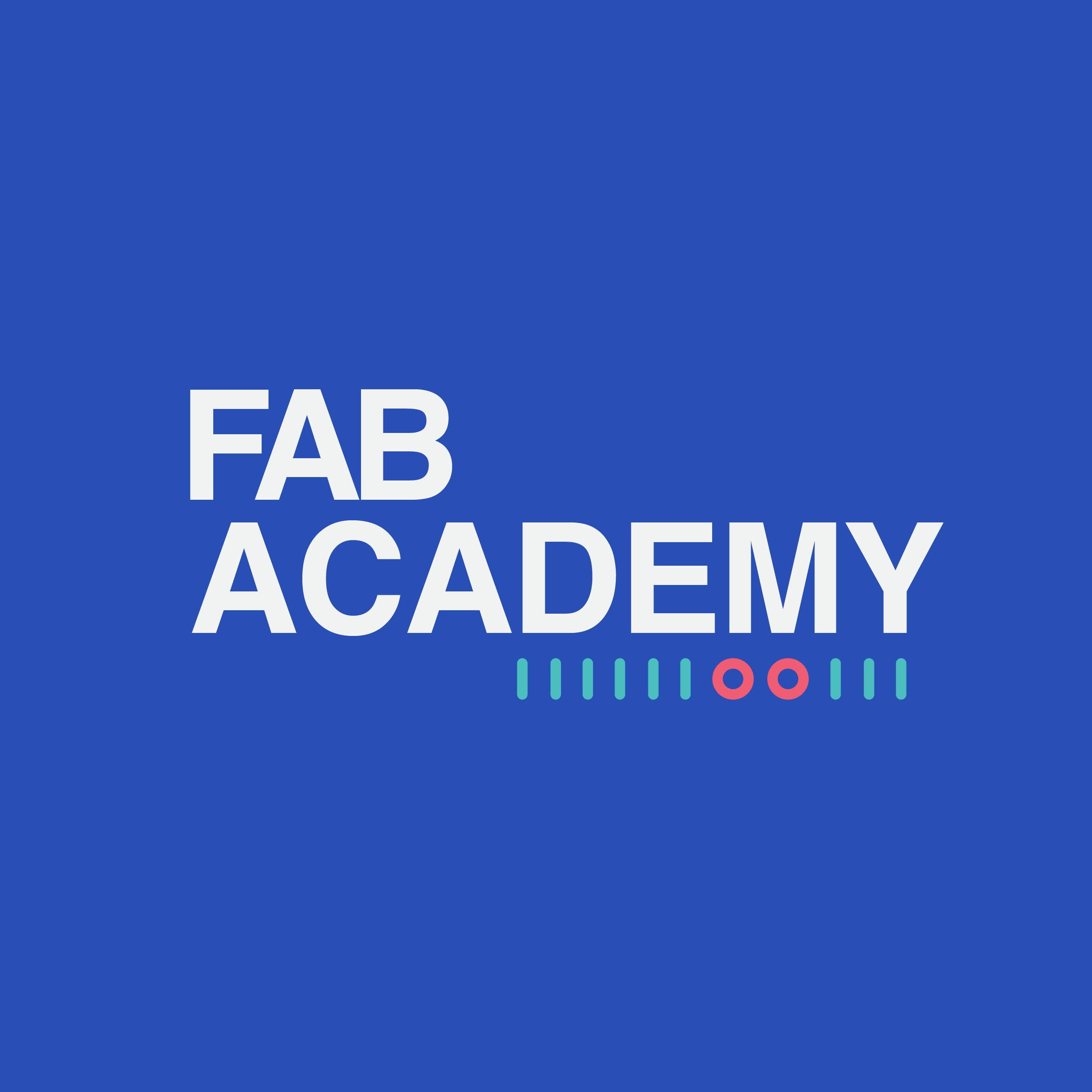
embedded_programming
Week | 4 |
---|---|
Assessment Guide | http://fabacademy.org/2023/nueval/embedded_programming.html |
Lecture Notes | Week 4 - Embedded Programming |
Progress Estimate | 70% |
Student Objectives
Student Objectives
Assignments
Individual
- Browse through the datasheet for your microcontroller
- Program a microcontroller development board to interact and communicate
Group
- Compare the performance and development workflows for other architectures
- Document your work to the group work page and reflect on your individual page what you learned
Student Tasks
Student Tasks
- Linked to the group assignment page
- Documented what you learned from browsing through a microcontroller datasheet.
