text("ON", 10, 30);
} else {
port.write('0');
fill( 255, 0, 0 );
text("OFF", 10, 30);
}
delay(50);
ellipse(200, 100, 50, 50);
if ( dist( mouseX, mouseY, 100, 100 ) < 25 ) {
port.write('0');
fill( 255, 0, 0 );
text("ON", 10, 30);
} else {
port.write('1');
fill( 0, 255, 0 );
text("OFF", 10, 30);
}
delay(50);
}
Arduino (.ino Code)
int state;
void setup() {
pinMode(13, OUTPUT);
Serial.begin(9600);
}
void loop() {
if (Serial.available() > 0) {
state = Serial.read();
if (state == '1') {
digitalWrite(13, HIGH);
}
if (state == '0') {
digitalWrite(13, LOW);
}
}
delay(50);
}