- Programmed your board to interact and communicate
- Described the programming process(es) you used
- Included your source code
- Included ‘hero shot(s)’
Pre-work, or crystal ball Parallel Design
Emailed back and forth with Scott, my instructor/advisor, to ensure I had access to materials for Embedded Programming since I’m remote and having to secure supplies in advance instead of relying on my Fab Academy node’s stock. Admittedly some of the back and forth was just me having to ask “what’s that?” while we figured out if I had what I’d need.
During Week 3 while the lasercutters were still down in my makerspace, our VP of Classes & Activities, and resident NeoPixels aficionado, James O’Dell offered a workshop in Circuit Python. Seeing an opportunity to become a paradox and simultaneously be ahead and behind, I jumped at the opportunity for in-person instruction and hands-on time with hardware. My notes from that session are recorded here.
Circuit Python Workshop with James O’Dell
- Libraries are drag and drop - just drag whichever one’s you want to use to the “lib” folder, but not more.
- your code will run almost immediately upon doing this, so be ready for blinking lights/etc
- Can download a Project Bundle directly from learn.adafruit.com, and then copy over the libraries and code.py to device
- Modify code.py file (in Mu) to change behaviors of the contoller, such as color/speed of blinking lights

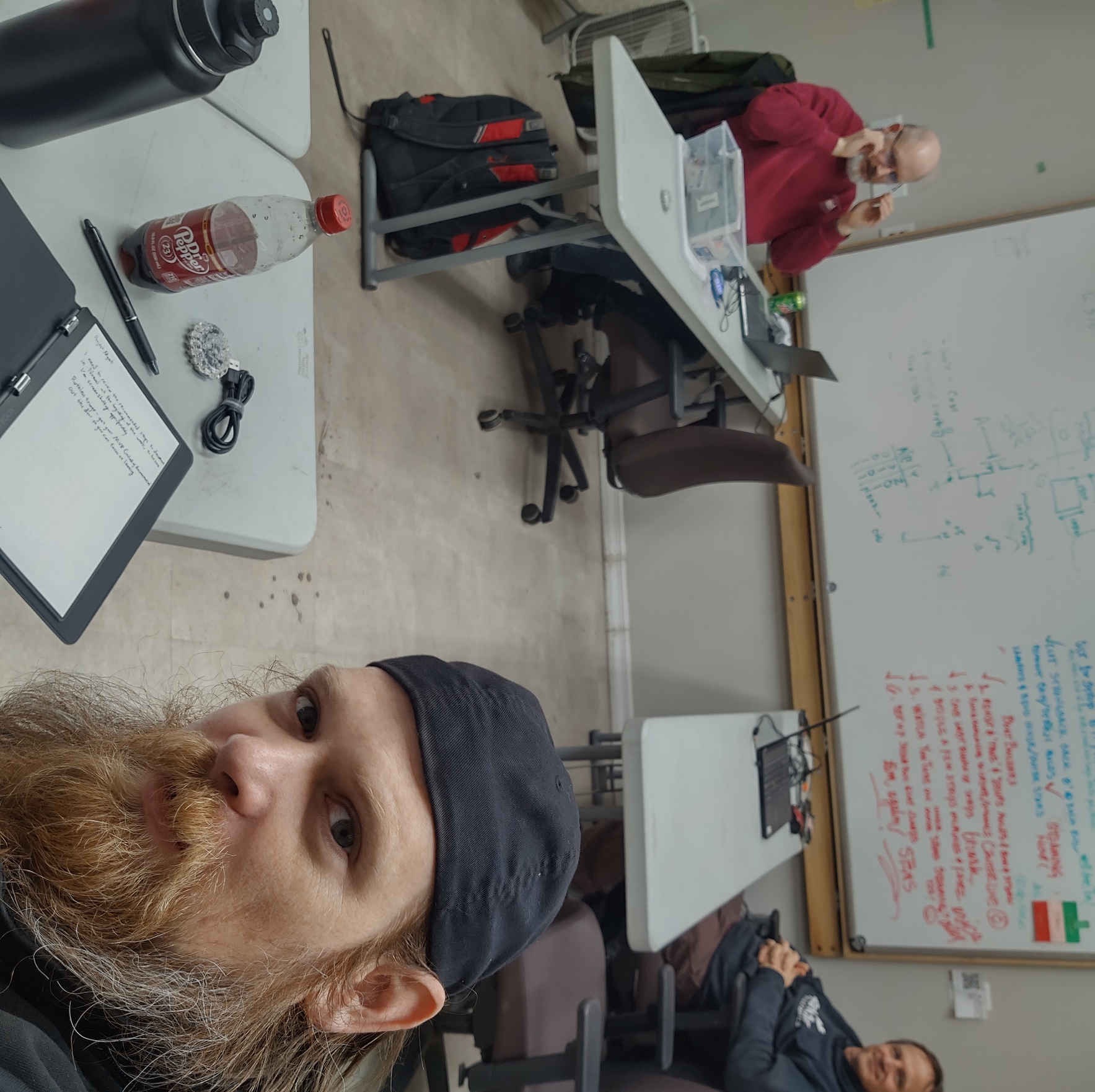
Our workshop took place in the makerspace’s Classroom zone, with James demoing on a screenshare, and going through a slidedeck, which he was kind enough to provide me a copy of for documentation.
Attendees were provided an Adafruit Circuit Playground Express and MicroUSB to USBA cable, to demo it with.
To get started, we downloaded Mu
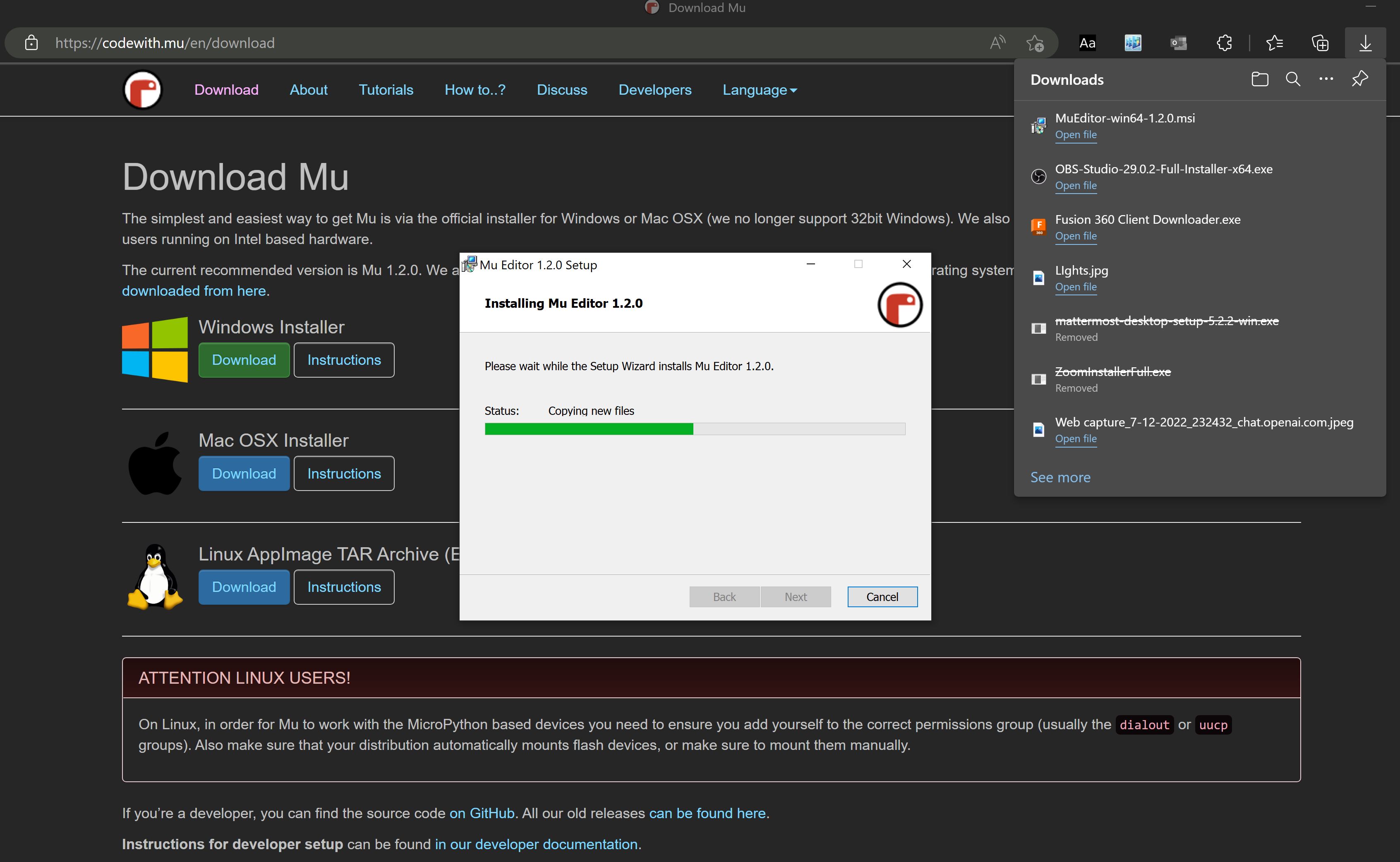
OUTLINE:
- Attend Class
- details, format
- materials
- describe device
- features
- CircPy, Arduino, MS Make
- chip: • ATSAMD21 ARM Cortex M0 Processor, running at 3.3V and 48MHz
- already set up to use
- updates in ~4 seconds
- describe device
- Install software
- discuss what
- demo live
- basically dragged and dropped
- shown resources (Adafruit, circuitpy.com)
- Shown REPL live editing
- Played around with values in code.py
- codebox of code.py
from adafruit_circuitplayground import cp import time cp.pixels.brightness = 0.3 while True: for i in range(10): cp.pixels[i] = (255, 0, 0) time.sleep(0.1) cp.pixels[i] = (0, 0, 0) time.sleep(0.1) for i in range(10, 0, -1): cp.pixels[i-1] = (0, 0, 255) time.sleep(0.1) cp.pixels[i-1] = (0, 0, 0) time.sleep(0.1)
- The code is surprisingly straightforward. It loads a library for timing, and another for controlling the LED colors. It starts by running a For Loop that starts at the first LED going clockwise, sets it to Red, waits for a specified amount of time, and then sets the LED to black, waiting another specified amount of time, before finally looping to the next LED clockwise. After that loop completes, it does the same thing except looping counterclockwise from the last LED, turning each LED blue.
- I altered the color values to a yellow and teal combo I found more fun, ```(150, 150, 0) ; (0, 150, 150)``` and changed seconds values on the timers at the end of the loops, both for visual effect. I attempted to set a variable for that time that would increase with each loop, but the Circuit Playground would error out and flash red leds all at once when it ran that line of the code.
- Changed the values back
- codebox of code.py
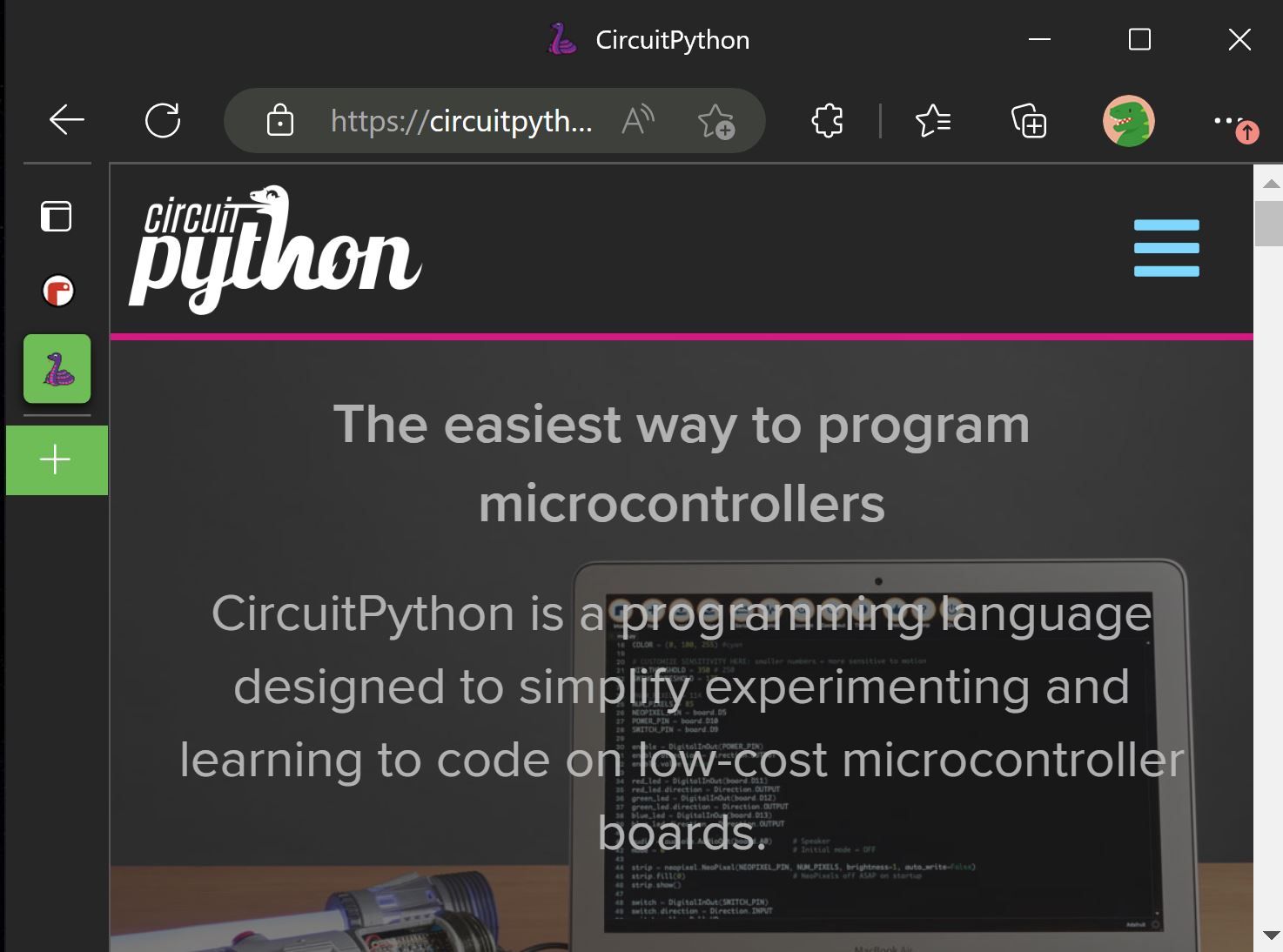
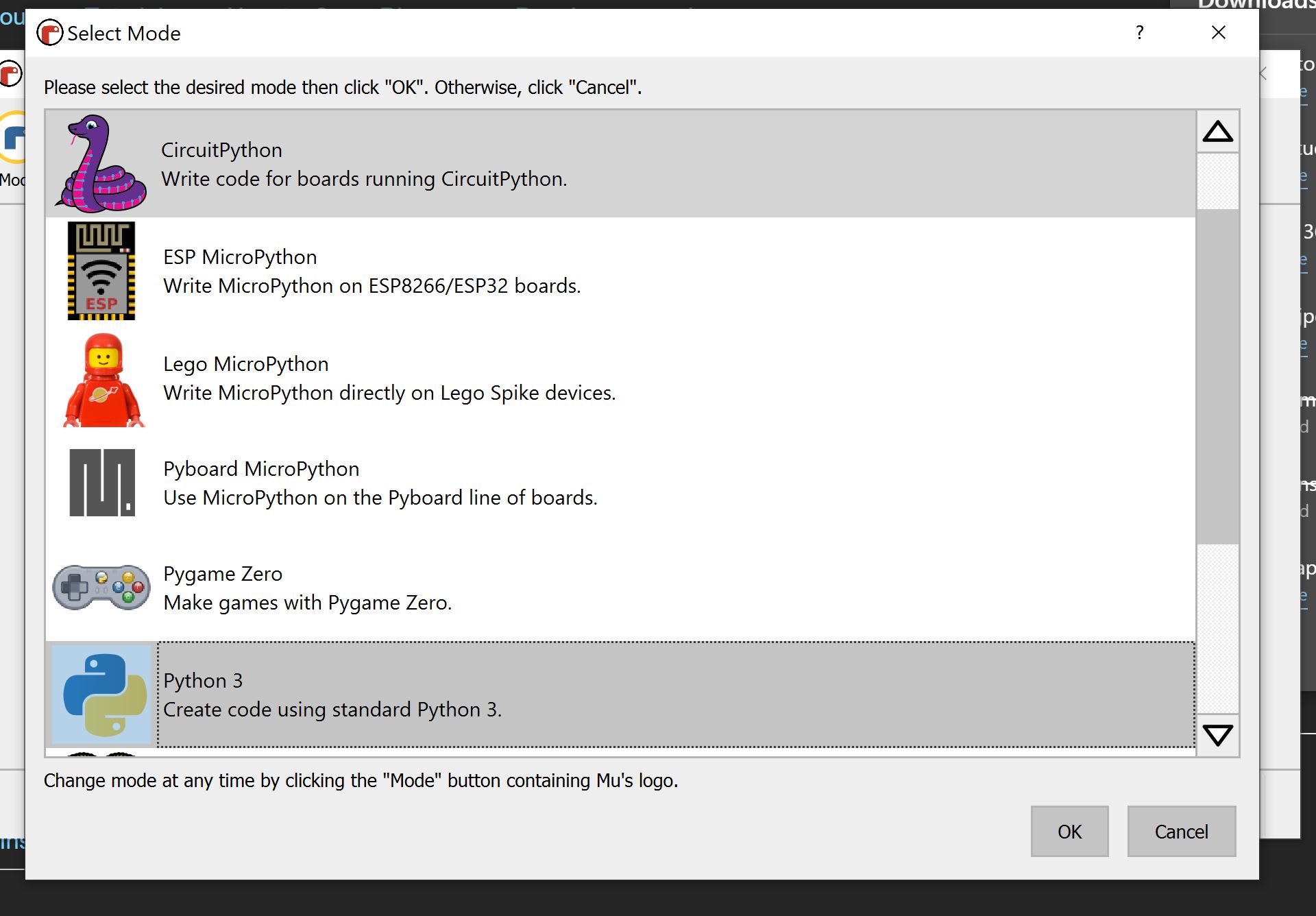
In The Weeds
During
After The Fact, or Playing Catch-up
